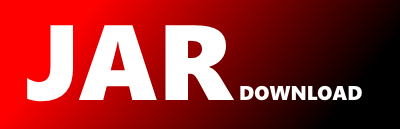
com.google.api.services.content.model.Product Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Required product attributes are primarily defined by the products data specification. See the
* Products Data Specification Help Center article for information.
*
* Some attributes are country-specific, so make sure you select the appropriate country in the
* drop-down selector at the top of the page.
*
* Product data. After inserting, updating, or deleting a product, it may take several minutes
* before changes take effect.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Product extends com.google.api.client.json.GenericJson {
/**
* Additional URLs of images of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List additionalImageLinks;
/**
* Used to group items in an arbitrary way. Only for CPA%, discouraged otherwise.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adsGrouping;
/**
* Similar to ads_grouping, but only works on CPC.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List adsLabels;
/**
* Allows advertisers to override the item URL when the product is shown within the context of
* Product Ads.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adsRedirect;
/**
* Set to true if the item is targeted towards adults.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean adult;
/**
* Target age group of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ageGroup;
/**
* Availability status of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String availability;
/**
* The day a pre-ordered product becomes available for delivery, in ISO 8601 format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String availabilityDate;
/**
* Brand of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String brand;
/**
* Required. The item's channel (online or local).
*
* Acceptable values are: - "`local`" - "`online`"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String channel;
/**
* Color of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String color;
/**
* Condition or state of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String condition;
/**
* Required. The two-letter ISO 639-1 language code for the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contentLanguage;
/**
* Cost of goods sold. Used for gross profit reporting.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Price costOfGoodsSold;
/**
* A list of custom (merchant-provided) attributes. It can also be used for submitting any
* attribute of the feed specification in its generic form (e.g., `{ "name": "size type", "value":
* "regular" }`). This is useful for submitting attributes not explicitly exposed by the API, such
* as additional attributes used for Shopping Actions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List customAttributes;
static {
// hack to force ProGuard to consider CustomAttribute used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(CustomAttribute.class);
}
/**
* Custom label 0 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel0;
/**
* Custom label 1 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel1;
/**
* Custom label 2 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel2;
/**
* Custom label 3 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel3;
/**
* Custom label 4 for custom grouping of items in a Shopping campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customLabel4;
/**
* Description of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* An identifier for an item for dynamic remarketing campaigns.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayAdsId;
/**
* URL directly to your item's landing page for dynamic remarketing campaigns.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayAdsLink;
/**
* Advertiser-specified recommendations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List displayAdsSimilarIds;
/**
* Title of an item for dynamic remarketing campaigns.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayAdsTitle;
/**
* Offer margin for dynamic remarketing campaigns.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double displayAdsValue;
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String energyEfficiencyClass;
/**
* The list of destinations to exclude for this target (corresponds to unchecked check boxes in
* Merchant Center).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List excludedDestinations;
/**
* Date on which the item should expire, as specified upon insertion, in ISO 8601 format. The
* actual expiration date in Google Shopping is exposed in `productstatuses` as
* `googleExpirationDate` and might be earlier if `expirationDate` is too far in the future.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String expirationDate;
/**
* Target gender of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gender;
/**
* Google's category of the item (see Google product taxonomy).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String googleProductCategory;
/**
* Global Trade Item Number (GTIN) of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gtin;
/**
* The REST ID of the product. Content API methods that operate on products take this as their
* `productId` parameter. The REST ID for a product is of the form
* channel:contentLanguage:targetCountry: offerId.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* False when the item does not have unique product identifiers appropriate to its category, such
* as GTIN, MPN, and brand. Required according to the Unique Product Identifier Rules for all
* target countries except for Canada.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean identifierExists;
/**
* URL of an image of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imageLink;
/**
* The list of destinations to include for this target (corresponds to checked check boxes in
* Merchant Center). Default destinations are always included unless provided in
* `excludedDestinations`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includedDestinations;
/**
* Number and amount of installments to pay for an item. Brazil only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Installment installment;
/**
* Whether the item is a merchant-defined bundle. A bundle is a custom grouping of different
* products sold by a merchant for a single price.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isBundle;
/**
* Shared identifier for all variants of the same product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String itemGroupId;
/**
* Identifies what kind of resource this is. Value: the fixed string "`content#product`"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* URL directly linking to your item's page on your website.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String link;
/**
* Loyalty points that users receive after purchasing the item. Japan only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LoyaltyPoints loyaltyPoints;
/**
* The material of which the item is made.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String material;
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String maxEnergyEfficiencyClass;
/**
* Maximal product handling time (in business days).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long maxHandlingTime;
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String minEnergyEfficiencyClass;
/**
* Minimal product handling time (in business days).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long minHandlingTime;
/**
* Link to a mobile-optimized version of the landing page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mobileLink;
/**
* Manufacturer Part Number (MPN) of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mpn;
/**
* The number of identical products in a merchant-defined multipack.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long multipack;
/**
* Required. A unique identifier for the item. Leading and trailing whitespaces are stripped and
* multiple whitespaces are replaced by a single whitespace upon submission. Only valid unicode
* characters are accepted. See the products feed specification for details. Note: Content API
* methods that operate on products take the REST ID of the product, not this identifier.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String offerId;
/**
* The item's pattern (e.g. polka dots).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pattern;
/**
* Price of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Price price;
/**
* Categories of the item (formatted as in products data specification).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List productTypes;
/**
* The unique ID of a promotion.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List promotionIds;
/**
* Advertised sale price of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Price salePrice;
/**
* Date range during which the item is on sale (see products data specification).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String salePriceEffectiveDate;
/**
* The quantity of the product that is available for selling on Google. Supported only for online
* products.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long sellOnGoogleQuantity;
/**
* Shipping rules.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List shipping;
/**
* Height of the item for shipping.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductShippingDimension shippingHeight;
/**
* The shipping label of the product, used to group product in account-level shipping rules.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String shippingLabel;
/**
* Length of the item for shipping.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductShippingDimension shippingLength;
/**
* Weight of the item for shipping.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductShippingWeight shippingWeight;
/**
* Width of the item for shipping.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductShippingDimension shippingWidth;
/**
* System in which the size is specified. Recommended for apparel items.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sizeSystem;
/**
* The cut of the item. Recommended for apparel items.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sizeType;
/**
* Size of the item. Only one value is allowed. For variants with different sizes, insert a
* separate product for each size with the same `itemGroupId` value (see size definition).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sizes;
/**
* The source of the offer, i.e., how the offer was created.
*
* Acceptable values are: - "`api`" - "`crawl`" - "`feed`"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String source;
/**
* Required. The CLDR territory code for the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String targetCountry;
/**
* The tax category of the product, used to configure detailed tax nexus in account-level tax
* settings.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String taxCategory;
/**
* Tax information.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List taxes;
/**
* Title of the item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The transit time label of the product, used to group product in account-level transit time
* tables.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String transitTimeLabel;
/**
* The preference of the denominator of the unit price.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductUnitPricingBaseMeasure unitPricingBaseMeasure;
/**
* The measure and dimension of an item.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductUnitPricingMeasure unitPricingMeasure;
/**
* Additional URLs of images of the item.
* @return value or {@code null} for none
*/
public java.util.List getAdditionalImageLinks() {
return additionalImageLinks;
}
/**
* Additional URLs of images of the item.
* @param additionalImageLinks additionalImageLinks or {@code null} for none
*/
public Product setAdditionalImageLinks(java.util.List additionalImageLinks) {
this.additionalImageLinks = additionalImageLinks;
return this;
}
/**
* Used to group items in an arbitrary way. Only for CPA%, discouraged otherwise.
* @return value or {@code null} for none
*/
public java.lang.String getAdsGrouping() {
return adsGrouping;
}
/**
* Used to group items in an arbitrary way. Only for CPA%, discouraged otherwise.
* @param adsGrouping adsGrouping or {@code null} for none
*/
public Product setAdsGrouping(java.lang.String adsGrouping) {
this.adsGrouping = adsGrouping;
return this;
}
/**
* Similar to ads_grouping, but only works on CPC.
* @return value or {@code null} for none
*/
public java.util.List getAdsLabels() {
return adsLabels;
}
/**
* Similar to ads_grouping, but only works on CPC.
* @param adsLabels adsLabels or {@code null} for none
*/
public Product setAdsLabels(java.util.List adsLabels) {
this.adsLabels = adsLabels;
return this;
}
/**
* Allows advertisers to override the item URL when the product is shown within the context of
* Product Ads.
* @return value or {@code null} for none
*/
public java.lang.String getAdsRedirect() {
return adsRedirect;
}
/**
* Allows advertisers to override the item URL when the product is shown within the context of
* Product Ads.
* @param adsRedirect adsRedirect or {@code null} for none
*/
public Product setAdsRedirect(java.lang.String adsRedirect) {
this.adsRedirect = adsRedirect;
return this;
}
/**
* Set to true if the item is targeted towards adults.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAdult() {
return adult;
}
/**
* Set to true if the item is targeted towards adults.
* @param adult adult or {@code null} for none
*/
public Product setAdult(java.lang.Boolean adult) {
this.adult = adult;
return this;
}
/**
* Target age group of the item.
* @return value or {@code null} for none
*/
public java.lang.String getAgeGroup() {
return ageGroup;
}
/**
* Target age group of the item.
* @param ageGroup ageGroup or {@code null} for none
*/
public Product setAgeGroup(java.lang.String ageGroup) {
this.ageGroup = ageGroup;
return this;
}
/**
* Availability status of the item.
* @return value or {@code null} for none
*/
public java.lang.String getAvailability() {
return availability;
}
/**
* Availability status of the item.
* @param availability availability or {@code null} for none
*/
public Product setAvailability(java.lang.String availability) {
this.availability = availability;
return this;
}
/**
* The day a pre-ordered product becomes available for delivery, in ISO 8601 format.
* @return value or {@code null} for none
*/
public java.lang.String getAvailabilityDate() {
return availabilityDate;
}
/**
* The day a pre-ordered product becomes available for delivery, in ISO 8601 format.
* @param availabilityDate availabilityDate or {@code null} for none
*/
public Product setAvailabilityDate(java.lang.String availabilityDate) {
this.availabilityDate = availabilityDate;
return this;
}
/**
* Brand of the item.
* @return value or {@code null} for none
*/
public java.lang.String getBrand() {
return brand;
}
/**
* Brand of the item.
* @param brand brand or {@code null} for none
*/
public Product setBrand(java.lang.String brand) {
this.brand = brand;
return this;
}
/**
* Required. The item's channel (online or local).
*
* Acceptable values are: - "`local`" - "`online`"
* @return value or {@code null} for none
*/
public java.lang.String getChannel() {
return channel;
}
/**
* Required. The item's channel (online or local).
*
* Acceptable values are: - "`local`" - "`online`"
* @param channel channel or {@code null} for none
*/
public Product setChannel(java.lang.String channel) {
this.channel = channel;
return this;
}
/**
* Color of the item.
* @return value or {@code null} for none
*/
public java.lang.String getColor() {
return color;
}
/**
* Color of the item.
* @param color color or {@code null} for none
*/
public Product setColor(java.lang.String color) {
this.color = color;
return this;
}
/**
* Condition or state of the item.
* @return value or {@code null} for none
*/
public java.lang.String getCondition() {
return condition;
}
/**
* Condition or state of the item.
* @param condition condition or {@code null} for none
*/
public Product setCondition(java.lang.String condition) {
this.condition = condition;
return this;
}
/**
* Required. The two-letter ISO 639-1 language code for the item.
* @return value or {@code null} for none
*/
public java.lang.String getContentLanguage() {
return contentLanguage;
}
/**
* Required. The two-letter ISO 639-1 language code for the item.
* @param contentLanguage contentLanguage or {@code null} for none
*/
public Product setContentLanguage(java.lang.String contentLanguage) {
this.contentLanguage = contentLanguage;
return this;
}
/**
* Cost of goods sold. Used for gross profit reporting.
* @return value or {@code null} for none
*/
public Price getCostOfGoodsSold() {
return costOfGoodsSold;
}
/**
* Cost of goods sold. Used for gross profit reporting.
* @param costOfGoodsSold costOfGoodsSold or {@code null} for none
*/
public Product setCostOfGoodsSold(Price costOfGoodsSold) {
this.costOfGoodsSold = costOfGoodsSold;
return this;
}
/**
* A list of custom (merchant-provided) attributes. It can also be used for submitting any
* attribute of the feed specification in its generic form (e.g., `{ "name": "size type", "value":
* "regular" }`). This is useful for submitting attributes not explicitly exposed by the API, such
* as additional attributes used for Shopping Actions.
* @return value or {@code null} for none
*/
public java.util.List getCustomAttributes() {
return customAttributes;
}
/**
* A list of custom (merchant-provided) attributes. It can also be used for submitting any
* attribute of the feed specification in its generic form (e.g., `{ "name": "size type", "value":
* "regular" }`). This is useful for submitting attributes not explicitly exposed by the API, such
* as additional attributes used for Shopping Actions.
* @param customAttributes customAttributes or {@code null} for none
*/
public Product setCustomAttributes(java.util.List customAttributes) {
this.customAttributes = customAttributes;
return this;
}
/**
* Custom label 0 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel0() {
return customLabel0;
}
/**
* Custom label 0 for custom grouping of items in a Shopping campaign.
* @param customLabel0 customLabel0 or {@code null} for none
*/
public Product setCustomLabel0(java.lang.String customLabel0) {
this.customLabel0 = customLabel0;
return this;
}
/**
* Custom label 1 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel1() {
return customLabel1;
}
/**
* Custom label 1 for custom grouping of items in a Shopping campaign.
* @param customLabel1 customLabel1 or {@code null} for none
*/
public Product setCustomLabel1(java.lang.String customLabel1) {
this.customLabel1 = customLabel1;
return this;
}
/**
* Custom label 2 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel2() {
return customLabel2;
}
/**
* Custom label 2 for custom grouping of items in a Shopping campaign.
* @param customLabel2 customLabel2 or {@code null} for none
*/
public Product setCustomLabel2(java.lang.String customLabel2) {
this.customLabel2 = customLabel2;
return this;
}
/**
* Custom label 3 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel3() {
return customLabel3;
}
/**
* Custom label 3 for custom grouping of items in a Shopping campaign.
* @param customLabel3 customLabel3 or {@code null} for none
*/
public Product setCustomLabel3(java.lang.String customLabel3) {
this.customLabel3 = customLabel3;
return this;
}
/**
* Custom label 4 for custom grouping of items in a Shopping campaign.
* @return value or {@code null} for none
*/
public java.lang.String getCustomLabel4() {
return customLabel4;
}
/**
* Custom label 4 for custom grouping of items in a Shopping campaign.
* @param customLabel4 customLabel4 or {@code null} for none
*/
public Product setCustomLabel4(java.lang.String customLabel4) {
this.customLabel4 = customLabel4;
return this;
}
/**
* Description of the item.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Description of the item.
* @param description description or {@code null} for none
*/
public Product setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* An identifier for an item for dynamic remarketing campaigns.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayAdsId() {
return displayAdsId;
}
/**
* An identifier for an item for dynamic remarketing campaigns.
* @param displayAdsId displayAdsId or {@code null} for none
*/
public Product setDisplayAdsId(java.lang.String displayAdsId) {
this.displayAdsId = displayAdsId;
return this;
}
/**
* URL directly to your item's landing page for dynamic remarketing campaigns.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayAdsLink() {
return displayAdsLink;
}
/**
* URL directly to your item's landing page for dynamic remarketing campaigns.
* @param displayAdsLink displayAdsLink or {@code null} for none
*/
public Product setDisplayAdsLink(java.lang.String displayAdsLink) {
this.displayAdsLink = displayAdsLink;
return this;
}
/**
* Advertiser-specified recommendations.
* @return value or {@code null} for none
*/
public java.util.List getDisplayAdsSimilarIds() {
return displayAdsSimilarIds;
}
/**
* Advertiser-specified recommendations.
* @param displayAdsSimilarIds displayAdsSimilarIds or {@code null} for none
*/
public Product setDisplayAdsSimilarIds(java.util.List displayAdsSimilarIds) {
this.displayAdsSimilarIds = displayAdsSimilarIds;
return this;
}
/**
* Title of an item for dynamic remarketing campaigns.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayAdsTitle() {
return displayAdsTitle;
}
/**
* Title of an item for dynamic remarketing campaigns.
* @param displayAdsTitle displayAdsTitle or {@code null} for none
*/
public Product setDisplayAdsTitle(java.lang.String displayAdsTitle) {
this.displayAdsTitle = displayAdsTitle;
return this;
}
/**
* Offer margin for dynamic remarketing campaigns.
* @return value or {@code null} for none
*/
public java.lang.Double getDisplayAdsValue() {
return displayAdsValue;
}
/**
* Offer margin for dynamic remarketing campaigns.
* @param displayAdsValue displayAdsValue or {@code null} for none
*/
public Product setDisplayAdsValue(java.lang.Double displayAdsValue) {
this.displayAdsValue = displayAdsValue;
return this;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @return value or {@code null} for none
*/
public java.lang.String getEnergyEfficiencyClass() {
return energyEfficiencyClass;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @param energyEfficiencyClass energyEfficiencyClass or {@code null} for none
*/
public Product setEnergyEfficiencyClass(java.lang.String energyEfficiencyClass) {
this.energyEfficiencyClass = energyEfficiencyClass;
return this;
}
/**
* The list of destinations to exclude for this target (corresponds to unchecked check boxes in
* Merchant Center).
* @return value or {@code null} for none
*/
public java.util.List getExcludedDestinations() {
return excludedDestinations;
}
/**
* The list of destinations to exclude for this target (corresponds to unchecked check boxes in
* Merchant Center).
* @param excludedDestinations excludedDestinations or {@code null} for none
*/
public Product setExcludedDestinations(java.util.List excludedDestinations) {
this.excludedDestinations = excludedDestinations;
return this;
}
/**
* Date on which the item should expire, as specified upon insertion, in ISO 8601 format. The
* actual expiration date in Google Shopping is exposed in `productstatuses` as
* `googleExpirationDate` and might be earlier if `expirationDate` is too far in the future.
* @return value or {@code null} for none
*/
public java.lang.String getExpirationDate() {
return expirationDate;
}
/**
* Date on which the item should expire, as specified upon insertion, in ISO 8601 format. The
* actual expiration date in Google Shopping is exposed in `productstatuses` as
* `googleExpirationDate` and might be earlier if `expirationDate` is too far in the future.
* @param expirationDate expirationDate or {@code null} for none
*/
public Product setExpirationDate(java.lang.String expirationDate) {
this.expirationDate = expirationDate;
return this;
}
/**
* Target gender of the item.
* @return value or {@code null} for none
*/
public java.lang.String getGender() {
return gender;
}
/**
* Target gender of the item.
* @param gender gender or {@code null} for none
*/
public Product setGender(java.lang.String gender) {
this.gender = gender;
return this;
}
/**
* Google's category of the item (see Google product taxonomy).
* @return value or {@code null} for none
*/
public java.lang.String getGoogleProductCategory() {
return googleProductCategory;
}
/**
* Google's category of the item (see Google product taxonomy).
* @param googleProductCategory googleProductCategory or {@code null} for none
*/
public Product setGoogleProductCategory(java.lang.String googleProductCategory) {
this.googleProductCategory = googleProductCategory;
return this;
}
/**
* Global Trade Item Number (GTIN) of the item.
* @return value or {@code null} for none
*/
public java.lang.String getGtin() {
return gtin;
}
/**
* Global Trade Item Number (GTIN) of the item.
* @param gtin gtin or {@code null} for none
*/
public Product setGtin(java.lang.String gtin) {
this.gtin = gtin;
return this;
}
/**
* The REST ID of the product. Content API methods that operate on products take this as their
* `productId` parameter. The REST ID for a product is of the form
* channel:contentLanguage:targetCountry: offerId.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The REST ID of the product. Content API methods that operate on products take this as their
* `productId` parameter. The REST ID for a product is of the form
* channel:contentLanguage:targetCountry: offerId.
* @param id id or {@code null} for none
*/
public Product setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* False when the item does not have unique product identifiers appropriate to its category, such
* as GTIN, MPN, and brand. Required according to the Unique Product Identifier Rules for all
* target countries except for Canada.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIdentifierExists() {
return identifierExists;
}
/**
* False when the item does not have unique product identifiers appropriate to its category, such
* as GTIN, MPN, and brand. Required according to the Unique Product Identifier Rules for all
* target countries except for Canada.
* @param identifierExists identifierExists or {@code null} for none
*/
public Product setIdentifierExists(java.lang.Boolean identifierExists) {
this.identifierExists = identifierExists;
return this;
}
/**
* URL of an image of the item.
* @return value or {@code null} for none
*/
public java.lang.String getImageLink() {
return imageLink;
}
/**
* URL of an image of the item.
* @param imageLink imageLink or {@code null} for none
*/
public Product setImageLink(java.lang.String imageLink) {
this.imageLink = imageLink;
return this;
}
/**
* The list of destinations to include for this target (corresponds to checked check boxes in
* Merchant Center). Default destinations are always included unless provided in
* `excludedDestinations`.
* @return value or {@code null} for none
*/
public java.util.List getIncludedDestinations() {
return includedDestinations;
}
/**
* The list of destinations to include for this target (corresponds to checked check boxes in
* Merchant Center). Default destinations are always included unless provided in
* `excludedDestinations`.
* @param includedDestinations includedDestinations or {@code null} for none
*/
public Product setIncludedDestinations(java.util.List includedDestinations) {
this.includedDestinations = includedDestinations;
return this;
}
/**
* Number and amount of installments to pay for an item. Brazil only.
* @return value or {@code null} for none
*/
public Installment getInstallment() {
return installment;
}
/**
* Number and amount of installments to pay for an item. Brazil only.
* @param installment installment or {@code null} for none
*/
public Product setInstallment(Installment installment) {
this.installment = installment;
return this;
}
/**
* Whether the item is a merchant-defined bundle. A bundle is a custom grouping of different
* products sold by a merchant for a single price.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsBundle() {
return isBundle;
}
/**
* Whether the item is a merchant-defined bundle. A bundle is a custom grouping of different
* products sold by a merchant for a single price.
* @param isBundle isBundle or {@code null} for none
*/
public Product setIsBundle(java.lang.Boolean isBundle) {
this.isBundle = isBundle;
return this;
}
/**
* Shared identifier for all variants of the same product.
* @return value or {@code null} for none
*/
public java.lang.String getItemGroupId() {
return itemGroupId;
}
/**
* Shared identifier for all variants of the same product.
* @param itemGroupId itemGroupId or {@code null} for none
*/
public Product setItemGroupId(java.lang.String itemGroupId) {
this.itemGroupId = itemGroupId;
return this;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "`content#product`"
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "`content#product`"
* @param kind kind or {@code null} for none
*/
public Product setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* URL directly linking to your item's page on your website.
* @return value or {@code null} for none
*/
public java.lang.String getLink() {
return link;
}
/**
* URL directly linking to your item's page on your website.
* @param link link or {@code null} for none
*/
public Product setLink(java.lang.String link) {
this.link = link;
return this;
}
/**
* Loyalty points that users receive after purchasing the item. Japan only.
* @return value or {@code null} for none
*/
public LoyaltyPoints getLoyaltyPoints() {
return loyaltyPoints;
}
/**
* Loyalty points that users receive after purchasing the item. Japan only.
* @param loyaltyPoints loyaltyPoints or {@code null} for none
*/
public Product setLoyaltyPoints(LoyaltyPoints loyaltyPoints) {
this.loyaltyPoints = loyaltyPoints;
return this;
}
/**
* The material of which the item is made.
* @return value or {@code null} for none
*/
public java.lang.String getMaterial() {
return material;
}
/**
* The material of which the item is made.
* @param material material or {@code null} for none
*/
public Product setMaterial(java.lang.String material) {
this.material = material;
return this;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @return value or {@code null} for none
*/
public java.lang.String getMaxEnergyEfficiencyClass() {
return maxEnergyEfficiencyClass;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @param maxEnergyEfficiencyClass maxEnergyEfficiencyClass or {@code null} for none
*/
public Product setMaxEnergyEfficiencyClass(java.lang.String maxEnergyEfficiencyClass) {
this.maxEnergyEfficiencyClass = maxEnergyEfficiencyClass;
return this;
}
/**
* Maximal product handling time (in business days).
* @return value or {@code null} for none
*/
public java.lang.Long getMaxHandlingTime() {
return maxHandlingTime;
}
/**
* Maximal product handling time (in business days).
* @param maxHandlingTime maxHandlingTime or {@code null} for none
*/
public Product setMaxHandlingTime(java.lang.Long maxHandlingTime) {
this.maxHandlingTime = maxHandlingTime;
return this;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @return value or {@code null} for none
*/
public java.lang.String getMinEnergyEfficiencyClass() {
return minEnergyEfficiencyClass;
}
/**
* The energy efficiency class as defined in EU directive 2010/30/EU.
* @param minEnergyEfficiencyClass minEnergyEfficiencyClass or {@code null} for none
*/
public Product setMinEnergyEfficiencyClass(java.lang.String minEnergyEfficiencyClass) {
this.minEnergyEfficiencyClass = minEnergyEfficiencyClass;
return this;
}
/**
* Minimal product handling time (in business days).
* @return value or {@code null} for none
*/
public java.lang.Long getMinHandlingTime() {
return minHandlingTime;
}
/**
* Minimal product handling time (in business days).
* @param minHandlingTime minHandlingTime or {@code null} for none
*/
public Product setMinHandlingTime(java.lang.Long minHandlingTime) {
this.minHandlingTime = minHandlingTime;
return this;
}
/**
* Link to a mobile-optimized version of the landing page.
* @return value or {@code null} for none
*/
public java.lang.String getMobileLink() {
return mobileLink;
}
/**
* Link to a mobile-optimized version of the landing page.
* @param mobileLink mobileLink or {@code null} for none
*/
public Product setMobileLink(java.lang.String mobileLink) {
this.mobileLink = mobileLink;
return this;
}
/**
* Manufacturer Part Number (MPN) of the item.
* @return value or {@code null} for none
*/
public java.lang.String getMpn() {
return mpn;
}
/**
* Manufacturer Part Number (MPN) of the item.
* @param mpn mpn or {@code null} for none
*/
public Product setMpn(java.lang.String mpn) {
this.mpn = mpn;
return this;
}
/**
* The number of identical products in a merchant-defined multipack.
* @return value or {@code null} for none
*/
public java.lang.Long getMultipack() {
return multipack;
}
/**
* The number of identical products in a merchant-defined multipack.
* @param multipack multipack or {@code null} for none
*/
public Product setMultipack(java.lang.Long multipack) {
this.multipack = multipack;
return this;
}
/**
* Required. A unique identifier for the item. Leading and trailing whitespaces are stripped and
* multiple whitespaces are replaced by a single whitespace upon submission. Only valid unicode
* characters are accepted. See the products feed specification for details. Note: Content API
* methods that operate on products take the REST ID of the product, not this identifier.
* @return value or {@code null} for none
*/
public java.lang.String getOfferId() {
return offerId;
}
/**
* Required. A unique identifier for the item. Leading and trailing whitespaces are stripped and
* multiple whitespaces are replaced by a single whitespace upon submission. Only valid unicode
* characters are accepted. See the products feed specification for details. Note: Content API
* methods that operate on products take the REST ID of the product, not this identifier.
* @param offerId offerId or {@code null} for none
*/
public Product setOfferId(java.lang.String offerId) {
this.offerId = offerId;
return this;
}
/**
* The item's pattern (e.g. polka dots).
* @return value or {@code null} for none
*/
public java.lang.String getPattern() {
return pattern;
}
/**
* The item's pattern (e.g. polka dots).
* @param pattern pattern or {@code null} for none
*/
public Product setPattern(java.lang.String pattern) {
this.pattern = pattern;
return this;
}
/**
* Price of the item.
* @return value or {@code null} for none
*/
public Price getPrice() {
return price;
}
/**
* Price of the item.
* @param price price or {@code null} for none
*/
public Product setPrice(Price price) {
this.price = price;
return this;
}
/**
* Categories of the item (formatted as in products data specification).
* @return value or {@code null} for none
*/
public java.util.List getProductTypes() {
return productTypes;
}
/**
* Categories of the item (formatted as in products data specification).
* @param productTypes productTypes or {@code null} for none
*/
public Product setProductTypes(java.util.List productTypes) {
this.productTypes = productTypes;
return this;
}
/**
* The unique ID of a promotion.
* @return value or {@code null} for none
*/
public java.util.List getPromotionIds() {
return promotionIds;
}
/**
* The unique ID of a promotion.
* @param promotionIds promotionIds or {@code null} for none
*/
public Product setPromotionIds(java.util.List promotionIds) {
this.promotionIds = promotionIds;
return this;
}
/**
* Advertised sale price of the item.
* @return value or {@code null} for none
*/
public Price getSalePrice() {
return salePrice;
}
/**
* Advertised sale price of the item.
* @param salePrice salePrice or {@code null} for none
*/
public Product setSalePrice(Price salePrice) {
this.salePrice = salePrice;
return this;
}
/**
* Date range during which the item is on sale (see products data specification).
* @return value or {@code null} for none
*/
public java.lang.String getSalePriceEffectiveDate() {
return salePriceEffectiveDate;
}
/**
* Date range during which the item is on sale (see products data specification).
* @param salePriceEffectiveDate salePriceEffectiveDate or {@code null} for none
*/
public Product setSalePriceEffectiveDate(java.lang.String salePriceEffectiveDate) {
this.salePriceEffectiveDate = salePriceEffectiveDate;
return this;
}
/**
* The quantity of the product that is available for selling on Google. Supported only for online
* products.
* @return value or {@code null} for none
*/
public java.lang.Long getSellOnGoogleQuantity() {
return sellOnGoogleQuantity;
}
/**
* The quantity of the product that is available for selling on Google. Supported only for online
* products.
* @param sellOnGoogleQuantity sellOnGoogleQuantity or {@code null} for none
*/
public Product setSellOnGoogleQuantity(java.lang.Long sellOnGoogleQuantity) {
this.sellOnGoogleQuantity = sellOnGoogleQuantity;
return this;
}
/**
* Shipping rules.
* @return value or {@code null} for none
*/
public java.util.List getShipping() {
return shipping;
}
/**
* Shipping rules.
* @param shipping shipping or {@code null} for none
*/
public Product setShipping(java.util.List shipping) {
this.shipping = shipping;
return this;
}
/**
* Height of the item for shipping.
* @return value or {@code null} for none
*/
public ProductShippingDimension getShippingHeight() {
return shippingHeight;
}
/**
* Height of the item for shipping.
* @param shippingHeight shippingHeight or {@code null} for none
*/
public Product setShippingHeight(ProductShippingDimension shippingHeight) {
this.shippingHeight = shippingHeight;
return this;
}
/**
* The shipping label of the product, used to group product in account-level shipping rules.
* @return value or {@code null} for none
*/
public java.lang.String getShippingLabel() {
return shippingLabel;
}
/**
* The shipping label of the product, used to group product in account-level shipping rules.
* @param shippingLabel shippingLabel or {@code null} for none
*/
public Product setShippingLabel(java.lang.String shippingLabel) {
this.shippingLabel = shippingLabel;
return this;
}
/**
* Length of the item for shipping.
* @return value or {@code null} for none
*/
public ProductShippingDimension getShippingLength() {
return shippingLength;
}
/**
* Length of the item for shipping.
* @param shippingLength shippingLength or {@code null} for none
*/
public Product setShippingLength(ProductShippingDimension shippingLength) {
this.shippingLength = shippingLength;
return this;
}
/**
* Weight of the item for shipping.
* @return value or {@code null} for none
*/
public ProductShippingWeight getShippingWeight() {
return shippingWeight;
}
/**
* Weight of the item for shipping.
* @param shippingWeight shippingWeight or {@code null} for none
*/
public Product setShippingWeight(ProductShippingWeight shippingWeight) {
this.shippingWeight = shippingWeight;
return this;
}
/**
* Width of the item for shipping.
* @return value or {@code null} for none
*/
public ProductShippingDimension getShippingWidth() {
return shippingWidth;
}
/**
* Width of the item for shipping.
* @param shippingWidth shippingWidth or {@code null} for none
*/
public Product setShippingWidth(ProductShippingDimension shippingWidth) {
this.shippingWidth = shippingWidth;
return this;
}
/**
* System in which the size is specified. Recommended for apparel items.
* @return value or {@code null} for none
*/
public java.lang.String getSizeSystem() {
return sizeSystem;
}
/**
* System in which the size is specified. Recommended for apparel items.
* @param sizeSystem sizeSystem or {@code null} for none
*/
public Product setSizeSystem(java.lang.String sizeSystem) {
this.sizeSystem = sizeSystem;
return this;
}
/**
* The cut of the item. Recommended for apparel items.
* @return value or {@code null} for none
*/
public java.lang.String getSizeType() {
return sizeType;
}
/**
* The cut of the item. Recommended for apparel items.
* @param sizeType sizeType or {@code null} for none
*/
public Product setSizeType(java.lang.String sizeType) {
this.sizeType = sizeType;
return this;
}
/**
* Size of the item. Only one value is allowed. For variants with different sizes, insert a
* separate product for each size with the same `itemGroupId` value (see size definition).
* @return value or {@code null} for none
*/
public java.util.List getSizes() {
return sizes;
}
/**
* Size of the item. Only one value is allowed. For variants with different sizes, insert a
* separate product for each size with the same `itemGroupId` value (see size definition).
* @param sizes sizes or {@code null} for none
*/
public Product setSizes(java.util.List sizes) {
this.sizes = sizes;
return this;
}
/**
* The source of the offer, i.e., how the offer was created.
*
* Acceptable values are: - "`api`" - "`crawl`" - "`feed`"
* @return value or {@code null} for none
*/
public java.lang.String getSource() {
return source;
}
/**
* The source of the offer, i.e., how the offer was created.
*
* Acceptable values are: - "`api`" - "`crawl`" - "`feed`"
* @param source source or {@code null} for none
*/
public Product setSource(java.lang.String source) {
this.source = source;
return this;
}
/**
* Required. The CLDR territory code for the item.
* @return value or {@code null} for none
*/
public java.lang.String getTargetCountry() {
return targetCountry;
}
/**
* Required. The CLDR territory code for the item.
* @param targetCountry targetCountry or {@code null} for none
*/
public Product setTargetCountry(java.lang.String targetCountry) {
this.targetCountry = targetCountry;
return this;
}
/**
* The tax category of the product, used to configure detailed tax nexus in account-level tax
* settings.
* @return value or {@code null} for none
*/
public java.lang.String getTaxCategory() {
return taxCategory;
}
/**
* The tax category of the product, used to configure detailed tax nexus in account-level tax
* settings.
* @param taxCategory taxCategory or {@code null} for none
*/
public Product setTaxCategory(java.lang.String taxCategory) {
this.taxCategory = taxCategory;
return this;
}
/**
* Tax information.
* @return value or {@code null} for none
*/
public java.util.List getTaxes() {
return taxes;
}
/**
* Tax information.
* @param taxes taxes or {@code null} for none
*/
public Product setTaxes(java.util.List taxes) {
this.taxes = taxes;
return this;
}
/**
* Title of the item.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Title of the item.
* @param title title or {@code null} for none
*/
public Product setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* The transit time label of the product, used to group product in account-level transit time
* tables.
* @return value or {@code null} for none
*/
public java.lang.String getTransitTimeLabel() {
return transitTimeLabel;
}
/**
* The transit time label of the product, used to group product in account-level transit time
* tables.
* @param transitTimeLabel transitTimeLabel or {@code null} for none
*/
public Product setTransitTimeLabel(java.lang.String transitTimeLabel) {
this.transitTimeLabel = transitTimeLabel;
return this;
}
/**
* The preference of the denominator of the unit price.
* @return value or {@code null} for none
*/
public ProductUnitPricingBaseMeasure getUnitPricingBaseMeasure() {
return unitPricingBaseMeasure;
}
/**
* The preference of the denominator of the unit price.
* @param unitPricingBaseMeasure unitPricingBaseMeasure or {@code null} for none
*/
public Product setUnitPricingBaseMeasure(ProductUnitPricingBaseMeasure unitPricingBaseMeasure) {
this.unitPricingBaseMeasure = unitPricingBaseMeasure;
return this;
}
/**
* The measure and dimension of an item.
* @return value or {@code null} for none
*/
public ProductUnitPricingMeasure getUnitPricingMeasure() {
return unitPricingMeasure;
}
/**
* The measure and dimension of an item.
* @param unitPricingMeasure unitPricingMeasure or {@code null} for none
*/
public Product setUnitPricingMeasure(ProductUnitPricingMeasure unitPricingMeasure) {
this.unitPricingMeasure = unitPricingMeasure;
return this;
}
@Override
public Product set(String fieldName, Object value) {
return (Product) super.set(fieldName, value);
}
@Override
public Product clone() {
return (Product) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy