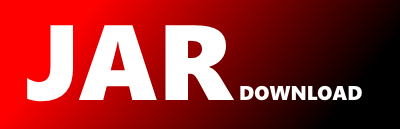
com.google.api.services.content.model.AccountImageImprovements Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* This improvement will attempt to automatically correct submitted images if they don't meet the
* [image requirements](https://support.google.com/merchants/answer/6324350), for example, removing
* overlays. If successful, the image will be replaced and approved. This improvement is only
* applied to images of disapproved offers. For more information see: [Automatic image
* improvements](https://support.google.com/merchants/answer/9242973)
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AccountImageImprovements extends com.google.api.client.json.GenericJson {
/**
* Determines how the images should be automatically updated. If this field is not present, then
* the settings will be deleted. If there are no settings for subaccount, they are inherited from
* aggregator.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AccountImageImprovementsSettings accountImageImprovementsSettings;
/**
* Output only. The effective value of allow_automatic_image_improvements. If
* account_image_improvements_settings is present, then this value is the same. Otherwise, it
* represents the inherited value of the parent account. Read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean effectiveAllowAutomaticImageImprovements;
/**
* Determines how the images should be automatically updated. If this field is not present, then
* the settings will be deleted. If there are no settings for subaccount, they are inherited from
* aggregator.
* @return value or {@code null} for none
*/
public AccountImageImprovementsSettings getAccountImageImprovementsSettings() {
return accountImageImprovementsSettings;
}
/**
* Determines how the images should be automatically updated. If this field is not present, then
* the settings will be deleted. If there are no settings for subaccount, they are inherited from
* aggregator.
* @param accountImageImprovementsSettings accountImageImprovementsSettings or {@code null} for none
*/
public AccountImageImprovements setAccountImageImprovementsSettings(AccountImageImprovementsSettings accountImageImprovementsSettings) {
this.accountImageImprovementsSettings = accountImageImprovementsSettings;
return this;
}
/**
* Output only. The effective value of allow_automatic_image_improvements. If
* account_image_improvements_settings is present, then this value is the same. Otherwise, it
* represents the inherited value of the parent account. Read-only.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEffectiveAllowAutomaticImageImprovements() {
return effectiveAllowAutomaticImageImprovements;
}
/**
* Output only. The effective value of allow_automatic_image_improvements. If
* account_image_improvements_settings is present, then this value is the same. Otherwise, it
* represents the inherited value of the parent account. Read-only.
* @param effectiveAllowAutomaticImageImprovements effectiveAllowAutomaticImageImprovements or {@code null} for none
*/
public AccountImageImprovements setEffectiveAllowAutomaticImageImprovements(java.lang.Boolean effectiveAllowAutomaticImageImprovements) {
this.effectiveAllowAutomaticImageImprovements = effectiveAllowAutomaticImageImprovements;
return this;
}
@Override
public AccountImageImprovements set(String fieldName, Object value) {
return (AccountImageImprovements) super.set(fieldName, value);
}
@Override
public AccountImageImprovements clone() {
return (AccountImageImprovements) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy