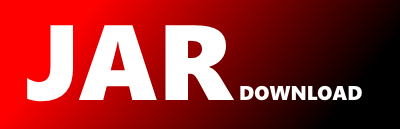
com.google.api.services.content.model.RepricingRuleRestriction Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Definition of a rule restriction. At least one of the following needs to be true: (1)
* use_auto_pricing_min_price is true (2) floor.price_delta exists (3) floor.percentage_delta exists
* If floor.price_delta and floor.percentage_delta are both set on a rule, the highest value will be
* chosen by the Repricer. In other words, for a product with a price of $50, if the
* `floor.percentage_delta` is "-10" and the floor.price_delta is "-12", the offer price will only
* be lowered $5 (10% lower than the original offer price).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RepricingRuleRestriction extends com.google.api.client.json.GenericJson {
/**
* The inclusive floor lower bound. The repricing rule only applies when new price >= floor.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RepricingRuleRestrictionBoundary floor;
/**
* If true, use the AUTO_PRICING_MIN_PRICE offer attribute as the lower bound of the rule. If
* use_auto_pricing_min_price is true, then only offers with `AUTO_PRICING_MIN_PRICE` existing on
* the offer will get Repricer treatment, even if a floor value is set on the rule. Also, if
* use_auto_pricing_min_price is true, the floor restriction will be ignored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useAutoPricingMinPrice;
/**
* The inclusive floor lower bound. The repricing rule only applies when new price >= floor.
* @return value or {@code null} for none
*/
public RepricingRuleRestrictionBoundary getFloor() {
return floor;
}
/**
* The inclusive floor lower bound. The repricing rule only applies when new price >= floor.
* @param floor floor or {@code null} for none
*/
public RepricingRuleRestriction setFloor(RepricingRuleRestrictionBoundary floor) {
this.floor = floor;
return this;
}
/**
* If true, use the AUTO_PRICING_MIN_PRICE offer attribute as the lower bound of the rule. If
* use_auto_pricing_min_price is true, then only offers with `AUTO_PRICING_MIN_PRICE` existing on
* the offer will get Repricer treatment, even if a floor value is set on the rule. Also, if
* use_auto_pricing_min_price is true, the floor restriction will be ignored.
* @return value or {@code null} for none
*/
public java.lang.Boolean getUseAutoPricingMinPrice() {
return useAutoPricingMinPrice;
}
/**
* If true, use the AUTO_PRICING_MIN_PRICE offer attribute as the lower bound of the rule. If
* use_auto_pricing_min_price is true, then only offers with `AUTO_PRICING_MIN_PRICE` existing on
* the offer will get Repricer treatment, even if a floor value is set on the rule. Also, if
* use_auto_pricing_min_price is true, the floor restriction will be ignored.
* @param useAutoPricingMinPrice useAutoPricingMinPrice or {@code null} for none
*/
public RepricingRuleRestriction setUseAutoPricingMinPrice(java.lang.Boolean useAutoPricingMinPrice) {
this.useAutoPricingMinPrice = useAutoPricingMinPrice;
return this;
}
@Override
public RepricingRuleRestriction set(String fieldName, Object value) {
return (RepricingRuleRestriction) super.set(fieldName, value);
}
@Override
public RepricingRuleRestriction clone() {
return (RepricingRuleRestriction) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy