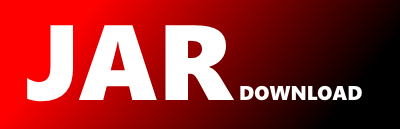
target.apidocs.com.google.api.services.content.model.OrderPromotion.html Maven / Gradle / Ivy
OrderPromotion (Content API for Shopping v2.1-rev20220413-1.32.1)
com.google.api.services.content.model
Class OrderPromotion
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.OrderPromotion
-
public final class OrderPromotion
extends GenericJson
Model definition for OrderPromotion.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
OrderPromotion()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
OrderPromotion
clone()
List<OrderPromotionItem>
getApplicableItems()
Items that this promotion may be applied to.
List<OrderPromotionItem>
getAppliedItems()
Items that this promotion have been applied to.
String
getEndTime()
Promotion end time in ISO 8601 format.
String
getFunder()
Required.
String
getMerchantPromotionId()
Required.
Price
getPriceValue()
Estimated discount applied to price.
String
getShortTitle()
A short title of the promotion to be shown on the checkout page.
String
getStartTime()
Promotion start time in ISO 8601 format.
String
getSubtype()
Required.
Price
getTaxValue()
Estimated discount applied to tax (if allowed by law).
String
getTitle()
Required.
String
getType()
Required.
OrderPromotion
set(String fieldName,
Object value)
OrderPromotion
setApplicableItems(List<OrderPromotionItem> applicableItems)
Items that this promotion may be applied to.
OrderPromotion
setAppliedItems(List<OrderPromotionItem> appliedItems)
Items that this promotion have been applied to.
OrderPromotion
setEndTime(String endTime)
Promotion end time in ISO 8601 format.
OrderPromotion
setFunder(String funder)
Required.
OrderPromotion
setMerchantPromotionId(String merchantPromotionId)
Required.
OrderPromotion
setPriceValue(Price priceValue)
Estimated discount applied to price.
OrderPromotion
setShortTitle(String shortTitle)
A short title of the promotion to be shown on the checkout page.
OrderPromotion
setStartTime(String startTime)
Promotion start time in ISO 8601 format.
OrderPromotion
setSubtype(String subtype)
Required.
OrderPromotion
setTaxValue(Price taxValue)
Estimated discount applied to tax (if allowed by law).
OrderPromotion
setTitle(String title)
Required.
OrderPromotion
setType(String type)
Required.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getApplicableItems
public List<OrderPromotionItem> getApplicableItems()
Items that this promotion may be applied to. If empty, there are no restrictions on applicable
items and quantity. This field will also be empty for shipping promotions because shipping is
not tied to any specific item.
- Returns:
- value or
null
for none
-
setApplicableItems
public OrderPromotion setApplicableItems(List<OrderPromotionItem> applicableItems)
Items that this promotion may be applied to. If empty, there are no restrictions on applicable
items and quantity. This field will also be empty for shipping promotions because shipping is
not tied to any specific item.
- Parameters:
applicableItems
- applicableItems or null
for none
-
getAppliedItems
public List<OrderPromotionItem> getAppliedItems()
Items that this promotion have been applied to. Do not provide for `orders.createtestorder`.
This field will be empty for shipping promotions because shipping is not tied to any specific
item.
- Returns:
- value or
null
for none
-
setAppliedItems
public OrderPromotion setAppliedItems(List<OrderPromotionItem> appliedItems)
Items that this promotion have been applied to. Do not provide for `orders.createtestorder`.
This field will be empty for shipping promotions because shipping is not tied to any specific
item.
- Parameters:
appliedItems
- appliedItems or null
for none
-
getEndTime
public String getEndTime()
Promotion end time in ISO 8601 format. Date, time, and offset required, for example,
"2020-01-02T09:00:00+01:00" or "2020-01-02T09:00:00Z".
- Returns:
- value or
null
for none
-
setEndTime
public OrderPromotion setEndTime(String endTime)
Promotion end time in ISO 8601 format. Date, time, and offset required, for example,
"2020-01-02T09:00:00+01:00" or "2020-01-02T09:00:00Z".
- Parameters:
endTime
- endTime or null
for none
-
getFunder
public String getFunder()
Required. The party funding the promotion. Only `merchant` is supported for
`orders.createtestorder`. Acceptable values are: - "`google`" - "`merchant`"
- Returns:
- value or
null
for none
-
setFunder
public OrderPromotion setFunder(String funder)
Required. The party funding the promotion. Only `merchant` is supported for
`orders.createtestorder`. Acceptable values are: - "`google`" - "`merchant`"
- Parameters:
funder
- funder or null
for none
-
getMerchantPromotionId
public String getMerchantPromotionId()
Required. This field is used to identify promotions within merchants' own systems.
- Returns:
- value or
null
for none
-
setMerchantPromotionId
public OrderPromotion setMerchantPromotionId(String merchantPromotionId)
Required. This field is used to identify promotions within merchants' own systems.
- Parameters:
merchantPromotionId
- merchantPromotionId or null
for none
-
getPriceValue
public Price getPriceValue()
Estimated discount applied to price. Amount is pre-tax or post-tax depending on location of
order.
- Returns:
- value or
null
for none
-
setPriceValue
public OrderPromotion setPriceValue(Price priceValue)
Estimated discount applied to price. Amount is pre-tax or post-tax depending on location of
order.
- Parameters:
priceValue
- priceValue or null
for none
-
getShortTitle
public String getShortTitle()
A short title of the promotion to be shown on the checkout page. Do not provide for
`orders.createtestorder`.
- Returns:
- value or
null
for none
-
setShortTitle
public OrderPromotion setShortTitle(String shortTitle)
A short title of the promotion to be shown on the checkout page. Do not provide for
`orders.createtestorder`.
- Parameters:
shortTitle
- shortTitle or null
for none
-
getStartTime
public String getStartTime()
Promotion start time in ISO 8601 format. Date, time, and offset required, for example,
"2020-01-02T09:00:00+01:00" or "2020-01-02T09:00:00Z".
- Returns:
- value or
null
for none
-
setStartTime
public OrderPromotion setStartTime(String startTime)
Promotion start time in ISO 8601 format. Date, time, and offset required, for example,
"2020-01-02T09:00:00+01:00" or "2020-01-02T09:00:00Z".
- Parameters:
startTime
- startTime or null
for none
-
getSubtype
public String getSubtype()
Required. The category of the promotion. Only `moneyOff` is supported for
`orders.createtestorder`. Acceptable values are: - "`buyMGetMoneyOff`" - "`buyMGetNMoneyOff`" -
"`buyMGetNPercentOff`" - "`buyMGetPercentOff`" - "`freeGift`" - "`freeGiftWithItemId`" -
"`freeGiftWithValue`" - "`freeShippingOvernight`" - "`freeShippingStandard`" -
"`freeShippingTwoDay`" - "`moneyOff`" - "`percentOff`" - "`rewardPoints`" - "`salePrice`"
- Returns:
- value or
null
for none
-
setSubtype
public OrderPromotion setSubtype(String subtype)
Required. The category of the promotion. Only `moneyOff` is supported for
`orders.createtestorder`. Acceptable values are: - "`buyMGetMoneyOff`" - "`buyMGetNMoneyOff`" -
"`buyMGetNPercentOff`" - "`buyMGetPercentOff`" - "`freeGift`" - "`freeGiftWithItemId`" -
"`freeGiftWithValue`" - "`freeShippingOvernight`" - "`freeShippingStandard`" -
"`freeShippingTwoDay`" - "`moneyOff`" - "`percentOff`" - "`rewardPoints`" - "`salePrice`"
- Parameters:
subtype
- subtype or null
for none
-
getTaxValue
public Price getTaxValue()
Estimated discount applied to tax (if allowed by law). Do not provide for
`orders.createtestorder`.
- Returns:
- value or
null
for none
-
setTaxValue
public OrderPromotion setTaxValue(Price taxValue)
Estimated discount applied to tax (if allowed by law). Do not provide for
`orders.createtestorder`.
- Parameters:
taxValue
- taxValue or null
for none
-
getTitle
public String getTitle()
Required. The title of the promotion.
- Returns:
- value or
null
for none
-
setTitle
public OrderPromotion setTitle(String title)
Required. The title of the promotion.
- Parameters:
title
- title or null
for none
-
getType
public String getType()
Required. The scope of the promotion. Only `product` is supported for `orders.createtestorder`.
Acceptable values are: - "`product`" - "`shipping`"
- Returns:
- value or
null
for none
-
setType
public OrderPromotion setType(String type)
Required. The scope of the promotion. Only `product` is supported for `orders.createtestorder`.
Acceptable values are: - "`product`" - "`shipping`"
- Parameters:
type
- type or null
for none
-
set
public OrderPromotion set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public OrderPromotion clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy