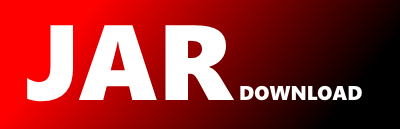
target.apidocs.com.google.api.services.content.model.OrdersUpdateShipmentRequest.html Maven / Gradle / Ivy
OrdersUpdateShipmentRequest (Content API for Shopping v2.1-rev20220413-1.32.1)
com.google.api.services.content.model
Class OrdersUpdateShipmentRequest
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.OrdersUpdateShipmentRequest
-
public final class OrdersUpdateShipmentRequest
extends GenericJson
Model definition for OrdersUpdateShipmentRequest.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
OrdersUpdateShipmentRequest()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
OrdersUpdateShipmentRequest
clone()
String
getCarrier()
The carrier handling the shipment.
String
getDeliveryDate()
Date on which the shipment has been delivered, in ISO 8601 format.
String
getLastPickupDate()
Date after which the pickup will expire, in ISO 8601 format.
String
getOperationId()
The ID of the operation.
String
getReadyPickupDate()
Date on which the shipment has been ready for pickup, in ISO 8601 format.
OrdersCustomBatchRequestEntryUpdateShipmentScheduledDeliveryDetails
getScheduledDeliveryDetails()
Delivery details of the shipment if scheduling is needed.
String
getShipmentId()
The ID of the shipment.
String
getStatus()
New status for the shipment.
String
getTrackingId()
The tracking ID for the shipment.
String
getUndeliveredDate()
Date on which the shipment has been undeliverable, in ISO 8601 format.
OrdersUpdateShipmentRequest
set(String fieldName,
Object value)
OrdersUpdateShipmentRequest
setCarrier(String carrier)
The carrier handling the shipment.
OrdersUpdateShipmentRequest
setDeliveryDate(String deliveryDate)
Date on which the shipment has been delivered, in ISO 8601 format.
OrdersUpdateShipmentRequest
setLastPickupDate(String lastPickupDate)
Date after which the pickup will expire, in ISO 8601 format.
OrdersUpdateShipmentRequest
setOperationId(String operationId)
The ID of the operation.
OrdersUpdateShipmentRequest
setReadyPickupDate(String readyPickupDate)
Date on which the shipment has been ready for pickup, in ISO 8601 format.
OrdersUpdateShipmentRequest
setScheduledDeliveryDetails(OrdersCustomBatchRequestEntryUpdateShipmentScheduledDeliveryDetails scheduledDeliveryDetails)
Delivery details of the shipment if scheduling is needed.
OrdersUpdateShipmentRequest
setShipmentId(String shipmentId)
The ID of the shipment.
OrdersUpdateShipmentRequest
setStatus(String status)
New status for the shipment.
OrdersUpdateShipmentRequest
setTrackingId(String trackingId)
The tracking ID for the shipment.
OrdersUpdateShipmentRequest
setUndeliveredDate(String undeliveredDate)
Date on which the shipment has been undeliverable, in ISO 8601 format.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCarrier
public String getCarrier()
The carrier handling the shipment. Not updated if missing. See `shipments[].carrier` in the
Orders resource representation for a list of acceptable values.
- Returns:
- value or
null
for none
-
setCarrier
public OrdersUpdateShipmentRequest setCarrier(String carrier)
The carrier handling the shipment. Not updated if missing. See `shipments[].carrier` in the
Orders resource representation for a list of acceptable values.
- Parameters:
carrier
- carrier or null
for none
-
getDeliveryDate
public String getDeliveryDate()
Date on which the shipment has been delivered, in ISO 8601 format. Optional and can be provided
only if `status` is `delivered`.
- Returns:
- value or
null
for none
-
setDeliveryDate
public OrdersUpdateShipmentRequest setDeliveryDate(String deliveryDate)
Date on which the shipment has been delivered, in ISO 8601 format. Optional and can be provided
only if `status` is `delivered`.
- Parameters:
deliveryDate
- deliveryDate or null
for none
-
getLastPickupDate
public String getLastPickupDate()
Date after which the pickup will expire, in ISO 8601 format. Required only when order is buy-
online-pickup-in-store(BOPIS) and `status` is `ready for pickup`.
- Returns:
- value or
null
for none
-
setLastPickupDate
public OrdersUpdateShipmentRequest setLastPickupDate(String lastPickupDate)
Date after which the pickup will expire, in ISO 8601 format. Required only when order is buy-
online-pickup-in-store(BOPIS) and `status` is `ready for pickup`.
- Parameters:
lastPickupDate
- lastPickupDate or null
for none
-
getOperationId
public String getOperationId()
The ID of the operation. Unique across all operations for a given order.
- Returns:
- value or
null
for none
-
setOperationId
public OrdersUpdateShipmentRequest setOperationId(String operationId)
The ID of the operation. Unique across all operations for a given order.
- Parameters:
operationId
- operationId or null
for none
-
getReadyPickupDate
public String getReadyPickupDate()
Date on which the shipment has been ready for pickup, in ISO 8601 format. Optional and can be
provided only if `status` is `ready for pickup`.
- Returns:
- value or
null
for none
-
setReadyPickupDate
public OrdersUpdateShipmentRequest setReadyPickupDate(String readyPickupDate)
Date on which the shipment has been ready for pickup, in ISO 8601 format. Optional and can be
provided only if `status` is `ready for pickup`.
- Parameters:
readyPickupDate
- readyPickupDate or null
for none
-
getScheduledDeliveryDetails
public OrdersCustomBatchRequestEntryUpdateShipmentScheduledDeliveryDetails getScheduledDeliveryDetails()
Delivery details of the shipment if scheduling is needed.
- Returns:
- value or
null
for none
-
setScheduledDeliveryDetails
public OrdersUpdateShipmentRequest setScheduledDeliveryDetails(OrdersCustomBatchRequestEntryUpdateShipmentScheduledDeliveryDetails scheduledDeliveryDetails)
Delivery details of the shipment if scheduling is needed.
- Parameters:
scheduledDeliveryDetails
- scheduledDeliveryDetails or null
for none
-
getShipmentId
public String getShipmentId()
The ID of the shipment.
- Returns:
- value or
null
for none
-
setShipmentId
public OrdersUpdateShipmentRequest setShipmentId(String shipmentId)
The ID of the shipment.
- Parameters:
shipmentId
- shipmentId or null
for none
-
getStatus
public String getStatus()
New status for the shipment. Not updated if missing. Acceptable values are: - "`delivered`" -
"`undeliverable`" - "`readyForPickup`"
- Returns:
- value or
null
for none
-
setStatus
public OrdersUpdateShipmentRequest setStatus(String status)
New status for the shipment. Not updated if missing. Acceptable values are: - "`delivered`" -
"`undeliverable`" - "`readyForPickup`"
- Parameters:
status
- status or null
for none
-
getTrackingId
public String getTrackingId()
The tracking ID for the shipment. Not updated if missing.
- Returns:
- value or
null
for none
-
setTrackingId
public OrdersUpdateShipmentRequest setTrackingId(String trackingId)
The tracking ID for the shipment. Not updated if missing.
- Parameters:
trackingId
- trackingId or null
for none
-
getUndeliveredDate
public String getUndeliveredDate()
Date on which the shipment has been undeliverable, in ISO 8601 format. Optional and can be
provided only if `status` is `undeliverable`.
- Returns:
- value or
null
for none
-
setUndeliveredDate
public OrdersUpdateShipmentRequest setUndeliveredDate(String undeliveredDate)
Date on which the shipment has been undeliverable, in ISO 8601 format. Optional and can be
provided only if `status` is `undeliverable`.
- Parameters:
undeliveredDate
- undeliveredDate or null
for none
-
set
public OrdersUpdateShipmentRequest set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public OrdersUpdateShipmentRequest clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2022 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy