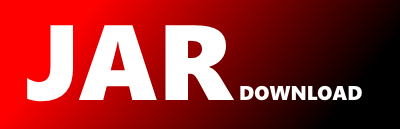
com.google.api.services.content.model.ProductView Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Product fields. Values are only set for fields requested explicitly in the request's search
* query.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ProductView extends com.google.api.client.json.GenericJson {
/**
* Aggregated destination status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String aggregatedDestinationStatus;
/**
* Availability of the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String availability;
/**
* Brand of the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String brand;
/**
* First level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String categoryL1;
/**
* Second level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String categoryL2;
/**
* Third level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String categoryL3;
/**
* Fourth level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String categoryL4;
/**
* Fifth level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String categoryL5;
/**
* Channel of the product (online versus local).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String channel;
/**
* Condition of the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String condition;
/**
* The time the merchant created the product in timestamp seconds.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String creationTime;
/**
* Product price currency code (for example, ISO 4217). Absent if product price is not available.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String currencyCode;
/**
* Expiration date for the product. Specified on insertion.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date expirationDate;
/**
* GTIN of the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List gtin;
/**
* The REST ID of the product, in the form of channel:contentLanguage:targetCountry:offerId.
* Content API methods that operate on products take this as their productId parameter. Should
* always be included in the SELECT clause.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* Item group ID provided by the merchant for grouping variants together.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String itemGroupId;
/**
* List of item issues for the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List itemIssues;
/**
* Language code of the product in BCP 47 format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/**
* Merchant-provided id of the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String offerId;
/**
* Product price specified as micros (1 millionth of a standard unit, 1 USD = 1000000 micros) in
* the product currency. Absent in case the information about the price of the product is not
* available.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long priceMicros;
/**
* First level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productTypeL1;
/**
* Second level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productTypeL2;
/**
* Third level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productTypeL3;
/**
* Fourth level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productTypeL4;
/**
* Fifth level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productTypeL5;
/**
* The normalized shipping label specified in the feed
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String shippingLabel;
/**
* Title of the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Aggregated destination status.
* @return value or {@code null} for none
*/
public java.lang.String getAggregatedDestinationStatus() {
return aggregatedDestinationStatus;
}
/**
* Aggregated destination status.
* @param aggregatedDestinationStatus aggregatedDestinationStatus or {@code null} for none
*/
public ProductView setAggregatedDestinationStatus(java.lang.String aggregatedDestinationStatus) {
this.aggregatedDestinationStatus = aggregatedDestinationStatus;
return this;
}
/**
* Availability of the product.
* @return value or {@code null} for none
*/
public java.lang.String getAvailability() {
return availability;
}
/**
* Availability of the product.
* @param availability availability or {@code null} for none
*/
public ProductView setAvailability(java.lang.String availability) {
this.availability = availability;
return this;
}
/**
* Brand of the product.
* @return value or {@code null} for none
*/
public java.lang.String getBrand() {
return brand;
}
/**
* Brand of the product.
* @param brand brand or {@code null} for none
*/
public ProductView setBrand(java.lang.String brand) {
this.brand = brand;
return this;
}
/**
* First level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getCategoryL1() {
return categoryL1;
}
/**
* First level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param categoryL1 categoryL1 or {@code null} for none
*/
public ProductView setCategoryL1(java.lang.String categoryL1) {
this.categoryL1 = categoryL1;
return this;
}
/**
* Second level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getCategoryL2() {
return categoryL2;
}
/**
* Second level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param categoryL2 categoryL2 or {@code null} for none
*/
public ProductView setCategoryL2(java.lang.String categoryL2) {
this.categoryL2 = categoryL2;
return this;
}
/**
* Third level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getCategoryL3() {
return categoryL3;
}
/**
* Third level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param categoryL3 categoryL3 or {@code null} for none
*/
public ProductView setCategoryL3(java.lang.String categoryL3) {
this.categoryL3 = categoryL3;
return this;
}
/**
* Fourth level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getCategoryL4() {
return categoryL4;
}
/**
* Fourth level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param categoryL4 categoryL4 or {@code null} for none
*/
public ProductView setCategoryL4(java.lang.String categoryL4) {
this.categoryL4 = categoryL4;
return this;
}
/**
* Fifth level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getCategoryL5() {
return categoryL5;
}
/**
* Fifth level of the product category in [Google's product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param categoryL5 categoryL5 or {@code null} for none
*/
public ProductView setCategoryL5(java.lang.String categoryL5) {
this.categoryL5 = categoryL5;
return this;
}
/**
* Channel of the product (online versus local).
* @return value or {@code null} for none
*/
public java.lang.String getChannel() {
return channel;
}
/**
* Channel of the product (online versus local).
* @param channel channel or {@code null} for none
*/
public ProductView setChannel(java.lang.String channel) {
this.channel = channel;
return this;
}
/**
* Condition of the product.
* @return value or {@code null} for none
*/
public java.lang.String getCondition() {
return condition;
}
/**
* Condition of the product.
* @param condition condition or {@code null} for none
*/
public ProductView setCondition(java.lang.String condition) {
this.condition = condition;
return this;
}
/**
* The time the merchant created the product in timestamp seconds.
* @return value or {@code null} for none
*/
public String getCreationTime() {
return creationTime;
}
/**
* The time the merchant created the product in timestamp seconds.
* @param creationTime creationTime or {@code null} for none
*/
public ProductView setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* Product price currency code (for example, ISO 4217). Absent if product price is not available.
* @return value or {@code null} for none
*/
public java.lang.String getCurrencyCode() {
return currencyCode;
}
/**
* Product price currency code (for example, ISO 4217). Absent if product price is not available.
* @param currencyCode currencyCode or {@code null} for none
*/
public ProductView setCurrencyCode(java.lang.String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/**
* Expiration date for the product. Specified on insertion.
* @return value or {@code null} for none
*/
public Date getExpirationDate() {
return expirationDate;
}
/**
* Expiration date for the product. Specified on insertion.
* @param expirationDate expirationDate or {@code null} for none
*/
public ProductView setExpirationDate(Date expirationDate) {
this.expirationDate = expirationDate;
return this;
}
/**
* GTIN of the product.
* @return value or {@code null} for none
*/
public java.util.List getGtin() {
return gtin;
}
/**
* GTIN of the product.
* @param gtin gtin or {@code null} for none
*/
public ProductView setGtin(java.util.List gtin) {
this.gtin = gtin;
return this;
}
/**
* The REST ID of the product, in the form of channel:contentLanguage:targetCountry:offerId.
* Content API methods that operate on products take this as their productId parameter. Should
* always be included in the SELECT clause.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The REST ID of the product, in the form of channel:contentLanguage:targetCountry:offerId.
* Content API methods that operate on products take this as their productId parameter. Should
* always be included in the SELECT clause.
* @param id id or {@code null} for none
*/
public ProductView setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Item group ID provided by the merchant for grouping variants together.
* @return value or {@code null} for none
*/
public java.lang.String getItemGroupId() {
return itemGroupId;
}
/**
* Item group ID provided by the merchant for grouping variants together.
* @param itemGroupId itemGroupId or {@code null} for none
*/
public ProductView setItemGroupId(java.lang.String itemGroupId) {
this.itemGroupId = itemGroupId;
return this;
}
/**
* List of item issues for the product.
* @return value or {@code null} for none
*/
public java.util.List getItemIssues() {
return itemIssues;
}
/**
* List of item issues for the product.
* @param itemIssues itemIssues or {@code null} for none
*/
public ProductView setItemIssues(java.util.List itemIssues) {
this.itemIssues = itemIssues;
return this;
}
/**
* Language code of the product in BCP 47 format.
* @return value or {@code null} for none
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* Language code of the product in BCP 47 format.
* @param languageCode languageCode or {@code null} for none
*/
public ProductView setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Merchant-provided id of the product.
* @return value or {@code null} for none
*/
public java.lang.String getOfferId() {
return offerId;
}
/**
* Merchant-provided id of the product.
* @param offerId offerId or {@code null} for none
*/
public ProductView setOfferId(java.lang.String offerId) {
this.offerId = offerId;
return this;
}
/**
* Product price specified as micros (1 millionth of a standard unit, 1 USD = 1000000 micros) in
* the product currency. Absent in case the information about the price of the product is not
* available.
* @return value or {@code null} for none
*/
public java.lang.Long getPriceMicros() {
return priceMicros;
}
/**
* Product price specified as micros (1 millionth of a standard unit, 1 USD = 1000000 micros) in
* the product currency. Absent in case the information about the price of the product is not
* available.
* @param priceMicros priceMicros or {@code null} for none
*/
public ProductView setPriceMicros(java.lang.Long priceMicros) {
this.priceMicros = priceMicros;
return this;
}
/**
* First level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getProductTypeL1() {
return productTypeL1;
}
/**
* First level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param productTypeL1 productTypeL1 or {@code null} for none
*/
public ProductView setProductTypeL1(java.lang.String productTypeL1) {
this.productTypeL1 = productTypeL1;
return this;
}
/**
* Second level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getProductTypeL2() {
return productTypeL2;
}
/**
* Second level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param productTypeL2 productTypeL2 or {@code null} for none
*/
public ProductView setProductTypeL2(java.lang.String productTypeL2) {
this.productTypeL2 = productTypeL2;
return this;
}
/**
* Third level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getProductTypeL3() {
return productTypeL3;
}
/**
* Third level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param productTypeL3 productTypeL3 or {@code null} for none
*/
public ProductView setProductTypeL3(java.lang.String productTypeL3) {
this.productTypeL3 = productTypeL3;
return this;
}
/**
* Fourth level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getProductTypeL4() {
return productTypeL4;
}
/**
* Fourth level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param productTypeL4 productTypeL4 or {@code null} for none
*/
public ProductView setProductTypeL4(java.lang.String productTypeL4) {
this.productTypeL4 = productTypeL4;
return this;
}
/**
* Fifth level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @return value or {@code null} for none
*/
public java.lang.String getProductTypeL5() {
return productTypeL5;
}
/**
* Fifth level of the product type in merchant's own [product
* taxonomy](https://support.google.com/merchants/answer/6324436).
* @param productTypeL5 productTypeL5 or {@code null} for none
*/
public ProductView setProductTypeL5(java.lang.String productTypeL5) {
this.productTypeL5 = productTypeL5;
return this;
}
/**
* The normalized shipping label specified in the feed
* @return value or {@code null} for none
*/
public java.lang.String getShippingLabel() {
return shippingLabel;
}
/**
* The normalized shipping label specified in the feed
* @param shippingLabel shippingLabel or {@code null} for none
*/
public ProductView setShippingLabel(java.lang.String shippingLabel) {
this.shippingLabel = shippingLabel;
return this;
}
/**
* Title of the product.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Title of the product.
* @param title title or {@code null} for none
*/
public ProductView setTitle(java.lang.String title) {
this.title = title;
return this;
}
@Override
public ProductView set(String fieldName, Object value) {
return (ProductView) super.set(fieldName, value);
}
@Override
public ProductView clone() {
return (ProductView) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy