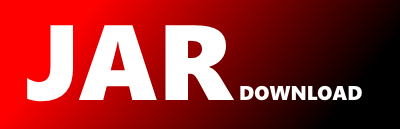
com.google.api.services.content.model.ReportRow Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Result row returned from the search query.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ReportRow extends com.google.api.client.json.GenericJson {
/**
* Best sellers fields requested by the merchant in the query. Field values are only set if the
* merchant queries `BestSellersProductClusterView` or `BestSellersBrandView`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BestSellers bestSellers;
/**
* Brand fields requested by the merchant in the query. Field values are only set if the merchant
* queries `BestSellersBrandView`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Brand brand;
/**
* Competitive visibility fields requested by the merchant in the query. Field values are only set
* if the merchant queries `CompetitiveVisibilityTopMerchantView`,
* `CompetitiveVisibilityBenchmarkView` or `CompetitiveVisibilityCompetitorView`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CompetitiveVisibility competitiveVisibility;
/**
* Metrics requested by the merchant in the query. Metric values are only set for metrics
* requested explicitly in the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Metrics metrics;
/**
* Price competitiveness fields requested by the merchant in the query. Field values are only set
* if the merchant queries `PriceCompetitivenessProductView`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PriceCompetitiveness priceCompetitiveness;
/**
* Price insights fields requested by the merchant in the query. Field values are only set if the
* merchant queries `PriceInsightsProductView`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PriceInsights priceInsights;
/**
* Product cluster fields requested by the merchant in the query. Field values are only set if the
* merchant queries `BestSellersProductClusterView`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductCluster productCluster;
/**
* Product fields requested by the merchant in the query. Field values are only set if the
* merchant queries `ProductView`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductView productView;
/**
* Segmentation dimensions requested by the merchant in the query. Dimension values are only set
* for dimensions requested explicitly in the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Segments segments;
/**
* Best sellers fields requested by the merchant in the query. Field values are only set if the
* merchant queries `BestSellersProductClusterView` or `BestSellersBrandView`.
* @return value or {@code null} for none
*/
public BestSellers getBestSellers() {
return bestSellers;
}
/**
* Best sellers fields requested by the merchant in the query. Field values are only set if the
* merchant queries `BestSellersProductClusterView` or `BestSellersBrandView`.
* @param bestSellers bestSellers or {@code null} for none
*/
public ReportRow setBestSellers(BestSellers bestSellers) {
this.bestSellers = bestSellers;
return this;
}
/**
* Brand fields requested by the merchant in the query. Field values are only set if the merchant
* queries `BestSellersBrandView`.
* @return value or {@code null} for none
*/
public Brand getBrand() {
return brand;
}
/**
* Brand fields requested by the merchant in the query. Field values are only set if the merchant
* queries `BestSellersBrandView`.
* @param brand brand or {@code null} for none
*/
public ReportRow setBrand(Brand brand) {
this.brand = brand;
return this;
}
/**
* Competitive visibility fields requested by the merchant in the query. Field values are only set
* if the merchant queries `CompetitiveVisibilityTopMerchantView`,
* `CompetitiveVisibilityBenchmarkView` or `CompetitiveVisibilityCompetitorView`.
* @return value or {@code null} for none
*/
public CompetitiveVisibility getCompetitiveVisibility() {
return competitiveVisibility;
}
/**
* Competitive visibility fields requested by the merchant in the query. Field values are only set
* if the merchant queries `CompetitiveVisibilityTopMerchantView`,
* `CompetitiveVisibilityBenchmarkView` or `CompetitiveVisibilityCompetitorView`.
* @param competitiveVisibility competitiveVisibility or {@code null} for none
*/
public ReportRow setCompetitiveVisibility(CompetitiveVisibility competitiveVisibility) {
this.competitiveVisibility = competitiveVisibility;
return this;
}
/**
* Metrics requested by the merchant in the query. Metric values are only set for metrics
* requested explicitly in the query.
* @return value or {@code null} for none
*/
public Metrics getMetrics() {
return metrics;
}
/**
* Metrics requested by the merchant in the query. Metric values are only set for metrics
* requested explicitly in the query.
* @param metrics metrics or {@code null} for none
*/
public ReportRow setMetrics(Metrics metrics) {
this.metrics = metrics;
return this;
}
/**
* Price competitiveness fields requested by the merchant in the query. Field values are only set
* if the merchant queries `PriceCompetitivenessProductView`.
* @return value or {@code null} for none
*/
public PriceCompetitiveness getPriceCompetitiveness() {
return priceCompetitiveness;
}
/**
* Price competitiveness fields requested by the merchant in the query. Field values are only set
* if the merchant queries `PriceCompetitivenessProductView`.
* @param priceCompetitiveness priceCompetitiveness or {@code null} for none
*/
public ReportRow setPriceCompetitiveness(PriceCompetitiveness priceCompetitiveness) {
this.priceCompetitiveness = priceCompetitiveness;
return this;
}
/**
* Price insights fields requested by the merchant in the query. Field values are only set if the
* merchant queries `PriceInsightsProductView`.
* @return value or {@code null} for none
*/
public PriceInsights getPriceInsights() {
return priceInsights;
}
/**
* Price insights fields requested by the merchant in the query. Field values are only set if the
* merchant queries `PriceInsightsProductView`.
* @param priceInsights priceInsights or {@code null} for none
*/
public ReportRow setPriceInsights(PriceInsights priceInsights) {
this.priceInsights = priceInsights;
return this;
}
/**
* Product cluster fields requested by the merchant in the query. Field values are only set if the
* merchant queries `BestSellersProductClusterView`.
* @return value or {@code null} for none
*/
public ProductCluster getProductCluster() {
return productCluster;
}
/**
* Product cluster fields requested by the merchant in the query. Field values are only set if the
* merchant queries `BestSellersProductClusterView`.
* @param productCluster productCluster or {@code null} for none
*/
public ReportRow setProductCluster(ProductCluster productCluster) {
this.productCluster = productCluster;
return this;
}
/**
* Product fields requested by the merchant in the query. Field values are only set if the
* merchant queries `ProductView`.
* @return value or {@code null} for none
*/
public ProductView getProductView() {
return productView;
}
/**
* Product fields requested by the merchant in the query. Field values are only set if the
* merchant queries `ProductView`.
* @param productView productView or {@code null} for none
*/
public ReportRow setProductView(ProductView productView) {
this.productView = productView;
return this;
}
/**
* Segmentation dimensions requested by the merchant in the query. Dimension values are only set
* for dimensions requested explicitly in the query.
* @return value or {@code null} for none
*/
public Segments getSegments() {
return segments;
}
/**
* Segmentation dimensions requested by the merchant in the query. Dimension values are only set
* for dimensions requested explicitly in the query.
* @param segments segments or {@code null} for none
*/
public ReportRow setSegments(Segments segments) {
this.segments = segments;
return this;
}
@Override
public ReportRow set(String fieldName, Object value) {
return (ReportRow) super.set(fieldName, value);
}
@Override
public ReportRow clone() {
return (ReportRow) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy