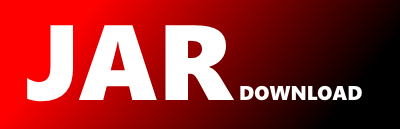
com.google.api.services.content.model.SettlementTransactionAmount Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* Model definition for SettlementTransactionAmount.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SettlementTransactionAmount extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SettlementTransactionAmountCommission commission;
/**
* The description of the event. Acceptable values are: - "`taxWithhold`" - "`principal`" -
* "`principalAdjustment`" - "`shippingFee`" - "`merchantRemittedSalesTax`" -
* "`googleRemittedSalesTax`" - "`merchantCoupon`" - "`merchantCouponTax`" -
* "`merchantRemittedDisposalTax`" - "`googleRemittedDisposalTax`" -
* "`merchantRemittedRedemptionFee`" - "`googleRemittedRedemptionFee`" - "`eeeEcoFee`" -
* "`furnitureEcoFee`" - "`copyPrivateFee`" - "`eeeEcoFeeCommission`" -
* "`furnitureEcoFeeCommission`" - "`copyPrivateFeeCommission`" - "`principalRefund`" -
* "`principalRefundTax`" - "`itemCommission`" - "`adjustmentCommission`" -
* "`shippingFeeCommission`" - "`commissionRefund`" - "`damaged`" - "`damagedOrDefectiveItem`" -
* "`expiredItem`" - "`faultyItem`" - "`incorrectItemReceived`" - "`itemMissing`" -
* "`qualityNotExpected`" - "`receivedTooLate`" - "`storePackageMissing`" -
* "`transitPackageMissing`" - "`unsuccessfulDeliveryUndeliverable`" - "`wrongChargeInStore`" -
* "`wrongItem`" - "`returns`" - "`undeliverable`" -
* "`issueRelatedRefundAndReplacementAmountDescription`" - "`refundFromMerchant`" -
* "`returnLabelShippingFee`" - "`lumpSumCorrection`" - "`pspFee`" - "`principalRefundDoesNotFit`"
* - "`principalRefundOrderedWrongItem`" - "`principalRefundQualityNotExpected`" -
* "`principalRefundBetterPriceFound`" - "`principalRefundNoLongerNeeded`" -
* "`principalRefundChangedMind`" - "`principalRefundReceivedTooLate`" -
* "`principalRefundIncorrectItemReceived`" - "`principalRefundDamagedOrDefectiveItem`" -
* "`principalRefundDidNotMatchDescription`" - "`principalRefundExpiredItem`"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The amount that contributes to the line item price.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Price transactionAmount;
/**
* The type of the amount. Acceptable values are: - "`itemPrice`" - "`orderPrice`" - "`refund`" -
* "`earlyRefund`" - "`courtesyRefund`" - "`returnRefund`" - "`returnLabelShippingFeeAmount`" -
* "`lumpSumCorrectionAmount`"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* @return value or {@code null} for none
*/
public SettlementTransactionAmountCommission getCommission() {
return commission;
}
/**
* @param commission commission or {@code null} for none
*/
public SettlementTransactionAmount setCommission(SettlementTransactionAmountCommission commission) {
this.commission = commission;
return this;
}
/**
* The description of the event. Acceptable values are: - "`taxWithhold`" - "`principal`" -
* "`principalAdjustment`" - "`shippingFee`" - "`merchantRemittedSalesTax`" -
* "`googleRemittedSalesTax`" - "`merchantCoupon`" - "`merchantCouponTax`" -
* "`merchantRemittedDisposalTax`" - "`googleRemittedDisposalTax`" -
* "`merchantRemittedRedemptionFee`" - "`googleRemittedRedemptionFee`" - "`eeeEcoFee`" -
* "`furnitureEcoFee`" - "`copyPrivateFee`" - "`eeeEcoFeeCommission`" -
* "`furnitureEcoFeeCommission`" - "`copyPrivateFeeCommission`" - "`principalRefund`" -
* "`principalRefundTax`" - "`itemCommission`" - "`adjustmentCommission`" -
* "`shippingFeeCommission`" - "`commissionRefund`" - "`damaged`" - "`damagedOrDefectiveItem`" -
* "`expiredItem`" - "`faultyItem`" - "`incorrectItemReceived`" - "`itemMissing`" -
* "`qualityNotExpected`" - "`receivedTooLate`" - "`storePackageMissing`" -
* "`transitPackageMissing`" - "`unsuccessfulDeliveryUndeliverable`" - "`wrongChargeInStore`" -
* "`wrongItem`" - "`returns`" - "`undeliverable`" -
* "`issueRelatedRefundAndReplacementAmountDescription`" - "`refundFromMerchant`" -
* "`returnLabelShippingFee`" - "`lumpSumCorrection`" - "`pspFee`" - "`principalRefundDoesNotFit`"
* - "`principalRefundOrderedWrongItem`" - "`principalRefundQualityNotExpected`" -
* "`principalRefundBetterPriceFound`" - "`principalRefundNoLongerNeeded`" -
* "`principalRefundChangedMind`" - "`principalRefundReceivedTooLate`" -
* "`principalRefundIncorrectItemReceived`" - "`principalRefundDamagedOrDefectiveItem`" -
* "`principalRefundDidNotMatchDescription`" - "`principalRefundExpiredItem`"
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The description of the event. Acceptable values are: - "`taxWithhold`" - "`principal`" -
* "`principalAdjustment`" - "`shippingFee`" - "`merchantRemittedSalesTax`" -
* "`googleRemittedSalesTax`" - "`merchantCoupon`" - "`merchantCouponTax`" -
* "`merchantRemittedDisposalTax`" - "`googleRemittedDisposalTax`" -
* "`merchantRemittedRedemptionFee`" - "`googleRemittedRedemptionFee`" - "`eeeEcoFee`" -
* "`furnitureEcoFee`" - "`copyPrivateFee`" - "`eeeEcoFeeCommission`" -
* "`furnitureEcoFeeCommission`" - "`copyPrivateFeeCommission`" - "`principalRefund`" -
* "`principalRefundTax`" - "`itemCommission`" - "`adjustmentCommission`" -
* "`shippingFeeCommission`" - "`commissionRefund`" - "`damaged`" - "`damagedOrDefectiveItem`" -
* "`expiredItem`" - "`faultyItem`" - "`incorrectItemReceived`" - "`itemMissing`" -
* "`qualityNotExpected`" - "`receivedTooLate`" - "`storePackageMissing`" -
* "`transitPackageMissing`" - "`unsuccessfulDeliveryUndeliverable`" - "`wrongChargeInStore`" -
* "`wrongItem`" - "`returns`" - "`undeliverable`" -
* "`issueRelatedRefundAndReplacementAmountDescription`" - "`refundFromMerchant`" -
* "`returnLabelShippingFee`" - "`lumpSumCorrection`" - "`pspFee`" - "`principalRefundDoesNotFit`"
* - "`principalRefundOrderedWrongItem`" - "`principalRefundQualityNotExpected`" -
* "`principalRefundBetterPriceFound`" - "`principalRefundNoLongerNeeded`" -
* "`principalRefundChangedMind`" - "`principalRefundReceivedTooLate`" -
* "`principalRefundIncorrectItemReceived`" - "`principalRefundDamagedOrDefectiveItem`" -
* "`principalRefundDidNotMatchDescription`" - "`principalRefundExpiredItem`"
* @param description description or {@code null} for none
*/
public SettlementTransactionAmount setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The amount that contributes to the line item price.
* @return value or {@code null} for none
*/
public Price getTransactionAmount() {
return transactionAmount;
}
/**
* The amount that contributes to the line item price.
* @param transactionAmount transactionAmount or {@code null} for none
*/
public SettlementTransactionAmount setTransactionAmount(Price transactionAmount) {
this.transactionAmount = transactionAmount;
return this;
}
/**
* The type of the amount. Acceptable values are: - "`itemPrice`" - "`orderPrice`" - "`refund`" -
* "`earlyRefund`" - "`courtesyRefund`" - "`returnRefund`" - "`returnLabelShippingFeeAmount`" -
* "`lumpSumCorrectionAmount`"
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The type of the amount. Acceptable values are: - "`itemPrice`" - "`orderPrice`" - "`refund`" -
* "`earlyRefund`" - "`courtesyRefund`" - "`returnRefund`" - "`returnLabelShippingFeeAmount`" -
* "`lumpSumCorrectionAmount`"
* @param type type or {@code null} for none
*/
public SettlementTransactionAmount setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public SettlementTransactionAmount set(String fieldName, Object value) {
return (SettlementTransactionAmount) super.set(fieldName, value);
}
@Override
public SettlementTransactionAmount clone() {
return (SettlementTransactionAmount) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy