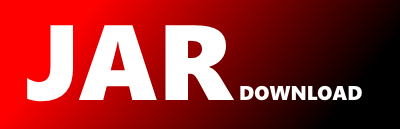
target.apidocs.com.google.api.services.content.model.Account.html Maven / Gradle / Ivy
Account (Content API for Shopping v2.1-rev20240609-2.0.0)
com.google.api.services.content.model
Class Account
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.Account
-
public final class Account
extends com.google.api.client.json.GenericJson
Account data. After the creation of a new account it may take a few minutes before it's fully
operational. The methods delete, insert, and update require the admin role.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Account()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Account
clone()
String
getAccountManagement()
Output only.
List<AccountAdsLink>
getAdsLinks()
Linked Ads accounts that are active or pending approval.
Boolean
getAdultContent()
Indicates whether the merchant sells adult content.
AccountAutomaticImprovements
getAutomaticImprovements()
The automatic improvements of the account can be used to automatically update items, improve
images and shipping.
List<BigInteger>
getAutomaticLabelIds()
Automatically created label IDs that are assigned to the account by CSS Center.
AccountBusinessIdentity
getBusinessIdentity()
The business identity attributes can be used to self-declare attributes that let customers know
more about your business.
AccountBusinessInformation
getBusinessInformation()
The business information of the account.
AccountConversionSettings
getConversionSettings()
Settings for conversion tracking.
BigInteger
getCssId()
ID of CSS the account belongs to.
AccountGoogleMyBusinessLink
getGoogleMyBusinessLink()
The Business Profile which is linked or in the process of being linked with the Merchant Center
account.
BigInteger
getId()
Required.
String
getKind()
Identifies what kind of resource this is.
List<BigInteger>
getLabelIds()
Manually created label IDs that are assigned to the account by CSS.
String
getName()
Required.
String
getSellerId()
Client-specific, locally-unique, internal ID for the child account.
List<AccountUser>
getUsers()
Users with access to the account.
String
getWebsiteUrl()
The merchant's website.
List<AccountYouTubeChannelLink>
getYoutubeChannelLinks()
Linked YouTube channels that are active or pending approval.
Account
set(String fieldName,
Object value)
Account
setAccountManagement(String accountManagement)
Output only.
Account
setAdsLinks(List<AccountAdsLink> adsLinks)
Linked Ads accounts that are active or pending approval.
Account
setAdultContent(Boolean adultContent)
Indicates whether the merchant sells adult content.
Account
setAutomaticImprovements(AccountAutomaticImprovements automaticImprovements)
The automatic improvements of the account can be used to automatically update items, improve
images and shipping.
Account
setAutomaticLabelIds(List<BigInteger> automaticLabelIds)
Automatically created label IDs that are assigned to the account by CSS Center.
Account
setBusinessIdentity(AccountBusinessIdentity businessIdentity)
The business identity attributes can be used to self-declare attributes that let customers know
more about your business.
Account
setBusinessInformation(AccountBusinessInformation businessInformation)
The business information of the account.
Account
setConversionSettings(AccountConversionSettings conversionSettings)
Settings for conversion tracking.
Account
setCssId(BigInteger cssId)
ID of CSS the account belongs to.
Account
setGoogleMyBusinessLink(AccountGoogleMyBusinessLink googleMyBusinessLink)
The Business Profile which is linked or in the process of being linked with the Merchant Center
account.
Account
setId(BigInteger id)
Required.
Account
setKind(String kind)
Identifies what kind of resource this is.
Account
setLabelIds(List<BigInteger> labelIds)
Manually created label IDs that are assigned to the account by CSS.
Account
setName(String name)
Required.
Account
setSellerId(String sellerId)
Client-specific, locally-unique, internal ID for the child account.
Account
setUsers(List<AccountUser> users)
Users with access to the account.
Account
setWebsiteUrl(String websiteUrl)
The merchant's website.
Account
setYoutubeChannelLinks(List<AccountYouTubeChannelLink> youtubeChannelLinks)
Linked YouTube channels that are active or pending approval.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAccountManagement
public String getAccountManagement()
Output only. How the account is managed. Acceptable values are: - "`manual`" - "`automatic`"
- Returns:
- value or
null
for none
-
setAccountManagement
public Account setAccountManagement(String accountManagement)
Output only. How the account is managed. Acceptable values are: - "`manual`" - "`automatic`"
- Parameters:
accountManagement
- accountManagement or null
for none
-
getAdsLinks
public List<AccountAdsLink> getAdsLinks()
Linked Ads accounts that are active or pending approval. To create a new link request, add a
new link with status `active` to the list. It will remain in a `pending` state until approved
or rejected either in the Ads interface or through the Google Ads API. To delete an active
link, or to cancel a link request, remove it from the list.
- Returns:
- value or
null
for none
-
setAdsLinks
public Account setAdsLinks(List<AccountAdsLink> adsLinks)
Linked Ads accounts that are active or pending approval. To create a new link request, add a
new link with status `active` to the list. It will remain in a `pending` state until approved
or rejected either in the Ads interface or through the Google Ads API. To delete an active
link, or to cancel a link request, remove it from the list.
- Parameters:
adsLinks
- adsLinks or null
for none
-
getAdultContent
public Boolean getAdultContent()
Indicates whether the merchant sells adult content.
- Returns:
- value or
null
for none
-
setAdultContent
public Account setAdultContent(Boolean adultContent)
Indicates whether the merchant sells adult content.
- Parameters:
adultContent
- adultContent or null
for none
-
getAutomaticImprovements
public AccountAutomaticImprovements getAutomaticImprovements()
The automatic improvements of the account can be used to automatically update items, improve
images and shipping. Each section inside AutomaticImprovements is updated separately.
- Returns:
- value or
null
for none
-
setAutomaticImprovements
public Account setAutomaticImprovements(AccountAutomaticImprovements automaticImprovements)
The automatic improvements of the account can be used to automatically update items, improve
images and shipping. Each section inside AutomaticImprovements is updated separately.
- Parameters:
automaticImprovements
- automaticImprovements or null
for none
-
getAutomaticLabelIds
public List<BigInteger> getAutomaticLabelIds()
Automatically created label IDs that are assigned to the account by CSS Center.
- Returns:
- value or
null
for none
-
setAutomaticLabelIds
public Account setAutomaticLabelIds(List<BigInteger> automaticLabelIds)
Automatically created label IDs that are assigned to the account by CSS Center.
- Parameters:
automaticLabelIds
- automaticLabelIds or null
for none
-
getBusinessIdentity
public AccountBusinessIdentity getBusinessIdentity()
The business identity attributes can be used to self-declare attributes that let customers know
more about your business.
- Returns:
- value or
null
for none
-
setBusinessIdentity
public Account setBusinessIdentity(AccountBusinessIdentity businessIdentity)
The business identity attributes can be used to self-declare attributes that let customers know
more about your business.
- Parameters:
businessIdentity
- businessIdentity or null
for none
-
getBusinessInformation
public AccountBusinessInformation getBusinessInformation()
The business information of the account.
- Returns:
- value or
null
for none
-
setBusinessInformation
public Account setBusinessInformation(AccountBusinessInformation businessInformation)
The business information of the account.
- Parameters:
businessInformation
- businessInformation or null
for none
-
getConversionSettings
public AccountConversionSettings getConversionSettings()
Settings for conversion tracking.
- Returns:
- value or
null
for none
-
setConversionSettings
public Account setConversionSettings(AccountConversionSettings conversionSettings)
Settings for conversion tracking.
- Parameters:
conversionSettings
- conversionSettings or null
for none
-
getCssId
public BigInteger getCssId()
ID of CSS the account belongs to.
- Returns:
- value or
null
for none
-
setCssId
public Account setCssId(BigInteger cssId)
ID of CSS the account belongs to.
- Parameters:
cssId
- cssId or null
for none
-
getGoogleMyBusinessLink
public AccountGoogleMyBusinessLink getGoogleMyBusinessLink()
The Business Profile which is linked or in the process of being linked with the Merchant Center
account.
- Returns:
- value or
null
for none
-
setGoogleMyBusinessLink
public Account setGoogleMyBusinessLink(AccountGoogleMyBusinessLink googleMyBusinessLink)
The Business Profile which is linked or in the process of being linked with the Merchant Center
account.
- Parameters:
googleMyBusinessLink
- googleMyBusinessLink or null
for none
-
getId
public BigInteger getId()
Required. 64-bit Merchant Center account ID.
- Returns:
- value or
null
for none
-
setId
public Account setId(BigInteger id)
Required. 64-bit Merchant Center account ID.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
Identifies what kind of resource this is. Value: the fixed string "`content#account`".
- Returns:
- value or
null
for none
-
setKind
public Account setKind(String kind)
Identifies what kind of resource this is. Value: the fixed string "`content#account`".
- Parameters:
kind
- kind or null
for none
-
getLabelIds
public List<BigInteger> getLabelIds()
Manually created label IDs that are assigned to the account by CSS.
- Returns:
- value or
null
for none
-
setLabelIds
public Account setLabelIds(List<BigInteger> labelIds)
Manually created label IDs that are assigned to the account by CSS.
- Parameters:
labelIds
- labelIds or null
for none
-
getName
public String getName()
Required. Display name for the account.
- Returns:
- value or
null
for none
-
setName
public Account setName(String name)
Required. Display name for the account.
- Parameters:
name
- name or null
for none
-
getSellerId
public String getSellerId()
Client-specific, locally-unique, internal ID for the child account.
- Returns:
- value or
null
for none
-
setSellerId
public Account setSellerId(String sellerId)
Client-specific, locally-unique, internal ID for the child account.
- Parameters:
sellerId
- sellerId or null
for none
-
getUsers
public List<AccountUser> getUsers()
Users with access to the account. Every account (except for subaccounts) must have at least one
admin user.
- Returns:
- value or
null
for none
-
setUsers
public Account setUsers(List<AccountUser> users)
Users with access to the account. Every account (except for subaccounts) must have at least one
admin user.
- Parameters:
users
- users or null
for none
-
getWebsiteUrl
public String getWebsiteUrl()
The merchant's website.
- Returns:
- value or
null
for none
-
setWebsiteUrl
public Account setWebsiteUrl(String websiteUrl)
The merchant's website.
- Parameters:
websiteUrl
- websiteUrl or null
for none
-
getYoutubeChannelLinks
public List<AccountYouTubeChannelLink> getYoutubeChannelLinks()
Linked YouTube channels that are active or pending approval. To create a new link request, add
a new link with status `active` to the list. It will remain in a `pending` state until approved
or rejected in the YT Creator Studio interface. To delete an active link, or to cancel a link
request, remove it from the list.
- Returns:
- value or
null
for none
-
setYoutubeChannelLinks
public Account setYoutubeChannelLinks(List<AccountYouTubeChannelLink> youtubeChannelLinks)
Linked YouTube channels that are active or pending approval. To create a new link request, add
a new link with status `active` to the list. It will remain in a `pending` state until approved
or rejected in the YT Creator Studio interface. To delete an active link, or to cancel a link
request, remove it from the list.
- Parameters:
youtubeChannelLinks
- youtubeChannelLinks or null
for none
-
set
public Account set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Account clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy