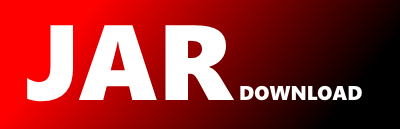
target.apidocs.com.google.api.services.content.model.CheckoutSettings.html Maven / Gradle / Ivy
CheckoutSettings (Content API for Shopping v2.1-rev20240609-2.0.0)
com.google.api.services.content.model
Class CheckoutSettings
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.CheckoutSettings
-
public final class CheckoutSettings
extends com.google.api.client.json.GenericJson
`CheckoutSettings` for a specific merchant ID.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
CheckoutSettings()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
CheckoutSettings
clone()
String
getEffectiveEnrollmentState()
Output only.
String
getEffectiveReviewState()
Output only.
UrlSettings
getEffectiveUriSettings()
The effective value of `url_settings` for a given merchant ID.
String
getEnrollmentState()
Output only.
Long
getMerchantId()
Required.
String
getReviewState()
Output only.
UrlSettings
getUriSettings()
URL settings for cart or checkout URL.
CheckoutSettings
set(String fieldName,
Object value)
CheckoutSettings
setEffectiveEnrollmentState(String effectiveEnrollmentState)
Output only.
CheckoutSettings
setEffectiveReviewState(String effectiveReviewState)
Output only.
CheckoutSettings
setEffectiveUriSettings(UrlSettings effectiveUriSettings)
The effective value of `url_settings` for a given merchant ID.
CheckoutSettings
setEnrollmentState(String enrollmentState)
Output only.
CheckoutSettings
setMerchantId(Long merchantId)
Required.
CheckoutSettings
setReviewState(String reviewState)
Output only.
CheckoutSettings
setUriSettings(UrlSettings uriSettings)
URL settings for cart or checkout URL.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getEffectiveEnrollmentState
public String getEffectiveEnrollmentState()
Output only. The effective value of enrollment state for a given merchant ID. If account level
settings are present then this value will be a copy of the account level settings. Otherwise,
it will have the value of the parent account.
- Returns:
- value or
null
for none
-
setEffectiveEnrollmentState
public CheckoutSettings setEffectiveEnrollmentState(String effectiveEnrollmentState)
Output only. The effective value of enrollment state for a given merchant ID. If account level
settings are present then this value will be a copy of the account level settings. Otherwise,
it will have the value of the parent account.
- Parameters:
effectiveEnrollmentState
- effectiveEnrollmentState or null
for none
-
getEffectiveReviewState
public String getEffectiveReviewState()
Output only. The effective value of review state for a given merchant ID. If account level
settings are present then this value will be a copy of the account level settings. Otherwise,
it will have the value of the parent account.
- Returns:
- value or
null
for none
-
setEffectiveReviewState
public CheckoutSettings setEffectiveReviewState(String effectiveReviewState)
Output only. The effective value of review state for a given merchant ID. If account level
settings are present then this value will be a copy of the account level settings. Otherwise,
it will have the value of the parent account.
- Parameters:
effectiveReviewState
- effectiveReviewState or null
for none
-
getEffectiveUriSettings
public UrlSettings getEffectiveUriSettings()
The effective value of `url_settings` for a given merchant ID. If account level settings are
present then this value will be a copy of the account level settings. Otherwise, it will have
the value of the parent account.
- Returns:
- value or
null
for none
-
setEffectiveUriSettings
public CheckoutSettings setEffectiveUriSettings(UrlSettings effectiveUriSettings)
The effective value of `url_settings` for a given merchant ID. If account level settings are
present then this value will be a copy of the account level settings. Otherwise, it will have
the value of the parent account.
- Parameters:
effectiveUriSettings
- effectiveUriSettings or null
for none
-
getEnrollmentState
public String getEnrollmentState()
Output only. Reflects the merchant enrollment state in `Checkout` feature.
- Returns:
- value or
null
for none
-
setEnrollmentState
public CheckoutSettings setEnrollmentState(String enrollmentState)
Output only. Reflects the merchant enrollment state in `Checkout` feature.
- Parameters:
enrollmentState
- enrollmentState or null
for none
-
getMerchantId
public Long getMerchantId()
Required. The ID of the account.
- Returns:
- value or
null
for none
-
setMerchantId
public CheckoutSettings setMerchantId(Long merchantId)
Required. The ID of the account.
- Parameters:
merchantId
- merchantId or null
for none
-
getReviewState
public String getReviewState()
Output only. Reflects the merchant review state in `Checkout` feature. This is set based on the
data quality reviews of the URL provided by the merchant. A merchant with enrollment state as
`ENROLLED` can be in the following review states: `IN_REVIEW`, `APPROVED` or `DISAPPROVED`. A
merchant must be in an enrollment_state of `ENROLLED` before a review can begin for the
merchant.
- Returns:
- value or
null
for none
-
setReviewState
public CheckoutSettings setReviewState(String reviewState)
Output only. Reflects the merchant review state in `Checkout` feature. This is set based on the
data quality reviews of the URL provided by the merchant. A merchant with enrollment state as
`ENROLLED` can be in the following review states: `IN_REVIEW`, `APPROVED` or `DISAPPROVED`. A
merchant must be in an enrollment_state of `ENROLLED` before a review can begin for the
merchant.
- Parameters:
reviewState
- reviewState or null
for none
-
getUriSettings
public UrlSettings getUriSettings()
URL settings for cart or checkout URL.
- Returns:
- value or
null
for none
-
setUriSettings
public CheckoutSettings setUriSettings(UrlSettings uriSettings)
URL settings for cart or checkout URL.
- Parameters:
uriSettings
- uriSettings or null
for none
-
set
public CheckoutSettings set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public CheckoutSettings clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy