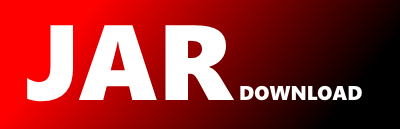
target.apidocs.com.google.api.services.content.model.DateTime.html Maven / Gradle / Ivy
DateTime (Content API for Shopping v2.1-rev20240609-2.0.0)
com.google.api.services.content.model
Class DateTime
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.DateTime
-
public final class DateTime
extends com.google.api.client.json.GenericJson
Represents civil time (or occasionally physical time). This type can represent a civil time in
one of a few possible ways: * When utc_offset is set and time_zone is unset: a civil time on a
calendar day with a particular offset from UTC. * When time_zone is set and utc_offset is unset:
a civil time on a calendar day in a particular time zone. * When neither time_zone nor utc_offset
is set: a civil time on a calendar day in local time. The date is relative to the Proleptic
Gregorian Calendar. If year, month, or day are 0, the DateTime is considered not to have a
specific year, month, or day respectively. This type may also be used to represent a physical
time if all the date and time fields are set and either case of the `time_offset` oneof is set.
Consider using `Timestamp` message for physical time instead. If your use case also would like to
store the user's timezone, that can be done in another field. This type is more flexible than
some applications may want. Make sure to document and validate your application's limitations.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
DateTime()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
DateTime
clone()
Integer
getDay()
Optional.
Integer
getHours()
Optional.
Integer
getMinutes()
Optional.
Integer
getMonth()
Optional.
Integer
getNanos()
Optional.
Integer
getSeconds()
Optional.
TimeZone
getTimeZone()
Time zone.
String
getUtcOffset()
UTC offset.
Integer
getYear()
Optional.
DateTime
set(String fieldName,
Object value)
DateTime
setDay(Integer day)
Optional.
DateTime
setHours(Integer hours)
Optional.
DateTime
setMinutes(Integer minutes)
Optional.
DateTime
setMonth(Integer month)
Optional.
DateTime
setNanos(Integer nanos)
Optional.
DateTime
setSeconds(Integer seconds)
Optional.
DateTime
setTimeZone(TimeZone timeZone)
Time zone.
DateTime
setUtcOffset(String utcOffset)
UTC offset.
DateTime
setYear(Integer year)
Optional.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getDay
public Integer getDay()
Optional. Day of month. Must be from 1 to 31 and valid for the year and month, or 0 if
specifying a datetime without a day.
- Returns:
- value or
null
for none
-
setDay
public DateTime setDay(Integer day)
Optional. Day of month. Must be from 1 to 31 and valid for the year and month, or 0 if
specifying a datetime without a day.
- Parameters:
day
- day or null
for none
-
getHours
public Integer getHours()
Optional. Hours of day in 24 hour format. Should be from 0 to 23, defaults to 0 (midnight). An
API may choose to allow the value "24:00:00" for scenarios like business closing time.
- Returns:
- value or
null
for none
-
setHours
public DateTime setHours(Integer hours)
Optional. Hours of day in 24 hour format. Should be from 0 to 23, defaults to 0 (midnight). An
API may choose to allow the value "24:00:00" for scenarios like business closing time.
- Parameters:
hours
- hours or null
for none
-
getMinutes
public Integer getMinutes()
Optional. Minutes of hour of day. Must be from 0 to 59, defaults to 0.
- Returns:
- value or
null
for none
-
setMinutes
public DateTime setMinutes(Integer minutes)
Optional. Minutes of hour of day. Must be from 0 to 59, defaults to 0.
- Parameters:
minutes
- minutes or null
for none
-
getMonth
public Integer getMonth()
Optional. Month of year. Must be from 1 to 12, or 0 if specifying a datetime without a month.
- Returns:
- value or
null
for none
-
setMonth
public DateTime setMonth(Integer month)
Optional. Month of year. Must be from 1 to 12, or 0 if specifying a datetime without a month.
- Parameters:
month
- month or null
for none
-
getNanos
public Integer getNanos()
Optional. Fractions of seconds in nanoseconds. Must be from 0 to 999,999,999, defaults to 0.
- Returns:
- value or
null
for none
-
setNanos
public DateTime setNanos(Integer nanos)
Optional. Fractions of seconds in nanoseconds. Must be from 0 to 999,999,999, defaults to 0.
- Parameters:
nanos
- nanos or null
for none
-
getSeconds
public Integer getSeconds()
Optional. Seconds of minutes of the time. Must normally be from 0 to 59, defaults to 0. An API
may allow the value 60 if it allows leap-seconds.
- Returns:
- value or
null
for none
-
setSeconds
public DateTime setSeconds(Integer seconds)
Optional. Seconds of minutes of the time. Must normally be from 0 to 59, defaults to 0. An API
may allow the value 60 if it allows leap-seconds.
- Parameters:
seconds
- seconds or null
for none
-
getTimeZone
public TimeZone getTimeZone()
Time zone.
- Returns:
- value or
null
for none
-
setTimeZone
public DateTime setTimeZone(TimeZone timeZone)
Time zone.
- Parameters:
timeZone
- timeZone or null
for none
-
getUtcOffset
public String getUtcOffset()
UTC offset. Must be whole seconds, between -18 hours and +18 hours. For example, a UTC offset
of -4:00 would be represented as { seconds: -14400 }.
- Returns:
- value or
null
for none
-
setUtcOffset
public DateTime setUtcOffset(String utcOffset)
UTC offset. Must be whole seconds, between -18 hours and +18 hours. For example, a UTC offset
of -4:00 would be represented as { seconds: -14400 }.
- Parameters:
utcOffset
- utcOffset or null
for none
-
getYear
public Integer getYear()
Optional. Year of date. Must be from 1 to 9999, or 0 if specifying a datetime without a year.
- Returns:
- value or
null
for none
-
setYear
public DateTime setYear(Integer year)
Optional. Year of date. Must be from 1 to 9999, or 0 if specifying a datetime without a year.
- Parameters:
year
- year or null
for none
-
set
public DateTime set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public DateTime clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy