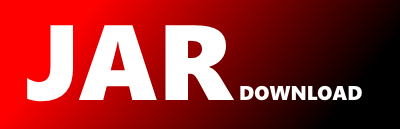
target.apidocs.com.google.api.services.content.model.Headers.html Maven / Gradle / Ivy
Headers (Content API for Shopping v2.1-rev20240609-2.0.0)
com.google.api.services.content.model
Class Headers
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.Headers
-
public final class Headers
extends com.google.api.client.json.GenericJson
A non-empty list of row or column headers for a table. Exactly one of `prices`, `weights`,
`numItems`, `postalCodeGroupNames`, or `location` must be set.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Headers()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Headers
clone()
List<LocationIdSet>
getLocations()
A list of location ID sets.
List<String>
getNumberOfItems()
A list of inclusive number of items upper bounds.
List<String>
getPostalCodeGroupNames()
A list of postal group names.
List<Price>
getPrices()
A list of inclusive order price upper bounds.
List<Weight>
getWeights()
A list of inclusive order weight upper bounds.
Headers
set(String fieldName,
Object value)
Headers
setLocations(List<LocationIdSet> locations)
A list of location ID sets.
Headers
setNumberOfItems(List<String> numberOfItems)
A list of inclusive number of items upper bounds.
Headers
setPostalCodeGroupNames(List<String> postalCodeGroupNames)
A list of postal group names.
Headers
setPrices(List<Price> prices)
A list of inclusive order price upper bounds.
Headers
setWeights(List<Weight> weights)
A list of inclusive order weight upper bounds.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getLocations
public List<LocationIdSet> getLocations()
A list of location ID sets. Must be non-empty. Can only be set if all other fields are not set.
- Returns:
- value or
null
for none
-
setLocations
public Headers setLocations(List<LocationIdSet> locations)
A list of location ID sets. Must be non-empty. Can only be set if all other fields are not set.
- Parameters:
locations
- locations or null
for none
-
getNumberOfItems
public List<String> getNumberOfItems()
A list of inclusive number of items upper bounds. The last value can be `"infinity"`. For
example `["10", "50", "infinity"]` represents the headers "<= 10 items", "<= 50 items", and ">
50 items". Must be non-empty. Can only be set if all other fields are not set.
- Returns:
- value or
null
for none
-
setNumberOfItems
public Headers setNumberOfItems(List<String> numberOfItems)
A list of inclusive number of items upper bounds. The last value can be `"infinity"`. For
example `["10", "50", "infinity"]` represents the headers "<= 10 items", "<= 50 items", and ">
50 items". Must be non-empty. Can only be set if all other fields are not set.
- Parameters:
numberOfItems
- numberOfItems or null
for none
-
getPostalCodeGroupNames
public List<String> getPostalCodeGroupNames()
A list of postal group names. The last value can be `"all other locations"`. Example: `["zone
1", "zone 2", "all other locations"]`. The referred postal code groups must match the delivery
country of the service. Must be non-empty. Can only be set if all other fields are not set.
- Returns:
- value or
null
for none
-
setPostalCodeGroupNames
public Headers setPostalCodeGroupNames(List<String> postalCodeGroupNames)
A list of postal group names. The last value can be `"all other locations"`. Example: `["zone
1", "zone 2", "all other locations"]`. The referred postal code groups must match the delivery
country of the service. Must be non-empty. Can only be set if all other fields are not set.
- Parameters:
postalCodeGroupNames
- postalCodeGroupNames or null
for none
-
getPrices
public List<Price> getPrices()
A list of inclusive order price upper bounds. The last price's value can be `"infinity"`. For
example `[{"value": "10", "currency": "USD"}, {"value": "500", "currency": "USD"}, {"value":
"infinity", "currency": "USD"}]` represents the headers "<= $10", "<= $500", and "> $500". All
prices within a service must have the same currency. Must be non-empty. Can only be set if all
other fields are not set.
- Returns:
- value or
null
for none
-
setPrices
public Headers setPrices(List<Price> prices)
A list of inclusive order price upper bounds. The last price's value can be `"infinity"`. For
example `[{"value": "10", "currency": "USD"}, {"value": "500", "currency": "USD"}, {"value":
"infinity", "currency": "USD"}]` represents the headers "<= $10", "<= $500", and "> $500". All
prices within a service must have the same currency. Must be non-empty. Can only be set if all
other fields are not set.
- Parameters:
prices
- prices or null
for none
-
getWeights
public List<Weight> getWeights()
A list of inclusive order weight upper bounds. The last weight's value can be `"infinity"`. For
example `[{"value": "10", "unit": "kg"}, {"value": "50", "unit": "kg"}, {"value": "infinity",
"unit": "kg"}]` represents the headers "<= 10kg", "<= 50kg", and "> 50kg". All weights within a
service must have the same unit. Must be non-empty. Can only be set if all other fields are not
set.
- Returns:
- value or
null
for none
-
setWeights
public Headers setWeights(List<Weight> weights)
A list of inclusive order weight upper bounds. The last weight's value can be `"infinity"`. For
example `[{"value": "10", "unit": "kg"}, {"value": "50", "unit": "kg"}, {"value": "infinity",
"unit": "kg"}]` represents the headers "<= 10kg", "<= 50kg", and "> 50kg". All weights within a
service must have the same unit. Must be non-empty. Can only be set if all other fields are not
set.
- Parameters:
weights
- weights or null
for none
-
set
public Headers set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Headers clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy