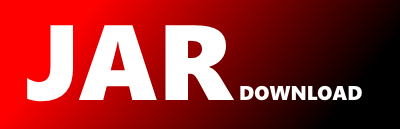
target.apidocs.com.google.api.services.content.model.OrderShipment.html Maven / Gradle / Ivy
OrderShipment (Content API for Shopping v2.1-rev20240609-2.0.0)
com.google.api.services.content.model
Class OrderShipment
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.content.model.OrderShipment
-
public final class OrderShipment
extends com.google.api.client.json.GenericJson
Model definition for OrderShipment.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
OrderShipment()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
OrderShipment
clone()
String
getCarrier()
The carrier handling the shipment.
String
getCreationDate()
Date on which the shipment has been created, in ISO 8601 format.
String
getDeliveryDate()
Date on which the shipment has been delivered, in ISO 8601 format.
String
getId()
The ID of the shipment.
List<OrderShipmentLineItemShipment>
getLineItems()
The line items that are shipped.
OrderShipmentScheduledDeliveryDetails
getScheduledDeliveryDetails()
Delivery details of the shipment if scheduling is needed.
String
getShipmentGroupId()
The shipment group ID of the shipment.
String
getStatus()
The status of the shipment.
String
getTrackingId()
The tracking ID for the shipment.
OrderShipment
set(String fieldName,
Object value)
OrderShipment
setCarrier(String carrier)
The carrier handling the shipment.
OrderShipment
setCreationDate(String creationDate)
Date on which the shipment has been created, in ISO 8601 format.
OrderShipment
setDeliveryDate(String deliveryDate)
Date on which the shipment has been delivered, in ISO 8601 format.
OrderShipment
setId(String id)
The ID of the shipment.
OrderShipment
setLineItems(List<OrderShipmentLineItemShipment> lineItems)
The line items that are shipped.
OrderShipment
setScheduledDeliveryDetails(OrderShipmentScheduledDeliveryDetails scheduledDeliveryDetails)
Delivery details of the shipment if scheduling is needed.
OrderShipment
setShipmentGroupId(String shipmentGroupId)
The shipment group ID of the shipment.
OrderShipment
setStatus(String status)
The status of the shipment.
OrderShipment
setTrackingId(String trackingId)
The tracking ID for the shipment.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCarrier
public String getCarrier()
The carrier handling the shipment. For supported carriers, Google includes the carrier name and
tracking URL in emails to customers. For select supported carriers, Google also automatically
updates the shipment status based on the provided shipment ID. *Note:* You can also use
unsupported carriers, but emails to customers won't include the carrier name or tracking URL,
and there will be no automatic order status updates. Supported carriers for "US" are: - "`ups`"
(United Parcel Service) *automatic status updates* - "`usps`" (United States Postal Service)
*automatic status updates* - "`fedex`" (FedEx) *automatic status updates * - "`dhl`" (DHL
eCommerce) *automatic status updates* (US only) - "`ontrac`" (OnTrac) *automatic status updates
* - "`dhl express`" (DHL Express) - "`deliv`" (Deliv) - "`dynamex`" (TForce) - "`lasership`"
(LaserShip) - "`mpx`" (Military Parcel Xpress) - "`uds`" (United Delivery Service) - "`efw`"
(Estes Forwarding Worldwide) - "`jd logistics`" (JD Logistics) - "`yunexpress`" (YunExpress) -
"`china post`" (China Post) - "`china ems`" (China Post Express Mail Service) - "`singapore
post`" (Singapore Post) - "`pos malaysia`" (Pos Malaysia) - "`postnl`" (PostNL) - "`ptt`" (PTT
Turkish Post) - "`eub`" (ePacket) - "`chukou1`" (Chukou1 Logistics) - "`bestex`" (Best Express)
- "`canada post`" (Canada Post) - "`purolator`" (Purolator) - "`canpar`" (Canpar) - "`india
post`" (India Post) - "`blue dart`" (Blue Dart) - "`delhivery`" (Delhivery) - "`dtdc`" (DTDC) -
"`tpc india`" (TPC India) - "`lso`" (Lone Star Overnight) - "`tww`" (Team Worldwide) -
"`deliver-it`" (Deliver-IT) - "`cdl last mile`" (CDL Last Mile) Supported carriers for FR are:
- "`la poste`" (La Poste) *automatic status updates * - "`colissimo`" (Colissimo by La Poste)
*automatic status updates* - "`ups`" (United Parcel Service) *automatic status updates * -
"`chronopost`" (Chronopost by La Poste) - "`gls`" (General Logistics Systems France) - "`dpd`"
(DPD Group by GeoPost) - "`bpost`" (Belgian Post Group) - "`colis prive`" (Colis Privé) -
"`boxtal`" (Boxtal) - "`geodis`" (GEODIS) - "`tnt`" (TNT) - "`db schenker`" (DB Schenker) -
"`aramex`" (Aramex)
- Returns:
- value or
null
for none
-
setCarrier
public OrderShipment setCarrier(String carrier)
The carrier handling the shipment. For supported carriers, Google includes the carrier name and
tracking URL in emails to customers. For select supported carriers, Google also automatically
updates the shipment status based on the provided shipment ID. *Note:* You can also use
unsupported carriers, but emails to customers won't include the carrier name or tracking URL,
and there will be no automatic order status updates. Supported carriers for "US" are: - "`ups`"
(United Parcel Service) *automatic status updates* - "`usps`" (United States Postal Service)
*automatic status updates* - "`fedex`" (FedEx) *automatic status updates * - "`dhl`" (DHL
eCommerce) *automatic status updates* (US only) - "`ontrac`" (OnTrac) *automatic status updates
* - "`dhl express`" (DHL Express) - "`deliv`" (Deliv) - "`dynamex`" (TForce) - "`lasership`"
(LaserShip) - "`mpx`" (Military Parcel Xpress) - "`uds`" (United Delivery Service) - "`efw`"
(Estes Forwarding Worldwide) - "`jd logistics`" (JD Logistics) - "`yunexpress`" (YunExpress) -
"`china post`" (China Post) - "`china ems`" (China Post Express Mail Service) - "`singapore
post`" (Singapore Post) - "`pos malaysia`" (Pos Malaysia) - "`postnl`" (PostNL) - "`ptt`" (PTT
Turkish Post) - "`eub`" (ePacket) - "`chukou1`" (Chukou1 Logistics) - "`bestex`" (Best Express)
- "`canada post`" (Canada Post) - "`purolator`" (Purolator) - "`canpar`" (Canpar) - "`india
post`" (India Post) - "`blue dart`" (Blue Dart) - "`delhivery`" (Delhivery) - "`dtdc`" (DTDC) -
"`tpc india`" (TPC India) - "`lso`" (Lone Star Overnight) - "`tww`" (Team Worldwide) -
"`deliver-it`" (Deliver-IT) - "`cdl last mile`" (CDL Last Mile) Supported carriers for FR are:
- "`la poste`" (La Poste) *automatic status updates * - "`colissimo`" (Colissimo by La Poste)
*automatic status updates* - "`ups`" (United Parcel Service) *automatic status updates * -
"`chronopost`" (Chronopost by La Poste) - "`gls`" (General Logistics Systems France) - "`dpd`"
(DPD Group by GeoPost) - "`bpost`" (Belgian Post Group) - "`colis prive`" (Colis Privé) -
"`boxtal`" (Boxtal) - "`geodis`" (GEODIS) - "`tnt`" (TNT) - "`db schenker`" (DB Schenker) -
"`aramex`" (Aramex)
- Parameters:
carrier
- carrier or null
for none
-
getCreationDate
public String getCreationDate()
Date on which the shipment has been created, in ISO 8601 format.
- Returns:
- value or
null
for none
-
setCreationDate
public OrderShipment setCreationDate(String creationDate)
Date on which the shipment has been created, in ISO 8601 format.
- Parameters:
creationDate
- creationDate or null
for none
-
getDeliveryDate
public String getDeliveryDate()
Date on which the shipment has been delivered, in ISO 8601 format. Present only if `status` is
`delivered`
- Returns:
- value or
null
for none
-
setDeliveryDate
public OrderShipment setDeliveryDate(String deliveryDate)
Date on which the shipment has been delivered, in ISO 8601 format. Present only if `status` is
`delivered`
- Parameters:
deliveryDate
- deliveryDate or null
for none
-
getId
public String getId()
The ID of the shipment.
- Returns:
- value or
null
for none
-
setId
public OrderShipment setId(String id)
The ID of the shipment.
- Parameters:
id
- id or null
for none
-
getLineItems
public List<OrderShipmentLineItemShipment> getLineItems()
The line items that are shipped.
- Returns:
- value or
null
for none
-
setLineItems
public OrderShipment setLineItems(List<OrderShipmentLineItemShipment> lineItems)
The line items that are shipped.
- Parameters:
lineItems
- lineItems or null
for none
-
getScheduledDeliveryDetails
public OrderShipmentScheduledDeliveryDetails getScheduledDeliveryDetails()
Delivery details of the shipment if scheduling is needed.
- Returns:
- value or
null
for none
-
setScheduledDeliveryDetails
public OrderShipment setScheduledDeliveryDetails(OrderShipmentScheduledDeliveryDetails scheduledDeliveryDetails)
Delivery details of the shipment if scheduling is needed.
- Parameters:
scheduledDeliveryDetails
- scheduledDeliveryDetails or null
for none
-
getShipmentGroupId
public String getShipmentGroupId()
The shipment group ID of the shipment. This is set in shiplineitems request.
- Returns:
- value or
null
for none
-
setShipmentGroupId
public OrderShipment setShipmentGroupId(String shipmentGroupId)
The shipment group ID of the shipment. This is set in shiplineitems request.
- Parameters:
shipmentGroupId
- shipmentGroupId or null
for none
-
getStatus
public String getStatus()
The status of the shipment. Acceptable values are: - "`delivered`" - "`readyForPickup`" -
"`shipped`" - "`undeliverable`"
- Returns:
- value or
null
for none
-
setStatus
public OrderShipment setStatus(String status)
The status of the shipment. Acceptable values are: - "`delivered`" - "`readyForPickup`" -
"`shipped`" - "`undeliverable`"
- Parameters:
status
- status or null
for none
-
getTrackingId
public String getTrackingId()
The tracking ID for the shipment.
- Returns:
- value or
null
for none
-
setTrackingId
public OrderShipment setTrackingId(String trackingId)
The tracking ID for the shipment.
- Parameters:
trackingId
- trackingId or null
for none
-
set
public OrderShipment set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public OrderShipment clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy