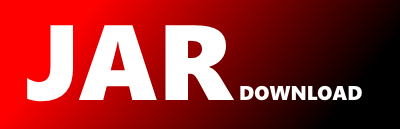
com.google.api.services.content.model.DatafeedFetchSchedule Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2020-03-07 at 08:13:03 UTC
* Modify at your own risk.
*/
package com.google.api.services.content.model;
/**
* The required fields vary based on the frequency of fetching. For a monthly fetch schedule,
* day_of_month and hour are required. For a weekly fetch schedule, weekday and hour are required.
* For a daily fetch schedule, only hour is required.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Content API for Shopping. For a detailed explanation
* see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DatafeedFetchSchedule extends com.google.api.client.json.GenericJson {
/**
* The day of the month the feed file should be fetched (1-31).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long dayOfMonth;
/**
* The URL where the feed file can be fetched. Google Merchant Center will support automatic
* scheduled uploads using the HTTP, HTTPS, FTP, or SFTP protocols, so the value will need to be a
* valid link using one of those four protocols.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fetchUrl;
/**
* The hour of the day the feed file should be fetched (0-23).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long hour;
/**
* The minute of the hour the feed file should be fetched (0-59). Read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long minuteOfHour;
/**
* An optional password for fetch_url.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String password;
/**
* Whether the scheduled fetch is paused or not.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean paused;
/**
* Time zone used for schedule. UTC by default. E.g., "America/Los_Angeles".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String timeZone;
/**
* An optional user name for fetch_url.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String username;
/**
* The day of the week the feed file should be fetched.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String weekday;
/**
* The day of the month the feed file should be fetched (1-31).
* @return value or {@code null} for none
*/
public java.lang.Long getDayOfMonth() {
return dayOfMonth;
}
/**
* The day of the month the feed file should be fetched (1-31).
* @param dayOfMonth dayOfMonth or {@code null} for none
*/
public DatafeedFetchSchedule setDayOfMonth(java.lang.Long dayOfMonth) {
this.dayOfMonth = dayOfMonth;
return this;
}
/**
* The URL where the feed file can be fetched. Google Merchant Center will support automatic
* scheduled uploads using the HTTP, HTTPS, FTP, or SFTP protocols, so the value will need to be a
* valid link using one of those four protocols.
* @return value or {@code null} for none
*/
public java.lang.String getFetchUrl() {
return fetchUrl;
}
/**
* The URL where the feed file can be fetched. Google Merchant Center will support automatic
* scheduled uploads using the HTTP, HTTPS, FTP, or SFTP protocols, so the value will need to be a
* valid link using one of those four protocols.
* @param fetchUrl fetchUrl or {@code null} for none
*/
public DatafeedFetchSchedule setFetchUrl(java.lang.String fetchUrl) {
this.fetchUrl = fetchUrl;
return this;
}
/**
* The hour of the day the feed file should be fetched (0-23).
* @return value or {@code null} for none
*/
public java.lang.Long getHour() {
return hour;
}
/**
* The hour of the day the feed file should be fetched (0-23).
* @param hour hour or {@code null} for none
*/
public DatafeedFetchSchedule setHour(java.lang.Long hour) {
this.hour = hour;
return this;
}
/**
* The minute of the hour the feed file should be fetched (0-59). Read-only.
* @return value or {@code null} for none
*/
public java.lang.Long getMinuteOfHour() {
return minuteOfHour;
}
/**
* The minute of the hour the feed file should be fetched (0-59). Read-only.
* @param minuteOfHour minuteOfHour or {@code null} for none
*/
public DatafeedFetchSchedule setMinuteOfHour(java.lang.Long minuteOfHour) {
this.minuteOfHour = minuteOfHour;
return this;
}
/**
* An optional password for fetch_url.
* @return value or {@code null} for none
*/
public java.lang.String getPassword() {
return password;
}
/**
* An optional password for fetch_url.
* @param password password or {@code null} for none
*/
public DatafeedFetchSchedule setPassword(java.lang.String password) {
this.password = password;
return this;
}
/**
* Whether the scheduled fetch is paused or not.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPaused() {
return paused;
}
/**
* Whether the scheduled fetch is paused or not.
* @param paused paused or {@code null} for none
*/
public DatafeedFetchSchedule setPaused(java.lang.Boolean paused) {
this.paused = paused;
return this;
}
/**
* Time zone used for schedule. UTC by default. E.g., "America/Los_Angeles".
* @return value or {@code null} for none
*/
public java.lang.String getTimeZone() {
return timeZone;
}
/**
* Time zone used for schedule. UTC by default. E.g., "America/Los_Angeles".
* @param timeZone timeZone or {@code null} for none
*/
public DatafeedFetchSchedule setTimeZone(java.lang.String timeZone) {
this.timeZone = timeZone;
return this;
}
/**
* An optional user name for fetch_url.
* @return value or {@code null} for none
*/
public java.lang.String getUsername() {
return username;
}
/**
* An optional user name for fetch_url.
* @param username username or {@code null} for none
*/
public DatafeedFetchSchedule setUsername(java.lang.String username) {
this.username = username;
return this;
}
/**
* The day of the week the feed file should be fetched.
* @return value or {@code null} for none
*/
public java.lang.String getWeekday() {
return weekday;
}
/**
* The day of the week the feed file should be fetched.
* @param weekday weekday or {@code null} for none
*/
public DatafeedFetchSchedule setWeekday(java.lang.String weekday) {
this.weekday = weekday;
return this;
}
@Override
public DatafeedFetchSchedule set(String fieldName, Object value) {
return (DatafeedFetchSchedule) super.set(fieldName, value);
}
@Override
public DatafeedFetchSchedule clone() {
return (DatafeedFetchSchedule) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy