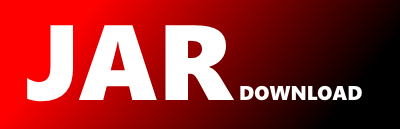
com.google.api.services.content.ShoppingContent Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-01-08 17:48:37 UTC)
* on 2016-01-18 at 16:36:08 UTC
* Modify at your own risk.
*/
package com.google.api.services.content;
/**
* Service definition for ShoppingContent (v2sandbox).
*
*
* Manage product items, inventory, and Merchant Center accounts for Google Shopping.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link ShoppingContentRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class ShoppingContent extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.21.0 of the Content API for Shopping library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "content/v2sandbox/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public ShoppingContent(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
ShoppingContent(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Orders collection.
*
* The typical use is:
*
* {@code ShoppingContent content = new ShoppingContent(...);}
* {@code ShoppingContent.Orders.List request = content.orders().list(parameters ...)}
*
*
* @return the resource collection
*/
public Orders orders() {
return new Orders();
}
/**
* The "orders" collection of methods.
*/
public class Orders {
/**
* Marks an order as acknowledged.
*
* Create a request for the method "orders.acknowledge".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Acknowledge#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersAcknowledgeRequest}
* @return the request
*/
public Acknowledge acknowledge(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersAcknowledgeRequest content) throws java.io.IOException {
Acknowledge result = new Acknowledge(merchantId, orderId, content);
initialize(result);
return result;
}
public class Acknowledge extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/acknowledge";
/**
* Marks an order as acknowledged.
*
* Create a request for the method "orders.acknowledge".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Acknowledge#execute()} method to invoke the remote operation.
* {@link
* Acknowledge#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersAcknowledgeRequest}
* @since 1.13
*/
protected Acknowledge(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersAcknowledgeRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersAcknowledgeResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Acknowledge setAlt(java.lang.String alt) {
return (Acknowledge) super.setAlt(alt);
}
@Override
public Acknowledge setFields(java.lang.String fields) {
return (Acknowledge) super.setFields(fields);
}
@Override
public Acknowledge setKey(java.lang.String key) {
return (Acknowledge) super.setKey(key);
}
@Override
public Acknowledge setOauthToken(java.lang.String oauthToken) {
return (Acknowledge) super.setOauthToken(oauthToken);
}
@Override
public Acknowledge setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Acknowledge) super.setPrettyPrint(prettyPrint);
}
@Override
public Acknowledge setQuotaUser(java.lang.String quotaUser) {
return (Acknowledge) super.setQuotaUser(quotaUser);
}
@Override
public Acknowledge setUserIp(java.lang.String userIp) {
return (Acknowledge) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Acknowledge setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Acknowledge setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Acknowledge set(String parameterName, Object value) {
return (Acknowledge) super.set(parameterName, value);
}
}
/**
* Sandbox only. Moves a test order from state "inProgress" to state "pendingShipment".
*
* Create a request for the method "orders.advancetestorder".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Advancetestorder#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the test order to modify.
* @return the request
*/
public Advancetestorder advancetestorder(java.math.BigInteger merchantId, java.lang.String orderId) throws java.io.IOException {
Advancetestorder result = new Advancetestorder(merchantId, orderId);
initialize(result);
return result;
}
public class Advancetestorder extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/testorders/{orderId}/advance";
/**
* Sandbox only. Moves a test order from state "inProgress" to state "pendingShipment".
*
* Create a request for the method "orders.advancetestorder".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Advancetestorder#execute()} method to invoke the remote operation.
* {@link Advancetestorder#initialize(com.google.api.client.googleapis.services.AbstractGoogle
* ClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the test order to modify.
* @since 1.13
*/
protected Advancetestorder(java.math.BigInteger merchantId, java.lang.String orderId) {
super(ShoppingContent.this, "POST", REST_PATH, null, com.google.api.services.content.model.OrdersAdvanceTestOrderResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Advancetestorder setAlt(java.lang.String alt) {
return (Advancetestorder) super.setAlt(alt);
}
@Override
public Advancetestorder setFields(java.lang.String fields) {
return (Advancetestorder) super.setFields(fields);
}
@Override
public Advancetestorder setKey(java.lang.String key) {
return (Advancetestorder) super.setKey(key);
}
@Override
public Advancetestorder setOauthToken(java.lang.String oauthToken) {
return (Advancetestorder) super.setOauthToken(oauthToken);
}
@Override
public Advancetestorder setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Advancetestorder) super.setPrettyPrint(prettyPrint);
}
@Override
public Advancetestorder setQuotaUser(java.lang.String quotaUser) {
return (Advancetestorder) super.setQuotaUser(quotaUser);
}
@Override
public Advancetestorder setUserIp(java.lang.String userIp) {
return (Advancetestorder) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Advancetestorder setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the test order to modify. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the test order to modify.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the test order to modify. */
public Advancetestorder setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Advancetestorder set(String parameterName, Object value) {
return (Advancetestorder) super.set(parameterName, value);
}
}
/**
* Cancels all line items in an order.
*
* Create a request for the method "orders.cancel".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order to cancel.
* @param content the {@link com.google.api.services.content.model.OrdersCancelRequest}
* @return the request
*/
public Cancel cancel(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelRequest content) throws java.io.IOException {
Cancel result = new Cancel(merchantId, orderId, content);
initialize(result);
return result;
}
public class Cancel extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/cancel";
/**
* Cancels all line items in an order.
*
* Create a request for the method "orders.cancel".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order to cancel.
* @param content the {@link com.google.api.services.content.model.OrdersCancelRequest}
* @since 1.13
*/
protected Cancel(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCancelResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUserIp(java.lang.String userIp) {
return (Cancel) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Cancel setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order to cancel. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order to cancel.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order to cancel. */
public Cancel setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Cancels a line item.
*
* Create a request for the method "orders.cancellineitem".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Cancellineitem#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersCancelLineItemRequest}
* @return the request
*/
public Cancellineitem cancellineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelLineItemRequest content) throws java.io.IOException {
Cancellineitem result = new Cancellineitem(merchantId, orderId, content);
initialize(result);
return result;
}
public class Cancellineitem extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/cancelLineItem";
/**
* Cancels a line item.
*
* Create a request for the method "orders.cancellineitem".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Cancellineitem#execute()} method to invoke the remote operation.
* {@link Cancellineitem#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersCancelLineItemRequest}
* @since 1.13
*/
protected Cancellineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersCancelLineItemRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCancelLineItemResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Cancellineitem setAlt(java.lang.String alt) {
return (Cancellineitem) super.setAlt(alt);
}
@Override
public Cancellineitem setFields(java.lang.String fields) {
return (Cancellineitem) super.setFields(fields);
}
@Override
public Cancellineitem setKey(java.lang.String key) {
return (Cancellineitem) super.setKey(key);
}
@Override
public Cancellineitem setOauthToken(java.lang.String oauthToken) {
return (Cancellineitem) super.setOauthToken(oauthToken);
}
@Override
public Cancellineitem setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancellineitem) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancellineitem setQuotaUser(java.lang.String quotaUser) {
return (Cancellineitem) super.setQuotaUser(quotaUser);
}
@Override
public Cancellineitem setUserIp(java.lang.String userIp) {
return (Cancellineitem) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Cancellineitem setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Cancellineitem setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Cancellineitem set(String parameterName, Object value) {
return (Cancellineitem) super.set(parameterName, value);
}
}
/**
* Sandbox only. Creates a test order.
*
* Create a request for the method "orders.createtestorder".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Createtestorder#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param content the {@link com.google.api.services.content.model.OrdersCreateTestOrderRequest}
* @return the request
*/
public Createtestorder createtestorder(java.math.BigInteger merchantId, com.google.api.services.content.model.OrdersCreateTestOrderRequest content) throws java.io.IOException {
Createtestorder result = new Createtestorder(merchantId, content);
initialize(result);
return result;
}
public class Createtestorder extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/testorders";
/**
* Sandbox only. Creates a test order.
*
* Create a request for the method "orders.createtestorder".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Createtestorder#execute()} method to invoke the remote operation.
* {@link Createtestorder#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the managing account.
* @param content the {@link com.google.api.services.content.model.OrdersCreateTestOrderRequest}
* @since 1.13
*/
protected Createtestorder(java.math.BigInteger merchantId, com.google.api.services.content.model.OrdersCreateTestOrderRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCreateTestOrderResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public Createtestorder setAlt(java.lang.String alt) {
return (Createtestorder) super.setAlt(alt);
}
@Override
public Createtestorder setFields(java.lang.String fields) {
return (Createtestorder) super.setFields(fields);
}
@Override
public Createtestorder setKey(java.lang.String key) {
return (Createtestorder) super.setKey(key);
}
@Override
public Createtestorder setOauthToken(java.lang.String oauthToken) {
return (Createtestorder) super.setOauthToken(oauthToken);
}
@Override
public Createtestorder setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Createtestorder) super.setPrettyPrint(prettyPrint);
}
@Override
public Createtestorder setQuotaUser(java.lang.String quotaUser) {
return (Createtestorder) super.setQuotaUser(quotaUser);
}
@Override
public Createtestorder setUserIp(java.lang.String userIp) {
return (Createtestorder) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Createtestorder setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
@Override
public Createtestorder set(String parameterName, Object value) {
return (Createtestorder) super.set(parameterName, value);
}
}
/**
* Retrieves or modifies multiple orders in a single request.
*
* Create a request for the method "orders.custombatch".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.content.model.OrdersCustomBatchRequest}
* @return the request
*/
public Custombatch custombatch(com.google.api.services.content.model.OrdersCustomBatchRequest content) throws java.io.IOException {
Custombatch result = new Custombatch(content);
initialize(result);
return result;
}
public class Custombatch extends ShoppingContentRequest {
private static final String REST_PATH = "orders/batch";
/**
* Retrieves or modifies multiple orders in a single request.
*
* Create a request for the method "orders.custombatch".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Custombatch#execute()} method to invoke the remote operation.
* {@link
* Custombatch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.content.model.OrdersCustomBatchRequest}
* @since 1.13
*/
protected Custombatch(com.google.api.services.content.model.OrdersCustomBatchRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersCustomBatchResponse.class);
}
@Override
public Custombatch setAlt(java.lang.String alt) {
return (Custombatch) super.setAlt(alt);
}
@Override
public Custombatch setFields(java.lang.String fields) {
return (Custombatch) super.setFields(fields);
}
@Override
public Custombatch setKey(java.lang.String key) {
return (Custombatch) super.setKey(key);
}
@Override
public Custombatch setOauthToken(java.lang.String oauthToken) {
return (Custombatch) super.setOauthToken(oauthToken);
}
@Override
public Custombatch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Custombatch) super.setPrettyPrint(prettyPrint);
}
@Override
public Custombatch setQuotaUser(java.lang.String quotaUser) {
return (Custombatch) super.setQuotaUser(quotaUser);
}
@Override
public Custombatch setUserIp(java.lang.String userIp) {
return (Custombatch) super.setUserIp(userIp);
}
@Override
public Custombatch set(String parameterName, Object value) {
return (Custombatch) super.set(parameterName, value);
}
}
/**
* Retrieves an order from your Merchant Center account.
*
* Create a request for the method "orders.get".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @return the request
*/
public Get get(java.math.BigInteger merchantId, java.lang.String orderId) throws java.io.IOException {
Get result = new Get(merchantId, orderId);
initialize(result);
return result;
}
public class Get extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}";
/**
* Retrieves an order from your Merchant Center account.
*
* Create a request for the method "orders.get".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @since 1.13
*/
protected Get(java.math.BigInteger merchantId, java.lang.String orderId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.Order.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Get setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Get setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves an order using merchant order id.
*
* Create a request for the method "orders.getbymerchantorderid".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Getbymerchantorderid#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the managing account.
* @param merchantOrderId The merchant order id to be looked for.
* @return the request
*/
public Getbymerchantorderid getbymerchantorderid(java.math.BigInteger merchantId, java.lang.String merchantOrderId) throws java.io.IOException {
Getbymerchantorderid result = new Getbymerchantorderid(merchantId, merchantOrderId);
initialize(result);
return result;
}
public class Getbymerchantorderid extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/ordersbymerchantid/{merchantOrderId}";
/**
* Retrieves an order using merchant order id.
*
* Create a request for the method "orders.getbymerchantorderid".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Getbymerchantorderid#execute()} method to invoke the remote
* operation. {@link Getbymerchantorderid#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @param merchantOrderId The merchant order id to be looked for.
* @since 1.13
*/
protected Getbymerchantorderid(java.math.BigInteger merchantId, java.lang.String merchantOrderId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.OrdersGetByMerchantOrderIdResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.merchantOrderId = com.google.api.client.util.Preconditions.checkNotNull(merchantOrderId, "Required parameter merchantOrderId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Getbymerchantorderid setAlt(java.lang.String alt) {
return (Getbymerchantorderid) super.setAlt(alt);
}
@Override
public Getbymerchantorderid setFields(java.lang.String fields) {
return (Getbymerchantorderid) super.setFields(fields);
}
@Override
public Getbymerchantorderid setKey(java.lang.String key) {
return (Getbymerchantorderid) super.setKey(key);
}
@Override
public Getbymerchantorderid setOauthToken(java.lang.String oauthToken) {
return (Getbymerchantorderid) super.setOauthToken(oauthToken);
}
@Override
public Getbymerchantorderid setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Getbymerchantorderid) super.setPrettyPrint(prettyPrint);
}
@Override
public Getbymerchantorderid setQuotaUser(java.lang.String quotaUser) {
return (Getbymerchantorderid) super.setQuotaUser(quotaUser);
}
@Override
public Getbymerchantorderid setUserIp(java.lang.String userIp) {
return (Getbymerchantorderid) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Getbymerchantorderid setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The merchant order id to be looked for. */
@com.google.api.client.util.Key
private java.lang.String merchantOrderId;
/** The merchant order id to be looked for.
*/
public java.lang.String getMerchantOrderId() {
return merchantOrderId;
}
/** The merchant order id to be looked for. */
public Getbymerchantorderid setMerchantOrderId(java.lang.String merchantOrderId) {
this.merchantOrderId = merchantOrderId;
return this;
}
@Override
public Getbymerchantorderid set(String parameterName, Object value) {
return (Getbymerchantorderid) super.set(parameterName, value);
}
}
/**
* Sandbox only. Retrieves an order template that can be used to quickly create a new order in
* sandbox.
*
* Create a request for the method "orders.gettestordertemplate".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Gettestordertemplate#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the managing account.
* @param templateName The name of the template to retrieve.
* @return the request
*/
public Gettestordertemplate gettestordertemplate(java.math.BigInteger merchantId, java.lang.String templateName) throws java.io.IOException {
Gettestordertemplate result = new Gettestordertemplate(merchantId, templateName);
initialize(result);
return result;
}
public class Gettestordertemplate extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/testordertemplates/{templateName}";
/**
* Sandbox only. Retrieves an order template that can be used to quickly create a new order in
* sandbox.
*
* Create a request for the method "orders.gettestordertemplate".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Gettestordertemplate#execute()} method to invoke the remote
* operation. {@link Gettestordertemplate#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @param templateName The name of the template to retrieve.
* @since 1.13
*/
protected Gettestordertemplate(java.math.BigInteger merchantId, java.lang.String templateName) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.OrdersGetTestOrderTemplateResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.templateName = com.google.api.client.util.Preconditions.checkNotNull(templateName, "Required parameter templateName must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Gettestordertemplate setAlt(java.lang.String alt) {
return (Gettestordertemplate) super.setAlt(alt);
}
@Override
public Gettestordertemplate setFields(java.lang.String fields) {
return (Gettestordertemplate) super.setFields(fields);
}
@Override
public Gettestordertemplate setKey(java.lang.String key) {
return (Gettestordertemplate) super.setKey(key);
}
@Override
public Gettestordertemplate setOauthToken(java.lang.String oauthToken) {
return (Gettestordertemplate) super.setOauthToken(oauthToken);
}
@Override
public Gettestordertemplate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Gettestordertemplate) super.setPrettyPrint(prettyPrint);
}
@Override
public Gettestordertemplate setQuotaUser(java.lang.String quotaUser) {
return (Gettestordertemplate) super.setQuotaUser(quotaUser);
}
@Override
public Gettestordertemplate setUserIp(java.lang.String userIp) {
return (Gettestordertemplate) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Gettestordertemplate setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The name of the template to retrieve. */
@com.google.api.client.util.Key
private java.lang.String templateName;
/** The name of the template to retrieve.
*/
public java.lang.String getTemplateName() {
return templateName;
}
/** The name of the template to retrieve. */
public Gettestordertemplate setTemplateName(java.lang.String templateName) {
this.templateName = templateName;
return this;
}
@Override
public Gettestordertemplate set(String parameterName, Object value) {
return (Gettestordertemplate) super.set(parameterName, value);
}
}
/**
* Lists the orders in your Merchant Center account.
*
* Create a request for the method "orders.list".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @return the request
*/
public List list(java.math.BigInteger merchantId) throws java.io.IOException {
List result = new List(merchantId);
initialize(result);
return result;
}
public class List extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders";
/**
* Lists the orders in your Merchant Center account.
*
* Create a request for the method "orders.list".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @since 1.13
*/
protected List(java.math.BigInteger merchantId) {
super(ShoppingContent.this, "GET", REST_PATH, null, com.google.api.services.content.model.OrdersListResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public List setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/**
* The ordering of the returned list. The only supported value are placedDate desc and
* placedDate asc for now, which returns orders sorted by placement date. "placedDate desc"
* stands for listing orders by placement date, from oldest to most recent. "placedDate asc"
* stands for listing orders by placement date, from most recent to oldest. In future releases
* we'll support other sorting criteria.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** The ordering of the returned list. The only supported value are placedDate desc and placedDate asc
for now, which returns orders sorted by placement date. "placedDate desc" stands for listing orders
by placement date, from oldest to most recent. "placedDate asc" stands for listing orders by
placement date, from most recent to oldest. In future releases we'll support other sorting
criteria.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* The ordering of the returned list. The only supported value are placedDate desc and
* placedDate asc for now, which returns orders sorted by placement date. "placedDate desc"
* stands for listing orders by placement date, from oldest to most recent. "placedDate asc"
* stands for listing orders by placement date, from most recent to oldest. In future releases
* we'll support other sorting criteria.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/** Obtains orders placed before this date (exclusively), in ISO 8601 format. */
@com.google.api.client.util.Key
private java.lang.String placedDateEnd;
/** Obtains orders placed before this date (exclusively), in ISO 8601 format.
*/
public java.lang.String getPlacedDateEnd() {
return placedDateEnd;
}
/** Obtains orders placed before this date (exclusively), in ISO 8601 format. */
public List setPlacedDateEnd(java.lang.String placedDateEnd) {
this.placedDateEnd = placedDateEnd;
return this;
}
/**
* Obtains orders that match the acknowledgement status. When set to true, obtains orders that
* have been acknowledged. When false, obtains orders that have not been acknowledged. We
* recommend using this filter set to false, in conjunction with the acknowledge call, such
* that only un-acknowledged orders are returned.
*/
@com.google.api.client.util.Key
private java.lang.Boolean acknowledged;
/** Obtains orders that match the acknowledgement status. When set to true, obtains orders that have
been acknowledged. When false, obtains orders that have not been acknowledged. We recommend using
this filter set to false, in conjunction with the acknowledge call, such that only un-acknowledged
orders are returned.
*/
public java.lang.Boolean getAcknowledged() {
return acknowledged;
}
/**
* Obtains orders that match the acknowledgement status. When set to true, obtains orders that
* have been acknowledged. When false, obtains orders that have not been acknowledged. We
* recommend using this filter set to false, in conjunction with the acknowledge call, such
* that only un-acknowledged orders are returned.
*/
public List setAcknowledged(java.lang.Boolean acknowledged) {
this.acknowledged = acknowledged;
return this;
}
/**
* The maximum number of orders to return in the response, used for paging. The default value
* is 25 orders per page, and the maximum allowed value is 250 orders per page. Known issue:
* All List calls will return all Orders without limit regardless of the value of this field.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of orders to return in the response, used for paging. The default value is 25
orders per page, and the maximum allowed value is 250 orders per page. Known issue: All List calls
will return all Orders without limit regardless of the value of this field.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of orders to return in the response, used for paging. The default value
* is 25 orders per page, and the maximum allowed value is 250 orders per page. Known issue:
* All List calls will return all Orders without limit regardless of the value of this field.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Obtains orders placed after this date (inclusively), in ISO 8601 format. */
@com.google.api.client.util.Key
private java.lang.String placedDateStart;
/** Obtains orders placed after this date (inclusively), in ISO 8601 format.
*/
public java.lang.String getPlacedDateStart() {
return placedDateStart;
}
/** Obtains orders placed after this date (inclusively), in ISO 8601 format. */
public List setPlacedDateStart(java.lang.String placedDateStart) {
this.placedDateStart = placedDateStart;
return this;
}
/**
* Obtains orders that match any of the specified statuses. Multiple values can be specified
* with comma separation. Additionally, please note that active is a shortcut for
* pendingShipment and partiallyShipped, and completed is a shortcut for shipped ,
* partiallyDelivered, delivered, partiallyReturned, returned, and canceled.
*/
@com.google.api.client.util.Key
private java.util.List statuses;
/** Obtains orders that match any of the specified statuses. Multiple values can be specified with
comma separation. Additionally, please note that active is a shortcut for pendingShipment and
partiallyShipped, and completed is a shortcut for shipped , partiallyDelivered, delivered,
partiallyReturned, returned, and canceled.
*/
public java.util.List getStatuses() {
return statuses;
}
/**
* Obtains orders that match any of the specified statuses. Multiple values can be specified
* with comma separation. Additionally, please note that active is a shortcut for
* pendingShipment and partiallyShipped, and completed is a shortcut for shipped ,
* partiallyDelivered, delivered, partiallyReturned, returned, and canceled.
*/
public List setStatuses(java.util.List statuses) {
this.statuses = statuses;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Refund a portion of the order, up to the full amount paid.
*
* Create a request for the method "orders.refund".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Refund#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order to refund.
* @param content the {@link com.google.api.services.content.model.OrdersRefundRequest}
* @return the request
*/
public Refund refund(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersRefundRequest content) throws java.io.IOException {
Refund result = new Refund(merchantId, orderId, content);
initialize(result);
return result;
}
public class Refund extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/refund";
/**
* Refund a portion of the order, up to the full amount paid.
*
* Create a request for the method "orders.refund".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Refund#execute()} method to invoke the remote operation. {@link
* Refund#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order to refund.
* @param content the {@link com.google.api.services.content.model.OrdersRefundRequest}
* @since 1.13
*/
protected Refund(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersRefundRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersRefundResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Refund setAlt(java.lang.String alt) {
return (Refund) super.setAlt(alt);
}
@Override
public Refund setFields(java.lang.String fields) {
return (Refund) super.setFields(fields);
}
@Override
public Refund setKey(java.lang.String key) {
return (Refund) super.setKey(key);
}
@Override
public Refund setOauthToken(java.lang.String oauthToken) {
return (Refund) super.setOauthToken(oauthToken);
}
@Override
public Refund setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Refund) super.setPrettyPrint(prettyPrint);
}
@Override
public Refund setQuotaUser(java.lang.String quotaUser) {
return (Refund) super.setQuotaUser(quotaUser);
}
@Override
public Refund setUserIp(java.lang.String userIp) {
return (Refund) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Refund setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order to refund. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order to refund.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order to refund. */
public Refund setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Refund set(String parameterName, Object value) {
return (Refund) super.set(parameterName, value);
}
}
/**
* Returns a line item.
*
* Create a request for the method "orders.returnlineitem".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Returnlineitem#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersReturnLineItemRequest}
* @return the request
*/
public Returnlineitem returnlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersReturnLineItemRequest content) throws java.io.IOException {
Returnlineitem result = new Returnlineitem(merchantId, orderId, content);
initialize(result);
return result;
}
public class Returnlineitem extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/returnLineItem";
/**
* Returns a line item.
*
* Create a request for the method "orders.returnlineitem".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Returnlineitem#execute()} method to invoke the remote operation.
* {@link Returnlineitem#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersReturnLineItemRequest}
* @since 1.13
*/
protected Returnlineitem(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersReturnLineItemRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersReturnLineItemResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Returnlineitem setAlt(java.lang.String alt) {
return (Returnlineitem) super.setAlt(alt);
}
@Override
public Returnlineitem setFields(java.lang.String fields) {
return (Returnlineitem) super.setFields(fields);
}
@Override
public Returnlineitem setKey(java.lang.String key) {
return (Returnlineitem) super.setKey(key);
}
@Override
public Returnlineitem setOauthToken(java.lang.String oauthToken) {
return (Returnlineitem) super.setOauthToken(oauthToken);
}
@Override
public Returnlineitem setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Returnlineitem) super.setPrettyPrint(prettyPrint);
}
@Override
public Returnlineitem setQuotaUser(java.lang.String quotaUser) {
return (Returnlineitem) super.setQuotaUser(quotaUser);
}
@Override
public Returnlineitem setUserIp(java.lang.String userIp) {
return (Returnlineitem) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Returnlineitem setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Returnlineitem setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Returnlineitem set(String parameterName, Object value) {
return (Returnlineitem) super.set(parameterName, value);
}
}
/**
* Marks line item(s) as shipped.
*
* Create a request for the method "orders.shiplineitems".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Shiplineitems#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersShipLineItemsRequest}
* @return the request
*/
public Shiplineitems shiplineitems(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersShipLineItemsRequest content) throws java.io.IOException {
Shiplineitems result = new Shiplineitems(merchantId, orderId, content);
initialize(result);
return result;
}
public class Shiplineitems extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/shipLineItems";
/**
* Marks line item(s) as shipped.
*
* Create a request for the method "orders.shiplineitems".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Shiplineitems#execute()} method to invoke the remote operation.
* {@link Shiplineitems#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientR
* equest)} must be called to initialize this instance immediately after invoking the constructor.
*
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersShipLineItemsRequest}
* @since 1.13
*/
protected Shiplineitems(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersShipLineItemsRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersShipLineItemsResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Shiplineitems setAlt(java.lang.String alt) {
return (Shiplineitems) super.setAlt(alt);
}
@Override
public Shiplineitems setFields(java.lang.String fields) {
return (Shiplineitems) super.setFields(fields);
}
@Override
public Shiplineitems setKey(java.lang.String key) {
return (Shiplineitems) super.setKey(key);
}
@Override
public Shiplineitems setOauthToken(java.lang.String oauthToken) {
return (Shiplineitems) super.setOauthToken(oauthToken);
}
@Override
public Shiplineitems setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Shiplineitems) super.setPrettyPrint(prettyPrint);
}
@Override
public Shiplineitems setQuotaUser(java.lang.String quotaUser) {
return (Shiplineitems) super.setQuotaUser(quotaUser);
}
@Override
public Shiplineitems setUserIp(java.lang.String userIp) {
return (Shiplineitems) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Shiplineitems setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Shiplineitems setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Shiplineitems set(String parameterName, Object value) {
return (Shiplineitems) super.set(parameterName, value);
}
}
/**
* Updates the merchant order ID for a given order.
*
* Create a request for the method "orders.updatemerchantorderid".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Updatemerchantorderid#execute()} method to invoke the remote
* operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest}
* @return the request
*/
public Updatemerchantorderid updatemerchantorderid(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest content) throws java.io.IOException {
Updatemerchantorderid result = new Updatemerchantorderid(merchantId, orderId, content);
initialize(result);
return result;
}
public class Updatemerchantorderid extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/updateMerchantOrderId";
/**
* Updates the merchant order ID for a given order.
*
* Create a request for the method "orders.updatemerchantorderid".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Updatemerchantorderid#execute()} method to invoke the remote
* operation. {@link Updatemerchantorderid#initialize(com.google.api.client.googleapis.service
* s.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest}
* @since 1.13
*/
protected Updatemerchantorderid(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateMerchantOrderIdRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersUpdateMerchantOrderIdResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Updatemerchantorderid setAlt(java.lang.String alt) {
return (Updatemerchantorderid) super.setAlt(alt);
}
@Override
public Updatemerchantorderid setFields(java.lang.String fields) {
return (Updatemerchantorderid) super.setFields(fields);
}
@Override
public Updatemerchantorderid setKey(java.lang.String key) {
return (Updatemerchantorderid) super.setKey(key);
}
@Override
public Updatemerchantorderid setOauthToken(java.lang.String oauthToken) {
return (Updatemerchantorderid) super.setOauthToken(oauthToken);
}
@Override
public Updatemerchantorderid setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Updatemerchantorderid) super.setPrettyPrint(prettyPrint);
}
@Override
public Updatemerchantorderid setQuotaUser(java.lang.String quotaUser) {
return (Updatemerchantorderid) super.setQuotaUser(quotaUser);
}
@Override
public Updatemerchantorderid setUserIp(java.lang.String userIp) {
return (Updatemerchantorderid) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Updatemerchantorderid setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Updatemerchantorderid setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Updatemerchantorderid set(String parameterName, Object value) {
return (Updatemerchantorderid) super.set(parameterName, value);
}
}
/**
* Updates a shipment's status, carrier, and/or tracking ID.
*
* Create a request for the method "orders.updateshipment".
*
* This request holds the parameters needed by the content server. After setting any optional
* parameters, call the {@link Updateshipment#execute()} method to invoke the remote operation.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateShipmentRequest}
* @return the request
*/
public Updateshipment updateshipment(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateShipmentRequest content) throws java.io.IOException {
Updateshipment result = new Updateshipment(merchantId, orderId, content);
initialize(result);
return result;
}
public class Updateshipment extends ShoppingContentRequest {
private static final String REST_PATH = "{merchantId}/orders/{orderId}/updateShipment";
/**
* Updates a shipment's status, carrier, and/or tracking ID.
*
* Create a request for the method "orders.updateshipment".
*
* This request holds the parameters needed by the the content server. After setting any optional
* parameters, call the {@link Updateshipment#execute()} method to invoke the remote operation.
* {@link Updateshipment#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param merchantId The ID of the managing account.
* @param orderId The ID of the order.
* @param content the {@link com.google.api.services.content.model.OrdersUpdateShipmentRequest}
* @since 1.13
*/
protected Updateshipment(java.math.BigInteger merchantId, java.lang.String orderId, com.google.api.services.content.model.OrdersUpdateShipmentRequest content) {
super(ShoppingContent.this, "POST", REST_PATH, content, com.google.api.services.content.model.OrdersUpdateShipmentResponse.class);
this.merchantId = com.google.api.client.util.Preconditions.checkNotNull(merchantId, "Required parameter merchantId must be specified.");
this.orderId = com.google.api.client.util.Preconditions.checkNotNull(orderId, "Required parameter orderId must be specified.");
}
@Override
public Updateshipment setAlt(java.lang.String alt) {
return (Updateshipment) super.setAlt(alt);
}
@Override
public Updateshipment setFields(java.lang.String fields) {
return (Updateshipment) super.setFields(fields);
}
@Override
public Updateshipment setKey(java.lang.String key) {
return (Updateshipment) super.setKey(key);
}
@Override
public Updateshipment setOauthToken(java.lang.String oauthToken) {
return (Updateshipment) super.setOauthToken(oauthToken);
}
@Override
public Updateshipment setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Updateshipment) super.setPrettyPrint(prettyPrint);
}
@Override
public Updateshipment setQuotaUser(java.lang.String quotaUser) {
return (Updateshipment) super.setQuotaUser(quotaUser);
}
@Override
public Updateshipment setUserIp(java.lang.String userIp) {
return (Updateshipment) super.setUserIp(userIp);
}
/** The ID of the managing account. */
@com.google.api.client.util.Key
private java.math.BigInteger merchantId;
/** The ID of the managing account.
*/
public java.math.BigInteger getMerchantId() {
return merchantId;
}
/** The ID of the managing account. */
public Updateshipment setMerchantId(java.math.BigInteger merchantId) {
this.merchantId = merchantId;
return this;
}
/** The ID of the order. */
@com.google.api.client.util.Key
private java.lang.String orderId;
/** The ID of the order.
*/
public java.lang.String getOrderId() {
return orderId;
}
/** The ID of the order. */
public Updateshipment setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
@Override
public Updateshipment set(String parameterName, Object value) {
return (Updateshipment) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link ShoppingContent}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link ShoppingContent}. */
@Override
public ShoppingContent build() {
return new ShoppingContent(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link ShoppingContentRequestInitializer}.
*
* @since 1.12
*/
public Builder setShoppingContentRequestInitializer(
ShoppingContentRequestInitializer shoppingcontentRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(shoppingcontentRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}