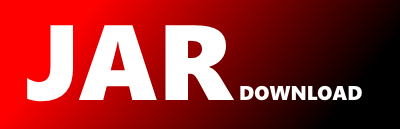
com.google.api.services.coordinate.Coordinate Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2016-01-08 17:48:37 UTC)
* on 2016-02-11 at 11:42:59 UTC
* Modify at your own risk.
*/
package com.google.api.services.coordinate;
/**
* Service definition for Coordinate (v1).
*
*
* Lets you view and manage jobs in a Coordinate team.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CoordinateRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Coordinate extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.20.0 of the Google Maps Coordinate API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "coordinate/v1/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Coordinate(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Coordinate(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the CustomFieldDef collection.
*
* The typical use is:
*
* {@code Coordinate coordinate = new Coordinate(...);}
* {@code Coordinate.CustomFieldDef.List request = coordinate.customFieldDef().list(parameters ...)}
*
*
* @return the resource collection
*/
public CustomFieldDef customFieldDef() {
return new CustomFieldDef();
}
/**
* The "customFieldDef" collection of methods.
*/
public class CustomFieldDef {
/**
* Retrieves a list of custom field definitions for a team.
*
* Create a request for the method "customFieldDef.list".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @return the request
*/
public List list(java.lang.String teamId) throws java.io.IOException {
List result = new List(teamId);
initialize(result);
return result;
}
public class List extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/custom_fields";
/**
* Retrieves a list of custom field definitions for a team.
*
* Create a request for the method "customFieldDef.list".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @since 1.13
*/
protected List(java.lang.String teamId) {
super(Coordinate.this, "GET", REST_PATH, null, com.google.api.services.coordinate.model.CustomFieldDefListResponse.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public List setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Jobs collection.
*
* The typical use is:
*
* {@code Coordinate coordinate = new Coordinate(...);}
* {@code Coordinate.Jobs.List request = coordinate.jobs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Jobs jobs() {
return new Jobs();
}
/**
* The "jobs" collection of methods.
*/
public class Jobs {
/**
* Retrieves a job, including all the changes made to the job.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param jobId Job number
* @return the request
*/
public Get get(java.lang.String teamId, java.math.BigInteger jobId) throws java.io.IOException {
Get result = new Get(teamId, jobId);
initialize(result);
return result;
}
public class Get extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs/{jobId}";
/**
* Retrieves a job, including all the changes made to the job.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param jobId Job number
* @since 1.13
*/
protected Get(java.lang.String teamId, java.math.BigInteger jobId) {
super(Coordinate.this, "GET", REST_PATH, null, com.google.api.services.coordinate.model.Job.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public Get setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Job number */
@com.google.api.client.util.Key
private java.math.BigInteger jobId;
/** Job number
*/
public java.math.BigInteger getJobId() {
return jobId;
}
/** Job number */
public Get setJobId(java.math.BigInteger jobId) {
this.jobId = jobId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new job. Only the state field of the job should be set.
*
* Create a request for the method "jobs.insert".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param address Job address as newline (Unix) separated string
* @param lat The latitude coordinate of this job's location.
* @param lng The longitude coordinate of this job's location.
* @param title Job title
* @param content the {@link com.google.api.services.coordinate.model.Job}
* @return the request
*/
public Insert insert(java.lang.String teamId, java.lang.String address, java.lang.Double lat, java.lang.Double lng, java.lang.String title, com.google.api.services.coordinate.model.Job content) throws java.io.IOException {
Insert result = new Insert(teamId, address, lat, lng, title, content);
initialize(result);
return result;
}
public class Insert extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs";
/**
* Inserts a new job. Only the state field of the job should be set.
*
* Create a request for the method "jobs.insert".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param address Job address as newline (Unix) separated string
* @param lat The latitude coordinate of this job's location.
* @param lng The longitude coordinate of this job's location.
* @param title Job title
* @param content the {@link com.google.api.services.coordinate.model.Job}
* @since 1.13
*/
protected Insert(java.lang.String teamId, java.lang.String address, java.lang.Double lat, java.lang.Double lng, java.lang.String title, com.google.api.services.coordinate.model.Job content) {
super(Coordinate.this, "POST", REST_PATH, content, com.google.api.services.coordinate.model.Job.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.address = com.google.api.client.util.Preconditions.checkNotNull(address, "Required parameter address must be specified.");
this.lat = com.google.api.client.util.Preconditions.checkNotNull(lat, "Required parameter lat must be specified.");
this.lng = com.google.api.client.util.Preconditions.checkNotNull(lng, "Required parameter lng must be specified.");
this.title = com.google.api.client.util.Preconditions.checkNotNull(title, "Required parameter title must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public Insert setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Job address as newline (Unix) separated string */
@com.google.api.client.util.Key
private java.lang.String address;
/** Job address as newline (Unix) separated string
*/
public java.lang.String getAddress() {
return address;
}
/** Job address as newline (Unix) separated string */
public Insert setAddress(java.lang.String address) {
this.address = address;
return this;
}
/** The latitude coordinate of this job's location. */
@com.google.api.client.util.Key
private java.lang.Double lat;
/** The latitude coordinate of this job's location.
*/
public java.lang.Double getLat() {
return lat;
}
/** The latitude coordinate of this job's location. */
public Insert setLat(java.lang.Double lat) {
this.lat = lat;
return this;
}
/** The longitude coordinate of this job's location. */
@com.google.api.client.util.Key
private java.lang.Double lng;
/** The longitude coordinate of this job's location.
*/
public java.lang.Double getLng() {
return lng;
}
/** The longitude coordinate of this job's location. */
public Insert setLng(java.lang.Double lng) {
this.lng = lng;
return this;
}
/** Job title */
@com.google.api.client.util.Key
private java.lang.String title;
/** Job title
*/
public java.lang.String getTitle() {
return title;
}
/** Job title */
public Insert setTitle(java.lang.String title) {
this.title = title;
return this;
}
/** Customer name */
@com.google.api.client.util.Key
private java.lang.String customerName;
/** Customer name
*/
public java.lang.String getCustomerName() {
return customerName;
}
/** Customer name */
public Insert setCustomerName(java.lang.String customerName) {
this.customerName = customerName;
return this;
}
/** Job note as newline (Unix) separated string */
@com.google.api.client.util.Key
private java.lang.String note;
/** Job note as newline (Unix) separated string
*/
public java.lang.String getNote() {
return note;
}
/** Job note as newline (Unix) separated string */
public Insert setNote(java.lang.String note) {
this.note = note;
return this;
}
/** Assignee email address, or empty string to unassign. */
@com.google.api.client.util.Key
private java.lang.String assignee;
/** Assignee email address, or empty string to unassign.
*/
public java.lang.String getAssignee() {
return assignee;
}
/** Assignee email address, or empty string to unassign. */
public Insert setAssignee(java.lang.String assignee) {
this.assignee = assignee;
return this;
}
/** Customer phone number */
@com.google.api.client.util.Key
private java.lang.String customerPhoneNumber;
/** Customer phone number
*/
public java.lang.String getCustomerPhoneNumber() {
return customerPhoneNumber;
}
/** Customer phone number */
public Insert setCustomerPhoneNumber(java.lang.String customerPhoneNumber) {
this.customerPhoneNumber = customerPhoneNumber;
return this;
}
/**
* Sets the value of custom fields. To set a custom field, pass the field id (from
* /team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a
* parameter. For example, customField=12%3DAlice. Repeat the parameter for each custom field.
* Note that '=' cannot appear in the parameter value. Specifying an invalid, or inactive enum
* field will result in an error 500.
*/
@com.google.api.client.util.Key
private java.util.List customField;
/** Sets the value of custom fields. To set a custom field, pass the field id (from
/team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a parameter. For
example, customField=12%3DAlice. Repeat the parameter for each custom field. Note that '=' cannot
appear in the parameter value. Specifying an invalid, or inactive enum field will result in an
error 500.
*/
public java.util.List getCustomField() {
return customField;
}
/**
* Sets the value of custom fields. To set a custom field, pass the field id (from
* /team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a
* parameter. For example, customField=12%3DAlice. Repeat the parameter for each custom field.
* Note that '=' cannot appear in the parameter value. Specifying an invalid, or inactive enum
* field will result in an error 500.
*/
public Insert setCustomField(java.util.List customField) {
this.customField = customField;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves jobs created or modified since the given timestamp.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @return the request
*/
public List list(java.lang.String teamId) throws java.io.IOException {
List result = new List(teamId);
initialize(result);
return result;
}
public class List extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs";
/**
* Retrieves jobs created or modified since the given timestamp.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @since 1.13
*/
protected List(java.lang.String teamId) {
super(Coordinate.this, "GET", REST_PATH, null, com.google.api.services.coordinate.model.JobListResponse.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public List setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Minimum time a job was modified in milliseconds since epoch. */
@com.google.api.client.util.Key
private java.math.BigInteger minModifiedTimestampMs;
/** Minimum time a job was modified in milliseconds since epoch.
*/
public java.math.BigInteger getMinModifiedTimestampMs() {
return minModifiedTimestampMs;
}
/** Minimum time a job was modified in milliseconds since epoch. */
public List setMinModifiedTimestampMs(java.math.BigInteger minModifiedTimestampMs) {
this.minModifiedTimestampMs = minModifiedTimestampMs;
return this;
}
/** Continuation token */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation token
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation token */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum number of results to return in one page. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return in one page.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return in one page. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Whether to omit detail job history information. */
@com.google.api.client.util.Key
private java.lang.Boolean omitJobChanges;
/** Whether to omit detail job history information.
*/
public java.lang.Boolean getOmitJobChanges() {
return omitJobChanges;
}
/** Whether to omit detail job history information. */
public List setOmitJobChanges(java.lang.Boolean omitJobChanges) {
this.omitJobChanges = omitJobChanges;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a job. Fields that are set in the job state will be updated. This method supports patch
* semantics.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Job}
* @return the request
*/
public Patch patch(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Job content) throws java.io.IOException {
Patch result = new Patch(teamId, jobId, content);
initialize(result);
return result;
}
public class Patch extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs/{jobId}";
/**
* Updates a job. Fields that are set in the job state will be updated. This method supports patch
* semantics.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Job}
* @since 1.13
*/
protected Patch(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Job content) {
super(Coordinate.this, "PATCH", REST_PATH, content, com.google.api.services.coordinate.model.Job.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public Patch setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Job number */
@com.google.api.client.util.Key
private java.math.BigInteger jobId;
/** Job number
*/
public java.math.BigInteger getJobId() {
return jobId;
}
/** Job number */
public Patch setJobId(java.math.BigInteger jobId) {
this.jobId = jobId;
return this;
}
/** Customer name */
@com.google.api.client.util.Key
private java.lang.String customerName;
/** Customer name
*/
public java.lang.String getCustomerName() {
return customerName;
}
/** Customer name */
public Patch setCustomerName(java.lang.String customerName) {
this.customerName = customerName;
return this;
}
/** Job title */
@com.google.api.client.util.Key
private java.lang.String title;
/** Job title
*/
public java.lang.String getTitle() {
return title;
}
/** Job title */
public Patch setTitle(java.lang.String title) {
this.title = title;
return this;
}
/** Job note as newline (Unix) separated string */
@com.google.api.client.util.Key
private java.lang.String note;
/** Job note as newline (Unix) separated string
*/
public java.lang.String getNote() {
return note;
}
/** Job note as newline (Unix) separated string */
public Patch setNote(java.lang.String note) {
this.note = note;
return this;
}
/** Assignee email address, or empty string to unassign. */
@com.google.api.client.util.Key
private java.lang.String assignee;
/** Assignee email address, or empty string to unassign.
*/
public java.lang.String getAssignee() {
return assignee;
}
/** Assignee email address, or empty string to unassign. */
public Patch setAssignee(java.lang.String assignee) {
this.assignee = assignee;
return this;
}
/** Customer phone number */
@com.google.api.client.util.Key
private java.lang.String customerPhoneNumber;
/** Customer phone number
*/
public java.lang.String getCustomerPhoneNumber() {
return customerPhoneNumber;
}
/** Customer phone number */
public Patch setCustomerPhoneNumber(java.lang.String customerPhoneNumber) {
this.customerPhoneNumber = customerPhoneNumber;
return this;
}
/** Job address as newline (Unix) separated string */
@com.google.api.client.util.Key
private java.lang.String address;
/** Job address as newline (Unix) separated string
*/
public java.lang.String getAddress() {
return address;
}
/** Job address as newline (Unix) separated string */
public Patch setAddress(java.lang.String address) {
this.address = address;
return this;
}
/** The latitude coordinate of this job's location. */
@com.google.api.client.util.Key
private java.lang.Double lat;
/** The latitude coordinate of this job's location.
*/
public java.lang.Double getLat() {
return lat;
}
/** The latitude coordinate of this job's location. */
public Patch setLat(java.lang.Double lat) {
this.lat = lat;
return this;
}
/** Job progress */
@com.google.api.client.util.Key
private java.lang.String progress;
/** Job progress
*/
public java.lang.String getProgress() {
return progress;
}
/** Job progress */
public Patch setProgress(java.lang.String progress) {
this.progress = progress;
return this;
}
/** The longitude coordinate of this job's location. */
@com.google.api.client.util.Key
private java.lang.Double lng;
/** The longitude coordinate of this job's location.
*/
public java.lang.Double getLng() {
return lng;
}
/** The longitude coordinate of this job's location. */
public Patch setLng(java.lang.Double lng) {
this.lng = lng;
return this;
}
/**
* Sets the value of custom fields. To set a custom field, pass the field id (from
* /team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a
* parameter. For example, customField=12%3DAlice. Repeat the parameter for each custom field.
* Note that '=' cannot appear in the parameter value. Specifying an invalid, or inactive enum
* field will result in an error 500.
*/
@com.google.api.client.util.Key
private java.util.List customField;
/** Sets the value of custom fields. To set a custom field, pass the field id (from
/team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a parameter. For
example, customField=12%3DAlice. Repeat the parameter for each custom field. Note that '=' cannot
appear in the parameter value. Specifying an invalid, or inactive enum field will result in an
error 500.
*/
public java.util.List getCustomField() {
return customField;
}
/**
* Sets the value of custom fields. To set a custom field, pass the field id (from
* /team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a
* parameter. For example, customField=12%3DAlice. Repeat the parameter for each custom field.
* Note that '=' cannot appear in the parameter value. Specifying an invalid, or inactive enum
* field will result in an error 500.
*/
public Patch setCustomField(java.util.List customField) {
this.customField = customField;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates a job. Fields that are set in the job state will be updated.
*
* Create a request for the method "jobs.update".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Job}
* @return the request
*/
public Update update(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Job content) throws java.io.IOException {
Update result = new Update(teamId, jobId, content);
initialize(result);
return result;
}
public class Update extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs/{jobId}";
/**
* Updates a job. Fields that are set in the job state will be updated.
*
* Create a request for the method "jobs.update".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Job}
* @since 1.13
*/
protected Update(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Job content) {
super(Coordinate.this, "PUT", REST_PATH, content, com.google.api.services.coordinate.model.Job.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public Update setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Job number */
@com.google.api.client.util.Key
private java.math.BigInteger jobId;
/** Job number
*/
public java.math.BigInteger getJobId() {
return jobId;
}
/** Job number */
public Update setJobId(java.math.BigInteger jobId) {
this.jobId = jobId;
return this;
}
/** Customer name */
@com.google.api.client.util.Key
private java.lang.String customerName;
/** Customer name
*/
public java.lang.String getCustomerName() {
return customerName;
}
/** Customer name */
public Update setCustomerName(java.lang.String customerName) {
this.customerName = customerName;
return this;
}
/** Job title */
@com.google.api.client.util.Key
private java.lang.String title;
/** Job title
*/
public java.lang.String getTitle() {
return title;
}
/** Job title */
public Update setTitle(java.lang.String title) {
this.title = title;
return this;
}
/** Job note as newline (Unix) separated string */
@com.google.api.client.util.Key
private java.lang.String note;
/** Job note as newline (Unix) separated string
*/
public java.lang.String getNote() {
return note;
}
/** Job note as newline (Unix) separated string */
public Update setNote(java.lang.String note) {
this.note = note;
return this;
}
/** Assignee email address, or empty string to unassign. */
@com.google.api.client.util.Key
private java.lang.String assignee;
/** Assignee email address, or empty string to unassign.
*/
public java.lang.String getAssignee() {
return assignee;
}
/** Assignee email address, or empty string to unassign. */
public Update setAssignee(java.lang.String assignee) {
this.assignee = assignee;
return this;
}
/** Customer phone number */
@com.google.api.client.util.Key
private java.lang.String customerPhoneNumber;
/** Customer phone number
*/
public java.lang.String getCustomerPhoneNumber() {
return customerPhoneNumber;
}
/** Customer phone number */
public Update setCustomerPhoneNumber(java.lang.String customerPhoneNumber) {
this.customerPhoneNumber = customerPhoneNumber;
return this;
}
/** Job address as newline (Unix) separated string */
@com.google.api.client.util.Key
private java.lang.String address;
/** Job address as newline (Unix) separated string
*/
public java.lang.String getAddress() {
return address;
}
/** Job address as newline (Unix) separated string */
public Update setAddress(java.lang.String address) {
this.address = address;
return this;
}
/** The latitude coordinate of this job's location. */
@com.google.api.client.util.Key
private java.lang.Double lat;
/** The latitude coordinate of this job's location.
*/
public java.lang.Double getLat() {
return lat;
}
/** The latitude coordinate of this job's location. */
public Update setLat(java.lang.Double lat) {
this.lat = lat;
return this;
}
/** Job progress */
@com.google.api.client.util.Key
private java.lang.String progress;
/** Job progress
*/
public java.lang.String getProgress() {
return progress;
}
/** Job progress */
public Update setProgress(java.lang.String progress) {
this.progress = progress;
return this;
}
/** The longitude coordinate of this job's location. */
@com.google.api.client.util.Key
private java.lang.Double lng;
/** The longitude coordinate of this job's location.
*/
public java.lang.Double getLng() {
return lng;
}
/** The longitude coordinate of this job's location. */
public Update setLng(java.lang.Double lng) {
this.lng = lng;
return this;
}
/**
* Sets the value of custom fields. To set a custom field, pass the field id (from
* /team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a
* parameter. For example, customField=12%3DAlice. Repeat the parameter for each custom field.
* Note that '=' cannot appear in the parameter value. Specifying an invalid, or inactive enum
* field will result in an error 500.
*/
@com.google.api.client.util.Key
private java.util.List customField;
/** Sets the value of custom fields. To set a custom field, pass the field id (from
/team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a parameter. For
example, customField=12%3DAlice. Repeat the parameter for each custom field. Note that '=' cannot
appear in the parameter value. Specifying an invalid, or inactive enum field will result in an
error 500.
*/
public java.util.List getCustomField() {
return customField;
}
/**
* Sets the value of custom fields. To set a custom field, pass the field id (from
* /team/teamId/custom_fields), a URL escaped '=' character, and the desired value as a
* parameter. For example, customField=12%3DAlice. Repeat the parameter for each custom field.
* Note that '=' cannot appear in the parameter value. Specifying an invalid, or inactive enum
* field will result in an error 500.
*/
public Update setCustomField(java.util.List customField) {
this.customField = customField;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Location collection.
*
* The typical use is:
*
* {@code Coordinate coordinate = new Coordinate(...);}
* {@code Coordinate.Location.List request = coordinate.location().list(parameters ...)}
*
*
* @return the resource collection
*/
public Location location() {
return new Location();
}
/**
* The "location" collection of methods.
*/
public class Location {
/**
* Retrieves a list of locations for a worker.
*
* Create a request for the method "location.list".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param workerEmail Worker email address.
* @param startTimestampMs Start timestamp in milliseconds since the epoch.
* @return the request
*/
public List list(java.lang.String teamId, java.lang.String workerEmail, java.math.BigInteger startTimestampMs) throws java.io.IOException {
List result = new List(teamId, workerEmail, startTimestampMs);
initialize(result);
return result;
}
public class List extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/workers/{workerEmail}/locations";
/**
* Retrieves a list of locations for a worker.
*
* Create a request for the method "location.list".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param workerEmail Worker email address.
* @param startTimestampMs Start timestamp in milliseconds since the epoch.
* @since 1.13
*/
protected List(java.lang.String teamId, java.lang.String workerEmail, java.math.BigInteger startTimestampMs) {
super(Coordinate.this, "GET", REST_PATH, null, com.google.api.services.coordinate.model.LocationListResponse.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.workerEmail = com.google.api.client.util.Preconditions.checkNotNull(workerEmail, "Required parameter workerEmail must be specified.");
this.startTimestampMs = com.google.api.client.util.Preconditions.checkNotNull(startTimestampMs, "Required parameter startTimestampMs must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public List setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Worker email address. */
@com.google.api.client.util.Key
private java.lang.String workerEmail;
/** Worker email address.
*/
public java.lang.String getWorkerEmail() {
return workerEmail;
}
/** Worker email address. */
public List setWorkerEmail(java.lang.String workerEmail) {
this.workerEmail = workerEmail;
return this;
}
/** Start timestamp in milliseconds since the epoch. */
@com.google.api.client.util.Key
private java.math.BigInteger startTimestampMs;
/** Start timestamp in milliseconds since the epoch.
*/
public java.math.BigInteger getStartTimestampMs() {
return startTimestampMs;
}
/** Start timestamp in milliseconds since the epoch. */
public List setStartTimestampMs(java.math.BigInteger startTimestampMs) {
this.startTimestampMs = startTimestampMs;
return this;
}
/** Continuation token */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation token
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation token */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum number of results to return in one page. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of results to return in one page.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of results to return in one page. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Schedule collection.
*
* The typical use is:
*
* {@code Coordinate coordinate = new Coordinate(...);}
* {@code Coordinate.Schedule.List request = coordinate.schedule().list(parameters ...)}
*
*
* @return the resource collection
*/
public Schedule schedule() {
return new Schedule();
}
/**
* The "schedule" collection of methods.
*/
public class Schedule {
/**
* Retrieves the schedule for a job.
*
* Create a request for the method "schedule.get".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param jobId Job number
* @return the request
*/
public Get get(java.lang.String teamId, java.math.BigInteger jobId) throws java.io.IOException {
Get result = new Get(teamId, jobId);
initialize(result);
return result;
}
public class Get extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs/{jobId}/schedule";
/**
* Retrieves the schedule for a job.
*
* Create a request for the method "schedule.get".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param jobId Job number
* @since 1.13
*/
protected Get(java.lang.String teamId, java.math.BigInteger jobId) {
super(Coordinate.this, "GET", REST_PATH, null, com.google.api.services.coordinate.model.Schedule.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public Get setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Job number */
@com.google.api.client.util.Key
private java.math.BigInteger jobId;
/** Job number
*/
public java.math.BigInteger getJobId() {
return jobId;
}
/** Job number */
public Get setJobId(java.math.BigInteger jobId) {
this.jobId = jobId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Replaces the schedule of a job with the provided schedule. This method supports patch semantics.
*
* Create a request for the method "schedule.patch".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Schedule}
* @return the request
*/
public Patch patch(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Schedule content) throws java.io.IOException {
Patch result = new Patch(teamId, jobId, content);
initialize(result);
return result;
}
public class Patch extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs/{jobId}/schedule";
/**
* Replaces the schedule of a job with the provided schedule. This method supports patch
* semantics.
*
* Create a request for the method "schedule.patch".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Schedule}
* @since 1.13
*/
protected Patch(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Schedule content) {
super(Coordinate.this, "PATCH", REST_PATH, content, com.google.api.services.coordinate.model.Schedule.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public Patch setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Job number */
@com.google.api.client.util.Key
private java.math.BigInteger jobId;
/** Job number
*/
public java.math.BigInteger getJobId() {
return jobId;
}
/** Job number */
public Patch setJobId(java.math.BigInteger jobId) {
this.jobId = jobId;
return this;
}
/**
* Whether the job is scheduled for the whole day. Time of day in start/end times is ignored
* if this is true.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allDay;
/** Whether the job is scheduled for the whole day. Time of day in start/end times is ignored if this
is true.
*/
public java.lang.Boolean getAllDay() {
return allDay;
}
/**
* Whether the job is scheduled for the whole day. Time of day in start/end times is ignored
* if this is true.
*/
public Patch setAllDay(java.lang.Boolean allDay) {
this.allDay = allDay;
return this;
}
/** Scheduled start time in milliseconds since epoch. */
@com.google.api.client.util.Key
private java.math.BigInteger startTime;
/** Scheduled start time in milliseconds since epoch.
*/
public java.math.BigInteger getStartTime() {
return startTime;
}
/** Scheduled start time in milliseconds since epoch. */
public Patch setStartTime(java.math.BigInteger startTime) {
this.startTime = startTime;
return this;
}
/** Job duration in milliseconds. */
@com.google.api.client.util.Key
private java.math.BigInteger duration;
/** Job duration in milliseconds.
*/
public java.math.BigInteger getDuration() {
return duration;
}
/** Job duration in milliseconds. */
public Patch setDuration(java.math.BigInteger duration) {
this.duration = duration;
return this;
}
/** Scheduled end time in milliseconds since epoch. */
@com.google.api.client.util.Key
private java.math.BigInteger endTime;
/** Scheduled end time in milliseconds since epoch.
*/
public java.math.BigInteger getEndTime() {
return endTime;
}
/** Scheduled end time in milliseconds since epoch. */
public Patch setEndTime(java.math.BigInteger endTime) {
this.endTime = endTime;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Replaces the schedule of a job with the provided schedule.
*
* Create a request for the method "schedule.update".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Schedule}
* @return the request
*/
public Update update(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Schedule content) throws java.io.IOException {
Update result = new Update(teamId, jobId, content);
initialize(result);
return result;
}
public class Update extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/jobs/{jobId}/schedule";
/**
* Replaces the schedule of a job with the provided schedule.
*
* Create a request for the method "schedule.update".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @param jobId Job number
* @param content the {@link com.google.api.services.coordinate.model.Schedule}
* @since 1.13
*/
protected Update(java.lang.String teamId, java.math.BigInteger jobId, com.google.api.services.coordinate.model.Schedule content) {
super(Coordinate.this, "PUT", REST_PATH, content, com.google.api.services.coordinate.model.Schedule.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public Update setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
/** Job number */
@com.google.api.client.util.Key
private java.math.BigInteger jobId;
/** Job number
*/
public java.math.BigInteger getJobId() {
return jobId;
}
/** Job number */
public Update setJobId(java.math.BigInteger jobId) {
this.jobId = jobId;
return this;
}
/**
* Whether the job is scheduled for the whole day. Time of day in start/end times is ignored
* if this is true.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allDay;
/** Whether the job is scheduled for the whole day. Time of day in start/end times is ignored if this
is true.
*/
public java.lang.Boolean getAllDay() {
return allDay;
}
/**
* Whether the job is scheduled for the whole day. Time of day in start/end times is ignored
* if this is true.
*/
public Update setAllDay(java.lang.Boolean allDay) {
this.allDay = allDay;
return this;
}
/** Scheduled start time in milliseconds since epoch. */
@com.google.api.client.util.Key
private java.math.BigInteger startTime;
/** Scheduled start time in milliseconds since epoch.
*/
public java.math.BigInteger getStartTime() {
return startTime;
}
/** Scheduled start time in milliseconds since epoch. */
public Update setStartTime(java.math.BigInteger startTime) {
this.startTime = startTime;
return this;
}
/** Job duration in milliseconds. */
@com.google.api.client.util.Key
private java.math.BigInteger duration;
/** Job duration in milliseconds.
*/
public java.math.BigInteger getDuration() {
return duration;
}
/** Job duration in milliseconds. */
public Update setDuration(java.math.BigInteger duration) {
this.duration = duration;
return this;
}
/** Scheduled end time in milliseconds since epoch. */
@com.google.api.client.util.Key
private java.math.BigInteger endTime;
/** Scheduled end time in milliseconds since epoch.
*/
public java.math.BigInteger getEndTime() {
return endTime;
}
/** Scheduled end time in milliseconds since epoch. */
public Update setEndTime(java.math.BigInteger endTime) {
this.endTime = endTime;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Team collection.
*
* The typical use is:
*
* {@code Coordinate coordinate = new Coordinate(...);}
* {@code Coordinate.Team.List request = coordinate.team().list(parameters ...)}
*
*
* @return the resource collection
*/
public Team team() {
return new Team();
}
/**
* The "team" collection of methods.
*/
public class Team {
/**
* Retrieves a list of teams for a user.
*
* Create a request for the method "team.list".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CoordinateRequest {
private static final String REST_PATH = "teams";
/**
* Retrieves a list of teams for a user.
*
* Create a request for the method "team.list".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Coordinate.this, "GET", REST_PATH, null, com.google.api.services.coordinate.model.TeamListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Whether to include teams for which the user has the Admin role. */
@com.google.api.client.util.Key
private java.lang.Boolean admin;
/** Whether to include teams for which the user has the Admin role.
*/
public java.lang.Boolean getAdmin() {
return admin;
}
/** Whether to include teams for which the user has the Admin role. */
public List setAdmin(java.lang.Boolean admin) {
this.admin = admin;
return this;
}
/** Whether to include teams for which the user has the Worker role. */
@com.google.api.client.util.Key
private java.lang.Boolean worker;
/** Whether to include teams for which the user has the Worker role.
*/
public java.lang.Boolean getWorker() {
return worker;
}
/** Whether to include teams for which the user has the Worker role. */
public List setWorker(java.lang.Boolean worker) {
this.worker = worker;
return this;
}
/** Whether to include teams for which the user has the Dispatcher role. */
@com.google.api.client.util.Key
private java.lang.Boolean dispatcher;
/** Whether to include teams for which the user has the Dispatcher role.
*/
public java.lang.Boolean getDispatcher() {
return dispatcher;
}
/** Whether to include teams for which the user has the Dispatcher role. */
public List setDispatcher(java.lang.Boolean dispatcher) {
this.dispatcher = dispatcher;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Worker collection.
*
* The typical use is:
*
* {@code Coordinate coordinate = new Coordinate(...);}
* {@code Coordinate.Worker.List request = coordinate.worker().list(parameters ...)}
*
*
* @return the resource collection
*/
public Worker worker() {
return new Worker();
}
/**
* The "worker" collection of methods.
*/
public class Worker {
/**
* Retrieves a list of workers in a team.
*
* Create a request for the method "worker.list".
*
* This request holds the parameters needed by the coordinate server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param teamId Team ID
* @return the request
*/
public List list(java.lang.String teamId) throws java.io.IOException {
List result = new List(teamId);
initialize(result);
return result;
}
public class List extends CoordinateRequest {
private static final String REST_PATH = "teams/{teamId}/workers";
/**
* Retrieves a list of workers in a team.
*
* Create a request for the method "worker.list".
*
* This request holds the parameters needed by the the coordinate server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param teamId Team ID
* @since 1.13
*/
protected List(java.lang.String teamId) {
super(Coordinate.this, "GET", REST_PATH, null, com.google.api.services.coordinate.model.WorkerListResponse.class);
this.teamId = com.google.api.client.util.Preconditions.checkNotNull(teamId, "Required parameter teamId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Team ID */
@com.google.api.client.util.Key
private java.lang.String teamId;
/** Team ID
*/
public java.lang.String getTeamId() {
return teamId;
}
/** Team ID */
public List setTeamId(java.lang.String teamId) {
this.teamId = teamId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Coordinate}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Coordinate}. */
@Override
public Coordinate build() {
return new Coordinate(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CoordinateRequestInitializer}.
*
* @since 1.12
*/
public Builder setCoordinateRequestInitializer(
CoordinateRequestInitializer coordinateRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(coordinateRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}