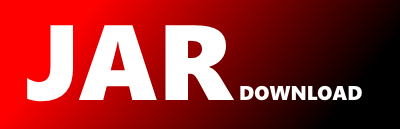
com.google.api.services.customsearch.v1.Customsearch Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.customsearch.v1;
/**
* Service definition for Customsearch (v1).
*
*
* Searches over a website or collection of websites
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CustomsearchRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Customsearch extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.30.9 of the Custom Search API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://customsearch.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Customsearch(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Customsearch(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Cse collection.
*
* The typical use is:
*
* {@code Customsearch customsearch = new Customsearch(...);}
* {@code Customsearch.Cse.List request = customsearch.cse().list(parameters ...)}
*
*
* @return the resource collection
*/
public Cse cse() {
return new Cse();
}
/**
* The "cse" collection of methods.
*/
public class Cse {
/**
* Returns metadata about the search performed, metadata about the custom search engine used for the
* search, and the search results.
*
* Create a request for the method "cse.list".
*
* This request holds the parameters needed by the customsearch server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CustomsearchRequest {
private static final String REST_PATH = "customsearch/v1";
/**
* Returns metadata about the search performed, metadata about the custom search engine used for
* the search, and the search results.
*
* Create a request for the method "cse.list".
*
* This request holds the parameters needed by the the customsearch server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Customsearch.this, "GET", REST_PATH, null, com.google.api.services.customsearch.v1.model.Search.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Turns off the translation between zh-CN and zh-TW. */
@com.google.api.client.util.Key
private java.lang.String c2coff;
/** Turns off the translation between zh-CN and zh-TW.
*/
public java.lang.String getC2coff() {
return c2coff;
}
/** Turns off the translation between zh-CN and zh-TW. */
public List setC2coff(java.lang.String c2coff) {
this.c2coff = c2coff;
return this;
}
/** Country restrict(s). */
@com.google.api.client.util.Key
private java.lang.String cr;
/** Country restrict(s).
*/
public java.lang.String getCr() {
return cr;
}
/** Country restrict(s). */
public List setCr(java.lang.String cr) {
this.cr = cr;
return this;
}
/** The custom search engine ID to scope this search query */
@com.google.api.client.util.Key
private java.lang.String cx;
/** The custom search engine ID to scope this search query
*/
public java.lang.String getCx() {
return cx;
}
/** The custom search engine ID to scope this search query */
public List setCx(java.lang.String cx) {
this.cx = cx;
return this;
}
/** Specifies all search results are from a time period */
@com.google.api.client.util.Key
private java.lang.String dateRestrict;
/** Specifies all search results are from a time period
*/
public java.lang.String getDateRestrict() {
return dateRestrict;
}
/** Specifies all search results are from a time period */
public List setDateRestrict(java.lang.String dateRestrict) {
this.dateRestrict = dateRestrict;
return this;
}
/** Identifies a phrase that all documents in the search results must contain */
@com.google.api.client.util.Key
private java.lang.String exactTerms;
/** Identifies a phrase that all documents in the search results must contain
*/
public java.lang.String getExactTerms() {
return exactTerms;
}
/** Identifies a phrase that all documents in the search results must contain */
public List setExactTerms(java.lang.String exactTerms) {
this.exactTerms = exactTerms;
return this;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results
*/
@com.google.api.client.util.Key
private java.lang.String excludeTerms;
/** Identifies a word or phrase that should not appear in any documents in the search results
*/
public java.lang.String getExcludeTerms() {
return excludeTerms;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results
*/
public List setExcludeTerms(java.lang.String excludeTerms) {
this.excludeTerms = excludeTerms;
return this;
}
/**
* Returns images of a specified type. Some of the allowed values are: bmp, gif, png, jpg,
* svg, pdf, ...
*/
@com.google.api.client.util.Key
private java.lang.String fileType;
/** Returns images of a specified type. Some of the allowed values are: bmp, gif, png, jpg, svg, pdf,
...
*/
public java.lang.String getFileType() {
return fileType;
}
/**
* Returns images of a specified type. Some of the allowed values are: bmp, gif, png, jpg,
* svg, pdf, ...
*/
public List setFileType(java.lang.String fileType) {
this.fileType = fileType;
return this;
}
/** Controls turning on (1) or off (0) the duplicate content filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Controls turning on (1) or off (0) the duplicate content filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** Controls turning on (1) or off (0) the duplicate content filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Geolocation of end user. */
@com.google.api.client.util.Key
private java.lang.String gl;
/** Geolocation of end user.
*/
public java.lang.String getGl() {
return gl;
}
/** Geolocation of end user. */
public List setGl(java.lang.String gl) {
this.gl = gl;
return this;
}
/** The local Google domain to use to perform the search. */
@com.google.api.client.util.Key
private java.lang.String googlehost;
/** The local Google domain to use to perform the search.
*/
public java.lang.String getGooglehost() {
return googlehost;
}
/** The local Google domain to use to perform the search. */
public List setGooglehost(java.lang.String googlehost) {
this.googlehost = googlehost;
return this;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
@com.google.api.client.util.Key
private java.lang.String highRange;
/** Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public java.lang.String getHighRange() {
return highRange;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public List setHighRange(java.lang.String highRange) {
this.highRange = highRange;
return this;
}
/** Sets the user interface language. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Sets the user interface language.
*/
public java.lang.String getHl() {
return hl;
}
/** Sets the user interface language. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
/** Appends the extra hidden query terms to the query. */
@com.google.api.client.util.Key
private java.lang.String hq;
/** Appends the extra hidden query terms to the query.
*/
public java.lang.String getHq() {
return hq;
}
/** Appends the extra hidden query terms to the query. */
public List setHq(java.lang.String hq) {
this.hq = hq;
return this;
}
/**
* Returns black and white, grayscale, transparent-background or color images: mono, gray,
* trans, and color.
*/
@com.google.api.client.util.Key
private java.lang.String imgColorType;
/** Returns black and white, grayscale, transparent-background or color images: mono, gray, trans, and
color.
*/
public java.lang.String getImgColorType() {
return imgColorType;
}
/**
* Returns black and white, grayscale, transparent-background or color images: mono, gray,
* trans, and color.
*/
public List setImgColorType(java.lang.String imgColorType) {
this.imgColorType = imgColorType;
return this;
}
/**
* Returns images of a specific dominant color: red, orange, yellow, green, teal, blue,
* purple, pink, white, gray, black and brown.
*/
@com.google.api.client.util.Key
private java.lang.String imgDominantColor;
/** Returns images of a specific dominant color: red, orange, yellow, green, teal, blue, purple, pink,
white, gray, black and brown.
*/
public java.lang.String getImgDominantColor() {
return imgDominantColor;
}
/**
* Returns images of a specific dominant color: red, orange, yellow, green, teal, blue,
* purple, pink, white, gray, black and brown.
*/
public List setImgDominantColor(java.lang.String imgDominantColor) {
this.imgDominantColor = imgDominantColor;
return this;
}
/**
* Returns images of a specified size, where size can be one of: icon, small, medium, large,
* xlarge, xxlarge, and huge.
*/
@com.google.api.client.util.Key
private java.lang.String imgSize;
/** Returns images of a specified size, where size can be one of: icon, small, medium, large, xlarge,
xxlarge, and huge.
*/
public java.lang.String getImgSize() {
return imgSize;
}
/**
* Returns images of a specified size, where size can be one of: icon, small, medium, large,
* xlarge, xxlarge, and huge.
*/
public List setImgSize(java.lang.String imgSize) {
this.imgSize = imgSize;
return this;
}
/**
* Returns images of a type, which can be one of: clipart, face, lineart, stock, photo, and
* animated.
*/
@com.google.api.client.util.Key
private java.lang.String imgType;
/** Returns images of a type, which can be one of: clipart, face, lineart, stock, photo, and animated.
*/
public java.lang.String getImgType() {
return imgType;
}
/**
* Returns images of a type, which can be one of: clipart, face, lineart, stock, photo, and
* animated.
*/
public List setImgType(java.lang.String imgType) {
this.imgType = imgType;
return this;
}
/** Specifies that all search results should contain a link to a particular URL */
@com.google.api.client.util.Key
private java.lang.String linkSite;
/** Specifies that all search results should contain a link to a particular URL
*/
public java.lang.String getLinkSite() {
return linkSite;
}
/** Specifies that all search results should contain a link to a particular URL */
public List setLinkSite(java.lang.String linkSite) {
this.linkSite = linkSite;
return this;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
@com.google.api.client.util.Key
private java.lang.String lowRange;
/** Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public java.lang.String getLowRange() {
return lowRange;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public List setLowRange(java.lang.String lowRange) {
this.lowRange = lowRange;
return this;
}
/** The language restriction for the search results */
@com.google.api.client.util.Key
private java.lang.String lr;
/** The language restriction for the search results
*/
public java.lang.String getLr() {
return lr;
}
/** The language restriction for the search results */
public List setLr(java.lang.String lr) {
this.lr = lr;
return this;
}
/** Number of search results to return */
@com.google.api.client.util.Key
private java.lang.Integer num;
/** Number of search results to return
*/
public java.lang.Integer getNum() {
return num;
}
/** Number of search results to return */
public List setNum(java.lang.Integer num) {
this.num = num;
return this;
}
/**
* Provides additional search terms to check for in a document, where each document in the
* search results must contain at least one of the additional search terms
*/
@com.google.api.client.util.Key
private java.lang.String orTerms;
/** Provides additional search terms to check for in a document, where each document in the search
results must contain at least one of the additional search terms
*/
public java.lang.String getOrTerms() {
return orTerms;
}
/**
* Provides additional search terms to check for in a document, where each document in the
* search results must contain at least one of the additional search terms
*/
public List setOrTerms(java.lang.String orTerms) {
this.orTerms = orTerms;
return this;
}
/** Query */
@com.google.api.client.util.Key
private java.lang.String q;
/** Query
*/
public java.lang.String getQ() {
return q;
}
/** Query */
public List setQ(java.lang.String q) {
this.q = q;
return this;
}
/**
* Specifies that all search results should be pages that are related to the specified URL
*/
@com.google.api.client.util.Key
private java.lang.String relatedSite;
/** Specifies that all search results should be pages that are related to the specified URL
*/
public java.lang.String getRelatedSite() {
return relatedSite;
}
/**
* Specifies that all search results should be pages that are related to the specified URL
*/
public List setRelatedSite(java.lang.String relatedSite) {
this.relatedSite = relatedSite;
return this;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain, cc_attribute,
* cc_sharealike, cc_noncommercial, cc_nonderived and combinations of these. See
* https://wiki.creativecommons.org/wiki/CC_Search_integration for typical combinations.
*/
@com.google.api.client.util.Key
private java.lang.String rights;
/** Filters based on licensing. Supported values include: cc_publicdomain, cc_attribute, cc_sharealike,
cc_noncommercial, cc_nonderived and combinations of these. See
https://wiki.creativecommons.org/wiki/CC_Search_integration for typical combinations.
*/
public java.lang.String getRights() {
return rights;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain, cc_attribute,
* cc_sharealike, cc_noncommercial, cc_nonderived and combinations of these. See
* https://wiki.creativecommons.org/wiki/CC_Search_integration for typical combinations.
*/
public List setRights(java.lang.String rights) {
this.rights = rights;
return this;
}
/** Search safety level (active, off) (high, medium are same as active) */
@com.google.api.client.util.Key
private java.lang.String safe;
/** Search safety level (active, off) (high, medium are same as active)
*/
public java.lang.String getSafe() {
return safe;
}
/** Search safety level (active, off) (high, medium are same as active) */
public List setSafe(java.lang.String safe) {
this.safe = safe;
return this;
}
/** Specifies the search type: image. */
@com.google.api.client.util.Key
private java.lang.String searchType;
/** Specifies the search type: image.
*/
public java.lang.String getSearchType() {
return searchType;
}
/** Specifies the search type: image. */
public List setSearchType(java.lang.String searchType) {
this.searchType = searchType;
return this;
}
/** Specifies all search results should be pages from a given site */
@com.google.api.client.util.Key
private java.lang.String siteSearch;
/** Specifies all search results should be pages from a given site
*/
public java.lang.String getSiteSearch() {
return siteSearch;
}
/** Specifies all search results should be pages from a given site */
public List setSiteSearch(java.lang.String siteSearch) {
this.siteSearch = siteSearch;
return this;
}
/**
* Controls whether to include (i) or exclude (e) results from the site named in the
* siteSearch parameter
*/
@com.google.api.client.util.Key
private java.lang.String siteSearchFilter;
/** Controls whether to include (i) or exclude (e) results from the site named in the siteSearch
parameter
*/
public java.lang.String getSiteSearchFilter() {
return siteSearchFilter;
}
/**
* Controls whether to include (i) or exclude (e) results from the site named in the
* siteSearch parameter
*/
public List setSiteSearchFilter(java.lang.String siteSearchFilter) {
this.siteSearchFilter = siteSearchFilter;
return this;
}
/** The sort expression to apply to the results */
@com.google.api.client.util.Key
private java.lang.String sort;
/** The sort expression to apply to the results
*/
public java.lang.String getSort() {
return sort;
}
/** The sort expression to apply to the results */
public List setSort(java.lang.String sort) {
this.sort = sort;
return this;
}
/** The index of the first result to return */
@com.google.api.client.util.Key
private java.lang.Long start;
/** The index of the first result to return
*/
public java.lang.Long getStart() {
return start;
}
/** The index of the first result to return */
public List setStart(java.lang.Long start) {
this.start = start;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Siterestrict collection.
*
* The typical use is:
*
* {@code Customsearch customsearch = new Customsearch(...);}
* {@code Customsearch.Siterestrict.List request = customsearch.siterestrict().list(parameters ...)}
*
*
* @return the resource collection
*/
public Siterestrict siterestrict() {
return new Siterestrict();
}
/**
* The "siterestrict" collection of methods.
*/
public class Siterestrict {
/**
* Returns metadata about the search performed, metadata about the custom search engine used for the
* search, and the search results. Uses a small set of url patterns.
*
* Create a request for the method "siterestrict.list".
*
* This request holds the parameters needed by the customsearch server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends CustomsearchRequest {
private static final String REST_PATH = "customsearch/v1/siterestrict";
/**
* Returns metadata about the search performed, metadata about the custom search engine used for
* the search, and the search results. Uses a small set of url patterns.
*
* Create a request for the method "siterestrict.list".
*
* This request holds the parameters needed by the the customsearch server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Customsearch.this, "GET", REST_PATH, null, com.google.api.services.customsearch.v1.model.Search.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Turns off the translation between zh-CN and zh-TW. */
@com.google.api.client.util.Key
private java.lang.String c2coff;
/** Turns off the translation between zh-CN and zh-TW.
*/
public java.lang.String getC2coff() {
return c2coff;
}
/** Turns off the translation between zh-CN and zh-TW. */
public List setC2coff(java.lang.String c2coff) {
this.c2coff = c2coff;
return this;
}
/** Country restrict(s). */
@com.google.api.client.util.Key
private java.lang.String cr;
/** Country restrict(s).
*/
public java.lang.String getCr() {
return cr;
}
/** Country restrict(s). */
public List setCr(java.lang.String cr) {
this.cr = cr;
return this;
}
/** The custom search engine ID to scope this search query */
@com.google.api.client.util.Key
private java.lang.String cx;
/** The custom search engine ID to scope this search query
*/
public java.lang.String getCx() {
return cx;
}
/** The custom search engine ID to scope this search query */
public List setCx(java.lang.String cx) {
this.cx = cx;
return this;
}
/** Specifies all search results are from a time period */
@com.google.api.client.util.Key
private java.lang.String dateRestrict;
/** Specifies all search results are from a time period
*/
public java.lang.String getDateRestrict() {
return dateRestrict;
}
/** Specifies all search results are from a time period */
public List setDateRestrict(java.lang.String dateRestrict) {
this.dateRestrict = dateRestrict;
return this;
}
/** Identifies a phrase that all documents in the search results must contain */
@com.google.api.client.util.Key
private java.lang.String exactTerms;
/** Identifies a phrase that all documents in the search results must contain
*/
public java.lang.String getExactTerms() {
return exactTerms;
}
/** Identifies a phrase that all documents in the search results must contain */
public List setExactTerms(java.lang.String exactTerms) {
this.exactTerms = exactTerms;
return this;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results
*/
@com.google.api.client.util.Key
private java.lang.String excludeTerms;
/** Identifies a word or phrase that should not appear in any documents in the search results
*/
public java.lang.String getExcludeTerms() {
return excludeTerms;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results
*/
public List setExcludeTerms(java.lang.String excludeTerms) {
this.excludeTerms = excludeTerms;
return this;
}
/**
* Returns images of a specified type. Some of the allowed values are: bmp, gif, png, jpg,
* svg, pdf, ...
*/
@com.google.api.client.util.Key
private java.lang.String fileType;
/** Returns images of a specified type. Some of the allowed values are: bmp, gif, png, jpg, svg, pdf,
...
*/
public java.lang.String getFileType() {
return fileType;
}
/**
* Returns images of a specified type. Some of the allowed values are: bmp, gif, png, jpg,
* svg, pdf, ...
*/
public List setFileType(java.lang.String fileType) {
this.fileType = fileType;
return this;
}
/** Controls turning on (1) or off (0) the duplicate content filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Controls turning on (1) or off (0) the duplicate content filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** Controls turning on (1) or off (0) the duplicate content filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Geolocation of end user. */
@com.google.api.client.util.Key
private java.lang.String gl;
/** Geolocation of end user.
*/
public java.lang.String getGl() {
return gl;
}
/** Geolocation of end user. */
public List setGl(java.lang.String gl) {
this.gl = gl;
return this;
}
/** The local Google domain to use to perform the search. */
@com.google.api.client.util.Key
private java.lang.String googlehost;
/** The local Google domain to use to perform the search.
*/
public java.lang.String getGooglehost() {
return googlehost;
}
/** The local Google domain to use to perform the search. */
public List setGooglehost(java.lang.String googlehost) {
this.googlehost = googlehost;
return this;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
@com.google.api.client.util.Key
private java.lang.String highRange;
/** Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public java.lang.String getHighRange() {
return highRange;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public List setHighRange(java.lang.String highRange) {
this.highRange = highRange;
return this;
}
/** Sets the user interface language. */
@com.google.api.client.util.Key
private java.lang.String hl;
/** Sets the user interface language.
*/
public java.lang.String getHl() {
return hl;
}
/** Sets the user interface language. */
public List setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
/** Appends the extra hidden query terms to the query. */
@com.google.api.client.util.Key
private java.lang.String hq;
/** Appends the extra hidden query terms to the query.
*/
public java.lang.String getHq() {
return hq;
}
/** Appends the extra hidden query terms to the query. */
public List setHq(java.lang.String hq) {
this.hq = hq;
return this;
}
/**
* Returns black and white, grayscale, transparent-background or color images: mono, gray,
* trans, and color.
*/
@com.google.api.client.util.Key
private java.lang.String imgColorType;
/** Returns black and white, grayscale, transparent-background or color images: mono, gray, trans, and
color.
*/
public java.lang.String getImgColorType() {
return imgColorType;
}
/**
* Returns black and white, grayscale, transparent-background or color images: mono, gray,
* trans, and color.
*/
public List setImgColorType(java.lang.String imgColorType) {
this.imgColorType = imgColorType;
return this;
}
/**
* Returns images of a specific dominant color: red, orange, yellow, green, teal, blue,
* purple, pink, white, gray, black and brown.
*/
@com.google.api.client.util.Key
private java.lang.String imgDominantColor;
/** Returns images of a specific dominant color: red, orange, yellow, green, teal, blue, purple, pink,
white, gray, black and brown.
*/
public java.lang.String getImgDominantColor() {
return imgDominantColor;
}
/**
* Returns images of a specific dominant color: red, orange, yellow, green, teal, blue,
* purple, pink, white, gray, black and brown.
*/
public List setImgDominantColor(java.lang.String imgDominantColor) {
this.imgDominantColor = imgDominantColor;
return this;
}
/**
* Returns images of a specified size, where size can be one of: icon, small, medium, large,
* xlarge, xxlarge, and huge.
*/
@com.google.api.client.util.Key
private java.lang.String imgSize;
/** Returns images of a specified size, where size can be one of: icon, small, medium, large, xlarge,
xxlarge, and huge.
*/
public java.lang.String getImgSize() {
return imgSize;
}
/**
* Returns images of a specified size, where size can be one of: icon, small, medium, large,
* xlarge, xxlarge, and huge.
*/
public List setImgSize(java.lang.String imgSize) {
this.imgSize = imgSize;
return this;
}
/**
* Returns images of a type, which can be one of: clipart, face, lineart, stock, photo, and
* animated.
*/
@com.google.api.client.util.Key
private java.lang.String imgType;
/** Returns images of a type, which can be one of: clipart, face, lineart, stock, photo, and animated.
*/
public java.lang.String getImgType() {
return imgType;
}
/**
* Returns images of a type, which can be one of: clipart, face, lineart, stock, photo, and
* animated.
*/
public List setImgType(java.lang.String imgType) {
this.imgType = imgType;
return this;
}
/** Specifies that all search results should contain a link to a particular URL */
@com.google.api.client.util.Key
private java.lang.String linkSite;
/** Specifies that all search results should contain a link to a particular URL
*/
public java.lang.String getLinkSite() {
return linkSite;
}
/** Specifies that all search results should contain a link to a particular URL */
public List setLinkSite(java.lang.String linkSite) {
this.linkSite = linkSite;
return this;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
@com.google.api.client.util.Key
private java.lang.String lowRange;
/** Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public java.lang.String getLowRange() {
return lowRange;
}
/**
* Creates a range in form as_nlo value..as_nhi value and attempts to append it to query
*/
public List setLowRange(java.lang.String lowRange) {
this.lowRange = lowRange;
return this;
}
/** The language restriction for the search results */
@com.google.api.client.util.Key
private java.lang.String lr;
/** The language restriction for the search results
*/
public java.lang.String getLr() {
return lr;
}
/** The language restriction for the search results */
public List setLr(java.lang.String lr) {
this.lr = lr;
return this;
}
/** Number of search results to return */
@com.google.api.client.util.Key
private java.lang.Integer num;
/** Number of search results to return
*/
public java.lang.Integer getNum() {
return num;
}
/** Number of search results to return */
public List setNum(java.lang.Integer num) {
this.num = num;
return this;
}
/**
* Provides additional search terms to check for in a document, where each document in the
* search results must contain at least one of the additional search terms
*/
@com.google.api.client.util.Key
private java.lang.String orTerms;
/** Provides additional search terms to check for in a document, where each document in the search
results must contain at least one of the additional search terms
*/
public java.lang.String getOrTerms() {
return orTerms;
}
/**
* Provides additional search terms to check for in a document, where each document in the
* search results must contain at least one of the additional search terms
*/
public List setOrTerms(java.lang.String orTerms) {
this.orTerms = orTerms;
return this;
}
/** Query */
@com.google.api.client.util.Key
private java.lang.String q;
/** Query
*/
public java.lang.String getQ() {
return q;
}
/** Query */
public List setQ(java.lang.String q) {
this.q = q;
return this;
}
/**
* Specifies that all search results should be pages that are related to the specified URL
*/
@com.google.api.client.util.Key
private java.lang.String relatedSite;
/** Specifies that all search results should be pages that are related to the specified URL
*/
public java.lang.String getRelatedSite() {
return relatedSite;
}
/**
* Specifies that all search results should be pages that are related to the specified URL
*/
public List setRelatedSite(java.lang.String relatedSite) {
this.relatedSite = relatedSite;
return this;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain, cc_attribute,
* cc_sharealike, cc_noncommercial, cc_nonderived and combinations of these. See
* https://wiki.creativecommons.org/wiki/CC_Search_integration for typical combinations.
*/
@com.google.api.client.util.Key
private java.lang.String rights;
/** Filters based on licensing. Supported values include: cc_publicdomain, cc_attribute, cc_sharealike,
cc_noncommercial, cc_nonderived and combinations of these. See
https://wiki.creativecommons.org/wiki/CC_Search_integration for typical combinations.
*/
public java.lang.String getRights() {
return rights;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain, cc_attribute,
* cc_sharealike, cc_noncommercial, cc_nonderived and combinations of these. See
* https://wiki.creativecommons.org/wiki/CC_Search_integration for typical combinations.
*/
public List setRights(java.lang.String rights) {
this.rights = rights;
return this;
}
/** Search safety level (active, off) (high, medium are same as active) */
@com.google.api.client.util.Key
private java.lang.String safe;
/** Search safety level (active, off) (high, medium are same as active)
*/
public java.lang.String getSafe() {
return safe;
}
/** Search safety level (active, off) (high, medium are same as active) */
public List setSafe(java.lang.String safe) {
this.safe = safe;
return this;
}
/** Specifies the search type: image. */
@com.google.api.client.util.Key
private java.lang.String searchType;
/** Specifies the search type: image.
*/
public java.lang.String getSearchType() {
return searchType;
}
/** Specifies the search type: image. */
public List setSearchType(java.lang.String searchType) {
this.searchType = searchType;
return this;
}
/** Specifies all search results should be pages from a given site */
@com.google.api.client.util.Key
private java.lang.String siteSearch;
/** Specifies all search results should be pages from a given site
*/
public java.lang.String getSiteSearch() {
return siteSearch;
}
/** Specifies all search results should be pages from a given site */
public List setSiteSearch(java.lang.String siteSearch) {
this.siteSearch = siteSearch;
return this;
}
/**
* Controls whether to include (i) or exclude (e) results from the site named in the
* siteSearch parameter
*/
@com.google.api.client.util.Key
private java.lang.String siteSearchFilter;
/** Controls whether to include (i) or exclude (e) results from the site named in the siteSearch
parameter
*/
public java.lang.String getSiteSearchFilter() {
return siteSearchFilter;
}
/**
* Controls whether to include (i) or exclude (e) results from the site named in the
* siteSearch parameter
*/
public List setSiteSearchFilter(java.lang.String siteSearchFilter) {
this.siteSearchFilter = siteSearchFilter;
return this;
}
/** The sort expression to apply to the results */
@com.google.api.client.util.Key
private java.lang.String sort;
/** The sort expression to apply to the results
*/
public java.lang.String getSort() {
return sort;
}
/** The sort expression to apply to the results */
public List setSort(java.lang.String sort) {
this.sort = sort;
return this;
}
/** The index of the first result to return */
@com.google.api.client.util.Key
private java.lang.Long start;
/** The index of the first result to return
*/
public java.lang.Long getStart() {
return start;
}
/** The index of the first result to return */
public List setStart(java.lang.Long start) {
this.start = start;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Customsearch}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Customsearch}. */
@Override
public Customsearch build() {
return new Customsearch(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CustomsearchRequestInitializer}.
*
* @since 1.12
*/
public Builder setCustomsearchRequestInitializer(
CustomsearchRequestInitializer customsearchRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(customsearchRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}