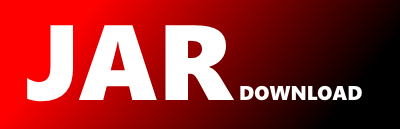
com.google.api.services.customsearch.v1.model.Search Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.customsearch.v1.model;
/**
* Response to a custom search request.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Custom Search API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Search extends com.google.api.client.json.GenericJson {
/**
* Metadata and refinements associated with the given search engine.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map context;
/**
* The current set of custom search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List items;
static {
// hack to force ProGuard to consider Result used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Result.class);
}
/**
* Unique identifier for the type of current object. For this API, it is customsearch#search.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The set of promotions. Present only if the custom search engine's configuration files define
* any promotions for the given query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List promotions;
static {
// hack to force ProGuard to consider Promotion used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Promotion.class);
}
/**
* Query metadata for the previous, current, and next pages of results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Queries queries;
/**
* Metadata about a search operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SearchInformation searchInformation;
/**
* Spell correction information for a query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Spelling spelling;
/**
* OpenSearch template and URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Url url;
/**
* Metadata and refinements associated with the given search engine.
* @return value or {@code null} for none
*/
public java.util.Map getContext() {
return context;
}
/**
* Metadata and refinements associated with the given search engine.
* @param context context or {@code null} for none
*/
public Search setContext(java.util.Map context) {
this.context = context;
return this;
}
/**
* The current set of custom search results.
* @return value or {@code null} for none
*/
public java.util.List getItems() {
return items;
}
/**
* The current set of custom search results.
* @param items items or {@code null} for none
*/
public Search setItems(java.util.List items) {
this.items = items;
return this;
}
/**
* Unique identifier for the type of current object. For this API, it is customsearch#search.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Unique identifier for the type of current object. For this API, it is customsearch#search.
* @param kind kind or {@code null} for none
*/
public Search setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The set of promotions. Present only if the custom search engine's configuration files define
* any promotions for the given query.
* @return value or {@code null} for none
*/
public java.util.List getPromotions() {
return promotions;
}
/**
* The set of promotions. Present only if the custom search engine's configuration files define
* any promotions for the given query.
* @param promotions promotions or {@code null} for none
*/
public Search setPromotions(java.util.List promotions) {
this.promotions = promotions;
return this;
}
/**
* Query metadata for the previous, current, and next pages of results.
* @return value or {@code null} for none
*/
public Queries getQueries() {
return queries;
}
/**
* Query metadata for the previous, current, and next pages of results.
* @param queries queries or {@code null} for none
*/
public Search setQueries(Queries queries) {
this.queries = queries;
return this;
}
/**
* Metadata about a search operation.
* @return value or {@code null} for none
*/
public SearchInformation getSearchInformation() {
return searchInformation;
}
/**
* Metadata about a search operation.
* @param searchInformation searchInformation or {@code null} for none
*/
public Search setSearchInformation(SearchInformation searchInformation) {
this.searchInformation = searchInformation;
return this;
}
/**
* Spell correction information for a query.
* @return value or {@code null} for none
*/
public Spelling getSpelling() {
return spelling;
}
/**
* Spell correction information for a query.
* @param spelling spelling or {@code null} for none
*/
public Search setSpelling(Spelling spelling) {
this.spelling = spelling;
return this;
}
/**
* OpenSearch template and URL.
* @return value or {@code null} for none
*/
public Url getUrl() {
return url;
}
/**
* OpenSearch template and URL.
* @param url url or {@code null} for none
*/
public Search setUrl(Url url) {
this.url = url;
return this;
}
@Override
public Search set(String fieldName, Object value) {
return (Search) super.set(fieldName, value);
}
@Override
public Search clone() {
return (Search) super.clone();
}
/**
* Query metadata for the previous, current, and next pages of results.
*/
public static final class Queries extends com.google.api.client.json.GenericJson {
/**
* Metadata representing the next page of results, if applicable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List nextPage;
static {
// hack to force ProGuard to consider NextPage used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(NextPage.class);
}
/**
* Metadata representing the previous page of results, if applicable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List previousPage;
static {
// hack to force ProGuard to consider PreviousPage used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(PreviousPage.class);
}
/**
* Metadata representing the current request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List request;
static {
// hack to force ProGuard to consider Request used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Request.class);
}
/**
* Metadata representing the next page of results, if applicable.
* @return value or {@code null} for none
*/
public java.util.List getNextPage() {
return nextPage;
}
/**
* Metadata representing the next page of results, if applicable.
* @param nextPage nextPage or {@code null} for none
*/
public Queries setNextPage(java.util.List nextPage) {
this.nextPage = nextPage;
return this;
}
/**
* Metadata representing the previous page of results, if applicable.
* @return value or {@code null} for none
*/
public java.util.List getPreviousPage() {
return previousPage;
}
/**
* Metadata representing the previous page of results, if applicable.
* @param previousPage previousPage or {@code null} for none
*/
public Queries setPreviousPage(java.util.List previousPage) {
this.previousPage = previousPage;
return this;
}
/**
* Metadata representing the current request.
* @return value or {@code null} for none
*/
public java.util.List getRequest() {
return request;
}
/**
* Metadata representing the current request.
* @param request request or {@code null} for none
*/
public Queries setRequest(java.util.List request) {
this.request = request;
return this;
}
@Override
public Queries set(String fieldName, Object value) {
return (Queries) super.set(fieldName, value);
}
@Override
public Queries clone() {
return (Queries) super.clone();
}
/**
* Custom search request metadata.
*/
public static final class NextPage extends com.google.api.client.json.GenericJson {
/**
* Number of search results returned in this set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer count;
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cr;
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cx;
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dateRestrict;
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String disableCnTwTranslation;
/**
* Identifies a phrase that all documents in the search results must contain.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String exactTerms;
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String excludeTerms;
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fileType;
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gl;
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String googleHost;
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String highRange;
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hl;
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hq;
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgColorType;
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgDominantColor;
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgSize;
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgType;
/**
* The character encoding supported for search requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String inputEncoding;
/**
* The language of the search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String language;
/**
* Specifies that all results should contain a link to a specific URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String linkSite;
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lowRange;
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orTerms;
/**
* The character encoding supported for search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String outputEncoding;
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String relatedSite;
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rights;
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String safe;
/**
* The search terms entered by the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String searchTerms;
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String searchType;
/**
* Restricts results to URLs from a specified site.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String siteSearch;
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String siteSearchFilter;
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sort;
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startIndex;
/**
* The page number of this set of results, where the page length is set by the count property.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startPage;
/**
* A description of the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Estimated number of total search results. May not be accurate.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalResults;
/**
* Number of search results returned in this set.
* @return value or {@code null} for none
*/
public java.lang.Integer getCount() {
return count;
}
/**
* Number of search results returned in this set.
* @param count count or {@code null} for none
*/
public NextPage setCount(java.lang.Integer count) {
this.count = count;
return this;
}
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* @return value or {@code null} for none
*/
public java.lang.String getCr() {
return cr;
}
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* @param cr cr or {@code null} for none
*/
public NextPage setCr(java.lang.String cr) {
this.cr = cr;
return this;
}
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* @return value or {@code null} for none
*/
public java.lang.String getCx() {
return cx;
}
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* @param cx cx or {@code null} for none
*/
public NextPage setCx(java.lang.String cx) {
this.cx = cx;
return this;
}
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* @return value or {@code null} for none
*/
public java.lang.String getDateRestrict() {
return dateRestrict;
}
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* @param dateRestrict dateRestrict or {@code null} for none
*/
public NextPage setDateRestrict(java.lang.String dateRestrict) {
this.dateRestrict = dateRestrict;
return this;
}
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* @return value or {@code null} for none
*/
public java.lang.String getDisableCnTwTranslation() {
return disableCnTwTranslation;
}
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* @param disableCnTwTranslation disableCnTwTranslation or {@code null} for none
*/
public NextPage setDisableCnTwTranslation(java.lang.String disableCnTwTranslation) {
this.disableCnTwTranslation = disableCnTwTranslation;
return this;
}
/**
* Identifies a phrase that all documents in the search results must contain.
* @return value or {@code null} for none
*/
public java.lang.String getExactTerms() {
return exactTerms;
}
/**
* Identifies a phrase that all documents in the search results must contain.
* @param exactTerms exactTerms or {@code null} for none
*/
public NextPage setExactTerms(java.lang.String exactTerms) {
this.exactTerms = exactTerms;
return this;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* @return value or {@code null} for none
*/
public java.lang.String getExcludeTerms() {
return excludeTerms;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* @param excludeTerms excludeTerms or {@code null} for none
*/
public NextPage setExcludeTerms(java.lang.String excludeTerms) {
this.excludeTerms = excludeTerms;
return this;
}
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* @return value or {@code null} for none
*/
public java.lang.String getFileType() {
return fileType;
}
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* @param fileType fileType or {@code null} for none
*/
public NextPage setFileType(java.lang.String fileType) {
this.fileType = fileType;
return this;
}
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* @return value or {@code null} for none
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* @param filter filter or {@code null} for none
*/
public NextPage setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* @return value or {@code null} for none
*/
public java.lang.String getGl() {
return gl;
}
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* @param gl gl or {@code null} for none
*/
public NextPage setGl(java.lang.String gl) {
this.gl = gl;
return this;
}
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* @return value or {@code null} for none
*/
public java.lang.String getGoogleHost() {
return googleHost;
}
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* @param googleHost googleHost or {@code null} for none
*/
public NextPage setGoogleHost(java.lang.String googleHost) {
this.googleHost = googleHost;
return this;
}
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* @return value or {@code null} for none
*/
public java.lang.String getHighRange() {
return highRange;
}
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* @param highRange highRange or {@code null} for none
*/
public NextPage setHighRange(java.lang.String highRange) {
this.highRange = highRange;
return this;
}
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* @return value or {@code null} for none
*/
public java.lang.String getHl() {
return hl;
}
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* @param hl hl or {@code null} for none
*/
public NextPage setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* @return value or {@code null} for none
*/
public java.lang.String getHq() {
return hq;
}
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* @param hq hq or {@code null} for none
*/
public NextPage setHq(java.lang.String hq) {
this.hq = hq;
return this;
}
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* @return value or {@code null} for none
*/
public java.lang.String getImgColorType() {
return imgColorType;
}
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* @param imgColorType imgColorType or {@code null} for none
*/
public NextPage setImgColorType(java.lang.String imgColorType) {
this.imgColorType = imgColorType;
return this;
}
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* @return value or {@code null} for none
*/
public java.lang.String getImgDominantColor() {
return imgDominantColor;
}
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* @param imgDominantColor imgDominantColor or {@code null} for none
*/
public NextPage setImgDominantColor(java.lang.String imgDominantColor) {
this.imgDominantColor = imgDominantColor;
return this;
}
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* @return value or {@code null} for none
*/
public java.lang.String getImgSize() {
return imgSize;
}
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* @param imgSize imgSize or {@code null} for none
*/
public NextPage setImgSize(java.lang.String imgSize) {
this.imgSize = imgSize;
return this;
}
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* @return value or {@code null} for none
*/
public java.lang.String getImgType() {
return imgType;
}
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* @param imgType imgType or {@code null} for none
*/
public NextPage setImgType(java.lang.String imgType) {
this.imgType = imgType;
return this;
}
/**
* The character encoding supported for search requests.
* @return value or {@code null} for none
*/
public java.lang.String getInputEncoding() {
return inputEncoding;
}
/**
* The character encoding supported for search requests.
* @param inputEncoding inputEncoding or {@code null} for none
*/
public NextPage setInputEncoding(java.lang.String inputEncoding) {
this.inputEncoding = inputEncoding;
return this;
}
/**
* The language of the search results.
* @return value or {@code null} for none
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language of the search results.
* @param language language or {@code null} for none
*/
public NextPage setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* Specifies that all results should contain a link to a specific URL.
* @return value or {@code null} for none
*/
public java.lang.String getLinkSite() {
return linkSite;
}
/**
* Specifies that all results should contain a link to a specific URL.
* @param linkSite linkSite or {@code null} for none
*/
public NextPage setLinkSite(java.lang.String linkSite) {
this.linkSite = linkSite;
return this;
}
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* @return value or {@code null} for none
*/
public java.lang.String getLowRange() {
return lowRange;
}
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* @param lowRange lowRange or {@code null} for none
*/
public NextPage setLowRange(java.lang.String lowRange) {
this.lowRange = lowRange;
return this;
}
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* @return value or {@code null} for none
*/
public java.lang.String getOrTerms() {
return orTerms;
}
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* @param orTerms orTerms or {@code null} for none
*/
public NextPage setOrTerms(java.lang.String orTerms) {
this.orTerms = orTerms;
return this;
}
/**
* The character encoding supported for search results.
* @return value or {@code null} for none
*/
public java.lang.String getOutputEncoding() {
return outputEncoding;
}
/**
* The character encoding supported for search results.
* @param outputEncoding outputEncoding or {@code null} for none
*/
public NextPage setOutputEncoding(java.lang.String outputEncoding) {
this.outputEncoding = outputEncoding;
return this;
}
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* @return value or {@code null} for none
*/
public java.lang.String getRelatedSite() {
return relatedSite;
}
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* @param relatedSite relatedSite or {@code null} for none
*/
public NextPage setRelatedSite(java.lang.String relatedSite) {
this.relatedSite = relatedSite;
return this;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* @return value or {@code null} for none
*/
public java.lang.String getRights() {
return rights;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* @param rights rights or {@code null} for none
*/
public NextPage setRights(java.lang.String rights) {
this.rights = rights;
return this;
}
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* @return value or {@code null} for none
*/
public java.lang.String getSafe() {
return safe;
}
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* @param safe safe or {@code null} for none
*/
public NextPage setSafe(java.lang.String safe) {
this.safe = safe;
return this;
}
/**
* The search terms entered by the user.
* @return value or {@code null} for none
*/
public java.lang.String getSearchTerms() {
return searchTerms;
}
/**
* The search terms entered by the user.
* @param searchTerms searchTerms or {@code null} for none
*/
public NextPage setSearchTerms(java.lang.String searchTerms) {
this.searchTerms = searchTerms;
return this;
}
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* @return value or {@code null} for none
*/
public java.lang.String getSearchType() {
return searchType;
}
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* @param searchType searchType or {@code null} for none
*/
public NextPage setSearchType(java.lang.String searchType) {
this.searchType = searchType;
return this;
}
/**
* Restricts results to URLs from a specified site.
* @return value or {@code null} for none
*/
public java.lang.String getSiteSearch() {
return siteSearch;
}
/**
* Restricts results to URLs from a specified site.
* @param siteSearch siteSearch or {@code null} for none
*/
public NextPage setSiteSearch(java.lang.String siteSearch) {
this.siteSearch = siteSearch;
return this;
}
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* @return value or {@code null} for none
*/
public java.lang.String getSiteSearchFilter() {
return siteSearchFilter;
}
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* @param siteSearchFilter siteSearchFilter or {@code null} for none
*/
public NextPage setSiteSearchFilter(java.lang.String siteSearchFilter) {
this.siteSearchFilter = siteSearchFilter;
return this;
}
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* @return value or {@code null} for none
*/
public java.lang.String getSort() {
return sort;
}
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* @param sort sort or {@code null} for none
*/
public NextPage setSort(java.lang.String sort) {
this.sort = sort;
return this;
}
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartIndex() {
return startIndex;
}
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* @param startIndex startIndex or {@code null} for none
*/
public NextPage setStartIndex(java.lang.Integer startIndex) {
this.startIndex = startIndex;
return this;
}
/**
* The page number of this set of results, where the page length is set by the count property.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartPage() {
return startPage;
}
/**
* The page number of this set of results, where the page length is set by the count property.
* @param startPage startPage or {@code null} for none
*/
public NextPage setStartPage(java.lang.Integer startPage) {
this.startPage = startPage;
return this;
}
/**
* A description of the query.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* A description of the query.
* @param title title or {@code null} for none
*/
public NextPage setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* Estimated number of total search results. May not be accurate.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalResults() {
return totalResults;
}
/**
* Estimated number of total search results. May not be accurate.
* @param totalResults totalResults or {@code null} for none
*/
public NextPage setTotalResults(java.lang.Long totalResults) {
this.totalResults = totalResults;
return this;
}
@Override
public NextPage set(String fieldName, Object value) {
return (NextPage) super.set(fieldName, value);
}
@Override
public NextPage clone() {
return (NextPage) super.clone();
}
}
/**
* Custom search request metadata.
*/
public static final class PreviousPage extends com.google.api.client.json.GenericJson {
/**
* Number of search results returned in this set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer count;
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cr;
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cx;
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dateRestrict;
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String disableCnTwTranslation;
/**
* Identifies a phrase that all documents in the search results must contain.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String exactTerms;
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String excludeTerms;
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fileType;
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gl;
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String googleHost;
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String highRange;
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hl;
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hq;
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgColorType;
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgDominantColor;
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgSize;
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgType;
/**
* The character encoding supported for search requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String inputEncoding;
/**
* The language of the search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String language;
/**
* Specifies that all results should contain a link to a specific URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String linkSite;
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lowRange;
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orTerms;
/**
* The character encoding supported for search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String outputEncoding;
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String relatedSite;
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rights;
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String safe;
/**
* The search terms entered by the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String searchTerms;
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String searchType;
/**
* Restricts results to URLs from a specified site.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String siteSearch;
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String siteSearchFilter;
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sort;
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startIndex;
/**
* The page number of this set of results, where the page length is set by the count property.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startPage;
/**
* A description of the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Estimated number of total search results. May not be accurate.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalResults;
/**
* Number of search results returned in this set.
* @return value or {@code null} for none
*/
public java.lang.Integer getCount() {
return count;
}
/**
* Number of search results returned in this set.
* @param count count or {@code null} for none
*/
public PreviousPage setCount(java.lang.Integer count) {
this.count = count;
return this;
}
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* @return value or {@code null} for none
*/
public java.lang.String getCr() {
return cr;
}
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* @param cr cr or {@code null} for none
*/
public PreviousPage setCr(java.lang.String cr) {
this.cr = cr;
return this;
}
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* @return value or {@code null} for none
*/
public java.lang.String getCx() {
return cx;
}
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* @param cx cx or {@code null} for none
*/
public PreviousPage setCx(java.lang.String cx) {
this.cx = cx;
return this;
}
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* @return value or {@code null} for none
*/
public java.lang.String getDateRestrict() {
return dateRestrict;
}
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* @param dateRestrict dateRestrict or {@code null} for none
*/
public PreviousPage setDateRestrict(java.lang.String dateRestrict) {
this.dateRestrict = dateRestrict;
return this;
}
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* @return value or {@code null} for none
*/
public java.lang.String getDisableCnTwTranslation() {
return disableCnTwTranslation;
}
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* @param disableCnTwTranslation disableCnTwTranslation or {@code null} for none
*/
public PreviousPage setDisableCnTwTranslation(java.lang.String disableCnTwTranslation) {
this.disableCnTwTranslation = disableCnTwTranslation;
return this;
}
/**
* Identifies a phrase that all documents in the search results must contain.
* @return value or {@code null} for none
*/
public java.lang.String getExactTerms() {
return exactTerms;
}
/**
* Identifies a phrase that all documents in the search results must contain.
* @param exactTerms exactTerms or {@code null} for none
*/
public PreviousPage setExactTerms(java.lang.String exactTerms) {
this.exactTerms = exactTerms;
return this;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* @return value or {@code null} for none
*/
public java.lang.String getExcludeTerms() {
return excludeTerms;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* @param excludeTerms excludeTerms or {@code null} for none
*/
public PreviousPage setExcludeTerms(java.lang.String excludeTerms) {
this.excludeTerms = excludeTerms;
return this;
}
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* @return value or {@code null} for none
*/
public java.lang.String getFileType() {
return fileType;
}
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* @param fileType fileType or {@code null} for none
*/
public PreviousPage setFileType(java.lang.String fileType) {
this.fileType = fileType;
return this;
}
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* @return value or {@code null} for none
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* @param filter filter or {@code null} for none
*/
public PreviousPage setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* @return value or {@code null} for none
*/
public java.lang.String getGl() {
return gl;
}
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* @param gl gl or {@code null} for none
*/
public PreviousPage setGl(java.lang.String gl) {
this.gl = gl;
return this;
}
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* @return value or {@code null} for none
*/
public java.lang.String getGoogleHost() {
return googleHost;
}
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* @param googleHost googleHost or {@code null} for none
*/
public PreviousPage setGoogleHost(java.lang.String googleHost) {
this.googleHost = googleHost;
return this;
}
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* @return value or {@code null} for none
*/
public java.lang.String getHighRange() {
return highRange;
}
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* @param highRange highRange or {@code null} for none
*/
public PreviousPage setHighRange(java.lang.String highRange) {
this.highRange = highRange;
return this;
}
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* @return value or {@code null} for none
*/
public java.lang.String getHl() {
return hl;
}
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* @param hl hl or {@code null} for none
*/
public PreviousPage setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* @return value or {@code null} for none
*/
public java.lang.String getHq() {
return hq;
}
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* @param hq hq or {@code null} for none
*/
public PreviousPage setHq(java.lang.String hq) {
this.hq = hq;
return this;
}
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* @return value or {@code null} for none
*/
public java.lang.String getImgColorType() {
return imgColorType;
}
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* @param imgColorType imgColorType or {@code null} for none
*/
public PreviousPage setImgColorType(java.lang.String imgColorType) {
this.imgColorType = imgColorType;
return this;
}
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* @return value or {@code null} for none
*/
public java.lang.String getImgDominantColor() {
return imgDominantColor;
}
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* @param imgDominantColor imgDominantColor or {@code null} for none
*/
public PreviousPage setImgDominantColor(java.lang.String imgDominantColor) {
this.imgDominantColor = imgDominantColor;
return this;
}
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* @return value or {@code null} for none
*/
public java.lang.String getImgSize() {
return imgSize;
}
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* @param imgSize imgSize or {@code null} for none
*/
public PreviousPage setImgSize(java.lang.String imgSize) {
this.imgSize = imgSize;
return this;
}
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* @return value or {@code null} for none
*/
public java.lang.String getImgType() {
return imgType;
}
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* @param imgType imgType or {@code null} for none
*/
public PreviousPage setImgType(java.lang.String imgType) {
this.imgType = imgType;
return this;
}
/**
* The character encoding supported for search requests.
* @return value or {@code null} for none
*/
public java.lang.String getInputEncoding() {
return inputEncoding;
}
/**
* The character encoding supported for search requests.
* @param inputEncoding inputEncoding or {@code null} for none
*/
public PreviousPage setInputEncoding(java.lang.String inputEncoding) {
this.inputEncoding = inputEncoding;
return this;
}
/**
* The language of the search results.
* @return value or {@code null} for none
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language of the search results.
* @param language language or {@code null} for none
*/
public PreviousPage setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* Specifies that all results should contain a link to a specific URL.
* @return value or {@code null} for none
*/
public java.lang.String getLinkSite() {
return linkSite;
}
/**
* Specifies that all results should contain a link to a specific URL.
* @param linkSite linkSite or {@code null} for none
*/
public PreviousPage setLinkSite(java.lang.String linkSite) {
this.linkSite = linkSite;
return this;
}
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* @return value or {@code null} for none
*/
public java.lang.String getLowRange() {
return lowRange;
}
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* @param lowRange lowRange or {@code null} for none
*/
public PreviousPage setLowRange(java.lang.String lowRange) {
this.lowRange = lowRange;
return this;
}
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* @return value or {@code null} for none
*/
public java.lang.String getOrTerms() {
return orTerms;
}
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* @param orTerms orTerms or {@code null} for none
*/
public PreviousPage setOrTerms(java.lang.String orTerms) {
this.orTerms = orTerms;
return this;
}
/**
* The character encoding supported for search results.
* @return value or {@code null} for none
*/
public java.lang.String getOutputEncoding() {
return outputEncoding;
}
/**
* The character encoding supported for search results.
* @param outputEncoding outputEncoding or {@code null} for none
*/
public PreviousPage setOutputEncoding(java.lang.String outputEncoding) {
this.outputEncoding = outputEncoding;
return this;
}
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* @return value or {@code null} for none
*/
public java.lang.String getRelatedSite() {
return relatedSite;
}
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* @param relatedSite relatedSite or {@code null} for none
*/
public PreviousPage setRelatedSite(java.lang.String relatedSite) {
this.relatedSite = relatedSite;
return this;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* @return value or {@code null} for none
*/
public java.lang.String getRights() {
return rights;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* @param rights rights or {@code null} for none
*/
public PreviousPage setRights(java.lang.String rights) {
this.rights = rights;
return this;
}
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* @return value or {@code null} for none
*/
public java.lang.String getSafe() {
return safe;
}
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* @param safe safe or {@code null} for none
*/
public PreviousPage setSafe(java.lang.String safe) {
this.safe = safe;
return this;
}
/**
* The search terms entered by the user.
* @return value or {@code null} for none
*/
public java.lang.String getSearchTerms() {
return searchTerms;
}
/**
* The search terms entered by the user.
* @param searchTerms searchTerms or {@code null} for none
*/
public PreviousPage setSearchTerms(java.lang.String searchTerms) {
this.searchTerms = searchTerms;
return this;
}
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* @return value or {@code null} for none
*/
public java.lang.String getSearchType() {
return searchType;
}
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* @param searchType searchType or {@code null} for none
*/
public PreviousPage setSearchType(java.lang.String searchType) {
this.searchType = searchType;
return this;
}
/**
* Restricts results to URLs from a specified site.
* @return value or {@code null} for none
*/
public java.lang.String getSiteSearch() {
return siteSearch;
}
/**
* Restricts results to URLs from a specified site.
* @param siteSearch siteSearch or {@code null} for none
*/
public PreviousPage setSiteSearch(java.lang.String siteSearch) {
this.siteSearch = siteSearch;
return this;
}
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* @return value or {@code null} for none
*/
public java.lang.String getSiteSearchFilter() {
return siteSearchFilter;
}
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* @param siteSearchFilter siteSearchFilter or {@code null} for none
*/
public PreviousPage setSiteSearchFilter(java.lang.String siteSearchFilter) {
this.siteSearchFilter = siteSearchFilter;
return this;
}
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* @return value or {@code null} for none
*/
public java.lang.String getSort() {
return sort;
}
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* @param sort sort or {@code null} for none
*/
public PreviousPage setSort(java.lang.String sort) {
this.sort = sort;
return this;
}
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartIndex() {
return startIndex;
}
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* @param startIndex startIndex or {@code null} for none
*/
public PreviousPage setStartIndex(java.lang.Integer startIndex) {
this.startIndex = startIndex;
return this;
}
/**
* The page number of this set of results, where the page length is set by the count property.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartPage() {
return startPage;
}
/**
* The page number of this set of results, where the page length is set by the count property.
* @param startPage startPage or {@code null} for none
*/
public PreviousPage setStartPage(java.lang.Integer startPage) {
this.startPage = startPage;
return this;
}
/**
* A description of the query.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* A description of the query.
* @param title title or {@code null} for none
*/
public PreviousPage setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* Estimated number of total search results. May not be accurate.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalResults() {
return totalResults;
}
/**
* Estimated number of total search results. May not be accurate.
* @param totalResults totalResults or {@code null} for none
*/
public PreviousPage setTotalResults(java.lang.Long totalResults) {
this.totalResults = totalResults;
return this;
}
@Override
public PreviousPage set(String fieldName, Object value) {
return (PreviousPage) super.set(fieldName, value);
}
@Override
public PreviousPage clone() {
return (PreviousPage) super.clone();
}
}
/**
* Custom search request metadata.
*/
public static final class Request extends com.google.api.client.json.GenericJson {
/**
* Number of search results returned in this set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer count;
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cr;
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cx;
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dateRestrict;
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String disableCnTwTranslation;
/**
* Identifies a phrase that all documents in the search results must contain.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String exactTerms;
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String excludeTerms;
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fileType;
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gl;
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String googleHost;
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String highRange;
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hl;
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hq;
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgColorType;
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgDominantColor;
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgSize;
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imgType;
/**
* The character encoding supported for search requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String inputEncoding;
/**
* The language of the search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String language;
/**
* Specifies that all results should contain a link to a specific URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String linkSite;
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lowRange;
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orTerms;
/**
* The character encoding supported for search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String outputEncoding;
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String relatedSite;
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rights;
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String safe;
/**
* The search terms entered by the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String searchTerms;
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String searchType;
/**
* Restricts results to URLs from a specified site.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String siteSearch;
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String siteSearchFilter;
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sort;
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startIndex;
/**
* The page number of this set of results, where the page length is set by the count property.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startPage;
/**
* A description of the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Estimated number of total search results. May not be accurate.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalResults;
/**
* Number of search results returned in this set.
* @return value or {@code null} for none
*/
public java.lang.Integer getCount() {
return count;
}
/**
* Number of search results returned in this set.
* @param count count or {@code null} for none
*/
public Request setCount(java.lang.Integer count) {
this.count = count;
return this;
}
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* @return value or {@code null} for none
*/
public java.lang.String getCr() {
return cr;
}
/**
* Restricts search results to documents originating in a particular country. You may use Boolean
* operators in the cr parameter's value.
* @param cr cr or {@code null} for none
*/
public Request setCr(java.lang.String cr) {
this.cr = cr;
return this;
}
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* @return value or {@code null} for none
*/
public java.lang.String getCx() {
return cx;
}
/**
* The identifier of a custom search engine created using the Custom Search Control Panel, if
* specified in request. This is a custom property not defined in the OpenSearch spec.
* @param cx cx or {@code null} for none
*/
public Request setCx(java.lang.String cx) {
this.cx = cx;
return this;
}
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* @return value or {@code null} for none
*/
public java.lang.String getDateRestrict() {
return dateRestrict;
}
/**
* Restricts results to URLs based on date. Supported values include: d[number]: requests results
* from the specified number of past days. w[number]: requests results from the specified number
* of past weeks. m[number]: requests results from the specified number of past months. y[number]:
* requests results from the specified number of past years.
* @param dateRestrict dateRestrict or {@code null} for none
*/
public Request setDateRestrict(java.lang.String dateRestrict) {
this.dateRestrict = dateRestrict;
return this;
}
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* @return value or {@code null} for none
*/
public java.lang.String getDisableCnTwTranslation() {
return disableCnTwTranslation;
}
/**
* Enables or disables the Simplified and Traditional Chinese Search feature. Supported values
* are: 0: enabled (default) 1: disabled
* @param disableCnTwTranslation disableCnTwTranslation or {@code null} for none
*/
public Request setDisableCnTwTranslation(java.lang.String disableCnTwTranslation) {
this.disableCnTwTranslation = disableCnTwTranslation;
return this;
}
/**
* Identifies a phrase that all documents in the search results must contain.
* @return value or {@code null} for none
*/
public java.lang.String getExactTerms() {
return exactTerms;
}
/**
* Identifies a phrase that all documents in the search results must contain.
* @param exactTerms exactTerms or {@code null} for none
*/
public Request setExactTerms(java.lang.String exactTerms) {
this.exactTerms = exactTerms;
return this;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* @return value or {@code null} for none
*/
public java.lang.String getExcludeTerms() {
return excludeTerms;
}
/**
* Identifies a word or phrase that should not appear in any documents in the search results.
* @param excludeTerms excludeTerms or {@code null} for none
*/
public Request setExcludeTerms(java.lang.String excludeTerms) {
this.excludeTerms = excludeTerms;
return this;
}
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* @return value or {@code null} for none
*/
public java.lang.String getFileType() {
return fileType;
}
/**
* Restricts results to files of a specified extension. Filetypes supported by Google include:
* Adobe Portable Document Format (pdf) Adobe PostScript (ps) Lotus 1-2-3 (wk1, wk2, wk3, wk4,
* wk5, wki, wks, wku) Lotus WordPro (lwp) Macwrite (mw) Microsoft Excel (xls) Microsoft
* PowerPoint (ppt) Microsoft Word (doc) Microsoft Works (wks, wps, wdb) Microsoft Write (wri)
* Rich Text Format (rtf) Shockwave Flash (swf) Text (ans, txt).
* @param fileType fileType or {@code null} for none
*/
public Request setFileType(java.lang.String fileType) {
this.fileType = fileType;
return this;
}
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* @return value or {@code null} for none
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Activates or deactivates the automatic filtering of Google search results. The default value
* for the filter parameter is 1, which indicates that the feature is enabled. Valid values for
* this parameter are: 0: Disabled 1: Enabled
* @param filter filter or {@code null} for none
*/
public Request setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* @return value or {@code null} for none
*/
public java.lang.String getGl() {
return gl;
}
/**
* Boosts search results whose country of origin matches the parameter value. Specifying a gl
* parameter value in WebSearch requests should improve the relevance of results. This is
* particularly true for international customers and, even more specifically, for customers in
* English-speaking countries other than the United States.
* @param gl gl or {@code null} for none
*/
public Request setGl(java.lang.String gl) {
this.gl = gl;
return this;
}
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* @return value or {@code null} for none
*/
public java.lang.String getGoogleHost() {
return googleHost;
}
/**
* Specifies the Google domain (for example, google.com, google.de, or google.fr) to which the
* search should be limited.
* @param googleHost googleHost or {@code null} for none
*/
public Request setGoogleHost(java.lang.String googleHost) {
this.googleHost = googleHost;
return this;
}
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* @return value or {@code null} for none
*/
public java.lang.String getHighRange() {
return highRange;
}
/**
* Specifies the ending value for a search range. Use cse:lowRange and cse:highrange to append an
* inclusive search range of lowRange...highRange to the query.
* @param highRange highRange or {@code null} for none
*/
public Request setHighRange(java.lang.String highRange) {
this.highRange = highRange;
return this;
}
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* @return value or {@code null} for none
*/
public java.lang.String getHl() {
return hl;
}
/**
* Specifies the interface language (host language) of your user interface. Explicitly setting
* this parameter improves the performance and the quality of your search results.
* @param hl hl or {@code null} for none
*/
public Request setHl(java.lang.String hl) {
this.hl = hl;
return this;
}
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* @return value or {@code null} for none
*/
public java.lang.String getHq() {
return hq;
}
/**
* Appends the specified query terms to the query, as if they were combined with a logical AND
* operator.
* @param hq hq or {@code null} for none
*/
public Request setHq(java.lang.String hq) {
this.hq = hq;
return this;
}
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* @return value or {@code null} for none
*/
public java.lang.String getImgColorType() {
return imgColorType;
}
/**
* Restricts results to images of a specified color type. Supported values are: mono (black and
* white) gray (grayscale) color (color)
* @param imgColorType imgColorType or {@code null} for none
*/
public Request setImgColorType(java.lang.String imgColorType) {
this.imgColorType = imgColorType;
return this;
}
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* @return value or {@code null} for none
*/
public java.lang.String getImgDominantColor() {
return imgDominantColor;
}
/**
* Restricts results to images with a specific dominant color. Supported values are: red orange
* yellow green teal blue purple pink white gray black brown
* @param imgDominantColor imgDominantColor or {@code null} for none
*/
public Request setImgDominantColor(java.lang.String imgDominantColor) {
this.imgDominantColor = imgDominantColor;
return this;
}
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* @return value or {@code null} for none
*/
public java.lang.String getImgSize() {
return imgSize;
}
/**
* Restricts results to images of a specified size. Supported values are: icon (small)
* small|medium|large|xlarge (medium) xxlarge (large) huge (extra-large)
* @param imgSize imgSize or {@code null} for none
*/
public Request setImgSize(java.lang.String imgSize) {
this.imgSize = imgSize;
return this;
}
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* @return value or {@code null} for none
*/
public java.lang.String getImgType() {
return imgType;
}
/**
* Restricts results to images of a specified type. Supported values are: clipart (Clip art) face
* (Face) lineart (Line drawing) photo (Photo) animated (Animated) stock (Stock)
* @param imgType imgType or {@code null} for none
*/
public Request setImgType(java.lang.String imgType) {
this.imgType = imgType;
return this;
}
/**
* The character encoding supported for search requests.
* @return value or {@code null} for none
*/
public java.lang.String getInputEncoding() {
return inputEncoding;
}
/**
* The character encoding supported for search requests.
* @param inputEncoding inputEncoding or {@code null} for none
*/
public Request setInputEncoding(java.lang.String inputEncoding) {
this.inputEncoding = inputEncoding;
return this;
}
/**
* The language of the search results.
* @return value or {@code null} for none
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language of the search results.
* @param language language or {@code null} for none
*/
public Request setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* Specifies that all results should contain a link to a specific URL.
* @return value or {@code null} for none
*/
public java.lang.String getLinkSite() {
return linkSite;
}
/**
* Specifies that all results should contain a link to a specific URL.
* @param linkSite linkSite or {@code null} for none
*/
public Request setLinkSite(java.lang.String linkSite) {
this.linkSite = linkSite;
return this;
}
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* @return value or {@code null} for none
*/
public java.lang.String getLowRange() {
return lowRange;
}
/**
* Specifies the starting value for a search range. Use cse:lowRange and cse:highrange to append
* an inclusive search range of lowRange...highRange to the query.
* @param lowRange lowRange or {@code null} for none
*/
public Request setLowRange(java.lang.String lowRange) {
this.lowRange = lowRange;
return this;
}
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* @return value or {@code null} for none
*/
public java.lang.String getOrTerms() {
return orTerms;
}
/**
* Provides additional search terms to check for in a document, where each document in the search
* results must contain at least one of the additional search terms. You can also use the Boolean
* OR query term for this type of query.
* @param orTerms orTerms or {@code null} for none
*/
public Request setOrTerms(java.lang.String orTerms) {
this.orTerms = orTerms;
return this;
}
/**
* The character encoding supported for search results.
* @return value or {@code null} for none
*/
public java.lang.String getOutputEncoding() {
return outputEncoding;
}
/**
* The character encoding supported for search results.
* @param outputEncoding outputEncoding or {@code null} for none
*/
public Request setOutputEncoding(java.lang.String outputEncoding) {
this.outputEncoding = outputEncoding;
return this;
}
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* @return value or {@code null} for none
*/
public java.lang.String getRelatedSite() {
return relatedSite;
}
/**
* Specifies that all search results should be pages that are related to the specified URL. The
* parameter value should be a URL.
* @param relatedSite relatedSite or {@code null} for none
*/
public Request setRelatedSite(java.lang.String relatedSite) {
this.relatedSite = relatedSite;
return this;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* @return value or {@code null} for none
*/
public java.lang.String getRights() {
return rights;
}
/**
* Filters based on licensing. Supported values include: cc_publicdomain cc_attribute
* cc_sharealike cc_noncommercial cc_nonderived
* @param rights rights or {@code null} for none
*/
public Request setRights(java.lang.String rights) {
this.rights = rights;
return this;
}
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* @return value or {@code null} for none
*/
public java.lang.String getSafe() {
return safe;
}
/**
* Specifies the SafeSearch level used for filtering out adult results. This is a custom property
* not defined in the OpenSearch spec. Valid parameter values are: off: Disable SafeSearch active:
* Enable SafeSearch
* @param safe safe or {@code null} for none
*/
public Request setSafe(java.lang.String safe) {
this.safe = safe;
return this;
}
/**
* The search terms entered by the user.
* @return value or {@code null} for none
*/
public java.lang.String getSearchTerms() {
return searchTerms;
}
/**
* The search terms entered by the user.
* @param searchTerms searchTerms or {@code null} for none
*/
public Request setSearchTerms(java.lang.String searchTerms) {
this.searchTerms = searchTerms;
return this;
}
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* @return value or {@code null} for none
*/
public java.lang.String getSearchType() {
return searchType;
}
/**
* Allowed values are web or image. If unspecified, results are limited to webpages.
* @param searchType searchType or {@code null} for none
*/
public Request setSearchType(java.lang.String searchType) {
this.searchType = searchType;
return this;
}
/**
* Restricts results to URLs from a specified site.
* @return value or {@code null} for none
*/
public java.lang.String getSiteSearch() {
return siteSearch;
}
/**
* Restricts results to URLs from a specified site.
* @param siteSearch siteSearch or {@code null} for none
*/
public Request setSiteSearch(java.lang.String siteSearch) {
this.siteSearch = siteSearch;
return this;
}
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* @return value or {@code null} for none
*/
public java.lang.String getSiteSearchFilter() {
return siteSearchFilter;
}
/**
* Specifies whether to include or exclude results from the site named in the sitesearch
* parameter. Supported values are: i: include content from site e: exclude content from site
* @param siteSearchFilter siteSearchFilter or {@code null} for none
*/
public Request setSiteSearchFilter(java.lang.String siteSearchFilter) {
this.siteSearchFilter = siteSearchFilter;
return this;
}
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* @return value or {@code null} for none
*/
public java.lang.String getSort() {
return sort;
}
/**
* Specifies that results should be sorted according to the specified expression. For example,
* sort by date.
* @param sort sort or {@code null} for none
*/
public Request setSort(java.lang.String sort) {
this.sort = sort;
return this;
}
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartIndex() {
return startIndex;
}
/**
* The index of the current set of search results into the total set of results, where the index
* of the first result is 1.
* @param startIndex startIndex or {@code null} for none
*/
public Request setStartIndex(java.lang.Integer startIndex) {
this.startIndex = startIndex;
return this;
}
/**
* The page number of this set of results, where the page length is set by the count property.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartPage() {
return startPage;
}
/**
* The page number of this set of results, where the page length is set by the count property.
* @param startPage startPage or {@code null} for none
*/
public Request setStartPage(java.lang.Integer startPage) {
this.startPage = startPage;
return this;
}
/**
* A description of the query.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* A description of the query.
* @param title title or {@code null} for none
*/
public Request setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* Estimated number of total search results. May not be accurate.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalResults() {
return totalResults;
}
/**
* Estimated number of total search results. May not be accurate.
* @param totalResults totalResults or {@code null} for none
*/
public Request setTotalResults(java.lang.Long totalResults) {
this.totalResults = totalResults;
return this;
}
@Override
public Request set(String fieldName, Object value) {
return (Request) super.set(fieldName, value);
}
@Override
public Request clone() {
return (Request) super.clone();
}
}
}
/**
* Metadata about a search operation.
*/
public static final class SearchInformation extends com.google.api.client.json.GenericJson {
/**
* The time taken for the server to return search results, formatted according to locale style.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String formattedSearchTime;
/**
* The total number of search results, formatted according to locale style.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String formattedTotalResults;
/**
* The time taken for the server to return search results.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double searchTime;
/**
* The total number of search results returned by the query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String totalResults;
/**
* The time taken for the server to return search results, formatted according to locale style.
* @return value or {@code null} for none
*/
public java.lang.String getFormattedSearchTime() {
return formattedSearchTime;
}
/**
* The time taken for the server to return search results, formatted according to locale style.
* @param formattedSearchTime formattedSearchTime or {@code null} for none
*/
public SearchInformation setFormattedSearchTime(java.lang.String formattedSearchTime) {
this.formattedSearchTime = formattedSearchTime;
return this;
}
/**
* The total number of search results, formatted according to locale style.
* @return value or {@code null} for none
*/
public java.lang.String getFormattedTotalResults() {
return formattedTotalResults;
}
/**
* The total number of search results, formatted according to locale style.
* @param formattedTotalResults formattedTotalResults or {@code null} for none
*/
public SearchInformation setFormattedTotalResults(java.lang.String formattedTotalResults) {
this.formattedTotalResults = formattedTotalResults;
return this;
}
/**
* The time taken for the server to return search results.
* @return value or {@code null} for none
*/
public java.lang.Double getSearchTime() {
return searchTime;
}
/**
* The time taken for the server to return search results.
* @param searchTime searchTime or {@code null} for none
*/
public SearchInformation setSearchTime(java.lang.Double searchTime) {
this.searchTime = searchTime;
return this;
}
/**
* The total number of search results returned by the query.
* @return value or {@code null} for none
*/
public java.lang.String getTotalResults() {
return totalResults;
}
/**
* The total number of search results returned by the query.
* @param totalResults totalResults or {@code null} for none
*/
public SearchInformation setTotalResults(java.lang.String totalResults) {
this.totalResults = totalResults;
return this;
}
@Override
public SearchInformation set(String fieldName, Object value) {
return (SearchInformation) super.set(fieldName, value);
}
@Override
public SearchInformation clone() {
return (SearchInformation) super.clone();
}
}
/**
* Spell correction information for a query.
*/
public static final class Spelling extends com.google.api.client.json.GenericJson {
/**
* The corrected query.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String correctedQuery;
/**
* The corrected query, formatted in HTML.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String htmlCorrectedQuery;
/**
* The corrected query.
* @return value or {@code null} for none
*/
public java.lang.String getCorrectedQuery() {
return correctedQuery;
}
/**
* The corrected query.
* @param correctedQuery correctedQuery or {@code null} for none
*/
public Spelling setCorrectedQuery(java.lang.String correctedQuery) {
this.correctedQuery = correctedQuery;
return this;
}
/**
* The corrected query, formatted in HTML.
* @return value or {@code null} for none
*/
public java.lang.String getHtmlCorrectedQuery() {
return htmlCorrectedQuery;
}
/**
* The corrected query, formatted in HTML.
* @param htmlCorrectedQuery htmlCorrectedQuery or {@code null} for none
*/
public Spelling setHtmlCorrectedQuery(java.lang.String htmlCorrectedQuery) {
this.htmlCorrectedQuery = htmlCorrectedQuery;
return this;
}
@Override
public Spelling set(String fieldName, Object value) {
return (Spelling) super.set(fieldName, value);
}
@Override
public Spelling clone() {
return (Spelling) super.clone();
}
}
/**
* OpenSearch template and URL.
*/
public static final class Url extends com.google.api.client.json.GenericJson {
/**
* The actual OpenSearch template for this API.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String template;
/**
* The MIME type of the OpenSearch URL template for the Custom Search API.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The actual OpenSearch template for this API.
* @return value or {@code null} for none
*/
public java.lang.String getTemplate() {
return template;
}
/**
* The actual OpenSearch template for this API.
* @param template template or {@code null} for none
*/
public Url setTemplate(java.lang.String template) {
this.template = template;
return this;
}
/**
* The MIME type of the OpenSearch URL template for the Custom Search API.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The MIME type of the OpenSearch URL template for the Custom Search API.
* @param type type or {@code null} for none
*/
public Url setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public Url set(String fieldName, Object value) {
return (Url) super.set(fieldName, value);
}
@Override
public Url clone() {
return (Url) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy