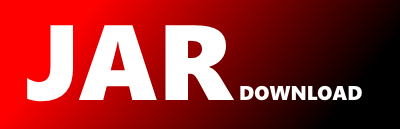
com.google.api.services.datacatalog.v1.DataCatalog Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datacatalog.v1;
/**
* Service definition for DataCatalog (v1).
*
*
* A fully managed and highly scalable data discovery and metadata management service.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link DataCatalogRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class DataCatalog extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Google Cloud Data Catalog API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://datacatalog.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://datacatalog.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public DataCatalog(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
DataCatalog(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Catalog collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Catalog.List request = datacatalog.catalog().list(parameters ...)}
*
*
* @return the resource collection
*/
public Catalog catalog() {
return new Catalog();
}
/**
* The "catalog" collection of methods.
*/
public class Catalog {
/**
* Searches Data Catalog for multiple resources like entries and tags that match a query. This is a
* [Custom Method] (https://cloud.google.com/apis/design/custom_methods) that doesn't return all
* information on a resource, only its ID and high level fields. To get more information, you can
* subsequently call specific get methods. Note: Data Catalog search queries don't guarantee full
* recall. Results that match your query might not be returned, even in subsequent result pages.
* Additionally, returned (and not returned) results can vary if you repeat search queries. For more
* information, see [Data Catalog search syntax] (https://cloud.google.com/data-catalog/docs/how-
* to/search-reference).
*
* Create a request for the method "catalog.search".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SearchCatalogRequest}
* @return the request
*/
public Search search(com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SearchCatalogRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends DataCatalogRequest {
private static final String REST_PATH = "v1/catalog:search";
/**
* Searches Data Catalog for multiple resources like entries and tags that match a query. This is
* a [Custom Method] (https://cloud.google.com/apis/design/custom_methods) that doesn't return all
* information on a resource, only its ID and high level fields. To get more information, you can
* subsequently call specific get methods. Note: Data Catalog search queries don't guarantee full
* recall. Results that match your query might not be returned, even in subsequent result pages.
* Additionally, returned (and not returned) results can vary if you repeat search queries. For
* more information, see [Data Catalog search syntax] (https://cloud.google.com/data-
* catalog/docs/how-to/search-reference).
*
* Create a request for the method "catalog.search".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SearchCatalogRequest}
* @since 1.13
*/
protected Search(com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SearchCatalogRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SearchCatalogResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Entries collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Entries.List request = datacatalog.entries().list(parameters ...)}
*
*
* @return the resource collection
*/
public Entries entries() {
return new Entries();
}
/**
* The "entries" collection of methods.
*/
public class Entries {
/**
* Gets an entry by its target resource name. The resource name comes from the source Google Cloud
* Platform service.
*
* Create a request for the method "entries.lookup".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Lookup lookup() throws java.io.IOException {
Lookup result = new Lookup();
initialize(result);
return result;
}
public class Lookup extends DataCatalogRequest {
private static final String REST_PATH = "v1/entries:lookup";
/**
* Gets an entry by its target resource name. The resource name comes from the source Google Cloud
* Platform service.
*
* Create a request for the method "entries.lookup".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Lookup#execute()} method to invoke the remote operation.
* {@link
* Lookup#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Lookup() {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Lookup set$Xgafv(java.lang.String $Xgafv) {
return (Lookup) super.set$Xgafv($Xgafv);
}
@Override
public Lookup setAccessToken(java.lang.String accessToken) {
return (Lookup) super.setAccessToken(accessToken);
}
@Override
public Lookup setAlt(java.lang.String alt) {
return (Lookup) super.setAlt(alt);
}
@Override
public Lookup setCallback(java.lang.String callback) {
return (Lookup) super.setCallback(callback);
}
@Override
public Lookup setFields(java.lang.String fields) {
return (Lookup) super.setFields(fields);
}
@Override
public Lookup setKey(java.lang.String key) {
return (Lookup) super.setKey(key);
}
@Override
public Lookup setOauthToken(java.lang.String oauthToken) {
return (Lookup) super.setOauthToken(oauthToken);
}
@Override
public Lookup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Lookup) super.setPrettyPrint(prettyPrint);
}
@Override
public Lookup setQuotaUser(java.lang.String quotaUser) {
return (Lookup) super.setQuotaUser(quotaUser);
}
@Override
public Lookup setUploadType(java.lang.String uploadType) {
return (Lookup) super.setUploadType(uploadType);
}
@Override
public Lookup setUploadProtocol(java.lang.String uploadProtocol) {
return (Lookup) super.setUploadProtocol(uploadProtocol);
}
/**
* [Fully Qualified Name (FQN)](https://cloud.google.com//data-catalog/docs/fully-qualified-
* names) of the resource. FQNs take two forms: * For non-regionalized resources:
* `{SYSTEM}:{PROJECT}.{PATH_TO_RESOURCE_SEPARATED_WITH_DOTS}` * For regionalized resources:
* `{SYSTEM}:{PROJECT}.{LOCATION_ID}.{PATH_TO_RESOURCE_SEPARATED_WITH_DOTS}` Example for a
* DPMS table:
* `dataproc_metastore:{PROJECT_ID}.{LOCATION_ID}.{INSTANCE_ID}.{DATABASE_ID}.{TABLE_ID}`
*/
@com.google.api.client.util.Key
private java.lang.String fullyQualifiedName;
/**[ Fully Qualified Name (FQN)](https://cloud.google.com//data-catalog/docs/fully-qualified-names) of
[ the resource. FQNs take two forms: * For non-regionalized resources:
[ `{SYSTEM}:{PROJECT}.{PATH_TO_RESOURCE_SEPARATED_WITH_DOTS}` * For regionalized resources:
[ `{SYSTEM}:{PROJECT}.{LOCATION_ID}.{PATH_TO_RESOURCE_SEPARATED_WITH_DOTS}` Example for a DPMS
[ table: `dataproc_metastore:{PROJECT_ID}.{LOCATION_ID}.{INSTANCE_ID}.{DATABASE_ID}.{TABLE_ID}`
[
*/
public java.lang.String getFullyQualifiedName() {
return fullyQualifiedName;
}
/**
* [Fully Qualified Name (FQN)](https://cloud.google.com//data-catalog/docs/fully-qualified-
* names) of the resource. FQNs take two forms: * For non-regionalized resources:
* `{SYSTEM}:{PROJECT}.{PATH_TO_RESOURCE_SEPARATED_WITH_DOTS}` * For regionalized resources:
* `{SYSTEM}:{PROJECT}.{LOCATION_ID}.{PATH_TO_RESOURCE_SEPARATED_WITH_DOTS}` Example for a
* DPMS table:
* `dataproc_metastore:{PROJECT_ID}.{LOCATION_ID}.{INSTANCE_ID}.{DATABASE_ID}.{TABLE_ID}`
*/
public Lookup setFullyQualifiedName(java.lang.String fullyQualifiedName) {
this.fullyQualifiedName = fullyQualifiedName;
return this;
}
/**
* The full name of the Google Cloud Platform resource the Data Catalog entry represents. For
* more information, see [Full Resource Name]
* (https://cloud.google.com/apis/design/resource_names#full_resource_name). Full names are
* case-sensitive. For example: *
* `//bigquery.googleapis.com/projects/{PROJECT_ID}/datasets/{DATASET_ID}/tables/{TABLE_ID}` *
* `//pubsub.googleapis.com/projects/{PROJECT_ID}/topics/{TOPIC_ID}`
*/
@com.google.api.client.util.Key
private java.lang.String linkedResource;
/** The full name of the Google Cloud Platform resource the Data Catalog entry represents. For more
information, see [Full Resource Name]
(https://cloud.google.com/apis/design/resource_names#full_resource_name). Full names are case-
sensitive. For example: *
`//bigquery.googleapis.com/projects/{PROJECT_ID}/datasets/{DATASET_ID}/tables/{TABLE_ID}` *
`//pubsub.googleapis.com/projects/{PROJECT_ID}/topics/{TOPIC_ID}`
*/
public java.lang.String getLinkedResource() {
return linkedResource;
}
/**
* The full name of the Google Cloud Platform resource the Data Catalog entry represents. For
* more information, see [Full Resource Name]
* (https://cloud.google.com/apis/design/resource_names#full_resource_name). Full names are
* case-sensitive. For example: *
* `//bigquery.googleapis.com/projects/{PROJECT_ID}/datasets/{DATASET_ID}/tables/{TABLE_ID}` *
* `//pubsub.googleapis.com/projects/{PROJECT_ID}/topics/{TOPIC_ID}`
*/
public Lookup setLinkedResource(java.lang.String linkedResource) {
this.linkedResource = linkedResource;
return this;
}
/**
* Location where the lookup should be performed. Required to lookup entry that is not a part
* of `DPMS` or `DATAPLEX` `integrated_system` using its `fully_qualified_name`. Ignored in
* other cases.
*/
@com.google.api.client.util.Key
private java.lang.String location;
/** Location where the lookup should be performed. Required to lookup entry that is not a part of
`DPMS` or `DATAPLEX` `integrated_system` using its `fully_qualified_name`. Ignored in other cases.
*/
public java.lang.String getLocation() {
return location;
}
/**
* Location where the lookup should be performed. Required to lookup entry that is not a part
* of `DPMS` or `DATAPLEX` `integrated_system` using its `fully_qualified_name`. Ignored in
* other cases.
*/
public Lookup setLocation(java.lang.String location) {
this.location = location;
return this;
}
/**
* Project where the lookup should be performed. Required to lookup entry that is not a part
* of `DPMS` or `DATAPLEX` `integrated_system` using its `fully_qualified_name`. Ignored in
* other cases.
*/
@com.google.api.client.util.Key
private java.lang.String project;
/** Project where the lookup should be performed. Required to lookup entry that is not a part of `DPMS`
or `DATAPLEX` `integrated_system` using its `fully_qualified_name`. Ignored in other cases.
*/
public java.lang.String getProject() {
return project;
}
/**
* Project where the lookup should be performed. Required to lookup entry that is not a part
* of `DPMS` or `DATAPLEX` `integrated_system` using its `fully_qualified_name`. Ignored in
* other cases.
*/
public Lookup setProject(java.lang.String project) {
this.project = project;
return this;
}
/**
* The SQL name of the entry. SQL names are case-sensitive. Examples: *
* `pubsub.topic.{PROJECT_ID}.{TOPIC_ID}` *
* `pubsub.topic.{PROJECT_ID}.`\``{TOPIC.ID.SEPARATED.WITH.DOTS}`\` *
* `bigquery.table.{PROJECT_ID}.{DATASET_ID}.{TABLE_ID}` *
* `bigquery.dataset.{PROJECT_ID}.{DATASET_ID}` *
* `datacatalog.entry.{PROJECT_ID}.{LOCATION_ID}.{ENTRY_GROUP_ID}.{ENTRY_ID}` Identifiers
* (`*_ID`) should comply with the [Lexical structure in Standard SQL]
* (https://cloud.google.com/bigquery/docs/reference/standard-sql/lexical).
*/
@com.google.api.client.util.Key
private java.lang.String sqlResource;
/** The SQL name of the entry. SQL names are case-sensitive. Examples: *
`pubsub.topic.{PROJECT_ID}.{TOPIC_ID}` *
`pubsub.topic.{PROJECT_ID}.`\``{TOPIC.ID.SEPARATED.WITH.DOTS}`\` *
`bigquery.table.{PROJECT_ID}.{DATASET_ID}.{TABLE_ID}` *
`bigquery.dataset.{PROJECT_ID}.{DATASET_ID}` *
`datacatalog.entry.{PROJECT_ID}.{LOCATION_ID}.{ENTRY_GROUP_ID}.{ENTRY_ID}` Identifiers (`*_ID`)
should comply with the [Lexical structure in Standard SQL]
(https://cloud.google.com/bigquery/docs/reference/standard-sql/lexical).
*/
public java.lang.String getSqlResource() {
return sqlResource;
}
/**
* The SQL name of the entry. SQL names are case-sensitive. Examples: *
* `pubsub.topic.{PROJECT_ID}.{TOPIC_ID}` *
* `pubsub.topic.{PROJECT_ID}.`\``{TOPIC.ID.SEPARATED.WITH.DOTS}`\` *
* `bigquery.table.{PROJECT_ID}.{DATASET_ID}.{TABLE_ID}` *
* `bigquery.dataset.{PROJECT_ID}.{DATASET_ID}` *
* `datacatalog.entry.{PROJECT_ID}.{LOCATION_ID}.{ENTRY_GROUP_ID}.{ENTRY_ID}` Identifiers
* (`*_ID`) should comply with the [Lexical structure in Standard SQL]
* (https://cloud.google.com/bigquery/docs/reference/standard-sql/lexical).
*/
public Lookup setSqlResource(java.lang.String sqlResource) {
this.sqlResource = sqlResource;
return this;
}
@Override
public Lookup set(String parameterName, Object value) {
return (Lookup) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Organizations collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Organizations.List request = datacatalog.organizations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Organizations organizations() {
return new Organizations();
}
/**
* The "organizations" collection of methods.
*/
public class Organizations {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Locations.List request = datacatalog.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Retrieves the configuration related to the migration from Data Catalog to Dataplex for a specific
* organization, including all the projects under it which have a separate configuration set.
*
* Create a request for the method "locations.retrieveConfig".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link RetrieveConfig#execute()} method to invoke the remote operation.
*
* @param name Required. The organization whose config is being retrieved.
* @return the request
*/
public RetrieveConfig retrieveConfig(java.lang.String name) throws java.io.IOException {
RetrieveConfig result = new RetrieveConfig(name);
initialize(result);
return result;
}
public class RetrieveConfig extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:retrieveConfig";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Retrieves the configuration related to the migration from Data Catalog to Dataplex for a
* specific organization, including all the projects under it which have a separate configuration
* set.
*
* Create a request for the method "locations.retrieveConfig".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link RetrieveConfig#execute()} method to invoke the remote
* operation. {@link RetrieveConfig#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param name Required. The organization whose config is being retrieved.
* @since 1.13
*/
protected RetrieveConfig(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1OrganizationConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public RetrieveConfig set$Xgafv(java.lang.String $Xgafv) {
return (RetrieveConfig) super.set$Xgafv($Xgafv);
}
@Override
public RetrieveConfig setAccessToken(java.lang.String accessToken) {
return (RetrieveConfig) super.setAccessToken(accessToken);
}
@Override
public RetrieveConfig setAlt(java.lang.String alt) {
return (RetrieveConfig) super.setAlt(alt);
}
@Override
public RetrieveConfig setCallback(java.lang.String callback) {
return (RetrieveConfig) super.setCallback(callback);
}
@Override
public RetrieveConfig setFields(java.lang.String fields) {
return (RetrieveConfig) super.setFields(fields);
}
@Override
public RetrieveConfig setKey(java.lang.String key) {
return (RetrieveConfig) super.setKey(key);
}
@Override
public RetrieveConfig setOauthToken(java.lang.String oauthToken) {
return (RetrieveConfig) super.setOauthToken(oauthToken);
}
@Override
public RetrieveConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RetrieveConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public RetrieveConfig setQuotaUser(java.lang.String quotaUser) {
return (RetrieveConfig) super.setQuotaUser(quotaUser);
}
@Override
public RetrieveConfig setUploadType(java.lang.String uploadType) {
return (RetrieveConfig) super.setUploadType(uploadType);
}
@Override
public RetrieveConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (RetrieveConfig) super.setUploadProtocol(uploadProtocol);
}
/** Required. The organization whose config is being retrieved. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The organization whose config is being retrieved.
*/
public java.lang.String getName() {
return name;
}
/** Required. The organization whose config is being retrieved. */
public RetrieveConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public RetrieveConfig set(String parameterName, Object value) {
return (RetrieveConfig) super.set(parameterName, value);
}
}
/**
* Retrieves the effective configuration related to the migration from Data Catalog to Dataplex for
* a specific organization or project. If there is no specific configuration set for the resource,
* the setting is checked hierarchicahlly through the ancestors of the resource, starting from the
* resource itself.
*
* Create a request for the method "locations.retrieveEffectiveConfig".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link RetrieveEffectiveConfig#execute()} method to invoke the remote
* operation.
*
* @param name Required. The resource whose effective config is being retrieved.
* @return the request
*/
public RetrieveEffectiveConfig retrieveEffectiveConfig(java.lang.String name) throws java.io.IOException {
RetrieveEffectiveConfig result = new RetrieveEffectiveConfig(name);
initialize(result);
return result;
}
public class RetrieveEffectiveConfig extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:retrieveEffectiveConfig";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Retrieves the effective configuration related to the migration from Data Catalog to Dataplex
* for a specific organization or project. If there is no specific configuration set for the
* resource, the setting is checked hierarchicahlly through the ancestors of the resource,
* starting from the resource itself.
*
* Create a request for the method "locations.retrieveEffectiveConfig".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link RetrieveEffectiveConfig#execute()} method to invoke the
* remote operation. {@link RetrieveEffectiveConfig#initialize(com.google.api.client.googleapi
* s.services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The resource whose effective config is being retrieved.
* @since 1.13
*/
protected RetrieveEffectiveConfig(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1MigrationConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public RetrieveEffectiveConfig set$Xgafv(java.lang.String $Xgafv) {
return (RetrieveEffectiveConfig) super.set$Xgafv($Xgafv);
}
@Override
public RetrieveEffectiveConfig setAccessToken(java.lang.String accessToken) {
return (RetrieveEffectiveConfig) super.setAccessToken(accessToken);
}
@Override
public RetrieveEffectiveConfig setAlt(java.lang.String alt) {
return (RetrieveEffectiveConfig) super.setAlt(alt);
}
@Override
public RetrieveEffectiveConfig setCallback(java.lang.String callback) {
return (RetrieveEffectiveConfig) super.setCallback(callback);
}
@Override
public RetrieveEffectiveConfig setFields(java.lang.String fields) {
return (RetrieveEffectiveConfig) super.setFields(fields);
}
@Override
public RetrieveEffectiveConfig setKey(java.lang.String key) {
return (RetrieveEffectiveConfig) super.setKey(key);
}
@Override
public RetrieveEffectiveConfig setOauthToken(java.lang.String oauthToken) {
return (RetrieveEffectiveConfig) super.setOauthToken(oauthToken);
}
@Override
public RetrieveEffectiveConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RetrieveEffectiveConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public RetrieveEffectiveConfig setQuotaUser(java.lang.String quotaUser) {
return (RetrieveEffectiveConfig) super.setQuotaUser(quotaUser);
}
@Override
public RetrieveEffectiveConfig setUploadType(java.lang.String uploadType) {
return (RetrieveEffectiveConfig) super.setUploadType(uploadType);
}
@Override
public RetrieveEffectiveConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (RetrieveEffectiveConfig) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource whose effective config is being retrieved. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource whose effective config is being retrieved.
*/
public java.lang.String getName() {
return name;
}
/** Required. The resource whose effective config is being retrieved. */
public RetrieveEffectiveConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public RetrieveEffectiveConfig set(String parameterName, Object value) {
return (RetrieveEffectiveConfig) super.set(parameterName, value);
}
}
/**
* Sets the configuration related to the migration to Dataplex for an organization or project.
*
* Create a request for the method "locations.setConfig".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link SetConfig#execute()} method to invoke the remote operation.
*
* @param name Required. The organization or project whose config is being specified.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest}
* @return the request
*/
public SetConfig setConfig(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest content) throws java.io.IOException {
SetConfig result = new SetConfig(name, content);
initialize(result);
return result;
}
public class SetConfig extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:setConfig";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/locations/[^/]+$");
/**
* Sets the configuration related to the migration to Dataplex for an organization or project.
*
* Create a request for the method "locations.setConfig".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link SetConfig#execute()} method to invoke the remote
* operation. {@link
* SetConfig#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The organization or project whose config is being specified.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest}
* @since 1.13
*/
protected SetConfig(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1MigrationConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
}
@Override
public SetConfig set$Xgafv(java.lang.String $Xgafv) {
return (SetConfig) super.set$Xgafv($Xgafv);
}
@Override
public SetConfig setAccessToken(java.lang.String accessToken) {
return (SetConfig) super.setAccessToken(accessToken);
}
@Override
public SetConfig setAlt(java.lang.String alt) {
return (SetConfig) super.setAlt(alt);
}
@Override
public SetConfig setCallback(java.lang.String callback) {
return (SetConfig) super.setCallback(callback);
}
@Override
public SetConfig setFields(java.lang.String fields) {
return (SetConfig) super.setFields(fields);
}
@Override
public SetConfig setKey(java.lang.String key) {
return (SetConfig) super.setKey(key);
}
@Override
public SetConfig setOauthToken(java.lang.String oauthToken) {
return (SetConfig) super.setOauthToken(oauthToken);
}
@Override
public SetConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public SetConfig setQuotaUser(java.lang.String quotaUser) {
return (SetConfig) super.setQuotaUser(quotaUser);
}
@Override
public SetConfig setUploadType(java.lang.String uploadType) {
return (SetConfig) super.setUploadType(uploadType);
}
@Override
public SetConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (SetConfig) super.setUploadProtocol(uploadProtocol);
}
/** Required. The organization or project whose config is being specified. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The organization or project whose config is being specified.
*/
public java.lang.String getName() {
return name;
}
/** Required. The organization or project whose config is being specified. */
public SetConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public SetConfig set(String parameterName, Object value) {
return (SetConfig) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Projects.List request = datacatalog.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Locations.List request = datacatalog.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Retrieves the effective configuration related to the migration from Data Catalog to Dataplex for
* a specific organization or project. If there is no specific configuration set for the resource,
* the setting is checked hierarchicahlly through the ancestors of the resource, starting from the
* resource itself.
*
* Create a request for the method "locations.retrieveEffectiveConfig".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link RetrieveEffectiveConfig#execute()} method to invoke the remote
* operation.
*
* @param name Required. The resource whose effective config is being retrieved.
* @return the request
*/
public RetrieveEffectiveConfig retrieveEffectiveConfig(java.lang.String name) throws java.io.IOException {
RetrieveEffectiveConfig result = new RetrieveEffectiveConfig(name);
initialize(result);
return result;
}
public class RetrieveEffectiveConfig extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:retrieveEffectiveConfig";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Retrieves the effective configuration related to the migration from Data Catalog to Dataplex
* for a specific organization or project. If there is no specific configuration set for the
* resource, the setting is checked hierarchicahlly through the ancestors of the resource,
* starting from the resource itself.
*
* Create a request for the method "locations.retrieveEffectiveConfig".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link RetrieveEffectiveConfig#execute()} method to invoke the
* remote operation. {@link RetrieveEffectiveConfig#initialize(com.google.api.client.googleapi
* s.services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The resource whose effective config is being retrieved.
* @since 1.13
*/
protected RetrieveEffectiveConfig(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1MigrationConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public RetrieveEffectiveConfig set$Xgafv(java.lang.String $Xgafv) {
return (RetrieveEffectiveConfig) super.set$Xgafv($Xgafv);
}
@Override
public RetrieveEffectiveConfig setAccessToken(java.lang.String accessToken) {
return (RetrieveEffectiveConfig) super.setAccessToken(accessToken);
}
@Override
public RetrieveEffectiveConfig setAlt(java.lang.String alt) {
return (RetrieveEffectiveConfig) super.setAlt(alt);
}
@Override
public RetrieveEffectiveConfig setCallback(java.lang.String callback) {
return (RetrieveEffectiveConfig) super.setCallback(callback);
}
@Override
public RetrieveEffectiveConfig setFields(java.lang.String fields) {
return (RetrieveEffectiveConfig) super.setFields(fields);
}
@Override
public RetrieveEffectiveConfig setKey(java.lang.String key) {
return (RetrieveEffectiveConfig) super.setKey(key);
}
@Override
public RetrieveEffectiveConfig setOauthToken(java.lang.String oauthToken) {
return (RetrieveEffectiveConfig) super.setOauthToken(oauthToken);
}
@Override
public RetrieveEffectiveConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RetrieveEffectiveConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public RetrieveEffectiveConfig setQuotaUser(java.lang.String quotaUser) {
return (RetrieveEffectiveConfig) super.setQuotaUser(quotaUser);
}
@Override
public RetrieveEffectiveConfig setUploadType(java.lang.String uploadType) {
return (RetrieveEffectiveConfig) super.setUploadType(uploadType);
}
@Override
public RetrieveEffectiveConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (RetrieveEffectiveConfig) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource whose effective config is being retrieved. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource whose effective config is being retrieved.
*/
public java.lang.String getName() {
return name;
}
/** Required. The resource whose effective config is being retrieved. */
public RetrieveEffectiveConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public RetrieveEffectiveConfig set(String parameterName, Object value) {
return (RetrieveEffectiveConfig) super.set(parameterName, value);
}
}
/**
* Sets the configuration related to the migration to Dataplex for an organization or project.
*
* Create a request for the method "locations.setConfig".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link SetConfig#execute()} method to invoke the remote operation.
*
* @param name Required. The organization or project whose config is being specified.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest}
* @return the request
*/
public SetConfig setConfig(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest content) throws java.io.IOException {
SetConfig result = new SetConfig(name, content);
initialize(result);
return result;
}
public class SetConfig extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:setConfig";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Sets the configuration related to the migration to Dataplex for an organization or project.
*
* Create a request for the method "locations.setConfig".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link SetConfig#execute()} method to invoke the remote
* operation. {@link
* SetConfig#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The organization or project whose config is being specified.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest}
* @since 1.13
*/
protected SetConfig(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1SetConfigRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1MigrationConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public SetConfig set$Xgafv(java.lang.String $Xgafv) {
return (SetConfig) super.set$Xgafv($Xgafv);
}
@Override
public SetConfig setAccessToken(java.lang.String accessToken) {
return (SetConfig) super.setAccessToken(accessToken);
}
@Override
public SetConfig setAlt(java.lang.String alt) {
return (SetConfig) super.setAlt(alt);
}
@Override
public SetConfig setCallback(java.lang.String callback) {
return (SetConfig) super.setCallback(callback);
}
@Override
public SetConfig setFields(java.lang.String fields) {
return (SetConfig) super.setFields(fields);
}
@Override
public SetConfig setKey(java.lang.String key) {
return (SetConfig) super.setKey(key);
}
@Override
public SetConfig setOauthToken(java.lang.String oauthToken) {
return (SetConfig) super.setOauthToken(oauthToken);
}
@Override
public SetConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public SetConfig setQuotaUser(java.lang.String quotaUser) {
return (SetConfig) super.setQuotaUser(quotaUser);
}
@Override
public SetConfig setUploadType(java.lang.String uploadType) {
return (SetConfig) super.setUploadType(uploadType);
}
@Override
public SetConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (SetConfig) super.setUploadProtocol(uploadProtocol);
}
/** Required. The organization or project whose config is being specified. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The organization or project whose config is being specified.
*/
public java.lang.String getName() {
return name;
}
/** Required. The organization or project whose config is being specified. */
public SetConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public SetConfig set(String parameterName, Object value) {
return (SetConfig) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the EntryGroups collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.EntryGroups.List request = datacatalog.entryGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public EntryGroups entryGroups() {
return new EntryGroups();
}
/**
* The "entryGroups" collection of methods.
*/
public class EntryGroups {
/**
* Creates an entry group. An entry group contains logically related entries together with [Cloud
* Identity and Access Management](/data-catalog/docs/concepts/iam) policies. These policies specify
* users who can create, edit, and view entries within entry groups. Data Catalog automatically
* creates entry groups with names that start with the `@` symbol for the following resources: *
* BigQuery entries (`@bigquery`) * Pub/Sub topics (`@pubsub`) * Dataproc Metastore services
* (`@dataproc_metastore_{SERVICE_NAME_HASH}`) You can create your own entry groups for Cloud
* Storage fileset entries and custom entries together with the corresponding IAM policies. User-
* created entry groups can't contain the `@` symbol, it is reserved for automatically created
* groups. Entry groups, like entries, can be searched. A maximum of 10,000 entry groups may be
* created per organization across all locations. You must enable the Data Catalog API in the
* project identified by the `parent` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entryGroups.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The names of the project and location that the new entry group belongs to. Note: The entry
* group itself and its child resources might not be stored in the location specified in its
* name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/entryGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates an entry group. An entry group contains logically related entries together with [Cloud
* Identity and Access Management](/data-catalog/docs/concepts/iam) policies. These policies
* specify users who can create, edit, and view entries within entry groups. Data Catalog
* automatically creates entry groups with names that start with the `@` symbol for the following
* resources: * BigQuery entries (`@bigquery`) * Pub/Sub topics (`@pubsub`) * Dataproc Metastore
* services (`@dataproc_metastore_{SERVICE_NAME_HASH}`) You can create your own entry groups for
* Cloud Storage fileset entries and custom entries together with the corresponding IAM policies.
* User-created entry groups can't contain the `@` symbol, it is reserved for automatically
* created groups. Entry groups, like entries, can be searched. A maximum of 10,000 entry groups
* may be created per organization across all locations. You must enable the Data Catalog API in
* the project identified by the `parent` parameter. For more information, see [Data Catalog
* resource project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entryGroups.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The names of the project and location that the new entry group belongs to. Note: The entry
* group itself and its child resources might not be stored in the location specified in its
* name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The names of the project and location that the new entry group belongs to.
* Note: The entry group itself and its child resources might not be stored in the
* location specified in its name.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The names of the project and location that the new entry group belongs to. Note: The
entry group itself and its child resources might not be stored in the location specified in its
name.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The names of the project and location that the new entry group belongs to.
* Note: The entry group itself and its child resources might not be stored in the
* location specified in its name.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID of the entry group to create. The ID must contain only letters (a-z,
* A-Z), numbers (0-9), underscores (_), and must start with a letter or underscore. The
* maximum size is 64 bytes when encoded in UTF-8.
*/
@com.google.api.client.util.Key
private java.lang.String entryGroupId;
/** Required. The ID of the entry group to create. The ID must contain only letters (a-z, A-Z), numbers
(0-9), underscores (_), and must start with a letter or underscore. The maximum size is 64 bytes
when encoded in UTF-8.
*/
public java.lang.String getEntryGroupId() {
return entryGroupId;
}
/**
* Required. The ID of the entry group to create. The ID must contain only letters (a-z,
* A-Z), numbers (0-9), underscores (_), and must start with a letter or underscore. The
* maximum size is 64 bytes when encoded in UTF-8.
*/
public Create setEntryGroupId(java.lang.String entryGroupId) {
this.entryGroupId = entryGroupId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an entry group. You must enable the Data Catalog API in the project identified by the
* `name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entryGroups.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entry group to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Deletes an entry group. You must enable the Data Catalog API in the project identified by the
* `name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entryGroups.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entry group to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the entry group to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entry group to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the entry group to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.name = name;
return this;
}
/** Optional. If true, deletes all entries in the entry group. */
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Optional. If true, deletes all entries in the entry group.
*/
public java.lang.Boolean getForce() {
return force;
}
/** Optional. If true, deletes all entries in the entry group. */
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an entry group.
*
* Create a request for the method "entryGroups.get".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entry group to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Gets an entry group.
*
* Create a request for the method "entryGroups.get".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entry group to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the entry group to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entry group to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the entry group to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.name = name;
return this;
}
/** The fields to return. If empty or omitted, all fields are returned. */
@com.google.api.client.util.Key
private String readMask;
/** The fields to return. If empty or omitted, all fields are returned.
*/
public String getReadMask() {
return readMask;
}
/** The fields to return. If empty or omitted, all fields are returned. */
public Get setReadMask(String readMask) {
this.readMask = readMask;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. May return: * A`NOT_FOUND` error if the resource
* doesn't exist or you don't have the permission to view it. * An empty policy if the resource
* exists but doesn't have a set policy. Supported resources are: - Tag templates - Entry groups
* Note: This method doesn't get policies from Google Cloud Platform resources ingested into Data
* Catalog. To call this method, you must have the following Google IAM permissions: -
* `datacatalog.tagTemplates.getIamPolicy` to get policies on tag templates. -
* `datacatalog.entryGroups.getIamPolicy` to get policies on entry groups.
*
* Create a request for the method "entryGroups.getIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Gets the access control policy for a resource. May return: * A`NOT_FOUND` error if the resource
* doesn't exist or you don't have the permission to view it. * An empty policy if the resource
* exists but doesn't have a set policy. Supported resources are: - Tag templates - Entry groups
* Note: This method doesn't get policies from Google Cloud Platform resources ingested into Data
* Catalog. To call this method, you must have the following Google IAM permissions: -
* `datacatalog.tagTemplates.getIamPolicy` to get policies on tag templates. -
* `datacatalog.entryGroups.getIamPolicy` to get policies on entry groups.
*
* Create a request for the method "entryGroups.getIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists entry groups.
*
* Create a request for the method "entryGroups.list".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the location that contains the entry groups to list. Can be provided as a URL.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/entryGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists entry groups.
*
* Create a request for the method "entryGroups.list".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the location that contains the entry groups to list. Can be provided as a URL.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ListEntryGroupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the location that contains the entry groups to list. Can be
* provided as a URL.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the location that contains the entry groups to list. Can be provided as a
URL.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the location that contains the entry groups to list. Can be
* provided as a URL.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of items to return. Default is 10. Maximum limit is 1000.
* Throws an invalid argument if `page_size` is greater than 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of items to return. Default is 10. Maximum limit is 1000. Throws an
invalid argument if `page_size` is greater than 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of items to return. Default is 10. Maximum limit is 1000.
* Throws an invalid argument if `page_size` is greater than 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. Pagination token that specifies the next page to return. If empty, returns
* the first page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. Pagination token that specifies the next page to return. If empty, returns the first
page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. Pagination token that specifies the next page to return. If empty, returns
* the first page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an entry group. You must enable the Data Catalog API in the project identified by the
* `entry_group.name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entryGroups.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. The resource name of the entry group in URL format. Note: The entry group itself and its
* child resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Updates an entry group. You must enable the Data Catalog API in the project identified by the
* `entry_group.name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entryGroups.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. The resource name of the entry group in URL format. Note: The entry group itself and its
* child resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. The resource name of the entry group in URL format. Note: The entry group
* itself and its child resources might not be stored in the location specified in its
* name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. The resource name of the entry group in URL format. Note: The entry group itself and
its child resources might not be stored in the location specified in its name.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. The resource name of the entry group in URL format. Note: The entry group
* itself and its child resources might not be stored in the location specified in its
* name.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* Names of fields whose values to overwrite on an entry group. If this parameter is
* absent or empty, all modifiable fields are overwritten. If such fields are non-required
* and omitted in the request body, their values are emptied.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Names of fields whose values to overwrite on an entry group. If this parameter is absent or empty,
all modifiable fields are overwritten. If such fields are non-required and omitted in the request
body, their values are emptied.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Names of fields whose values to overwrite on an entry group. If this parameter is
* absent or empty, all modifiable fields are overwritten. If such fields are non-required
* and omitted in the request body, their values are emptied.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets an access control policy for a resource. Replaces any existing policy. Supported resources
* are: - Tag templates - Entry groups Note: This method sets policies only within Data Catalog and
* can't be used to manage policies in BigQuery, Pub/Sub, Dataproc Metastore, and any external
* Google Cloud Platform resources synced with the Data Catalog. To call this method, you must have
* the following Google IAM permissions: - `datacatalog.tagTemplates.setIamPolicy` to set policies
* on tag templates. - `datacatalog.entryGroups.setIamPolicy` to set policies on entry groups.
*
* Create a request for the method "entryGroups.setIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Sets an access control policy for a resource. Replaces any existing policy. Supported resources
* are: - Tag templates - Entry groups Note: This method sets policies only within Data Catalog
* and can't be used to manage policies in BigQuery, Pub/Sub, Dataproc Metastore, and any external
* Google Cloud Platform resources synced with the Data Catalog. To call this method, you must
* have the following Google IAM permissions: - `datacatalog.tagTemplates.setIamPolicy` to set
* policies on tag templates. - `datacatalog.entryGroups.setIamPolicy` to set policies on entry
* groups.
*
* Create a request for the method "entryGroups.setIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Gets your permissions on a resource. Returns an empty set of permissions if the resource doesn't
* exist. Supported resources are: - Tag templates - Entry groups Note: This method gets policies
* only within Data Catalog and can't be used to get policies from BigQuery, Pub/Sub, Dataproc
* Metastore, and any external Google Cloud Platform resources ingested into Data Catalog. No Google
* IAM permissions are required to call this method.
*
* Create a request for the method "entryGroups.testIamPermissions".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Gets your permissions on a resource. Returns an empty set of permissions if the resource
* doesn't exist. Supported resources are: - Tag templates - Entry groups Note: This method gets
* policies only within Data Catalog and can't be used to get policies from BigQuery, Pub/Sub,
* Dataproc Metastore, and any external Google Cloud Platform resources ingested into Data
* Catalog. No Google IAM permissions are required to call this method.
*
* Create a request for the method "entryGroups.testIamPermissions".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Entries collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Entries.List request = datacatalog.entries().list(parameters ...)}
*
*
* @return the resource collection
*/
public Entries entries() {
return new Entries();
}
/**
* The "entries" collection of methods.
*/
public class Entries {
/**
* Creates an entry. You can create entries only with 'FILESET', 'CLUSTER', 'DATA_STREAM', or custom
* types. Data Catalog automatically creates entries with other types during metadata ingestion from
* integrated systems. You must enable the Data Catalog API in the project identified by the
* `parent` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project). An entry group
* can have a maximum of 100,000 entries.
*
* Create a request for the method "entries.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the entry group this entry belongs to. Note: The entry itself and its child
* resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/entries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Creates an entry. You can create entries only with 'FILESET', 'CLUSTER', 'DATA_STREAM', or
* custom types. Data Catalog automatically creates entries with other types during metadata
* ingestion from integrated systems. You must enable the Data Catalog API in the project
* identified by the `parent` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project). An entry group
* can have a maximum of 100,000 entries.
*
* Create a request for the method "entries.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the entry group this entry belongs to. Note: The entry itself and its child
* resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the entry group this entry belongs to. Note: The entry itself
* and its child resources might not be stored in the location specified in its name.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the entry group this entry belongs to. Note: The entry itself and its child
resources might not be stored in the location specified in its name.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the entry group this entry belongs to. Note: The entry itself
* and its child resources might not be stored in the location specified in its name.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID of the entry to create. The ID must contain only letters (a-z, A-Z),
* numbers (0-9), and underscores (_). The maximum size is 64 bytes when encoded in
* UTF-8.
*/
@com.google.api.client.util.Key
private java.lang.String entryId;
/** Required. The ID of the entry to create. The ID must contain only letters (a-z, A-Z), numbers
(0-9), and underscores (_). The maximum size is 64 bytes when encoded in UTF-8.
*/
public java.lang.String getEntryId() {
return entryId;
}
/**
* Required. The ID of the entry to create. The ID must contain only letters (a-z, A-Z),
* numbers (0-9), and underscores (_). The maximum size is 64 bytes when encoded in
* UTF-8.
*/
public Create setEntryId(java.lang.String entryId) {
this.entryId = entryId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an existing entry. You can delete only the entries created by the CreateEntry method. You
* must enable the Data Catalog API in the project identified by the `name` parameter. For more
* information, see [Data Catalog resource project](https://cloud.google.com/data-
* catalog/docs/concepts/resource-project).
*
* Create a request for the method "entries.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entry to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Deletes an existing entry. You can delete only the entries created by the CreateEntry method.
* You must enable the Data Catalog API in the project identified by the `name` parameter. For
* more information, see [Data Catalog resource project](https://cloud.google.com/data-
* catalog/docs/concepts/resource-project).
*
* Create a request for the method "entries.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entry to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the entry to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entry to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the entry to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an entry.
*
* Create a request for the method "entries.get".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entry to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Gets an entry.
*
* Create a request for the method "entries.get".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entry to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the entry to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entry to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the entry to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. May return: * A`NOT_FOUND` error if the resource
* doesn't exist or you don't have the permission to view it. * An empty policy if the resource
* exists but doesn't have a set policy. Supported resources are: - Tag templates - Entry groups
* Note: This method doesn't get policies from Google Cloud Platform resources ingested into Data
* Catalog. To call this method, you must have the following Google IAM permissions: -
* `datacatalog.tagTemplates.getIamPolicy` to get policies on tag templates. -
* `datacatalog.entryGroups.getIamPolicy` to get policies on entry groups.
*
* Create a request for the method "entries.getIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Gets the access control policy for a resource. May return: * A`NOT_FOUND` error if the resource
* doesn't exist or you don't have the permission to view it. * An empty policy if the resource
* exists but doesn't have a set policy. Supported resources are: - Tag templates - Entry groups
* Note: This method doesn't get policies from Google Cloud Platform resources ingested into Data
* Catalog. To call this method, you must have the following Google IAM permissions: -
* `datacatalog.tagTemplates.getIamPolicy` to get policies on tag templates. -
* `datacatalog.entryGroups.getIamPolicy` to get policies on entry groups.
*
* Create a request for the method "entries.getIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Imports entries from a source, such as data previously dumped into a Cloud Storage bucket, into
* Data Catalog. Import of entries is a sync operation that reconciles the state of the third-party
* system with the Data Catalog. `ImportEntries` accepts source data snapshots of a third-party
* system. Snapshot should be delivered as a .wire or base65-encoded .txt file containing a sequence
* of Protocol Buffer messages of DumpItem type. `ImportEntries` returns a long-running operation
* resource that can be queried with Operations.GetOperation to return ImportEntriesMetadata and an
* ImportEntriesResponse message.
*
* Create a request for the method "entries.import".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link DataCatalogImport#execute()} method to invoke the remote operation.
*
* @param parent Required. Target entry group for ingested entries.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportEntriesRequest}
* @return the request
*/
public DataCatalogImport datacatalogImport(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportEntriesRequest content) throws java.io.IOException {
DataCatalogImport result = new DataCatalogImport(parent, content);
initialize(result);
return result;
}
public class DataCatalogImport extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/entries:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Imports entries from a source, such as data previously dumped into a Cloud Storage bucket, into
* Data Catalog. Import of entries is a sync operation that reconciles the state of the third-
* party system with the Data Catalog. `ImportEntries` accepts source data snapshots of a third-
* party system. Snapshot should be delivered as a .wire or base65-encoded .txt file containing a
* sequence of Protocol Buffer messages of DumpItem type. `ImportEntries` returns a long-running
* operation resource that can be queried with Operations.GetOperation to return
* ImportEntriesMetadata and an ImportEntriesResponse message.
*
* Create a request for the method "entries.import".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link DataCatalogImport#execute()} method to invoke the remote
* operation. {@link DataCatalogImport#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. Target entry group for ingested entries.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportEntriesRequest}
* @since 1.13
*/
protected DataCatalogImport(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportEntriesRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public DataCatalogImport set$Xgafv(java.lang.String $Xgafv) {
return (DataCatalogImport) super.set$Xgafv($Xgafv);
}
@Override
public DataCatalogImport setAccessToken(java.lang.String accessToken) {
return (DataCatalogImport) super.setAccessToken(accessToken);
}
@Override
public DataCatalogImport setAlt(java.lang.String alt) {
return (DataCatalogImport) super.setAlt(alt);
}
@Override
public DataCatalogImport setCallback(java.lang.String callback) {
return (DataCatalogImport) super.setCallback(callback);
}
@Override
public DataCatalogImport setFields(java.lang.String fields) {
return (DataCatalogImport) super.setFields(fields);
}
@Override
public DataCatalogImport setKey(java.lang.String key) {
return (DataCatalogImport) super.setKey(key);
}
@Override
public DataCatalogImport setOauthToken(java.lang.String oauthToken) {
return (DataCatalogImport) super.setOauthToken(oauthToken);
}
@Override
public DataCatalogImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DataCatalogImport) super.setPrettyPrint(prettyPrint);
}
@Override
public DataCatalogImport setQuotaUser(java.lang.String quotaUser) {
return (DataCatalogImport) super.setQuotaUser(quotaUser);
}
@Override
public DataCatalogImport setUploadType(java.lang.String uploadType) {
return (DataCatalogImport) super.setUploadType(uploadType);
}
@Override
public DataCatalogImport setUploadProtocol(java.lang.String uploadProtocol) {
return (DataCatalogImport) super.setUploadProtocol(uploadProtocol);
}
/** Required. Target entry group for ingested entries. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Target entry group for ingested entries.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Target entry group for ingested entries. */
public DataCatalogImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public DataCatalogImport set(String parameterName, Object value) {
return (DataCatalogImport) super.set(parameterName, value);
}
}
/**
* Lists entries. Note: Currently, this method can list only custom entries. To get a list of both
* custom and automatically created entries, use SearchCatalog.
*
* Create a request for the method "entries.list".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the entry group that contains the entries to list. Can be provided in URL
* format.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/entries";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Lists entries. Note: Currently, this method can list only custom entries. To get a list of both
* custom and automatically created entries, use SearchCatalog.
*
* Create a request for the method "entries.list".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the entry group that contains the entries to list. Can be provided in URL
* format.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ListEntriesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the entry group that contains the entries to list. Can be
* provided in URL format.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the entry group that contains the entries to list. Can be provided in URL
format.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the entry group that contains the entries to list. Can be
* provided in URL format.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return. Default is 10. Maximum limit is 1000. Throws
* an invalid argument if `page_size` is more than 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return. Default is 10. Maximum limit is 1000. Throws an invalid
argument if `page_size` is more than 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return. Default is 10. Maximum limit is 1000. Throws
* an invalid argument if `page_size` is more than 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Pagination token that specifies the next page to return. If empty, the first page is
* returned.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Pagination token that specifies the next page to return. If empty, the first page is returned.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Pagination token that specifies the next page to return. If empty, the first page is
* returned.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The fields to return for each entry. If empty or omitted, all fields are returned.
* For example, to return a list of entries with only the `name` field, set `read_mask`
* to only one path with the `name` value.
*/
@com.google.api.client.util.Key
private String readMask;
/** The fields to return for each entry. If empty or omitted, all fields are returned. For example, to
return a list of entries with only the `name` field, set `read_mask` to only one path with the
`name` value.
*/
public String getReadMask() {
return readMask;
}
/**
* The fields to return for each entry. If empty or omitted, all fields are returned.
* For example, to return a list of entries with only the `name` field, set `read_mask`
* to only one path with the `name` value.
*/
public List setReadMask(String readMask) {
this.readMask = readMask;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies contacts, part of the business context of an Entry. To call this method, you must have
* the `datacatalog.entries.updateContacts` IAM permission on the corresponding project.
*
* Create a request for the method "entries.modifyEntryContacts".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link ModifyEntryContacts#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the entry.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryContactsRequest}
* @return the request
*/
public ModifyEntryContacts modifyEntryContacts(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryContactsRequest content) throws java.io.IOException {
ModifyEntryContacts result = new ModifyEntryContacts(name, content);
initialize(result);
return result;
}
public class ModifyEntryContacts extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:modifyEntryContacts";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Modifies contacts, part of the business context of an Entry. To call this method, you must have
* the `datacatalog.entries.updateContacts` IAM permission on the corresponding project.
*
* Create a request for the method "entries.modifyEntryContacts".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link ModifyEntryContacts#execute()} method to invoke the remote
* operation. {@link ModifyEntryContacts#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The full resource name of the entry.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryContactsRequest}
* @since 1.13
*/
protected ModifyEntryContacts(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryContactsRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Contacts.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public ModifyEntryContacts set$Xgafv(java.lang.String $Xgafv) {
return (ModifyEntryContacts) super.set$Xgafv($Xgafv);
}
@Override
public ModifyEntryContacts setAccessToken(java.lang.String accessToken) {
return (ModifyEntryContacts) super.setAccessToken(accessToken);
}
@Override
public ModifyEntryContacts setAlt(java.lang.String alt) {
return (ModifyEntryContacts) super.setAlt(alt);
}
@Override
public ModifyEntryContacts setCallback(java.lang.String callback) {
return (ModifyEntryContacts) super.setCallback(callback);
}
@Override
public ModifyEntryContacts setFields(java.lang.String fields) {
return (ModifyEntryContacts) super.setFields(fields);
}
@Override
public ModifyEntryContacts setKey(java.lang.String key) {
return (ModifyEntryContacts) super.setKey(key);
}
@Override
public ModifyEntryContacts setOauthToken(java.lang.String oauthToken) {
return (ModifyEntryContacts) super.setOauthToken(oauthToken);
}
@Override
public ModifyEntryContacts setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ModifyEntryContacts) super.setPrettyPrint(prettyPrint);
}
@Override
public ModifyEntryContacts setQuotaUser(java.lang.String quotaUser) {
return (ModifyEntryContacts) super.setQuotaUser(quotaUser);
}
@Override
public ModifyEntryContacts setUploadType(java.lang.String uploadType) {
return (ModifyEntryContacts) super.setUploadType(uploadType);
}
@Override
public ModifyEntryContacts setUploadProtocol(java.lang.String uploadProtocol) {
return (ModifyEntryContacts) super.setUploadProtocol(uploadProtocol);
}
/** Required. The full resource name of the entry. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the entry.
*/
public java.lang.String getName() {
return name;
}
/** Required. The full resource name of the entry. */
public ModifyEntryContacts setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ModifyEntryContacts set(String parameterName, Object value) {
return (ModifyEntryContacts) super.set(parameterName, value);
}
}
/**
* Modifies entry overview, part of the business context of an Entry. To call this method, you must
* have the `datacatalog.entries.updateOverview` IAM permission on the corresponding project.
*
* Create a request for the method "entries.modifyEntryOverview".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link ModifyEntryOverview#execute()} method to invoke the remote operation.
*
* @param name Required. The full resource name of the entry.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryOverviewRequest}
* @return the request
*/
public ModifyEntryOverview modifyEntryOverview(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryOverviewRequest content) throws java.io.IOException {
ModifyEntryOverview result = new ModifyEntryOverview(name, content);
initialize(result);
return result;
}
public class ModifyEntryOverview extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:modifyEntryOverview";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Modifies entry overview, part of the business context of an Entry. To call this method, you
* must have the `datacatalog.entries.updateOverview` IAM permission on the corresponding project.
*
* Create a request for the method "entries.modifyEntryOverview".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link ModifyEntryOverview#execute()} method to invoke the remote
* operation. {@link ModifyEntryOverview#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The full resource name of the entry.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryOverviewRequest}
* @since 1.13
*/
protected ModifyEntryOverview(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ModifyEntryOverviewRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1EntryOverview.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public ModifyEntryOverview set$Xgafv(java.lang.String $Xgafv) {
return (ModifyEntryOverview) super.set$Xgafv($Xgafv);
}
@Override
public ModifyEntryOverview setAccessToken(java.lang.String accessToken) {
return (ModifyEntryOverview) super.setAccessToken(accessToken);
}
@Override
public ModifyEntryOverview setAlt(java.lang.String alt) {
return (ModifyEntryOverview) super.setAlt(alt);
}
@Override
public ModifyEntryOverview setCallback(java.lang.String callback) {
return (ModifyEntryOverview) super.setCallback(callback);
}
@Override
public ModifyEntryOverview setFields(java.lang.String fields) {
return (ModifyEntryOverview) super.setFields(fields);
}
@Override
public ModifyEntryOverview setKey(java.lang.String key) {
return (ModifyEntryOverview) super.setKey(key);
}
@Override
public ModifyEntryOverview setOauthToken(java.lang.String oauthToken) {
return (ModifyEntryOverview) super.setOauthToken(oauthToken);
}
@Override
public ModifyEntryOverview setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ModifyEntryOverview) super.setPrettyPrint(prettyPrint);
}
@Override
public ModifyEntryOverview setQuotaUser(java.lang.String quotaUser) {
return (ModifyEntryOverview) super.setQuotaUser(quotaUser);
}
@Override
public ModifyEntryOverview setUploadType(java.lang.String uploadType) {
return (ModifyEntryOverview) super.setUploadType(uploadType);
}
@Override
public ModifyEntryOverview setUploadProtocol(java.lang.String uploadProtocol) {
return (ModifyEntryOverview) super.setUploadProtocol(uploadProtocol);
}
/** Required. The full resource name of the entry. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The full resource name of the entry.
*/
public java.lang.String getName() {
return name;
}
/** Required. The full resource name of the entry. */
public ModifyEntryOverview setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ModifyEntryOverview set(String parameterName, Object value) {
return (ModifyEntryOverview) super.set(parameterName, value);
}
}
/**
* Updates an existing entry. You must enable the Data Catalog API in the project identified by the
* `entry.name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entries.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Identifier. The resource name of an entry in URL format. Note: The entry itself and its
* child resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Updates an existing entry. You must enable the Data Catalog API in the project identified by
* the `entry.name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "entries.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Identifier. The resource name of an entry in URL format. Note: The entry itself and its
* child resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Entry.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Identifier. The resource name of an entry in URL format. Note: The entry
* itself and its child resources might not be stored in the location specified in its
* name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Identifier. The resource name of an entry in URL format. Note: The entry itself and
its child resources might not be stored in the location specified in its name.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Identifier. The resource name of an entry in URL format. Note: The entry
* itself and its child resources might not be stored in the location specified in its
* name.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.name = name;
return this;
}
/**
* Names of fields whose values to overwrite on an entry. If this parameter is absent or
* empty, all modifiable fields are overwritten. If such fields are non-required and
* omitted in the request body, their values are emptied. You can modify only the fields
* listed below. For entries with type `DATA_STREAM`: * `schema` For entries with type
* `FILESET`: * `schema` * `display_name` * `description` * `gcs_fileset_spec` *
* `gcs_fileset_spec.file_patterns` For entries with `user_specified_type`: * `schema` *
* `display_name` * `description` * `user_specified_type` * `user_specified_system` *
* `linked_resource` * `source_system_timestamps`
*/
@com.google.api.client.util.Key
private String updateMask;
/** Names of fields whose values to overwrite on an entry. If this parameter is absent or empty, all
modifiable fields are overwritten. If such fields are non-required and omitted in the request body,
their values are emptied. You can modify only the fields listed below. For entries with type
`DATA_STREAM`: * `schema` For entries with type `FILESET`: * `schema` * `display_name` *
`description` * `gcs_fileset_spec` * `gcs_fileset_spec.file_patterns` For entries with
`user_specified_type`: * `schema` * `display_name` * `description` * `user_specified_type` *
`user_specified_system` * `linked_resource` * `source_system_timestamps`
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Names of fields whose values to overwrite on an entry. If this parameter is absent or
* empty, all modifiable fields are overwritten. If such fields are non-required and
* omitted in the request body, their values are emptied. You can modify only the fields
* listed below. For entries with type `DATA_STREAM`: * `schema` For entries with type
* `FILESET`: * `schema` * `display_name` * `description` * `gcs_fileset_spec` *
* `gcs_fileset_spec.file_patterns` For entries with `user_specified_type`: * `schema` *
* `display_name` * `description` * `user_specified_type` * `user_specified_system` *
* `linked_resource` * `source_system_timestamps`
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Marks an Entry as starred by the current user. Starring information is private to each user.
*
* Create a request for the method "entries.star".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Star#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entry to mark as starred.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1StarEntryRequest}
* @return the request
*/
public Star star(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1StarEntryRequest content) throws java.io.IOException {
Star result = new Star(name, content);
initialize(result);
return result;
}
public class Star extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:star";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Marks an Entry as starred by the current user. Starring information is private to each user.
*
* Create a request for the method "entries.star".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Star#execute()} method to invoke the remote operation.
* {@link Star#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entry to mark as starred.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1StarEntryRequest}
* @since 1.13
*/
protected Star(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1StarEntryRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1StarEntryResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public Star set$Xgafv(java.lang.String $Xgafv) {
return (Star) super.set$Xgafv($Xgafv);
}
@Override
public Star setAccessToken(java.lang.String accessToken) {
return (Star) super.setAccessToken(accessToken);
}
@Override
public Star setAlt(java.lang.String alt) {
return (Star) super.setAlt(alt);
}
@Override
public Star setCallback(java.lang.String callback) {
return (Star) super.setCallback(callback);
}
@Override
public Star setFields(java.lang.String fields) {
return (Star) super.setFields(fields);
}
@Override
public Star setKey(java.lang.String key) {
return (Star) super.setKey(key);
}
@Override
public Star setOauthToken(java.lang.String oauthToken) {
return (Star) super.setOauthToken(oauthToken);
}
@Override
public Star setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Star) super.setPrettyPrint(prettyPrint);
}
@Override
public Star setQuotaUser(java.lang.String quotaUser) {
return (Star) super.setQuotaUser(quotaUser);
}
@Override
public Star setUploadType(java.lang.String uploadType) {
return (Star) super.setUploadType(uploadType);
}
@Override
public Star setUploadProtocol(java.lang.String uploadProtocol) {
return (Star) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the entry to mark as starred. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entry to mark as starred.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the entry to mark as starred. */
public Star setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Star set(String parameterName, Object value) {
return (Star) super.set(parameterName, value);
}
}
/**
* Gets your permissions on a resource. Returns an empty set of permissions if the resource doesn't
* exist. Supported resources are: - Tag templates - Entry groups Note: This method gets policies
* only within Data Catalog and can't be used to get policies from BigQuery, Pub/Sub, Dataproc
* Metastore, and any external Google Cloud Platform resources ingested into Data Catalog. No Google
* IAM permissions are required to call this method.
*
* Create a request for the method "entries.testIamPermissions".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Gets your permissions on a resource. Returns an empty set of permissions if the resource
* doesn't exist. Supported resources are: - Tag templates - Entry groups Note: This method gets
* policies only within Data Catalog and can't be used to get policies from BigQuery, Pub/Sub,
* Dataproc Metastore, and any external Google Cloud Platform resources ingested into Data
* Catalog. No Google IAM permissions are required to call this method.
*
* Create a request for the method "entries.testIamPermissions".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Marks an Entry as NOT starred by the current user. Starring information is private to each user.
*
* Create a request for the method "entries.unstar".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Unstar#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entry to mark as **not** starred.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1UnstarEntryRequest}
* @return the request
*/
public Unstar unstar(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1UnstarEntryRequest content) throws java.io.IOException {
Unstar result = new Unstar(name, content);
initialize(result);
return result;
}
public class Unstar extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:unstar";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Marks an Entry as NOT starred by the current user. Starring information is private to each
* user.
*
* Create a request for the method "entries.unstar".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Unstar#execute()} method to invoke the remote operation.
* {@link
* Unstar#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entry to mark as **not** starred.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1UnstarEntryRequest}
* @since 1.13
*/
protected Unstar(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1UnstarEntryRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1UnstarEntryResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public Unstar set$Xgafv(java.lang.String $Xgafv) {
return (Unstar) super.set$Xgafv($Xgafv);
}
@Override
public Unstar setAccessToken(java.lang.String accessToken) {
return (Unstar) super.setAccessToken(accessToken);
}
@Override
public Unstar setAlt(java.lang.String alt) {
return (Unstar) super.setAlt(alt);
}
@Override
public Unstar setCallback(java.lang.String callback) {
return (Unstar) super.setCallback(callback);
}
@Override
public Unstar setFields(java.lang.String fields) {
return (Unstar) super.setFields(fields);
}
@Override
public Unstar setKey(java.lang.String key) {
return (Unstar) super.setKey(key);
}
@Override
public Unstar setOauthToken(java.lang.String oauthToken) {
return (Unstar) super.setOauthToken(oauthToken);
}
@Override
public Unstar setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unstar) super.setPrettyPrint(prettyPrint);
}
@Override
public Unstar setQuotaUser(java.lang.String quotaUser) {
return (Unstar) super.setQuotaUser(quotaUser);
}
@Override
public Unstar setUploadType(java.lang.String uploadType) {
return (Unstar) super.setUploadType(uploadType);
}
@Override
public Unstar setUploadProtocol(java.lang.String uploadProtocol) {
return (Unstar) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the entry to mark as **not** starred. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entry to mark as **not** starred.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the entry to mark as **not** starred. */
public Unstar setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Unstar set(String parameterName, Object value) {
return (Unstar) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Tags collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Tags.List request = datacatalog.tags().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tags tags() {
return new Tags();
}
/**
* The "tags" collection of methods.
*/
public class Tags {
/**
* Creates a tag and assigns it to: * An Entry if the method name is
* `projects.locations.entryGroups.entries.tags.create`. * Or EntryGroupif the method name is
* `projects.locations.entryGroups.tags.create`. Note: The project identified by the `parent`
* parameter for the [tag] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries.tags/create#path-
* parameters) and the [tag template] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.tagTemplates/create#path-parameters) used to
* create the tag must be in the same organization.
*
* Create a request for the method "tags.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the resource to attach this tag to. Tags can be attached to entries or entry
* groups. An entry can have up to 1000 attached tags. Note: The tag and its child resources
* might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/tags";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Creates a tag and assigns it to: * An Entry if the method name is
* `projects.locations.entryGroups.entries.tags.create`. * Or EntryGroupif the method name is
* `projects.locations.entryGroups.tags.create`. Note: The project identified by the `parent`
* parameter for the [tag] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries.tags/create#path-
* parameters) and the [tag template] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.tagTemplates/create#path-parameters) used to
* create the tag must be in the same organization.
*
* Create a request for the method "tags.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the resource to attach this tag to. Tags can be attached to entries or entry
* groups. An entry can have up to 1000 attached tags. Note: The tag and its child resources
* might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource to attach this tag to. Tags can be attached to
* entries or entry groups. An entry can have up to 1000 attached tags. Note: The tag
* and its child resources might not be stored in the location specified in its name.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the resource to attach this tag to. Tags can be attached to entries or entry
groups. An entry can have up to 1000 attached tags. Note: The tag and its child resources might not
be stored in the location specified in its name.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the resource to attach this tag to. Tags can be attached to
* entries or entry groups. An entry can have up to 1000 attached tags. Note: The tag
* and its child resources might not be stored in the location specified in its name.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a tag.
*
* Create a request for the method "tags.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the tag to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+/tags/[^/]+$");
/**
* Deletes a tag.
*
* Create a request for the method "tags.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the tag to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+/tags/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the tag to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the tag to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the tag to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+/tags/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists tags assigned to an Entry. The columns in the response are lowercased.
*
* Create a request for the method "tags.list".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the Data Catalog resource to list the tags of. The resource can be an Entry or
* an EntryGroup (without `/entries/{entries}` at the end).
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/tags";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* Lists tags assigned to an Entry. The columns in the response are lowercased.
*
* Create a request for the method "tags.list".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the Data Catalog resource to list the tags of. The resource can be an Entry or
* an EntryGroup (without `/entries/{entries}` at the end).
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ListTagsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Data Catalog resource to list the tags of. The resource
* can be an Entry or an EntryGroup (without `/entries/{entries}` at the end).
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the Data Catalog resource to list the tags of. The resource can be an Entry
or an EntryGroup (without `/entries/{entries}` at the end).
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the Data Catalog resource to list the tags of. The resource
* can be an Entry or an EntryGroup (without `/entries/{entries}` at the end).
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of tags to return. Default is 10. Maximum limit is 1000. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of tags to return. Default is 10. Maximum limit is 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of tags to return. Default is 10. Maximum limit is 1000. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Pagination token that specifies the next page to return. If empty, the first page
* is returned.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Pagination token that specifies the next page to return. If empty, the first page is returned.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Pagination token that specifies the next page to return. If empty, the first page
* is returned.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing tag.
*
* Create a request for the method "tags.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. The resource name of the tag in URL format where tag ID is a system-generated
* identifier. Note: The tag itself might not be stored in the location specified in its
* name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+/tags/[^/]+$");
/**
* Updates an existing tag.
*
* Create a request for the method "tags.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. The resource name of the tag in URL format where tag ID is a system-generated
* identifier. Note: The tag itself might not be stored in the location specified in its
* name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+/tags/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. The resource name of the tag in URL format where tag ID is a system-
* generated identifier. Note: The tag itself might not be stored in the location
* specified in its name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. The resource name of the tag in URL format where tag ID is a system-generated
identifier. Note: The tag itself might not be stored in the location specified in its name.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. The resource name of the tag in URL format where tag ID is a system-
* generated identifier. Note: The tag itself might not be stored in the location
* specified in its name.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+/tags/[^/]+$");
}
this.name = name;
return this;
}
/**
* Names of fields whose values to overwrite on a tag. Currently, a tag has the only
* modifiable field with the name `fields`. In general, if this parameter is absent or
* empty, all modifiable fields are overwritten. If such fields are non-required and
* omitted in the request body, their values are emptied.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Names of fields whose values to overwrite on a tag. Currently, a tag has the only modifiable field
with the name `fields`. In general, if this parameter is absent or empty, all modifiable fields are
overwritten. If such fields are non-required and omitted in the request body, their values are
emptied.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Names of fields whose values to overwrite on a tag. Currently, a tag has the only
* modifiable field with the name `fields`. In general, if this parameter is absent or
* empty, all modifiable fields are overwritten. If such fields are non-required and
* omitted in the request body, their values are emptied.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* `ReconcileTags` creates or updates a list of tags on the entry. If the
* ReconcileTagsRequest.force_delete_missing parameter is set, the operation deletes tags not
* included in the input tag list. `ReconcileTags` returns a long-running operation resource that
* can be queried with Operations.GetOperation to return ReconcileTagsMetadata and a
* ReconcileTagsResponse message.
*
* Create a request for the method "tags.reconcile".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Reconcile#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of Entry to be tagged.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReconcileTagsRequest}
* @return the request
*/
public Reconcile reconcile(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReconcileTagsRequest content) throws java.io.IOException {
Reconcile result = new Reconcile(parent, content);
initialize(result);
return result;
}
public class Reconcile extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/tags:reconcile";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
/**
* `ReconcileTags` creates or updates a list of tags on the entry. If the
* ReconcileTagsRequest.force_delete_missing parameter is set, the operation deletes tags not
* included in the input tag list. `ReconcileTags` returns a long-running operation resource that
* can be queried with Operations.GetOperation to return ReconcileTagsMetadata and a
* ReconcileTagsResponse message.
*
* Create a request for the method "tags.reconcile".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Reconcile#execute()} method to invoke the remote
* operation. {@link
* Reconcile#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of Entry to be tagged.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReconcileTagsRequest}
* @since 1.13
*/
protected Reconcile(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReconcileTagsRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
}
@Override
public Reconcile set$Xgafv(java.lang.String $Xgafv) {
return (Reconcile) super.set$Xgafv($Xgafv);
}
@Override
public Reconcile setAccessToken(java.lang.String accessToken) {
return (Reconcile) super.setAccessToken(accessToken);
}
@Override
public Reconcile setAlt(java.lang.String alt) {
return (Reconcile) super.setAlt(alt);
}
@Override
public Reconcile setCallback(java.lang.String callback) {
return (Reconcile) super.setCallback(callback);
}
@Override
public Reconcile setFields(java.lang.String fields) {
return (Reconcile) super.setFields(fields);
}
@Override
public Reconcile setKey(java.lang.String key) {
return (Reconcile) super.setKey(key);
}
@Override
public Reconcile setOauthToken(java.lang.String oauthToken) {
return (Reconcile) super.setOauthToken(oauthToken);
}
@Override
public Reconcile setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Reconcile) super.setPrettyPrint(prettyPrint);
}
@Override
public Reconcile setQuotaUser(java.lang.String quotaUser) {
return (Reconcile) super.setQuotaUser(quotaUser);
}
@Override
public Reconcile setUploadType(java.lang.String uploadType) {
return (Reconcile) super.setUploadType(uploadType);
}
@Override
public Reconcile setUploadProtocol(java.lang.String uploadProtocol) {
return (Reconcile) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of Entry to be tagged. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of Entry to be tagged.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Name of Entry to be tagged. */
public Reconcile setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/entries/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Reconcile set(String parameterName, Object value) {
return (Reconcile) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Tags collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Tags.List request = datacatalog.tags().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tags tags() {
return new Tags();
}
/**
* The "tags" collection of methods.
*/
public class Tags {
/**
* Creates a tag and assigns it to: * An Entry if the method name is
* `projects.locations.entryGroups.entries.tags.create`. * Or EntryGroupif the method name is
* `projects.locations.entryGroups.tags.create`. Note: The project identified by the `parent`
* parameter for the [tag] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries.tags/create#path-
* parameters) and the [tag template] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.tagTemplates/create#path-parameters) used to
* create the tag must be in the same organization.
*
* Create a request for the method "tags.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the resource to attach this tag to. Tags can be attached to entries or entry
* groups. An entry can have up to 1000 attached tags. Note: The tag and its child resources
* might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/tags";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Creates a tag and assigns it to: * An Entry if the method name is
* `projects.locations.entryGroups.entries.tags.create`. * Or EntryGroupif the method name is
* `projects.locations.entryGroups.tags.create`. Note: The project identified by the `parent`
* parameter for the [tag] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.entryGroups.entries.tags/create#path-
* parameters) and the [tag template] (https://cloud.google.com/data-
* catalog/docs/reference/rest/v1/projects.locations.tagTemplates/create#path-parameters) used to
* create the tag must be in the same organization.
*
* Create a request for the method "tags.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the resource to attach this tag to. Tags can be attached to entries or entry
* groups. An entry can have up to 1000 attached tags. Note: The tag and its child resources
* might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource to attach this tag to. Tags can be attached to
* entries or entry groups. An entry can have up to 1000 attached tags. Note: The tag
* and its child resources might not be stored in the location specified in its name.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the resource to attach this tag to. Tags can be attached to entries or entry
groups. An entry can have up to 1000 attached tags. Note: The tag and its child resources might not
be stored in the location specified in its name.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the resource to attach this tag to. Tags can be attached to
* entries or entry groups. An entry can have up to 1000 attached tags. Note: The tag
* and its child resources might not be stored in the location specified in its name.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a tag.
*
* Create a request for the method "tags.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the tag to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/tags/[^/]+$");
/**
* Deletes a tag.
*
* Create a request for the method "tags.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the tag to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/tags/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the tag to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the tag to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the tag to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/tags/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists tags assigned to an Entry. The columns in the response are lowercased.
*
* Create a request for the method "tags.list".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the Data Catalog resource to list the tags of. The resource can be an Entry or
* an EntryGroup (without `/entries/{entries}` at the end).
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/tags";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
/**
* Lists tags assigned to an Entry. The columns in the response are lowercased.
*
* Create a request for the method "tags.list".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the Data Catalog resource to list the tags of. The resource can be an Entry or
* an EntryGroup (without `/entries/{entries}` at the end).
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ListTagsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Data Catalog resource to list the tags of. The resource can
* be an Entry or an EntryGroup (without `/entries/{entries}` at the end).
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the Data Catalog resource to list the tags of. The resource can be an Entry
or an EntryGroup (without `/entries/{entries}` at the end).
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the Data Catalog resource to list the tags of. The resource can
* be an Entry or an EntryGroup (without `/entries/{entries}` at the end).
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of tags to return. Default is 10. Maximum limit is 1000. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of tags to return. Default is 10. Maximum limit is 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of tags to return. Default is 10. Maximum limit is 1000. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Pagination token that specifies the next page to return. If empty, the first page is
* returned.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Pagination token that specifies the next page to return. If empty, the first page is returned.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Pagination token that specifies the next page to return. If empty, the first page is
* returned.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing tag.
*
* Create a request for the method "tags.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. The resource name of the tag in URL format where tag ID is a system-generated
* identifier. Note: The tag itself might not be stored in the location specified in its
* name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/tags/[^/]+$");
/**
* Updates an existing tag.
*
* Create a request for the method "tags.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. The resource name of the tag in URL format where tag ID is a system-generated
* identifier. Note: The tag itself might not be stored in the location specified in its
* name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Tag.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/tags/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. The resource name of the tag in URL format where tag ID is a system-
* generated identifier. Note: The tag itself might not be stored in the location
* specified in its name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. The resource name of the tag in URL format where tag ID is a system-generated
identifier. Note: The tag itself might not be stored in the location specified in its name.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. The resource name of the tag in URL format where tag ID is a system-
* generated identifier. Note: The tag itself might not be stored in the location
* specified in its name.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/entryGroups/[^/]+/tags/[^/]+$");
}
this.name = name;
return this;
}
/**
* Names of fields whose values to overwrite on a tag. Currently, a tag has the only
* modifiable field with the name `fields`. In general, if this parameter is absent or
* empty, all modifiable fields are overwritten. If such fields are non-required and
* omitted in the request body, their values are emptied.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Names of fields whose values to overwrite on a tag. Currently, a tag has the only modifiable field
with the name `fields`. In general, if this parameter is absent or empty, all modifiable fields are
overwritten. If such fields are non-required and omitted in the request body, their values are
emptied.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Names of fields whose values to overwrite on a tag. Currently, a tag has the only
* modifiable field with the name `fields`. In general, if this parameter is absent or
* empty, all modifiable fields are overwritten. If such fields are non-required and
* omitted in the request body, their values are emptied.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Operations.List request = datacatalog.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of `1`, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of `1`, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(DataCatalog.this, "POST", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the TagTemplates collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.TagTemplates.List request = datacatalog.tagTemplates().list(parameters ...)}
*
*
* @return the resource collection
*/
public TagTemplates tagTemplates() {
return new TagTemplates();
}
/**
* The "tagTemplates" collection of methods.
*/
public class TagTemplates {
/**
* Creates a tag template. You must enable the Data Catalog API in the project identified by the
* `parent` parameter. For more information, see [Data Catalog resource project]
* (https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "tagTemplates.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project and the template location [region](https://cloud.google.com/data-
* catalog/docs/concepts/regions).
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/tagTemplates";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a tag template. You must enable the Data Catalog API in the project identified by the
* `parent` parameter. For more information, see [Data Catalog resource project]
* (https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "tagTemplates.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project and the template location [region](https://cloud.google.com/data-
* catalog/docs/concepts/regions).
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project and the template location
* [region](https://cloud.google.com/data-catalog/docs/concepts/regions).
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project and the template location [region](https://cloud.google.com/data-
catalog/docs/concepts/regions).
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project and the template location
* [region](https://cloud.google.com/data-catalog/docs/concepts/regions).
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID of the tag template to create. The ID must contain only lowercase
* letters (a-z), numbers (0-9), or underscores (_), and must start with a letter or
* underscore. The maximum size is 64 bytes when encoded in UTF-8.
*/
@com.google.api.client.util.Key
private java.lang.String tagTemplateId;
/** Required. The ID of the tag template to create. The ID must contain only lowercase letters (a-z),
numbers (0-9), or underscores (_), and must start with a letter or underscore. The maximum size is
64 bytes when encoded in UTF-8.
*/
public java.lang.String getTagTemplateId() {
return tagTemplateId;
}
/**
* Required. The ID of the tag template to create. The ID must contain only lowercase
* letters (a-z), numbers (0-9), or underscores (_), and must start with a letter or
* underscore. The maximum size is 64 bytes when encoded in UTF-8.
*/
public Create setTagTemplateId(java.lang.String tagTemplateId) {
this.tagTemplateId = tagTemplateId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a tag template and all tags that use it. You must enable the Data Catalog API in the
* project identified by the `name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "tagTemplates.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the tag template to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
/**
* Deletes a tag template and all tags that use it. You must enable the Data Catalog API in the
* project identified by the `name` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "tagTemplates.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the tag template to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the tag template to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the tag template to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the tag template to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. If true, deletes all tags that use this template. Currently, `true` is the
* only supported value.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Required. If true, deletes all tags that use this template. Currently, `true` is the only supported
value.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Required. If true, deletes all tags that use this template. Currently, `true` is the
* only supported value.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a tag template.
*
* Create a request for the method "tagTemplates.get".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the tag template to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
/**
* Gets a tag template.
*
* Create a request for the method "tagTemplates.get".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the tag template to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the tag template to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the tag template to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the tag template to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. May return: * A`NOT_FOUND` error if the resource
* doesn't exist or you don't have the permission to view it. * An empty policy if the resource
* exists but doesn't have a set policy. Supported resources are: - Tag templates - Entry groups
* Note: This method doesn't get policies from Google Cloud Platform resources ingested into Data
* Catalog. To call this method, you must have the following Google IAM permissions: -
* `datacatalog.tagTemplates.getIamPolicy` to get policies on tag templates. -
* `datacatalog.entryGroups.getIamPolicy` to get policies on entry groups.
*
* Create a request for the method "tagTemplates.getIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
/**
* Gets the access control policy for a resource. May return: * A`NOT_FOUND` error if the resource
* doesn't exist or you don't have the permission to view it. * An empty policy if the resource
* exists but doesn't have a set policy. Supported resources are: - Tag templates - Entry groups
* Note: This method doesn't get policies from Google Cloud Platform resources ingested into Data
* Catalog. To call this method, you must have the following Google IAM permissions: -
* `datacatalog.tagTemplates.getIamPolicy` to get policies on tag templates. -
* `datacatalog.entryGroups.getIamPolicy` to get policies on entry groups.
*
* Create a request for the method "tagTemplates.getIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Updates a tag template. You can't update template fields with this method. These fields are
* separate resources with their own create, update, and delete methods. You must enable the Data
* Catalog API in the project identified by the `tag_template.name` parameter. For more information,
* see [Data Catalog resource project](https://cloud.google.com/data-catalog/docs/concepts/resource-
* project).
*
* Create a request for the method "tagTemplates.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. The resource name of the tag template in URL format. Note: The tag template itself and
* its child resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
/**
* Updates a tag template. You can't update template fields with this method. These fields are
* separate resources with their own create, update, and delete methods. You must enable the Data
* Catalog API in the project identified by the `tag_template.name` parameter. For more
* information, see [Data Catalog resource project](https://cloud.google.com/data-
* catalog/docs/concepts/resource-project).
*
* Create a request for the method "tagTemplates.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. The resource name of the tag template in URL format. Note: The tag template itself and
* its child resources might not be stored in the location specified in its name.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplate.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. The resource name of the tag template in URL format. Note: The tag template
* itself and its child resources might not be stored in the location specified in its
* name.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. The resource name of the tag template in URL format. Note: The tag template itself and
its child resources might not be stored in the location specified in its name.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. The resource name of the tag template in URL format. Note: The tag template
* itself and its child resources might not be stored in the location specified in its
* name.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Names of fields whose values to overwrite on a tag template. Currently, only
* `display_name` and `is_publicly_readable` can be overwritten. If this parameter is
* absent or empty, all modifiable fields are overwritten. If such fields are non-required
* and omitted in the request body, their values are emptied. Note: Updating the
* `is_publicly_readable` field may require up to 12 hours to take effect in search
* results.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Names of fields whose values to overwrite on a tag template. Currently, only `display_name` and
`is_publicly_readable` can be overwritten. If this parameter is absent or empty, all modifiable
fields are overwritten. If such fields are non-required and omitted in the request body, their
values are emptied. Note: Updating the `is_publicly_readable` field may require up to 12 hours to
take effect in search results.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Names of fields whose values to overwrite on a tag template. Currently, only
* `display_name` and `is_publicly_readable` can be overwritten. If this parameter is
* absent or empty, all modifiable fields are overwritten. If such fields are non-required
* and omitted in the request body, their values are emptied. Note: Updating the
* `is_publicly_readable` field may require up to 12 hours to take effect in search
* results.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets an access control policy for a resource. Replaces any existing policy. Supported resources
* are: - Tag templates - Entry groups Note: This method sets policies only within Data Catalog and
* can't be used to manage policies in BigQuery, Pub/Sub, Dataproc Metastore, and any external
* Google Cloud Platform resources synced with the Data Catalog. To call this method, you must have
* the following Google IAM permissions: - `datacatalog.tagTemplates.setIamPolicy` to set policies
* on tag templates. - `datacatalog.entryGroups.setIamPolicy` to set policies on entry groups.
*
* Create a request for the method "tagTemplates.setIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
/**
* Sets an access control policy for a resource. Replaces any existing policy. Supported resources
* are: - Tag templates - Entry groups Note: This method sets policies only within Data Catalog
* and can't be used to manage policies in BigQuery, Pub/Sub, Dataproc Metastore, and any external
* Google Cloud Platform resources synced with the Data Catalog. To call this method, you must
* have the following Google IAM permissions: - `datacatalog.tagTemplates.setIamPolicy` to set
* policies on tag templates. - `datacatalog.entryGroups.setIamPolicy` to set policies on entry
* groups.
*
* Create a request for the method "tagTemplates.setIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Gets your permissions on a resource. Returns an empty set of permissions if the resource doesn't
* exist. Supported resources are: - Tag templates - Entry groups Note: This method gets policies
* only within Data Catalog and can't be used to get policies from BigQuery, Pub/Sub, Dataproc
* Metastore, and any external Google Cloud Platform resources ingested into Data Catalog. No Google
* IAM permissions are required to call this method.
*
* Create a request for the method "tagTemplates.testIamPermissions".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
/**
* Gets your permissions on a resource. Returns an empty set of permissions if the resource
* doesn't exist. Supported resources are: - Tag templates - Entry groups Note: This method gets
* policies only within Data Catalog and can't be used to get policies from BigQuery, Pub/Sub,
* Dataproc Metastore, and any external Google Cloud Platform resources ingested into Data
* Catalog. No Google IAM permissions are required to call this method.
*
* Create a request for the method "tagTemplates.testIamPermissions".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Fields collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Fields.List request = datacatalog.fields().list(parameters ...)}
*
*
* @return the resource collection
*/
public Fields fields() {
return new Fields();
}
/**
* The "fields" collection of methods.
*/
public class Fields {
/**
* Creates a field in a tag template. You must enable the Data Catalog API in the project identified
* by the `parent` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the project and the template location [region](https://cloud.google.com/data-
* catalog/docs/concepts/regions).
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/fields";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
/**
* Creates a field in a tag template. You must enable the Data Catalog API in the project
* identified by the `parent` parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the project and the template location [region](https://cloud.google.com/data-
* catalog/docs/concepts/regions).
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the project and the template location
* [region](https://cloud.google.com/data-catalog/docs/concepts/regions).
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the project and the template location [region](https://cloud.google.com/data-
catalog/docs/concepts/regions).
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the project and the template location
* [region](https://cloud.google.com/data-catalog/docs/concepts/regions).
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID of the tag template field to create. Note: Adding a required field
* to an existing template is *not* allowed. Field IDs can contain letters (both
* uppercase and lowercase), numbers (0-9), underscores (_) and dashes (-). Field IDs
* must be at least 1 character long and at most 128 characters long. Field IDs must
* also be unique within their template.
*/
@com.google.api.client.util.Key
private java.lang.String tagTemplateFieldId;
/** Required. The ID of the tag template field to create. Note: Adding a required field to an existing
template is *not* allowed. Field IDs can contain letters (both uppercase and lowercase), numbers
(0-9), underscores (_) and dashes (-). Field IDs must be at least 1 character long and at most 128
characters long. Field IDs must also be unique within their template.
*/
public java.lang.String getTagTemplateFieldId() {
return tagTemplateFieldId;
}
/**
* Required. The ID of the tag template field to create. Note: Adding a required field
* to an existing template is *not* allowed. Field IDs can contain letters (both
* uppercase and lowercase), numbers (0-9), underscores (_) and dashes (-). Field IDs
* must be at least 1 character long and at most 128 characters long. Field IDs must
* also be unique within their template.
*/
public Create setTagTemplateFieldId(java.lang.String tagTemplateFieldId) {
this.tagTemplateFieldId = tagTemplateFieldId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a field in a tag template and all uses of this field from the tags based on this
* template. You must enable the Data Catalog API in the project identified by the `name` parameter.
* For more information, see [Data Catalog resource project](https://cloud.google.com/data-
* catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the tag template field to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
/**
* Deletes a field in a tag template and all uses of this field from the tags based on this
* template. You must enable the Data Catalog API in the project identified by the `name`
* parameter. For more information, see [Data Catalog resource
* project](https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the tag template field to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the tag template field to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the tag template field to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the tag template field to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. If true, deletes this field from any tags that use it. Currently, `true` is
* the only supported value.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Required. If true, deletes this field from any tags that use it. Currently, `true` is the only
supported value.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Required. If true, deletes this field from any tags that use it. Currently, `true` is
* the only supported value.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Updates a field in a tag template. You can't update the field type with this method. You must
* enable the Data Catalog API in the project identified by the `name` parameter. For more
* information, see [Data Catalog resource project](https://cloud.google.com/data-
* catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the tag template field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
/**
* Updates a field in a tag template. You can't update the field type with this method. You must
* enable the Data Catalog API in the project identified by the `name` parameter. For more
* information, see [Data Catalog resource project](https://cloud.google.com/data-
* catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the tag template field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the tag template field. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the tag template field.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the tag template field. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Names of fields whose values to overwrite on an individual field of a tag
* template. The following fields are modifiable: * `display_name` * `type.enum_type` *
* `is_required` If this parameter is absent or empty, all modifiable fields are
* overwritten. If such fields are non-required and omitted in the request body, their
* values are emptied with one exception: when updating an enum type, the provided
* values are merged with the existing values. Therefore, enum values can only be added,
* existing enum values cannot be deleted or renamed. Additionally, updating a template
* field from optional to required is *not* allowed.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. Names of fields whose values to overwrite on an individual field of a tag template. The
following fields are modifiable: * `display_name` * `type.enum_type` * `is_required` If this
parameter is absent or empty, all modifiable fields are overwritten. If such fields are non-
required and omitted in the request body, their values are emptied with one exception: when
updating an enum type, the provided values are merged with the existing values. Therefore, enum
values can only be added, existing enum values cannot be deleted or renamed. Additionally, updating
a template field from optional to required is *not* allowed.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. Names of fields whose values to overwrite on an individual field of a tag
* template. The following fields are modifiable: * `display_name` * `type.enum_type` *
* `is_required` If this parameter is absent or empty, all modifiable fields are
* overwritten. If such fields are non-required and omitted in the request body, their
* values are emptied with one exception: when updating an enum type, the provided
* values are merged with the existing values. Therefore, enum values can only be added,
* existing enum values cannot be deleted or renamed. Additionally, updating a template
* field from optional to required is *not* allowed.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Renames a field in a tag template. You must enable the Data Catalog API in the project identified
* by the `name` parameter. For more information, see [Data Catalog resource project]
* (https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.rename".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Rename#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the tag template field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldRequest}
* @return the request
*/
public Rename rename(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldRequest content) throws java.io.IOException {
Rename result = new Rename(name, content);
initialize(result);
return result;
}
public class Rename extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:rename";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
/**
* Renames a field in a tag template. You must enable the Data Catalog API in the project
* identified by the `name` parameter. For more information, see [Data Catalog resource project]
* (https://cloud.google.com/data-catalog/docs/concepts/resource-project).
*
* Create a request for the method "fields.rename".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Rename#execute()} method to invoke the remote operation.
* {@link
* Rename#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the tag template field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldRequest}
* @since 1.13
*/
protected Rename(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
}
}
@Override
public Rename set$Xgafv(java.lang.String $Xgafv) {
return (Rename) super.set$Xgafv($Xgafv);
}
@Override
public Rename setAccessToken(java.lang.String accessToken) {
return (Rename) super.setAccessToken(accessToken);
}
@Override
public Rename setAlt(java.lang.String alt) {
return (Rename) super.setAlt(alt);
}
@Override
public Rename setCallback(java.lang.String callback) {
return (Rename) super.setCallback(callback);
}
@Override
public Rename setFields(java.lang.String fields) {
return (Rename) super.setFields(fields);
}
@Override
public Rename setKey(java.lang.String key) {
return (Rename) super.setKey(key);
}
@Override
public Rename setOauthToken(java.lang.String oauthToken) {
return (Rename) super.setOauthToken(oauthToken);
}
@Override
public Rename setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rename) super.setPrettyPrint(prettyPrint);
}
@Override
public Rename setQuotaUser(java.lang.String quotaUser) {
return (Rename) super.setQuotaUser(quotaUser);
}
@Override
public Rename setUploadType(java.lang.String uploadType) {
return (Rename) super.setUploadType(uploadType);
}
@Override
public Rename setUploadProtocol(java.lang.String uploadProtocol) {
return (Rename) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the tag template field. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the tag template field.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the tag template field. */
public Rename setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Rename set(String parameterName, Object value) {
return (Rename) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the EnumValues collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.EnumValues.List request = datacatalog.enumValues().list(parameters ...)}
*
*
* @return the resource collection
*/
public EnumValues enumValues() {
return new EnumValues();
}
/**
* The "enumValues" collection of methods.
*/
public class EnumValues {
/**
* Renames an enum value in a tag template. Within a single enum field, enum values must be unique.
*
* Create a request for the method "enumValues.rename".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Rename#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the enum field value.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldEnumValueRequest}
* @return the request
*/
public Rename rename(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldEnumValueRequest content) throws java.io.IOException {
Rename result = new Rename(name, content);
initialize(result);
return result;
}
public class Rename extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:rename";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+/enumValues/[^/]+$");
/**
* Renames an enum value in a tag template. Within a single enum field, enum values must be
* unique.
*
* Create a request for the method "enumValues.rename".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Rename#execute()} method to invoke the remote operation.
* {@link
* Rename#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the enum field value.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldEnumValueRequest}
* @since 1.13
*/
protected Rename(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1RenameTagTemplateFieldEnumValueRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1TagTemplateField.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+/enumValues/[^/]+$");
}
}
@Override
public Rename set$Xgafv(java.lang.String $Xgafv) {
return (Rename) super.set$Xgafv($Xgafv);
}
@Override
public Rename setAccessToken(java.lang.String accessToken) {
return (Rename) super.setAccessToken(accessToken);
}
@Override
public Rename setAlt(java.lang.String alt) {
return (Rename) super.setAlt(alt);
}
@Override
public Rename setCallback(java.lang.String callback) {
return (Rename) super.setCallback(callback);
}
@Override
public Rename setFields(java.lang.String fields) {
return (Rename) super.setFields(fields);
}
@Override
public Rename setKey(java.lang.String key) {
return (Rename) super.setKey(key);
}
@Override
public Rename setOauthToken(java.lang.String oauthToken) {
return (Rename) super.setOauthToken(oauthToken);
}
@Override
public Rename setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rename) super.setPrettyPrint(prettyPrint);
}
@Override
public Rename setQuotaUser(java.lang.String quotaUser) {
return (Rename) super.setQuotaUser(quotaUser);
}
@Override
public Rename setUploadType(java.lang.String uploadType) {
return (Rename) super.setUploadType(uploadType);
}
@Override
public Rename setUploadProtocol(java.lang.String uploadProtocol) {
return (Rename) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the enum field value. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the enum field value.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the enum field value. */
public Rename setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/tagTemplates/[^/]+/fields/[^/]+/enumValues/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Rename set(String parameterName, Object value) {
return (Rename) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Taxonomies collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.Taxonomies.List request = datacatalog.taxonomies().list(parameters ...)}
*
*
* @return the resource collection
*/
public Taxonomies taxonomies() {
return new Taxonomies();
}
/**
* The "taxonomies" collection of methods.
*/
public class Taxonomies {
/**
* Creates a taxonomy in a specified project. The taxonomy is initially empty, that is, it doesn't
* contain policy tags.
*
* Create a request for the method "taxonomies.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the project that the taxonomy will belong to.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/taxonomies";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a taxonomy in a specified project. The taxonomy is initially empty, that is, it doesn't
* contain policy tags.
*
* Create a request for the method "taxonomies.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the project that the taxonomy will belong to.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the project that the taxonomy will belong to. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the project that the taxonomy will belong to.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Resource name of the project that the taxonomy will belong to. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a taxonomy, including all policy tags in this taxonomy, their associated policies, and
* the policy tags references from BigQuery columns.
*
* Create a request for the method "taxonomies.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the taxonomy to delete. Note: All policy tags in this taxonomy are also
* deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Deletes a taxonomy, including all policy tags in this taxonomy, their associated policies, and
* the policy tags references from BigQuery columns.
*
* Create a request for the method "taxonomies.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the taxonomy to delete. Note: All policy tags in this taxonomy are also
* deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the taxonomy to delete. Note: All policy tags in this
* taxonomy are also deleted.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the taxonomy to delete. Note: All policy tags in this taxonomy are also
deleted.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the taxonomy to delete. Note: All policy tags in this
* taxonomy are also deleted.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Exports taxonomies in the requested type and returns them, including their policy tags. The
* requested taxonomies must belong to the same project. This method generates `SerializedTaxonomy`
* protocol buffers with nested policy tags that can be used as input for `ImportTaxonomies` calls.
*
* Create a request for the method "taxonomies.export".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the project that the exported taxonomies belong to.
* @return the request
*/
public Export export(java.lang.String parent) throws java.io.IOException {
Export result = new Export(parent);
initialize(result);
return result;
}
public class Export extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/taxonomies:export";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Exports taxonomies in the requested type and returns them, including their policy tags. The
* requested taxonomies must belong to the same project. This method generates
* `SerializedTaxonomy` protocol buffers with nested policy tags that can be used as input for
* `ImportTaxonomies` calls.
*
* Create a request for the method "taxonomies.export".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the project that the exported taxonomies belong to.
* @since 1.13
*/
protected Export(java.lang.String parent) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ExportTaxonomiesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the project that the exported taxonomies belong to. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the project that the exported taxonomies belong to.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Resource name of the project that the exported taxonomies belong to. */
public Export setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Serialized export taxonomies that contain all the policy tags as nested protocol
* buffers.
*/
@com.google.api.client.util.Key
private java.lang.Boolean serializedTaxonomies;
/** Serialized export taxonomies that contain all the policy tags as nested protocol buffers.
*/
public java.lang.Boolean getSerializedTaxonomies() {
return serializedTaxonomies;
}
/**
* Serialized export taxonomies that contain all the policy tags as nested protocol
* buffers.
*/
public Export setSerializedTaxonomies(java.lang.Boolean serializedTaxonomies) {
this.serializedTaxonomies = serializedTaxonomies;
return this;
}
/** Required. Resource names of the taxonomies to export. */
@com.google.api.client.util.Key
private java.util.List taxonomies;
/** Required. Resource names of the taxonomies to export.
*/
public java.util.List getTaxonomies() {
return taxonomies;
}
/** Required. Resource names of the taxonomies to export. */
public Export setTaxonomies(java.util.List taxonomies) {
this.taxonomies = taxonomies;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Gets a taxonomy.
*
* Create a request for the method "taxonomies.get".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the taxonomy to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Gets a taxonomy.
*
* Create a request for the method "taxonomies.get".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the taxonomy to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the taxonomy to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the taxonomy to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. Resource name of the taxonomy to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "taxonomies.getIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Gets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "taxonomies.getIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Creates new taxonomies (including their policy tags) in a given project by importing from inlined
* or cross-regional sources. For a cross-regional source, new taxonomies are created by copying
* from a source in another region. For an inlined source, taxonomies and policy tags are created in
* bulk using nested protocol buffer structures.
*
* Create a request for the method "taxonomies.import".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link DataCatalogImport#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of project that the imported taxonomies will belong to.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportTaxonomiesRequest}
* @return the request
*/
public DataCatalogImport datacatalogImport(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportTaxonomiesRequest content) throws java.io.IOException {
DataCatalogImport result = new DataCatalogImport(parent, content);
initialize(result);
return result;
}
public class DataCatalogImport extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/taxonomies:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates new taxonomies (including their policy tags) in a given project by importing from
* inlined or cross-regional sources. For a cross-regional source, new taxonomies are created by
* copying from a source in another region. For an inlined source, taxonomies and policy tags are
* created in bulk using nested protocol buffer structures.
*
* Create a request for the method "taxonomies.import".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link DataCatalogImport#execute()} method to invoke the remote
* operation. {@link DataCatalogImport#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. Resource name of project that the imported taxonomies will belong to.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportTaxonomiesRequest}
* @since 1.13
*/
protected DataCatalogImport(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportTaxonomiesRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ImportTaxonomiesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public DataCatalogImport set$Xgafv(java.lang.String $Xgafv) {
return (DataCatalogImport) super.set$Xgafv($Xgafv);
}
@Override
public DataCatalogImport setAccessToken(java.lang.String accessToken) {
return (DataCatalogImport) super.setAccessToken(accessToken);
}
@Override
public DataCatalogImport setAlt(java.lang.String alt) {
return (DataCatalogImport) super.setAlt(alt);
}
@Override
public DataCatalogImport setCallback(java.lang.String callback) {
return (DataCatalogImport) super.setCallback(callback);
}
@Override
public DataCatalogImport setFields(java.lang.String fields) {
return (DataCatalogImport) super.setFields(fields);
}
@Override
public DataCatalogImport setKey(java.lang.String key) {
return (DataCatalogImport) super.setKey(key);
}
@Override
public DataCatalogImport setOauthToken(java.lang.String oauthToken) {
return (DataCatalogImport) super.setOauthToken(oauthToken);
}
@Override
public DataCatalogImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DataCatalogImport) super.setPrettyPrint(prettyPrint);
}
@Override
public DataCatalogImport setQuotaUser(java.lang.String quotaUser) {
return (DataCatalogImport) super.setQuotaUser(quotaUser);
}
@Override
public DataCatalogImport setUploadType(java.lang.String uploadType) {
return (DataCatalogImport) super.setUploadType(uploadType);
}
@Override
public DataCatalogImport setUploadProtocol(java.lang.String uploadProtocol) {
return (DataCatalogImport) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of project that the imported taxonomies will belong to. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of project that the imported taxonomies will belong to.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Resource name of project that the imported taxonomies will belong to. */
public DataCatalogImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public DataCatalogImport set(String parameterName, Object value) {
return (DataCatalogImport) super.set(parameterName, value);
}
}
/**
* Lists all taxonomies in a project in a particular location that you have a permission to view.
*
* Create a request for the method "taxonomies.list".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the project to list the taxonomies of.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/taxonomies";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists all taxonomies in a project in a particular location that you have a permission to view.
*
* Create a request for the method "taxonomies.list".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the project to list the taxonomies of.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ListTaxonomiesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the project to list the taxonomies of. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the project to list the taxonomies of.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Resource name of the project to list the taxonomies of. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Supported field for filter is 'service' and value is 'dataplex'. Eg: service=dataplex.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Supported field for filter is 'service' and value is 'dataplex'. Eg: service=dataplex.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Supported field for filter is 'service' and value is 'dataplex'. Eg: service=dataplex.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of items to return. Must be a value between 1 and 1000 inclusively.
* If not set, defaults to 50.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return. Must be a value between 1 and 1000 inclusively. If not set,
defaults to 50.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return. Must be a value between 1 and 1000 inclusively.
* If not set, defaults to 50.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The pagination token of the next results page. If not set, the first page is returned.
* The token is returned in the response to a previous list request.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The pagination token of the next results page. If not set, the first page is returned. The token is
returned in the response to a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The pagination token of the next results page. If not set, the first page is returned.
* The token is returned in the response to a previous list request.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a taxonomy, including its display name, description, and activated policy types.
*
* Create a request for the method "taxonomies.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. Resource name of this taxonomy in URL format. Note: Policy tag manager generates unique
* taxonomy IDs.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Updates a taxonomy, including its display name, description, and activated policy types.
*
* Create a request for the method "taxonomies.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. Resource name of this taxonomy in URL format. Note: Policy tag manager generates unique
* taxonomy IDs.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. Resource name of this taxonomy in URL format. Note: Policy tag manager
* generates unique taxonomy IDs.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. Resource name of this taxonomy in URL format. Note: Policy tag manager generates unique
taxonomy IDs.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Resource name of this taxonomy in URL format. Note: Policy tag manager
* generates unique taxonomy IDs.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.name = name;
return this;
}
/**
* Specifies fields to update. If not set, defaults to all fields you can update. For more
* information, see [FieldMask] (https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask).
*/
@com.google.api.client.util.Key
private String updateMask;
/** Specifies fields to update. If not set, defaults to all fields you can update. For more
information, see [FieldMask] (https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask).
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Specifies fields to update. If not set, defaults to all fields you can update. For more
* information, see [FieldMask] (https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask).
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Replaces (updates) a taxonomy and all its policy tags. The taxonomy and its entire hierarchy of
* policy tags must be represented literally by `SerializedTaxonomy` and the nested
* `SerializedPolicyTag` messages. This operation automatically does the following: - Deletes the
* existing policy tags that are missing from the `SerializedPolicyTag`. - Creates policy tags that
* don't have resource names. They are considered new. - Updates policy tags with valid resources
* names accordingly.
*
* Create a request for the method "taxonomies.replace".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Replace#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the taxonomy to update.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReplaceTaxonomyRequest}
* @return the request
*/
public Replace replace(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReplaceTaxonomyRequest content) throws java.io.IOException {
Replace result = new Replace(name, content);
initialize(result);
return result;
}
public class Replace extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}:replace";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Replaces (updates) a taxonomy and all its policy tags. The taxonomy and its entire hierarchy of
* policy tags must be represented literally by `SerializedTaxonomy` and the nested
* `SerializedPolicyTag` messages. This operation automatically does the following: - Deletes the
* existing policy tags that are missing from the `SerializedPolicyTag`. - Creates policy tags
* that don't have resource names. They are considered new. - Updates policy tags with valid
* resources names accordingly.
*
* Create a request for the method "taxonomies.replace".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Replace#execute()} method to invoke the remote operation.
* {@link
* Replace#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the taxonomy to update.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReplaceTaxonomyRequest}
* @since 1.13
*/
protected Replace(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ReplaceTaxonomyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1Taxonomy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public Replace set$Xgafv(java.lang.String $Xgafv) {
return (Replace) super.set$Xgafv($Xgafv);
}
@Override
public Replace setAccessToken(java.lang.String accessToken) {
return (Replace) super.setAccessToken(accessToken);
}
@Override
public Replace setAlt(java.lang.String alt) {
return (Replace) super.setAlt(alt);
}
@Override
public Replace setCallback(java.lang.String callback) {
return (Replace) super.setCallback(callback);
}
@Override
public Replace setFields(java.lang.String fields) {
return (Replace) super.setFields(fields);
}
@Override
public Replace setKey(java.lang.String key) {
return (Replace) super.setKey(key);
}
@Override
public Replace setOauthToken(java.lang.String oauthToken) {
return (Replace) super.setOauthToken(oauthToken);
}
@Override
public Replace setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Replace) super.setPrettyPrint(prettyPrint);
}
@Override
public Replace setQuotaUser(java.lang.String quotaUser) {
return (Replace) super.setQuotaUser(quotaUser);
}
@Override
public Replace setUploadType(java.lang.String uploadType) {
return (Replace) super.setUploadType(uploadType);
}
@Override
public Replace setUploadProtocol(java.lang.String uploadProtocol) {
return (Replace) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the taxonomy to update. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the taxonomy to update.
*/
public java.lang.String getName() {
return name;
}
/** Required. Resource name of the taxonomy to update. */
public Replace setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Replace set(String parameterName, Object value) {
return (Replace) super.set(parameterName, value);
}
}
/**
* Sets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "taxonomies.setIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Sets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "taxonomies.setIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns your permissions on a specified policy tag or taxonomy.
*
* Create a request for the method "taxonomies.testIamPermissions".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Returns your permissions on a specified policy tag or taxonomy.
*
* Create a request for the method "taxonomies.testIamPermissions".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the PolicyTags collection.
*
* The typical use is:
*
* {@code DataCatalog datacatalog = new DataCatalog(...);}
* {@code DataCatalog.PolicyTags.List request = datacatalog.policyTags().list(parameters ...)}
*
*
* @return the resource collection
*/
public PolicyTags policyTags() {
return new PolicyTags();
}
/**
* The "policyTags" collection of methods.
*/
public class PolicyTags {
/**
* Creates a policy tag in a taxonomy.
*
* Create a request for the method "policyTags.create".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the taxonomy that the policy tag will belong to.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/policyTags";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Creates a policy tag in a taxonomy.
*
* Create a request for the method "policyTags.create".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the taxonomy that the policy tag will belong to.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the taxonomy that the policy tag will belong to. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the taxonomy that the policy tag will belong to.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Resource name of the taxonomy that the policy tag will belong to. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a policy tag together with the following: * All of its descendant policy tags, if any *
* Policies associated with the policy tag and its descendants * References from BigQuery table
* schema of the policy tag and its descendants
*
* Create a request for the method "policyTags.delete".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the policy tag to delete. Note: All of its descendant policy tags are
* also deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
/**
* Deletes a policy tag together with the following: * All of its descendant policy tags, if any *
* Policies associated with the policy tag and its descendants * References from BigQuery table
* schema of the policy tag and its descendants
*
* Create a request for the method "policyTags.delete".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the policy tag to delete. Note: All of its descendant policy tags are
* also deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DataCatalog.this, "DELETE", REST_PATH, null, com.google.api.services.datacatalog.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the policy tag to delete. Note: All of its descendant
* policy tags are also deleted.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the policy tag to delete. Note: All of its descendant policy tags are
also deleted.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the policy tag to delete. Note: All of its descendant
* policy tags are also deleted.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a policy tag.
*
* Create a request for the method "policyTags.get".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the policy tag.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
/**
* Gets a policy tag.
*
* Create a request for the method "policyTags.get".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the policy tag.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the policy tag. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the policy tag.
*/
public java.lang.String getName() {
return name;
}
/** Required. Resource name of the policy tag. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "policyTags.getIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
/**
* Gets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "policyTags.getIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.GetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists all policy tags in a taxonomy.
*
* Create a request for the method "policyTags.list".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name of the taxonomy to list the policy tags of.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+parent}/policyTags";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
/**
* Lists all policy tags in a taxonomy.
*
* Create a request for the method "policyTags.list".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name of the taxonomy to list the policy tags of.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DataCatalog.this, "GET", REST_PATH, null, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1ListPolicyTagsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. Resource name of the taxonomy to list the policy tags of. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name of the taxonomy to list the policy tags of.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Resource name of the taxonomy to list the policy tags of. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return. Must be a value between 1 and 1000
* inclusively. If not set, defaults to 50.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return. Must be a value between 1 and 1000 inclusively. If not set,
defaults to 50.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return. Must be a value between 1 and 1000
* inclusively. If not set, defaults to 50.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The pagination token of the next results page. If not set, returns the first page.
* The token is returned in the response to a previous list request.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The pagination token of the next results page. If not set, returns the first page. The token is
returned in the response to a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The pagination token of the next results page. If not set, returns the first page.
* The token is returned in the response to a previous list request.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a policy tag, including its display name, description, and parent policy tag.
*
* Create a request for the method "policyTags.patch".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. Resource name of this policy tag in the URL format. The policy tag manager generates
* unique taxonomy IDs and policy tag IDs.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
/**
* Updates a policy tag, including its display name, description, and parent policy tag.
*
* Create a request for the method "policyTags.patch".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. Resource name of this policy tag in the URL format. The policy tag manager generates
* unique taxonomy IDs and policy tag IDs.
* @param content the {@link com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag content) {
super(DataCatalog.this, "PATCH", REST_PATH, content, com.google.api.services.datacatalog.v1.model.GoogleCloudDatacatalogV1PolicyTag.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. Resource name of this policy tag in the URL format. The policy tag
* manager generates unique taxonomy IDs and policy tag IDs.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. Resource name of this policy tag in the URL format. The policy tag manager generates
unique taxonomy IDs and policy tag IDs.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Resource name of this policy tag in the URL format. The policy tag
* manager generates unique taxonomy IDs and policy tag IDs.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
this.name = name;
return this;
}
/**
* Specifies the fields to update. You can update only display name, description, and
* parent policy tag. If not set, defaults to all updatable fields. For more
* information, see [FieldMask] (https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask).
*/
@com.google.api.client.util.Key
private String updateMask;
/** Specifies the fields to update. You can update only display name, description, and parent policy
tag. If not set, defaults to all updatable fields. For more information, see [FieldMask]
(https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask).
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Specifies the fields to update. You can update only display name, description, and
* parent policy tag. If not set, defaults to all updatable fields. For more
* information, see [FieldMask] (https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask).
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "policyTags.setIamPolicy".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
/**
* Sets the IAM policy for a policy tag or a taxonomy.
*
* Create a request for the method "policyTags.setIamPolicy".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datacatalog.v1.model.SetIamPolicyRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns your permissions on a specified policy tag or taxonomy.
*
* Create a request for the method "policyTags.testIamPermissions".
*
* This request holds the parameters needed by the datacatalog server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataCatalogRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
/**
* Returns your permissions on a specified policy tag or taxonomy.
*
* Create a request for the method "policyTags.testIamPermissions".
*
* This request holds the parameters needed by the the datacatalog server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datacatalog.v1.model.TestIamPermissionsRequest content) {
super(DataCatalog.this, "POST", REST_PATH, content, com.google.api.services.datacatalog.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/taxonomies/[^/]+/policyTags/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
}
}
}
/**
* Builder for {@link DataCatalog}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link DataCatalog}. */
@Override
public DataCatalog build() {
return new DataCatalog(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link DataCatalogRequestInitializer}.
*
* @since 1.12
*/
public Builder setDataCatalogRequestInitializer(
DataCatalogRequestInitializer datacatalogRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(datacatalogRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}