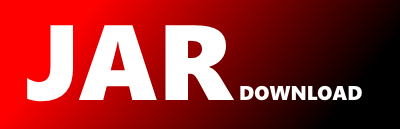
com.google.api.services.dataflow.model.ParameterMetadata Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataflow.model;
/**
* Metadata for a specific parameter.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dataflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ParameterMetadata extends com.google.api.client.json.GenericJson {
/**
* Optional. Additional metadata for describing this parameter.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map customMetadata;
/**
* Optional. The default values will pre-populate the parameter with the given value from the
* proto. If default_value is left empty, the parameter will be populated with a default of the
* relevant type, e.g. false for a boolean.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultValue;
/**
* Optional. The options shown when ENUM ParameterType is specified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List enumOptions;
/**
* Optional. Specifies a group name for this parameter to be rendered under. Group header text
* will be rendered exactly as specified in this field. Only considered when parent_name is NOT
* provided.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String groupName;
/**
* Required. The help text to display for the parameter.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String helpText;
/**
* Optional. Whether the parameter is optional. Defaults to false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isOptional;
/**
* Required. The label to display for the parameter.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String label;
/**
* Required. The name of the parameter.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Optional. The type of the parameter. Used for selecting input picker.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String paramType;
/**
* Optional. Specifies the name of the parent parameter. Used in conjunction with
* 'parent_trigger_values' to make this parameter conditional (will only be rendered
* conditionally). Should be mappable to a ParameterMetadata.name field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String parentName;
/**
* Optional. The value(s) of the 'parent_name' parameter which will trigger this parameter to be
* shown. If left empty, ANY non-empty value in parent_name will trigger this parameter to be
* shown. Only considered when this parameter is conditional (when 'parent_name' has been
* provided).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List parentTriggerValues;
/**
* Optional. Regexes that the parameter must match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List regexes;
/**
* Optional. Additional metadata for describing this parameter.
* @return value or {@code null} for none
*/
public java.util.Map getCustomMetadata() {
return customMetadata;
}
/**
* Optional. Additional metadata for describing this parameter.
* @param customMetadata customMetadata or {@code null} for none
*/
public ParameterMetadata setCustomMetadata(java.util.Map customMetadata) {
this.customMetadata = customMetadata;
return this;
}
/**
* Optional. The default values will pre-populate the parameter with the given value from the
* proto. If default_value is left empty, the parameter will be populated with a default of the
* relevant type, e.g. false for a boolean.
* @return value or {@code null} for none
*/
public java.lang.String getDefaultValue() {
return defaultValue;
}
/**
* Optional. The default values will pre-populate the parameter with the given value from the
* proto. If default_value is left empty, the parameter will be populated with a default of the
* relevant type, e.g. false for a boolean.
* @param defaultValue defaultValue or {@code null} for none
*/
public ParameterMetadata setDefaultValue(java.lang.String defaultValue) {
this.defaultValue = defaultValue;
return this;
}
/**
* Optional. The options shown when ENUM ParameterType is specified.
* @return value or {@code null} for none
*/
public java.util.List getEnumOptions() {
return enumOptions;
}
/**
* Optional. The options shown when ENUM ParameterType is specified.
* @param enumOptions enumOptions or {@code null} for none
*/
public ParameterMetadata setEnumOptions(java.util.List enumOptions) {
this.enumOptions = enumOptions;
return this;
}
/**
* Optional. Specifies a group name for this parameter to be rendered under. Group header text
* will be rendered exactly as specified in this field. Only considered when parent_name is NOT
* provided.
* @return value or {@code null} for none
*/
public java.lang.String getGroupName() {
return groupName;
}
/**
* Optional. Specifies a group name for this parameter to be rendered under. Group header text
* will be rendered exactly as specified in this field. Only considered when parent_name is NOT
* provided.
* @param groupName groupName or {@code null} for none
*/
public ParameterMetadata setGroupName(java.lang.String groupName) {
this.groupName = groupName;
return this;
}
/**
* Required. The help text to display for the parameter.
* @return value or {@code null} for none
*/
public java.lang.String getHelpText() {
return helpText;
}
/**
* Required. The help text to display for the parameter.
* @param helpText helpText or {@code null} for none
*/
public ParameterMetadata setHelpText(java.lang.String helpText) {
this.helpText = helpText;
return this;
}
/**
* Optional. Whether the parameter is optional. Defaults to false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsOptional() {
return isOptional;
}
/**
* Optional. Whether the parameter is optional. Defaults to false.
* @param isOptional isOptional or {@code null} for none
*/
public ParameterMetadata setIsOptional(java.lang.Boolean isOptional) {
this.isOptional = isOptional;
return this;
}
/**
* Required. The label to display for the parameter.
* @return value or {@code null} for none
*/
public java.lang.String getLabel() {
return label;
}
/**
* Required. The label to display for the parameter.
* @param label label or {@code null} for none
*/
public ParameterMetadata setLabel(java.lang.String label) {
this.label = label;
return this;
}
/**
* Required. The name of the parameter.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the parameter.
* @param name name or {@code null} for none
*/
public ParameterMetadata setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Optional. The type of the parameter. Used for selecting input picker.
* @return value or {@code null} for none
*/
public java.lang.String getParamType() {
return paramType;
}
/**
* Optional. The type of the parameter. Used for selecting input picker.
* @param paramType paramType or {@code null} for none
*/
public ParameterMetadata setParamType(java.lang.String paramType) {
this.paramType = paramType;
return this;
}
/**
* Optional. Specifies the name of the parent parameter. Used in conjunction with
* 'parent_trigger_values' to make this parameter conditional (will only be rendered
* conditionally). Should be mappable to a ParameterMetadata.name field.
* @return value or {@code null} for none
*/
public java.lang.String getParentName() {
return parentName;
}
/**
* Optional. Specifies the name of the parent parameter. Used in conjunction with
* 'parent_trigger_values' to make this parameter conditional (will only be rendered
* conditionally). Should be mappable to a ParameterMetadata.name field.
* @param parentName parentName or {@code null} for none
*/
public ParameterMetadata setParentName(java.lang.String parentName) {
this.parentName = parentName;
return this;
}
/**
* Optional. The value(s) of the 'parent_name' parameter which will trigger this parameter to be
* shown. If left empty, ANY non-empty value in parent_name will trigger this parameter to be
* shown. Only considered when this parameter is conditional (when 'parent_name' has been
* provided).
* @return value or {@code null} for none
*/
public java.util.List getParentTriggerValues() {
return parentTriggerValues;
}
/**
* Optional. The value(s) of the 'parent_name' parameter which will trigger this parameter to be
* shown. If left empty, ANY non-empty value in parent_name will trigger this parameter to be
* shown. Only considered when this parameter is conditional (when 'parent_name' has been
* provided).
* @param parentTriggerValues parentTriggerValues or {@code null} for none
*/
public ParameterMetadata setParentTriggerValues(java.util.List parentTriggerValues) {
this.parentTriggerValues = parentTriggerValues;
return this;
}
/**
* Optional. Regexes that the parameter must match.
* @return value or {@code null} for none
*/
public java.util.List getRegexes() {
return regexes;
}
/**
* Optional. Regexes that the parameter must match.
* @param regexes regexes or {@code null} for none
*/
public ParameterMetadata setRegexes(java.util.List regexes) {
this.regexes = regexes;
return this;
}
@Override
public ParameterMetadata set(String fieldName, Object value) {
return (ParameterMetadata) super.set(fieldName, value);
}
@Override
public ParameterMetadata clone() {
return (ParameterMetadata) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy