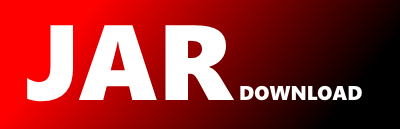
com.google.api.services.dataflow.model.Snapshot Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataflow.model;
/**
* Represents a snapshot of a job.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dataflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Snapshot extends com.google.api.client.json.GenericJson {
/**
* The time this snapshot was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String creationTime;
/**
* User specified description of the snapshot. Maybe empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The disk byte size of the snapshot. Only available for snapshots in READY state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long diskSizeBytes;
/**
* The unique ID of this snapshot.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The project this snapshot belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/**
* Pub/Sub snapshot metadata.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List pubsubMetadata;
static {
// hack to force ProGuard to consider PubsubSnapshotMetadata used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(PubsubSnapshotMetadata.class);
}
/**
* Cloud region where this snapshot lives in, e.g., "us-central1".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String region;
/**
* The job this snapshot was created from.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sourceJobId;
/**
* State of the snapshot.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* The time after which this snapshot will be automatically deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String ttl;
/**
* The time this snapshot was created.
* @return value or {@code null} for none
*/
public String getCreationTime() {
return creationTime;
}
/**
* The time this snapshot was created.
* @param creationTime creationTime or {@code null} for none
*/
public Snapshot setCreationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* User specified description of the snapshot. Maybe empty.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* User specified description of the snapshot. Maybe empty.
* @param description description or {@code null} for none
*/
public Snapshot setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The disk byte size of the snapshot. Only available for snapshots in READY state.
* @return value or {@code null} for none
*/
public java.lang.Long getDiskSizeBytes() {
return diskSizeBytes;
}
/**
* The disk byte size of the snapshot. Only available for snapshots in READY state.
* @param diskSizeBytes diskSizeBytes or {@code null} for none
*/
public Snapshot setDiskSizeBytes(java.lang.Long diskSizeBytes) {
this.diskSizeBytes = diskSizeBytes;
return this;
}
/**
* The unique ID of this snapshot.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The unique ID of this snapshot.
* @param id id or {@code null} for none
*/
public Snapshot setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The project this snapshot belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* The project this snapshot belongs to.
* @param projectId projectId or {@code null} for none
*/
public Snapshot setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Pub/Sub snapshot metadata.
* @return value or {@code null} for none
*/
public java.util.List getPubsubMetadata() {
return pubsubMetadata;
}
/**
* Pub/Sub snapshot metadata.
* @param pubsubMetadata pubsubMetadata or {@code null} for none
*/
public Snapshot setPubsubMetadata(java.util.List pubsubMetadata) {
this.pubsubMetadata = pubsubMetadata;
return this;
}
/**
* Cloud region where this snapshot lives in, e.g., "us-central1".
* @return value or {@code null} for none
*/
public java.lang.String getRegion() {
return region;
}
/**
* Cloud region where this snapshot lives in, e.g., "us-central1".
* @param region region or {@code null} for none
*/
public Snapshot setRegion(java.lang.String region) {
this.region = region;
return this;
}
/**
* The job this snapshot was created from.
* @return value or {@code null} for none
*/
public java.lang.String getSourceJobId() {
return sourceJobId;
}
/**
* The job this snapshot was created from.
* @param sourceJobId sourceJobId or {@code null} for none
*/
public Snapshot setSourceJobId(java.lang.String sourceJobId) {
this.sourceJobId = sourceJobId;
return this;
}
/**
* State of the snapshot.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* State of the snapshot.
* @param state state or {@code null} for none
*/
public Snapshot setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* The time after which this snapshot will be automatically deleted.
* @return value or {@code null} for none
*/
public String getTtl() {
return ttl;
}
/**
* The time after which this snapshot will be automatically deleted.
* @param ttl ttl or {@code null} for none
*/
public Snapshot setTtl(String ttl) {
this.ttl = ttl;
return this;
}
@Override
public Snapshot set(String fieldName, Object value) {
return (Snapshot) super.set(fieldName, value);
}
@Override
public Snapshot clone() {
return (Snapshot) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy