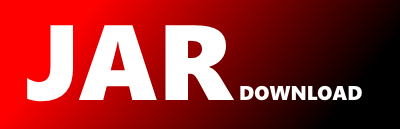
com.google.api.services.dataflow.model.Source Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataflow.model;
/**
* A source that records can be read and decoded from.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dataflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Source extends com.google.api.client.json.GenericJson {
/**
* While splitting, sources may specify the produced bundles as differences against another
* source, in order to save backend-side memory and allow bigger jobs. For details, see
* SourceSplitRequest. To support this use case, the full set of parameters of the source is
* logically obtained by taking the latest explicitly specified value of each parameter in the
* order: base_specs (later items win), spec (overrides anything in base_specs).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List> baseSpecs;
/**
* The codec to use to decode data read from the source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map codec;
/**
* Setting this value to true hints to the framework that the source doesn't need splitting, and
* using SourceSplitRequest on it would yield SOURCE_SPLIT_OUTCOME_USE_CURRENT. E.g. a file
* splitter may set this to true when splitting a single file into a set of byte ranges of
* appropriate size, and set this to false when splitting a filepattern into individual files.
* However, for efficiency, a file splitter may decide to produce file subranges directly from the
* filepattern to avoid a splitting round-trip. See SourceSplitRequest for an overview of the
* splitting process. This field is meaningful only in the Source objects populated by the user
* (e.g. when filling in a DerivedSource). Source objects supplied by the framework to the user
* don't have this field populated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean doesNotNeedSplitting;
/**
* Optionally, metadata for this source can be supplied right away, avoiding a
* SourceGetMetadataOperation roundtrip (see SourceOperationRequest). This field is meaningful
* only in the Source objects populated by the user (e.g. when filling in a DerivedSource). Source
* objects supplied by the framework to the user don't have this field populated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SourceMetadata metadata;
/**
* The source to read from, plus its parameters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map spec;
/**
* While splitting, sources may specify the produced bundles as differences against another
* source, in order to save backend-side memory and allow bigger jobs. For details, see
* SourceSplitRequest. To support this use case, the full set of parameters of the source is
* logically obtained by taking the latest explicitly specified value of each parameter in the
* order: base_specs (later items win), spec (overrides anything in base_specs).
* @return value or {@code null} for none
*/
public java.util.List> getBaseSpecs() {
return baseSpecs;
}
/**
* While splitting, sources may specify the produced bundles as differences against another
* source, in order to save backend-side memory and allow bigger jobs. For details, see
* SourceSplitRequest. To support this use case, the full set of parameters of the source is
* logically obtained by taking the latest explicitly specified value of each parameter in the
* order: base_specs (later items win), spec (overrides anything in base_specs).
* @param baseSpecs baseSpecs or {@code null} for none
*/
public Source setBaseSpecs(java.util.List> baseSpecs) {
this.baseSpecs = baseSpecs;
return this;
}
/**
* The codec to use to decode data read from the source.
* @return value or {@code null} for none
*/
public java.util.Map getCodec() {
return codec;
}
/**
* The codec to use to decode data read from the source.
* @param codec codec or {@code null} for none
*/
public Source setCodec(java.util.Map codec) {
this.codec = codec;
return this;
}
/**
* Setting this value to true hints to the framework that the source doesn't need splitting, and
* using SourceSplitRequest on it would yield SOURCE_SPLIT_OUTCOME_USE_CURRENT. E.g. a file
* splitter may set this to true when splitting a single file into a set of byte ranges of
* appropriate size, and set this to false when splitting a filepattern into individual files.
* However, for efficiency, a file splitter may decide to produce file subranges directly from the
* filepattern to avoid a splitting round-trip. See SourceSplitRequest for an overview of the
* splitting process. This field is meaningful only in the Source objects populated by the user
* (e.g. when filling in a DerivedSource). Source objects supplied by the framework to the user
* don't have this field populated.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDoesNotNeedSplitting() {
return doesNotNeedSplitting;
}
/**
* Setting this value to true hints to the framework that the source doesn't need splitting, and
* using SourceSplitRequest on it would yield SOURCE_SPLIT_OUTCOME_USE_CURRENT. E.g. a file
* splitter may set this to true when splitting a single file into a set of byte ranges of
* appropriate size, and set this to false when splitting a filepattern into individual files.
* However, for efficiency, a file splitter may decide to produce file subranges directly from the
* filepattern to avoid a splitting round-trip. See SourceSplitRequest for an overview of the
* splitting process. This field is meaningful only in the Source objects populated by the user
* (e.g. when filling in a DerivedSource). Source objects supplied by the framework to the user
* don't have this field populated.
* @param doesNotNeedSplitting doesNotNeedSplitting or {@code null} for none
*/
public Source setDoesNotNeedSplitting(java.lang.Boolean doesNotNeedSplitting) {
this.doesNotNeedSplitting = doesNotNeedSplitting;
return this;
}
/**
* Optionally, metadata for this source can be supplied right away, avoiding a
* SourceGetMetadataOperation roundtrip (see SourceOperationRequest). This field is meaningful
* only in the Source objects populated by the user (e.g. when filling in a DerivedSource). Source
* objects supplied by the framework to the user don't have this field populated.
* @return value or {@code null} for none
*/
public SourceMetadata getMetadata() {
return metadata;
}
/**
* Optionally, metadata for this source can be supplied right away, avoiding a
* SourceGetMetadataOperation roundtrip (see SourceOperationRequest). This field is meaningful
* only in the Source objects populated by the user (e.g. when filling in a DerivedSource). Source
* objects supplied by the framework to the user don't have this field populated.
* @param metadata metadata or {@code null} for none
*/
public Source setMetadata(SourceMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* The source to read from, plus its parameters.
* @return value or {@code null} for none
*/
public java.util.Map getSpec() {
return spec;
}
/**
* The source to read from, plus its parameters.
* @param spec spec or {@code null} for none
*/
public Source setSpec(java.util.Map spec) {
this.spec = spec;
return this;
}
@Override
public Source set(String fieldName, Object value) {
return (Source) super.set(fieldName, value);
}
@Override
public Source clone() {
return (Source) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy