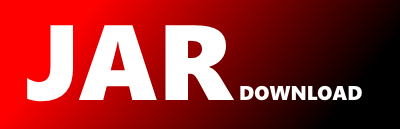
com.google.api.services.dataflow.model.WorkerMessage Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataflow.model;
/**
* WorkerMessage provides information to the backend about a worker.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dataflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class WorkerMessage extends com.google.api.client.json.GenericJson {
/**
* Optional. Contains metrics related to go/dataflow-data-sampling-telemetry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DataSamplingReport dataSamplingReport;
/**
* Labels are used to group WorkerMessages. For example, a worker_message about a particular
* container might have the labels: { "JOB_ID": "2015-04-22", "WORKER_ID": "wordcount-vm-2015…"
* "CONTAINER_TYPE": "worker", "CONTAINER_ID": "ac1234def"} Label tags typically correspond to
* Label enum values. However, for ease of development other strings can be used as tags.
* LABEL_UNSPECIFIED should not be used here.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* The timestamp of the worker_message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String time;
/**
* The health of a worker.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WorkerHealthReport workerHealthReport;
/**
* Record of worker lifecycle events.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WorkerLifecycleEvent workerLifecycleEvent;
/**
* A worker message code.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WorkerMessageCode workerMessageCode;
/**
* Resource metrics reported by workers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ResourceUtilizationReport workerMetrics;
/**
* Shutdown notice by workers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WorkerShutdownNotice workerShutdownNotice;
/**
* Thread scaling information reported by workers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WorkerThreadScalingReport workerThreadScalingReport;
/**
* Optional. Contains metrics related to go/dataflow-data-sampling-telemetry.
* @return value or {@code null} for none
*/
public DataSamplingReport getDataSamplingReport() {
return dataSamplingReport;
}
/**
* Optional. Contains metrics related to go/dataflow-data-sampling-telemetry.
* @param dataSamplingReport dataSamplingReport or {@code null} for none
*/
public WorkerMessage setDataSamplingReport(DataSamplingReport dataSamplingReport) {
this.dataSamplingReport = dataSamplingReport;
return this;
}
/**
* Labels are used to group WorkerMessages. For example, a worker_message about a particular
* container might have the labels: { "JOB_ID": "2015-04-22", "WORKER_ID": "wordcount-vm-2015…"
* "CONTAINER_TYPE": "worker", "CONTAINER_ID": "ac1234def"} Label tags typically correspond to
* Label enum values. However, for ease of development other strings can be used as tags.
* LABEL_UNSPECIFIED should not be used here.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Labels are used to group WorkerMessages. For example, a worker_message about a particular
* container might have the labels: { "JOB_ID": "2015-04-22", "WORKER_ID": "wordcount-vm-2015…"
* "CONTAINER_TYPE": "worker", "CONTAINER_ID": "ac1234def"} Label tags typically correspond to
* Label enum values. However, for ease of development other strings can be used as tags.
* LABEL_UNSPECIFIED should not be used here.
* @param labels labels or {@code null} for none
*/
public WorkerMessage setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* The timestamp of the worker_message.
* @return value or {@code null} for none
*/
public String getTime() {
return time;
}
/**
* The timestamp of the worker_message.
* @param time time or {@code null} for none
*/
public WorkerMessage setTime(String time) {
this.time = time;
return this;
}
/**
* The health of a worker.
* @return value or {@code null} for none
*/
public WorkerHealthReport getWorkerHealthReport() {
return workerHealthReport;
}
/**
* The health of a worker.
* @param workerHealthReport workerHealthReport or {@code null} for none
*/
public WorkerMessage setWorkerHealthReport(WorkerHealthReport workerHealthReport) {
this.workerHealthReport = workerHealthReport;
return this;
}
/**
* Record of worker lifecycle events.
* @return value or {@code null} for none
*/
public WorkerLifecycleEvent getWorkerLifecycleEvent() {
return workerLifecycleEvent;
}
/**
* Record of worker lifecycle events.
* @param workerLifecycleEvent workerLifecycleEvent or {@code null} for none
*/
public WorkerMessage setWorkerLifecycleEvent(WorkerLifecycleEvent workerLifecycleEvent) {
this.workerLifecycleEvent = workerLifecycleEvent;
return this;
}
/**
* A worker message code.
* @return value or {@code null} for none
*/
public WorkerMessageCode getWorkerMessageCode() {
return workerMessageCode;
}
/**
* A worker message code.
* @param workerMessageCode workerMessageCode or {@code null} for none
*/
public WorkerMessage setWorkerMessageCode(WorkerMessageCode workerMessageCode) {
this.workerMessageCode = workerMessageCode;
return this;
}
/**
* Resource metrics reported by workers.
* @return value or {@code null} for none
*/
public ResourceUtilizationReport getWorkerMetrics() {
return workerMetrics;
}
/**
* Resource metrics reported by workers.
* @param workerMetrics workerMetrics or {@code null} for none
*/
public WorkerMessage setWorkerMetrics(ResourceUtilizationReport workerMetrics) {
this.workerMetrics = workerMetrics;
return this;
}
/**
* Shutdown notice by workers.
* @return value or {@code null} for none
*/
public WorkerShutdownNotice getWorkerShutdownNotice() {
return workerShutdownNotice;
}
/**
* Shutdown notice by workers.
* @param workerShutdownNotice workerShutdownNotice or {@code null} for none
*/
public WorkerMessage setWorkerShutdownNotice(WorkerShutdownNotice workerShutdownNotice) {
this.workerShutdownNotice = workerShutdownNotice;
return this;
}
/**
* Thread scaling information reported by workers.
* @return value or {@code null} for none
*/
public WorkerThreadScalingReport getWorkerThreadScalingReport() {
return workerThreadScalingReport;
}
/**
* Thread scaling information reported by workers.
* @param workerThreadScalingReport workerThreadScalingReport or {@code null} for none
*/
public WorkerMessage setWorkerThreadScalingReport(WorkerThreadScalingReport workerThreadScalingReport) {
this.workerThreadScalingReport = workerThreadScalingReport;
return this;
}
@Override
public WorkerMessage set(String fieldName, Object value) {
return (WorkerMessage) super.set(fieldName, value);
}
@Override
public WorkerMessage clone() {
return (WorkerMessage) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy