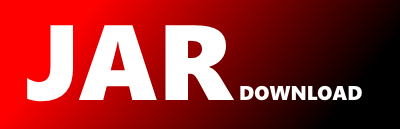
target.apidocs.com.google.api.services.dataflow.model.DisplayData.html Maven / Gradle / Ivy
DisplayData (Dataflow API v1b3-rev20231112-2.0.0)
com.google.api.services.dataflow.model
Class DisplayData
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.dataflow.model.DisplayData
-
public final class DisplayData
extends com.google.api.client.json.GenericJson
Data provided with a pipeline or transform to provide descriptive info.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Dataflow API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
DisplayData()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
DisplayData
clone()
Boolean
getBoolValue()
Contains value if the data is of a boolean type.
String
getDurationValue()
Contains value if the data is of duration type.
Float
getFloatValue()
Contains value if the data is of float type.
Long
getInt64Value()
Contains value if the data is of int64 type.
String
getJavaClassValue()
Contains value if the data is of java class type.
String
getKey()
The key identifying the display data.
String
getLabel()
An optional label to display in a dax UI for the element.
String
getNamespace()
The namespace for the key.
String
getShortStrValue()
A possible additional shorter value to display.
String
getStrValue()
Contains value if the data is of string type.
String
getTimestampValue()
Contains value if the data is of timestamp type.
String
getUrl()
An optional full URL.
DisplayData
set(String fieldName,
Object value)
DisplayData
setBoolValue(Boolean boolValue)
Contains value if the data is of a boolean type.
DisplayData
setDurationValue(String durationValue)
Contains value if the data is of duration type.
DisplayData
setFloatValue(Float floatValue)
Contains value if the data is of float type.
DisplayData
setInt64Value(Long int64Value)
Contains value if the data is of int64 type.
DisplayData
setJavaClassValue(String javaClassValue)
Contains value if the data is of java class type.
DisplayData
setKey(String key)
The key identifying the display data.
DisplayData
setLabel(String label)
An optional label to display in a dax UI for the element.
DisplayData
setNamespace(String namespace)
The namespace for the key.
DisplayData
setShortStrValue(String shortStrValue)
A possible additional shorter value to display.
DisplayData
setStrValue(String strValue)
Contains value if the data is of string type.
DisplayData
setTimestampValue(String timestampValue)
Contains value if the data is of timestamp type.
DisplayData
setUrl(String url)
An optional full URL.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getBoolValue
public Boolean getBoolValue()
Contains value if the data is of a boolean type.
- Returns:
- value or
null
for none
-
setBoolValue
public DisplayData setBoolValue(Boolean boolValue)
Contains value if the data is of a boolean type.
- Parameters:
boolValue
- boolValue or null
for none
-
getDurationValue
public String getDurationValue()
Contains value if the data is of duration type.
- Returns:
- value or
null
for none
-
setDurationValue
public DisplayData setDurationValue(String durationValue)
Contains value if the data is of duration type.
- Parameters:
durationValue
- durationValue or null
for none
-
getFloatValue
public Float getFloatValue()
Contains value if the data is of float type.
- Returns:
- value or
null
for none
-
setFloatValue
public DisplayData setFloatValue(Float floatValue)
Contains value if the data is of float type.
- Parameters:
floatValue
- floatValue or null
for none
-
getInt64Value
public Long getInt64Value()
Contains value if the data is of int64 type.
- Returns:
- value or
null
for none
-
setInt64Value
public DisplayData setInt64Value(Long int64Value)
Contains value if the data is of int64 type.
- Parameters:
int64Value
- int64Value or null
for none
-
getJavaClassValue
public String getJavaClassValue()
Contains value if the data is of java class type.
- Returns:
- value or
null
for none
-
setJavaClassValue
public DisplayData setJavaClassValue(String javaClassValue)
Contains value if the data is of java class type.
- Parameters:
javaClassValue
- javaClassValue or null
for none
-
getKey
public String getKey()
The key identifying the display data. This is intended to be used as a label for the display
data when viewed in a dax monitoring system.
- Returns:
- value or
null
for none
-
setKey
public DisplayData setKey(String key)
The key identifying the display data. This is intended to be used as a label for the display
data when viewed in a dax monitoring system.
- Parameters:
key
- key or null
for none
-
getLabel
public String getLabel()
An optional label to display in a dax UI for the element.
- Returns:
- value or
null
for none
-
setLabel
public DisplayData setLabel(String label)
An optional label to display in a dax UI for the element.
- Parameters:
label
- label or null
for none
-
getNamespace
public String getNamespace()
The namespace for the key. This is usually a class name or programming language namespace (i.e.
python module) which defines the display data. This allows a dax monitoring system to specially
handle the data and perform custom rendering.
- Returns:
- value or
null
for none
-
setNamespace
public DisplayData setNamespace(String namespace)
The namespace for the key. This is usually a class name or programming language namespace (i.e.
python module) which defines the display data. This allows a dax monitoring system to specially
handle the data and perform custom rendering.
- Parameters:
namespace
- namespace or null
for none
-
getShortStrValue
public String getShortStrValue()
A possible additional shorter value to display. For example a java_class_name_value of
com.mypackage.MyDoFn will be stored with MyDoFn as the short_str_value and com.mypackage.MyDoFn
as the java_class_name value. short_str_value can be displayed and java_class_name_value will
be displayed as a tooltip.
- Returns:
- value or
null
for none
-
setShortStrValue
public DisplayData setShortStrValue(String shortStrValue)
A possible additional shorter value to display. For example a java_class_name_value of
com.mypackage.MyDoFn will be stored with MyDoFn as the short_str_value and com.mypackage.MyDoFn
as the java_class_name value. short_str_value can be displayed and java_class_name_value will
be displayed as a tooltip.
- Parameters:
shortStrValue
- shortStrValue or null
for none
-
getStrValue
public String getStrValue()
Contains value if the data is of string type.
- Returns:
- value or
null
for none
-
setStrValue
public DisplayData setStrValue(String strValue)
Contains value if the data is of string type.
- Parameters:
strValue
- strValue or null
for none
-
getTimestampValue
public String getTimestampValue()
Contains value if the data is of timestamp type.
- Returns:
- value or
null
for none
-
setTimestampValue
public DisplayData setTimestampValue(String timestampValue)
Contains value if the data is of timestamp type.
- Parameters:
timestampValue
- timestampValue or null
for none
-
getUrl
public String getUrl()
An optional full URL.
- Returns:
- value or
null
for none
-
setUrl
public DisplayData setUrl(String url)
An optional full URL.
- Parameters:
url
- url or null
for none
-
set
public DisplayData set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public DisplayData clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2023 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy