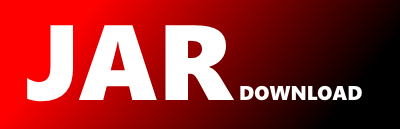
com.google.api.services.datamigration.v1.model.ColumnEntity Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datamigration.v1.model;
/**
* Column is not used as an independent entity, it is retrieved as part of a Table entity.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Database Migration API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ColumnEntity extends com.google.api.client.json.GenericJson {
/**
* Is the column of array type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean array;
/**
* If the column is array, of which length.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer arrayLength;
/**
* Is the column auto-generated/identity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoGenerated;
/**
* Charset override - instead of table level charset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String charset;
/**
* Collation override - instead of table level collation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String collation;
/**
* Comment associated with the column.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String comment;
/**
* Custom engine specific features.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map customFeatures;
/**
* Column data type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dataType;
/**
* Default value of the column.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultValue;
/**
* Column fractional second precision - used for timestamp based datatypes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer fractionalSecondsPrecision;
/**
* Column length - e.g. varchar (50).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long length;
/**
* Column name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Is the column nullable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean nullable;
/**
* Column order in the table.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer ordinalPosition;
/**
* Column precision - when relevant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer precision;
/**
* Column scale - when relevant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer scale;
/**
* Specifies the list of values allowed in the column. Only used for set data type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List setValues;
/**
* Is the column a UDT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean udt;
/**
* Is the column of array type.
* @return value or {@code null} for none
*/
public java.lang.Boolean getArray() {
return array;
}
/**
* Is the column of array type.
* @param array array or {@code null} for none
*/
public ColumnEntity setArray(java.lang.Boolean array) {
this.array = array;
return this;
}
/**
* If the column is array, of which length.
* @return value or {@code null} for none
*/
public java.lang.Integer getArrayLength() {
return arrayLength;
}
/**
* If the column is array, of which length.
* @param arrayLength arrayLength or {@code null} for none
*/
public ColumnEntity setArrayLength(java.lang.Integer arrayLength) {
this.arrayLength = arrayLength;
return this;
}
/**
* Is the column auto-generated/identity.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAutoGenerated() {
return autoGenerated;
}
/**
* Is the column auto-generated/identity.
* @param autoGenerated autoGenerated or {@code null} for none
*/
public ColumnEntity setAutoGenerated(java.lang.Boolean autoGenerated) {
this.autoGenerated = autoGenerated;
return this;
}
/**
* Charset override - instead of table level charset.
* @return value or {@code null} for none
*/
public java.lang.String getCharset() {
return charset;
}
/**
* Charset override - instead of table level charset.
* @param charset charset or {@code null} for none
*/
public ColumnEntity setCharset(java.lang.String charset) {
this.charset = charset;
return this;
}
/**
* Collation override - instead of table level collation.
* @return value or {@code null} for none
*/
public java.lang.String getCollation() {
return collation;
}
/**
* Collation override - instead of table level collation.
* @param collation collation or {@code null} for none
*/
public ColumnEntity setCollation(java.lang.String collation) {
this.collation = collation;
return this;
}
/**
* Comment associated with the column.
* @return value or {@code null} for none
*/
public java.lang.String getComment() {
return comment;
}
/**
* Comment associated with the column.
* @param comment comment or {@code null} for none
*/
public ColumnEntity setComment(java.lang.String comment) {
this.comment = comment;
return this;
}
/**
* Custom engine specific features.
* @return value or {@code null} for none
*/
public java.util.Map getCustomFeatures() {
return customFeatures;
}
/**
* Custom engine specific features.
* @param customFeatures customFeatures or {@code null} for none
*/
public ColumnEntity setCustomFeatures(java.util.Map customFeatures) {
this.customFeatures = customFeatures;
return this;
}
/**
* Column data type.
* @return value or {@code null} for none
*/
public java.lang.String getDataType() {
return dataType;
}
/**
* Column data type.
* @param dataType dataType or {@code null} for none
*/
public ColumnEntity setDataType(java.lang.String dataType) {
this.dataType = dataType;
return this;
}
/**
* Default value of the column.
* @return value or {@code null} for none
*/
public java.lang.String getDefaultValue() {
return defaultValue;
}
/**
* Default value of the column.
* @param defaultValue defaultValue or {@code null} for none
*/
public ColumnEntity setDefaultValue(java.lang.String defaultValue) {
this.defaultValue = defaultValue;
return this;
}
/**
* Column fractional second precision - used for timestamp based datatypes.
* @return value or {@code null} for none
*/
public java.lang.Integer getFractionalSecondsPrecision() {
return fractionalSecondsPrecision;
}
/**
* Column fractional second precision - used for timestamp based datatypes.
* @param fractionalSecondsPrecision fractionalSecondsPrecision or {@code null} for none
*/
public ColumnEntity setFractionalSecondsPrecision(java.lang.Integer fractionalSecondsPrecision) {
this.fractionalSecondsPrecision = fractionalSecondsPrecision;
return this;
}
/**
* Column length - e.g. varchar (50).
* @return value or {@code null} for none
*/
public java.lang.Long getLength() {
return length;
}
/**
* Column length - e.g. varchar (50).
* @param length length or {@code null} for none
*/
public ColumnEntity setLength(java.lang.Long length) {
this.length = length;
return this;
}
/**
* Column name.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Column name.
* @param name name or {@code null} for none
*/
public ColumnEntity setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Is the column nullable.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNullable() {
return nullable;
}
/**
* Is the column nullable.
* @param nullable nullable or {@code null} for none
*/
public ColumnEntity setNullable(java.lang.Boolean nullable) {
this.nullable = nullable;
return this;
}
/**
* Column order in the table.
* @return value or {@code null} for none
*/
public java.lang.Integer getOrdinalPosition() {
return ordinalPosition;
}
/**
* Column order in the table.
* @param ordinalPosition ordinalPosition or {@code null} for none
*/
public ColumnEntity setOrdinalPosition(java.lang.Integer ordinalPosition) {
this.ordinalPosition = ordinalPosition;
return this;
}
/**
* Column precision - when relevant.
* @return value or {@code null} for none
*/
public java.lang.Integer getPrecision() {
return precision;
}
/**
* Column precision - when relevant.
* @param precision precision or {@code null} for none
*/
public ColumnEntity setPrecision(java.lang.Integer precision) {
this.precision = precision;
return this;
}
/**
* Column scale - when relevant.
* @return value or {@code null} for none
*/
public java.lang.Integer getScale() {
return scale;
}
/**
* Column scale - when relevant.
* @param scale scale or {@code null} for none
*/
public ColumnEntity setScale(java.lang.Integer scale) {
this.scale = scale;
return this;
}
/**
* Specifies the list of values allowed in the column. Only used for set data type.
* @return value or {@code null} for none
*/
public java.util.List getSetValues() {
return setValues;
}
/**
* Specifies the list of values allowed in the column. Only used for set data type.
* @param setValues setValues or {@code null} for none
*/
public ColumnEntity setSetValues(java.util.List setValues) {
this.setValues = setValues;
return this;
}
/**
* Is the column a UDT.
* @return value or {@code null} for none
*/
public java.lang.Boolean getUdt() {
return udt;
}
/**
* Is the column a UDT.
* @param udt udt or {@code null} for none
*/
public ColumnEntity setUdt(java.lang.Boolean udt) {
this.udt = udt;
return this;
}
@Override
public ColumnEntity set(String fieldName, Object value) {
return (ColumnEntity) super.set(fieldName, value);
}
@Override
public ColumnEntity clone() {
return (ColumnEntity) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy