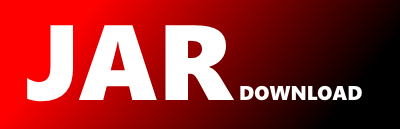
com.google.api.services.datamigration.v1.model.SequenceEntity Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datamigration.v1.model;
/**
* Sequence's parent is a schema.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Database Migration API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SequenceEntity extends com.google.api.client.json.GenericJson {
/**
* Indicates number of entries to cache / precreate.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long cache;
/**
* Custom engine specific features.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map customFeatures;
/**
* Indicates whether the sequence value should cycle through.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean cycle;
/**
* Increment value for the sequence.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long increment;
/**
* Maximum number for the sequence represented as bytes to accommodate large. numbers
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String maxValue;
/**
* Minimum number for the sequence represented as bytes to accommodate large. numbers
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String minValue;
/**
* Start number for the sequence represented as bytes to accommodate large. numbers
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String startValue;
/**
* Indicates number of entries to cache / precreate.
* @return value or {@code null} for none
*/
public java.lang.Long getCache() {
return cache;
}
/**
* Indicates number of entries to cache / precreate.
* @param cache cache or {@code null} for none
*/
public SequenceEntity setCache(java.lang.Long cache) {
this.cache = cache;
return this;
}
/**
* Custom engine specific features.
* @return value or {@code null} for none
*/
public java.util.Map getCustomFeatures() {
return customFeatures;
}
/**
* Custom engine specific features.
* @param customFeatures customFeatures or {@code null} for none
*/
public SequenceEntity setCustomFeatures(java.util.Map customFeatures) {
this.customFeatures = customFeatures;
return this;
}
/**
* Indicates whether the sequence value should cycle through.
* @return value or {@code null} for none
*/
public java.lang.Boolean getCycle() {
return cycle;
}
/**
* Indicates whether the sequence value should cycle through.
* @param cycle cycle or {@code null} for none
*/
public SequenceEntity setCycle(java.lang.Boolean cycle) {
this.cycle = cycle;
return this;
}
/**
* Increment value for the sequence.
* @return value or {@code null} for none
*/
public java.lang.Long getIncrement() {
return increment;
}
/**
* Increment value for the sequence.
* @param increment increment or {@code null} for none
*/
public SequenceEntity setIncrement(java.lang.Long increment) {
this.increment = increment;
return this;
}
/**
* Maximum number for the sequence represented as bytes to accommodate large. numbers
* @see #decodeMaxValue()
* @return value or {@code null} for none
*/
public java.lang.String getMaxValue() {
return maxValue;
}
/**
* Maximum number for the sequence represented as bytes to accommodate large. numbers
* @see #getMaxValue()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeMaxValue() {
return com.google.api.client.util.Base64.decodeBase64(maxValue);
}
/**
* Maximum number for the sequence represented as bytes to accommodate large. numbers
* @see #encodeMaxValue()
* @param maxValue maxValue or {@code null} for none
*/
public SequenceEntity setMaxValue(java.lang.String maxValue) {
this.maxValue = maxValue;
return this;
}
/**
* Maximum number for the sequence represented as bytes to accommodate large. numbers
* @see #setMaxValue()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public SequenceEntity encodeMaxValue(byte[] maxValue) {
this.maxValue = com.google.api.client.util.Base64.encodeBase64URLSafeString(maxValue);
return this;
}
/**
* Minimum number for the sequence represented as bytes to accommodate large. numbers
* @see #decodeMinValue()
* @return value or {@code null} for none
*/
public java.lang.String getMinValue() {
return minValue;
}
/**
* Minimum number for the sequence represented as bytes to accommodate large. numbers
* @see #getMinValue()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeMinValue() {
return com.google.api.client.util.Base64.decodeBase64(minValue);
}
/**
* Minimum number for the sequence represented as bytes to accommodate large. numbers
* @see #encodeMinValue()
* @param minValue minValue or {@code null} for none
*/
public SequenceEntity setMinValue(java.lang.String minValue) {
this.minValue = minValue;
return this;
}
/**
* Minimum number for the sequence represented as bytes to accommodate large. numbers
* @see #setMinValue()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public SequenceEntity encodeMinValue(byte[] minValue) {
this.minValue = com.google.api.client.util.Base64.encodeBase64URLSafeString(minValue);
return this;
}
/**
* Start number for the sequence represented as bytes to accommodate large. numbers
* @see #decodeStartValue()
* @return value or {@code null} for none
*/
public java.lang.String getStartValue() {
return startValue;
}
/**
* Start number for the sequence represented as bytes to accommodate large. numbers
* @see #getStartValue()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeStartValue() {
return com.google.api.client.util.Base64.decodeBase64(startValue);
}
/**
* Start number for the sequence represented as bytes to accommodate large. numbers
* @see #encodeStartValue()
* @param startValue startValue or {@code null} for none
*/
public SequenceEntity setStartValue(java.lang.String startValue) {
this.startValue = startValue;
return this;
}
/**
* Start number for the sequence represented as bytes to accommodate large. numbers
* @see #setStartValue()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public SequenceEntity encodeStartValue(byte[] startValue) {
this.startValue = com.google.api.client.util.Base64.encodeBase64URLSafeString(startValue);
return this;
}
@Override
public SequenceEntity set(String fieldName, Object value) {
return (SequenceEntity) super.set(fieldName, value);
}
@Override
public SequenceEntity clone() {
return (SequenceEntity) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy