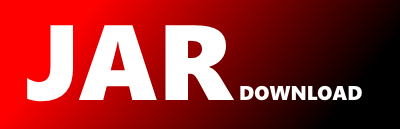
com.google.api.services.datamigration.v1.model.DatabaseEntity Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datamigration.v1.model;
/**
* The base entity type for all the database related entities. The message contains the entity name,
* the name of its parent, the entity type, and the specific details per entity type.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Database Migration API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DatabaseEntity extends com.google.api.client.json.GenericJson {
/**
* Database.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DatabaseInstanceEntity database;
/**
* Function.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FunctionEntity databaseFunction;
/**
* Package.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PackageEntity databasePackage;
/**
* Details about the entity DDL script. Multiple DDL scripts are provided for child entities such
* as a table entity will have one DDL for the table with additional DDLs for each index,
* constraint and such.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List entityDdl;
/**
* The type of the database entity (table, view, index, ...).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String entityType;
/**
* Details about the various issues found for the entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List issues;
/**
* Details about entity mappings. For source tree entities, this holds the draft entities which
* were generated by the mapping rules. For draft tree entities, this holds the source entities
* which were converted to form the draft entity. Destination entities will have no mapping
* details.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List mappings;
/**
* Materialized view.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MaterializedViewEntity materializedView;
/**
* The full name of the parent entity (e.g. schema name).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String parentEntity;
/**
* Schema.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SchemaEntity schema;
/**
* Sequence.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SequenceEntity sequence;
/**
* The short name (e.g. table name) of the entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String shortName;
/**
* Stored procedure.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StoredProcedureEntity storedProcedure;
/**
* Synonym.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SynonymEntity synonym;
/**
* Table.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TableEntity table;
/**
* The type of tree the entity belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String tree;
/**
* UDT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private UDTEntity udt;
/**
* View.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ViewEntity view;
/**
* Database.
* @return value or {@code null} for none
*/
public DatabaseInstanceEntity getDatabase() {
return database;
}
/**
* Database.
* @param database database or {@code null} for none
*/
public DatabaseEntity setDatabase(DatabaseInstanceEntity database) {
this.database = database;
return this;
}
/**
* Function.
* @return value or {@code null} for none
*/
public FunctionEntity getDatabaseFunction() {
return databaseFunction;
}
/**
* Function.
* @param databaseFunction databaseFunction or {@code null} for none
*/
public DatabaseEntity setDatabaseFunction(FunctionEntity databaseFunction) {
this.databaseFunction = databaseFunction;
return this;
}
/**
* Package.
* @return value or {@code null} for none
*/
public PackageEntity getDatabasePackage() {
return databasePackage;
}
/**
* Package.
* @param databasePackage databasePackage or {@code null} for none
*/
public DatabaseEntity setDatabasePackage(PackageEntity databasePackage) {
this.databasePackage = databasePackage;
return this;
}
/**
* Details about the entity DDL script. Multiple DDL scripts are provided for child entities such
* as a table entity will have one DDL for the table with additional DDLs for each index,
* constraint and such.
* @return value or {@code null} for none
*/
public java.util.List getEntityDdl() {
return entityDdl;
}
/**
* Details about the entity DDL script. Multiple DDL scripts are provided for child entities such
* as a table entity will have one DDL for the table with additional DDLs for each index,
* constraint and such.
* @param entityDdl entityDdl or {@code null} for none
*/
public DatabaseEntity setEntityDdl(java.util.List entityDdl) {
this.entityDdl = entityDdl;
return this;
}
/**
* The type of the database entity (table, view, index, ...).
* @return value or {@code null} for none
*/
public java.lang.String getEntityType() {
return entityType;
}
/**
* The type of the database entity (table, view, index, ...).
* @param entityType entityType or {@code null} for none
*/
public DatabaseEntity setEntityType(java.lang.String entityType) {
this.entityType = entityType;
return this;
}
/**
* Details about the various issues found for the entity.
* @return value or {@code null} for none
*/
public java.util.List getIssues() {
return issues;
}
/**
* Details about the various issues found for the entity.
* @param issues issues or {@code null} for none
*/
public DatabaseEntity setIssues(java.util.List issues) {
this.issues = issues;
return this;
}
/**
* Details about entity mappings. For source tree entities, this holds the draft entities which
* were generated by the mapping rules. For draft tree entities, this holds the source entities
* which were converted to form the draft entity. Destination entities will have no mapping
* details.
* @return value or {@code null} for none
*/
public java.util.List getMappings() {
return mappings;
}
/**
* Details about entity mappings. For source tree entities, this holds the draft entities which
* were generated by the mapping rules. For draft tree entities, this holds the source entities
* which were converted to form the draft entity. Destination entities will have no mapping
* details.
* @param mappings mappings or {@code null} for none
*/
public DatabaseEntity setMappings(java.util.List mappings) {
this.mappings = mappings;
return this;
}
/**
* Materialized view.
* @return value or {@code null} for none
*/
public MaterializedViewEntity getMaterializedView() {
return materializedView;
}
/**
* Materialized view.
* @param materializedView materializedView or {@code null} for none
*/
public DatabaseEntity setMaterializedView(MaterializedViewEntity materializedView) {
this.materializedView = materializedView;
return this;
}
/**
* The full name of the parent entity (e.g. schema name).
* @return value or {@code null} for none
*/
public java.lang.String getParentEntity() {
return parentEntity;
}
/**
* The full name of the parent entity (e.g. schema name).
* @param parentEntity parentEntity or {@code null} for none
*/
public DatabaseEntity setParentEntity(java.lang.String parentEntity) {
this.parentEntity = parentEntity;
return this;
}
/**
* Schema.
* @return value or {@code null} for none
*/
public SchemaEntity getSchema() {
return schema;
}
/**
* Schema.
* @param schema schema or {@code null} for none
*/
public DatabaseEntity setSchema(SchemaEntity schema) {
this.schema = schema;
return this;
}
/**
* Sequence.
* @return value or {@code null} for none
*/
public SequenceEntity getSequence() {
return sequence;
}
/**
* Sequence.
* @param sequence sequence or {@code null} for none
*/
public DatabaseEntity setSequence(SequenceEntity sequence) {
this.sequence = sequence;
return this;
}
/**
* The short name (e.g. table name) of the entity.
* @return value or {@code null} for none
*/
public java.lang.String getShortName() {
return shortName;
}
/**
* The short name (e.g. table name) of the entity.
* @param shortName shortName or {@code null} for none
*/
public DatabaseEntity setShortName(java.lang.String shortName) {
this.shortName = shortName;
return this;
}
/**
* Stored procedure.
* @return value or {@code null} for none
*/
public StoredProcedureEntity getStoredProcedure() {
return storedProcedure;
}
/**
* Stored procedure.
* @param storedProcedure storedProcedure or {@code null} for none
*/
public DatabaseEntity setStoredProcedure(StoredProcedureEntity storedProcedure) {
this.storedProcedure = storedProcedure;
return this;
}
/**
* Synonym.
* @return value or {@code null} for none
*/
public SynonymEntity getSynonym() {
return synonym;
}
/**
* Synonym.
* @param synonym synonym or {@code null} for none
*/
public DatabaseEntity setSynonym(SynonymEntity synonym) {
this.synonym = synonym;
return this;
}
/**
* Table.
* @return value or {@code null} for none
*/
public TableEntity getTable() {
return table;
}
/**
* Table.
* @param table table or {@code null} for none
*/
public DatabaseEntity setTable(TableEntity table) {
this.table = table;
return this;
}
/**
* The type of tree the entity belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getTree() {
return tree;
}
/**
* The type of tree the entity belongs to.
* @param tree tree or {@code null} for none
*/
public DatabaseEntity setTree(java.lang.String tree) {
this.tree = tree;
return this;
}
/**
* UDT.
* @return value or {@code null} for none
*/
public UDTEntity getUdt() {
return udt;
}
/**
* UDT.
* @param udt udt or {@code null} for none
*/
public DatabaseEntity setUdt(UDTEntity udt) {
this.udt = udt;
return this;
}
/**
* View.
* @return value or {@code null} for none
*/
public ViewEntity getView() {
return view;
}
/**
* View.
* @param view view or {@code null} for none
*/
public DatabaseEntity setView(ViewEntity view) {
this.view = view;
return this;
}
@Override
public DatabaseEntity set(String fieldName, Object value) {
return (DatabaseEntity) super.set(fieldName, value);
}
@Override
public DatabaseEntity clone() {
return (DatabaseEntity) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy