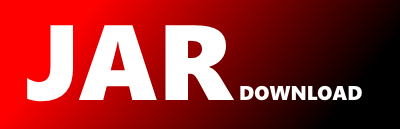
com.google.api.services.datamigration.v1.model.SingleColumnChange Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datamigration.v1.model;
/**
* Options to configure rule type SingleColumnChange. The rule is used to change the properties of a
* column. The rule filter field can refer to one entity. The rule scope can be one of: Column. When
* using this rule, if a field is not specified than the destination column's configuration will be
* the same as the one in the source column..
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Database Migration API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SingleColumnChange extends com.google.api.client.json.GenericJson {
/**
* Optional. Is the column of array type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean array;
/**
* Optional. The length of the array, only relevant if the column type is an array.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer arrayLength;
/**
* Optional. Is the column auto-generated/identity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoGenerated;
/**
* Optional. Charset override - instead of table level charset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String charset;
/**
* Optional. Collation override - instead of table level collation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String collation;
/**
* Optional. Comment associated with the column.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String comment;
/**
* Optional. Custom engine specific features.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map customFeatures;
/**
* Optional. Column data type name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dataType;
/**
* Optional. Column fractional seconds precision - e.g. 2 as in timestamp (2) - when relevant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer fractionalSecondsPrecision;
/**
* Optional. Column length - e.g. 50 as in varchar (50) - when relevant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long length;
/**
* Optional. Is the column nullable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean nullable;
/**
* Optional. Column precision - e.g. 8 as in double (8,2) - when relevant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer precision;
/**
* Optional. Column scale - e.g. 2 as in double (8,2) - when relevant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer scale;
/**
* Optional. Specifies the list of values allowed in the column.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List setValues;
/**
* Optional. Is the column a UDT (User-defined Type).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean udt;
/**
* Optional. Is the column of array type.
* @return value or {@code null} for none
*/
public java.lang.Boolean getArray() {
return array;
}
/**
* Optional. Is the column of array type.
* @param array array or {@code null} for none
*/
public SingleColumnChange setArray(java.lang.Boolean array) {
this.array = array;
return this;
}
/**
* Optional. The length of the array, only relevant if the column type is an array.
* @return value or {@code null} for none
*/
public java.lang.Integer getArrayLength() {
return arrayLength;
}
/**
* Optional. The length of the array, only relevant if the column type is an array.
* @param arrayLength arrayLength or {@code null} for none
*/
public SingleColumnChange setArrayLength(java.lang.Integer arrayLength) {
this.arrayLength = arrayLength;
return this;
}
/**
* Optional. Is the column auto-generated/identity.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAutoGenerated() {
return autoGenerated;
}
/**
* Optional. Is the column auto-generated/identity.
* @param autoGenerated autoGenerated or {@code null} for none
*/
public SingleColumnChange setAutoGenerated(java.lang.Boolean autoGenerated) {
this.autoGenerated = autoGenerated;
return this;
}
/**
* Optional. Charset override - instead of table level charset.
* @return value or {@code null} for none
*/
public java.lang.String getCharset() {
return charset;
}
/**
* Optional. Charset override - instead of table level charset.
* @param charset charset or {@code null} for none
*/
public SingleColumnChange setCharset(java.lang.String charset) {
this.charset = charset;
return this;
}
/**
* Optional. Collation override - instead of table level collation.
* @return value or {@code null} for none
*/
public java.lang.String getCollation() {
return collation;
}
/**
* Optional. Collation override - instead of table level collation.
* @param collation collation or {@code null} for none
*/
public SingleColumnChange setCollation(java.lang.String collation) {
this.collation = collation;
return this;
}
/**
* Optional. Comment associated with the column.
* @return value or {@code null} for none
*/
public java.lang.String getComment() {
return comment;
}
/**
* Optional. Comment associated with the column.
* @param comment comment or {@code null} for none
*/
public SingleColumnChange setComment(java.lang.String comment) {
this.comment = comment;
return this;
}
/**
* Optional. Custom engine specific features.
* @return value or {@code null} for none
*/
public java.util.Map getCustomFeatures() {
return customFeatures;
}
/**
* Optional. Custom engine specific features.
* @param customFeatures customFeatures or {@code null} for none
*/
public SingleColumnChange setCustomFeatures(java.util.Map customFeatures) {
this.customFeatures = customFeatures;
return this;
}
/**
* Optional. Column data type name.
* @return value or {@code null} for none
*/
public java.lang.String getDataType() {
return dataType;
}
/**
* Optional. Column data type name.
* @param dataType dataType or {@code null} for none
*/
public SingleColumnChange setDataType(java.lang.String dataType) {
this.dataType = dataType;
return this;
}
/**
* Optional. Column fractional seconds precision - e.g. 2 as in timestamp (2) - when relevant.
* @return value or {@code null} for none
*/
public java.lang.Integer getFractionalSecondsPrecision() {
return fractionalSecondsPrecision;
}
/**
* Optional. Column fractional seconds precision - e.g. 2 as in timestamp (2) - when relevant.
* @param fractionalSecondsPrecision fractionalSecondsPrecision or {@code null} for none
*/
public SingleColumnChange setFractionalSecondsPrecision(java.lang.Integer fractionalSecondsPrecision) {
this.fractionalSecondsPrecision = fractionalSecondsPrecision;
return this;
}
/**
* Optional. Column length - e.g. 50 as in varchar (50) - when relevant.
* @return value or {@code null} for none
*/
public java.lang.Long getLength() {
return length;
}
/**
* Optional. Column length - e.g. 50 as in varchar (50) - when relevant.
* @param length length or {@code null} for none
*/
public SingleColumnChange setLength(java.lang.Long length) {
this.length = length;
return this;
}
/**
* Optional. Is the column nullable.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNullable() {
return nullable;
}
/**
* Optional. Is the column nullable.
* @param nullable nullable or {@code null} for none
*/
public SingleColumnChange setNullable(java.lang.Boolean nullable) {
this.nullable = nullable;
return this;
}
/**
* Optional. Column precision - e.g. 8 as in double (8,2) - when relevant.
* @return value or {@code null} for none
*/
public java.lang.Integer getPrecision() {
return precision;
}
/**
* Optional. Column precision - e.g. 8 as in double (8,2) - when relevant.
* @param precision precision or {@code null} for none
*/
public SingleColumnChange setPrecision(java.lang.Integer precision) {
this.precision = precision;
return this;
}
/**
* Optional. Column scale - e.g. 2 as in double (8,2) - when relevant.
* @return value or {@code null} for none
*/
public java.lang.Integer getScale() {
return scale;
}
/**
* Optional. Column scale - e.g. 2 as in double (8,2) - when relevant.
* @param scale scale or {@code null} for none
*/
public SingleColumnChange setScale(java.lang.Integer scale) {
this.scale = scale;
return this;
}
/**
* Optional. Specifies the list of values allowed in the column.
* @return value or {@code null} for none
*/
public java.util.List getSetValues() {
return setValues;
}
/**
* Optional. Specifies the list of values allowed in the column.
* @param setValues setValues or {@code null} for none
*/
public SingleColumnChange setSetValues(java.util.List setValues) {
this.setValues = setValues;
return this;
}
/**
* Optional. Is the column a UDT (User-defined Type).
* @return value or {@code null} for none
*/
public java.lang.Boolean getUdt() {
return udt;
}
/**
* Optional. Is the column a UDT (User-defined Type).
* @param udt udt or {@code null} for none
*/
public SingleColumnChange setUdt(java.lang.Boolean udt) {
this.udt = udt;
return this;
}
@Override
public SingleColumnChange set(String fieldName, Object value) {
return (SingleColumnChange) super.set(fieldName, value);
}
@Override
public SingleColumnChange clone() {
return (SingleColumnChange) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy