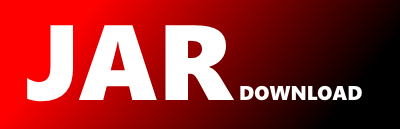
com.google.api.services.datamigration.v1.model.ValueTransformation Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datamigration.v1.model;
/**
* Description of data transformation during migration as part of the ConditionalColumnSetValue.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Database Migration API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ValueTransformation extends com.google.api.client.json.GenericJson {
/**
* Optional. Applies a hash function on the data
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ApplyHash applyHash;
/**
* Optional. Set to max_value - if integer or numeric, will use int.maxvalue, etc
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Empty assignMaxValue;
/**
* Optional. Set to min_value - if integer or numeric, will use int.minvalue, etc
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Empty assignMinValue;
/**
* Optional. Set to null
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Empty assignNull;
/**
* Optional. Set to a specific value (value is converted to fit the target data type)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AssignSpecificValue assignSpecificValue;
/**
* Optional. Filter on relation between source value and compare value of type double.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DoubleComparisonFilter doubleComparison;
/**
* Optional. Filter on relation between source value and compare value of type integer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private IntComparisonFilter intComparison;
/**
* Optional. Value is null
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Empty isNull;
/**
* Optional. Allows the data to change scale
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RoundToScale roundScale;
/**
* Optional. Value is found in the specified list.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ValueListFilter valueList;
/**
* Optional. Applies a hash function on the data
* @return value or {@code null} for none
*/
public ApplyHash getApplyHash() {
return applyHash;
}
/**
* Optional. Applies a hash function on the data
* @param applyHash applyHash or {@code null} for none
*/
public ValueTransformation setApplyHash(ApplyHash applyHash) {
this.applyHash = applyHash;
return this;
}
/**
* Optional. Set to max_value - if integer or numeric, will use int.maxvalue, etc
* @return value or {@code null} for none
*/
public Empty getAssignMaxValue() {
return assignMaxValue;
}
/**
* Optional. Set to max_value - if integer or numeric, will use int.maxvalue, etc
* @param assignMaxValue assignMaxValue or {@code null} for none
*/
public ValueTransformation setAssignMaxValue(Empty assignMaxValue) {
this.assignMaxValue = assignMaxValue;
return this;
}
/**
* Optional. Set to min_value - if integer or numeric, will use int.minvalue, etc
* @return value or {@code null} for none
*/
public Empty getAssignMinValue() {
return assignMinValue;
}
/**
* Optional. Set to min_value - if integer or numeric, will use int.minvalue, etc
* @param assignMinValue assignMinValue or {@code null} for none
*/
public ValueTransformation setAssignMinValue(Empty assignMinValue) {
this.assignMinValue = assignMinValue;
return this;
}
/**
* Optional. Set to null
* @return value or {@code null} for none
*/
public Empty getAssignNull() {
return assignNull;
}
/**
* Optional. Set to null
* @param assignNull assignNull or {@code null} for none
*/
public ValueTransformation setAssignNull(Empty assignNull) {
this.assignNull = assignNull;
return this;
}
/**
* Optional. Set to a specific value (value is converted to fit the target data type)
* @return value or {@code null} for none
*/
public AssignSpecificValue getAssignSpecificValue() {
return assignSpecificValue;
}
/**
* Optional. Set to a specific value (value is converted to fit the target data type)
* @param assignSpecificValue assignSpecificValue or {@code null} for none
*/
public ValueTransformation setAssignSpecificValue(AssignSpecificValue assignSpecificValue) {
this.assignSpecificValue = assignSpecificValue;
return this;
}
/**
* Optional. Filter on relation between source value and compare value of type double.
* @return value or {@code null} for none
*/
public DoubleComparisonFilter getDoubleComparison() {
return doubleComparison;
}
/**
* Optional. Filter on relation between source value and compare value of type double.
* @param doubleComparison doubleComparison or {@code null} for none
*/
public ValueTransformation setDoubleComparison(DoubleComparisonFilter doubleComparison) {
this.doubleComparison = doubleComparison;
return this;
}
/**
* Optional. Filter on relation between source value and compare value of type integer.
* @return value or {@code null} for none
*/
public IntComparisonFilter getIntComparison() {
return intComparison;
}
/**
* Optional. Filter on relation between source value and compare value of type integer.
* @param intComparison intComparison or {@code null} for none
*/
public ValueTransformation setIntComparison(IntComparisonFilter intComparison) {
this.intComparison = intComparison;
return this;
}
/**
* Optional. Value is null
* @return value or {@code null} for none
*/
public Empty getIsNull() {
return isNull;
}
/**
* Optional. Value is null
* @param isNull isNull or {@code null} for none
*/
public ValueTransformation setIsNull(Empty isNull) {
this.isNull = isNull;
return this;
}
/**
* Optional. Allows the data to change scale
* @return value or {@code null} for none
*/
public RoundToScale getRoundScale() {
return roundScale;
}
/**
* Optional. Allows the data to change scale
* @param roundScale roundScale or {@code null} for none
*/
public ValueTransformation setRoundScale(RoundToScale roundScale) {
this.roundScale = roundScale;
return this;
}
/**
* Optional. Value is found in the specified list.
* @return value or {@code null} for none
*/
public ValueListFilter getValueList() {
return valueList;
}
/**
* Optional. Value is found in the specified list.
* @param valueList valueList or {@code null} for none
*/
public ValueTransformation setValueList(ValueListFilter valueList) {
this.valueList = valueList;
return this;
}
@Override
public ValueTransformation set(String fieldName, Object value) {
return (ValueTransformation) super.set(fieldName, value);
}
@Override
public ValueTransformation clone() {
return (ValueTransformation) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy