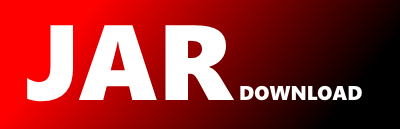
com.google.api.services.datamigration.v1.model.MappingRule Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datamigration.v1.model;
/**
* Definition of a transformation that is to be applied to a group of entities in the source schema.
* Several such transformations can be applied to an entity sequentially to define the corresponding
* entity in the target schema.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Database Migration API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class MappingRule extends com.google.api.client.json.GenericJson {
/**
* Optional. Rule to specify how the data contained in a column should be transformed (such as
* trimmed, rounded, etc) provided that the data meets certain criteria.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ConditionalColumnSetValue conditionalColumnSetValue;
/**
* Optional. Rule to specify how multiple tables should be converted with an additional rowid
* column.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ConvertRowIdToColumn convertRowidColumn;
/**
* Optional. A human readable name
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* Optional. Rule to specify how multiple entities should be relocated into a different schema.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private EntityMove entityMove;
/**
* Required. The rule filter
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MappingRuleFilter filter;
/**
* Optional. Rule to specify the list of columns to include or exclude from a table.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FilterTableColumns filterTableColumns;
/**
* Optional. Rule to specify how multiple columns should be converted to a different data type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MultiColumnDatatypeChange multiColumnDataTypeChange;
/**
* Optional. Rule to specify how multiple entities should be renamed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MultiEntityRename multiEntityRename;
/**
* Full name of the mapping rule resource, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{set}/mappingRule/{rule}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. The timestamp that the revision was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String revisionCreateTime;
/**
* Output only. The revision ID of the mapping rule. A new revision is committed whenever the
* mapping rule is changed in any way. The format is an 8-character hexadecimal string.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String revisionId;
/**
* Required. The order in which the rule is applied. Lower order rules are applied before higher
* value rules so they may end up being overridden.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long ruleOrder;
/**
* Required. The rule scope
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ruleScope;
/**
* Optional. Rule to specify the primary key for a table
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SetTablePrimaryKey setTablePrimaryKey;
/**
* Optional. Rule to specify how a single column is converted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SingleColumnChange singleColumnChange;
/**
* Optional. Rule to specify how a single entity should be renamed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SingleEntityRename singleEntityRename;
/**
* Optional. Rule to specify how a single package is converted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SinglePackageChange singlePackageChange;
/**
* Optional. Rule to change the sql code for an entity, for example, function, procedure.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SourceSqlChange sourceSqlChange;
/**
* Optional. The mapping rule state
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Optional. Rule to specify how the data contained in a column should be transformed (such as
* trimmed, rounded, etc) provided that the data meets certain criteria.
* @return value or {@code null} for none
*/
public ConditionalColumnSetValue getConditionalColumnSetValue() {
return conditionalColumnSetValue;
}
/**
* Optional. Rule to specify how the data contained in a column should be transformed (such as
* trimmed, rounded, etc) provided that the data meets certain criteria.
* @param conditionalColumnSetValue conditionalColumnSetValue or {@code null} for none
*/
public MappingRule setConditionalColumnSetValue(ConditionalColumnSetValue conditionalColumnSetValue) {
this.conditionalColumnSetValue = conditionalColumnSetValue;
return this;
}
/**
* Optional. Rule to specify how multiple tables should be converted with an additional rowid
* column.
* @return value or {@code null} for none
*/
public ConvertRowIdToColumn getConvertRowidColumn() {
return convertRowidColumn;
}
/**
* Optional. Rule to specify how multiple tables should be converted with an additional rowid
* column.
* @param convertRowidColumn convertRowidColumn or {@code null} for none
*/
public MappingRule setConvertRowidColumn(ConvertRowIdToColumn convertRowidColumn) {
this.convertRowidColumn = convertRowidColumn;
return this;
}
/**
* Optional. A human readable name
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* Optional. A human readable name
* @param displayName displayName or {@code null} for none
*/
public MappingRule setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* Optional. Rule to specify how multiple entities should be relocated into a different schema.
* @return value or {@code null} for none
*/
public EntityMove getEntityMove() {
return entityMove;
}
/**
* Optional. Rule to specify how multiple entities should be relocated into a different schema.
* @param entityMove entityMove or {@code null} for none
*/
public MappingRule setEntityMove(EntityMove entityMove) {
this.entityMove = entityMove;
return this;
}
/**
* Required. The rule filter
* @return value or {@code null} for none
*/
public MappingRuleFilter getFilter() {
return filter;
}
/**
* Required. The rule filter
* @param filter filter or {@code null} for none
*/
public MappingRule setFilter(MappingRuleFilter filter) {
this.filter = filter;
return this;
}
/**
* Optional. Rule to specify the list of columns to include or exclude from a table.
* @return value or {@code null} for none
*/
public FilterTableColumns getFilterTableColumns() {
return filterTableColumns;
}
/**
* Optional. Rule to specify the list of columns to include or exclude from a table.
* @param filterTableColumns filterTableColumns or {@code null} for none
*/
public MappingRule setFilterTableColumns(FilterTableColumns filterTableColumns) {
this.filterTableColumns = filterTableColumns;
return this;
}
/**
* Optional. Rule to specify how multiple columns should be converted to a different data type.
* @return value or {@code null} for none
*/
public MultiColumnDatatypeChange getMultiColumnDataTypeChange() {
return multiColumnDataTypeChange;
}
/**
* Optional. Rule to specify how multiple columns should be converted to a different data type.
* @param multiColumnDataTypeChange multiColumnDataTypeChange or {@code null} for none
*/
public MappingRule setMultiColumnDataTypeChange(MultiColumnDatatypeChange multiColumnDataTypeChange) {
this.multiColumnDataTypeChange = multiColumnDataTypeChange;
return this;
}
/**
* Optional. Rule to specify how multiple entities should be renamed.
* @return value or {@code null} for none
*/
public MultiEntityRename getMultiEntityRename() {
return multiEntityRename;
}
/**
* Optional. Rule to specify how multiple entities should be renamed.
* @param multiEntityRename multiEntityRename or {@code null} for none
*/
public MappingRule setMultiEntityRename(MultiEntityRename multiEntityRename) {
this.multiEntityRename = multiEntityRename;
return this;
}
/**
* Full name of the mapping rule resource, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{set}/mappingRule/{rule}.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Full name of the mapping rule resource, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{set}/mappingRule/{rule}.
* @param name name or {@code null} for none
*/
public MappingRule setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. The timestamp that the revision was created.
* @return value or {@code null} for none
*/
public String getRevisionCreateTime() {
return revisionCreateTime;
}
/**
* Output only. The timestamp that the revision was created.
* @param revisionCreateTime revisionCreateTime or {@code null} for none
*/
public MappingRule setRevisionCreateTime(String revisionCreateTime) {
this.revisionCreateTime = revisionCreateTime;
return this;
}
/**
* Output only. The revision ID of the mapping rule. A new revision is committed whenever the
* mapping rule is changed in any way. The format is an 8-character hexadecimal string.
* @return value or {@code null} for none
*/
public java.lang.String getRevisionId() {
return revisionId;
}
/**
* Output only. The revision ID of the mapping rule. A new revision is committed whenever the
* mapping rule is changed in any way. The format is an 8-character hexadecimal string.
* @param revisionId revisionId or {@code null} for none
*/
public MappingRule setRevisionId(java.lang.String revisionId) {
this.revisionId = revisionId;
return this;
}
/**
* Required. The order in which the rule is applied. Lower order rules are applied before higher
* value rules so they may end up being overridden.
* @return value or {@code null} for none
*/
public java.lang.Long getRuleOrder() {
return ruleOrder;
}
/**
* Required. The order in which the rule is applied. Lower order rules are applied before higher
* value rules so they may end up being overridden.
* @param ruleOrder ruleOrder or {@code null} for none
*/
public MappingRule setRuleOrder(java.lang.Long ruleOrder) {
this.ruleOrder = ruleOrder;
return this;
}
/**
* Required. The rule scope
* @return value or {@code null} for none
*/
public java.lang.String getRuleScope() {
return ruleScope;
}
/**
* Required. The rule scope
* @param ruleScope ruleScope or {@code null} for none
*/
public MappingRule setRuleScope(java.lang.String ruleScope) {
this.ruleScope = ruleScope;
return this;
}
/**
* Optional. Rule to specify the primary key for a table
* @return value or {@code null} for none
*/
public SetTablePrimaryKey getSetTablePrimaryKey() {
return setTablePrimaryKey;
}
/**
* Optional. Rule to specify the primary key for a table
* @param setTablePrimaryKey setTablePrimaryKey or {@code null} for none
*/
public MappingRule setSetTablePrimaryKey(SetTablePrimaryKey setTablePrimaryKey) {
this.setTablePrimaryKey = setTablePrimaryKey;
return this;
}
/**
* Optional. Rule to specify how a single column is converted.
* @return value or {@code null} for none
*/
public SingleColumnChange getSingleColumnChange() {
return singleColumnChange;
}
/**
* Optional. Rule to specify how a single column is converted.
* @param singleColumnChange singleColumnChange or {@code null} for none
*/
public MappingRule setSingleColumnChange(SingleColumnChange singleColumnChange) {
this.singleColumnChange = singleColumnChange;
return this;
}
/**
* Optional. Rule to specify how a single entity should be renamed.
* @return value or {@code null} for none
*/
public SingleEntityRename getSingleEntityRename() {
return singleEntityRename;
}
/**
* Optional. Rule to specify how a single entity should be renamed.
* @param singleEntityRename singleEntityRename or {@code null} for none
*/
public MappingRule setSingleEntityRename(SingleEntityRename singleEntityRename) {
this.singleEntityRename = singleEntityRename;
return this;
}
/**
* Optional. Rule to specify how a single package is converted.
* @return value or {@code null} for none
*/
public SinglePackageChange getSinglePackageChange() {
return singlePackageChange;
}
/**
* Optional. Rule to specify how a single package is converted.
* @param singlePackageChange singlePackageChange or {@code null} for none
*/
public MappingRule setSinglePackageChange(SinglePackageChange singlePackageChange) {
this.singlePackageChange = singlePackageChange;
return this;
}
/**
* Optional. Rule to change the sql code for an entity, for example, function, procedure.
* @return value or {@code null} for none
*/
public SourceSqlChange getSourceSqlChange() {
return sourceSqlChange;
}
/**
* Optional. Rule to change the sql code for an entity, for example, function, procedure.
* @param sourceSqlChange sourceSqlChange or {@code null} for none
*/
public MappingRule setSourceSqlChange(SourceSqlChange sourceSqlChange) {
this.sourceSqlChange = sourceSqlChange;
return this;
}
/**
* Optional. The mapping rule state
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Optional. The mapping rule state
* @param state state or {@code null} for none
*/
public MappingRule setState(java.lang.String state) {
this.state = state;
return this;
}
@Override
public MappingRule set(String fieldName, Object value) {
return (MappingRule) super.set(fieldName, value);
}
@Override
public MappingRule clone() {
return (MappingRule) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy