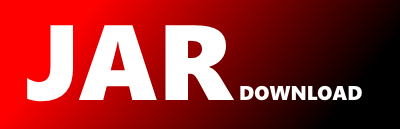
com.google.api.services.datamigration.v1.DatabaseMigrationService Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.datamigration.v1;
/**
* Service definition for DatabaseMigrationService (v1).
*
*
* Manage Cloud Database Migration Service resources on Google Cloud Platform.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link DatabaseMigrationServiceRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class DatabaseMigrationService extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Database Migration API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://datamigration.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://datamigration.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public DatabaseMigrationService(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
DatabaseMigrationService(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.Projects.List request = datamigration.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.Locations.List request = datamigration.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Fetches a set of static IP addresses that need to be allowlisted by the customer when using the
* static-IP connectivity method.
*
* Create a request for the method "locations.fetchStaticIps".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link FetchStaticIps#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name for the location for which static IPs should be returned. Must be in the
* format `projects/locations`.
* @return the request
*/
public FetchStaticIps fetchStaticIps(java.lang.String name) throws java.io.IOException {
FetchStaticIps result = new FetchStaticIps(name);
initialize(result);
return result;
}
public class FetchStaticIps extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:fetchStaticIps";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Fetches a set of static IP addresses that need to be allowlisted by the customer when using the
* static-IP connectivity method.
*
* Create a request for the method "locations.fetchStaticIps".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link FetchStaticIps#execute()} method to invoke the remote
* operation. {@link FetchStaticIps#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param name Required. The resource name for the location for which static IPs should be returned. Must be in the
* format `projects/locations`.
* @since 1.13
*/
protected FetchStaticIps(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.FetchStaticIpsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public FetchStaticIps set$Xgafv(java.lang.String $Xgafv) {
return (FetchStaticIps) super.set$Xgafv($Xgafv);
}
@Override
public FetchStaticIps setAccessToken(java.lang.String accessToken) {
return (FetchStaticIps) super.setAccessToken(accessToken);
}
@Override
public FetchStaticIps setAlt(java.lang.String alt) {
return (FetchStaticIps) super.setAlt(alt);
}
@Override
public FetchStaticIps setCallback(java.lang.String callback) {
return (FetchStaticIps) super.setCallback(callback);
}
@Override
public FetchStaticIps setFields(java.lang.String fields) {
return (FetchStaticIps) super.setFields(fields);
}
@Override
public FetchStaticIps setKey(java.lang.String key) {
return (FetchStaticIps) super.setKey(key);
}
@Override
public FetchStaticIps setOauthToken(java.lang.String oauthToken) {
return (FetchStaticIps) super.setOauthToken(oauthToken);
}
@Override
public FetchStaticIps setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FetchStaticIps) super.setPrettyPrint(prettyPrint);
}
@Override
public FetchStaticIps setQuotaUser(java.lang.String quotaUser) {
return (FetchStaticIps) super.setQuotaUser(quotaUser);
}
@Override
public FetchStaticIps setUploadType(java.lang.String uploadType) {
return (FetchStaticIps) super.setUploadType(uploadType);
}
@Override
public FetchStaticIps setUploadProtocol(java.lang.String uploadProtocol) {
return (FetchStaticIps) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name for the location for which static IPs should be returned.
* Must be in the format `projects/locations`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name for the location for which static IPs should be returned. Must be in
the format `projects/locations`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name for the location for which static IPs should be returned.
* Must be in the format `projects/locations`.
*/
public FetchStaticIps setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** Maximum number of IPs to return. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of IPs to return.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum number of IPs to return. */
public FetchStaticIps setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A page token, received from a previous `FetchStaticIps` call. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `FetchStaticIps` call.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A page token, received from a previous `FetchStaticIps` call. */
public FetchStaticIps setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public FetchStaticIps set(String parameterName, Object value) {
return (FetchStaticIps) super.set(parameterName, value);
}
}
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
`"displayName=tokyo"`, and is documented in more detail in [AIP-160](https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the `next_page_token` field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ConnectionProfiles collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.ConnectionProfiles.List request = datamigration.connectionProfiles().list(parameters ...)}
*
*
* @return the resource collection
*/
public ConnectionProfiles connectionProfiles() {
return new ConnectionProfiles();
}
/**
* The "connectionProfiles" collection of methods.
*/
public class ConnectionProfiles {
/**
* Creates a new connection profile in a given project and location.
*
* Create a request for the method "connectionProfiles.create".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent which owns this collection of connection profiles.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConnectionProfile}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datamigration.v1.model.ConnectionProfile content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/connectionProfiles";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new connection profile in a given project and location.
*
* Create a request for the method "connectionProfiles.create".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent which owns this collection of connection profiles.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConnectionProfile}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datamigration.v1.model.ConnectionProfile content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent which owns this collection of connection profiles. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent which owns this collection of connection profiles.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent which owns this collection of connection profiles. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Required. The connection profile identifier. */
@com.google.api.client.util.Key
private java.lang.String connectionProfileId;
/** Required. The connection profile identifier.
*/
public java.lang.String getConnectionProfileId() {
return connectionProfileId;
}
/** Required. The connection profile identifier. */
public Create setConnectionProfileId(java.lang.String connectionProfileId) {
this.connectionProfileId = connectionProfileId;
return this;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique ID used to identify the request. If the server receives two requests with the
same ID, then the second request is ignored. It is recommended to always set this value to a UUID.
The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Optional. Create the connection profile without validating it. The default is false.
* Only supported for Oracle connection profiles.
*/
@com.google.api.client.util.Key
private java.lang.Boolean skipValidation;
/** Optional. Create the connection profile without validating it. The default is false. Only supported
for Oracle connection profiles.
*/
public java.lang.Boolean getSkipValidation() {
return skipValidation;
}
/**
* Optional. Create the connection profile without validating it. The default is false.
* Only supported for Oracle connection profiles.
*/
public Create setSkipValidation(java.lang.Boolean skipValidation) {
this.skipValidation = skipValidation;
return this;
}
/**
* Optional. Only validate the connection profile, but don't create any resources. The
* default is false. Only supported for Oracle connection profiles.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. Only validate the connection profile, but don't create any resources. The default is
false. Only supported for Oracle connection profiles.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. Only validate the connection profile, but don't create any resources. The
* default is false. Only supported for Oracle connection profiles.
*/
public Create setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single Database Migration Service connection profile. A connection profile can only be
* deleted if it is not in use by any active migration jobs.
*
* Create a request for the method "connectionProfiles.delete".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the connection profile resource to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
/**
* Deletes a single Database Migration Service connection profile. A connection profile can only
* be deleted if it is not in use by any active migration jobs.
*
* Create a request for the method "connectionProfiles.delete".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the connection profile resource to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DatabaseMigrationService.this, "DELETE", REST_PATH, null, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the connection profile resource to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the connection profile resource to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the connection profile resource to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
this.name = name;
return this;
}
/**
* In case of force delete, the CloudSQL replica database is also deleted (only for
* CloudSQL connection profile).
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** In case of force delete, the CloudSQL replica database is also deleted (only for CloudSQL
connection profile).
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* In case of force delete, the CloudSQL replica database is also deleted (only for
* CloudSQL connection profile).
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique ID used to identify the request. If the server receives two requests with the same ID,
then the second request is ignored. It is recommended to always set this value to a UUID. The ID
must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum
length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single connection profile.
*
* Create a request for the method "connectionProfiles.get".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the connection profile resource to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
/**
* Gets details of a single connection profile.
*
* Create a request for the method "connectionProfiles.get".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the connection profile resource to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ConnectionProfile.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the connection profile resource to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the connection profile resource to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the connection profile resource to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "connectionProfiles.getIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "connectionProfiles.getIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Retrieves a list of all connection profiles in a given project and location.
*
* Create a request for the method "connectionProfiles.list".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent which owns this collection of connection profiles.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/connectionProfiles";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Retrieves a list of all connection profiles in a given project and location.
*
* Create a request for the method "connectionProfiles.list".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent which owns this collection of connection profiles.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ListConnectionProfilesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent which owns this collection of connection profiles. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent which owns this collection of connection profiles.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent which owns this collection of connection profiles. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that filters connection profiles listed in the response. The
* expression must specify the field name, a comparison operator, and the value that you
* want to use for filtering. The value must be a string, a number, or a boolean. The
* comparison operator must be either =, !=, >, or <. For example, list connection
* profiles created this year by specifying **createTime %gt;
* 2020-01-01T00:00:00.000000000Z**. You can also filter nested fields. For example, you
* could specify **mySql.username = %lt;my_username%gt;** to list all connection profiles
* configured to connect with a specific username.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters connection profiles listed in the response. The expression must
specify the field name, a comparison operator, and the value that you want to use for filtering.
The value must be a string, a number, or a boolean. The comparison operator must be either =, !=,
>, or <. For example, list connection profiles created this year by specifying **createTime %gt;
2020-01-01T00:00:00.000000000Z**. You can also filter nested fields. For example, you could specify
**mySql.username = %lt;my_username%gt;** to list all connection profiles configured to connect with
a specific username.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters connection profiles listed in the response. The
* expression must specify the field name, a comparison operator, and the value that you
* want to use for filtering. The value must be a string, a number, or a boolean. The
* comparison operator must be either =, !=, >, or <. For example, list connection
* profiles created this year by specifying **createTime %gt;
* 2020-01-01T00:00:00.000000000Z**. You can also filter nested fields. For example, you
* could specify **mySql.username = %lt;my_username%gt;** to list all connection profiles
* configured to connect with a specific username.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** A comma-separated list of fields to order results according to. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** A comma-separated list of fields to order results according to.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** A comma-separated list of fields to order results according to. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of connection profiles to return. The service may return fewer than
* this value. If unspecified, at most 50 connection profiles will be returned. The
* maximum value is 1000; values above 1000 are coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of connection profiles to return. The service may return fewer than this value.
If unspecified, at most 50 connection profiles will be returned. The maximum value is 1000; values
above 1000 are coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of connection profiles to return. The service may return fewer than
* this value. If unspecified, at most 50 connection profiles will be returned. The
* maximum value is 1000; values above 1000 are coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListConnectionProfiles` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListConnectionProfiles` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListConnectionProfiles` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListConnectionProfiles` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListConnectionProfiles` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListConnectionProfiles` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Update the configuration of a single connection profile.
*
* Create a request for the method "connectionProfiles.patch".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name of this connection profile resource in the form of
* projects/{project}/locations/{location}/connectionProfiles/{connectionProfile}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConnectionProfile}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datamigration.v1.model.ConnectionProfile content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
/**
* Update the configuration of a single connection profile.
*
* Create a request for the method "connectionProfiles.patch".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of this connection profile resource in the form of
* projects/{project}/locations/{location}/connectionProfiles/{connectionProfile}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConnectionProfile}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datamigration.v1.model.ConnectionProfile content) {
super(DatabaseMigrationService.this, "PATCH", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of this connection profile resource in the form of
* projects/{project}/locations/{location}/connectionProfiles/{connectionProfile}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of this connection profile resource in the form of
projects/{project}/locations/{location}/connectionProfiles/{connectionProfile}.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of this connection profile resource in the form of
* projects/{project}/locations/{location}/connectionProfiles/{connectionProfile}.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique ID used to identify the request. If the server receives two requests with the
same ID, then the second request is ignored. It is recommended to always set this value to a UUID.
The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Optional. Update the connection profile without validating it. The default is false.
* Only supported for Oracle connection profiles.
*/
@com.google.api.client.util.Key
private java.lang.Boolean skipValidation;
/** Optional. Update the connection profile without validating it. The default is false. Only supported
for Oracle connection profiles.
*/
public java.lang.Boolean getSkipValidation() {
return skipValidation;
}
/**
* Optional. Update the connection profile without validating it. The default is false.
* Only supported for Oracle connection profiles.
*/
public Patch setSkipValidation(java.lang.Boolean skipValidation) {
this.skipValidation = skipValidation;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten by the update in
* the conversion workspace resource.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten by the update in the
conversion workspace resource.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten by the update in
* the conversion workspace resource.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* Optional. Only validate the connection profile, but don't update any resources. The
* default is false. Only supported for Oracle connection profiles.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. Only validate the connection profile, but don't update any resources. The default is
false. Only supported for Oracle connection profiles.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. Only validate the connection profile, but don't update any resources. The
* default is false. Only supported for Oracle connection profiles.
*/
public Patch setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "connectionProfiles.setIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "connectionProfiles.setIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "connectionProfiles.testIamPermissions".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "connectionProfiles.testIamPermissions".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/connectionProfiles/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ConversionWorkspaces collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.ConversionWorkspaces.List request = datamigration.conversionWorkspaces().list(parameters ...)}
*
*
* @return the resource collection
*/
public ConversionWorkspaces conversionWorkspaces() {
return new ConversionWorkspaces();
}
/**
* The "conversionWorkspaces" collection of methods.
*/
public class ConversionWorkspaces {
/**
* Applies draft tree onto a specific destination database.
*
* Create a request for the method "conversionWorkspaces.apply".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Apply#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the conversion workspace resource for which to apply the draft tree. Must be
* in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ApplyConversionWorkspaceRequest}
* @return the request
*/
public Apply apply(java.lang.String name, com.google.api.services.datamigration.v1.model.ApplyConversionWorkspaceRequest content) throws java.io.IOException {
Apply result = new Apply(name, content);
initialize(result);
return result;
}
public class Apply extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:apply";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Applies draft tree onto a specific destination database.
*
* Create a request for the method "conversionWorkspaces.apply".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Apply#execute()} method to invoke the remote operation.
* {@link
* Apply#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the conversion workspace resource for which to apply the draft tree. Must be
* in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ApplyConversionWorkspaceRequest}
* @since 1.13
*/
protected Apply(java.lang.String name, com.google.api.services.datamigration.v1.model.ApplyConversionWorkspaceRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Apply set$Xgafv(java.lang.String $Xgafv) {
return (Apply) super.set$Xgafv($Xgafv);
}
@Override
public Apply setAccessToken(java.lang.String accessToken) {
return (Apply) super.setAccessToken(accessToken);
}
@Override
public Apply setAlt(java.lang.String alt) {
return (Apply) super.setAlt(alt);
}
@Override
public Apply setCallback(java.lang.String callback) {
return (Apply) super.setCallback(callback);
}
@Override
public Apply setFields(java.lang.String fields) {
return (Apply) super.setFields(fields);
}
@Override
public Apply setKey(java.lang.String key) {
return (Apply) super.setKey(key);
}
@Override
public Apply setOauthToken(java.lang.String oauthToken) {
return (Apply) super.setOauthToken(oauthToken);
}
@Override
public Apply setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Apply) super.setPrettyPrint(prettyPrint);
}
@Override
public Apply setQuotaUser(java.lang.String quotaUser) {
return (Apply) super.setQuotaUser(quotaUser);
}
@Override
public Apply setUploadType(java.lang.String uploadType) {
return (Apply) super.setUploadType(uploadType);
}
@Override
public Apply setUploadProtocol(java.lang.String uploadProtocol) {
return (Apply) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the conversion workspace resource for which to apply the draft
* tree. Must be in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the conversion workspace resource for which to apply the draft tree. Must be
in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the conversion workspace resource for which to apply the draft
* tree. Must be in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public Apply setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Apply set(String parameterName, Object value) {
return (Apply) super.set(parameterName, value);
}
}
/**
* Marks all the data in the conversion workspace as committed.
*
* Create a request for the method "conversionWorkspaces.commit".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Commit#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the conversion workspace resource to commit.
* @param content the {@link com.google.api.services.datamigration.v1.model.CommitConversionWorkspaceRequest}
* @return the request
*/
public Commit commit(java.lang.String name, com.google.api.services.datamigration.v1.model.CommitConversionWorkspaceRequest content) throws java.io.IOException {
Commit result = new Commit(name, content);
initialize(result);
return result;
}
public class Commit extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:commit";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Marks all the data in the conversion workspace as committed.
*
* Create a request for the method "conversionWorkspaces.commit".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Commit#execute()} method to invoke the remote operation.
* {@link
* Commit#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the conversion workspace resource to commit.
* @param content the {@link com.google.api.services.datamigration.v1.model.CommitConversionWorkspaceRequest}
* @since 1.13
*/
protected Commit(java.lang.String name, com.google.api.services.datamigration.v1.model.CommitConversionWorkspaceRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Commit set$Xgafv(java.lang.String $Xgafv) {
return (Commit) super.set$Xgafv($Xgafv);
}
@Override
public Commit setAccessToken(java.lang.String accessToken) {
return (Commit) super.setAccessToken(accessToken);
}
@Override
public Commit setAlt(java.lang.String alt) {
return (Commit) super.setAlt(alt);
}
@Override
public Commit setCallback(java.lang.String callback) {
return (Commit) super.setCallback(callback);
}
@Override
public Commit setFields(java.lang.String fields) {
return (Commit) super.setFields(fields);
}
@Override
public Commit setKey(java.lang.String key) {
return (Commit) super.setKey(key);
}
@Override
public Commit setOauthToken(java.lang.String oauthToken) {
return (Commit) super.setOauthToken(oauthToken);
}
@Override
public Commit setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Commit) super.setPrettyPrint(prettyPrint);
}
@Override
public Commit setQuotaUser(java.lang.String quotaUser) {
return (Commit) super.setQuotaUser(quotaUser);
}
@Override
public Commit setUploadType(java.lang.String uploadType) {
return (Commit) super.setUploadType(uploadType);
}
@Override
public Commit setUploadProtocol(java.lang.String uploadProtocol) {
return (Commit) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the conversion workspace resource to commit. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the conversion workspace resource to commit.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the conversion workspace resource to commit. */
public Commit setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Commit set(String parameterName, Object value) {
return (Commit) super.set(parameterName, value);
}
}
/**
* Creates a draft tree schema for the destination database.
*
* Create a request for the method "conversionWorkspaces.convert".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Convert#execute()} method to invoke the remote operation.
*
* @param name Name of the conversion workspace resource to convert in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConvertConversionWorkspaceRequest}
* @return the request
*/
public Convert convert(java.lang.String name, com.google.api.services.datamigration.v1.model.ConvertConversionWorkspaceRequest content) throws java.io.IOException {
Convert result = new Convert(name, content);
initialize(result);
return result;
}
public class Convert extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:convert";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Creates a draft tree schema for the destination database.
*
* Create a request for the method "conversionWorkspaces.convert".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Convert#execute()} method to invoke the remote operation.
* {@link
* Convert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the conversion workspace resource to convert in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConvertConversionWorkspaceRequest}
* @since 1.13
*/
protected Convert(java.lang.String name, com.google.api.services.datamigration.v1.model.ConvertConversionWorkspaceRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Convert set$Xgafv(java.lang.String $Xgafv) {
return (Convert) super.set$Xgafv($Xgafv);
}
@Override
public Convert setAccessToken(java.lang.String accessToken) {
return (Convert) super.setAccessToken(accessToken);
}
@Override
public Convert setAlt(java.lang.String alt) {
return (Convert) super.setAlt(alt);
}
@Override
public Convert setCallback(java.lang.String callback) {
return (Convert) super.setCallback(callback);
}
@Override
public Convert setFields(java.lang.String fields) {
return (Convert) super.setFields(fields);
}
@Override
public Convert setKey(java.lang.String key) {
return (Convert) super.setKey(key);
}
@Override
public Convert setOauthToken(java.lang.String oauthToken) {
return (Convert) super.setOauthToken(oauthToken);
}
@Override
public Convert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Convert) super.setPrettyPrint(prettyPrint);
}
@Override
public Convert setQuotaUser(java.lang.String quotaUser) {
return (Convert) super.setQuotaUser(quotaUser);
}
@Override
public Convert setUploadType(java.lang.String uploadType) {
return (Convert) super.setUploadType(uploadType);
}
@Override
public Convert setUploadProtocol(java.lang.String uploadProtocol) {
return (Convert) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the conversion workspace resource to convert in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the conversion workspace resource to convert in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the conversion workspace resource to convert in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public Convert setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Convert set(String parameterName, Object value) {
return (Convert) super.set(parameterName, value);
}
}
/**
* Creates a new conversion workspace in a given project and location.
*
* Create a request for the method "conversionWorkspaces.create".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent which owns this collection of conversion workspaces.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConversionWorkspace}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datamigration.v1.model.ConversionWorkspace content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/conversionWorkspaces";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new conversion workspace in a given project and location.
*
* Create a request for the method "conversionWorkspaces.create".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent which owns this collection of conversion workspaces.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConversionWorkspace}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datamigration.v1.model.ConversionWorkspace content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent which owns this collection of conversion workspaces. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent which owns this collection of conversion workspaces.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent which owns this collection of conversion workspaces. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Required. The ID of the conversion workspace to create. */
@com.google.api.client.util.Key
private java.lang.String conversionWorkspaceId;
/** Required. The ID of the conversion workspace to create.
*/
public java.lang.String getConversionWorkspaceId() {
return conversionWorkspaceId;
}
/** Required. The ID of the conversion workspace to create. */
public Create setConversionWorkspaceId(java.lang.String conversionWorkspaceId) {
this.conversionWorkspaceId = conversionWorkspaceId;
return this;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique ID used to identify the request. If the server receives two requests with the same ID,
then the second request is ignored. It is recommended to always set this value to a UUID. The ID
must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum
length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single conversion workspace.
*
* Create a request for the method "conversionWorkspaces.delete".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the conversion workspace resource to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Deletes a single conversion workspace.
*
* Create a request for the method "conversionWorkspaces.delete".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the conversion workspace resource to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DatabaseMigrationService.this, "DELETE", REST_PATH, null, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the conversion workspace resource to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the conversion workspace resource to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the conversion workspace resource to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
/**
* Force delete the conversion workspace, even if there's a running migration that is
* using the workspace.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Force delete the conversion workspace, even if there's a running migration that is using the
workspace.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Force delete the conversion workspace, even if there's a running migration that is
* using the workspace.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique ID used to identify the request. If the server receives two requests with the same ID,
then the second request is ignored. It is recommended to always set this value to a UUID. The ID
must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum
length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a list of committed revisions of a specific conversion workspace.
*
* Create a request for the method "conversionWorkspaces.describeConversionWorkspaceRevisions".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link DescribeConversionWorkspaceRevisions#execute()} method to invoke the
* remote operation.
*
* @param conversionWorkspace Required. Name of the conversion workspace resource whose revisions are listed. Must be in the form
* of: projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @return the request
*/
public DescribeConversionWorkspaceRevisions describeConversionWorkspaceRevisions(java.lang.String conversionWorkspace) throws java.io.IOException {
DescribeConversionWorkspaceRevisions result = new DescribeConversionWorkspaceRevisions(conversionWorkspace);
initialize(result);
return result;
}
public class DescribeConversionWorkspaceRevisions extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+conversionWorkspace}:describeConversionWorkspaceRevisions";
private final java.util.regex.Pattern CONVERSION_WORKSPACE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Retrieves a list of committed revisions of a specific conversion workspace.
*
* Create a request for the method "conversionWorkspaces.describeConversionWorkspaceRevisions".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link DescribeConversionWorkspaceRevisions#execute()} method to
* invoke the remote operation. {@link DescribeConversionWorkspaceRevisions#initialize(com.goo
* gle.api.client.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize
* this instance immediately after invoking the constructor.
*
* @param conversionWorkspace Required. Name of the conversion workspace resource whose revisions are listed. Must be in the form
* of: projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @since 1.13
*/
protected DescribeConversionWorkspaceRevisions(java.lang.String conversionWorkspace) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.DescribeConversionWorkspaceRevisionsResponse.class);
this.conversionWorkspace = com.google.api.client.util.Preconditions.checkNotNull(conversionWorkspace, "Required parameter conversionWorkspace must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CONVERSION_WORKSPACE_PATTERN.matcher(conversionWorkspace).matches(),
"Parameter conversionWorkspace must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public DescribeConversionWorkspaceRevisions set$Xgafv(java.lang.String $Xgafv) {
return (DescribeConversionWorkspaceRevisions) super.set$Xgafv($Xgafv);
}
@Override
public DescribeConversionWorkspaceRevisions setAccessToken(java.lang.String accessToken) {
return (DescribeConversionWorkspaceRevisions) super.setAccessToken(accessToken);
}
@Override
public DescribeConversionWorkspaceRevisions setAlt(java.lang.String alt) {
return (DescribeConversionWorkspaceRevisions) super.setAlt(alt);
}
@Override
public DescribeConversionWorkspaceRevisions setCallback(java.lang.String callback) {
return (DescribeConversionWorkspaceRevisions) super.setCallback(callback);
}
@Override
public DescribeConversionWorkspaceRevisions setFields(java.lang.String fields) {
return (DescribeConversionWorkspaceRevisions) super.setFields(fields);
}
@Override
public DescribeConversionWorkspaceRevisions setKey(java.lang.String key) {
return (DescribeConversionWorkspaceRevisions) super.setKey(key);
}
@Override
public DescribeConversionWorkspaceRevisions setOauthToken(java.lang.String oauthToken) {
return (DescribeConversionWorkspaceRevisions) super.setOauthToken(oauthToken);
}
@Override
public DescribeConversionWorkspaceRevisions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DescribeConversionWorkspaceRevisions) super.setPrettyPrint(prettyPrint);
}
@Override
public DescribeConversionWorkspaceRevisions setQuotaUser(java.lang.String quotaUser) {
return (DescribeConversionWorkspaceRevisions) super.setQuotaUser(quotaUser);
}
@Override
public DescribeConversionWorkspaceRevisions setUploadType(java.lang.String uploadType) {
return (DescribeConversionWorkspaceRevisions) super.setUploadType(uploadType);
}
@Override
public DescribeConversionWorkspaceRevisions setUploadProtocol(java.lang.String uploadProtocol) {
return (DescribeConversionWorkspaceRevisions) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the conversion workspace resource whose revisions are listed. Must be
* in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String conversionWorkspace;
/** Required. Name of the conversion workspace resource whose revisions are listed. Must be in the form
of: projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getConversionWorkspace() {
return conversionWorkspace;
}
/**
* Required. Name of the conversion workspace resource whose revisions are listed. Must be
* in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public DescribeConversionWorkspaceRevisions setConversionWorkspace(java.lang.String conversionWorkspace) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CONVERSION_WORKSPACE_PATTERN.matcher(conversionWorkspace).matches(),
"Parameter conversionWorkspace must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.conversionWorkspace = conversionWorkspace;
return this;
}
/** Optional. Optional filter to request a specific commit ID. */
@com.google.api.client.util.Key
private java.lang.String commitId;
/** Optional. Optional filter to request a specific commit ID.
*/
public java.lang.String getCommitId() {
return commitId;
}
/** Optional. Optional filter to request a specific commit ID. */
public DescribeConversionWorkspaceRevisions setCommitId(java.lang.String commitId) {
this.commitId = commitId;
return this;
}
@Override
public DescribeConversionWorkspaceRevisions set(String parameterName, Object value) {
return (DescribeConversionWorkspaceRevisions) super.set(parameterName, value);
}
}
/**
* Describes the database entities tree for a specific conversion workspace and a specific tree
* type. Database entities are not resources like conversion workspaces or mapping rules, and they
* can't be created, updated or deleted. Instead, they are simple data objects describing the
* structure of the client database.
*
* Create a request for the method "conversionWorkspaces.describeDatabaseEntities".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link DescribeDatabaseEntities#execute()} method to invoke the remote
* operation.
*
* @param conversionWorkspace Required. Name of the conversion workspace resource whose database entities are described. Must be
* in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @return the request
*/
public DescribeDatabaseEntities describeDatabaseEntities(java.lang.String conversionWorkspace) throws java.io.IOException {
DescribeDatabaseEntities result = new DescribeDatabaseEntities(conversionWorkspace);
initialize(result);
return result;
}
public class DescribeDatabaseEntities extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+conversionWorkspace}:describeDatabaseEntities";
private final java.util.regex.Pattern CONVERSION_WORKSPACE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Describes the database entities tree for a specific conversion workspace and a specific tree
* type. Database entities are not resources like conversion workspaces or mapping rules, and they
* can't be created, updated or deleted. Instead, they are simple data objects describing the
* structure of the client database.
*
* Create a request for the method "conversionWorkspaces.describeDatabaseEntities".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link DescribeDatabaseEntities#execute()} method to invoke the
* remote operation. {@link DescribeDatabaseEntities#initialize(com.google.api.client.googleap
* is.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param conversionWorkspace Required. Name of the conversion workspace resource whose database entities are described. Must be
* in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @since 1.13
*/
protected DescribeDatabaseEntities(java.lang.String conversionWorkspace) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.DescribeDatabaseEntitiesResponse.class);
this.conversionWorkspace = com.google.api.client.util.Preconditions.checkNotNull(conversionWorkspace, "Required parameter conversionWorkspace must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CONVERSION_WORKSPACE_PATTERN.matcher(conversionWorkspace).matches(),
"Parameter conversionWorkspace must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public DescribeDatabaseEntities set$Xgafv(java.lang.String $Xgafv) {
return (DescribeDatabaseEntities) super.set$Xgafv($Xgafv);
}
@Override
public DescribeDatabaseEntities setAccessToken(java.lang.String accessToken) {
return (DescribeDatabaseEntities) super.setAccessToken(accessToken);
}
@Override
public DescribeDatabaseEntities setAlt(java.lang.String alt) {
return (DescribeDatabaseEntities) super.setAlt(alt);
}
@Override
public DescribeDatabaseEntities setCallback(java.lang.String callback) {
return (DescribeDatabaseEntities) super.setCallback(callback);
}
@Override
public DescribeDatabaseEntities setFields(java.lang.String fields) {
return (DescribeDatabaseEntities) super.setFields(fields);
}
@Override
public DescribeDatabaseEntities setKey(java.lang.String key) {
return (DescribeDatabaseEntities) super.setKey(key);
}
@Override
public DescribeDatabaseEntities setOauthToken(java.lang.String oauthToken) {
return (DescribeDatabaseEntities) super.setOauthToken(oauthToken);
}
@Override
public DescribeDatabaseEntities setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DescribeDatabaseEntities) super.setPrettyPrint(prettyPrint);
}
@Override
public DescribeDatabaseEntities setQuotaUser(java.lang.String quotaUser) {
return (DescribeDatabaseEntities) super.setQuotaUser(quotaUser);
}
@Override
public DescribeDatabaseEntities setUploadType(java.lang.String uploadType) {
return (DescribeDatabaseEntities) super.setUploadType(uploadType);
}
@Override
public DescribeDatabaseEntities setUploadProtocol(java.lang.String uploadProtocol) {
return (DescribeDatabaseEntities) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the conversion workspace resource whose database entities are
* described. Must be in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String conversionWorkspace;
/** Required. Name of the conversion workspace resource whose database entities are described. Must be
in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getConversionWorkspace() {
return conversionWorkspace;
}
/**
* Required. Name of the conversion workspace resource whose database entities are
* described. Must be in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public DescribeDatabaseEntities setConversionWorkspace(java.lang.String conversionWorkspace) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CONVERSION_WORKSPACE_PATTERN.matcher(conversionWorkspace).matches(),
"Parameter conversionWorkspace must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.conversionWorkspace = conversionWorkspace;
return this;
}
/**
* Optional. Request a specific commit ID. If not specified, the entities from the latest
* commit are returned.
*/
@com.google.api.client.util.Key
private java.lang.String commitId;
/** Optional. Request a specific commit ID. If not specified, the entities from the latest commit are
returned.
*/
public java.lang.String getCommitId() {
return commitId;
}
/**
* Optional. Request a specific commit ID. If not specified, the entities from the latest
* commit are returned.
*/
public DescribeDatabaseEntities setCommitId(java.lang.String commitId) {
this.commitId = commitId;
return this;
}
/** Optional. Filter the returned entities based on AIP-160 standard. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filter the returned entities based on AIP-160 standard.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filter the returned entities based on AIP-160 standard. */
public DescribeDatabaseEntities setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. The maximum number of entities to return. The service may return fewer
* entities than the value specifies.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of entities to return. The service may return fewer entities than the
value specifies.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of entities to return. The service may return fewer
* entities than the value specifies.
*/
public DescribeDatabaseEntities setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. The nextPageToken value received in the previous call to
* conversionWorkspace.describeDatabaseEntities, used in the subsequent request to
* retrieve the next page of results. On first call this should be left blank. When
* paginating, all other parameters provided to
* conversionWorkspace.describeDatabaseEntities must match the call that provided the page
* token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The nextPageToken value received in the previous call to
conversionWorkspace.describeDatabaseEntities, used in the subsequent request to retrieve the next
page of results. On first call this should be left blank. When paginating, all other parameters
provided to conversionWorkspace.describeDatabaseEntities must match the call that provided the page
token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The nextPageToken value received in the previous call to
* conversionWorkspace.describeDatabaseEntities, used in the subsequent request to
* retrieve the next page of results. On first call this should be left blank. When
* paginating, all other parameters provided to
* conversionWorkspace.describeDatabaseEntities must match the call that provided the page
* token.
*/
public DescribeDatabaseEntities setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Required. The tree to fetch. */
@com.google.api.client.util.Key
private java.lang.String tree;
/** Required. The tree to fetch.
*/
public java.lang.String getTree() {
return tree;
}
/** Required. The tree to fetch. */
public DescribeDatabaseEntities setTree(java.lang.String tree) {
this.tree = tree;
return this;
}
/**
* Optional. Whether to retrieve the latest committed version of the entities or the
* latest version. This field is ignored if a specific commit_id is specified.
*/
@com.google.api.client.util.Key
private java.lang.Boolean uncommitted;
/** Optional. Whether to retrieve the latest committed version of the entities or the latest version.
This field is ignored if a specific commit_id is specified.
*/
public java.lang.Boolean getUncommitted() {
return uncommitted;
}
/**
* Optional. Whether to retrieve the latest committed version of the entities or the
* latest version. This field is ignored if a specific commit_id is specified.
*/
public DescribeDatabaseEntities setUncommitted(java.lang.Boolean uncommitted) {
this.uncommitted = uncommitted;
return this;
}
/** Optional. Results view based on AIP-157 */
@com.google.api.client.util.Key
private java.lang.String view;
/** Optional. Results view based on AIP-157
*/
public java.lang.String getView() {
return view;
}
/** Optional. Results view based on AIP-157 */
public DescribeDatabaseEntities setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public DescribeDatabaseEntities set(String parameterName, Object value) {
return (DescribeDatabaseEntities) super.set(parameterName, value);
}
}
/**
* Gets details of a single conversion workspace.
*
* Create a request for the method "conversionWorkspaces.get".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the conversion workspace resource to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Gets details of a single conversion workspace.
*
* Create a request for the method "conversionWorkspaces.get".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the conversion workspace resource to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ConversionWorkspace.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the conversion workspace resource to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the conversion workspace resource to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the conversion workspace resource to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "conversionWorkspaces.getIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "conversionWorkspaces.getIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists conversion workspaces in a given project and location.
*
* Create a request for the method "conversionWorkspaces.list".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent which owns this collection of conversion workspaces.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/conversionWorkspaces";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists conversion workspaces in a given project and location.
*
* Create a request for the method "conversionWorkspaces.list".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent which owns this collection of conversion workspaces.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ListConversionWorkspacesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent which owns this collection of conversion workspaces. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent which owns this collection of conversion workspaces.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent which owns this collection of conversion workspaces. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that filters conversion workspaces listed in the response. The
* expression must specify the field name, a comparison operator, and the value that you
* want to use for filtering. The value must be a string, a number, or a boolean. The
* comparison operator must be either =, !=, >, or <. For example, list conversion
* workspaces created this year by specifying **createTime %gt;
* 2020-01-01T00:00:00.000000000Z.** You can also filter nested fields. For example, you
* could specify **source.version = "12.c.1"** to select all conversion workspaces with
* source database version equal to 12.c.1.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters conversion workspaces listed in the response. The expression must
specify the field name, a comparison operator, and the value that you want to use for filtering.
The value must be a string, a number, or a boolean. The comparison operator must be either =, !=,
>, or <. For example, list conversion workspaces created this year by specifying **createTime %gt;
2020-01-01T00:00:00.000000000Z.** You can also filter nested fields. For example, you could specify
**source.version = "12.c.1"** to select all conversion workspaces with source database version
equal to 12.c.1.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters conversion workspaces listed in the response. The
* expression must specify the field name, a comparison operator, and the value that you
* want to use for filtering. The value must be a string, a number, or a boolean. The
* comparison operator must be either =, !=, >, or <. For example, list conversion
* workspaces created this year by specifying **createTime %gt;
* 2020-01-01T00:00:00.000000000Z.** You can also filter nested fields. For example, you
* could specify **source.version = "12.c.1"** to select all conversion workspaces with
* source database version equal to 12.c.1.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of conversion workspaces to return. The service may return fewer
* than this value. If unspecified, at most 50 sets are returned.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of conversion workspaces to return. The service may return fewer than this
value. If unspecified, at most 50 sets are returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of conversion workspaces to return. The service may return fewer
* than this value. If unspecified, at most 50 sets are returned.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The nextPageToken value received in the previous call to conversionWorkspaces.list,
* used in the subsequent request to retrieve the next page of results. On first call this
* should be left blank. When paginating, all other parameters provided to
* conversionWorkspaces.list must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The nextPageToken value received in the previous call to conversionWorkspaces.list, used in the
subsequent request to retrieve the next page of results. On first call this should be left blank.
When paginating, all other parameters provided to conversionWorkspaces.list must match the call
that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The nextPageToken value received in the previous call to conversionWorkspaces.list,
* used in the subsequent request to retrieve the next page of results. On first call this
* should be left blank. When paginating, all other parameters provided to
* conversionWorkspaces.list must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the parameters of a single conversion workspace.
*
* Create a request for the method "conversionWorkspaces.patch".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Full name of the workspace resource, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConversionWorkspace}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datamigration.v1.model.ConversionWorkspace content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Updates the parameters of a single conversion workspace.
*
* Create a request for the method "conversionWorkspaces.patch".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Full name of the workspace resource, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ConversionWorkspace}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datamigration.v1.model.ConversionWorkspace content) {
super(DatabaseMigrationService.this, "PATCH", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Full name of the workspace resource, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Full name of the workspace resource, in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getName() {
return name;
}
/**
* Full name of the workspace resource, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique ID used to identify the request. If the server receives two requests with the same ID,
then the second request is ignored. It is recommended to always set this value to a UUID. The ID
must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum
length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten by the update in
* the conversion workspace resource.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten by the update in the
conversion workspace resource.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten by the update in
* the conversion workspace resource.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Rolls back a conversion workspace to the last committed snapshot.
*
* Create a request for the method "conversionWorkspaces.rollback".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Rollback#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the conversion workspace resource to roll back to.
* @param content the {@link com.google.api.services.datamigration.v1.model.RollbackConversionWorkspaceRequest}
* @return the request
*/
public Rollback rollback(java.lang.String name, com.google.api.services.datamigration.v1.model.RollbackConversionWorkspaceRequest content) throws java.io.IOException {
Rollback result = new Rollback(name, content);
initialize(result);
return result;
}
public class Rollback extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:rollback";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Rolls back a conversion workspace to the last committed snapshot.
*
* Create a request for the method "conversionWorkspaces.rollback".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Rollback#execute()} method to invoke the remote operation.
* {@link
* Rollback#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the conversion workspace resource to roll back to.
* @param content the {@link com.google.api.services.datamigration.v1.model.RollbackConversionWorkspaceRequest}
* @since 1.13
*/
protected Rollback(java.lang.String name, com.google.api.services.datamigration.v1.model.RollbackConversionWorkspaceRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Rollback set$Xgafv(java.lang.String $Xgafv) {
return (Rollback) super.set$Xgafv($Xgafv);
}
@Override
public Rollback setAccessToken(java.lang.String accessToken) {
return (Rollback) super.setAccessToken(accessToken);
}
@Override
public Rollback setAlt(java.lang.String alt) {
return (Rollback) super.setAlt(alt);
}
@Override
public Rollback setCallback(java.lang.String callback) {
return (Rollback) super.setCallback(callback);
}
@Override
public Rollback setFields(java.lang.String fields) {
return (Rollback) super.setFields(fields);
}
@Override
public Rollback setKey(java.lang.String key) {
return (Rollback) super.setKey(key);
}
@Override
public Rollback setOauthToken(java.lang.String oauthToken) {
return (Rollback) super.setOauthToken(oauthToken);
}
@Override
public Rollback setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rollback) super.setPrettyPrint(prettyPrint);
}
@Override
public Rollback setQuotaUser(java.lang.String quotaUser) {
return (Rollback) super.setQuotaUser(quotaUser);
}
@Override
public Rollback setUploadType(java.lang.String uploadType) {
return (Rollback) super.setUploadType(uploadType);
}
@Override
public Rollback setUploadProtocol(java.lang.String uploadProtocol) {
return (Rollback) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the conversion workspace resource to roll back to. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the conversion workspace resource to roll back to.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the conversion workspace resource to roll back to. */
public Rollback setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Rollback set(String parameterName, Object value) {
return (Rollback) super.set(parameterName, value);
}
}
/**
* Searches/lists the background jobs for a specific conversion workspace. The background jobs are
* not resources like conversion workspaces or mapping rules, and they can't be created, updated or
* deleted. Instead, they are a way to expose the data plane jobs log.
*
* Create a request for the method "conversionWorkspaces.searchBackgroundJobs".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link SearchBackgroundJobs#execute()} method to invoke the remote
* operation.
*
* @param conversionWorkspace Required. Name of the conversion workspace resource whose jobs are listed, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @return the request
*/
public SearchBackgroundJobs searchBackgroundJobs(java.lang.String conversionWorkspace) throws java.io.IOException {
SearchBackgroundJobs result = new SearchBackgroundJobs(conversionWorkspace);
initialize(result);
return result;
}
public class SearchBackgroundJobs extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+conversionWorkspace}:searchBackgroundJobs";
private final java.util.regex.Pattern CONVERSION_WORKSPACE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Searches/lists the background jobs for a specific conversion workspace. The background jobs are
* not resources like conversion workspaces or mapping rules, and they can't be created, updated
* or deleted. Instead, they are a way to expose the data plane jobs log.
*
* Create a request for the method "conversionWorkspaces.searchBackgroundJobs".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link SearchBackgroundJobs#execute()} method to invoke the
* remote operation. {@link SearchBackgroundJobs#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param conversionWorkspace Required. Name of the conversion workspace resource whose jobs are listed, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @since 1.13
*/
protected SearchBackgroundJobs(java.lang.String conversionWorkspace) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.SearchBackgroundJobsResponse.class);
this.conversionWorkspace = com.google.api.client.util.Preconditions.checkNotNull(conversionWorkspace, "Required parameter conversionWorkspace must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CONVERSION_WORKSPACE_PATTERN.matcher(conversionWorkspace).matches(),
"Parameter conversionWorkspace must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public SearchBackgroundJobs set$Xgafv(java.lang.String $Xgafv) {
return (SearchBackgroundJobs) super.set$Xgafv($Xgafv);
}
@Override
public SearchBackgroundJobs setAccessToken(java.lang.String accessToken) {
return (SearchBackgroundJobs) super.setAccessToken(accessToken);
}
@Override
public SearchBackgroundJobs setAlt(java.lang.String alt) {
return (SearchBackgroundJobs) super.setAlt(alt);
}
@Override
public SearchBackgroundJobs setCallback(java.lang.String callback) {
return (SearchBackgroundJobs) super.setCallback(callback);
}
@Override
public SearchBackgroundJobs setFields(java.lang.String fields) {
return (SearchBackgroundJobs) super.setFields(fields);
}
@Override
public SearchBackgroundJobs setKey(java.lang.String key) {
return (SearchBackgroundJobs) super.setKey(key);
}
@Override
public SearchBackgroundJobs setOauthToken(java.lang.String oauthToken) {
return (SearchBackgroundJobs) super.setOauthToken(oauthToken);
}
@Override
public SearchBackgroundJobs setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SearchBackgroundJobs) super.setPrettyPrint(prettyPrint);
}
@Override
public SearchBackgroundJobs setQuotaUser(java.lang.String quotaUser) {
return (SearchBackgroundJobs) super.setQuotaUser(quotaUser);
}
@Override
public SearchBackgroundJobs setUploadType(java.lang.String uploadType) {
return (SearchBackgroundJobs) super.setUploadType(uploadType);
}
@Override
public SearchBackgroundJobs setUploadProtocol(java.lang.String uploadProtocol) {
return (SearchBackgroundJobs) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the conversion workspace resource whose jobs are listed, in the form
* of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String conversionWorkspace;
/** Required. Name of the conversion workspace resource whose jobs are listed, in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getConversionWorkspace() {
return conversionWorkspace;
}
/**
* Required. Name of the conversion workspace resource whose jobs are listed, in the form
* of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public SearchBackgroundJobs setConversionWorkspace(java.lang.String conversionWorkspace) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(CONVERSION_WORKSPACE_PATTERN.matcher(conversionWorkspace).matches(),
"Parameter conversionWorkspace must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.conversionWorkspace = conversionWorkspace;
return this;
}
/**
* Optional. If provided, only returns jobs that completed until (not including) the given
* timestamp.
*/
@com.google.api.client.util.Key
private String completedUntilTime;
/** Optional. If provided, only returns jobs that completed until (not including) the given timestamp.
*/
public String getCompletedUntilTime() {
return completedUntilTime;
}
/**
* Optional. If provided, only returns jobs that completed until (not including) the given
* timestamp.
*/
public SearchBackgroundJobs setCompletedUntilTime(String completedUntilTime) {
this.completedUntilTime = completedUntilTime;
return this;
}
/**
* Optional. The maximum number of jobs to return. The service may return fewer than this
* value. If unspecified, at most 100 jobs are returned. The maximum value is 100; values
* above 100 are coerced to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxSize;
/** Optional. The maximum number of jobs to return. The service may return fewer than this value. If
unspecified, at most 100 jobs are returned. The maximum value is 100; values above 100 are coerced
to 100.
*/
public java.lang.Integer getMaxSize() {
return maxSize;
}
/**
* Optional. The maximum number of jobs to return. The service may return fewer than this
* value. If unspecified, at most 100 jobs are returned. The maximum value is 100; values
* above 100 are coerced to 100.
*/
public SearchBackgroundJobs setMaxSize(java.lang.Integer maxSize) {
this.maxSize = maxSize;
return this;
}
/** Optional. Whether or not to return just the most recent job per job type, */
@com.google.api.client.util.Key
private java.lang.Boolean returnMostRecentPerJobType;
/** Optional. Whether or not to return just the most recent job per job type,
*/
public java.lang.Boolean getReturnMostRecentPerJobType() {
return returnMostRecentPerJobType;
}
/** Optional. Whether or not to return just the most recent job per job type, */
public SearchBackgroundJobs setReturnMostRecentPerJobType(java.lang.Boolean returnMostRecentPerJobType) {
this.returnMostRecentPerJobType = returnMostRecentPerJobType;
return this;
}
@Override
public SearchBackgroundJobs set(String parameterName, Object value) {
return (SearchBackgroundJobs) super.set(parameterName, value);
}
}
/**
* Imports a snapshot of the source database into the conversion workspace.
*
* Create a request for the method "conversionWorkspaces.seed".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Seed#execute()} method to invoke the remote operation.
*
* @param name Name of the conversion workspace resource to seed with new database structure, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.SeedConversionWorkspaceRequest}
* @return the request
*/
public Seed seed(java.lang.String name, com.google.api.services.datamigration.v1.model.SeedConversionWorkspaceRequest content) throws java.io.IOException {
Seed result = new Seed(name, content);
initialize(result);
return result;
}
public class Seed extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:seed";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Imports a snapshot of the source database into the conversion workspace.
*
* Create a request for the method "conversionWorkspaces.seed".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Seed#execute()} method to invoke the remote operation.
* {@link Seed#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the conversion workspace resource to seed with new database structure, in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.SeedConversionWorkspaceRequest}
* @since 1.13
*/
protected Seed(java.lang.String name, com.google.api.services.datamigration.v1.model.SeedConversionWorkspaceRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Seed set$Xgafv(java.lang.String $Xgafv) {
return (Seed) super.set$Xgafv($Xgafv);
}
@Override
public Seed setAccessToken(java.lang.String accessToken) {
return (Seed) super.setAccessToken(accessToken);
}
@Override
public Seed setAlt(java.lang.String alt) {
return (Seed) super.setAlt(alt);
}
@Override
public Seed setCallback(java.lang.String callback) {
return (Seed) super.setCallback(callback);
}
@Override
public Seed setFields(java.lang.String fields) {
return (Seed) super.setFields(fields);
}
@Override
public Seed setKey(java.lang.String key) {
return (Seed) super.setKey(key);
}
@Override
public Seed setOauthToken(java.lang.String oauthToken) {
return (Seed) super.setOauthToken(oauthToken);
}
@Override
public Seed setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Seed) super.setPrettyPrint(prettyPrint);
}
@Override
public Seed setQuotaUser(java.lang.String quotaUser) {
return (Seed) super.setQuotaUser(quotaUser);
}
@Override
public Seed setUploadType(java.lang.String uploadType) {
return (Seed) super.setUploadType(uploadType);
}
@Override
public Seed setUploadProtocol(java.lang.String uploadProtocol) {
return (Seed) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the conversion workspace resource to seed with new database structure, in the
* form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the conversion workspace resource to seed with new database structure, in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the conversion workspace resource to seed with new database structure, in the
* form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public Seed setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Seed set(String parameterName, Object value) {
return (Seed) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "conversionWorkspaces.setIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "conversionWorkspaces.setIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "conversionWorkspaces.testIamPermissions".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "conversionWorkspaces.testIamPermissions".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the MappingRules collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.MappingRules.List request = datamigration.mappingRules().list(parameters ...)}
*
*
* @return the resource collection
*/
public MappingRules mappingRules() {
return new MappingRules();
}
/**
* The "mappingRules" collection of methods.
*/
public class MappingRules {
/**
* Creates a new mapping rule for a given conversion workspace.
*
* Create a request for the method "mappingRules.create".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent which owns this collection of mapping rules.
* @param content the {@link com.google.api.services.datamigration.v1.model.MappingRule}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datamigration.v1.model.MappingRule content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/mappingRules";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Creates a new mapping rule for a given conversion workspace.
*
* Create a request for the method "mappingRules.create".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent which owns this collection of mapping rules.
* @param content the {@link com.google.api.services.datamigration.v1.model.MappingRule}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datamigration.v1.model.MappingRule content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.MappingRule.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent which owns this collection of mapping rules. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent which owns this collection of mapping rules.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent which owns this collection of mapping rules. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.parent = parent;
return this;
}
/** Required. The ID of the rule to create. */
@com.google.api.client.util.Key
private java.lang.String mappingRuleId;
/** Required. The ID of the rule to create.
*/
public java.lang.String getMappingRuleId() {
return mappingRuleId;
}
/** Required. The ID of the rule to create. */
public Create setMappingRuleId(java.lang.String mappingRuleId) {
this.mappingRuleId = mappingRuleId;
return this;
}
/**
* A unique ID used to identify the request. If the server receives two requests with
* the same ID, then the second request is ignored. It is recommended to always set this
* value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique ID used to identify the request. If the server receives two requests with the same ID,
then the second request is ignored. It is recommended to always set this value to a UUID. The ID
must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum
length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique ID used to identify the request. If the server receives two requests with
* the same ID, then the second request is ignored. It is recommended to always set this
* value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single mapping rule.
*
* Create a request for the method "mappingRules.delete".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the mapping rule resource to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+/mappingRules/[^/]+$");
/**
* Deletes a single mapping rule.
*
* Create a request for the method "mappingRules.delete".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the mapping rule resource to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DatabaseMigrationService.this, "DELETE", REST_PATH, null, com.google.api.services.datamigration.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+/mappingRules/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the mapping rule resource to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the mapping rule resource to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the mapping rule resource to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+/mappingRules/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two
* requests with the same ID, then the second request is ignored. It is recommended to
* always set this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers
* (0-9), underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique ID used to identify the request. If the server receives two requests with the
same ID, then the second request is ignored. It is recommended to always set this value to a UUID.
The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two
* requests with the same ID, then the second request is ignored. It is recommended to
* always set this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers
* (0-9), underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the details of a mapping rule.
*
* Create a request for the method "mappingRules.get".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the mapping rule resource to get. Example:
* conversionWorkspaces/123/mappingRules/rule123 In order to retrieve a previous revision of
* the mapping rule, also provide the revision ID. Example:
* conversionWorkspace/123/mappingRules/rule123@c7cfa2a8c7cfa2a8c7cfa2a8c7cfa2a8
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+/mappingRules/[^/]+$");
/**
* Gets the details of a mapping rule.
*
* Create a request for the method "mappingRules.get".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the mapping rule resource to get. Example:
* conversionWorkspaces/123/mappingRules/rule123 In order to retrieve a previous revision of
* the mapping rule, also provide the revision ID. Example:
* conversionWorkspace/123/mappingRules/rule123@c7cfa2a8c7cfa2a8c7cfa2a8c7cfa2a8
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.MappingRule.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+/mappingRules/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the mapping rule resource to get. Example:
* conversionWorkspaces/123/mappingRules/rule123 In order to retrieve a previous
* revision of the mapping rule, also provide the revision ID. Example:
* conversionWorkspace/123/mappingRules/rule123@c7cfa2a8c7cfa2a8c7cfa2a8c7cfa2a8
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the mapping rule resource to get. Example:
conversionWorkspaces/123/mappingRules/rule123 In order to retrieve a previous revision of the
mapping rule, also provide the revision ID. Example:
conversionWorkspace/123/mappingRules/rule123@c7cfa2a8c7cfa2a8c7cfa2a8c7cfa2a8
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the mapping rule resource to get. Example:
* conversionWorkspaces/123/mappingRules/rule123 In order to retrieve a previous
* revision of the mapping rule, also provide the revision ID. Example:
* conversionWorkspace/123/mappingRules/rule123@c7cfa2a8c7cfa2a8c7cfa2a8c7cfa2a8
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+/mappingRules/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports the mapping rules for a given conversion workspace. Supports various formats of external
* rules files.
*
* Create a request for the method "mappingRules.import".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link DatabaseMigrationServiceImport#execute()} method to invoke the remote
* operation.
*
* @param parent Required. Name of the conversion workspace resource to import the rules to in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ImportMappingRulesRequest}
* @return the request
*/
public DatabaseMigrationServiceImport datamigrationImport(java.lang.String parent, com.google.api.services.datamigration.v1.model.ImportMappingRulesRequest content) throws java.io.IOException {
DatabaseMigrationServiceImport result = new DatabaseMigrationServiceImport(parent, content);
initialize(result);
return result;
}
public class DatabaseMigrationServiceImport extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/mappingRules:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Imports the mapping rules for a given conversion workspace. Supports various formats of
* external rules files.
*
* Create a request for the method "mappingRules.import".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link DatabaseMigrationServiceImport#execute()} method to invoke
* the remote operation. {@link DatabaseMigrationServiceImport#initialize(com.google.api.clien
* t.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param parent Required. Name of the conversion workspace resource to import the rules to in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @param content the {@link com.google.api.services.datamigration.v1.model.ImportMappingRulesRequest}
* @since 1.13
*/
protected DatabaseMigrationServiceImport(java.lang.String parent, com.google.api.services.datamigration.v1.model.ImportMappingRulesRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public DatabaseMigrationServiceImport set$Xgafv(java.lang.String $Xgafv) {
return (DatabaseMigrationServiceImport) super.set$Xgafv($Xgafv);
}
@Override
public DatabaseMigrationServiceImport setAccessToken(java.lang.String accessToken) {
return (DatabaseMigrationServiceImport) super.setAccessToken(accessToken);
}
@Override
public DatabaseMigrationServiceImport setAlt(java.lang.String alt) {
return (DatabaseMigrationServiceImport) super.setAlt(alt);
}
@Override
public DatabaseMigrationServiceImport setCallback(java.lang.String callback) {
return (DatabaseMigrationServiceImport) super.setCallback(callback);
}
@Override
public DatabaseMigrationServiceImport setFields(java.lang.String fields) {
return (DatabaseMigrationServiceImport) super.setFields(fields);
}
@Override
public DatabaseMigrationServiceImport setKey(java.lang.String key) {
return (DatabaseMigrationServiceImport) super.setKey(key);
}
@Override
public DatabaseMigrationServiceImport setOauthToken(java.lang.String oauthToken) {
return (DatabaseMigrationServiceImport) super.setOauthToken(oauthToken);
}
@Override
public DatabaseMigrationServiceImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DatabaseMigrationServiceImport) super.setPrettyPrint(prettyPrint);
}
@Override
public DatabaseMigrationServiceImport setQuotaUser(java.lang.String quotaUser) {
return (DatabaseMigrationServiceImport) super.setQuotaUser(quotaUser);
}
@Override
public DatabaseMigrationServiceImport setUploadType(java.lang.String uploadType) {
return (DatabaseMigrationServiceImport) super.setUploadType(uploadType);
}
@Override
public DatabaseMigrationServiceImport setUploadProtocol(java.lang.String uploadProtocol) {
return (DatabaseMigrationServiceImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the conversion workspace resource to import the rules to in the
* form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the conversion workspace resource to import the rules to in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the conversion workspace resource to import the rules to in the
* form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public DatabaseMigrationServiceImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public DatabaseMigrationServiceImport set(String parameterName, Object value) {
return (DatabaseMigrationServiceImport) super.set(parameterName, value);
}
}
/**
* Lists the mapping rules for a specific conversion workspace.
*
* Create a request for the method "mappingRules.list".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Name of the conversion workspace resource whose mapping rules are listed in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/mappingRules";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
/**
* Lists the mapping rules for a specific conversion workspace.
*
* Create a request for the method "mappingRules.list".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Name of the conversion workspace resource whose mapping rules are listed in the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ListMappingRulesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the conversion workspace resource whose mapping rules are listed in
* the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Name of the conversion workspace resource whose mapping rules are listed in the form of:
projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Name of the conversion workspace resource whose mapping rules are listed in
* the form of:
* projects/{project}/locations/{location}/conversionWorkspaces/{conversion_workspace}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/conversionWorkspaces/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of rules to return. The service may return fewer than this value.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of rules to return. The service may return fewer than this value.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of rules to return. The service may return fewer than this value.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The nextPageToken value received in the previous call to mappingRules.list, used in
* the subsequent request to retrieve the next page of results. On first call this
* should be left blank. When paginating, all other parameters provided to
* mappingRules.list must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The nextPageToken value received in the previous call to mappingRules.list, used in the subsequent
request to retrieve the next page of results. On first call this should be left blank. When
paginating, all other parameters provided to mappingRules.list must match the call that provided
the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The nextPageToken value received in the previous call to mappingRules.list, used in
* the subsequent request to retrieve the next page of results. On first call this
* should be left blank. When paginating, all other parameters provided to
* mappingRules.list must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the MigrationJobs collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.MigrationJobs.List request = datamigration.migrationJobs().list(parameters ...)}
*
*
* @return the resource collection
*/
public MigrationJobs migrationJobs() {
return new MigrationJobs();
}
/**
* The "migrationJobs" collection of methods.
*/
public class MigrationJobs {
/**
* Creates a new migration job in a given project and location.
*
* Create a request for the method "migrationJobs.create".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent which owns this collection of migration jobs.
* @param content the {@link com.google.api.services.datamigration.v1.model.MigrationJob}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datamigration.v1.model.MigrationJob content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/migrationJobs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new migration job in a given project and location.
*
* Create a request for the method "migrationJobs.create".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent which owns this collection of migration jobs.
* @param content the {@link com.google.api.services.datamigration.v1.model.MigrationJob}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datamigration.v1.model.MigrationJob content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent which owns this collection of migration jobs. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent which owns this collection of migration jobs.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent which owns this collection of migration jobs. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Required. The ID of the instance to create. */
@com.google.api.client.util.Key
private java.lang.String migrationJobId;
/** Required. The ID of the instance to create.
*/
public java.lang.String getMigrationJobId() {
return migrationJobId;
}
/** Required. The ID of the instance to create. */
public Create setMigrationJobId(java.lang.String migrationJobId) {
this.migrationJobId = migrationJobId;
return this;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique ID used to identify the request. If the server receives two requests with the
same ID, then the second request is ignored. It is recommended to always set this value to a UUID.
The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single migration job.
*
* Create a request for the method "migrationJobs.delete".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the migration job resource to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Deletes a single migration job.
*
* Create a request for the method "migrationJobs.delete".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the migration job resource to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DatabaseMigrationService.this, "DELETE", REST_PATH, null, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the migration job resource to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the migration job resource to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the migration job resource to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
/**
* The destination CloudSQL connection profile is always deleted with the migration job.
* In case of force delete, the destination CloudSQL replica database is also deleted.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** The destination CloudSQL connection profile is always deleted with the migration job. In case of
force delete, the destination CloudSQL replica database is also deleted.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* The destination CloudSQL connection profile is always deleted with the migration job.
* In case of force delete, the destination CloudSQL replica database is also deleted.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique ID used to identify the request. If the server receives two requests with the same ID,
then the second request is ignored. It is recommended to always set this value to a UUID. The ID
must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum
length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Demotes the destination database to become a read replica of the source. This is applicable for
* the following migrations: 1. MySQL to Cloud SQL for MySQL 2. PostgreSQL to Cloud SQL for
* PostgreSQL 3. PostgreSQL to AlloyDB for PostgreSQL.
*
* Create a request for the method "migrationJobs.demoteDestination".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link DemoteDestination#execute()} method to invoke the remote operation.
*
* @param name Name of the migration job resource to demote its destination.
* @param content the {@link com.google.api.services.datamigration.v1.model.DemoteDestinationRequest}
* @return the request
*/
public DemoteDestination demoteDestination(java.lang.String name, com.google.api.services.datamigration.v1.model.DemoteDestinationRequest content) throws java.io.IOException {
DemoteDestination result = new DemoteDestination(name, content);
initialize(result);
return result;
}
public class DemoteDestination extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:demoteDestination";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Demotes the destination database to become a read replica of the source. This is applicable for
* the following migrations: 1. MySQL to Cloud SQL for MySQL 2. PostgreSQL to Cloud SQL for
* PostgreSQL 3. PostgreSQL to AlloyDB for PostgreSQL.
*
* Create a request for the method "migrationJobs.demoteDestination".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link DemoteDestination#execute()} method to invoke the remote
* operation. {@link DemoteDestination#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Name of the migration job resource to demote its destination.
* @param content the {@link com.google.api.services.datamigration.v1.model.DemoteDestinationRequest}
* @since 1.13
*/
protected DemoteDestination(java.lang.String name, com.google.api.services.datamigration.v1.model.DemoteDestinationRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public DemoteDestination set$Xgafv(java.lang.String $Xgafv) {
return (DemoteDestination) super.set$Xgafv($Xgafv);
}
@Override
public DemoteDestination setAccessToken(java.lang.String accessToken) {
return (DemoteDestination) super.setAccessToken(accessToken);
}
@Override
public DemoteDestination setAlt(java.lang.String alt) {
return (DemoteDestination) super.setAlt(alt);
}
@Override
public DemoteDestination setCallback(java.lang.String callback) {
return (DemoteDestination) super.setCallback(callback);
}
@Override
public DemoteDestination setFields(java.lang.String fields) {
return (DemoteDestination) super.setFields(fields);
}
@Override
public DemoteDestination setKey(java.lang.String key) {
return (DemoteDestination) super.setKey(key);
}
@Override
public DemoteDestination setOauthToken(java.lang.String oauthToken) {
return (DemoteDestination) super.setOauthToken(oauthToken);
}
@Override
public DemoteDestination setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DemoteDestination) super.setPrettyPrint(prettyPrint);
}
@Override
public DemoteDestination setQuotaUser(java.lang.String quotaUser) {
return (DemoteDestination) super.setQuotaUser(quotaUser);
}
@Override
public DemoteDestination setUploadType(java.lang.String uploadType) {
return (DemoteDestination) super.setUploadType(uploadType);
}
@Override
public DemoteDestination setUploadProtocol(java.lang.String uploadProtocol) {
return (DemoteDestination) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to demote its destination. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the migration job resource to demote its destination.
*/
public java.lang.String getName() {
return name;
}
/** Name of the migration job resource to demote its destination. */
public DemoteDestination setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public DemoteDestination set(String parameterName, Object value) {
return (DemoteDestination) super.set(parameterName, value);
}
}
/**
* Generate a SSH configuration script to configure the reverse SSH connectivity.
*
* Create a request for the method "migrationJobs.generateSshScript".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link GenerateSshScript#execute()} method to invoke the remote operation.
*
* @param migrationJob Name of the migration job resource to generate the SSH script.
* @param content the {@link com.google.api.services.datamigration.v1.model.GenerateSshScriptRequest}
* @return the request
*/
public GenerateSshScript generateSshScript(java.lang.String migrationJob, com.google.api.services.datamigration.v1.model.GenerateSshScriptRequest content) throws java.io.IOException {
GenerateSshScript result = new GenerateSshScript(migrationJob, content);
initialize(result);
return result;
}
public class GenerateSshScript extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+migrationJob}:generateSshScript";
private final java.util.regex.Pattern MIGRATION_JOB_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Generate a SSH configuration script to configure the reverse SSH connectivity.
*
* Create a request for the method "migrationJobs.generateSshScript".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link GenerateSshScript#execute()} method to invoke the remote
* operation. {@link GenerateSshScript#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param migrationJob Name of the migration job resource to generate the SSH script.
* @param content the {@link com.google.api.services.datamigration.v1.model.GenerateSshScriptRequest}
* @since 1.13
*/
protected GenerateSshScript(java.lang.String migrationJob, com.google.api.services.datamigration.v1.model.GenerateSshScriptRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.SshScript.class);
this.migrationJob = com.google.api.client.util.Preconditions.checkNotNull(migrationJob, "Required parameter migrationJob must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(MIGRATION_JOB_PATTERN.matcher(migrationJob).matches(),
"Parameter migrationJob must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public GenerateSshScript set$Xgafv(java.lang.String $Xgafv) {
return (GenerateSshScript) super.set$Xgafv($Xgafv);
}
@Override
public GenerateSshScript setAccessToken(java.lang.String accessToken) {
return (GenerateSshScript) super.setAccessToken(accessToken);
}
@Override
public GenerateSshScript setAlt(java.lang.String alt) {
return (GenerateSshScript) super.setAlt(alt);
}
@Override
public GenerateSshScript setCallback(java.lang.String callback) {
return (GenerateSshScript) super.setCallback(callback);
}
@Override
public GenerateSshScript setFields(java.lang.String fields) {
return (GenerateSshScript) super.setFields(fields);
}
@Override
public GenerateSshScript setKey(java.lang.String key) {
return (GenerateSshScript) super.setKey(key);
}
@Override
public GenerateSshScript setOauthToken(java.lang.String oauthToken) {
return (GenerateSshScript) super.setOauthToken(oauthToken);
}
@Override
public GenerateSshScript setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateSshScript) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateSshScript setQuotaUser(java.lang.String quotaUser) {
return (GenerateSshScript) super.setQuotaUser(quotaUser);
}
@Override
public GenerateSshScript setUploadType(java.lang.String uploadType) {
return (GenerateSshScript) super.setUploadType(uploadType);
}
@Override
public GenerateSshScript setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateSshScript) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to generate the SSH script. */
@com.google.api.client.util.Key
private java.lang.String migrationJob;
/** Name of the migration job resource to generate the SSH script.
*/
public java.lang.String getMigrationJob() {
return migrationJob;
}
/** Name of the migration job resource to generate the SSH script. */
public GenerateSshScript setMigrationJob(java.lang.String migrationJob) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(MIGRATION_JOB_PATTERN.matcher(migrationJob).matches(),
"Parameter migrationJob must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.migrationJob = migrationJob;
return this;
}
@Override
public GenerateSshScript set(String parameterName, Object value) {
return (GenerateSshScript) super.set(parameterName, value);
}
}
/**
* Generate a TCP Proxy configuration script to configure a cloud-hosted VM running a TCP Proxy.
*
* Create a request for the method "migrationJobs.generateTcpProxyScript".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link GenerateTcpProxyScript#execute()} method to invoke the remote
* operation.
*
* @param migrationJob Name of the migration job resource to generate the TCP Proxy script.
* @param content the {@link com.google.api.services.datamigration.v1.model.GenerateTcpProxyScriptRequest}
* @return the request
*/
public GenerateTcpProxyScript generateTcpProxyScript(java.lang.String migrationJob, com.google.api.services.datamigration.v1.model.GenerateTcpProxyScriptRequest content) throws java.io.IOException {
GenerateTcpProxyScript result = new GenerateTcpProxyScript(migrationJob, content);
initialize(result);
return result;
}
public class GenerateTcpProxyScript extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+migrationJob}:generateTcpProxyScript";
private final java.util.regex.Pattern MIGRATION_JOB_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Generate a TCP Proxy configuration script to configure a cloud-hosted VM running a TCP Proxy.
*
* Create a request for the method "migrationJobs.generateTcpProxyScript".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link GenerateTcpProxyScript#execute()} method to invoke the
* remote operation. {@link GenerateTcpProxyScript#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param migrationJob Name of the migration job resource to generate the TCP Proxy script.
* @param content the {@link com.google.api.services.datamigration.v1.model.GenerateTcpProxyScriptRequest}
* @since 1.13
*/
protected GenerateTcpProxyScript(java.lang.String migrationJob, com.google.api.services.datamigration.v1.model.GenerateTcpProxyScriptRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.TcpProxyScript.class);
this.migrationJob = com.google.api.client.util.Preconditions.checkNotNull(migrationJob, "Required parameter migrationJob must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(MIGRATION_JOB_PATTERN.matcher(migrationJob).matches(),
"Parameter migrationJob must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public GenerateTcpProxyScript set$Xgafv(java.lang.String $Xgafv) {
return (GenerateTcpProxyScript) super.set$Xgafv($Xgafv);
}
@Override
public GenerateTcpProxyScript setAccessToken(java.lang.String accessToken) {
return (GenerateTcpProxyScript) super.setAccessToken(accessToken);
}
@Override
public GenerateTcpProxyScript setAlt(java.lang.String alt) {
return (GenerateTcpProxyScript) super.setAlt(alt);
}
@Override
public GenerateTcpProxyScript setCallback(java.lang.String callback) {
return (GenerateTcpProxyScript) super.setCallback(callback);
}
@Override
public GenerateTcpProxyScript setFields(java.lang.String fields) {
return (GenerateTcpProxyScript) super.setFields(fields);
}
@Override
public GenerateTcpProxyScript setKey(java.lang.String key) {
return (GenerateTcpProxyScript) super.setKey(key);
}
@Override
public GenerateTcpProxyScript setOauthToken(java.lang.String oauthToken) {
return (GenerateTcpProxyScript) super.setOauthToken(oauthToken);
}
@Override
public GenerateTcpProxyScript setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateTcpProxyScript) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateTcpProxyScript setQuotaUser(java.lang.String quotaUser) {
return (GenerateTcpProxyScript) super.setQuotaUser(quotaUser);
}
@Override
public GenerateTcpProxyScript setUploadType(java.lang.String uploadType) {
return (GenerateTcpProxyScript) super.setUploadType(uploadType);
}
@Override
public GenerateTcpProxyScript setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateTcpProxyScript) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to generate the TCP Proxy script. */
@com.google.api.client.util.Key
private java.lang.String migrationJob;
/** Name of the migration job resource to generate the TCP Proxy script.
*/
public java.lang.String getMigrationJob() {
return migrationJob;
}
/** Name of the migration job resource to generate the TCP Proxy script. */
public GenerateTcpProxyScript setMigrationJob(java.lang.String migrationJob) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(MIGRATION_JOB_PATTERN.matcher(migrationJob).matches(),
"Parameter migrationJob must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.migrationJob = migrationJob;
return this;
}
@Override
public GenerateTcpProxyScript set(String parameterName, Object value) {
return (GenerateTcpProxyScript) super.set(parameterName, value);
}
}
/**
* Gets details of a single migration job.
*
* Create a request for the method "migrationJobs.get".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the migration job resource to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Gets details of a single migration job.
*
* Create a request for the method "migrationJobs.get".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the migration job resource to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.MigrationJob.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the migration job resource to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the migration job resource to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the migration job resource to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "migrationJobs.getIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "migrationJobs.getIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists migration jobs in a given project and location.
*
* Create a request for the method "migrationJobs.list".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent which owns this collection of migrationJobs.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/migrationJobs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists migration jobs in a given project and location.
*
* Create a request for the method "migrationJobs.list".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent which owns this collection of migrationJobs.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ListMigrationJobsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent which owns this collection of migrationJobs. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent which owns this collection of migrationJobs.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent which owns this collection of migrationJobs. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that filters migration jobs listed in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be either =, !=, >, or <. For example, list migration jobs created this
* year by specifying **createTime %gt; 2020-01-01T00:00:00.000000000Z.** You can also
* filter nested fields. For example, you could specify **reverseSshConnectivity.vmIp =
* "1.2.3.4"** to select all migration jobs connecting through the specific SSH tunnel
* bastion.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters migration jobs listed in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be either =, !=, >, or <.
For example, list migration jobs created this year by specifying **createTime %gt;
2020-01-01T00:00:00.000000000Z.** You can also filter nested fields. For example, you could specify
**reverseSshConnectivity.vmIp = "1.2.3.4"** to select all migration jobs connecting through the
specific SSH tunnel bastion.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters migration jobs listed in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be either =, !=, >, or <. For example, list migration jobs created this
* year by specifying **createTime %gt; 2020-01-01T00:00:00.000000000Z.** You can also
* filter nested fields. For example, you could specify **reverseSshConnectivity.vmIp =
* "1.2.3.4"** to select all migration jobs connecting through the specific SSH tunnel
* bastion.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sort the results based on the migration job name. Valid values are: "name", "name asc",
* and "name desc".
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sort the results based on the migration job name. Valid values are: "name", "name asc", and "name
desc".
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sort the results based on the migration job name. Valid values are: "name", "name asc",
* and "name desc".
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of migration jobs to return. The service may return fewer than this
* value. If unspecified, at most 50 migration jobs will be returned. The maximum value is
* 1000; values above 1000 are coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of migration jobs to return. The service may return fewer than this value. If
unspecified, at most 50 migration jobs will be returned. The maximum value is 1000; values above
1000 are coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of migration jobs to return. The service may return fewer than this
* value. If unspecified, at most 50 migration jobs will be returned. The maximum value is
* 1000; values above 1000 are coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The nextPageToken value received in the previous call to migrationJobs.list, used in
* the subsequent request to retrieve the next page of results. On first call this should
* be left blank. When paginating, all other parameters provided to migrationJobs.list
* must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The nextPageToken value received in the previous call to migrationJobs.list, used in the subsequent
request to retrieve the next page of results. On first call this should be left blank. When
paginating, all other parameters provided to migrationJobs.list must match the call that provided
the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The nextPageToken value received in the previous call to migrationJobs.list, used in
* the subsequent request to retrieve the next page of results. On first call this should
* be left blank. When paginating, all other parameters provided to migrationJobs.list
* must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the parameters of a single migration job.
*
* Create a request for the method "migrationJobs.patch".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name (URI) of this migration job resource, in the form of:
* projects/{project}/locations/{location}/migrationJobs/{migrationJob}.
* @param content the {@link com.google.api.services.datamigration.v1.model.MigrationJob}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.datamigration.v1.model.MigrationJob content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Updates the parameters of a single migration job.
*
* Create a request for the method "migrationJobs.patch".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name (URI) of this migration job resource, in the form of:
* projects/{project}/locations/{location}/migrationJobs/{migrationJob}.
* @param content the {@link com.google.api.services.datamigration.v1.model.MigrationJob}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.datamigration.v1.model.MigrationJob content) {
super(DatabaseMigrationService.this, "PATCH", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The name (URI) of this migration job resource, in the form of:
* projects/{project}/locations/{location}/migrationJobs/{migrationJob}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name (URI) of this migration job resource, in the form of:
projects/{project}/locations/{location}/migrationJobs/{migrationJob}.
*/
public java.lang.String getName() {
return name;
}
/**
* The name (URI) of this migration job resource, in the form of:
* projects/{project}/locations/{location}/migrationJobs/{migrationJob}.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique ID used to identify the request. If the server receives two requests with the same ID,
then the second request is ignored. It is recommended to always set this value to a UUID. The ID
must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum
length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique ID used to identify the request. If the server receives two requests with the
* same ID, then the second request is ignored. It is recommended to always set this value
* to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_),
* and hyphens (-). The maximum length is 40 characters.
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten by the update in
* the conversion workspace resource.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten by the update in the
conversion workspace resource.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten by the update in
* the conversion workspace resource.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Promote a migration job, stopping replication to the destination and promoting the destination to
* be a standalone database.
*
* Create a request for the method "migrationJobs.promote".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Promote#execute()} method to invoke the remote operation.
*
* @param name Name of the migration job resource to promote.
* @param content the {@link com.google.api.services.datamigration.v1.model.PromoteMigrationJobRequest}
* @return the request
*/
public Promote promote(java.lang.String name, com.google.api.services.datamigration.v1.model.PromoteMigrationJobRequest content) throws java.io.IOException {
Promote result = new Promote(name, content);
initialize(result);
return result;
}
public class Promote extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:promote";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Promote a migration job, stopping replication to the destination and promoting the destination
* to be a standalone database.
*
* Create a request for the method "migrationJobs.promote".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Promote#execute()} method to invoke the remote operation.
* {@link
* Promote#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the migration job resource to promote.
* @param content the {@link com.google.api.services.datamigration.v1.model.PromoteMigrationJobRequest}
* @since 1.13
*/
protected Promote(java.lang.String name, com.google.api.services.datamigration.v1.model.PromoteMigrationJobRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Promote set$Xgafv(java.lang.String $Xgafv) {
return (Promote) super.set$Xgafv($Xgafv);
}
@Override
public Promote setAccessToken(java.lang.String accessToken) {
return (Promote) super.setAccessToken(accessToken);
}
@Override
public Promote setAlt(java.lang.String alt) {
return (Promote) super.setAlt(alt);
}
@Override
public Promote setCallback(java.lang.String callback) {
return (Promote) super.setCallback(callback);
}
@Override
public Promote setFields(java.lang.String fields) {
return (Promote) super.setFields(fields);
}
@Override
public Promote setKey(java.lang.String key) {
return (Promote) super.setKey(key);
}
@Override
public Promote setOauthToken(java.lang.String oauthToken) {
return (Promote) super.setOauthToken(oauthToken);
}
@Override
public Promote setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Promote) super.setPrettyPrint(prettyPrint);
}
@Override
public Promote setQuotaUser(java.lang.String quotaUser) {
return (Promote) super.setQuotaUser(quotaUser);
}
@Override
public Promote setUploadType(java.lang.String uploadType) {
return (Promote) super.setUploadType(uploadType);
}
@Override
public Promote setUploadProtocol(java.lang.String uploadProtocol) {
return (Promote) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to promote. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the migration job resource to promote.
*/
public java.lang.String getName() {
return name;
}
/** Name of the migration job resource to promote. */
public Promote setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Promote set(String parameterName, Object value) {
return (Promote) super.set(parameterName, value);
}
}
/**
* Restart a stopped or failed migration job, resetting the destination instance to its original
* state and starting the migration process from scratch.
*
* Create a request for the method "migrationJobs.restart".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Restart#execute()} method to invoke the remote operation.
*
* @param name Name of the migration job resource to restart.
* @param content the {@link com.google.api.services.datamigration.v1.model.RestartMigrationJobRequest}
* @return the request
*/
public Restart restart(java.lang.String name, com.google.api.services.datamigration.v1.model.RestartMigrationJobRequest content) throws java.io.IOException {
Restart result = new Restart(name, content);
initialize(result);
return result;
}
public class Restart extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:restart";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Restart a stopped or failed migration job, resetting the destination instance to its original
* state and starting the migration process from scratch.
*
* Create a request for the method "migrationJobs.restart".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Restart#execute()} method to invoke the remote operation.
* {@link
* Restart#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the migration job resource to restart.
* @param content the {@link com.google.api.services.datamigration.v1.model.RestartMigrationJobRequest}
* @since 1.13
*/
protected Restart(java.lang.String name, com.google.api.services.datamigration.v1.model.RestartMigrationJobRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Restart set$Xgafv(java.lang.String $Xgafv) {
return (Restart) super.set$Xgafv($Xgafv);
}
@Override
public Restart setAccessToken(java.lang.String accessToken) {
return (Restart) super.setAccessToken(accessToken);
}
@Override
public Restart setAlt(java.lang.String alt) {
return (Restart) super.setAlt(alt);
}
@Override
public Restart setCallback(java.lang.String callback) {
return (Restart) super.setCallback(callback);
}
@Override
public Restart setFields(java.lang.String fields) {
return (Restart) super.setFields(fields);
}
@Override
public Restart setKey(java.lang.String key) {
return (Restart) super.setKey(key);
}
@Override
public Restart setOauthToken(java.lang.String oauthToken) {
return (Restart) super.setOauthToken(oauthToken);
}
@Override
public Restart setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Restart) super.setPrettyPrint(prettyPrint);
}
@Override
public Restart setQuotaUser(java.lang.String quotaUser) {
return (Restart) super.setQuotaUser(quotaUser);
}
@Override
public Restart setUploadType(java.lang.String uploadType) {
return (Restart) super.setUploadType(uploadType);
}
@Override
public Restart setUploadProtocol(java.lang.String uploadProtocol) {
return (Restart) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to restart. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the migration job resource to restart.
*/
public java.lang.String getName() {
return name;
}
/** Name of the migration job resource to restart. */
public Restart setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Restart set(String parameterName, Object value) {
return (Restart) super.set(parameterName, value);
}
}
/**
* Resume a migration job that is currently stopped and is resumable (was stopped during CDC phase).
*
* Create a request for the method "migrationJobs.resume".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Resume#execute()} method to invoke the remote operation.
*
* @param name Name of the migration job resource to resume.
* @param content the {@link com.google.api.services.datamigration.v1.model.ResumeMigrationJobRequest}
* @return the request
*/
public Resume resume(java.lang.String name, com.google.api.services.datamigration.v1.model.ResumeMigrationJobRequest content) throws java.io.IOException {
Resume result = new Resume(name, content);
initialize(result);
return result;
}
public class Resume extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:resume";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Resume a migration job that is currently stopped and is resumable (was stopped during CDC
* phase).
*
* Create a request for the method "migrationJobs.resume".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Resume#execute()} method to invoke the remote operation.
* {@link
* Resume#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the migration job resource to resume.
* @param content the {@link com.google.api.services.datamigration.v1.model.ResumeMigrationJobRequest}
* @since 1.13
*/
protected Resume(java.lang.String name, com.google.api.services.datamigration.v1.model.ResumeMigrationJobRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Resume set$Xgafv(java.lang.String $Xgafv) {
return (Resume) super.set$Xgafv($Xgafv);
}
@Override
public Resume setAccessToken(java.lang.String accessToken) {
return (Resume) super.setAccessToken(accessToken);
}
@Override
public Resume setAlt(java.lang.String alt) {
return (Resume) super.setAlt(alt);
}
@Override
public Resume setCallback(java.lang.String callback) {
return (Resume) super.setCallback(callback);
}
@Override
public Resume setFields(java.lang.String fields) {
return (Resume) super.setFields(fields);
}
@Override
public Resume setKey(java.lang.String key) {
return (Resume) super.setKey(key);
}
@Override
public Resume setOauthToken(java.lang.String oauthToken) {
return (Resume) super.setOauthToken(oauthToken);
}
@Override
public Resume setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Resume) super.setPrettyPrint(prettyPrint);
}
@Override
public Resume setQuotaUser(java.lang.String quotaUser) {
return (Resume) super.setQuotaUser(quotaUser);
}
@Override
public Resume setUploadType(java.lang.String uploadType) {
return (Resume) super.setUploadType(uploadType);
}
@Override
public Resume setUploadProtocol(java.lang.String uploadProtocol) {
return (Resume) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to resume. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the migration job resource to resume.
*/
public java.lang.String getName() {
return name;
}
/** Name of the migration job resource to resume. */
public Resume setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Resume set(String parameterName, Object value) {
return (Resume) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "migrationJobs.setIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "migrationJobs.setIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Start an already created migration job.
*
* Create a request for the method "migrationJobs.start".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Start#execute()} method to invoke the remote operation.
*
* @param name Name of the migration job resource to start.
* @param content the {@link com.google.api.services.datamigration.v1.model.StartMigrationJobRequest}
* @return the request
*/
public Start start(java.lang.String name, com.google.api.services.datamigration.v1.model.StartMigrationJobRequest content) throws java.io.IOException {
Start result = new Start(name, content);
initialize(result);
return result;
}
public class Start extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:start";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Start an already created migration job.
*
* Create a request for the method "migrationJobs.start".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Start#execute()} method to invoke the remote operation.
* {@link
* Start#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the migration job resource to start.
* @param content the {@link com.google.api.services.datamigration.v1.model.StartMigrationJobRequest}
* @since 1.13
*/
protected Start(java.lang.String name, com.google.api.services.datamigration.v1.model.StartMigrationJobRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Start set$Xgafv(java.lang.String $Xgafv) {
return (Start) super.set$Xgafv($Xgafv);
}
@Override
public Start setAccessToken(java.lang.String accessToken) {
return (Start) super.setAccessToken(accessToken);
}
@Override
public Start setAlt(java.lang.String alt) {
return (Start) super.setAlt(alt);
}
@Override
public Start setCallback(java.lang.String callback) {
return (Start) super.setCallback(callback);
}
@Override
public Start setFields(java.lang.String fields) {
return (Start) super.setFields(fields);
}
@Override
public Start setKey(java.lang.String key) {
return (Start) super.setKey(key);
}
@Override
public Start setOauthToken(java.lang.String oauthToken) {
return (Start) super.setOauthToken(oauthToken);
}
@Override
public Start setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Start) super.setPrettyPrint(prettyPrint);
}
@Override
public Start setQuotaUser(java.lang.String quotaUser) {
return (Start) super.setQuotaUser(quotaUser);
}
@Override
public Start setUploadType(java.lang.String uploadType) {
return (Start) super.setUploadType(uploadType);
}
@Override
public Start setUploadProtocol(java.lang.String uploadProtocol) {
return (Start) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to start. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the migration job resource to start.
*/
public java.lang.String getName() {
return name;
}
/** Name of the migration job resource to start. */
public Start setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Start set(String parameterName, Object value) {
return (Start) super.set(parameterName, value);
}
}
/**
* Stops a running migration job.
*
* Create a request for the method "migrationJobs.stop".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Stop#execute()} method to invoke the remote operation.
*
* @param name Name of the migration job resource to stop.
* @param content the {@link com.google.api.services.datamigration.v1.model.StopMigrationJobRequest}
* @return the request
*/
public Stop stop(java.lang.String name, com.google.api.services.datamigration.v1.model.StopMigrationJobRequest content) throws java.io.IOException {
Stop result = new Stop(name, content);
initialize(result);
return result;
}
public class Stop extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:stop";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Stops a running migration job.
*
* Create a request for the method "migrationJobs.stop".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Stop#execute()} method to invoke the remote operation.
* {@link Stop#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the migration job resource to stop.
* @param content the {@link com.google.api.services.datamigration.v1.model.StopMigrationJobRequest}
* @since 1.13
*/
protected Stop(java.lang.String name, com.google.api.services.datamigration.v1.model.StopMigrationJobRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Stop set$Xgafv(java.lang.String $Xgafv) {
return (Stop) super.set$Xgafv($Xgafv);
}
@Override
public Stop setAccessToken(java.lang.String accessToken) {
return (Stop) super.setAccessToken(accessToken);
}
@Override
public Stop setAlt(java.lang.String alt) {
return (Stop) super.setAlt(alt);
}
@Override
public Stop setCallback(java.lang.String callback) {
return (Stop) super.setCallback(callback);
}
@Override
public Stop setFields(java.lang.String fields) {
return (Stop) super.setFields(fields);
}
@Override
public Stop setKey(java.lang.String key) {
return (Stop) super.setKey(key);
}
@Override
public Stop setOauthToken(java.lang.String oauthToken) {
return (Stop) super.setOauthToken(oauthToken);
}
@Override
public Stop setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Stop) super.setPrettyPrint(prettyPrint);
}
@Override
public Stop setQuotaUser(java.lang.String quotaUser) {
return (Stop) super.setQuotaUser(quotaUser);
}
@Override
public Stop setUploadType(java.lang.String uploadType) {
return (Stop) super.setUploadType(uploadType);
}
@Override
public Stop setUploadProtocol(java.lang.String uploadProtocol) {
return (Stop) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to stop. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the migration job resource to stop.
*/
public java.lang.String getName() {
return name;
}
/** Name of the migration job resource to stop. */
public Stop setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Stop set(String parameterName, Object value) {
return (Stop) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "migrationJobs.testIamPermissions".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "migrationJobs.testIamPermissions".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Verify a migration job, making sure the destination can reach the source and that all
* configuration and prerequisites are met.
*
* Create a request for the method "migrationJobs.verify".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Verify#execute()} method to invoke the remote operation.
*
* @param name Name of the migration job resource to verify.
* @param content the {@link com.google.api.services.datamigration.v1.model.VerifyMigrationJobRequest}
* @return the request
*/
public Verify verify(java.lang.String name, com.google.api.services.datamigration.v1.model.VerifyMigrationJobRequest content) throws java.io.IOException {
Verify result = new Verify(name, content);
initialize(result);
return result;
}
public class Verify extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:verify";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
/**
* Verify a migration job, making sure the destination can reach the source and that all
* configuration and prerequisites are met.
*
* Create a request for the method "migrationJobs.verify".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Verify#execute()} method to invoke the remote operation.
* {@link
* Verify#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the migration job resource to verify.
* @param content the {@link com.google.api.services.datamigration.v1.model.VerifyMigrationJobRequest}
* @since 1.13
*/
protected Verify(java.lang.String name, com.google.api.services.datamigration.v1.model.VerifyMigrationJobRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
}
@Override
public Verify set$Xgafv(java.lang.String $Xgafv) {
return (Verify) super.set$Xgafv($Xgafv);
}
@Override
public Verify setAccessToken(java.lang.String accessToken) {
return (Verify) super.setAccessToken(accessToken);
}
@Override
public Verify setAlt(java.lang.String alt) {
return (Verify) super.setAlt(alt);
}
@Override
public Verify setCallback(java.lang.String callback) {
return (Verify) super.setCallback(callback);
}
@Override
public Verify setFields(java.lang.String fields) {
return (Verify) super.setFields(fields);
}
@Override
public Verify setKey(java.lang.String key) {
return (Verify) super.setKey(key);
}
@Override
public Verify setOauthToken(java.lang.String oauthToken) {
return (Verify) super.setOauthToken(oauthToken);
}
@Override
public Verify setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Verify) super.setPrettyPrint(prettyPrint);
}
@Override
public Verify setQuotaUser(java.lang.String quotaUser) {
return (Verify) super.setQuotaUser(quotaUser);
}
@Override
public Verify setUploadType(java.lang.String uploadType) {
return (Verify) super.setUploadType(uploadType);
}
@Override
public Verify setUploadProtocol(java.lang.String uploadProtocol) {
return (Verify) super.setUploadProtocol(uploadProtocol);
}
/** Name of the migration job resource to verify. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the migration job resource to verify.
*/
public java.lang.String getName() {
return name;
}
/** Name of the migration job resource to verify. */
public Verify setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Verify set(String parameterName, Object value) {
return (Verify) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Objects collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.Objects.List request = datamigration.objects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Objects objects() {
return new Objects();
}
/**
* The "objects" collection of methods.
*/
public class Objects {
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "objects.getIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "objects.getIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "objects.setIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "objects.setIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "objects.testIamPermissions".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "objects.testIamPermissions".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/migrationJobs/[^/]+/objects/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.Operations.List request = datamigration.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.datamigration.v1.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.datamigration.v1.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.datamigration.v1.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.datamigration.v1.model.CancelOperationRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DatabaseMigrationService.this, "DELETE", REST_PATH, null, com.google.api.services.datamigration.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the PrivateConnections collection.
*
* The typical use is:
*
* {@code DatabaseMigrationService datamigration = new DatabaseMigrationService(...);}
* {@code DatabaseMigrationService.PrivateConnections.List request = datamigration.privateConnections().list(parameters ...)}
*
*
* @return the resource collection
*/
public PrivateConnections privateConnections() {
return new PrivateConnections();
}
/**
* The "privateConnections" collection of methods.
*/
public class PrivateConnections {
/**
* Creates a new private connection in a given project and location.
*
* Create a request for the method "privateConnections.create".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent that owns the collection of PrivateConnections.
* @param content the {@link com.google.api.services.datamigration.v1.model.PrivateConnection}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.datamigration.v1.model.PrivateConnection content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/privateConnections";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new private connection in a given project and location.
*
* Create a request for the method "privateConnections.create".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent that owns the collection of PrivateConnections.
* @param content the {@link com.google.api.services.datamigration.v1.model.PrivateConnection}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.datamigration.v1.model.PrivateConnection content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent that owns the collection of PrivateConnections. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent that owns the collection of PrivateConnections.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent that owns the collection of PrivateConnections. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Required. The private connection identifier. */
@com.google.api.client.util.Key
private java.lang.String privateConnectionId;
/** Required. The private connection identifier.
*/
public java.lang.String getPrivateConnectionId() {
return privateConnectionId;
}
/** Required. The private connection identifier. */
public Create setPrivateConnectionId(java.lang.String privateConnectionId) {
this.privateConnectionId = privateConnectionId;
return this;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique ID used to identify the request. If the server receives two requests with the
same ID, then the second request is ignored. It is recommended to always set this value to a UUID.
The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/** Optional. If set to true, will skip validations. */
@com.google.api.client.util.Key
private java.lang.Boolean skipValidation;
/** Optional. If set to true, will skip validations.
*/
public java.lang.Boolean getSkipValidation() {
return skipValidation;
}
/** Optional. If set to true, will skip validations. */
public Create setSkipValidation(java.lang.Boolean skipValidation) {
this.skipValidation = skipValidation;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single Database Migration Service private connection.
*
* Create a request for the method "privateConnections.delete".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the private connection to delete.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Deletes a single Database Migration Service private connection.
*
* Create a request for the method "privateConnections.delete".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the private connection to delete.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(DatabaseMigrationService.this, "DELETE", REST_PATH, null, com.google.api.services.datamigration.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the private connection to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the private connection to delete.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the private connection to delete. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique ID used to identify the request. If the server receives two requests with the
same ID, then the second request is ignored. It is recommended to always set this value to a UUID.
The ID must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique ID used to identify the request. If the server receives two requests
* with the same ID, then the second request is ignored. It is recommended to always set
* this value to a UUID. The ID must contain only letters (a-z, A-Z), numbers (0-9),
* underscores (_), and hyphens (-). The maximum length is 40 characters.
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single private connection.
*
* Create a request for the method "privateConnections.get".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the private connection to get.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Gets details of a single private connection.
*
* Create a request for the method "privateConnections.get".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the private connection to get.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.PrivateConnection.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the private connection to get. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the private connection to get.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the private connection to get. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "privateConnections.getIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "privateConnections.getIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Retrieves a list of private connections in a given project and location.
*
* Create a request for the method "privateConnections.list".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent that owns the collection of private connections.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+parent}/privateConnections";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Retrieves a list of private connections in a given project and location.
*
* Create a request for the method "privateConnections.list".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent that owns the collection of private connections.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(DatabaseMigrationService.this, "GET", REST_PATH, null, com.google.api.services.datamigration.v1.model.ListPrivateConnectionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The parent that owns the collection of private connections. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent that owns the collection of private connections.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The parent that owns the collection of private connections. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that filters private connections listed in the response. The
* expression must specify the field name, a comparison operator, and the value that you
* want to use for filtering. The value must be a string, a number, or a boolean. The
* comparison operator must be either =, !=, >, or <. For example, list private
* connections created this year by specifying **createTime %gt;
* 2021-01-01T00:00:00.000000000Z**.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters private connections listed in the response. The expression must
specify the field name, a comparison operator, and the value that you want to use for filtering.
The value must be a string, a number, or a boolean. The comparison operator must be either =, !=,
>, or <. For example, list private connections created this year by specifying **createTime %gt;
2021-01-01T00:00:00.000000000Z**.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters private connections listed in the response. The
* expression must specify the field name, a comparison operator, and the value that you
* want to use for filtering. The value must be a string, a number, or a boolean. The
* comparison operator must be either =, !=, >, or <. For example, list private
* connections created this year by specifying **createTime %gt;
* 2021-01-01T00:00:00.000000000Z**.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Order by fields for the result. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Order by fields for the result.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Order by fields for the result. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Maximum number of private connections to return. If unspecified, at most 50 private
* connections that are returned. The maximum value is 1000; values above 1000 are coerced
* to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum number of private connections to return. If unspecified, at most 50 private connections
that are returned. The maximum value is 1000; values above 1000 are coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Maximum number of private connections to return. If unspecified, at most 50 private
* connections that are returned. The maximum value is 1000; values above 1000 are coerced
* to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Page token received from a previous `ListPrivateConnections` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPrivateConnections` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Page token received from a previous `ListPrivateConnections` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListPrivateConnections` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Page token received from a previous `ListPrivateConnections` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPrivateConnections` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "privateConnections.setIamPolicy".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "privateConnections.setIamPolicy".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.datamigration.v1.model.SetIamPolicyRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "privateConnections.testIamPermissions".
*
* This request holds the parameters needed by the datamigration server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DatabaseMigrationServiceRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "privateConnections.testIamPermissions".
*
* This request holds the parameters needed by the the datamigration server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.datamigration.v1.model.TestIamPermissionsRequest content) {
super(DatabaseMigrationService.this, "POST", REST_PATH, content, com.google.api.services.datamigration.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link DatabaseMigrationService}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link DatabaseMigrationService}. */
@Override
public DatabaseMigrationService build() {
return new DatabaseMigrationService(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link DatabaseMigrationServiceRequestInitializer}.
*
* @since 1.12
*/
public Builder setDatabaseMigrationServiceRequestInitializer(
DatabaseMigrationServiceRequestInitializer databasemigrationserviceRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(databasemigrationserviceRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}