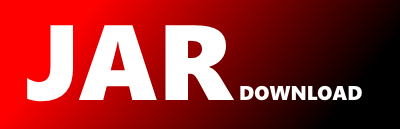
com.google.api.services.dataplex.v1.model.GoogleCloudDataplexV1AssetDiscoverySpec Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataplex.v1.model;
/**
* Settings to manage the metadata discovery and publishing for an asset.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataplex API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudDataplexV1AssetDiscoverySpec extends com.google.api.client.json.GenericJson {
/**
* Optional. Configuration for CSV data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDataplexV1AssetDiscoverySpecCsvOptions csvOptions;
/**
* Optional. Whether discovery is enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enabled;
/**
* Optional. The list of patterns to apply for selecting data to exclude during discovery. For
* Cloud Storage bucket assets, these are interpreted as glob patterns used to match object names.
* For BigQuery dataset assets, these are interpreted as patterns to match table names.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List excludePatterns;
/**
* Optional. The list of patterns to apply for selecting data to include during discovery if only
* a subset of the data should considered. For Cloud Storage bucket assets, these are interpreted
* as glob patterns used to match object names. For BigQuery dataset assets, these are interpreted
* as patterns to match table names.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List includePatterns;
/**
* Optional. Configuration for Json data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDataplexV1AssetDiscoverySpecJsonOptions jsonOptions;
/**
* Optional. Cron schedule (https://en.wikipedia.org/wiki/Cron) for running discovery
* periodically. Successive discovery runs must be scheduled at least 60 minutes apart. The
* default value is to run discovery every 60 minutes. To explicitly set a timezone to the cron
* tab, apply a prefix in the cron tab: "CRON_TZ=${IANA_TIME_ZONE}" or TZ=${IANA_TIME_ZONE}". The
* ${IANA_TIME_ZONE} may only be a valid string from IANA time zone database. For example,
* CRON_TZ=America/New_York 1 * * * *, or TZ=America/New_York 1 * * * *.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String schedule;
/**
* Optional. Configuration for CSV data.
* @return value or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpecCsvOptions getCsvOptions() {
return csvOptions;
}
/**
* Optional. Configuration for CSV data.
* @param csvOptions csvOptions or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpec setCsvOptions(GoogleCloudDataplexV1AssetDiscoverySpecCsvOptions csvOptions) {
this.csvOptions = csvOptions;
return this;
}
/**
* Optional. Whether discovery is enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnabled() {
return enabled;
}
/**
* Optional. Whether discovery is enabled.
* @param enabled enabled or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpec setEnabled(java.lang.Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Optional. The list of patterns to apply for selecting data to exclude during discovery. For
* Cloud Storage bucket assets, these are interpreted as glob patterns used to match object names.
* For BigQuery dataset assets, these are interpreted as patterns to match table names.
* @return value or {@code null} for none
*/
public java.util.List getExcludePatterns() {
return excludePatterns;
}
/**
* Optional. The list of patterns to apply for selecting data to exclude during discovery. For
* Cloud Storage bucket assets, these are interpreted as glob patterns used to match object names.
* For BigQuery dataset assets, these are interpreted as patterns to match table names.
* @param excludePatterns excludePatterns or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpec setExcludePatterns(java.util.List excludePatterns) {
this.excludePatterns = excludePatterns;
return this;
}
/**
* Optional. The list of patterns to apply for selecting data to include during discovery if only
* a subset of the data should considered. For Cloud Storage bucket assets, these are interpreted
* as glob patterns used to match object names. For BigQuery dataset assets, these are interpreted
* as patterns to match table names.
* @return value or {@code null} for none
*/
public java.util.List getIncludePatterns() {
return includePatterns;
}
/**
* Optional. The list of patterns to apply for selecting data to include during discovery if only
* a subset of the data should considered. For Cloud Storage bucket assets, these are interpreted
* as glob patterns used to match object names. For BigQuery dataset assets, these are interpreted
* as patterns to match table names.
* @param includePatterns includePatterns or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpec setIncludePatterns(java.util.List includePatterns) {
this.includePatterns = includePatterns;
return this;
}
/**
* Optional. Configuration for Json data.
* @return value or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpecJsonOptions getJsonOptions() {
return jsonOptions;
}
/**
* Optional. Configuration for Json data.
* @param jsonOptions jsonOptions or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpec setJsonOptions(GoogleCloudDataplexV1AssetDiscoverySpecJsonOptions jsonOptions) {
this.jsonOptions = jsonOptions;
return this;
}
/**
* Optional. Cron schedule (https://en.wikipedia.org/wiki/Cron) for running discovery
* periodically. Successive discovery runs must be scheduled at least 60 minutes apart. The
* default value is to run discovery every 60 minutes. To explicitly set a timezone to the cron
* tab, apply a prefix in the cron tab: "CRON_TZ=${IANA_TIME_ZONE}" or TZ=${IANA_TIME_ZONE}". The
* ${IANA_TIME_ZONE} may only be a valid string from IANA time zone database. For example,
* CRON_TZ=America/New_York 1 * * * *, or TZ=America/New_York 1 * * * *.
* @return value or {@code null} for none
*/
public java.lang.String getSchedule() {
return schedule;
}
/**
* Optional. Cron schedule (https://en.wikipedia.org/wiki/Cron) for running discovery
* periodically. Successive discovery runs must be scheduled at least 60 minutes apart. The
* default value is to run discovery every 60 minutes. To explicitly set a timezone to the cron
* tab, apply a prefix in the cron tab: "CRON_TZ=${IANA_TIME_ZONE}" or TZ=${IANA_TIME_ZONE}". The
* ${IANA_TIME_ZONE} may only be a valid string from IANA time zone database. For example,
* CRON_TZ=America/New_York 1 * * * *, or TZ=America/New_York 1 * * * *.
* @param schedule schedule or {@code null} for none
*/
public GoogleCloudDataplexV1AssetDiscoverySpec setSchedule(java.lang.String schedule) {
this.schedule = schedule;
return this;
}
@Override
public GoogleCloudDataplexV1AssetDiscoverySpec set(String fieldName, Object value) {
return (GoogleCloudDataplexV1AssetDiscoverySpec) super.set(fieldName, value);
}
@Override
public GoogleCloudDataplexV1AssetDiscoverySpec clone() {
return (GoogleCloudDataplexV1AssetDiscoverySpec) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy