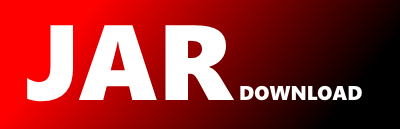
target.apidocs.com.google.api.services.dataproc.model.ExecutionConfig.html Maven / Gradle / Ivy
ExecutionConfig (Cloud Dataproc API v1-rev20240605-2.0.0)
com.google.api.services.dataproc.model
Class ExecutionConfig
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.dataproc.model.ExecutionConfig
-
public final class ExecutionConfig
extends com.google.api.client.json.GenericJson
Execution configuration for a workload.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
ExecutionConfig()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
ExecutionConfig
clone()
String
getIdleTtl()
Optional.
String
getKmsKey()
Optional.
List<String>
getNetworkTags()
Optional.
String
getNetworkUri()
Optional.
String
getServiceAccount()
Optional.
String
getStagingBucket()
Optional.
String
getSubnetworkUri()
Optional.
String
getTtl()
Optional.
ExecutionConfig
set(String fieldName,
Object value)
ExecutionConfig
setIdleTtl(String idleTtl)
Optional.
ExecutionConfig
setKmsKey(String kmsKey)
Optional.
ExecutionConfig
setNetworkTags(List<String> networkTags)
Optional.
ExecutionConfig
setNetworkUri(String networkUri)
Optional.
ExecutionConfig
setServiceAccount(String serviceAccount)
Optional.
ExecutionConfig
setStagingBucket(String stagingBucket)
Optional.
ExecutionConfig
setSubnetworkUri(String subnetworkUri)
Optional.
ExecutionConfig
setTtl(String ttl)
Optional.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getIdleTtl
public String getIdleTtl()
Optional. Applies to sessions only. The duration to keep the session alive while it's idling.
Exceeding this threshold causes the session to terminate. This field cannot be set on a batch
workload. Minimum value is 10 minutes; maximum value is 14 days (see JSON representation of
Duration (https://developers.google.com/protocol-buffers/docs/proto3#json)). Defaults to 1 hour
if not set. If both ttl and idle_ttl are specified for an interactive session, the conditions
are treated as OR conditions: the workload will be terminated when it has been idle for
idle_ttl or when ttl has been exceeded, whichever occurs first.
- Returns:
- value or
null
for none
-
setIdleTtl
public ExecutionConfig setIdleTtl(String idleTtl)
Optional. Applies to sessions only. The duration to keep the session alive while it's idling.
Exceeding this threshold causes the session to terminate. This field cannot be set on a batch
workload. Minimum value is 10 minutes; maximum value is 14 days (see JSON representation of
Duration (https://developers.google.com/protocol-buffers/docs/proto3#json)). Defaults to 1 hour
if not set. If both ttl and idle_ttl are specified for an interactive session, the conditions
are treated as OR conditions: the workload will be terminated when it has been idle for
idle_ttl or when ttl has been exceeded, whichever occurs first.
- Parameters:
idleTtl
- idleTtl or null
for none
-
getKmsKey
public String getKmsKey()
Optional. The Cloud KMS key to use for encryption.
- Returns:
- value or
null
for none
-
setKmsKey
public ExecutionConfig setKmsKey(String kmsKey)
Optional. The Cloud KMS key to use for encryption.
- Parameters:
kmsKey
- kmsKey or null
for none
-
getNetworkTags
public List<String> getNetworkTags()
Optional. Tags used for network traffic control.
- Returns:
- value or
null
for none
-
setNetworkTags
public ExecutionConfig setNetworkTags(List<String> networkTags)
Optional. Tags used for network traffic control.
- Parameters:
networkTags
- networkTags or null
for none
-
getNetworkUri
public String getNetworkUri()
Optional. Network URI to connect workload to.
- Returns:
- value or
null
for none
-
setNetworkUri
public ExecutionConfig setNetworkUri(String networkUri)
Optional. Network URI to connect workload to.
- Parameters:
networkUri
- networkUri or null
for none
-
getServiceAccount
public String getServiceAccount()
Optional. Service account that used to execute workload.
- Returns:
- value or
null
for none
-
setServiceAccount
public ExecutionConfig setServiceAccount(String serviceAccount)
Optional. Service account that used to execute workload.
- Parameters:
serviceAccount
- serviceAccount or null
for none
-
getStagingBucket
public String getStagingBucket()
Optional. A Cloud Storage bucket used to stage workload dependencies, config files, and store
workload output and other ephemeral data, such as Spark history files. If you do not specify a
staging bucket, Cloud Dataproc will determine a Cloud Storage location according to the region
where your workload is running, and then create and manage project-level, per-location staging
and temporary buckets. This field requires a Cloud Storage bucket name, not a gs://... URI to a
Cloud Storage bucket.
- Returns:
- value or
null
for none
-
setStagingBucket
public ExecutionConfig setStagingBucket(String stagingBucket)
Optional. A Cloud Storage bucket used to stage workload dependencies, config files, and store
workload output and other ephemeral data, such as Spark history files. If you do not specify a
staging bucket, Cloud Dataproc will determine a Cloud Storage location according to the region
where your workload is running, and then create and manage project-level, per-location staging
and temporary buckets. This field requires a Cloud Storage bucket name, not a gs://... URI to a
Cloud Storage bucket.
- Parameters:
stagingBucket
- stagingBucket or null
for none
-
getSubnetworkUri
public String getSubnetworkUri()
Optional. Subnetwork URI to connect workload to.
- Returns:
- value or
null
for none
-
setSubnetworkUri
public ExecutionConfig setSubnetworkUri(String subnetworkUri)
Optional. Subnetwork URI to connect workload to.
- Parameters:
subnetworkUri
- subnetworkUri or null
for none
-
getTtl
public String getTtl()
Optional. The duration after which the workload will be terminated, specified as the JSON
representation for Duration (https://protobuf.dev/programming-guides/proto3/#json). When the
workload exceeds this duration, it will be unconditionally terminated without waiting for
ongoing work to finish. If ttl is not specified for a batch workload, the workload will be
allowed to run until it exits naturally (or run forever without exiting). If ttl is not
specified for an interactive session, it defaults to 24 hours. If ttl is not specified for a
batch that uses 2.1+ runtime version, it defaults to 4 hours. Minimum value is 10 minutes;
maximum value is 14 days. If both ttl and idle_ttl are specified (for an interactive session),
the conditions are treated as OR conditions: the workload will be terminated when it has been
idle for idle_ttl or when ttl has been exceeded, whichever occurs first.
- Returns:
- value or
null
for none
-
setTtl
public ExecutionConfig setTtl(String ttl)
Optional. The duration after which the workload will be terminated, specified as the JSON
representation for Duration (https://protobuf.dev/programming-guides/proto3/#json). When the
workload exceeds this duration, it will be unconditionally terminated without waiting for
ongoing work to finish. If ttl is not specified for a batch workload, the workload will be
allowed to run until it exits naturally (or run forever without exiting). If ttl is not
specified for an interactive session, it defaults to 24 hours. If ttl is not specified for a
batch that uses 2.1+ runtime version, it defaults to 4 hours. Minimum value is 10 minutes;
maximum value is 14 days. If both ttl and idle_ttl are specified (for an interactive session),
the conditions are treated as OR conditions: the workload will be terminated when it has been
idle for idle_ttl or when ttl has been exceeded, whichever occurs first.
- Parameters:
ttl
- ttl or null
for none
-
set
public ExecutionConfig set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public ExecutionConfig clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy