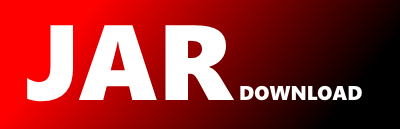
target.apidocs.com.google.api.services.dataproc.model.Job.html Maven / Gradle / Ivy
Job (Cloud Dataproc API v1-rev20240605-2.0.0)
com.google.api.services.dataproc.model
Class Job
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.dataproc.model.Job
-
public final class Job
extends com.google.api.client.json.GenericJson
A Dataproc job resource.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Job()
-
Method Summary
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getDone
public Boolean getDone()
Output only. Indicates whether the job is completed. If the value is false, the job is still in
progress. If true, the job is completed, and status.state field will indicate if it was
successful, failed, or cancelled.
- Returns:
- value or
null
for none
-
setDone
public Job setDone(Boolean done)
Output only. Indicates whether the job is completed. If the value is false, the job is still in
progress. If true, the job is completed, and status.state field will indicate if it was
successful, failed, or cancelled.
- Parameters:
done
- done or null
for none
-
getDriverControlFilesUri
public String getDriverControlFilesUri()
Output only. If present, the location of miscellaneous control files which can be used as part
of job setup and handling. If not present, control files might be placed in the same location
as driver_output_uri.
- Returns:
- value or
null
for none
-
setDriverControlFilesUri
public Job setDriverControlFilesUri(String driverControlFilesUri)
Output only. If present, the location of miscellaneous control files which can be used as part
of job setup and handling. If not present, control files might be placed in the same location
as driver_output_uri.
- Parameters:
driverControlFilesUri
- driverControlFilesUri or null
for none
-
getDriverOutputResourceUri
public String getDriverOutputResourceUri()
Output only. A URI pointing to the location of the stdout of the job's driver program.
- Returns:
- value or
null
for none
-
setDriverOutputResourceUri
public Job setDriverOutputResourceUri(String driverOutputResourceUri)
Output only. A URI pointing to the location of the stdout of the job's driver program.
- Parameters:
driverOutputResourceUri
- driverOutputResourceUri or null
for none
-
getDriverSchedulingConfig
public DriverSchedulingConfig getDriverSchedulingConfig()
Optional. Driver scheduling configuration.
- Returns:
- value or
null
for none
-
setDriverSchedulingConfig
public Job setDriverSchedulingConfig(DriverSchedulingConfig driverSchedulingConfig)
Optional. Driver scheduling configuration.
- Parameters:
driverSchedulingConfig
- driverSchedulingConfig or null
for none
-
getFlinkJob
public FlinkJob getFlinkJob()
Optional. Job is a Flink job.
- Returns:
- value or
null
for none
-
setFlinkJob
public Job setFlinkJob(FlinkJob flinkJob)
Optional. Job is a Flink job.
- Parameters:
flinkJob
- flinkJob or null
for none
-
getHadoopJob
public HadoopJob getHadoopJob()
Optional. Job is a Hadoop job.
- Returns:
- value or
null
for none
-
setHadoopJob
public Job setHadoopJob(HadoopJob hadoopJob)
Optional. Job is a Hadoop job.
- Parameters:
hadoopJob
- hadoopJob or null
for none
-
getHiveJob
public HiveJob getHiveJob()
Optional. Job is a Hive job.
- Returns:
- value or
null
for none
-
setHiveJob
public Job setHiveJob(HiveJob hiveJob)
Optional. Job is a Hive job.
- Parameters:
hiveJob
- hiveJob or null
for none
-
getJobUuid
public String getJobUuid()
Output only. A UUID that uniquely identifies a job within the project over time. This is in
contrast to a user-settable reference.job_id that might be reused over time.
- Returns:
- value or
null
for none
-
setJobUuid
public Job setJobUuid(String jobUuid)
Output only. A UUID that uniquely identifies a job within the project over time. This is in
contrast to a user-settable reference.job_id that might be reused over time.
- Parameters:
jobUuid
- jobUuid or null
for none
-
getLabels
public Map<String,String> getLabels()
Optional. The labels to associate with this job. Label keys must contain 1 to 63 characters,
and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). Label values can be empty,
but, if present, must contain 1 to 63 characters, and must conform to RFC 1035
(https://www.ietf.org/rfc/rfc1035.txt). No more than 32 labels can be associated with a job.
- Returns:
- value or
null
for none
-
setLabels
public Job setLabels(Map<String,String> labels)
Optional. The labels to associate with this job. Label keys must contain 1 to 63 characters,
and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). Label values can be empty,
but, if present, must contain 1 to 63 characters, and must conform to RFC 1035
(https://www.ietf.org/rfc/rfc1035.txt). No more than 32 labels can be associated with a job.
- Parameters:
labels
- labels or null
for none
-
getPigJob
public PigJob getPigJob()
Optional. Job is a Pig job.
- Returns:
- value or
null
for none
-
setPigJob
public Job setPigJob(PigJob pigJob)
Optional. Job is a Pig job.
- Parameters:
pigJob
- pigJob or null
for none
-
getPlacement
public JobPlacement getPlacement()
Required. Job information, including how, when, and where to run the job.
- Returns:
- value or
null
for none
-
setPlacement
public Job setPlacement(JobPlacement placement)
Required. Job information, including how, when, and where to run the job.
- Parameters:
placement
- placement or null
for none
-
getPrestoJob
public PrestoJob getPrestoJob()
Optional. Job is a Presto job.
- Returns:
- value or
null
for none
-
setPrestoJob
public Job setPrestoJob(PrestoJob prestoJob)
Optional. Job is a Presto job.
- Parameters:
prestoJob
- prestoJob or null
for none
-
getPysparkJob
public PySparkJob getPysparkJob()
Optional. Job is a PySpark job.
- Returns:
- value or
null
for none
-
setPysparkJob
public Job setPysparkJob(PySparkJob pysparkJob)
Optional. Job is a PySpark job.
- Parameters:
pysparkJob
- pysparkJob or null
for none
-
getReference
public JobReference getReference()
Optional. The fully qualified reference to the job, which can be used to obtain the equivalent
REST path of the job resource. If this property is not specified when a job is created, the
server generates a job_id.
- Returns:
- value or
null
for none
-
setReference
public Job setReference(JobReference reference)
Optional. The fully qualified reference to the job, which can be used to obtain the equivalent
REST path of the job resource. If this property is not specified when a job is created, the
server generates a job_id.
- Parameters:
reference
- reference or null
for none
-
getScheduling
public JobScheduling getScheduling()
Optional. Job scheduling configuration.
- Returns:
- value or
null
for none
-
setScheduling
public Job setScheduling(JobScheduling scheduling)
Optional. Job scheduling configuration.
- Parameters:
scheduling
- scheduling or null
for none
-
getSparkJob
public SparkJob getSparkJob()
Optional. Job is a Spark job.
- Returns:
- value or
null
for none
-
setSparkJob
public Job setSparkJob(SparkJob sparkJob)
Optional. Job is a Spark job.
- Parameters:
sparkJob
- sparkJob or null
for none
-
getSparkRJob
public SparkRJob getSparkRJob()
Optional. Job is a SparkR job.
- Returns:
- value or
null
for none
-
setSparkRJob
public Job setSparkRJob(SparkRJob sparkRJob)
Optional. Job is a SparkR job.
- Parameters:
sparkRJob
- sparkRJob or null
for none
-
getSparkSqlJob
public SparkSqlJob getSparkSqlJob()
Optional. Job is a SparkSql job.
- Returns:
- value or
null
for none
-
setSparkSqlJob
public Job setSparkSqlJob(SparkSqlJob sparkSqlJob)
Optional. Job is a SparkSql job.
- Parameters:
sparkSqlJob
- sparkSqlJob or null
for none
-
getStatus
public JobStatus getStatus()
Output only. The job status. Additional application-specific status information might be
contained in the type_job and yarn_applications fields.
- Returns:
- value or
null
for none
-
setStatus
public Job setStatus(JobStatus status)
Output only. The job status. Additional application-specific status information might be
contained in the type_job and yarn_applications fields.
- Parameters:
status
- status or null
for none
-
getStatusHistory
public List<JobStatus> getStatusHistory()
Output only. The previous job status.
- Returns:
- value or
null
for none
-
setStatusHistory
public Job setStatusHistory(List<JobStatus> statusHistory)
Output only. The previous job status.
- Parameters:
statusHistory
- statusHistory or null
for none
-
getTrinoJob
public TrinoJob getTrinoJob()
Optional. Job is a Trino job.
- Returns:
- value or
null
for none
-
setTrinoJob
public Job setTrinoJob(TrinoJob trinoJob)
Optional. Job is a Trino job.
- Parameters:
trinoJob
- trinoJob or null
for none
-
getYarnApplications
public List<YarnApplication> getYarnApplications()
Output only. The collection of YARN applications spun up by this job.Beta Feature: This report
is available for testing purposes only. It might be changed before final release.
- Returns:
- value or
null
for none
-
setYarnApplications
public Job setYarnApplications(List<YarnApplication> yarnApplications)
Output only. The collection of YARN applications spun up by this job.Beta Feature: This report
is available for testing purposes only. It might be changed before final release.
- Parameters:
yarnApplications
- yarnApplications or null
for none
-
set
public Job set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Job clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy