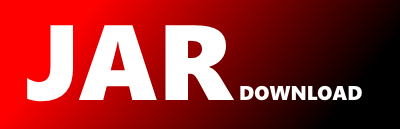
com.google.api.services.dataproc.model.Cluster Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Describes the identifying information, config, and status of a Dataproc cluster
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Cluster extends com.google.api.client.json.GenericJson {
/**
* Required. The cluster name, which must be unique within a project. The name must start with a
* lowercase letter, and can contain up to 51 lowercase letters, numbers, and hyphens. It cannot
* end with a hyphen. The name of a deleted cluster can be reused.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clusterName;
/**
* Output only. A cluster UUID (Unique Universal Identifier). Dataproc generates this value when
* it creates the cluster.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clusterUuid;
/**
* Optional. The cluster config for a cluster of Compute Engine Instances. Note that Dataproc may
* set default values, and values may change when clusters are updated.Exactly one of
* ClusterConfig or VirtualClusterConfig must be specified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ClusterConfig config;
/**
* Optional. The labels to associate with this cluster. Label keys must contain 1 to 63
* characters, and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). Label values
* may be empty, but, if present, must contain 1 to 63 characters, and must conform to RFC 1035
* (https://www.ietf.org/rfc/rfc1035.txt). No more than 32 labels can be associated with a
* cluster.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Output only. Contains cluster daemon metrics such as HDFS and YARN stats.Beta Feature: This
* report is available for testing purposes only. It may be changed before final release.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ClusterMetrics metrics;
/**
* Required. The Google Cloud Platform project ID that the cluster belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/**
* Output only. Cluster status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ClusterStatus status;
/**
* Output only. The previous cluster status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List statusHistory;
/**
* Optional. The virtual cluster config is used when creating a Dataproc cluster that does not
* directly control the underlying compute resources, for example, when creating a Dataproc-on-GKE
* cluster (https://cloud.google.com/dataproc/docs/guides/dpgke/dataproc-gke-overview). Dataproc
* may set default values, and values may change when clusters are updated. Exactly one of config
* or virtual_cluster_config must be specified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private VirtualClusterConfig virtualClusterConfig;
/**
* Required. The cluster name, which must be unique within a project. The name must start with a
* lowercase letter, and can contain up to 51 lowercase letters, numbers, and hyphens. It cannot
* end with a hyphen. The name of a deleted cluster can be reused.
* @return value or {@code null} for none
*/
public java.lang.String getClusterName() {
return clusterName;
}
/**
* Required. The cluster name, which must be unique within a project. The name must start with a
* lowercase letter, and can contain up to 51 lowercase letters, numbers, and hyphens. It cannot
* end with a hyphen. The name of a deleted cluster can be reused.
* @param clusterName clusterName or {@code null} for none
*/
public Cluster setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Output only. A cluster UUID (Unique Universal Identifier). Dataproc generates this value when
* it creates the cluster.
* @return value or {@code null} for none
*/
public java.lang.String getClusterUuid() {
return clusterUuid;
}
/**
* Output only. A cluster UUID (Unique Universal Identifier). Dataproc generates this value when
* it creates the cluster.
* @param clusterUuid clusterUuid or {@code null} for none
*/
public Cluster setClusterUuid(java.lang.String clusterUuid) {
this.clusterUuid = clusterUuid;
return this;
}
/**
* Optional. The cluster config for a cluster of Compute Engine Instances. Note that Dataproc may
* set default values, and values may change when clusters are updated.Exactly one of
* ClusterConfig or VirtualClusterConfig must be specified.
* @return value or {@code null} for none
*/
public ClusterConfig getConfig() {
return config;
}
/**
* Optional. The cluster config for a cluster of Compute Engine Instances. Note that Dataproc may
* set default values, and values may change when clusters are updated.Exactly one of
* ClusterConfig or VirtualClusterConfig must be specified.
* @param config config or {@code null} for none
*/
public Cluster setConfig(ClusterConfig config) {
this.config = config;
return this;
}
/**
* Optional. The labels to associate with this cluster. Label keys must contain 1 to 63
* characters, and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). Label values
* may be empty, but, if present, must contain 1 to 63 characters, and must conform to RFC 1035
* (https://www.ietf.org/rfc/rfc1035.txt). No more than 32 labels can be associated with a
* cluster.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Optional. The labels to associate with this cluster. Label keys must contain 1 to 63
* characters, and must conform to RFC 1035 (https://www.ietf.org/rfc/rfc1035.txt). Label values
* may be empty, but, if present, must contain 1 to 63 characters, and must conform to RFC 1035
* (https://www.ietf.org/rfc/rfc1035.txt). No more than 32 labels can be associated with a
* cluster.
* @param labels labels or {@code null} for none
*/
public Cluster setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Output only. Contains cluster daemon metrics such as HDFS and YARN stats.Beta Feature: This
* report is available for testing purposes only. It may be changed before final release.
* @return value or {@code null} for none
*/
public ClusterMetrics getMetrics() {
return metrics;
}
/**
* Output only. Contains cluster daemon metrics such as HDFS and YARN stats.Beta Feature: This
* report is available for testing purposes only. It may be changed before final release.
* @param metrics metrics or {@code null} for none
*/
public Cluster setMetrics(ClusterMetrics metrics) {
this.metrics = metrics;
return this;
}
/**
* Required. The Google Cloud Platform project ID that the cluster belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* Required. The Google Cloud Platform project ID that the cluster belongs to.
* @param projectId projectId or {@code null} for none
*/
public Cluster setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* Output only. Cluster status.
* @return value or {@code null} for none
*/
public ClusterStatus getStatus() {
return status;
}
/**
* Output only. Cluster status.
* @param status status or {@code null} for none
*/
public Cluster setStatus(ClusterStatus status) {
this.status = status;
return this;
}
/**
* Output only. The previous cluster status.
* @return value or {@code null} for none
*/
public java.util.List getStatusHistory() {
return statusHistory;
}
/**
* Output only. The previous cluster status.
* @param statusHistory statusHistory or {@code null} for none
*/
public Cluster setStatusHistory(java.util.List statusHistory) {
this.statusHistory = statusHistory;
return this;
}
/**
* Optional. The virtual cluster config is used when creating a Dataproc cluster that does not
* directly control the underlying compute resources, for example, when creating a Dataproc-on-GKE
* cluster (https://cloud.google.com/dataproc/docs/guides/dpgke/dataproc-gke-overview). Dataproc
* may set default values, and values may change when clusters are updated. Exactly one of config
* or virtual_cluster_config must be specified.
* @return value or {@code null} for none
*/
public VirtualClusterConfig getVirtualClusterConfig() {
return virtualClusterConfig;
}
/**
* Optional. The virtual cluster config is used when creating a Dataproc cluster that does not
* directly control the underlying compute resources, for example, when creating a Dataproc-on-GKE
* cluster (https://cloud.google.com/dataproc/docs/guides/dpgke/dataproc-gke-overview). Dataproc
* may set default values, and values may change when clusters are updated. Exactly one of config
* or virtual_cluster_config must be specified.
* @param virtualClusterConfig virtualClusterConfig or {@code null} for none
*/
public Cluster setVirtualClusterConfig(VirtualClusterConfig virtualClusterConfig) {
this.virtualClusterConfig = virtualClusterConfig;
return this;
}
@Override
public Cluster set(String fieldName, Object value) {
return (Cluster) super.set(fieldName, value);
}
@Override
public Cluster clone() {
return (Cluster) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy