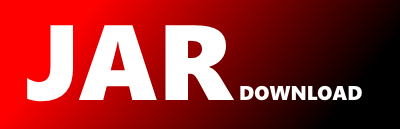
com.google.api.services.dataproc.model.DiskConfig Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Specifies the config of disk options for a group of VM instances.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DiskConfig extends com.google.api.client.json.GenericJson {
/**
* Optional. Indicates how many IOPS to provision for the disk. This sets the number of I/O
* operations per second that the disk can handle. Note: This field is only supported if
* boot_disk_type is hyperdisk-balanced.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bootDiskProvisionedIops;
/**
* Optional. Indicates how much throughput to provision for the disk. This sets the number of
* throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
* Note: This field is only supported if boot_disk_type is hyperdisk-balanced.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long bootDiskProvisionedThroughput;
/**
* Optional. Size in GB of the boot disk (default is 500GB).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer bootDiskSizeGb;
/**
* Optional. Type of the boot disk (default is "pd-standard"). Valid values: "pd-balanced"
* (Persistent Disk Balanced Solid State Drive), "pd-ssd" (Persistent Disk Solid State Drive), or
* "pd-standard" (Persistent Disk Hard Disk Drive). See Disk types
* (https://cloud.google.com/compute/docs/disks#disk-types).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String bootDiskType;
/**
* Optional. Interface type of local SSDs (default is "scsi"). Valid values: "scsi" (Small
* Computer System Interface), "nvme" (Non-Volatile Memory Express). See local SSD performance
* (https://cloud.google.com/compute/docs/disks/local-ssd#performance).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String localSsdInterface;
/**
* Optional. Number of attached SSDs, from 0 to 8 (default is 0). If SSDs are not attached, the
* boot disk is used to store runtime logs and HDFS
* (https://hadoop.apache.org/docs/r1.2.1/hdfs_user_guide.html) data. If one or more SSDs are
* attached, this runtime bulk data is spread across them, and the boot disk contains only basic
* config and installed binaries.Note: Local SSD options may vary by machine type and number of
* vCPUs selected.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numLocalSsds;
/**
* Optional. Indicates how many IOPS to provision for the disk. This sets the number of I/O
* operations per second that the disk can handle. Note: This field is only supported if
* boot_disk_type is hyperdisk-balanced.
* @return value or {@code null} for none
*/
public java.lang.Long getBootDiskProvisionedIops() {
return bootDiskProvisionedIops;
}
/**
* Optional. Indicates how many IOPS to provision for the disk. This sets the number of I/O
* operations per second that the disk can handle. Note: This field is only supported if
* boot_disk_type is hyperdisk-balanced.
* @param bootDiskProvisionedIops bootDiskProvisionedIops or {@code null} for none
*/
public DiskConfig setBootDiskProvisionedIops(java.lang.Long bootDiskProvisionedIops) {
this.bootDiskProvisionedIops = bootDiskProvisionedIops;
return this;
}
/**
* Optional. Indicates how much throughput to provision for the disk. This sets the number of
* throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
* Note: This field is only supported if boot_disk_type is hyperdisk-balanced.
* @return value or {@code null} for none
*/
public java.lang.Long getBootDiskProvisionedThroughput() {
return bootDiskProvisionedThroughput;
}
/**
* Optional. Indicates how much throughput to provision for the disk. This sets the number of
* throughput mb per second that the disk can handle. Values must be greater than or equal to 1.
* Note: This field is only supported if boot_disk_type is hyperdisk-balanced.
* @param bootDiskProvisionedThroughput bootDiskProvisionedThroughput or {@code null} for none
*/
public DiskConfig setBootDiskProvisionedThroughput(java.lang.Long bootDiskProvisionedThroughput) {
this.bootDiskProvisionedThroughput = bootDiskProvisionedThroughput;
return this;
}
/**
* Optional. Size in GB of the boot disk (default is 500GB).
* @return value or {@code null} for none
*/
public java.lang.Integer getBootDiskSizeGb() {
return bootDiskSizeGb;
}
/**
* Optional. Size in GB of the boot disk (default is 500GB).
* @param bootDiskSizeGb bootDiskSizeGb or {@code null} for none
*/
public DiskConfig setBootDiskSizeGb(java.lang.Integer bootDiskSizeGb) {
this.bootDiskSizeGb = bootDiskSizeGb;
return this;
}
/**
* Optional. Type of the boot disk (default is "pd-standard"). Valid values: "pd-balanced"
* (Persistent Disk Balanced Solid State Drive), "pd-ssd" (Persistent Disk Solid State Drive), or
* "pd-standard" (Persistent Disk Hard Disk Drive). See Disk types
* (https://cloud.google.com/compute/docs/disks#disk-types).
* @return value or {@code null} for none
*/
public java.lang.String getBootDiskType() {
return bootDiskType;
}
/**
* Optional. Type of the boot disk (default is "pd-standard"). Valid values: "pd-balanced"
* (Persistent Disk Balanced Solid State Drive), "pd-ssd" (Persistent Disk Solid State Drive), or
* "pd-standard" (Persistent Disk Hard Disk Drive). See Disk types
* (https://cloud.google.com/compute/docs/disks#disk-types).
* @param bootDiskType bootDiskType or {@code null} for none
*/
public DiskConfig setBootDiskType(java.lang.String bootDiskType) {
this.bootDiskType = bootDiskType;
return this;
}
/**
* Optional. Interface type of local SSDs (default is "scsi"). Valid values: "scsi" (Small
* Computer System Interface), "nvme" (Non-Volatile Memory Express). See local SSD performance
* (https://cloud.google.com/compute/docs/disks/local-ssd#performance).
* @return value or {@code null} for none
*/
public java.lang.String getLocalSsdInterface() {
return localSsdInterface;
}
/**
* Optional. Interface type of local SSDs (default is "scsi"). Valid values: "scsi" (Small
* Computer System Interface), "nvme" (Non-Volatile Memory Express). See local SSD performance
* (https://cloud.google.com/compute/docs/disks/local-ssd#performance).
* @param localSsdInterface localSsdInterface or {@code null} for none
*/
public DiskConfig setLocalSsdInterface(java.lang.String localSsdInterface) {
this.localSsdInterface = localSsdInterface;
return this;
}
/**
* Optional. Number of attached SSDs, from 0 to 8 (default is 0). If SSDs are not attached, the
* boot disk is used to store runtime logs and HDFS
* (https://hadoop.apache.org/docs/r1.2.1/hdfs_user_guide.html) data. If one or more SSDs are
* attached, this runtime bulk data is spread across them, and the boot disk contains only basic
* config and installed binaries.Note: Local SSD options may vary by machine type and number of
* vCPUs selected.
* @return value or {@code null} for none
*/
public java.lang.Integer getNumLocalSsds() {
return numLocalSsds;
}
/**
* Optional. Number of attached SSDs, from 0 to 8 (default is 0). If SSDs are not attached, the
* boot disk is used to store runtime logs and HDFS
* (https://hadoop.apache.org/docs/r1.2.1/hdfs_user_guide.html) data. If one or more SSDs are
* attached, this runtime bulk data is spread across them, and the boot disk contains only basic
* config and installed binaries.Note: Local SSD options may vary by machine type and number of
* vCPUs selected.
* @param numLocalSsds numLocalSsds or {@code null} for none
*/
public DiskConfig setNumLocalSsds(java.lang.Integer numLocalSsds) {
this.numLocalSsds = numLocalSsds;
return this;
}
@Override
public DiskConfig set(String fieldName, Object value) {
return (DiskConfig) super.set(fieldName, value);
}
@Override
public DiskConfig clone() {
return (DiskConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy