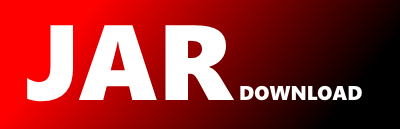
com.google.api.services.dataproc.model.ExecutionConfig Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Execution configuration for a workload.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ExecutionConfig extends com.google.api.client.json.GenericJson {
/**
* Optional. Applies to sessions only. The duration to keep the session alive while it's idling.
* Exceeding this threshold causes the session to terminate. This field cannot be set on a batch
* workload. Minimum value is 10 minutes; maximum value is 14 days (see JSON representation of
* Duration (https://developers.google.com/protocol-buffers/docs/proto3#json)). Defaults to 1 hour
* if not set. If both ttl and idle_ttl are specified for an interactive session, the conditions
* are treated as OR conditions: the workload will be terminated when it has been idle for
* idle_ttl or when ttl has been exceeded, whichever occurs first.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String idleTtl;
/**
* Optional. The Cloud KMS key to use for encryption.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kmsKey;
/**
* Optional. Tags used for network traffic control.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List networkTags;
/**
* Optional. Network URI to connect workload to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String networkUri;
/**
* Optional. Service account that used to execute workload.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceAccount;
/**
* Optional. A Cloud Storage bucket used to stage workload dependencies, config files, and store
* workload output and other ephemeral data, such as Spark history files. If you do not specify a
* staging bucket, Cloud Dataproc will determine a Cloud Storage location according to the region
* where your workload is running, and then create and manage project-level, per-location staging
* and temporary buckets. This field requires a Cloud Storage bucket name, not a gs://... URI to a
* Cloud Storage bucket.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String stagingBucket;
/**
* Optional. Subnetwork URI to connect workload to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String subnetworkUri;
/**
* Optional. The duration after which the workload will be terminated, specified as the JSON
* representation for Duration (https://protobuf.dev/programming-guides/proto3/#json). When the
* workload exceeds this duration, it will be unconditionally terminated without waiting for
* ongoing work to finish. If ttl is not specified for a batch workload, the workload will be
* allowed to run until it exits naturally (or run forever without exiting). If ttl is not
* specified for an interactive session, it defaults to 24 hours. If ttl is not specified for a
* batch that uses 2.1+ runtime version, it defaults to 4 hours. Minimum value is 10 minutes;
* maximum value is 14 days. If both ttl and idle_ttl are specified (for an interactive session),
* the conditions are treated as OR conditions: the workload will be terminated when it has been
* idle for idle_ttl or when ttl has been exceeded, whichever occurs first.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String ttl;
/**
* Optional. Applies to sessions only. The duration to keep the session alive while it's idling.
* Exceeding this threshold causes the session to terminate. This field cannot be set on a batch
* workload. Minimum value is 10 minutes; maximum value is 14 days (see JSON representation of
* Duration (https://developers.google.com/protocol-buffers/docs/proto3#json)). Defaults to 1 hour
* if not set. If both ttl and idle_ttl are specified for an interactive session, the conditions
* are treated as OR conditions: the workload will be terminated when it has been idle for
* idle_ttl or when ttl has been exceeded, whichever occurs first.
* @return value or {@code null} for none
*/
public String getIdleTtl() {
return idleTtl;
}
/**
* Optional. Applies to sessions only. The duration to keep the session alive while it's idling.
* Exceeding this threshold causes the session to terminate. This field cannot be set on a batch
* workload. Minimum value is 10 minutes; maximum value is 14 days (see JSON representation of
* Duration (https://developers.google.com/protocol-buffers/docs/proto3#json)). Defaults to 1 hour
* if not set. If both ttl and idle_ttl are specified for an interactive session, the conditions
* are treated as OR conditions: the workload will be terminated when it has been idle for
* idle_ttl or when ttl has been exceeded, whichever occurs first.
* @param idleTtl idleTtl or {@code null} for none
*/
public ExecutionConfig setIdleTtl(String idleTtl) {
this.idleTtl = idleTtl;
return this;
}
/**
* Optional. The Cloud KMS key to use for encryption.
* @return value or {@code null} for none
*/
public java.lang.String getKmsKey() {
return kmsKey;
}
/**
* Optional. The Cloud KMS key to use for encryption.
* @param kmsKey kmsKey or {@code null} for none
*/
public ExecutionConfig setKmsKey(java.lang.String kmsKey) {
this.kmsKey = kmsKey;
return this;
}
/**
* Optional. Tags used for network traffic control.
* @return value or {@code null} for none
*/
public java.util.List getNetworkTags() {
return networkTags;
}
/**
* Optional. Tags used for network traffic control.
* @param networkTags networkTags or {@code null} for none
*/
public ExecutionConfig setNetworkTags(java.util.List networkTags) {
this.networkTags = networkTags;
return this;
}
/**
* Optional. Network URI to connect workload to.
* @return value or {@code null} for none
*/
public java.lang.String getNetworkUri() {
return networkUri;
}
/**
* Optional. Network URI to connect workload to.
* @param networkUri networkUri or {@code null} for none
*/
public ExecutionConfig setNetworkUri(java.lang.String networkUri) {
this.networkUri = networkUri;
return this;
}
/**
* Optional. Service account that used to execute workload.
* @return value or {@code null} for none
*/
public java.lang.String getServiceAccount() {
return serviceAccount;
}
/**
* Optional. Service account that used to execute workload.
* @param serviceAccount serviceAccount or {@code null} for none
*/
public ExecutionConfig setServiceAccount(java.lang.String serviceAccount) {
this.serviceAccount = serviceAccount;
return this;
}
/**
* Optional. A Cloud Storage bucket used to stage workload dependencies, config files, and store
* workload output and other ephemeral data, such as Spark history files. If you do not specify a
* staging bucket, Cloud Dataproc will determine a Cloud Storage location according to the region
* where your workload is running, and then create and manage project-level, per-location staging
* and temporary buckets. This field requires a Cloud Storage bucket name, not a gs://... URI to a
* Cloud Storage bucket.
* @return value or {@code null} for none
*/
public java.lang.String getStagingBucket() {
return stagingBucket;
}
/**
* Optional. A Cloud Storage bucket used to stage workload dependencies, config files, and store
* workload output and other ephemeral data, such as Spark history files. If you do not specify a
* staging bucket, Cloud Dataproc will determine a Cloud Storage location according to the region
* where your workload is running, and then create and manage project-level, per-location staging
* and temporary buckets. This field requires a Cloud Storage bucket name, not a gs://... URI to a
* Cloud Storage bucket.
* @param stagingBucket stagingBucket or {@code null} for none
*/
public ExecutionConfig setStagingBucket(java.lang.String stagingBucket) {
this.stagingBucket = stagingBucket;
return this;
}
/**
* Optional. Subnetwork URI to connect workload to.
* @return value or {@code null} for none
*/
public java.lang.String getSubnetworkUri() {
return subnetworkUri;
}
/**
* Optional. Subnetwork URI to connect workload to.
* @param subnetworkUri subnetworkUri or {@code null} for none
*/
public ExecutionConfig setSubnetworkUri(java.lang.String subnetworkUri) {
this.subnetworkUri = subnetworkUri;
return this;
}
/**
* Optional. The duration after which the workload will be terminated, specified as the JSON
* representation for Duration (https://protobuf.dev/programming-guides/proto3/#json). When the
* workload exceeds this duration, it will be unconditionally terminated without waiting for
* ongoing work to finish. If ttl is not specified for a batch workload, the workload will be
* allowed to run until it exits naturally (or run forever without exiting). If ttl is not
* specified for an interactive session, it defaults to 24 hours. If ttl is not specified for a
* batch that uses 2.1+ runtime version, it defaults to 4 hours. Minimum value is 10 minutes;
* maximum value is 14 days. If both ttl and idle_ttl are specified (for an interactive session),
* the conditions are treated as OR conditions: the workload will be terminated when it has been
* idle for idle_ttl or when ttl has been exceeded, whichever occurs first.
* @return value or {@code null} for none
*/
public String getTtl() {
return ttl;
}
/**
* Optional. The duration after which the workload will be terminated, specified as the JSON
* representation for Duration (https://protobuf.dev/programming-guides/proto3/#json). When the
* workload exceeds this duration, it will be unconditionally terminated without waiting for
* ongoing work to finish. If ttl is not specified for a batch workload, the workload will be
* allowed to run until it exits naturally (or run forever without exiting). If ttl is not
* specified for an interactive session, it defaults to 24 hours. If ttl is not specified for a
* batch that uses 2.1+ runtime version, it defaults to 4 hours. Minimum value is 10 minutes;
* maximum value is 14 days. If both ttl and idle_ttl are specified (for an interactive session),
* the conditions are treated as OR conditions: the workload will be terminated when it has been
* idle for idle_ttl or when ttl has been exceeded, whichever occurs first.
* @param ttl ttl or {@code null} for none
*/
public ExecutionConfig setTtl(String ttl) {
this.ttl = ttl;
return this;
}
@Override
public ExecutionConfig set(String fieldName, Object value) {
return (ExecutionConfig) super.set(fieldName, value);
}
@Override
public ExecutionConfig clone() {
return (ExecutionConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy