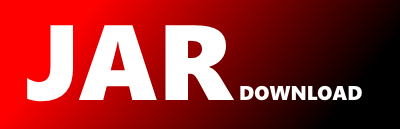
com.google.api.services.dataproc.model.ExecutorMetricsDistributions Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Model definition for ExecutorMetricsDistributions.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ExecutorMetricsDistributions extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List diskBytesSpilled;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List failedTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List inputBytes;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List inputRecords;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List killedTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List memoryBytesSpilled;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List outputBytes;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List outputRecords;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ExecutorPeakMetricsDistributions peakMemoryMetrics;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List quantiles;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List shuffleRead;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List shuffleReadRecords;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List shuffleWrite;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List shuffleWriteRecords;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List succeededTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List taskTimeMillis;
/**
* @return value or {@code null} for none
*/
public java.util.List getDiskBytesSpilled() {
return diskBytesSpilled;
}
/**
* @param diskBytesSpilled diskBytesSpilled or {@code null} for none
*/
public ExecutorMetricsDistributions setDiskBytesSpilled(java.util.List diskBytesSpilled) {
this.diskBytesSpilled = diskBytesSpilled;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getFailedTasks() {
return failedTasks;
}
/**
* @param failedTasks failedTasks or {@code null} for none
*/
public ExecutorMetricsDistributions setFailedTasks(java.util.List failedTasks) {
this.failedTasks = failedTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getInputBytes() {
return inputBytes;
}
/**
* @param inputBytes inputBytes or {@code null} for none
*/
public ExecutorMetricsDistributions setInputBytes(java.util.List inputBytes) {
this.inputBytes = inputBytes;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getInputRecords() {
return inputRecords;
}
/**
* @param inputRecords inputRecords or {@code null} for none
*/
public ExecutorMetricsDistributions setInputRecords(java.util.List inputRecords) {
this.inputRecords = inputRecords;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getKilledTasks() {
return killedTasks;
}
/**
* @param killedTasks killedTasks or {@code null} for none
*/
public ExecutorMetricsDistributions setKilledTasks(java.util.List killedTasks) {
this.killedTasks = killedTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getMemoryBytesSpilled() {
return memoryBytesSpilled;
}
/**
* @param memoryBytesSpilled memoryBytesSpilled or {@code null} for none
*/
public ExecutorMetricsDistributions setMemoryBytesSpilled(java.util.List memoryBytesSpilled) {
this.memoryBytesSpilled = memoryBytesSpilled;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getOutputBytes() {
return outputBytes;
}
/**
* @param outputBytes outputBytes or {@code null} for none
*/
public ExecutorMetricsDistributions setOutputBytes(java.util.List outputBytes) {
this.outputBytes = outputBytes;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getOutputRecords() {
return outputRecords;
}
/**
* @param outputRecords outputRecords or {@code null} for none
*/
public ExecutorMetricsDistributions setOutputRecords(java.util.List outputRecords) {
this.outputRecords = outputRecords;
return this;
}
/**
* @return value or {@code null} for none
*/
public ExecutorPeakMetricsDistributions getPeakMemoryMetrics() {
return peakMemoryMetrics;
}
/**
* @param peakMemoryMetrics peakMemoryMetrics or {@code null} for none
*/
public ExecutorMetricsDistributions setPeakMemoryMetrics(ExecutorPeakMetricsDistributions peakMemoryMetrics) {
this.peakMemoryMetrics = peakMemoryMetrics;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getQuantiles() {
return quantiles;
}
/**
* @param quantiles quantiles or {@code null} for none
*/
public ExecutorMetricsDistributions setQuantiles(java.util.List quantiles) {
this.quantiles = quantiles;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getShuffleRead() {
return shuffleRead;
}
/**
* @param shuffleRead shuffleRead or {@code null} for none
*/
public ExecutorMetricsDistributions setShuffleRead(java.util.List shuffleRead) {
this.shuffleRead = shuffleRead;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getShuffleReadRecords() {
return shuffleReadRecords;
}
/**
* @param shuffleReadRecords shuffleReadRecords or {@code null} for none
*/
public ExecutorMetricsDistributions setShuffleReadRecords(java.util.List shuffleReadRecords) {
this.shuffleReadRecords = shuffleReadRecords;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getShuffleWrite() {
return shuffleWrite;
}
/**
* @param shuffleWrite shuffleWrite or {@code null} for none
*/
public ExecutorMetricsDistributions setShuffleWrite(java.util.List shuffleWrite) {
this.shuffleWrite = shuffleWrite;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getShuffleWriteRecords() {
return shuffleWriteRecords;
}
/**
* @param shuffleWriteRecords shuffleWriteRecords or {@code null} for none
*/
public ExecutorMetricsDistributions setShuffleWriteRecords(java.util.List shuffleWriteRecords) {
this.shuffleWriteRecords = shuffleWriteRecords;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getSucceededTasks() {
return succeededTasks;
}
/**
* @param succeededTasks succeededTasks or {@code null} for none
*/
public ExecutorMetricsDistributions setSucceededTasks(java.util.List succeededTasks) {
this.succeededTasks = succeededTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getTaskTimeMillis() {
return taskTimeMillis;
}
/**
* @param taskTimeMillis taskTimeMillis or {@code null} for none
*/
public ExecutorMetricsDistributions setTaskTimeMillis(java.util.List taskTimeMillis) {
this.taskTimeMillis = taskTimeMillis;
return this;
}
@Override
public ExecutorMetricsDistributions set(String fieldName, Object value) {
return (ExecutorMetricsDistributions) super.set(fieldName, value);
}
@Override
public ExecutorMetricsDistributions clone() {
return (ExecutorMetricsDistributions) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy