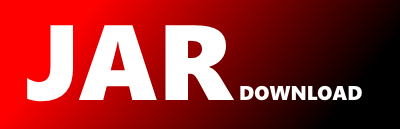
com.google.api.services.dataproc.model.GkeNodeConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Parameters that describe cluster nodes.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GkeNodeConfig extends com.google.api.client.json.GenericJson {
/**
* Optional. A list of hardware accelerators (https://cloud.google.com/compute/docs/gpus) to
* attach to each node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List accelerators;
/**
* Optional. The Customer Managed Encryption Key (CMEK) (https://cloud.google.com/kubernetes-
* engine/docs/how-to/using-cmek) used to encrypt the boot disk attached to each node in the node
* pool. Specify the key using the following format:
* projects/{project}/locations/{location}/keyRings/{key_ring}/cryptoKeys/{crypto_key}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String bootDiskKmsKey;
/**
* Optional. The number of local SSD disks to attach to the node, which is limited by the maximum
* number of disks allowable per zone (see Adding Local SSDs
* (https://cloud.google.com/compute/docs/disks/local-ssd)).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer localSsdCount;
/**
* Optional. The name of a Compute Engine machine type
* (https://cloud.google.com/compute/docs/machine-types).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String machineType;
/**
* Optional. Minimum CPU platform (https://cloud.google.com/compute/docs/instances/specify-min-
* cpu-platform) to be used by this instance. The instance may be scheduled on the specified or a
* newer CPU platform. Specify the friendly names of CPU platforms, such as "Intel Haswell"` or
* Intel Sandy Bridge".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String minCpuPlatform;
/**
* Optional. Whether the nodes are created as legacy preemptible VM instances
* (https://cloud.google.com/compute/docs/instances/preemptible). Also see Spot VMs, preemptible
* VM instances without a maximum lifetime. Legacy and Spot preemptible nodes cannot be used in a
* node pool with the CONTROLLER role or in the DEFAULT node pool if the CONTROLLER role is not
* assigned (the DEFAULT node pool will assume the CONTROLLER role).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean preemptible;
/**
* Optional. Whether the nodes are created as Spot VM instances
* (https://cloud.google.com/compute/docs/instances/spot). Spot VMs are the latest update to
* legacy preemptible VMs. Spot VMs do not have a maximum lifetime. Legacy and Spot preemptible
* nodes cannot be used in a node pool with the CONTROLLER role or in the DEFAULT node pool if the
* CONTROLLER role is not assigned (the DEFAULT node pool will assume the CONTROLLER role).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean spot;
/**
* Optional. A list of hardware accelerators (https://cloud.google.com/compute/docs/gpus) to
* attach to each node.
* @return value or {@code null} for none
*/
public java.util.List getAccelerators() {
return accelerators;
}
/**
* Optional. A list of hardware accelerators (https://cloud.google.com/compute/docs/gpus) to
* attach to each node.
* @param accelerators accelerators or {@code null} for none
*/
public GkeNodeConfig setAccelerators(java.util.List accelerators) {
this.accelerators = accelerators;
return this;
}
/**
* Optional. The Customer Managed Encryption Key (CMEK) (https://cloud.google.com/kubernetes-
* engine/docs/how-to/using-cmek) used to encrypt the boot disk attached to each node in the node
* pool. Specify the key using the following format:
* projects/{project}/locations/{location}/keyRings/{key_ring}/cryptoKeys/{crypto_key}
* @return value or {@code null} for none
*/
public java.lang.String getBootDiskKmsKey() {
return bootDiskKmsKey;
}
/**
* Optional. The Customer Managed Encryption Key (CMEK) (https://cloud.google.com/kubernetes-
* engine/docs/how-to/using-cmek) used to encrypt the boot disk attached to each node in the node
* pool. Specify the key using the following format:
* projects/{project}/locations/{location}/keyRings/{key_ring}/cryptoKeys/{crypto_key}
* @param bootDiskKmsKey bootDiskKmsKey or {@code null} for none
*/
public GkeNodeConfig setBootDiskKmsKey(java.lang.String bootDiskKmsKey) {
this.bootDiskKmsKey = bootDiskKmsKey;
return this;
}
/**
* Optional. The number of local SSD disks to attach to the node, which is limited by the maximum
* number of disks allowable per zone (see Adding Local SSDs
* (https://cloud.google.com/compute/docs/disks/local-ssd)).
* @return value or {@code null} for none
*/
public java.lang.Integer getLocalSsdCount() {
return localSsdCount;
}
/**
* Optional. The number of local SSD disks to attach to the node, which is limited by the maximum
* number of disks allowable per zone (see Adding Local SSDs
* (https://cloud.google.com/compute/docs/disks/local-ssd)).
* @param localSsdCount localSsdCount or {@code null} for none
*/
public GkeNodeConfig setLocalSsdCount(java.lang.Integer localSsdCount) {
this.localSsdCount = localSsdCount;
return this;
}
/**
* Optional. The name of a Compute Engine machine type
* (https://cloud.google.com/compute/docs/machine-types).
* @return value or {@code null} for none
*/
public java.lang.String getMachineType() {
return machineType;
}
/**
* Optional. The name of a Compute Engine machine type
* (https://cloud.google.com/compute/docs/machine-types).
* @param machineType machineType or {@code null} for none
*/
public GkeNodeConfig setMachineType(java.lang.String machineType) {
this.machineType = machineType;
return this;
}
/**
* Optional. Minimum CPU platform (https://cloud.google.com/compute/docs/instances/specify-min-
* cpu-platform) to be used by this instance. The instance may be scheduled on the specified or a
* newer CPU platform. Specify the friendly names of CPU platforms, such as "Intel Haswell"` or
* Intel Sandy Bridge".
* @return value or {@code null} for none
*/
public java.lang.String getMinCpuPlatform() {
return minCpuPlatform;
}
/**
* Optional. Minimum CPU platform (https://cloud.google.com/compute/docs/instances/specify-min-
* cpu-platform) to be used by this instance. The instance may be scheduled on the specified or a
* newer CPU platform. Specify the friendly names of CPU platforms, such as "Intel Haswell"` or
* Intel Sandy Bridge".
* @param minCpuPlatform minCpuPlatform or {@code null} for none
*/
public GkeNodeConfig setMinCpuPlatform(java.lang.String minCpuPlatform) {
this.minCpuPlatform = minCpuPlatform;
return this;
}
/**
* Optional. Whether the nodes are created as legacy preemptible VM instances
* (https://cloud.google.com/compute/docs/instances/preemptible). Also see Spot VMs, preemptible
* VM instances without a maximum lifetime. Legacy and Spot preemptible nodes cannot be used in a
* node pool with the CONTROLLER role or in the DEFAULT node pool if the CONTROLLER role is not
* assigned (the DEFAULT node pool will assume the CONTROLLER role).
* @return value or {@code null} for none
*/
public java.lang.Boolean getPreemptible() {
return preemptible;
}
/**
* Optional. Whether the nodes are created as legacy preemptible VM instances
* (https://cloud.google.com/compute/docs/instances/preemptible). Also see Spot VMs, preemptible
* VM instances without a maximum lifetime. Legacy and Spot preemptible nodes cannot be used in a
* node pool with the CONTROLLER role or in the DEFAULT node pool if the CONTROLLER role is not
* assigned (the DEFAULT node pool will assume the CONTROLLER role).
* @param preemptible preemptible or {@code null} for none
*/
public GkeNodeConfig setPreemptible(java.lang.Boolean preemptible) {
this.preemptible = preemptible;
return this;
}
/**
* Optional. Whether the nodes are created as Spot VM instances
* (https://cloud.google.com/compute/docs/instances/spot). Spot VMs are the latest update to
* legacy preemptible VMs. Spot VMs do not have a maximum lifetime. Legacy and Spot preemptible
* nodes cannot be used in a node pool with the CONTROLLER role or in the DEFAULT node pool if the
* CONTROLLER role is not assigned (the DEFAULT node pool will assume the CONTROLLER role).
* @return value or {@code null} for none
*/
public java.lang.Boolean getSpot() {
return spot;
}
/**
* Optional. Whether the nodes are created as Spot VM instances
* (https://cloud.google.com/compute/docs/instances/spot). Spot VMs are the latest update to
* legacy preemptible VMs. Spot VMs do not have a maximum lifetime. Legacy and Spot preemptible
* nodes cannot be used in a node pool with the CONTROLLER role or in the DEFAULT node pool if the
* CONTROLLER role is not assigned (the DEFAULT node pool will assume the CONTROLLER role).
* @param spot spot or {@code null} for none
*/
public GkeNodeConfig setSpot(java.lang.Boolean spot) {
this.spot = spot;
return this;
}
@Override
public GkeNodeConfig set(String fieldName, Object value) {
return (GkeNodeConfig) super.set(fieldName, value);
}
@Override
public GkeNodeConfig clone() {
return (GkeNodeConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy