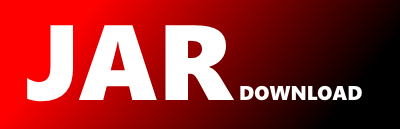
com.google.api.services.dataproc.model.JobData Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Data corresponding to a spark job.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class JobData extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String completionTime;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String jobGroup;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long jobId;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map killTasksSummary;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numActiveStages;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numActiveTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numCompletedIndices;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numCompletedStages;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numCompletedTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numFailedStages;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numFailedTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numKilledTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numSkippedStages;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numSkippedTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numTasks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List skippedStages;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long sqlExecutionId;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.List stageIds;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String submissionTime;
/**
* @return value or {@code null} for none
*/
public String getCompletionTime() {
return completionTime;
}
/**
* @param completionTime completionTime or {@code null} for none
*/
public JobData setCompletionTime(String completionTime) {
this.completionTime = completionTime;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* @param description description or {@code null} for none
*/
public JobData setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getJobGroup() {
return jobGroup;
}
/**
* @param jobGroup jobGroup or {@code null} for none
*/
public JobData setJobGroup(java.lang.String jobGroup) {
this.jobGroup = jobGroup;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getJobId() {
return jobId;
}
/**
* @param jobId jobId or {@code null} for none
*/
public JobData setJobId(java.lang.Long jobId) {
this.jobId = jobId;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.Map getKillTasksSummary() {
return killTasksSummary;
}
/**
* @param killTasksSummary killTasksSummary or {@code null} for none
*/
public JobData setKillTasksSummary(java.util.Map killTasksSummary) {
this.killTasksSummary = killTasksSummary;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* @param name name or {@code null} for none
*/
public JobData setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumActiveStages() {
return numActiveStages;
}
/**
* @param numActiveStages numActiveStages or {@code null} for none
*/
public JobData setNumActiveStages(java.lang.Integer numActiveStages) {
this.numActiveStages = numActiveStages;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumActiveTasks() {
return numActiveTasks;
}
/**
* @param numActiveTasks numActiveTasks or {@code null} for none
*/
public JobData setNumActiveTasks(java.lang.Integer numActiveTasks) {
this.numActiveTasks = numActiveTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumCompletedIndices() {
return numCompletedIndices;
}
/**
* @param numCompletedIndices numCompletedIndices or {@code null} for none
*/
public JobData setNumCompletedIndices(java.lang.Integer numCompletedIndices) {
this.numCompletedIndices = numCompletedIndices;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumCompletedStages() {
return numCompletedStages;
}
/**
* @param numCompletedStages numCompletedStages or {@code null} for none
*/
public JobData setNumCompletedStages(java.lang.Integer numCompletedStages) {
this.numCompletedStages = numCompletedStages;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumCompletedTasks() {
return numCompletedTasks;
}
/**
* @param numCompletedTasks numCompletedTasks or {@code null} for none
*/
public JobData setNumCompletedTasks(java.lang.Integer numCompletedTasks) {
this.numCompletedTasks = numCompletedTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumFailedStages() {
return numFailedStages;
}
/**
* @param numFailedStages numFailedStages or {@code null} for none
*/
public JobData setNumFailedStages(java.lang.Integer numFailedStages) {
this.numFailedStages = numFailedStages;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumFailedTasks() {
return numFailedTasks;
}
/**
* @param numFailedTasks numFailedTasks or {@code null} for none
*/
public JobData setNumFailedTasks(java.lang.Integer numFailedTasks) {
this.numFailedTasks = numFailedTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumKilledTasks() {
return numKilledTasks;
}
/**
* @param numKilledTasks numKilledTasks or {@code null} for none
*/
public JobData setNumKilledTasks(java.lang.Integer numKilledTasks) {
this.numKilledTasks = numKilledTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumSkippedStages() {
return numSkippedStages;
}
/**
* @param numSkippedStages numSkippedStages or {@code null} for none
*/
public JobData setNumSkippedStages(java.lang.Integer numSkippedStages) {
this.numSkippedStages = numSkippedStages;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumSkippedTasks() {
return numSkippedTasks;
}
/**
* @param numSkippedTasks numSkippedTasks or {@code null} for none
*/
public JobData setNumSkippedTasks(java.lang.Integer numSkippedTasks) {
this.numSkippedTasks = numSkippedTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Integer getNumTasks() {
return numTasks;
}
/**
* @param numTasks numTasks or {@code null} for none
*/
public JobData setNumTasks(java.lang.Integer numTasks) {
this.numTasks = numTasks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getSkippedStages() {
return skippedStages;
}
/**
* @param skippedStages skippedStages or {@code null} for none
*/
public JobData setSkippedStages(java.util.List skippedStages) {
this.skippedStages = skippedStages;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getSqlExecutionId() {
return sqlExecutionId;
}
/**
* @param sqlExecutionId sqlExecutionId or {@code null} for none
*/
public JobData setSqlExecutionId(java.lang.Long sqlExecutionId) {
this.sqlExecutionId = sqlExecutionId;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.List getStageIds() {
return stageIds;
}
/**
* @param stageIds stageIds or {@code null} for none
*/
public JobData setStageIds(java.util.List stageIds) {
this.stageIds = stageIds;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* @param status status or {@code null} for none
*/
public JobData setStatus(java.lang.String status) {
this.status = status;
return this;
}
/**
* @return value or {@code null} for none
*/
public String getSubmissionTime() {
return submissionTime;
}
/**
* @param submissionTime submissionTime or {@code null} for none
*/
public JobData setSubmissionTime(String submissionTime) {
this.submissionTime = submissionTime;
return this;
}
@Override
public JobData set(String fieldName, Object value) {
return (JobData) super.set(fieldName, value);
}
@Override
public JobData clone() {
return (JobData) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy