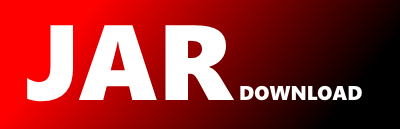
com.google.api.services.dataproc.model.ShufflePushReadMetrics Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Model definition for ShufflePushReadMetrics.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ShufflePushReadMetrics extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long corruptMergedBlockChunks;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long localMergedBlocksFetched;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long localMergedBytesRead;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long localMergedChunksFetched;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long mergedFetchFallbackCount;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long remoteMergedBlocksFetched;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long remoteMergedBytesRead;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long remoteMergedChunksFetched;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long remoteMergedReqsDuration;
/**
* @return value or {@code null} for none
*/
public java.lang.Long getCorruptMergedBlockChunks() {
return corruptMergedBlockChunks;
}
/**
* @param corruptMergedBlockChunks corruptMergedBlockChunks or {@code null} for none
*/
public ShufflePushReadMetrics setCorruptMergedBlockChunks(java.lang.Long corruptMergedBlockChunks) {
this.corruptMergedBlockChunks = corruptMergedBlockChunks;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getLocalMergedBlocksFetched() {
return localMergedBlocksFetched;
}
/**
* @param localMergedBlocksFetched localMergedBlocksFetched or {@code null} for none
*/
public ShufflePushReadMetrics setLocalMergedBlocksFetched(java.lang.Long localMergedBlocksFetched) {
this.localMergedBlocksFetched = localMergedBlocksFetched;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getLocalMergedBytesRead() {
return localMergedBytesRead;
}
/**
* @param localMergedBytesRead localMergedBytesRead or {@code null} for none
*/
public ShufflePushReadMetrics setLocalMergedBytesRead(java.lang.Long localMergedBytesRead) {
this.localMergedBytesRead = localMergedBytesRead;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getLocalMergedChunksFetched() {
return localMergedChunksFetched;
}
/**
* @param localMergedChunksFetched localMergedChunksFetched or {@code null} for none
*/
public ShufflePushReadMetrics setLocalMergedChunksFetched(java.lang.Long localMergedChunksFetched) {
this.localMergedChunksFetched = localMergedChunksFetched;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getMergedFetchFallbackCount() {
return mergedFetchFallbackCount;
}
/**
* @param mergedFetchFallbackCount mergedFetchFallbackCount or {@code null} for none
*/
public ShufflePushReadMetrics setMergedFetchFallbackCount(java.lang.Long mergedFetchFallbackCount) {
this.mergedFetchFallbackCount = mergedFetchFallbackCount;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getRemoteMergedBlocksFetched() {
return remoteMergedBlocksFetched;
}
/**
* @param remoteMergedBlocksFetched remoteMergedBlocksFetched or {@code null} for none
*/
public ShufflePushReadMetrics setRemoteMergedBlocksFetched(java.lang.Long remoteMergedBlocksFetched) {
this.remoteMergedBlocksFetched = remoteMergedBlocksFetched;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getRemoteMergedBytesRead() {
return remoteMergedBytesRead;
}
/**
* @param remoteMergedBytesRead remoteMergedBytesRead or {@code null} for none
*/
public ShufflePushReadMetrics setRemoteMergedBytesRead(java.lang.Long remoteMergedBytesRead) {
this.remoteMergedBytesRead = remoteMergedBytesRead;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getRemoteMergedChunksFetched() {
return remoteMergedChunksFetched;
}
/**
* @param remoteMergedChunksFetched remoteMergedChunksFetched or {@code null} for none
*/
public ShufflePushReadMetrics setRemoteMergedChunksFetched(java.lang.Long remoteMergedChunksFetched) {
this.remoteMergedChunksFetched = remoteMergedChunksFetched;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getRemoteMergedReqsDuration() {
return remoteMergedReqsDuration;
}
/**
* @param remoteMergedReqsDuration remoteMergedReqsDuration or {@code null} for none
*/
public ShufflePushReadMetrics setRemoteMergedReqsDuration(java.lang.Long remoteMergedReqsDuration) {
this.remoteMergedReqsDuration = remoteMergedReqsDuration;
return this;
}
@Override
public ShufflePushReadMetrics set(String fieldName, Object value) {
return (ShufflePushReadMetrics) super.set(fieldName, value);
}
@Override
public ShufflePushReadMetrics clone() {
return (ShufflePushReadMetrics) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy