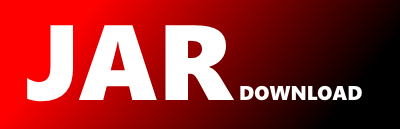
com.google.api.services.dataproc.model.StateOperatorProgress Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dataproc.model;
/**
* Model definition for StateOperatorProgress.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class StateOperatorProgress extends com.google.api.client.json.GenericJson {
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long allRemovalsTimeMs;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long allUpdatesTimeMs;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long commitTimeMs;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.Map customMetrics;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long memoryUsedBytes;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numRowsDroppedByWatermark;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numRowsRemoved;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numRowsTotal;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numRowsUpdated;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numShufflePartitions;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long numStateStoreInstances;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String operatorName;
/**
* @return value or {@code null} for none
*/
public java.lang.Long getAllRemovalsTimeMs() {
return allRemovalsTimeMs;
}
/**
* @param allRemovalsTimeMs allRemovalsTimeMs or {@code null} for none
*/
public StateOperatorProgress setAllRemovalsTimeMs(java.lang.Long allRemovalsTimeMs) {
this.allRemovalsTimeMs = allRemovalsTimeMs;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getAllUpdatesTimeMs() {
return allUpdatesTimeMs;
}
/**
* @param allUpdatesTimeMs allUpdatesTimeMs or {@code null} for none
*/
public StateOperatorProgress setAllUpdatesTimeMs(java.lang.Long allUpdatesTimeMs) {
this.allUpdatesTimeMs = allUpdatesTimeMs;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getCommitTimeMs() {
return commitTimeMs;
}
/**
* @param commitTimeMs commitTimeMs or {@code null} for none
*/
public StateOperatorProgress setCommitTimeMs(java.lang.Long commitTimeMs) {
this.commitTimeMs = commitTimeMs;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.util.Map getCustomMetrics() {
return customMetrics;
}
/**
* @param customMetrics customMetrics or {@code null} for none
*/
public StateOperatorProgress setCustomMetrics(java.util.Map customMetrics) {
this.customMetrics = customMetrics;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getMemoryUsedBytes() {
return memoryUsedBytes;
}
/**
* @param memoryUsedBytes memoryUsedBytes or {@code null} for none
*/
public StateOperatorProgress setMemoryUsedBytes(java.lang.Long memoryUsedBytes) {
this.memoryUsedBytes = memoryUsedBytes;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getNumRowsDroppedByWatermark() {
return numRowsDroppedByWatermark;
}
/**
* @param numRowsDroppedByWatermark numRowsDroppedByWatermark or {@code null} for none
*/
public StateOperatorProgress setNumRowsDroppedByWatermark(java.lang.Long numRowsDroppedByWatermark) {
this.numRowsDroppedByWatermark = numRowsDroppedByWatermark;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getNumRowsRemoved() {
return numRowsRemoved;
}
/**
* @param numRowsRemoved numRowsRemoved or {@code null} for none
*/
public StateOperatorProgress setNumRowsRemoved(java.lang.Long numRowsRemoved) {
this.numRowsRemoved = numRowsRemoved;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getNumRowsTotal() {
return numRowsTotal;
}
/**
* @param numRowsTotal numRowsTotal or {@code null} for none
*/
public StateOperatorProgress setNumRowsTotal(java.lang.Long numRowsTotal) {
this.numRowsTotal = numRowsTotal;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getNumRowsUpdated() {
return numRowsUpdated;
}
/**
* @param numRowsUpdated numRowsUpdated or {@code null} for none
*/
public StateOperatorProgress setNumRowsUpdated(java.lang.Long numRowsUpdated) {
this.numRowsUpdated = numRowsUpdated;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getNumShufflePartitions() {
return numShufflePartitions;
}
/**
* @param numShufflePartitions numShufflePartitions or {@code null} for none
*/
public StateOperatorProgress setNumShufflePartitions(java.lang.Long numShufflePartitions) {
this.numShufflePartitions = numShufflePartitions;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.Long getNumStateStoreInstances() {
return numStateStoreInstances;
}
/**
* @param numStateStoreInstances numStateStoreInstances or {@code null} for none
*/
public StateOperatorProgress setNumStateStoreInstances(java.lang.Long numStateStoreInstances) {
this.numStateStoreInstances = numStateStoreInstances;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getOperatorName() {
return operatorName;
}
/**
* @param operatorName operatorName or {@code null} for none
*/
public StateOperatorProgress setOperatorName(java.lang.String operatorName) {
this.operatorName = operatorName;
return this;
}
@Override
public StateOperatorProgress set(String fieldName, Object value) {
return (StateOperatorProgress) super.set(fieldName, value);
}
@Override
public StateOperatorProgress clone() {
return (StateOperatorProgress) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy