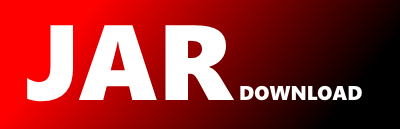
target.apidocs.com.google.api.services.dataproc.model.OrderedJob.html Maven / Gradle / Ivy
The newest version!
OrderedJob (Cloud Dataproc API v1-rev20241025-2.0.0)
com.google.api.services.dataproc.model
Class OrderedJob
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.dataproc.model.OrderedJob
-
public final class OrderedJob
extends com.google.api.client.json.GenericJson
A job executed by the workflow.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Cloud Dataproc API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
OrderedJob()
-
Method Summary
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getFlinkJob
public FlinkJob getFlinkJob()
Optional. Job is a Flink job.
- Returns:
- value or
null
for none
-
setFlinkJob
public OrderedJob setFlinkJob(FlinkJob flinkJob)
Optional. Job is a Flink job.
- Parameters:
flinkJob
- flinkJob or null
for none
-
getHadoopJob
public HadoopJob getHadoopJob()
Optional. Job is a Hadoop job.
- Returns:
- value or
null
for none
-
setHadoopJob
public OrderedJob setHadoopJob(HadoopJob hadoopJob)
Optional. Job is a Hadoop job.
- Parameters:
hadoopJob
- hadoopJob or null
for none
-
getHiveJob
public HiveJob getHiveJob()
Optional. Job is a Hive job.
- Returns:
- value or
null
for none
-
setHiveJob
public OrderedJob setHiveJob(HiveJob hiveJob)
Optional. Job is a Hive job.
- Parameters:
hiveJob
- hiveJob or null
for none
-
getLabels
public Map<String,String> getLabels()
Optional. The labels to associate with this job.Label keys must be between 1 and 63 characters
long, and must conform to the following regular expression: \p{Ll}\p{Lo}{0,62}Label values must
be between 1 and 63 characters long, and must conform to the following regular expression:
\p{Ll}\p{Lo}\p{N}_-{0,63}No more than 32 labels can be associated with a given job.
- Returns:
- value or
null
for none
-
setLabels
public OrderedJob setLabels(Map<String,String> labels)
Optional. The labels to associate with this job.Label keys must be between 1 and 63 characters
long, and must conform to the following regular expression: \p{Ll}\p{Lo}{0,62}Label values must
be between 1 and 63 characters long, and must conform to the following regular expression:
\p{Ll}\p{Lo}\p{N}_-{0,63}No more than 32 labels can be associated with a given job.
- Parameters:
labels
- labels or null
for none
-
getPigJob
public PigJob getPigJob()
Optional. Job is a Pig job.
- Returns:
- value or
null
for none
-
setPigJob
public OrderedJob setPigJob(PigJob pigJob)
Optional. Job is a Pig job.
- Parameters:
pigJob
- pigJob or null
for none
-
getPrerequisiteStepIds
public List<String> getPrerequisiteStepIds()
Optional. The optional list of prerequisite job step_ids. If not specified, the job will start
at the beginning of workflow.
- Returns:
- value or
null
for none
-
setPrerequisiteStepIds
public OrderedJob setPrerequisiteStepIds(List<String> prerequisiteStepIds)
Optional. The optional list of prerequisite job step_ids. If not specified, the job will start
at the beginning of workflow.
- Parameters:
prerequisiteStepIds
- prerequisiteStepIds or null
for none
-
getPrestoJob
public PrestoJob getPrestoJob()
Optional. Job is a Presto job.
- Returns:
- value or
null
for none
-
setPrestoJob
public OrderedJob setPrestoJob(PrestoJob prestoJob)
Optional. Job is a Presto job.
- Parameters:
prestoJob
- prestoJob or null
for none
-
getPysparkJob
public PySparkJob getPysparkJob()
Optional. Job is a PySpark job.
- Returns:
- value or
null
for none
-
setPysparkJob
public OrderedJob setPysparkJob(PySparkJob pysparkJob)
Optional. Job is a PySpark job.
- Parameters:
pysparkJob
- pysparkJob or null
for none
-
getScheduling
public JobScheduling getScheduling()
Optional. Job scheduling configuration.
- Returns:
- value or
null
for none
-
setScheduling
public OrderedJob setScheduling(JobScheduling scheduling)
Optional. Job scheduling configuration.
- Parameters:
scheduling
- scheduling or null
for none
-
getSparkJob
public SparkJob getSparkJob()
Optional. Job is a Spark job.
- Returns:
- value or
null
for none
-
setSparkJob
public OrderedJob setSparkJob(SparkJob sparkJob)
Optional. Job is a Spark job.
- Parameters:
sparkJob
- sparkJob or null
for none
-
getSparkRJob
public SparkRJob getSparkRJob()
Optional. Job is a SparkR job.
- Returns:
- value or
null
for none
-
setSparkRJob
public OrderedJob setSparkRJob(SparkRJob sparkRJob)
Optional. Job is a SparkR job.
- Parameters:
sparkRJob
- sparkRJob or null
for none
-
getSparkSqlJob
public SparkSqlJob getSparkSqlJob()
Optional. Job is a SparkSql job.
- Returns:
- value or
null
for none
-
setSparkSqlJob
public OrderedJob setSparkSqlJob(SparkSqlJob sparkSqlJob)
Optional. Job is a SparkSql job.
- Parameters:
sparkSqlJob
- sparkSqlJob or null
for none
-
getStepId
public String getStepId()
Required. The step id. The id must be unique among all jobs within the template.The step id is
used as prefix for job id, as job goog-dataproc-workflow-step-id label, and in
prerequisiteStepIds field from other steps.The id must contain only letters (a-z, A-Z), numbers
(0-9), underscores (_), and hyphens (-). Cannot begin or end with underscore or hyphen. Must
consist of between 3 and 50 characters.
- Returns:
- value or
null
for none
-
setStepId
public OrderedJob setStepId(String stepId)
Required. The step id. The id must be unique among all jobs within the template.The step id is
used as prefix for job id, as job goog-dataproc-workflow-step-id label, and in
prerequisiteStepIds field from other steps.The id must contain only letters (a-z, A-Z), numbers
(0-9), underscores (_), and hyphens (-). Cannot begin or end with underscore or hyphen. Must
consist of between 3 and 50 characters.
- Parameters:
stepId
- stepId or null
for none
-
getTrinoJob
public TrinoJob getTrinoJob()
Optional. Job is a Trino job.
- Returns:
- value or
null
for none
-
setTrinoJob
public OrderedJob setTrinoJob(TrinoJob trinoJob)
Optional. Job is a Trino job.
- Parameters:
trinoJob
- trinoJob or null
for none
-
set
public OrderedJob set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public OrderedJob clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy