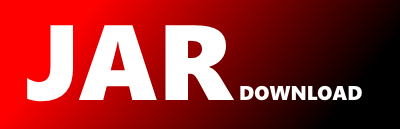
com.google.api.services.dataproc.v1beta2.Dataproc Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-05-04 17:28:03 UTC)
* on 2018-09-12 at 16:10:30 UTC
* Modify at your own risk.
*/
package com.google.api.services.dataproc.v1beta2;
/**
* Service definition for Dataproc (v1beta2).
*
*
* Manages Hadoop-based clusters and jobs on Google Cloud Platform.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link DataprocRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Dataproc extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Cloud Dataproc API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://dataproc.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Dataproc(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Dataproc(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.Projects.List request = dataproc.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.Locations.List request = dataproc.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* An accessor for creating requests from the WorkflowTemplates collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.WorkflowTemplates.List request = dataproc.workflowTemplates().list(parameters ...)}
*
*
* @return the resource collection
*/
public WorkflowTemplates workflowTemplates() {
return new WorkflowTemplates();
}
/**
* The "workflowTemplates" collection of methods.
*/
public class WorkflowTemplates {
/**
* Creates new workflow template.
*
* Create a request for the method "workflowTemplates.create".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+parent}/workflowTemplates";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates new workflow template.
*
* Create a request for the method "workflowTemplates.create".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The "resource name" of the region, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a workflow template. It does not cancel in-progress workflows.
*
* Create a request for the method "workflowTemplates.delete".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
/**
* Deletes a workflow template. It does not cancel in-progress workflows.
*
* Create a request for the method "workflowTemplates.delete".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dataproc.this, "DELETE", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The "resource name" of the workflow template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The version of workflow template to delete. If specified, will only delete
* the template if the current server version matches specified version.
*/
@com.google.api.client.util.Key
private java.lang.Integer version;
/** Optional. The version of workflow template to delete. If specified, will only delete the template
if the current server version matches specified version.
*/
public java.lang.Integer getVersion() {
return version;
}
/**
* Optional. The version of workflow template to delete. If specified, will only delete
* the template if the current server version matches specified version.
*/
public Delete setVersion(java.lang.Integer version) {
this.version = version;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by
* specifying optional version parameter.
*
* Create a request for the method "workflowTemplates.get".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by
* specifying optional version parameter.
*
* Create a request for the method "workflowTemplates.get".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The "resource name" of the workflow template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The version of workflow template to retrieve. Only previously instatiated
* versions can be retrieved.If unspecified, retrieves the current version.
*/
@com.google.api.client.util.Key
private java.lang.Integer version;
/** Optional. The version of workflow template to retrieve. Only previously instatiated versions can be
retrieved.If unspecified, retrieves the current version.
*/
public java.lang.Integer getVersion() {
return version;
}
/**
* Optional. The version of workflow template to retrieve. Only previously instatiated
* versions can be retrieved.If unspecified, retrieves the current version.
*/
public Get setVersion(java.lang.Integer version) {
this.version = version;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "workflowTemplates.getIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "workflowTemplates.getIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Instantiates a template and begins execution.The returned Operation can be used to track
* execution of workflow by polling operations.get. The Operation will complete when entire workflow
* is finished.The running workflow can be aborted via operations.cancel. This will cause any
* inflight jobs to be cancelled and workflow-owned clusters to be deleted.The Operation.metadata
* will be WorkflowMetadata.On successful completion, Operation.response will be Empty.
*
* Create a request for the method "workflowTemplates.instantiate".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Instantiate#execute()} method to invoke the remote operation.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest}
* @return the request
*/
public Instantiate instantiate(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest content) throws java.io.IOException {
Instantiate result = new Instantiate(name, content);
initialize(result);
return result;
}
public class Instantiate extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}:instantiate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
/**
* Instantiates a template and begins execution.The returned Operation can be used to track
* execution of workflow by polling operations.get. The Operation will complete when entire
* workflow is finished.The running workflow can be aborted via operations.cancel. This will cause
* any inflight jobs to be cancelled and workflow-owned clusters to be deleted.The
* Operation.metadata will be WorkflowMetadata.On successful completion, Operation.response will
* be Empty.
*
* Create a request for the method "workflowTemplates.instantiate".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Instantiate#execute()} method to invoke the remote
* operation. {@link
* Instantiate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest}
* @since 1.13
*/
protected Instantiate(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public Instantiate set$Xgafv(java.lang.String $Xgafv) {
return (Instantiate) super.set$Xgafv($Xgafv);
}
@Override
public Instantiate setAccessToken(java.lang.String accessToken) {
return (Instantiate) super.setAccessToken(accessToken);
}
@Override
public Instantiate setAlt(java.lang.String alt) {
return (Instantiate) super.setAlt(alt);
}
@Override
public Instantiate setCallback(java.lang.String callback) {
return (Instantiate) super.setCallback(callback);
}
@Override
public Instantiate setFields(java.lang.String fields) {
return (Instantiate) super.setFields(fields);
}
@Override
public Instantiate setKey(java.lang.String key) {
return (Instantiate) super.setKey(key);
}
@Override
public Instantiate setOauthToken(java.lang.String oauthToken) {
return (Instantiate) super.setOauthToken(oauthToken);
}
@Override
public Instantiate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Instantiate) super.setPrettyPrint(prettyPrint);
}
@Override
public Instantiate setQuotaUser(java.lang.String quotaUser) {
return (Instantiate) super.setQuotaUser(quotaUser);
}
@Override
public Instantiate setUploadType(java.lang.String uploadType) {
return (Instantiate) super.setUploadType(uploadType);
}
@Override
public Instantiate setUploadProtocol(java.lang.String uploadProtocol) {
return (Instantiate) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The "resource name" of the workflow template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Instantiate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Instantiate set(String parameterName, Object value) {
return (Instantiate) super.set(parameterName, value);
}
}
/**
* Instantiates a template and begins execution.This method is equivalent to executing the sequence
* CreateWorkflowTemplate, InstantiateWorkflowTemplate, DeleteWorkflowTemplate.The returned
* Operation can be used to track execution of workflow by polling operations.get. The Operation
* will complete when entire workflow is finished.The running workflow can be aborted via
* operations.cancel. This will cause any inflight jobs to be cancelled and workflow-owned clusters
* to be deleted.The Operation.metadata will be WorkflowMetadata.On successful completion,
* Operation.response will be Empty.
*
* Create a request for the method "workflowTemplates.instantiateInline".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link InstantiateInline#execute()} method to invoke the remote operation.
*
* @param parent Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @return the request
*/
public InstantiateInline instantiateInline(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) throws java.io.IOException {
InstantiateInline result = new InstantiateInline(parent, content);
initialize(result);
return result;
}
public class InstantiateInline extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+parent}/workflowTemplates:instantiateInline";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Instantiates a template and begins execution.This method is equivalent to executing the
* sequence CreateWorkflowTemplate, InstantiateWorkflowTemplate, DeleteWorkflowTemplate.The
* returned Operation can be used to track execution of workflow by polling operations.get. The
* Operation will complete when entire workflow is finished.The running workflow can be aborted
* via operations.cancel. This will cause any inflight jobs to be cancelled and workflow-owned
* clusters to be deleted.The Operation.metadata will be WorkflowMetadata.On successful
* completion, Operation.response will be Empty.
*
* Create a request for the method "workflowTemplates.instantiateInline".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link InstantiateInline#execute()} method to invoke the remote
* operation. {@link InstantiateInline#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @since 1.13
*/
protected InstantiateInline(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public InstantiateInline set$Xgafv(java.lang.String $Xgafv) {
return (InstantiateInline) super.set$Xgafv($Xgafv);
}
@Override
public InstantiateInline setAccessToken(java.lang.String accessToken) {
return (InstantiateInline) super.setAccessToken(accessToken);
}
@Override
public InstantiateInline setAlt(java.lang.String alt) {
return (InstantiateInline) super.setAlt(alt);
}
@Override
public InstantiateInline setCallback(java.lang.String callback) {
return (InstantiateInline) super.setCallback(callback);
}
@Override
public InstantiateInline setFields(java.lang.String fields) {
return (InstantiateInline) super.setFields(fields);
}
@Override
public InstantiateInline setKey(java.lang.String key) {
return (InstantiateInline) super.setKey(key);
}
@Override
public InstantiateInline setOauthToken(java.lang.String oauthToken) {
return (InstantiateInline) super.setOauthToken(oauthToken);
}
@Override
public InstantiateInline setPrettyPrint(java.lang.Boolean prettyPrint) {
return (InstantiateInline) super.setPrettyPrint(prettyPrint);
}
@Override
public InstantiateInline setQuotaUser(java.lang.String quotaUser) {
return (InstantiateInline) super.setQuotaUser(quotaUser);
}
@Override
public InstantiateInline setUploadType(java.lang.String uploadType) {
return (InstantiateInline) super.setUploadType(uploadType);
}
@Override
public InstantiateInline setUploadProtocol(java.lang.String uploadProtocol) {
return (InstantiateInline) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The "resource name" of the workflow template region, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
public InstantiateInline setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A tag that prevents multiple concurrent workflow instances with the same tag
* from running. This mitigates risk of concurrent instances started due to retries.It is
* recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The tag must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String instanceId;
/** Optional. A tag that prevents multiple concurrent workflow instances with the same tag from
running. This mitigates risk of concurrent instances started due to retries.It is recommended to
always set this value to a UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier).The
tag must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getInstanceId() {
return instanceId;
}
/**
* Optional. A tag that prevents multiple concurrent workflow instances with the same tag
* from running. This mitigates risk of concurrent instances started due to retries.It is
* recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The tag must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
public InstantiateInline setInstanceId(java.lang.String instanceId) {
this.instanceId = instanceId;
return this;
}
@Override
public InstantiateInline set(String parameterName, Object value) {
return (InstantiateInline) super.set(parameterName, value);
}
}
/**
* Lists workflows that match the specified filter in the request.
*
* Create a request for the method "workflowTemplates.list".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+parent}/workflowTemplates";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists workflows that match the specified filter in the request.
*
* Create a request for the method "workflowTemplates.list".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.ListWorkflowTemplatesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The "resource name" of the region, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The page token, returned by a previous call, to request the next page of
* results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The page token, returned by a previous call, to request the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The page token, returned by a previous call, to request the next page of
* results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Optional. The maximum number of results to return in each response. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return in each response.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The maximum number of results to return in each response. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "workflowTemplates.setIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "workflowTemplates.setIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "workflowTemplates.testIamPermissions".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "workflowTemplates.testIamPermissions".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Updates (replaces) workflow template. The updated template must contain version that matches the
* current server version.
*
* Create a request for the method "workflowTemplates.update".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param name Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @return the request
*/
public Update update(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) throws java.io.IOException {
Update result = new Update(name, content);
initialize(result);
return result;
}
public class Update extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
/**
* Updates (replaces) workflow template. The updated template must contain version that matches
* the current server version.
*
* Create a request for the method "workflowTemplates.update".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @since 1.13
*/
protected Update(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) {
super(Dataproc.this, "PUT", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The "resource name" of the template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Update setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Regions collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.Regions.List request = dataproc.regions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Regions regions() {
return new Regions();
}
/**
* The "regions" collection of methods.
*/
public class Regions {
/**
* An accessor for creating requests from the Clusters collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.Clusters.List request = dataproc.clusters().list(parameters ...)}
*
*
* @return the resource collection
*/
public Clusters clusters() {
return new Clusters();
}
/**
* The "clusters" collection of methods.
*/
public class Clusters {
/**
* Creates a cluster in a project.
*
* Create a request for the method "clusters.create".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.Cluster}
* @return the request
*/
public Create create(java.lang.String projectId, java.lang.String region, com.google.api.services.dataproc.v1beta2.model.Cluster content) throws java.io.IOException {
Create result = new Create(projectId, region, content);
initialize(result);
return result;
}
public class Create extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/clusters";
/**
* Creates a cluster in a project.
*
* Create a request for the method "clusters.create".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.Cluster}
* @since 1.13
*/
protected Create(java.lang.String projectId, java.lang.String region, com.google.api.services.dataproc.v1beta2.model.Cluster content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Create setRegion(java.lang.String region) {
this.region = region;
return this;
}
/**
* Optional. A unique id used to identify the request. If the server receives two
* CreateClusterRequest requests with the same id, then the second request will be ignored
* and the first google.longrunning.Operation created and stored in the backend is
* returned.It is recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The id must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique id used to identify the request. If the server receives two CreateClusterRequest
requests with the same id, then the second request will be ignored and the first
google.longrunning.Operation created and stored in the backend is returned.It is recommended to
always set this value to a UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier).The
id must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique id used to identify the request. If the server receives two
* CreateClusterRequest requests with the same id, then the second request will be ignored
* and the first google.longrunning.Operation created and stored in the backend is
* returned.It is recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The id must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a cluster in a project.
*
* Create a request for the method "clusters.delete".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @return the request
*/
public Delete delete(java.lang.String projectId, java.lang.String region, java.lang.String clusterName) throws java.io.IOException {
Delete result = new Delete(projectId, region, clusterName);
initialize(result);
return result;
}
public class Delete extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/clusters/{clusterName}";
/**
* Deletes a cluster in a project.
*
* Create a request for the method "clusters.delete".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @since 1.13
*/
protected Delete(java.lang.String projectId, java.lang.String region, java.lang.String clusterName) {
super(Dataproc.this, "DELETE", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.clusterName = com.google.api.client.util.Preconditions.checkNotNull(clusterName, "Required parameter clusterName must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
public Delete setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Delete setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The cluster name. */
@com.google.api.client.util.Key
private java.lang.String clusterName;
/** Required. The cluster name.
*/
public java.lang.String getClusterName() {
return clusterName;
}
/** Required. The cluster name. */
public Delete setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Optional. Specifying the cluster_uuid means the RPC should fail (with error NOT_FOUND)
* if cluster with specified UUID does not exist.
*/
@com.google.api.client.util.Key
private java.lang.String clusterUuid;
/** Optional. Specifying the cluster_uuid means the RPC should fail (with error NOT_FOUND) if cluster
with specified UUID does not exist.
*/
public java.lang.String getClusterUuid() {
return clusterUuid;
}
/**
* Optional. Specifying the cluster_uuid means the RPC should fail (with error NOT_FOUND)
* if cluster with specified UUID does not exist.
*/
public Delete setClusterUuid(java.lang.String clusterUuid) {
this.clusterUuid = clusterUuid;
return this;
}
/**
* Optional. A unique id used to identify the request. If the server receives two
* DeleteClusterRequest requests with the same id, then the second request will be ignored
* and the first google.longrunning.Operation created and stored in the backend is
* returned.It is recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The id must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique id used to identify the request. If the server receives two DeleteClusterRequest
requests with the same id, then the second request will be ignored and the first
google.longrunning.Operation created and stored in the backend is returned.It is recommended to
always set this value to a UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier).The
id must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique id used to identify the request. If the server receives two
* DeleteClusterRequest requests with the same id, then the second request will be ignored
* and the first google.longrunning.Operation created and stored in the backend is
* returned.It is recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The id must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets cluster diagnostic information. After the operation completes, the Operation.response field
* contains DiagnoseClusterOutputLocation.
*
* Create a request for the method "clusters.diagnose".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Diagnose#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.DiagnoseClusterRequest}
* @return the request
*/
public Diagnose diagnose(java.lang.String projectId, java.lang.String region, java.lang.String clusterName, com.google.api.services.dataproc.v1beta2.model.DiagnoseClusterRequest content) throws java.io.IOException {
Diagnose result = new Diagnose(projectId, region, clusterName, content);
initialize(result);
return result;
}
public class Diagnose extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/clusters/{clusterName}:diagnose";
/**
* Gets cluster diagnostic information. After the operation completes, the Operation.response
* field contains DiagnoseClusterOutputLocation.
*
* Create a request for the method "clusters.diagnose".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Diagnose#execute()} method to invoke the remote operation.
* {@link
* Diagnose#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.DiagnoseClusterRequest}
* @since 1.13
*/
protected Diagnose(java.lang.String projectId, java.lang.String region, java.lang.String clusterName, com.google.api.services.dataproc.v1beta2.model.DiagnoseClusterRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.clusterName = com.google.api.client.util.Preconditions.checkNotNull(clusterName, "Required parameter clusterName must be specified.");
}
@Override
public Diagnose set$Xgafv(java.lang.String $Xgafv) {
return (Diagnose) super.set$Xgafv($Xgafv);
}
@Override
public Diagnose setAccessToken(java.lang.String accessToken) {
return (Diagnose) super.setAccessToken(accessToken);
}
@Override
public Diagnose setAlt(java.lang.String alt) {
return (Diagnose) super.setAlt(alt);
}
@Override
public Diagnose setCallback(java.lang.String callback) {
return (Diagnose) super.setCallback(callback);
}
@Override
public Diagnose setFields(java.lang.String fields) {
return (Diagnose) super.setFields(fields);
}
@Override
public Diagnose setKey(java.lang.String key) {
return (Diagnose) super.setKey(key);
}
@Override
public Diagnose setOauthToken(java.lang.String oauthToken) {
return (Diagnose) super.setOauthToken(oauthToken);
}
@Override
public Diagnose setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Diagnose) super.setPrettyPrint(prettyPrint);
}
@Override
public Diagnose setQuotaUser(java.lang.String quotaUser) {
return (Diagnose) super.setQuotaUser(quotaUser);
}
@Override
public Diagnose setUploadType(java.lang.String uploadType) {
return (Diagnose) super.setUploadType(uploadType);
}
@Override
public Diagnose setUploadProtocol(java.lang.String uploadProtocol) {
return (Diagnose) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
public Diagnose setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Diagnose setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The cluster name. */
@com.google.api.client.util.Key
private java.lang.String clusterName;
/** Required. The cluster name.
*/
public java.lang.String getClusterName() {
return clusterName;
}
/** Required. The cluster name. */
public Diagnose setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
@Override
public Diagnose set(String parameterName, Object value) {
return (Diagnose) super.set(parameterName, value);
}
}
/**
* Gets the resource representation for a cluster in a project.
*
* Create a request for the method "clusters.get".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @return the request
*/
public Get get(java.lang.String projectId, java.lang.String region, java.lang.String clusterName) throws java.io.IOException {
Get result = new Get(projectId, region, clusterName);
initialize(result);
return result;
}
public class Get extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/clusters/{clusterName}";
/**
* Gets the resource representation for a cluster in a project.
*
* Create a request for the method "clusters.get".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @since 1.13
*/
protected Get(java.lang.String projectId, java.lang.String region, java.lang.String clusterName) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Cluster.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.clusterName = com.google.api.client.util.Preconditions.checkNotNull(clusterName, "Required parameter clusterName must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Get setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The cluster name. */
@com.google.api.client.util.Key
private java.lang.String clusterName;
/** Required. The cluster name.
*/
public java.lang.String getClusterName() {
return clusterName;
}
/** Required. The cluster name. */
public Get setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "clusters.getIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "clusters.getIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists all regions/{region}/clusters in a project.
*
* Create a request for the method "clusters.list".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @return the request
*/
public List list(java.lang.String projectId, java.lang.String region) throws java.io.IOException {
List result = new List(projectId, region);
initialize(result);
return result;
}
public class List extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/clusters";
/**
* Lists all regions/{region}/clusters in a project.
*
* Create a request for the method "clusters.list".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @since 1.13
*/
protected List(java.lang.String projectId, java.lang.String region) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.ListClustersResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the cluster belongs to. */
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public List setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Optional. The standard List page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The standard List page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. The standard List page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Optional. The standard List page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The standard List page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The standard List page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A filter constraining the clusters to list. Filters are case-sensitive and
* have the following syntax:field = value AND field = value ...where field is one of
* status.state, clusterName, or labels.[KEY], and [KEY] is a label key. value can be * to
* match all values. status.state can be one of the following: ACTIVE, INACTIVE, CREATING,
* RUNNING, ERROR, DELETING, or UPDATING. ACTIVE contains the CREATING, UPDATING, and
* RUNNING states. INACTIVE contains the DELETING and ERROR states. clusterName is the
* name of the cluster provided at creation time. Only the logical AND operator is
* supported; space-separated items are treated as having an implicit AND operator.Example
* filter:status.state = ACTIVE AND clusterName = mycluster AND labels.env = staging AND
* labels.starred = *
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter constraining the clusters to list. Filters are case-sensitive and have the
following syntax:field = value AND field = value ...where field is one of status.state,
clusterName, or labels.[KEY], and [KEY] is a label key. value can be * to match all values.
status.state can be one of the following: ACTIVE, INACTIVE, CREATING, RUNNING, ERROR, DELETING, or
UPDATING. ACTIVE contains the CREATING, UPDATING, and RUNNING states. INACTIVE contains the
DELETING and ERROR states. clusterName is the name of the cluster provided at creation time. Only
the logical AND operator is supported; space-separated items are treated as having an implicit AND
operator.Example filter:status.state = ACTIVE AND clusterName = mycluster AND labels.env = staging
AND labels.starred = *
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter constraining the clusters to list. Filters are case-sensitive and
* have the following syntax:field = value AND field = value ...where field is one of
* status.state, clusterName, or labels.[KEY], and [KEY] is a label key. value can be * to
* match all values. status.state can be one of the following: ACTIVE, INACTIVE, CREATING,
* RUNNING, ERROR, DELETING, or UPDATING. ACTIVE contains the CREATING, UPDATING, and
* RUNNING states. INACTIVE contains the DELETING and ERROR states. clusterName is the
* name of the cluster provided at creation time. Only the logical AND operator is
* supported; space-separated items are treated as having an implicit AND operator.Example
* filter:status.state = ACTIVE AND clusterName = mycluster AND labels.env = staging AND
* labels.starred = *
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a cluster in a project.
*
* Create a request for the method "clusters.patch".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.Cluster}
* @return the request
*/
public Patch patch(java.lang.String projectId, java.lang.String region, java.lang.String clusterName, com.google.api.services.dataproc.v1beta2.model.Cluster content) throws java.io.IOException {
Patch result = new Patch(projectId, region, clusterName, content);
initialize(result);
return result;
}
public class Patch extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/clusters/{clusterName}";
/**
* Updates a cluster in a project.
*
* Create a request for the method "clusters.patch".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project the cluster belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param clusterName Required. The cluster name.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.Cluster}
* @since 1.13
*/
protected Patch(java.lang.String projectId, java.lang.String region, java.lang.String clusterName, com.google.api.services.dataproc.v1beta2.model.Cluster content) {
super(Dataproc.this, "PATCH", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.clusterName = com.google.api.client.util.Preconditions.checkNotNull(clusterName, "Required parameter clusterName must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project the cluster belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project the cluster belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project the cluster belongs to. */
public Patch setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Patch setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The cluster name. */
@com.google.api.client.util.Key
private java.lang.String clusterName;
/** Required. The cluster name.
*/
public java.lang.String getClusterName() {
return clusterName;
}
/** Required. The cluster name. */
public Patch setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Required. Specifies the path, relative to Cluster, of the field to update. For example,
* to change the number of workers in a cluster to 5, the update_mask parameter would be
* specified as config.worker_config.num_instances, and the PATCH request body would
* specify the new value, as follows: { "config":{ "workerConfig":{ "numInstances":"5" } }
* } Similarly, to change the number of preemptible workers in a cluster to 5, the
* update_mask parameter would be config.secondary_worker_config.num_instances, and the
* PATCH request body would be set as follows: { "config":{ "secondaryWorkerConfig":{
* "numInstances":"5" } } } Note: currently only the following fields can be updated:
*
* MaskPurpose
*
* labelsUpdates labels
*
* config.worker_config.num_instancesResize primary worker group
*
* config.secondary_worker_config.num_instancesResize secondary worker group
*
* config.lifecycle_config.auto_delete_ttlReset MAX TTL duration
*
* config.lifecycle_config.auto_delete_timeUpdate MAX TTL deletion timestamp
*
* config.lifecycle_config.idle_delete_ttlUpdate Idle TTL duration
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Specifies the path, relative to Cluster, of the field to update. For example, to change
the number of workers in a cluster to 5, the update_mask parameter would be specified as
config.worker_config.num_instances, and the PATCH request body would specify the new value, as
follows: { "config":{ "workerConfig":{ "numInstances":"5" } } } Similarly, to change the number of
preemptible workers in a cluster to 5, the update_mask parameter would be
config.secondary_worker_config.num_instances, and the PATCH request body would be set as follows: {
"config":{ "secondaryWorkerConfig":{ "numInstances":"5" } } } Note: currently only the following
fields can be updated:
MaskPurpose
labelsUpdates labels
config.worker_config.num_instancesResize primary worker group
config.secondary_worker_config.num_instancesResize secondary worker group
config.lifecycle_config.auto_delete_ttlReset MAX TTL duration
config.lifecycle_config.auto_delete_timeUpdate MAX TTL deletion timestamp
config.lifecycle_config.idle_delete_ttlUpdate Idle TTL duration
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Specifies the path, relative to Cluster, of the field to update. For example,
* to change the number of workers in a cluster to 5, the update_mask parameter would be
* specified as config.worker_config.num_instances, and the PATCH request body would
* specify the new value, as follows: { "config":{ "workerConfig":{ "numInstances":"5" } }
* } Similarly, to change the number of preemptible workers in a cluster to 5, the
* update_mask parameter would be config.secondary_worker_config.num_instances, and the
* PATCH request body would be set as follows: { "config":{ "secondaryWorkerConfig":{
* "numInstances":"5" } } } Note: currently only the following fields can be updated:
*
* MaskPurpose
*
* labelsUpdates labels
*
* config.worker_config.num_instancesResize primary worker group
*
* config.secondary_worker_config.num_instancesResize secondary worker group
*
* config.lifecycle_config.auto_delete_ttlReset MAX TTL duration
*
* config.lifecycle_config.auto_delete_timeUpdate MAX TTL deletion timestamp
*
* config.lifecycle_config.idle_delete_ttlUpdate Idle TTL duration
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* Optional. Timeout for graceful YARN decomissioning. Graceful decommissioning allows
* removing nodes from the cluster without interrupting jobs in progress. Timeout
* specifies how long to wait for jobs in progress to finish before forcefully removing
* nodes (and potentially interrupting jobs). Default timeout is 0 (for forceful
* decommission), and the maximum allowed timeout is 1 day.Only supported on Dataproc
* image versions 1.2 and higher.
*/
@com.google.api.client.util.Key
private String gracefulDecommissionTimeout;
/** Optional. Timeout for graceful YARN decomissioning. Graceful decommissioning allows removing nodes
from the cluster without interrupting jobs in progress. Timeout specifies how long to wait for jobs
in progress to finish before forcefully removing nodes (and potentially interrupting jobs). Default
timeout is 0 (for forceful decommission), and the maximum allowed timeout is 1 day.Only supported
on Dataproc image versions 1.2 and higher.
*/
public String getGracefulDecommissionTimeout() {
return gracefulDecommissionTimeout;
}
/**
* Optional. Timeout for graceful YARN decomissioning. Graceful decommissioning allows
* removing nodes from the cluster without interrupting jobs in progress. Timeout
* specifies how long to wait for jobs in progress to finish before forcefully removing
* nodes (and potentially interrupting jobs). Default timeout is 0 (for forceful
* decommission), and the maximum allowed timeout is 1 day.Only supported on Dataproc
* image versions 1.2 and higher.
*/
public Patch setGracefulDecommissionTimeout(String gracefulDecommissionTimeout) {
this.gracefulDecommissionTimeout = gracefulDecommissionTimeout;
return this;
}
/**
* Optional. A unique id used to identify the request. If the server receives two
* UpdateClusterRequest requests with the same id, then the second request will be ignored
* and the first google.longrunning.Operation created and stored in the backend is
* returned.It is recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The id must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A unique id used to identify the request. If the server receives two UpdateClusterRequest
requests with the same id, then the second request will be ignored and the first
google.longrunning.Operation created and stored in the backend is returned.It is recommended to
always set this value to a UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier).The
id must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A unique id used to identify the request. If the server receives two
* UpdateClusterRequest requests with the same id, then the second request will be ignored
* and the first google.longrunning.Operation created and stored in the backend is
* returned.It is recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The id must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "clusters.setIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "clusters.setIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "clusters.testIamPermissions".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "clusters.testIamPermissions".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/clusters/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Jobs collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.Jobs.List request = dataproc.jobs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Jobs jobs() {
return new Jobs();
}
/**
* The "jobs" collection of methods.
*/
public class Jobs {
/**
* Starts a job cancellation request. To access the job resource after cancellation, call
* regions/{region}/jobs.list or regions/{region}/jobs.get.
*
* Create a request for the method "jobs.cancel".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.CancelJobRequest}
* @return the request
*/
public Cancel cancel(java.lang.String projectId, java.lang.String region, java.lang.String jobId, com.google.api.services.dataproc.v1beta2.model.CancelJobRequest content) throws java.io.IOException {
Cancel result = new Cancel(projectId, region, jobId, content);
initialize(result);
return result;
}
public class Cancel extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/jobs/{jobId}:cancel";
/**
* Starts a job cancellation request. To access the job resource after cancellation, call
* regions/{region}/jobs.list or regions/{region}/jobs.get.
*
* Create a request for the method "jobs.cancel".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.CancelJobRequest}
* @since 1.13
*/
protected Cancel(java.lang.String projectId, java.lang.String region, java.lang.String jobId, com.google.api.services.dataproc.v1beta2.model.CancelJobRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Job.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the job belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
public Cancel setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Cancel setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The job ID. */
@com.google.api.client.util.Key
private java.lang.String jobId;
/** Required. The job ID.
*/
public java.lang.String getJobId() {
return jobId;
}
/** Required. The job ID. */
public Cancel setJobId(java.lang.String jobId) {
this.jobId = jobId;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes the job from the project. If the job is active, the delete fails, and the response
* returns FAILED_PRECONDITION.
*
* Create a request for the method "jobs.delete".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @return the request
*/
public Delete delete(java.lang.String projectId, java.lang.String region, java.lang.String jobId) throws java.io.IOException {
Delete result = new Delete(projectId, region, jobId);
initialize(result);
return result;
}
public class Delete extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/jobs/{jobId}";
/**
* Deletes the job from the project. If the job is active, the delete fails, and the response
* returns FAILED_PRECONDITION.
*
* Create a request for the method "jobs.delete".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @since 1.13
*/
protected Delete(java.lang.String projectId, java.lang.String region, java.lang.String jobId) {
super(Dataproc.this, "DELETE", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Empty.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the job belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
public Delete setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Delete setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The job ID. */
@com.google.api.client.util.Key
private java.lang.String jobId;
/** Required. The job ID.
*/
public java.lang.String getJobId() {
return jobId;
}
/** Required. The job ID. */
public Delete setJobId(java.lang.String jobId) {
this.jobId = jobId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the resource representation for a job in a project.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @return the request
*/
public Get get(java.lang.String projectId, java.lang.String region, java.lang.String jobId) throws java.io.IOException {
Get result = new Get(projectId, region, jobId);
initialize(result);
return result;
}
public class Get extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/jobs/{jobId}";
/**
* Gets the resource representation for a job in a project.
*
* Create a request for the method "jobs.get".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @since 1.13
*/
protected Get(java.lang.String projectId, java.lang.String region, java.lang.String jobId) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Job.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the job belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Get setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The job ID. */
@com.google.api.client.util.Key
private java.lang.String jobId;
/** Required. The job ID.
*/
public java.lang.String getJobId() {
return jobId;
}
/** Required. The job ID. */
public Get setJobId(java.lang.String jobId) {
this.jobId = jobId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "jobs.getIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "jobs.getIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists regions/{region}/jobs in a project.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @return the request
*/
public List list(java.lang.String projectId, java.lang.String region) throws java.io.IOException {
List result = new List(projectId, region);
initialize(result);
return result;
}
public class List extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/jobs";
/**
* Lists regions/{region}/jobs in a project.
*
* Create a request for the method "jobs.list".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @since 1.13
*/
protected List(java.lang.String projectId, java.lang.String region) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.ListJobsResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the job belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public List setRegion(java.lang.String region) {
this.region = region;
return this;
}
/**
* Optional. The page token, returned by a previous call, to request the next page of
* results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The page token, returned by a previous call, to request the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The page token, returned by a previous call, to request the next page of
* results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Optional. The number of results to return in each response. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The number of results to return in each response.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The number of results to return in each response. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If set, the returned jobs list includes only jobs that were submitted to the
* named cluster.
*/
@com.google.api.client.util.Key
private java.lang.String clusterName;
/** Optional. If set, the returned jobs list includes only jobs that were submitted to the named
cluster.
*/
public java.lang.String getClusterName() {
return clusterName;
}
/**
* Optional. If set, the returned jobs list includes only jobs that were submitted to the
* named cluster.
*/
public List setClusterName(java.lang.String clusterName) {
this.clusterName = clusterName;
return this;
}
/**
* Optional. A filter constraining the jobs to list. Filters are case-sensitive and have
* the following syntax:field = value AND field = value ...where field is status.state or
* labels.[KEY], and [KEY] is a label key. value can be * to match all values.
* status.state can be either ACTIVE or NON_ACTIVE. Only the logical AND operator is
* supported; space-separated items are treated as having an implicit AND operator.Example
* filter:status.state = ACTIVE AND labels.env = staging AND labels.starred = *
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. A filter constraining the jobs to list. Filters are case-sensitive and have the following
syntax:field = value AND field = value ...where field is status.state or labels.[KEY], and [KEY] is
a label key. value can be * to match all values. status.state can be either ACTIVE or NON_ACTIVE.
Only the logical AND operator is supported; space-separated items are treated as having an implicit
AND operator.Example filter:status.state = ACTIVE AND labels.env = staging AND labels.starred = *
*/
public java.lang.String getFilter() {
return filter;
}
/**
* Optional. A filter constraining the jobs to list. Filters are case-sensitive and have
* the following syntax:field = value AND field = value ...where field is status.state or
* labels.[KEY], and [KEY] is a label key. value can be * to match all values.
* status.state can be either ACTIVE or NON_ACTIVE. Only the logical AND operator is
* supported; space-separated items are treated as having an implicit AND operator.Example
* filter:status.state = ACTIVE AND labels.env = staging AND labels.starred = *
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Specifies enumerated categories of jobs to list. (default = match ALL
* jobs).If filter is provided, jobStateMatcher will be ignored.
*/
@com.google.api.client.util.Key
private java.lang.String jobStateMatcher;
/** Optional. Specifies enumerated categories of jobs to list. (default = match ALL jobs).If filter is
provided, jobStateMatcher will be ignored.
*/
public java.lang.String getJobStateMatcher() {
return jobStateMatcher;
}
/**
* Optional. Specifies enumerated categories of jobs to list. (default = match ALL
* jobs).If filter is provided, jobStateMatcher will be ignored.
*/
public List setJobStateMatcher(java.lang.String jobStateMatcher) {
this.jobStateMatcher = jobStateMatcher;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a job in a project.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.Job}
* @return the request
*/
public Patch patch(java.lang.String projectId, java.lang.String region, java.lang.String jobId, com.google.api.services.dataproc.v1beta2.model.Job content) throws java.io.IOException {
Patch result = new Patch(projectId, region, jobId, content);
initialize(result);
return result;
}
public class Patch extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/jobs/{jobId}";
/**
* Updates a job in a project.
*
* Create a request for the method "jobs.patch".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param jobId Required. The job ID.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.Job}
* @since 1.13
*/
protected Patch(java.lang.String projectId, java.lang.String region, java.lang.String jobId, com.google.api.services.dataproc.v1beta2.model.Job content) {
super(Dataproc.this, "PATCH", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Job.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
this.jobId = com.google.api.client.util.Preconditions.checkNotNull(jobId, "Required parameter jobId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the job belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
public Patch setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Patch setRegion(java.lang.String region) {
this.region = region;
return this;
}
/** Required. The job ID. */
@com.google.api.client.util.Key
private java.lang.String jobId;
/** Required. The job ID.
*/
public java.lang.String getJobId() {
return jobId;
}
/** Required. The job ID. */
public Patch setJobId(java.lang.String jobId) {
this.jobId = jobId;
return this;
}
/**
* Required. Specifies the path, relative to Job, of the field to update. For example, to
* update the labels of a Job the update_mask parameter would be specified as labels, and
* the PATCH request body would specify the new value. Note: Currently, labels is the only
* field that can be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Specifies the path, relative to Job, of the field to update. For example, to update the
labels of a Job the update_mask parameter would be specified as labels, and the PATCH request body
would specify the new value. Note: Currently, labels is the only field that can be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Specifies the path, relative to Job, of the field to update. For example, to
* update the labels of a Job the update_mask parameter would be specified as labels, and
* the PATCH request body would specify the new value. Note: Currently, labels is the only
* field that can be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "jobs.setIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "jobs.setIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Submits a job to a cluster.
*
* Create a request for the method "jobs.submit".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Submit#execute()} method to invoke the remote operation.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SubmitJobRequest}
* @return the request
*/
public Submit submit(java.lang.String projectId, java.lang.String region, com.google.api.services.dataproc.v1beta2.model.SubmitJobRequest content) throws java.io.IOException {
Submit result = new Submit(projectId, region, content);
initialize(result);
return result;
}
public class Submit extends DataprocRequest {
private static final String REST_PATH = "v1beta2/projects/{projectId}/regions/{region}/jobs:submit";
/**
* Submits a job to a cluster.
*
* Create a request for the method "jobs.submit".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Submit#execute()} method to invoke the remote operation.
* {@link
* Submit#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId Required. The ID of the Google Cloud Platform project that the job belongs to.
* @param region Required. The Cloud Dataproc region in which to handle the request.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SubmitJobRequest}
* @since 1.13
*/
protected Submit(java.lang.String projectId, java.lang.String region, com.google.api.services.dataproc.v1beta2.model.SubmitJobRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Job.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.region = com.google.api.client.util.Preconditions.checkNotNull(region, "Required parameter region must be specified.");
}
@Override
public Submit set$Xgafv(java.lang.String $Xgafv) {
return (Submit) super.set$Xgafv($Xgafv);
}
@Override
public Submit setAccessToken(java.lang.String accessToken) {
return (Submit) super.setAccessToken(accessToken);
}
@Override
public Submit setAlt(java.lang.String alt) {
return (Submit) super.setAlt(alt);
}
@Override
public Submit setCallback(java.lang.String callback) {
return (Submit) super.setCallback(callback);
}
@Override
public Submit setFields(java.lang.String fields) {
return (Submit) super.setFields(fields);
}
@Override
public Submit setKey(java.lang.String key) {
return (Submit) super.setKey(key);
}
@Override
public Submit setOauthToken(java.lang.String oauthToken) {
return (Submit) super.setOauthToken(oauthToken);
}
@Override
public Submit setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Submit) super.setPrettyPrint(prettyPrint);
}
@Override
public Submit setQuotaUser(java.lang.String quotaUser) {
return (Submit) super.setQuotaUser(quotaUser);
}
@Override
public Submit setUploadType(java.lang.String uploadType) {
return (Submit) super.setUploadType(uploadType);
}
@Override
public Submit setUploadProtocol(java.lang.String uploadProtocol) {
return (Submit) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The ID of the Google Cloud Platform project that the job belongs to.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The ID of the Google Cloud Platform project that the job belongs to. */
public Submit setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
@com.google.api.client.util.Key
private java.lang.String region;
/** Required. The Cloud Dataproc region in which to handle the request.
*/
public java.lang.String getRegion() {
return region;
}
/** Required. The Cloud Dataproc region in which to handle the request. */
public Submit setRegion(java.lang.String region) {
this.region = region;
return this;
}
@Override
public Submit set(String parameterName, Object value) {
return (Submit) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "jobs.testIamPermissions".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "jobs.testIamPermissions".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/jobs/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.Operations.List request = dataproc.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Dataproc.this, "POST", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns google.rpc.Code.UNIMPLEMENTED.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns google.rpc.Code.UNIMPLEMENTED.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dataproc.this, "DELETE", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "operations.getIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "operations.getIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.NOTE: the name binding allows API services to override the
* binding to use different resource name schemes, such as users/operations. To override the
* binding, API services can add a binding such as "/v1/{name=users}/operations" to their service
* configuration. For backwards compatibility, the default name includes the operations collection
* id, however overriding users must ensure the name binding is the parent resource, without the
* operations collection id.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.NOTE: the name binding allows API services to override
* the binding to use different resource name schemes, such as users/operations. To override the
* binding, API services can add a binding such as "/v1/{name=users}/operations" to their service
* configuration. For backwards compatibility, the default name includes the operations collection
* id, however overriding users must ensure the name binding is the parent resource, without the
* operations collection id.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "operations.setIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "operations.setIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "operations.testIamPermissions".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "operations.testIamPermissions".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/operations/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the WorkflowTemplates collection.
*
* The typical use is:
*
* {@code Dataproc dataproc = new Dataproc(...);}
* {@code Dataproc.WorkflowTemplates.List request = dataproc.workflowTemplates().list(parameters ...)}
*
*
* @return the resource collection
*/
public WorkflowTemplates workflowTemplates() {
return new WorkflowTemplates();
}
/**
* The "workflowTemplates" collection of methods.
*/
public class WorkflowTemplates {
/**
* Creates new workflow template.
*
* Create a request for the method "workflowTemplates.create".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+parent}/workflowTemplates";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+$");
/**
* Creates new workflow template.
*
* Create a request for the method "workflowTemplates.create".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The "resource name" of the region, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a workflow template. It does not cancel in-progress workflows.
*
* Create a request for the method "workflowTemplates.delete".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
/**
* Deletes a workflow template. It does not cancel in-progress workflows.
*
* Create a request for the method "workflowTemplates.delete".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dataproc.this, "DELETE", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The "resource name" of the workflow template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The version of workflow template to delete. If specified, will only delete
* the template if the current server version matches specified version.
*/
@com.google.api.client.util.Key
private java.lang.Integer version;
/** Optional. The version of workflow template to delete. If specified, will only delete the template
if the current server version matches specified version.
*/
public java.lang.Integer getVersion() {
return version;
}
/**
* Optional. The version of workflow template to delete. If specified, will only delete
* the template if the current server version matches specified version.
*/
public Delete setVersion(java.lang.Integer version) {
this.version = version;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by
* specifying optional version parameter.
*
* Create a request for the method "workflowTemplates.get".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
/**
* Retrieves the latest workflow template.Can retrieve previously instantiated template by
* specifying optional version parameter.
*
* Create a request for the method "workflowTemplates.get".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The "resource name" of the workflow template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The version of workflow template to retrieve. Only previously instatiated
* versions can be retrieved.If unspecified, retrieves the current version.
*/
@com.google.api.client.util.Key
private java.lang.Integer version;
/** Optional. The version of workflow template to retrieve. Only previously instatiated versions can be
retrieved.If unspecified, retrieves the current version.
*/
public java.lang.Integer getVersion() {
return version;
}
/**
* Optional. The version of workflow template to retrieve. Only previously instatiated
* versions can be retrieved.If unspecified, retrieves the current version.
*/
public Get setVersion(java.lang.Integer version) {
this.version = version;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "workflowTemplates.getIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "workflowTemplates.getIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the operation documentation for
* the appropriate value for this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See the operation
* documentation for the appropriate value for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Instantiates a template and begins execution.The returned Operation can be used to track
* execution of workflow by polling operations.get. The Operation will complete when entire workflow
* is finished.The running workflow can be aborted via operations.cancel. This will cause any
* inflight jobs to be cancelled and workflow-owned clusters to be deleted.The Operation.metadata
* will be WorkflowMetadata.On successful completion, Operation.response will be Empty.
*
* Create a request for the method "workflowTemplates.instantiate".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Instantiate#execute()} method to invoke the remote operation.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest}
* @return the request
*/
public Instantiate instantiate(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest content) throws java.io.IOException {
Instantiate result = new Instantiate(name, content);
initialize(result);
return result;
}
public class Instantiate extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}:instantiate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
/**
* Instantiates a template and begins execution.The returned Operation can be used to track
* execution of workflow by polling operations.get. The Operation will complete when entire
* workflow is finished.The running workflow can be aborted via operations.cancel. This will cause
* any inflight jobs to be cancelled and workflow-owned clusters to be deleted.The
* Operation.metadata will be WorkflowMetadata.On successful completion, Operation.response will
* be Empty.
*
* Create a request for the method "workflowTemplates.instantiate".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Instantiate#execute()} method to invoke the remote
* operation. {@link
* Instantiate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest}
* @since 1.13
*/
protected Instantiate(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.InstantiateWorkflowTemplateRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public Instantiate set$Xgafv(java.lang.String $Xgafv) {
return (Instantiate) super.set$Xgafv($Xgafv);
}
@Override
public Instantiate setAccessToken(java.lang.String accessToken) {
return (Instantiate) super.setAccessToken(accessToken);
}
@Override
public Instantiate setAlt(java.lang.String alt) {
return (Instantiate) super.setAlt(alt);
}
@Override
public Instantiate setCallback(java.lang.String callback) {
return (Instantiate) super.setCallback(callback);
}
@Override
public Instantiate setFields(java.lang.String fields) {
return (Instantiate) super.setFields(fields);
}
@Override
public Instantiate setKey(java.lang.String key) {
return (Instantiate) super.setKey(key);
}
@Override
public Instantiate setOauthToken(java.lang.String oauthToken) {
return (Instantiate) super.setOauthToken(oauthToken);
}
@Override
public Instantiate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Instantiate) super.setPrettyPrint(prettyPrint);
}
@Override
public Instantiate setQuotaUser(java.lang.String quotaUser) {
return (Instantiate) super.setQuotaUser(quotaUser);
}
@Override
public Instantiate setUploadType(java.lang.String uploadType) {
return (Instantiate) super.setUploadType(uploadType);
}
@Override
public Instantiate setUploadProtocol(java.lang.String uploadProtocol) {
return (Instantiate) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The "resource name" of the workflow template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The "resource name" of the workflow template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Instantiate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Instantiate set(String parameterName, Object value) {
return (Instantiate) super.set(parameterName, value);
}
}
/**
* Instantiates a template and begins execution.This method is equivalent to executing the sequence
* CreateWorkflowTemplate, InstantiateWorkflowTemplate, DeleteWorkflowTemplate.The returned
* Operation can be used to track execution of workflow by polling operations.get. The Operation
* will complete when entire workflow is finished.The running workflow can be aborted via
* operations.cancel. This will cause any inflight jobs to be cancelled and workflow-owned clusters
* to be deleted.The Operation.metadata will be WorkflowMetadata.On successful completion,
* Operation.response will be Empty.
*
* Create a request for the method "workflowTemplates.instantiateInline".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link InstantiateInline#execute()} method to invoke the remote operation.
*
* @param parent Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @return the request
*/
public InstantiateInline instantiateInline(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) throws java.io.IOException {
InstantiateInline result = new InstantiateInline(parent, content);
initialize(result);
return result;
}
public class InstantiateInline extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+parent}/workflowTemplates:instantiateInline";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+$");
/**
* Instantiates a template and begins execution.This method is equivalent to executing the
* sequence CreateWorkflowTemplate, InstantiateWorkflowTemplate, DeleteWorkflowTemplate.The
* returned Operation can be used to track execution of workflow by polling operations.get. The
* Operation will complete when entire workflow is finished.The running workflow can be aborted
* via operations.cancel. This will cause any inflight jobs to be cancelled and workflow-owned
* clusters to be deleted.The Operation.metadata will be WorkflowMetadata.On successful
* completion, Operation.response will be Empty.
*
* Create a request for the method "workflowTemplates.instantiateInline".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link InstantiateInline#execute()} method to invoke the remote
* operation. {@link InstantiateInline#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @since 1.13
*/
protected InstantiateInline(java.lang.String parent, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+$");
}
}
@Override
public InstantiateInline set$Xgafv(java.lang.String $Xgafv) {
return (InstantiateInline) super.set$Xgafv($Xgafv);
}
@Override
public InstantiateInline setAccessToken(java.lang.String accessToken) {
return (InstantiateInline) super.setAccessToken(accessToken);
}
@Override
public InstantiateInline setAlt(java.lang.String alt) {
return (InstantiateInline) super.setAlt(alt);
}
@Override
public InstantiateInline setCallback(java.lang.String callback) {
return (InstantiateInline) super.setCallback(callback);
}
@Override
public InstantiateInline setFields(java.lang.String fields) {
return (InstantiateInline) super.setFields(fields);
}
@Override
public InstantiateInline setKey(java.lang.String key) {
return (InstantiateInline) super.setKey(key);
}
@Override
public InstantiateInline setOauthToken(java.lang.String oauthToken) {
return (InstantiateInline) super.setOauthToken(oauthToken);
}
@Override
public InstantiateInline setPrettyPrint(java.lang.Boolean prettyPrint) {
return (InstantiateInline) super.setPrettyPrint(prettyPrint);
}
@Override
public InstantiateInline setQuotaUser(java.lang.String quotaUser) {
return (InstantiateInline) super.setQuotaUser(quotaUser);
}
@Override
public InstantiateInline setUploadType(java.lang.String uploadType) {
return (InstantiateInline) super.setUploadType(uploadType);
}
@Override
public InstantiateInline setUploadProtocol(java.lang.String uploadProtocol) {
return (InstantiateInline) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The "resource name" of the workflow template region, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The "resource name" of the workflow template region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
public InstantiateInline setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A tag that prevents multiple concurrent workflow instances with the same tag
* from running. This mitigates risk of concurrent instances started due to retries.It is
* recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The tag must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
@com.google.api.client.util.Key
private java.lang.String instanceId;
/** Optional. A tag that prevents multiple concurrent workflow instances with the same tag from
running. This mitigates risk of concurrent instances started due to retries.It is recommended to
always set this value to a UUID (https://en.wikipedia.org/wiki/Universally_unique_identifier).The
tag must contain only letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The
maximum length is 40 characters.
*/
public java.lang.String getInstanceId() {
return instanceId;
}
/**
* Optional. A tag that prevents multiple concurrent workflow instances with the same tag
* from running. This mitigates risk of concurrent instances started due to retries.It is
* recommended to always set this value to a UUID
* (https://en.wikipedia.org/wiki/Universally_unique_identifier).The tag must contain only
* letters (a-z, A-Z), numbers (0-9), underscores (_), and hyphens (-). The maximum length
* is 40 characters.
*/
public InstantiateInline setInstanceId(java.lang.String instanceId) {
this.instanceId = instanceId;
return this;
}
@Override
public InstantiateInline set(String parameterName, Object value) {
return (InstantiateInline) super.set(parameterName, value);
}
}
/**
* Lists workflows that match the specified filter in the request.
*
* Create a request for the method "workflowTemplates.list".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+parent}/workflowTemplates";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+$");
/**
* Lists workflows that match the specified filter in the request.
*
* Create a request for the method "workflowTemplates.list".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dataproc.this, "GET", REST_PATH, null, com.google.api.services.dataproc.v1beta2.model.ListWorkflowTemplatesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The "resource name" of the region, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The "resource name" of the region, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The page token, returned by a previous call, to request the next page of
* results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The page token, returned by a previous call, to request the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The page token, returned by a previous call, to request the next page of
* results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Optional. The maximum number of results to return in each response. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of results to return in each response.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Optional. The maximum number of results to return in each response. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "workflowTemplates.setIamPolicy".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "workflowTemplates.setIamPolicy".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the operation documentation for
* the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.SetIamPolicyRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See the operation documentation for
the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See the operation
* documentation for the appropriate value for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "workflowTemplates.testIamPermissions".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a NOT_FOUND error.Note: This operation
* is designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "workflowTemplates.testIamPermissions".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the operation
* documentation for the appropriate value for this field.
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsRequest content) {
super(Dataproc.this, "POST", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See the operation
documentation for the appropriate value for this field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Updates (replaces) workflow template. The updated template must contain version that matches the
* current server version.
*
* Create a request for the method "workflowTemplates.update".
*
* This request holds the parameters needed by the dataproc server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param name Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @return the request
*/
public Update update(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) throws java.io.IOException {
Update result = new Update(name, content);
initialize(result);
return result;
}
public class Update extends DataprocRequest {
private static final String REST_PATH = "v1beta2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
/**
* Updates (replaces) workflow template. The updated template must contain version that matches
* the current server version.
*
* Create a request for the method "workflowTemplates.update".
*
* This request holds the parameters needed by the the dataproc server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
* @param content the {@link com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate}
* @since 1.13
*/
protected Update(java.lang.String name, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate content) {
super(Dataproc.this, "PUT", REST_PATH, content, com.google.api.services.dataproc.v1beta2.model.WorkflowTemplate.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The "resource name" of the template, as described in
https://cloud.google.com/apis/design/resource_names of the form
projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The "resource name" of the template, as described in
* https://cloud.google.com/apis/design/resource_names of the form
* projects/{project_id}/regions/{region}/workflowTemplates/{template_id}
*/
public Update setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/regions/[^/]+/workflowTemplates/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link Dataproc}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Dataproc}. */
@Override
public Dataproc build() {
return new Dataproc(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link DataprocRequestInitializer}.
*
* @since 1.12
*/
public Builder setDataprocRequestInitializer(
DataprocRequestInitializer dataprocRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(dataprocRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}