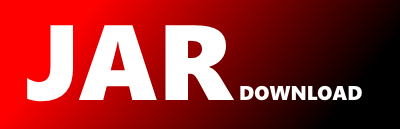
com.google.api.services.datastore.model.Query Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2016-01-08 17:48:37 UTC)
* on 2016-01-12 at 04:49:09 UTC
* Modify at your own risk.
*/
package com.google.api.services.datastore.model;
/**
* A query.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Cloud Datastore API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Query extends com.google.api.client.json.GenericJson {
/**
* An ending point for the query results. Optional. Query cursors are returned in query result
* batches.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String endCursor;
/**
* The filter to apply (optional).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Filter filter;
/**
* The properties to group by (if empty, no grouping is applied to the result set).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List groupBy;
static {
// hack to force ProGuard to consider PropertyReference used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(PropertyReference.class);
}
/**
* The kinds to query (if empty, returns entities from all kinds).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List kinds;
static {
// hack to force ProGuard to consider KindExpression used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(KindExpression.class);
}
/**
* The maximum number of results to return. Applies after all other constraints. Optional.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer limit;
/**
* The number of results to skip. Applies before limit, but after all other constraints (optional,
* defaults to 0).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer offset;
/**
* The order to apply to the query results (if empty, order is unspecified).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List order;
static {
// hack to force ProGuard to consider PropertyOrder used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(PropertyOrder.class);
}
/**
* The projection to return. If not set the entire entity is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List projection;
static {
// hack to force ProGuard to consider PropertyExpression used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(PropertyExpression.class);
}
/**
* A starting point for the query results. Optional. Query cursors are returned in query result
* batches.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String startCursor;
/**
* An ending point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #decodeEndCursor()
* @return value or {@code null} for none
*/
public java.lang.String getEndCursor() {
return endCursor;
}
/**
* An ending point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #getEndCursor()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeEndCursor() {
return com.google.api.client.util.Base64.decodeBase64(endCursor);
}
/**
* An ending point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #encodeEndCursor()
* @param endCursor endCursor or {@code null} for none
*/
public Query setEndCursor(java.lang.String endCursor) {
this.endCursor = endCursor;
return this;
}
/**
* An ending point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #setEndCursor()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public Query encodeEndCursor(byte[] endCursor) {
this.endCursor = com.google.api.client.util.Base64.encodeBase64URLSafeString(endCursor);
return this;
}
/**
* The filter to apply (optional).
* @return value or {@code null} for none
*/
public Filter getFilter() {
return filter;
}
/**
* The filter to apply (optional).
* @param filter filter or {@code null} for none
*/
public Query setFilter(Filter filter) {
this.filter = filter;
return this;
}
/**
* The properties to group by (if empty, no grouping is applied to the result set).
* @return value or {@code null} for none
*/
public java.util.List getGroupBy() {
return groupBy;
}
/**
* The properties to group by (if empty, no grouping is applied to the result set).
* @param groupBy groupBy or {@code null} for none
*/
public Query setGroupBy(java.util.List groupBy) {
this.groupBy = groupBy;
return this;
}
/**
* The kinds to query (if empty, returns entities from all kinds).
* @return value or {@code null} for none
*/
public java.util.List getKinds() {
return kinds;
}
/**
* The kinds to query (if empty, returns entities from all kinds).
* @param kinds kinds or {@code null} for none
*/
public Query setKinds(java.util.List kinds) {
this.kinds = kinds;
return this;
}
/**
* The maximum number of results to return. Applies after all other constraints. Optional.
* @return value or {@code null} for none
*/
public java.lang.Integer getLimit() {
return limit;
}
/**
* The maximum number of results to return. Applies after all other constraints. Optional.
* @param limit limit or {@code null} for none
*/
public Query setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/**
* The number of results to skip. Applies before limit, but after all other constraints (optional,
* defaults to 0).
* @return value or {@code null} for none
*/
public java.lang.Integer getOffset() {
return offset;
}
/**
* The number of results to skip. Applies before limit, but after all other constraints (optional,
* defaults to 0).
* @param offset offset or {@code null} for none
*/
public Query setOffset(java.lang.Integer offset) {
this.offset = offset;
return this;
}
/**
* The order to apply to the query results (if empty, order is unspecified).
* @return value or {@code null} for none
*/
public java.util.List getOrder() {
return order;
}
/**
* The order to apply to the query results (if empty, order is unspecified).
* @param order order or {@code null} for none
*/
public Query setOrder(java.util.List order) {
this.order = order;
return this;
}
/**
* The projection to return. If not set the entire entity is returned.
* @return value or {@code null} for none
*/
public java.util.List getProjection() {
return projection;
}
/**
* The projection to return. If not set the entire entity is returned.
* @param projection projection or {@code null} for none
*/
public Query setProjection(java.util.List projection) {
this.projection = projection;
return this;
}
/**
* A starting point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #decodeStartCursor()
* @return value or {@code null} for none
*/
public java.lang.String getStartCursor() {
return startCursor;
}
/**
* A starting point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #getStartCursor()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeStartCursor() {
return com.google.api.client.util.Base64.decodeBase64(startCursor);
}
/**
* A starting point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #encodeStartCursor()
* @param startCursor startCursor or {@code null} for none
*/
public Query setStartCursor(java.lang.String startCursor) {
this.startCursor = startCursor;
return this;
}
/**
* A starting point for the query results. Optional. Query cursors are returned in query result
* batches.
* @see #setStartCursor()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public Query encodeStartCursor(byte[] startCursor) {
this.startCursor = com.google.api.client.util.Base64.encodeBase64URLSafeString(startCursor);
return this;
}
@Override
public Query set(String fieldName, Object value) {
return (Query) super.set(fieldName, value);
}
@Override
public Query clone() {
return (Query) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy