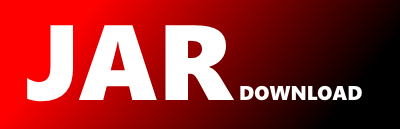
com.google.api.services.datastore.model.Mutation Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2016-04-08 17:16:44 UTC)
* on 2016-04-27 at 02:41:28 UTC
* Modify at your own risk.
*/
package com.google.api.services.datastore.model;
/**
* A set of changes to apply.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Cloud Datastore API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Mutation extends com.google.api.client.json.GenericJson {
/**
* Keys of entities to delete. Each key must have a complete key path and must not be reserved
* /read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List delete;
static {
// hack to force ProGuard to consider Key used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Key.class);
}
/**
* Ignore a user specified read-only period. Optional.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/**
* Entities to insert. Each inserted entity's key must have a complete path and must not be
* reserved/read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List insert;
static {
// hack to force ProGuard to consider Entity used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Entity.class);
}
/**
* Insert entities with a newly allocated ID. Each inserted entity's key must omit the final
* identifier in its path and must not be reserved/read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List insertAutoId;
static {
// hack to force ProGuard to consider Entity used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Entity.class);
}
/**
* Entities to update. Each updated entity's key must have a complete path and must not be
* reserved/read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List update;
static {
// hack to force ProGuard to consider Entity used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Entity.class);
}
/**
* Entities to upsert. Each upserted entity's key must have a complete path and must not be
* reserved/read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List upsert;
static {
// hack to force ProGuard to consider Entity used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Entity.class);
}
/**
* Keys of entities to delete. Each key must have a complete key path and must not be reserved
* /read-only.
* @return value or {@code null} for none
*/
public java.util.List getDelete() {
return delete;
}
/**
* Keys of entities to delete. Each key must have a complete key path and must not be reserved
* /read-only.
* @param delete delete or {@code null} for none
*/
public Mutation setDelete(java.util.List delete) {
this.delete = delete;
return this;
}
/**
* Ignore a user specified read-only period. Optional.
* @return value or {@code null} for none
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Ignore a user specified read-only period. Optional.
* @param force force or {@code null} for none
*/
public Mutation setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* Entities to insert. Each inserted entity's key must have a complete path and must not be
* reserved/read-only.
* @return value or {@code null} for none
*/
public java.util.List getInsert() {
return insert;
}
/**
* Entities to insert. Each inserted entity's key must have a complete path and must not be
* reserved/read-only.
* @param insert insert or {@code null} for none
*/
public Mutation setInsert(java.util.List insert) {
this.insert = insert;
return this;
}
/**
* Insert entities with a newly allocated ID. Each inserted entity's key must omit the final
* identifier in its path and must not be reserved/read-only.
* @return value or {@code null} for none
*/
public java.util.List getInsertAutoId() {
return insertAutoId;
}
/**
* Insert entities with a newly allocated ID. Each inserted entity's key must omit the final
* identifier in its path and must not be reserved/read-only.
* @param insertAutoId insertAutoId or {@code null} for none
*/
public Mutation setInsertAutoId(java.util.List insertAutoId) {
this.insertAutoId = insertAutoId;
return this;
}
/**
* Entities to update. Each updated entity's key must have a complete path and must not be
* reserved/read-only.
* @return value or {@code null} for none
*/
public java.util.List getUpdate() {
return update;
}
/**
* Entities to update. Each updated entity's key must have a complete path and must not be
* reserved/read-only.
* @param update update or {@code null} for none
*/
public Mutation setUpdate(java.util.List update) {
this.update = update;
return this;
}
/**
* Entities to upsert. Each upserted entity's key must have a complete path and must not be
* reserved/read-only.
* @return value or {@code null} for none
*/
public java.util.List getUpsert() {
return upsert;
}
/**
* Entities to upsert. Each upserted entity's key must have a complete path and must not be
* reserved/read-only.
* @param upsert upsert or {@code null} for none
*/
public Mutation setUpsert(java.util.List upsert) {
this.upsert = upsert;
return this;
}
@Override
public Mutation set(String fieldName, Object value) {
return (Mutation) super.set(fieldName, value);
}
@Override
public Mutation clone() {
return (Mutation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy