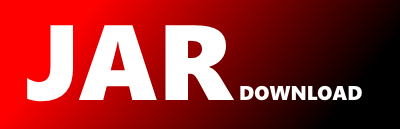
com.google.api.services.deploymentmanager.DeploymentManager Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.deploymentmanager;
/**
* Service definition for DeploymentManager (v2).
*
*
* The Google Cloud Deployment Manager v2 API provides services for configuring, deploying, and viewing Google Cloud services and APIs via templates which specify deployments of Cloud resources.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link DeploymentManagerRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class DeploymentManager extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Deployment Manager V2 API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://deploymentmanager.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://deploymentmanager.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public DeploymentManager(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
DeploymentManager(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Deployments collection.
*
* The typical use is:
*
* {@code DeploymentManager deploymentmanager = new DeploymentManager(...);}
* {@code DeploymentManager.Deployments.List request = deploymentmanager.deployments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Deployments deployments() {
return new Deployments();
}
/**
* The "deployments" collection of methods.
*/
public class Deployments {
/**
* Cancels and removes the preview currently associated with the deployment.
*
* Create a request for the method "deployments.cancelPreview".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link CancelPreview#execute()} method to invoke the remote
* operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.DeploymentsCancelPreviewRequest}
* @return the request
*/
public CancelPreview cancelPreview(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.DeploymentsCancelPreviewRequest content) throws java.io.IOException {
CancelPreview result = new CancelPreview(project, deployment, content);
initialize(result);
return result;
}
public class CancelPreview extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}/cancelPreview";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Cancels and removes the preview currently associated with the deployment.
*
* Create a request for the method "deployments.cancelPreview".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link CancelPreview#execute()} method to invoke the remote
* operation. {@link CancelPreview#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.DeploymentsCancelPreviewRequest}
* @since 1.13
*/
protected CancelPreview(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.DeploymentsCancelPreviewRequest content) {
super(DeploymentManager.this, "POST", REST_PATH, content, com.google.api.services.deploymentmanager.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public CancelPreview set$Xgafv(java.lang.String $Xgafv) {
return (CancelPreview) super.set$Xgafv($Xgafv);
}
@Override
public CancelPreview setAccessToken(java.lang.String accessToken) {
return (CancelPreview) super.setAccessToken(accessToken);
}
@Override
public CancelPreview setAlt(java.lang.String alt) {
return (CancelPreview) super.setAlt(alt);
}
@Override
public CancelPreview setCallback(java.lang.String callback) {
return (CancelPreview) super.setCallback(callback);
}
@Override
public CancelPreview setFields(java.lang.String fields) {
return (CancelPreview) super.setFields(fields);
}
@Override
public CancelPreview setKey(java.lang.String key) {
return (CancelPreview) super.setKey(key);
}
@Override
public CancelPreview setOauthToken(java.lang.String oauthToken) {
return (CancelPreview) super.setOauthToken(oauthToken);
}
@Override
public CancelPreview setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CancelPreview) super.setPrettyPrint(prettyPrint);
}
@Override
public CancelPreview setQuotaUser(java.lang.String quotaUser) {
return (CancelPreview) super.setQuotaUser(quotaUser);
}
@Override
public CancelPreview setUploadType(java.lang.String uploadType) {
return (CancelPreview) super.setUploadType(uploadType);
}
@Override
public CancelPreview setUploadProtocol(java.lang.String uploadProtocol) {
return (CancelPreview) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public CancelPreview setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public CancelPreview setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
@Override
public CancelPreview set(String parameterName, Object value) {
return (CancelPreview) super.set(parameterName, value);
}
}
/**
* Deletes a deployment and all of the resources in the deployment.
*
* Create a request for the method "deployments.delete".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @return the request
*/
public Delete delete(java.lang.String project, java.lang.String deployment) throws java.io.IOException {
Delete result = new Delete(project, deployment);
initialize(result);
return result;
}
public class Delete extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Deletes a deployment and all of the resources in the deployment.
*
* Create a request for the method "deployments.delete".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @since 1.13
*/
protected Delete(java.lang.String project, java.lang.String deployment) {
super(DeploymentManager.this, "DELETE", REST_PATH, null, com.google.api.services.deploymentmanager.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Delete setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public Delete setDeployment(java.lang.String deployment) {
this.deployment = deployment;
return this;
}
/** Sets the policy to use for deleting resources. */
@com.google.api.client.util.Key
private java.lang.String deletePolicy;
/** Sets the policy to use for deleting resources. [default: DELETE]
*/
public java.lang.String getDeletePolicy() {
return deletePolicy;
}
/** Sets the policy to use for deleting resources. */
public Delete setDeletePolicy(java.lang.String deletePolicy) {
this.deletePolicy = deletePolicy;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Delete setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about a specific deployment.
*
* Create a request for the method "deployments.get".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String deployment) throws java.io.IOException {
Get result = new Get(project, deployment);
initialize(result);
return result;
}
public class Get extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Gets information about a specific deployment.
*
* Create a request for the method "deployments.get".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String deployment) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.Deployment.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Get setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public Get setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Get setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. May be empty if no such policy or resource exists.
*
* Create a request for the method "deployments.getIamPolicy".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param resource Name or id of the resource for this request.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String project, java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(project, resource);
initialize(result);
return result;
}
public class GetIamPolicy extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{resource}/getIamPolicy";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
/**
* Gets the access control policy for a resource. May be empty if no such policy or resource
* exists.
*
* Create a request for the method "deployments.getIamPolicy".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param resource Name or id of the resource for this request.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String project, java.lang.String resource) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.Policy.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public GetIamPolicy setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name or id of the resource for this request. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name or id of the resource for this request.
*/
public java.lang.String getResource() {
return resource;
}
/** Name or id of the resource for this request. */
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
}
this.resource = resource;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public GetIamPolicy setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
/** Requested IAM Policy version. */
@com.google.api.client.util.Key
private java.lang.Integer optionsRequestedPolicyVersion;
/** Requested IAM Policy version.
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/** Requested IAM Policy version. */
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Creates a deployment and all of the resources described by the deployment manifest.
*
* Create a request for the method "deployments.insert".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.Deployment}
* @return the request
*/
public Insert insert(java.lang.String project, com.google.api.services.deploymentmanager.model.Deployment content) throws java.io.IOException {
Insert result = new Insert(project, content);
initialize(result);
return result;
}
public class Insert extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Creates a deployment and all of the resources described by the deployment manifest.
*
* Create a request for the method "deployments.insert".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Insert#execute()} method to invoke the remote
* operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.Deployment}
* @since 1.13
*/
protected Insert(java.lang.String project, com.google.api.services.deploymentmanager.model.Deployment content) {
super(DeploymentManager.this, "POST", REST_PATH, content, com.google.api.services.deploymentmanager.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Insert setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Sets the policy to use for creating new resources. */
@com.google.api.client.util.Key
private java.lang.String createPolicy;
/** Sets the policy to use for creating new resources. [default: CREATE_OR_ACQUIRE]
*/
public java.lang.String getCreatePolicy() {
return createPolicy;
}
/** Sets the policy to use for creating new resources. */
public Insert setCreatePolicy(java.lang.String createPolicy) {
this.createPolicy = createPolicy;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Insert setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
/**
* If set to true, creates a deployment and creates "shell" resources but does not actually
* instantiate these resources. This allows you to preview what your deployment looks like.
* After previewing a deployment, you can deploy your resources by making a request with the
* `update()` method or you can use the `cancelPreview()` method to cancel the preview
* altogether. Note that the deployment will still exist after you cancel the preview and you
* must separately delete this deployment if you want to remove it.
*/
@com.google.api.client.util.Key
private java.lang.Boolean preview;
/** If set to true, creates a deployment and creates "shell" resources but does not actually
instantiate these resources. This allows you to preview what your deployment looks like. After
previewing a deployment, you can deploy your resources by making a request with the `update()`
method or you can use the `cancelPreview()` method to cancel the preview altogether. Note that the
deployment will still exist after you cancel the preview and you must separately delete this
deployment if you want to remove it.
*/
public java.lang.Boolean getPreview() {
return preview;
}
/**
* If set to true, creates a deployment and creates "shell" resources but does not actually
* instantiate these resources. This allows you to preview what your deployment looks like.
* After previewing a deployment, you can deploy your resources by making a request with the
* `update()` method or you can use the `cancelPreview()` method to cancel the preview
* altogether. Note that the deployment will still exist after you cancel the preview and you
* must separately delete this deployment if you want to remove it.
*/
public Insert setPreview(java.lang.Boolean preview) {
this.preview = preview;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists all deployments for a given project.
*
* Create a request for the method "deployments.list".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Lists all deployments for a given project.
*
* Create a request for the method "deployments.list".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @since 1.13
*/
protected List(java.lang.String project) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.DeploymentsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters resources listed in the response. Most Compute resources support
two types of filter expressions: expressions that support regular expressions and expressions that
follow API improvement proposal AIP-160. These two types of filter expressions cannot be mixed in
one request. If you want to use AIP-160, your expression must specify the field name, an operator,
and the value that you want to use for filtering. The value must be a string, a number, or a
boolean. The operator must be either `=`, `!=`, `>`, `<`, `<=`, `>=` or `:`. For example, if you
are filtering Compute Engine instances, you can exclude instances named `example-instance` by
specifying `name != example-instance`. The `:*` comparison can be used to test whether a key has
been defined. For example, to find all objects with `owner` label use: ``` labels.owner:* ``` You
can also filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
to include instances only if they are not scheduled for automatic restarts. You can use filtering
on nested fields to filter based on resource labels. To filter on multiple expressions, provide
each separate expression within parentheses. For example: ``` (scheduling.automaticRestart = true)
(cpuPlatform = "Intel Skylake") ``` By default, each expression is an `AND` expression. However,
you can include `AND` and `OR` expressions explicitly. For example: ``` (cpuPlatform = "Intel
Skylake") OR (cpuPlatform = "Intel Broadwell") AND (scheduling.automaticRestart = true) ``` If you
want to use a regular expression, use the `eq` (equal) or `ne` (not equal) operator against a
single un-parenthesized expression with or without quotes or against multiple parenthesized
expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted literal'`
`fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne "literal")` The
literal value is interpreted as a regular expression using Google RE2 library syntax. The literal
value must match the entire field. For example, to filter for instances that do not end with name
"instance", you would use `name ne .*instance`. You cannot combine constraints on multiple fields
using regular expressions.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of results per page that should be returned. If the number of available results
is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be used to get the
next page of results in subsequent list requests. Acceptable values are `0` to `500`, inclusive.
(Default: `500`) [default: 500] [minimum: 0]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name. You can also sort results in descending order based on the creation
timestamp using `orderBy="creationTimestamp desc"`. This sorts results based on the
`creationTimestamp` field in reverse chronological order (newest result first). Use this to sort
resources like operations so that the newest operation is returned first. Currently, only sorting
by `name` or `creationTimestamp desc` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a previous list
request to get the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Patches a deployment and all of the resources described by the deployment manifest.
*
* Create a request for the method "deployments.patch".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.Deployment}
* @return the request
*/
public Patch patch(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.Deployment content) throws java.io.IOException {
Patch result = new Patch(project, deployment, content);
initialize(result);
return result;
}
public class Patch extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Patches a deployment and all of the resources described by the deployment manifest.
*
* Create a request for the method "deployments.patch".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.Deployment}
* @since 1.13
*/
protected Patch(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.Deployment content) {
super(DeploymentManager.this, "PATCH", REST_PATH, content, com.google.api.services.deploymentmanager.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Patch setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public Patch setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
/** Sets the policy to use for creating new resources. */
@com.google.api.client.util.Key
private java.lang.String createPolicy;
/** Sets the policy to use for creating new resources. [default: CREATE_OR_ACQUIRE]
*/
public java.lang.String getCreatePolicy() {
return createPolicy;
}
/** Sets the policy to use for creating new resources. */
public Patch setCreatePolicy(java.lang.String createPolicy) {
this.createPolicy = createPolicy;
return this;
}
/** Sets the policy to use for deleting resources. */
@com.google.api.client.util.Key
private java.lang.String deletePolicy;
/** Sets the policy to use for deleting resources. [default: DELETE]
*/
public java.lang.String getDeletePolicy() {
return deletePolicy;
}
/** Sets the policy to use for deleting resources. */
public Patch setDeletePolicy(java.lang.String deletePolicy) {
this.deletePolicy = deletePolicy;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Patch setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
/**
* If set to true, updates the deployment and creates and updates the "shell" resources but
* does not actually alter or instantiate these resources. This allows you to preview what
* your deployment will look like. You can use this intent to preview how an update would
* affect your deployment. You must provide a `target.config` with a configuration if this is
* set to true. After previewing a deployment, you can deploy your resources by making a
* request with the `update()` or you can `cancelPreview()` to remove the preview altogether.
* Note that the deployment will still exist after you cancel the preview and you must
* separately delete this deployment if you want to remove it.
*/
@com.google.api.client.util.Key
private java.lang.Boolean preview;
/** If set to true, updates the deployment and creates and updates the "shell" resources but does not
actually alter or instantiate these resources. This allows you to preview what your deployment will
look like. You can use this intent to preview how an update would affect your deployment. You must
provide a `target.config` with a configuration if this is set to true. After previewing a
deployment, you can deploy your resources by making a request with the `update()` or you can
`cancelPreview()` to remove the preview altogether. Note that the deployment will still exist after
you cancel the preview and you must separately delete this deployment if you want to remove it.
[default: false]
*/
public java.lang.Boolean getPreview() {
return preview;
}
/**
* If set to true, updates the deployment and creates and updates the "shell" resources but
* does not actually alter or instantiate these resources. This allows you to preview what
* your deployment will look like. You can use this intent to preview how an update would
* affect your deployment. You must provide a `target.config` with a configuration if this is
* set to true. After previewing a deployment, you can deploy your resources by making a
* request with the `update()` or you can `cancelPreview()` to remove the preview altogether.
* Note that the deployment will still exist after you cancel the preview and you must
* separately delete this deployment if you want to remove it.
*/
public Patch setPreview(java.lang.Boolean preview) {
this.preview = preview;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* If set to true, updates the deployment and creates and updates the "shell" resources but does not
actually alter or instantiate these resources. This allows you to preview what your deployment will
look like. You can use this intent to preview how an update would affect your deployment. You must
provide a `target.config` with a configuration if this is set to true. After previewing a
deployment, you can deploy your resources by making a request with the `update()` or you can
`cancelPreview()` to remove the preview altogether. Note that the deployment will still exist after
you cancel the preview and you must separately delete this deployment if you want to remove it.
*
*/
public boolean isPreview() {
if (preview == null || preview == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return preview;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "deployments.setIamPolicy".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param resource Name or id of the resource for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.GlobalSetPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String project, java.lang.String resource, com.google.api.services.deploymentmanager.model.GlobalSetPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(project, resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{resource}/setIamPolicy";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy.
*
* Create a request for the method "deployments.setIamPolicy".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project Project ID for this request.
* @param resource Name or id of the resource for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.GlobalSetPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String project, java.lang.String resource, com.google.api.services.deploymentmanager.model.GlobalSetPolicyRequest content) {
super(DeploymentManager.this, "POST", REST_PATH, content, com.google.api.services.deploymentmanager.model.Policy.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public SetIamPolicy setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name or id of the resource for this request. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name or id of the resource for this request.
*/
public java.lang.String getResource() {
return resource;
}
/** Name or id of the resource for this request. */
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Stops an ongoing operation. This does not roll back any work that has already been completed, but
* prevents any new work from being started.
*
* Create a request for the method "deployments.stop".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Stop#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.DeploymentsStopRequest}
* @return the request
*/
public Stop stop(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.DeploymentsStopRequest content) throws java.io.IOException {
Stop result = new Stop(project, deployment, content);
initialize(result);
return result;
}
public class Stop extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}/stop";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Stops an ongoing operation. This does not roll back any work that has already been completed,
* but prevents any new work from being started.
*
* Create a request for the method "deployments.stop".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Stop#execute()} method to invoke the remote operation.
* {@link
* Stop#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.DeploymentsStopRequest}
* @since 1.13
*/
protected Stop(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.DeploymentsStopRequest content) {
super(DeploymentManager.this, "POST", REST_PATH, content, com.google.api.services.deploymentmanager.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public Stop set$Xgafv(java.lang.String $Xgafv) {
return (Stop) super.set$Xgafv($Xgafv);
}
@Override
public Stop setAccessToken(java.lang.String accessToken) {
return (Stop) super.setAccessToken(accessToken);
}
@Override
public Stop setAlt(java.lang.String alt) {
return (Stop) super.setAlt(alt);
}
@Override
public Stop setCallback(java.lang.String callback) {
return (Stop) super.setCallback(callback);
}
@Override
public Stop setFields(java.lang.String fields) {
return (Stop) super.setFields(fields);
}
@Override
public Stop setKey(java.lang.String key) {
return (Stop) super.setKey(key);
}
@Override
public Stop setOauthToken(java.lang.String oauthToken) {
return (Stop) super.setOauthToken(oauthToken);
}
@Override
public Stop setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Stop) super.setPrettyPrint(prettyPrint);
}
@Override
public Stop setQuotaUser(java.lang.String quotaUser) {
return (Stop) super.setQuotaUser(quotaUser);
}
@Override
public Stop setUploadType(java.lang.String uploadType) {
return (Stop) super.setUploadType(uploadType);
}
@Override
public Stop setUploadProtocol(java.lang.String uploadProtocol) {
return (Stop) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Stop setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public Stop setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
@Override
public Stop set(String parameterName, Object value) {
return (Stop) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource.
*
* Create a request for the method "deployments.testIamPermissions".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param project Project ID for this request.
* @param resource Name or id of the resource for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.TestPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String project, java.lang.String resource, com.google.api.services.deploymentmanager.model.TestPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(project, resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{resource}/testIamPermissions";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
/**
* Returns permissions that a caller has on the specified resource.
*
* Create a request for the method "deployments.testIamPermissions".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param project Project ID for this request.
* @param resource Name or id of the resource for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.TestPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String project, java.lang.String resource, com.google.api.services.deploymentmanager.model.TestPermissionsRequest content) {
super(DeploymentManager.this, "POST", REST_PATH, content, com.google.api.services.deploymentmanager.model.TestPermissionsResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/** Project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** Project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** Project ID for this request. */
public TestIamPermissions setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z0-9](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** Name or id of the resource for this request. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** Name or id of the resource for this request.
*/
public java.lang.String getResource() {
return resource;
}
/** Name or id of the resource for this request. */
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"[a-z](?:[-a-z0-9_]{0,61}[a-z0-9])?|[1-9][0-9]{0,19}");
}
this.resource = resource;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public TestIamPermissions setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Updates a deployment and all of the resources described by the deployment manifest.
*
* Create a request for the method "deployments.update".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.Deployment}
* @return the request
*/
public Update update(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.Deployment content) throws java.io.IOException {
Update result = new Update(project, deployment, content);
initialize(result);
return result;
}
public class Update extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Updates a deployment and all of the resources described by the deployment manifest.
*
* Create a request for the method "deployments.update".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param content the {@link com.google.api.services.deploymentmanager.model.Deployment}
* @since 1.13
*/
protected Update(java.lang.String project, java.lang.String deployment, com.google.api.services.deploymentmanager.model.Deployment content) {
super(DeploymentManager.this, "PUT", REST_PATH, content, com.google.api.services.deploymentmanager.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Update setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public Update setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
/** Sets the policy to use for creating new resources. */
@com.google.api.client.util.Key
private java.lang.String createPolicy;
/** Sets the policy to use for creating new resources. [default: CREATE_OR_ACQUIRE]
*/
public java.lang.String getCreatePolicy() {
return createPolicy;
}
/** Sets the policy to use for creating new resources. */
public Update setCreatePolicy(java.lang.String createPolicy) {
this.createPolicy = createPolicy;
return this;
}
/** Sets the policy to use for deleting resources. */
@com.google.api.client.util.Key
private java.lang.String deletePolicy;
/** Sets the policy to use for deleting resources. [default: DELETE]
*/
public java.lang.String getDeletePolicy() {
return deletePolicy;
}
/** Sets the policy to use for deleting resources. */
public Update setDeletePolicy(java.lang.String deletePolicy) {
this.deletePolicy = deletePolicy;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Update setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
/**
* If set to true, updates the deployment and creates and updates the "shell" resources but
* does not actually alter or instantiate these resources. This allows you to preview what
* your deployment will look like. You can use this intent to preview how an update would
* affect your deployment. You must provide a `target.config` with a configuration if this is
* set to true. After previewing a deployment, you can deploy your resources by making a
* request with the `update()` or you can `cancelPreview()` to remove the preview altogether.
* Note that the deployment will still exist after you cancel the preview and you must
* separately delete this deployment if you want to remove it.
*/
@com.google.api.client.util.Key
private java.lang.Boolean preview;
/** If set to true, updates the deployment and creates and updates the "shell" resources but does not
actually alter or instantiate these resources. This allows you to preview what your deployment will
look like. You can use this intent to preview how an update would affect your deployment. You must
provide a `target.config` with a configuration if this is set to true. After previewing a
deployment, you can deploy your resources by making a request with the `update()` or you can
`cancelPreview()` to remove the preview altogether. Note that the deployment will still exist after
you cancel the preview and you must separately delete this deployment if you want to remove it.
[default: false]
*/
public java.lang.Boolean getPreview() {
return preview;
}
/**
* If set to true, updates the deployment and creates and updates the "shell" resources but
* does not actually alter or instantiate these resources. This allows you to preview what
* your deployment will look like. You can use this intent to preview how an update would
* affect your deployment. You must provide a `target.config` with a configuration if this is
* set to true. After previewing a deployment, you can deploy your resources by making a
* request with the `update()` or you can `cancelPreview()` to remove the preview altogether.
* Note that the deployment will still exist after you cancel the preview and you must
* separately delete this deployment if you want to remove it.
*/
public Update setPreview(java.lang.Boolean preview) {
this.preview = preview;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* If set to true, updates the deployment and creates and updates the "shell" resources but does not
actually alter or instantiate these resources. This allows you to preview what your deployment will
look like. You can use this intent to preview how an update would affect your deployment. You must
provide a `target.config` with a configuration if this is set to true. After previewing a
deployment, you can deploy your resources by making a request with the `update()` or you can
`cancelPreview()` to remove the preview altogether. Note that the deployment will still exist after
you cancel the preview and you must separately delete this deployment if you want to remove it.
*
*/
public boolean isPreview() {
if (preview == null || preview == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return preview;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Manifests collection.
*
* The typical use is:
*
* {@code DeploymentManager deploymentmanager = new DeploymentManager(...);}
* {@code DeploymentManager.Manifests.List request = deploymentmanager.manifests().list(parameters ...)}
*
*
* @return the resource collection
*/
public Manifests manifests() {
return new Manifests();
}
/**
* The "manifests" collection of methods.
*/
public class Manifests {
/**
* Gets information about a specific manifest.
*
* Create a request for the method "manifests.get".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param manifest The name of the manifest for this request.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String deployment, java.lang.String manifest) throws java.io.IOException {
Get result = new Get(project, deployment, manifest);
initialize(result);
return result;
}
public class Get extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}/manifests/{manifest}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
private final java.util.regex.Pattern MANIFEST_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Gets information about a specific manifest.
*
* Create a request for the method "manifests.get".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param manifest The name of the manifest for this request.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String deployment, java.lang.String manifest) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.Manifest.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.manifest = com.google.api.client.util.Preconditions.checkNotNull(manifest, "Required parameter manifest must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(MANIFEST_PATTERN.matcher(manifest).matches(),
"Parameter manifest must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Get setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public Get setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
/** The name of the manifest for this request. */
@com.google.api.client.util.Key
private java.lang.String manifest;
/** The name of the manifest for this request.
*/
public java.lang.String getManifest() {
return manifest;
}
/** The name of the manifest for this request. */
public Get setManifest(java.lang.String manifest) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(MANIFEST_PATTERN.matcher(manifest).matches(),
"Parameter manifest must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.manifest = manifest;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Get setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all manifests for a given deployment.
*
* Create a request for the method "manifests.list".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @return the request
*/
public List list(java.lang.String project, java.lang.String deployment) throws java.io.IOException {
List result = new List(project, deployment);
initialize(result);
return result;
}
public class List extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}/manifests";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Lists all manifests for a given deployment.
*
* Create a request for the method "manifests.list".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String deployment) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.ManifestsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public List setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters resources listed in the response. Most Compute resources support
two types of filter expressions: expressions that support regular expressions and expressions that
follow API improvement proposal AIP-160. These two types of filter expressions cannot be mixed in
one request. If you want to use AIP-160, your expression must specify the field name, an operator,
and the value that you want to use for filtering. The value must be a string, a number, or a
boolean. The operator must be either `=`, `!=`, `>`, `<`, `<=`, `>=` or `:`. For example, if you
are filtering Compute Engine instances, you can exclude instances named `example-instance` by
specifying `name != example-instance`. The `:*` comparison can be used to test whether a key has
been defined. For example, to find all objects with `owner` label use: ``` labels.owner:* ``` You
can also filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
to include instances only if they are not scheduled for automatic restarts. You can use filtering
on nested fields to filter based on resource labels. To filter on multiple expressions, provide
each separate expression within parentheses. For example: ``` (scheduling.automaticRestart = true)
(cpuPlatform = "Intel Skylake") ``` By default, each expression is an `AND` expression. However,
you can include `AND` and `OR` expressions explicitly. For example: ``` (cpuPlatform = "Intel
Skylake") OR (cpuPlatform = "Intel Broadwell") AND (scheduling.automaticRestart = true) ``` If you
want to use a regular expression, use the `eq` (equal) or `ne` (not equal) operator against a
single un-parenthesized expression with or without quotes or against multiple parenthesized
expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted literal'`
`fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne "literal")` The
literal value is interpreted as a regular expression using Google RE2 library syntax. The literal
value must match the entire field. For example, to filter for instances that do not end with name
"instance", you would use `name ne .*instance`. You cannot combine constraints on multiple fields
using regular expressions.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of results per page that should be returned. If the number of available results
is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be used to get the
next page of results in subsequent list requests. Acceptable values are `0` to `500`, inclusive.
(Default: `500`) [default: 500] [minimum: 0]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name. You can also sort results in descending order based on the creation
timestamp using `orderBy="creationTimestamp desc"`. This sorts results based on the
`creationTimestamp` field in reverse chronological order (newest result first). Use this to sort
resources like operations so that the newest operation is returned first. Currently, only sorting
by `name` or `creationTimestamp desc` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a previous list
request to get the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code DeploymentManager deploymentmanager = new DeploymentManager(...);}
* {@code DeploymentManager.Operations.List request = deploymentmanager.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets information about a specific operation.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param operation The name of the operation for this request.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String operation) throws java.io.IOException {
Get result = new Get(project, operation);
initialize(result);
return result;
}
public class Get extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/operations/{operation}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Gets information about a specific operation.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param operation The name of the operation for this request.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String operation) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.Operation.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.operation = com.google.api.client.util.Preconditions.checkNotNull(operation, "Required parameter operation must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Get setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the operation for this request. */
@com.google.api.client.util.Key
private java.lang.String operation;
/** The name of the operation for this request.
*/
public java.lang.String getOperation() {
return operation;
}
/** The name of the operation for this request. */
public Get setOperation(java.lang.String operation) {
this.operation = operation;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Get setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all operations for a project.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/operations";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Lists all operations for a project.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @since 1.13
*/
protected List(java.lang.String project) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.OperationsListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters resources listed in the response. Most Compute resources support
two types of filter expressions: expressions that support regular expressions and expressions that
follow API improvement proposal AIP-160. These two types of filter expressions cannot be mixed in
one request. If you want to use AIP-160, your expression must specify the field name, an operator,
and the value that you want to use for filtering. The value must be a string, a number, or a
boolean. The operator must be either `=`, `!=`, `>`, `<`, `<=`, `>=` or `:`. For example, if you
are filtering Compute Engine instances, you can exclude instances named `example-instance` by
specifying `name != example-instance`. The `:*` comparison can be used to test whether a key has
been defined. For example, to find all objects with `owner` label use: ``` labels.owner:* ``` You
can also filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
to include instances only if they are not scheduled for automatic restarts. You can use filtering
on nested fields to filter based on resource labels. To filter on multiple expressions, provide
each separate expression within parentheses. For example: ``` (scheduling.automaticRestart = true)
(cpuPlatform = "Intel Skylake") ``` By default, each expression is an `AND` expression. However,
you can include `AND` and `OR` expressions explicitly. For example: ``` (cpuPlatform = "Intel
Skylake") OR (cpuPlatform = "Intel Broadwell") AND (scheduling.automaticRestart = true) ``` If you
want to use a regular expression, use the `eq` (equal) or `ne` (not equal) operator against a
single un-parenthesized expression with or without quotes or against multiple parenthesized
expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted literal'`
`fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne "literal")` The
literal value is interpreted as a regular expression using Google RE2 library syntax. The literal
value must match the entire field. For example, to filter for instances that do not end with name
"instance", you would use `name ne .*instance`. You cannot combine constraints on multiple fields
using regular expressions.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of results per page that should be returned. If the number of available results
is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be used to get the
next page of results in subsequent list requests. Acceptable values are `0` to `500`, inclusive.
(Default: `500`) [default: 500] [minimum: 0]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name. You can also sort results in descending order based on the creation
timestamp using `orderBy="creationTimestamp desc"`. This sorts results based on the
`creationTimestamp` field in reverse chronological order (newest result first). Use this to sort
resources like operations so that the newest operation is returned first. Currently, only sorting
by `name` or `creationTimestamp desc` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a previous list
request to get the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Resources collection.
*
* The typical use is:
*
* {@code DeploymentManager deploymentmanager = new DeploymentManager(...);}
* {@code DeploymentManager.Resources.List request = deploymentmanager.resources().list(parameters ...)}
*
*
* @return the resource collection
*/
public Resources resources() {
return new Resources();
}
/**
* The "resources" collection of methods.
*/
public class Resources {
/**
* Gets information about a single resource.
*
* Create a request for the method "resources.get".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param resource The name of the resource for this request.
* @return the request
*/
public Get get(java.lang.String project, java.lang.String deployment, java.lang.String resource) throws java.io.IOException {
Get result = new Get(project, deployment, resource);
initialize(result);
return result;
}
public class Get extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}/resources/{resource}";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Gets information about a single resource.
*
* Create a request for the method "resources.get".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @param resource The name of the resource for this request.
* @since 1.13
*/
protected Get(java.lang.String project, java.lang.String deployment, java.lang.String resource) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.Resource.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public Get setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public Get setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
/** The name of the resource for this request. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** The name of the resource for this request.
*/
public java.lang.String getResource() {
return resource;
}
/** The name of the resource for this request. */
public Get setResource(java.lang.String resource) {
this.resource = resource;
return this;
}
@com.google.api.client.util.Key("header.bypassBillingFilter")
private java.lang.Boolean headerBypassBillingFilter;
/**
*/
public java.lang.Boolean getHeaderBypassBillingFilter() {
return headerBypassBillingFilter;
}
public Get setHeaderBypassBillingFilter(java.lang.Boolean headerBypassBillingFilter) {
this.headerBypassBillingFilter = headerBypassBillingFilter;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all resources in a given deployment.
*
* Create a request for the method "resources.list".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @return the request
*/
public List list(java.lang.String project, java.lang.String deployment) throws java.io.IOException {
List result = new List(project, deployment);
initialize(result);
return result;
}
public class List extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/deployments/{deployment}/resources";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
private final java.util.regex.Pattern DEPLOYMENT_PATTERN =
java.util.regex.Pattern.compile("[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
/**
* Lists all resources in a given deployment.
*
* Create a request for the method "resources.list".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @param deployment The name of the deployment for this request.
* @since 1.13
*/
protected List(java.lang.String project, java.lang.String deployment) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.ResourcesListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.deployment = com.google.api.client.util.Preconditions.checkNotNull(deployment, "Required parameter deployment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/** The name of the deployment for this request. */
@com.google.api.client.util.Key
private java.lang.String deployment;
/** The name of the deployment for this request.
*/
public java.lang.String getDeployment() {
return deployment;
}
/** The name of the deployment for this request. */
public List setDeployment(java.lang.String deployment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DEPLOYMENT_PATTERN.matcher(deployment).matches(),
"Parameter deployment must conform to the pattern " +
"[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?");
}
this.deployment = deployment;
return this;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters resources listed in the response. Most Compute resources support
two types of filter expressions: expressions that support regular expressions and expressions that
follow API improvement proposal AIP-160. These two types of filter expressions cannot be mixed in
one request. If you want to use AIP-160, your expression must specify the field name, an operator,
and the value that you want to use for filtering. The value must be a string, a number, or a
boolean. The operator must be either `=`, `!=`, `>`, `<`, `<=`, `>=` or `:`. For example, if you
are filtering Compute Engine instances, you can exclude instances named `example-instance` by
specifying `name != example-instance`. The `:*` comparison can be used to test whether a key has
been defined. For example, to find all objects with `owner` label use: ``` labels.owner:* ``` You
can also filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
to include instances only if they are not scheduled for automatic restarts. You can use filtering
on nested fields to filter based on resource labels. To filter on multiple expressions, provide
each separate expression within parentheses. For example: ``` (scheduling.automaticRestart = true)
(cpuPlatform = "Intel Skylake") ``` By default, each expression is an `AND` expression. However,
you can include `AND` and `OR` expressions explicitly. For example: ``` (cpuPlatform = "Intel
Skylake") OR (cpuPlatform = "Intel Broadwell") AND (scheduling.automaticRestart = true) ``` If you
want to use a regular expression, use the `eq` (equal) or `ne` (not equal) operator against a
single un-parenthesized expression with or without quotes or against multiple parenthesized
expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted literal'`
`fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne "literal")` The
literal value is interpreted as a regular expression using Google RE2 library syntax. The literal
value must match the entire field. For example, to filter for instances that do not end with name
"instance", you would use `name ne .*instance`. You cannot combine constraints on multiple fields
using regular expressions.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of results per page that should be returned. If the number of available results
is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be used to get the
next page of results in subsequent list requests. Acceptable values are `0` to `500`, inclusive.
(Default: `500`) [default: 500] [minimum: 0]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name. You can also sort results in descending order based on the creation
timestamp using `orderBy="creationTimestamp desc"`. This sorts results based on the
`creationTimestamp` field in reverse chronological order (newest result first). Use this to sort
resources like operations so that the newest operation is returned first. Currently, only sorting
by `name` or `creationTimestamp desc` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a previous list
request to get the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Types collection.
*
* The typical use is:
*
* {@code DeploymentManager deploymentmanager = new DeploymentManager(...);}
* {@code DeploymentManager.Types.List request = deploymentmanager.types().list(parameters ...)}
*
*
* @return the resource collection
*/
public Types types() {
return new Types();
}
/**
* The "types" collection of methods.
*/
public class Types {
/**
* Lists all resource types for Deployment Manager.
*
* Create a request for the method "types.list".
*
* This request holds the parameters needed by the deploymentmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param project The project ID for this request.
* @return the request
*/
public List list(java.lang.String project) throws java.io.IOException {
List result = new List(project);
initialize(result);
return result;
}
public class List extends DeploymentManagerRequest {
private static final String REST_PATH = "deploymentmanager/v2/projects/{project}/global/types";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
/**
* Lists all resource types for Deployment Manager.
*
* Create a request for the method "types.list".
*
* This request holds the parameters needed by the the deploymentmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param project The project ID for this request.
* @since 1.13
*/
protected List(java.lang.String project) {
super(DeploymentManager.this, "GET", REST_PATH, null, com.google.api.services.deploymentmanager.model.TypesListResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The project ID for this request. */
@com.google.api.client.util.Key
private java.lang.String project;
/** The project ID for this request.
*/
public java.lang.String getProject() {
return project;
}
/** The project ID for this request. */
public List setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"(?:(?:[-a-z0-9]{1,63}\\.)*(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?):)?(?:[0-9]{1,19}|(?:[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?))");
}
this.project = project;
return this;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that filters resources listed in the response. Most Compute resources support
two types of filter expressions: expressions that support regular expressions and expressions that
follow API improvement proposal AIP-160. These two types of filter expressions cannot be mixed in
one request. If you want to use AIP-160, your expression must specify the field name, an operator,
and the value that you want to use for filtering. The value must be a string, a number, or a
boolean. The operator must be either `=`, `!=`, `>`, `<`, `<=`, `>=` or `:`. For example, if you
are filtering Compute Engine instances, you can exclude instances named `example-instance` by
specifying `name != example-instance`. The `:*` comparison can be used to test whether a key has
been defined. For example, to find all objects with `owner` label use: ``` labels.owner:* ``` You
can also filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
to include instances only if they are not scheduled for automatic restarts. You can use filtering
on nested fields to filter based on resource labels. To filter on multiple expressions, provide
each separate expression within parentheses. For example: ``` (scheduling.automaticRestart = true)
(cpuPlatform = "Intel Skylake") ``` By default, each expression is an `AND` expression. However,
you can include `AND` and `OR` expressions explicitly. For example: ``` (cpuPlatform = "Intel
Skylake") OR (cpuPlatform = "Intel Broadwell") AND (scheduling.automaticRestart = true) ``` If you
want to use a regular expression, use the `eq` (equal) or `ne` (not equal) operator against a
single un-parenthesized expression with or without quotes or against multiple parenthesized
expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted literal'`
`fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne "literal")` The
literal value is interpreted as a regular expression using Google RE2 library syntax. The literal
value must match the entire field. For example, to filter for instances that do not end with name
"instance", you would use `name ne .*instance`. You cannot combine constraints on multiple fields
using regular expressions.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that filters resources listed in the response. Most Compute resources
* support two types of filter expressions: expressions that support regular expressions and
* expressions that follow API improvement proposal AIP-160. These two types of filter
* expressions cannot be mixed in one request. If you want to use AIP-160, your expression
* must specify the field name, an operator, and the value that you want to use for filtering.
* The value must be a string, a number, or a boolean. The operator must be either `=`, `!=`,
* `>`, `<`, `<=`, `>=` or `:`. For example, if you are filtering Compute Engine instances,
* you can exclude instances named `example-instance` by specifying `name != example-
* instance`. The `:*` comparison can be used to test whether a key has been defined. For
* example, to find all objects with `owner` label use: ``` labels.owner:* ``` You can also
* filter nested fields. For example, you could specify `scheduling.automaticRestart = false`
* to include instances only if they are not scheduled for automatic restarts. You can use
* filtering on nested fields to filter based on resource labels. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel
* Broadwell") AND (scheduling.automaticRestart = true) ``` If you want to use a regular
* expression, use the `eq` (equal) or `ne` (not equal) operator against a single un-
* parenthesized expression with or without quotes or against multiple parenthesized
* expressions. Examples: `fieldname eq unquoted literal` `fieldname eq 'single quoted
* literal'` `fieldname eq "double quoted literal"` `(fieldname1 eq literal) (fieldname2 ne
* "literal")` The literal value is interpreted as a regular expression using Google RE2
* library syntax. The literal value must match the entire field. For example, to filter for
* instances that do not end with name "instance", you would use `name ne .*instance`. You
* cannot combine constraints on multiple fields using regular expressions.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of results per page that should be returned. If the number of available results
is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be used to get the
next page of results in subsequent list requests. Acceptable values are `0` to `500`, inclusive.
(Default: `500`) [default: 500] [minimum: 0]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* The maximum number of results per page that should be returned. If the number of available
* results is larger than `maxResults`, Compute Engine returns a `nextPageToken` that can be
* used to get the next page of results in subsequent list requests. Acceptable values are `0`
* to `500`, inclusive. (Default: `500`)
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, results are returned in alphanumerical order
based on the resource name. You can also sort results in descending order based on the creation
timestamp using `orderBy="creationTimestamp desc"`. This sorts results based on the
`creationTimestamp` field in reverse chronological order (newest result first). Use this to sort
resources like operations so that the newest operation is returned first. Currently, only sorting
by `name` or `creationTimestamp desc` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, results are returned in alphanumerical
* order based on the resource name. You can also sort results in descending order based on
* the creation timestamp using `orderBy="creationTimestamp desc"`. This sorts results based
* on the `creationTimestamp` field in reverse chronological order (newest result first). Use
* this to sort resources like operations so that the newest operation is returned first.
* Currently, only sorting by `name` or `creationTimestamp desc` is supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a previous list
request to get the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Specifies a page token to use. Set `pageToken` to the `nextPageToken` returned by a
* previous list request to get the next page of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link DeploymentManager}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link DeploymentManager}. */
@Override
public DeploymentManager build() {
return new DeploymentManager(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link DeploymentManagerRequestInitializer}.
*
* @since 1.12
*/
public Builder setDeploymentManagerRequestInitializer(
DeploymentManagerRequestInitializer deploymentmanagerRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(deploymentmanagerRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}