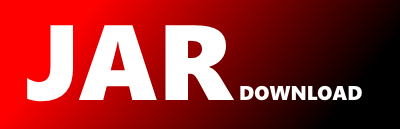
com.google.api.services.developerconnect.v1.model.GitHubEnterpriseConfig Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.developerconnect.v1.model;
/**
* Configuration for connections to an instance of GitHub Enterprise.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Developer Connect API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GitHubEnterpriseConfig extends com.google.api.client.json.GenericJson {
/**
* Optional. ID of the GitHub App created from the manifest.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long appId;
/**
* Optional. ID of the installation of the GitHub App.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long appInstallationId;
/**
* Output only. The URL-friendly name of the GitHub App.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String appSlug;
/**
* Required. The URI of the GitHub Enterprise host this connection is for.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hostUri;
/**
* Output only. The URI to navigate to in order to manage the installation associated with this
* GitHubEnterpriseConfig.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String installationUri;
/**
* Optional. SecretManager resource containing the private key of the GitHub App, formatted as
* `projects/secrets/versions`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String privateKeySecretVersion;
/**
* Output only. GitHub Enterprise version installed at the host_uri.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serverVersion;
/**
* Optional. Configuration for using Service Directory to privately connect to a GitHub Enterprise
* server. This should only be set if the GitHub Enterprise server is hosted on-premises and not
* reachable by public internet. If this field is left empty, calls to the GitHub Enterprise
* server will be made over the public internet.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ServiceDirectoryConfig serviceDirectoryConfig;
/**
* Optional. SSL certificate to use for requests to GitHub Enterprise.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sslCaCertificate;
/**
* Optional. SecretManager resource containing the webhook secret of the GitHub App, formatted as
* `projects/secrets/versions`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String webhookSecretSecretVersion;
/**
* Optional. ID of the GitHub App created from the manifest.
* @return value or {@code null} for none
*/
public java.lang.Long getAppId() {
return appId;
}
/**
* Optional. ID of the GitHub App created from the manifest.
* @param appId appId or {@code null} for none
*/
public GitHubEnterpriseConfig setAppId(java.lang.Long appId) {
this.appId = appId;
return this;
}
/**
* Optional. ID of the installation of the GitHub App.
* @return value or {@code null} for none
*/
public java.lang.Long getAppInstallationId() {
return appInstallationId;
}
/**
* Optional. ID of the installation of the GitHub App.
* @param appInstallationId appInstallationId or {@code null} for none
*/
public GitHubEnterpriseConfig setAppInstallationId(java.lang.Long appInstallationId) {
this.appInstallationId = appInstallationId;
return this;
}
/**
* Output only. The URL-friendly name of the GitHub App.
* @return value or {@code null} for none
*/
public java.lang.String getAppSlug() {
return appSlug;
}
/**
* Output only. The URL-friendly name of the GitHub App.
* @param appSlug appSlug or {@code null} for none
*/
public GitHubEnterpriseConfig setAppSlug(java.lang.String appSlug) {
this.appSlug = appSlug;
return this;
}
/**
* Required. The URI of the GitHub Enterprise host this connection is for.
* @return value or {@code null} for none
*/
public java.lang.String getHostUri() {
return hostUri;
}
/**
* Required. The URI of the GitHub Enterprise host this connection is for.
* @param hostUri hostUri or {@code null} for none
*/
public GitHubEnterpriseConfig setHostUri(java.lang.String hostUri) {
this.hostUri = hostUri;
return this;
}
/**
* Output only. The URI to navigate to in order to manage the installation associated with this
* GitHubEnterpriseConfig.
* @return value or {@code null} for none
*/
public java.lang.String getInstallationUri() {
return installationUri;
}
/**
* Output only. The URI to navigate to in order to manage the installation associated with this
* GitHubEnterpriseConfig.
* @param installationUri installationUri or {@code null} for none
*/
public GitHubEnterpriseConfig setInstallationUri(java.lang.String installationUri) {
this.installationUri = installationUri;
return this;
}
/**
* Optional. SecretManager resource containing the private key of the GitHub App, formatted as
* `projects/secrets/versions`.
* @return value or {@code null} for none
*/
public java.lang.String getPrivateKeySecretVersion() {
return privateKeySecretVersion;
}
/**
* Optional. SecretManager resource containing the private key of the GitHub App, formatted as
* `projects/secrets/versions`.
* @param privateKeySecretVersion privateKeySecretVersion or {@code null} for none
*/
public GitHubEnterpriseConfig setPrivateKeySecretVersion(java.lang.String privateKeySecretVersion) {
this.privateKeySecretVersion = privateKeySecretVersion;
return this;
}
/**
* Output only. GitHub Enterprise version installed at the host_uri.
* @return value or {@code null} for none
*/
public java.lang.String getServerVersion() {
return serverVersion;
}
/**
* Output only. GitHub Enterprise version installed at the host_uri.
* @param serverVersion serverVersion or {@code null} for none
*/
public GitHubEnterpriseConfig setServerVersion(java.lang.String serverVersion) {
this.serverVersion = serverVersion;
return this;
}
/**
* Optional. Configuration for using Service Directory to privately connect to a GitHub Enterprise
* server. This should only be set if the GitHub Enterprise server is hosted on-premises and not
* reachable by public internet. If this field is left empty, calls to the GitHub Enterprise
* server will be made over the public internet.
* @return value or {@code null} for none
*/
public ServiceDirectoryConfig getServiceDirectoryConfig() {
return serviceDirectoryConfig;
}
/**
* Optional. Configuration for using Service Directory to privately connect to a GitHub Enterprise
* server. This should only be set if the GitHub Enterprise server is hosted on-premises and not
* reachable by public internet. If this field is left empty, calls to the GitHub Enterprise
* server will be made over the public internet.
* @param serviceDirectoryConfig serviceDirectoryConfig or {@code null} for none
*/
public GitHubEnterpriseConfig setServiceDirectoryConfig(ServiceDirectoryConfig serviceDirectoryConfig) {
this.serviceDirectoryConfig = serviceDirectoryConfig;
return this;
}
/**
* Optional. SSL certificate to use for requests to GitHub Enterprise.
* @return value or {@code null} for none
*/
public java.lang.String getSslCaCertificate() {
return sslCaCertificate;
}
/**
* Optional. SSL certificate to use for requests to GitHub Enterprise.
* @param sslCaCertificate sslCaCertificate or {@code null} for none
*/
public GitHubEnterpriseConfig setSslCaCertificate(java.lang.String sslCaCertificate) {
this.sslCaCertificate = sslCaCertificate;
return this;
}
/**
* Optional. SecretManager resource containing the webhook secret of the GitHub App, formatted as
* `projects/secrets/versions`.
* @return value or {@code null} for none
*/
public java.lang.String getWebhookSecretSecretVersion() {
return webhookSecretSecretVersion;
}
/**
* Optional. SecretManager resource containing the webhook secret of the GitHub App, formatted as
* `projects/secrets/versions`.
* @param webhookSecretSecretVersion webhookSecretSecretVersion or {@code null} for none
*/
public GitHubEnterpriseConfig setWebhookSecretSecretVersion(java.lang.String webhookSecretSecretVersion) {
this.webhookSecretSecretVersion = webhookSecretSecretVersion;
return this;
}
@Override
public GitHubEnterpriseConfig set(String fieldName, Object value) {
return (GitHubEnterpriseConfig) super.set(fieldName, value);
}
@Override
public GitHubEnterpriseConfig clone() {
return (GitHubEnterpriseConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy