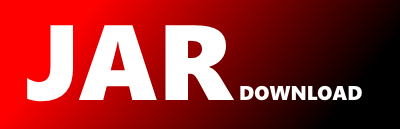
com.google.api.services.dfareporting.Dfareporting Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dfareporting;
/**
* Service definition for Dfareporting (v4).
*
*
* Build applications to efficiently manage large or complex trafficking, reporting, and attribution workflows for Campaign Manager 360.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link DfareportingRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Dfareporting extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Campaign Manager 360 API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://dfareporting.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://dfareporting.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "dfareporting/v4/";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Dfareporting(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Dfareporting(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the AccountActiveAdSummaries collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.AccountActiveAdSummaries.List request = dfareporting.accountActiveAdSummaries().list(parameters ...)}
*
*
* @return the resource collection
*/
public AccountActiveAdSummaries accountActiveAdSummaries() {
return new AccountActiveAdSummaries();
}
/**
* The "accountActiveAdSummaries" collection of methods.
*/
public class AccountActiveAdSummaries {
/**
* Gets the account's active ad summary by account ID.
*
* Create a request for the method "accountActiveAdSummaries.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param summaryAccountId Account ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long summaryAccountId) throws java.io.IOException {
Get result = new Get(profileId, summaryAccountId);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountActiveAdSummaries/{+summaryAccountId}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern SUMMARY_ACCOUNT_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets the account's active ad summary by account ID.
*
* Create a request for the method "accountActiveAdSummaries.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param summaryAccountId Account ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long summaryAccountId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountActiveAdSummary.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.summaryAccountId = com.google.api.client.util.Preconditions.checkNotNull(summaryAccountId, "Required parameter summaryAccountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Account ID. */
@com.google.api.client.util.Key
private java.lang.Long summaryAccountId;
/** Account ID.
*/
public java.lang.Long getSummaryAccountId() {
return summaryAccountId;
}
/** Account ID. */
public Get setSummaryAccountId(java.lang.Long summaryAccountId) {
this.summaryAccountId = summaryAccountId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AccountPermissionGroups collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.AccountPermissionGroups.List request = dfareporting.accountPermissionGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public AccountPermissionGroups accountPermissionGroups() {
return new AccountPermissionGroups();
}
/**
* The "accountPermissionGroups" collection of methods.
*/
public class AccountPermissionGroups {
/**
* Gets one account permission group by ID.
*
* Create a request for the method "accountPermissionGroups.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Account permission group ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountPermissionGroups/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one account permission group by ID.
*
* Create a request for the method "accountPermissionGroups.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Account permission group ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountPermissionGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Account permission group ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Account permission group ID.
*/
public java.lang.Long getId() {
return id;
}
/** Account permission group ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the list of account permission groups.
*
* Create a request for the method "accountPermissionGroups.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountPermissionGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves the list of account permission groups.
*
* Create a request for the method "accountPermissionGroups.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountPermissionGroupsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AccountPermissions collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.AccountPermissions.List request = dfareporting.accountPermissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public AccountPermissions accountPermissions() {
return new AccountPermissions();
}
/**
* The "accountPermissions" collection of methods.
*/
public class AccountPermissions {
/**
* Gets one account permission by ID.
*
* Create a request for the method "accountPermissions.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Account permission ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountPermissions/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one account permission by ID.
*
* Create a request for the method "accountPermissions.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Account permission ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountPermission.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Account permission ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Account permission ID.
*/
public java.lang.Long getId() {
return id;
}
/** Account permission ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the list of account permissions.
*
* Create a request for the method "accountPermissions.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountPermissions";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves the list of account permissions.
*
* Create a request for the method "accountPermissions.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountPermissionsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AccountUserProfiles collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.AccountUserProfiles.List request = dfareporting.accountUserProfiles().list(parameters ...)}
*
*
* @return the resource collection
*/
public AccountUserProfiles accountUserProfiles() {
return new AccountUserProfiles();
}
/**
* The "accountUserProfiles" collection of methods.
*/
public class AccountUserProfiles {
/**
* Gets one account user profile by ID.
*
* Create a request for the method "accountUserProfiles.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id User profile ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{profileId}/accountUserProfiles/{+id}";
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one account user profile by ID.
*
* Create a request for the method "accountUserProfiles.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id User profile ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountUserProfile.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** User profile ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** User profile ID.
*/
public java.lang.Long getId() {
return id;
}
/** User profile ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new account user profile.
*
* Create a request for the method "accountUserProfiles.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AccountUserProfile}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.AccountUserProfile content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountUserProfiles";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new account user profile.
*
* Create a request for the method "accountUserProfiles.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AccountUserProfile}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.AccountUserProfile content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.AccountUserProfile.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of account user profiles, possibly filtered. This method supports paging.
*
* Create a request for the method "accountUserProfiles.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountUserProfiles";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of account user profiles, possibly filtered. This method supports paging.
*
* Create a request for the method "accountUserProfiles.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountUserProfilesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only active user profiles. */
@com.google.api.client.util.Key
private java.lang.Boolean active;
/** Select only active user profiles.
*/
public java.lang.Boolean getActive() {
return active;
}
/** Select only active user profiles. */
public List setActive(java.lang.Boolean active) {
this.active = active;
return this;
}
/** Select only user profiles with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only user profiles with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only user profiles with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for objects by name, ID or email. Wildcards (*) are allowed. For example,
* "user profile*2015" will return objects with names like "user profile June 2015", "user
* profile April 2015", or simply "user profile 2015". Most of the searches also add wildcards
* implicitly at the start and the end of the search string. For example, a search string of
* "user profile" will match objects with name "my user profile", "user profile 2015", or
* simply "user profile".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name, ID or email. Wildcards (*) are allowed. For example, "user
profile*2015" will return objects with names like "user profile June 2015", "user profile April
2015", or simply "user profile 2015". Most of the searches also add wildcards implicitly at the
start and the end of the search string. For example, a search string of "user profile" will match
objects with name "my user profile", "user profile 2015", or simply "user profile".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name, ID or email. Wildcards (*) are allowed. For example,
* "user profile*2015" will return objects with names like "user profile June 2015", "user
* profile April 2015", or simply "user profile 2015". Most of the searches also add wildcards
* implicitly at the start and the end of the search string. For example, a search string of
* "user profile" will match objects with name "my user profile", "user profile 2015", or
* simply "user profile".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/** Select only user profiles with the specified subaccount ID. */
@com.google.api.client.util.Key
private java.lang.Long subaccountId;
/** Select only user profiles with the specified subaccount ID.
*/
public java.lang.Long getSubaccountId() {
return subaccountId;
}
/** Select only user profiles with the specified subaccount ID. */
public List setSubaccountId(java.lang.Long subaccountId) {
this.subaccountId = subaccountId;
return this;
}
/** Select only user profiles with the specified user role ID. */
@com.google.api.client.util.Key
private java.lang.Long userRoleId;
/** Select only user profiles with the specified user role ID.
*/
public java.lang.Long getUserRoleId() {
return userRoleId;
}
/** Select only user profiles with the specified user role ID. */
public List setUserRoleId(java.lang.Long userRoleId) {
this.userRoleId = userRoleId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing account user profile. This method supports patch semantics.
*
* Create a request for the method "accountUserProfiles.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. AccountUserProfile ID.
* @param content the {@link com.google.api.services.dfareporting.model.AccountUserProfile}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.AccountUserProfile content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountUserProfiles";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing account user profile. This method supports patch semantics.
*
* Create a request for the method "accountUserProfiles.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. AccountUserProfile ID.
* @param content the {@link com.google.api.services.dfareporting.model.AccountUserProfile}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.AccountUserProfile content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.AccountUserProfile.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. AccountUserProfile ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. AccountUserProfile ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. AccountUserProfile ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing account user profile.
*
* Create a request for the method "accountUserProfiles.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AccountUserProfile}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.AccountUserProfile content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accountUserProfiles";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing account user profile.
*
* Create a request for the method "accountUserProfiles.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AccountUserProfile}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.AccountUserProfile content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.AccountUserProfile.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Accounts collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Accounts.List request = dfareporting.accounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounts accounts() {
return new Accounts();
}
/**
* The "accounts" collection of methods.
*/
public class Accounts {
/**
* Gets one account by ID.
*
* Create a request for the method "accounts.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Account ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accounts/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one account by ID.
*
* Create a request for the method "accounts.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Account ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.Account.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Account ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Account ID.
*/
public java.lang.Long getId() {
return id;
}
/** Account ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the list of accounts, possibly filtered. This method supports paging.
*
* Create a request for the method "accounts.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accounts";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves the list of accounts, possibly filtered. This method supports paging.
*
* Create a request for the method "accounts.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AccountsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/**
* Select only active accounts. Don't set this field to select both active and non-active
* accounts.
*/
@com.google.api.client.util.Key
private java.lang.Boolean active;
/** Select only active accounts. Don't set this field to select both active and non-active accounts.
*/
public java.lang.Boolean getActive() {
return active;
}
/**
* Select only active accounts. Don't set this field to select both active and non-active
* accounts.
*/
public List setActive(java.lang.Boolean active) {
this.active = active;
return this;
}
/** Select only accounts with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only accounts with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only accounts with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "account*2015" will return objects with names like "account June 2015", "account April
* 2015", or simply "account 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "account" will
* match objects with name "my account", "account 2015", or simply "account".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name or ID. Wildcards (*) are allowed. For example, "account*2015"
will return objects with names like "account June 2015", "account April 2015", or simply "account
2015". Most of the searches also add wildcards implicitly at the start and the end of the search
string. For example, a search string of "account" will match objects with name "my account",
"account 2015", or simply "account".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "account*2015" will return objects with names like "account June 2015", "account April
* 2015", or simply "account 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "account" will
* match objects with name "my account", "account 2015", or simply "account".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing account. This method supports patch semantics.
*
* Create a request for the method "accounts.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Account ID.
* @param content the {@link com.google.api.services.dfareporting.model.Account}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Account content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accounts";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing account. This method supports patch semantics.
*
* Create a request for the method "accounts.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Account ID.
* @param content the {@link com.google.api.services.dfareporting.model.Account}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Account content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.Account.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. Account ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. Account ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. Account ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing account.
*
* Create a request for the method "accounts.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Account}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Account content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/accounts";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing account.
*
* Create a request for the method "accounts.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Account}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Account content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.Account.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Ads collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Ads.List request = dfareporting.ads().list(parameters ...)}
*
*
* @return the resource collection
*/
public Ads ads() {
return new Ads();
}
/**
* The "ads" collection of methods.
*/
public class Ads {
/**
* Gets one ad by ID.
*
* Create a request for the method "ads.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Ad ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/ads/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one ad by ID.
*
* Create a request for the method "ads.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Ad ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.Ad.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Ad ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Ad ID.
*/
public java.lang.Long getId() {
return id;
}
/** Ad ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new ad.
*
* Create a request for the method "ads.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Ad}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Ad content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/ads";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new ad.
*
* Create a request for the method "ads.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Ad}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Ad content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.Ad.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of ads, possibly filtered. This method supports paging.
*
* Create a request for the method "ads.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/ads";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of ads, possibly filtered. This method supports paging.
*
* Create a request for the method "ads.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AdsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only active ads. */
@com.google.api.client.util.Key
private java.lang.Boolean active;
/** Select only active ads.
*/
public java.lang.Boolean getActive() {
return active;
}
/** Select only active ads. */
public List setActive(java.lang.Boolean active) {
this.active = active;
return this;
}
/** Select only ads with this advertiser ID. */
@com.google.api.client.util.Key
private java.lang.Long advertiserId;
/** Select only ads with this advertiser ID.
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/** Select only ads with this advertiser ID. */
public List setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/** Select only archived ads. */
@com.google.api.client.util.Key
private java.lang.Boolean archived;
/** Select only archived ads.
*/
public java.lang.Boolean getArchived() {
return archived;
}
/** Select only archived ads. */
public List setArchived(java.lang.Boolean archived) {
this.archived = archived;
return this;
}
/** Select only ads with these audience segment IDs. */
@com.google.api.client.util.Key
private java.util.List audienceSegmentIds;
/** Select only ads with these audience segment IDs.
*/
public java.util.List getAudienceSegmentIds() {
return audienceSegmentIds;
}
/** Select only ads with these audience segment IDs. */
public List setAudienceSegmentIds(java.util.List audienceSegmentIds) {
this.audienceSegmentIds = audienceSegmentIds;
return this;
}
/** Select only ads with these campaign IDs. */
@com.google.api.client.util.Key
private java.util.List campaignIds;
/** Select only ads with these campaign IDs.
*/
public java.util.List getCampaignIds() {
return campaignIds;
}
/** Select only ads with these campaign IDs. */
public List setCampaignIds(java.util.List campaignIds) {
this.campaignIds = campaignIds;
return this;
}
/**
* Select default ads with the specified compatibility. Applicable when type is
* AD_SERVING_DEFAULT_AD. DISPLAY and DISPLAY_INTERSTITIAL refer to rendering either on
* desktop or on mobile devices for regular or interstitial ads, respectively. APP and
* APP_INTERSTITIAL are for rendering in mobile apps. IN_STREAM_VIDEO refers to rendering an
* in-stream video ads developed with the VAST standard.
*/
@com.google.api.client.util.Key
private java.lang.String compatibility;
/** Select default ads with the specified compatibility. Applicable when type is AD_SERVING_DEFAULT_AD.
DISPLAY and DISPLAY_INTERSTITIAL refer to rendering either on desktop or on mobile devices for
regular or interstitial ads, respectively. APP and APP_INTERSTITIAL are for rendering in mobile
apps. IN_STREAM_VIDEO refers to rendering an in-stream video ads developed with the VAST standard.
*/
public java.lang.String getCompatibility() {
return compatibility;
}
/**
* Select default ads with the specified compatibility. Applicable when type is
* AD_SERVING_DEFAULT_AD. DISPLAY and DISPLAY_INTERSTITIAL refer to rendering either on
* desktop or on mobile devices for regular or interstitial ads, respectively. APP and
* APP_INTERSTITIAL are for rendering in mobile apps. IN_STREAM_VIDEO refers to rendering an
* in-stream video ads developed with the VAST standard.
*/
public List setCompatibility(java.lang.String compatibility) {
this.compatibility = compatibility;
return this;
}
/** Select only ads with these creative IDs assigned. */
@com.google.api.client.util.Key
private java.util.List creativeIds;
/** Select only ads with these creative IDs assigned.
*/
public java.util.List getCreativeIds() {
return creativeIds;
}
/** Select only ads with these creative IDs assigned. */
public List setCreativeIds(java.util.List creativeIds) {
this.creativeIds = creativeIds;
return this;
}
/** Select only ads with these creative optimization configuration IDs. */
@com.google.api.client.util.Key
private java.util.List creativeOptimizationConfigurationIds;
/** Select only ads with these creative optimization configuration IDs.
*/
public java.util.List getCreativeOptimizationConfigurationIds() {
return creativeOptimizationConfigurationIds;
}
/** Select only ads with these creative optimization configuration IDs. */
public List setCreativeOptimizationConfigurationIds(java.util.List creativeOptimizationConfigurationIds) {
this.creativeOptimizationConfigurationIds = creativeOptimizationConfigurationIds;
return this;
}
/**
* Select only dynamic click trackers. Applicable when type is AD_SERVING_CLICK_TRACKER. If
* true, select dynamic click trackers. If false, select static click trackers. Leave unset to
* select both.
*/
@com.google.api.client.util.Key
private java.lang.Boolean dynamicClickTracker;
/** Select only dynamic click trackers. Applicable when type is AD_SERVING_CLICK_TRACKER. If true,
select dynamic click trackers. If false, select static click trackers. Leave unset to select both.
*/
public java.lang.Boolean getDynamicClickTracker() {
return dynamicClickTracker;
}
/**
* Select only dynamic click trackers. Applicable when type is AD_SERVING_CLICK_TRACKER. If
* true, select dynamic click trackers. If false, select static click trackers. Leave unset to
* select both.
*/
public List setDynamicClickTracker(java.lang.Boolean dynamicClickTracker) {
this.dynamicClickTracker = dynamicClickTracker;
return this;
}
/** Select only ads with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only ads with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only ads with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Select only ads with these landing page IDs. */
@com.google.api.client.util.Key
private java.util.List landingPageIds;
/** Select only ads with these landing page IDs.
*/
public java.util.List getLandingPageIds() {
return landingPageIds;
}
/** Select only ads with these landing page IDs. */
public List setLandingPageIds(java.util.List landingPageIds) {
this.landingPageIds = landingPageIds;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Select only ads with this event tag override ID. */
@com.google.api.client.util.Key
private java.lang.Long overriddenEventTagId;
/** Select only ads with this event tag override ID.
*/
public java.lang.Long getOverriddenEventTagId() {
return overriddenEventTagId;
}
/** Select only ads with this event tag override ID. */
public List setOverriddenEventTagId(java.lang.Long overriddenEventTagId) {
this.overriddenEventTagId = overriddenEventTagId;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Select only ads with these placement IDs assigned. */
@com.google.api.client.util.Key
private java.util.List placementIds;
/** Select only ads with these placement IDs assigned.
*/
public java.util.List getPlacementIds() {
return placementIds;
}
/** Select only ads with these placement IDs assigned. */
public List setPlacementIds(java.util.List placementIds) {
this.placementIds = placementIds;
return this;
}
/** Select only ads whose list targeting expression use these remarketing list IDs. */
@com.google.api.client.util.Key
private java.util.List remarketingListIds;
/** Select only ads whose list targeting expression use these remarketing list IDs.
*/
public java.util.List getRemarketingListIds() {
return remarketingListIds;
}
/** Select only ads whose list targeting expression use these remarketing list IDs. */
public List setRemarketingListIds(java.util.List remarketingListIds) {
this.remarketingListIds = remarketingListIds;
return this;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "ad*2015" will return objects with names like "ad June 2015", "ad April 2015", or simply
* "ad 2015". Most of the searches also add wildcards implicitly at the start and the end of
* the search string. For example, a search string of "ad" will match objects with name "my
* ad", "ad 2015", or simply "ad".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name or ID. Wildcards (*) are allowed. For example, "ad*2015" will
return objects with names like "ad June 2015", "ad April 2015", or simply "ad 2015". Most of the
searches also add wildcards implicitly at the start and the end of the search string. For example,
a search string of "ad" will match objects with name "my ad", "ad 2015", or simply "ad".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "ad*2015" will return objects with names like "ad June 2015", "ad April 2015", or simply
* "ad 2015". Most of the searches also add wildcards implicitly at the start and the end of
* the search string. For example, a search string of "ad" will match objects with name "my
* ad", "ad 2015", or simply "ad".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Select only ads with these size IDs. */
@com.google.api.client.util.Key
private java.util.List sizeIds;
/** Select only ads with these size IDs.
*/
public java.util.List getSizeIds() {
return sizeIds;
}
/** Select only ads with these size IDs. */
public List setSizeIds(java.util.List sizeIds) {
this.sizeIds = sizeIds;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/** Select only ads that are SSL-compliant. */
@com.google.api.client.util.Key
private java.lang.Boolean sslCompliant;
/** Select only ads that are SSL-compliant.
*/
public java.lang.Boolean getSslCompliant() {
return sslCompliant;
}
/** Select only ads that are SSL-compliant. */
public List setSslCompliant(java.lang.Boolean sslCompliant) {
this.sslCompliant = sslCompliant;
return this;
}
/** Select only ads that require SSL. */
@com.google.api.client.util.Key
private java.lang.Boolean sslRequired;
/** Select only ads that require SSL.
*/
public java.lang.Boolean getSslRequired() {
return sslRequired;
}
/** Select only ads that require SSL. */
public List setSslRequired(java.lang.Boolean sslRequired) {
this.sslRequired = sslRequired;
return this;
}
/** Select only ads with these types. */
@com.google.api.client.util.Key
private java.util.List type;
/** Select only ads with these types.
*/
public java.util.List getType() {
return type;
}
/** Select only ads with these types. */
public List setType(java.util.List type) {
this.type = type;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing ad. This method supports patch semantics.
*
* Create a request for the method "ads.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. RemarketingList ID.
* @param content the {@link com.google.api.services.dfareporting.model.Ad}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Ad content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/ads";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing ad. This method supports patch semantics.
*
* Create a request for the method "ads.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. RemarketingList ID.
* @param content the {@link com.google.api.services.dfareporting.model.Ad}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Ad content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.Ad.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. RemarketingList ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. RemarketingList ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. RemarketingList ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing ad.
*
* Create a request for the method "ads.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Ad}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Ad content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/ads";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing ad.
*
* Create a request for the method "ads.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Ad}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Ad content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.Ad.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AdvertiserGroups collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.AdvertiserGroups.List request = dfareporting.advertiserGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public AdvertiserGroups advertiserGroups() {
return new AdvertiserGroups();
}
/**
* The "advertiserGroups" collection of methods.
*/
public class AdvertiserGroups {
/**
* Deletes an existing advertiser group.
*
* Create a request for the method "advertiserGroups.delete".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Advertiser group ID.
* @return the request
*/
public Delete delete(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(profileId, id);
initialize(result);
return result;
}
public class Delete extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserGroups/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Deletes an existing advertiser group.
*
* Create a request for the method "advertiserGroups.delete".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Advertiser group ID.
* @since 1.13
*/
protected Delete(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "DELETE", REST_PATH, null, Void.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Delete setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Advertiser group ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Advertiser group ID.
*/
public java.lang.Long getId() {
return id;
}
/** Advertiser group ID. */
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets one advertiser group by ID.
*
* Create a request for the method "advertiserGroups.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Advertiser group ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserGroups/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one advertiser group by ID.
*
* Create a request for the method "advertiserGroups.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Advertiser group ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AdvertiserGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Advertiser group ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Advertiser group ID.
*/
public java.lang.Long getId() {
return id;
}
/** Advertiser group ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new advertiser group.
*
* Create a request for the method "advertiserGroups.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AdvertiserGroup}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.AdvertiserGroup content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new advertiser group.
*
* Create a request for the method "advertiserGroups.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AdvertiserGroup}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.AdvertiserGroup content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.AdvertiserGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of advertiser groups, possibly filtered. This method supports paging.
*
* Create a request for the method "advertiserGroups.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of advertiser groups, possibly filtered. This method supports paging.
*
* Create a request for the method "advertiserGroups.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AdvertiserGroupsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only advertiser groups with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only advertiser groups with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only advertiser groups with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "advertiser*2015" will return objects with names like "advertiser group June 2015",
* "advertiser group April 2015", or simply "advertiser group 2015". Most of the searches also
* add wildcards implicitly at the start and the end of the search string. For example, a
* search string of "advertisergroup" will match objects with name "my advertisergroup",
* "advertisergroup 2015", or simply "advertisergroup".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
"advertiser*2015" will return objects with names like "advertiser group June 2015", "advertiser
group April 2015", or simply "advertiser group 2015". Most of the searches also add wildcards
implicitly at the start and the end of the search string. For example, a search string of
"advertisergroup" will match objects with name "my advertisergroup", "advertisergroup 2015", or
simply "advertisergroup".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "advertiser*2015" will return objects with names like "advertiser group June 2015",
* "advertiser group April 2015", or simply "advertiser group 2015". Most of the searches also
* add wildcards implicitly at the start and the end of the search string. For example, a
* search string of "advertisergroup" will match objects with name "my advertisergroup",
* "advertisergroup 2015", or simply "advertisergroup".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing advertiser group. This method supports patch semantics.
*
* Create a request for the method "advertiserGroups.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Advertiser Group ID.
* @param content the {@link com.google.api.services.dfareporting.model.AdvertiserGroup}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.AdvertiserGroup content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing advertiser group. This method supports patch semantics.
*
* Create a request for the method "advertiserGroups.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Advertiser Group ID.
* @param content the {@link com.google.api.services.dfareporting.model.AdvertiserGroup}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.AdvertiserGroup content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.AdvertiserGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. Advertiser Group ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. Advertiser Group ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. Advertiser Group ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing advertiser group.
*
* Create a request for the method "advertiserGroups.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AdvertiserGroup}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.AdvertiserGroup content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing advertiser group.
*
* Create a request for the method "advertiserGroups.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.AdvertiserGroup}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.AdvertiserGroup content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.AdvertiserGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AdvertiserInvoices collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.AdvertiserInvoices.List request = dfareporting.advertiserInvoices().list(parameters ...)}
*
*
* @return the resource collection
*/
public AdvertiserInvoices advertiserInvoices() {
return new AdvertiserInvoices();
}
/**
* The "advertiserInvoices" collection of methods.
*/
public class AdvertiserInvoices {
/**
* Retrieves a list of invoices for a particular issue month. The api only works if the billing
* profile invoice level is set to either advertiser or campaign non-consolidated invoice level.
*
* Create a request for the method "advertiserInvoices.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param advertiserId Advertiser ID of this invoice.
* @return the request
*/
public List list(java.lang.Long profileId, java.lang.Long advertiserId) throws java.io.IOException {
List result = new List(profileId, advertiserId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertisers/{+advertiserId}/invoices";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ADVERTISER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of invoices for a particular issue month. The api only works if the billing
* profile invoice level is set to either advertiser or campaign non-consolidated invoice level.
*
* Create a request for the method "advertiserInvoices.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param advertiserId Advertiser ID of this invoice.
* @since 1.13
*/
protected List(java.lang.Long profileId, java.lang.Long advertiserId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AdvertiserInvoicesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.advertiserId = com.google.api.client.util.Preconditions.checkNotNull(advertiserId, "Required parameter advertiserId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Advertiser ID of this invoice. */
@com.google.api.client.util.Key
private java.lang.Long advertiserId;
/** Advertiser ID of this invoice.
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/** Advertiser ID of this invoice. */
public List setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/** Month for which invoices are needed in the format YYYYMM. Required field */
@com.google.api.client.util.Key
private java.lang.String issueMonth;
/** Month for which invoices are needed in the format YYYYMM. Required field
*/
public java.lang.String getIssueMonth() {
return issueMonth;
}
/** Month for which invoices are needed in the format YYYYMM. Required field */
public List setIssueMonth(java.lang.String issueMonth) {
this.issueMonth = issueMonth;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AdvertiserLandingPages collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.AdvertiserLandingPages.List request = dfareporting.advertiserLandingPages().list(parameters ...)}
*
*
* @return the resource collection
*/
public AdvertiserLandingPages advertiserLandingPages() {
return new AdvertiserLandingPages();
}
/**
* The "advertiserLandingPages" collection of methods.
*/
public class AdvertiserLandingPages {
/**
* Gets one landing page by ID.
*
* Create a request for the method "advertiserLandingPages.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Landing page ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserLandingPages/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one landing page by ID.
*
* Create a request for the method "advertiserLandingPages.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Landing page ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.LandingPage.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Landing page ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Landing page ID.
*/
public java.lang.Long getId() {
return id;
}
/** Landing page ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new landing page.
*
* Create a request for the method "advertiserLandingPages.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.LandingPage}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.LandingPage content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserLandingPages";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new landing page.
*
* Create a request for the method "advertiserLandingPages.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.LandingPage}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.LandingPage content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.LandingPage.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of landing pages.
*
* Create a request for the method "advertiserLandingPages.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserLandingPages";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of landing pages.
*
* Create a request for the method "advertiserLandingPages.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AdvertiserLandingPagesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only landing pages that belong to these advertisers. */
@com.google.api.client.util.Key
private java.util.List advertiserIds;
/** Select only landing pages that belong to these advertisers.
*/
public java.util.List getAdvertiserIds() {
return advertiserIds;
}
/** Select only landing pages that belong to these advertisers. */
public List setAdvertiserIds(java.util.List advertiserIds) {
this.advertiserIds = advertiserIds;
return this;
}
/**
* Select only archived landing pages. Don't set this field to select both archived and non-
* archived landing pages.
*/
@com.google.api.client.util.Key
private java.lang.Boolean archived;
/** Select only archived landing pages. Don't set this field to select both archived and non-archived
landing pages.
*/
public java.lang.Boolean getArchived() {
return archived;
}
/**
* Select only archived landing pages. Don't set this field to select both archived and non-
* archived landing pages.
*/
public List setArchived(java.lang.Boolean archived) {
this.archived = archived;
return this;
}
/** Select only landing pages that are associated with these campaigns. */
@com.google.api.client.util.Key
private java.util.List campaignIds;
/** Select only landing pages that are associated with these campaigns.
*/
public java.util.List getCampaignIds() {
return campaignIds;
}
/** Select only landing pages that are associated with these campaigns. */
public List setCampaignIds(java.util.List campaignIds) {
this.campaignIds = campaignIds;
return this;
}
/** Select only landing pages with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only landing pages with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only landing pages with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for landing pages by name or ID. Wildcards (*) are allowed. For example,
* "landingpage*2017" will return landing pages with names like "landingpage July 2017",
* "landingpage March 2017", or simply "landingpage 2017". Most of the searches also add
* wildcards implicitly at the start and the end of the search string. For example, a search
* string of "landingpage" will match campaigns with name "my landingpage", "landingpage
* 2015", or simply "landingpage".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for landing pages by name or ID. Wildcards (*) are allowed. For example,
"landingpage*2017" will return landing pages with names like "landingpage July 2017", "landingpage
March 2017", or simply "landingpage 2017". Most of the searches also add wildcards implicitly at
the start and the end of the search string. For example, a search string of "landingpage" will
match campaigns with name "my landingpage", "landingpage 2015", or simply "landingpage".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for landing pages by name or ID. Wildcards (*) are allowed. For example,
* "landingpage*2017" will return landing pages with names like "landingpage July 2017",
* "landingpage March 2017", or simply "landingpage 2017". Most of the searches also add
* wildcards implicitly at the start and the end of the search string. For example, a search
* string of "landingpage" will match campaigns with name "my landingpage", "landingpage
* 2015", or simply "landingpage".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/** Select only landing pages that belong to this subaccount. */
@com.google.api.client.util.Key
private java.lang.Long subaccountId;
/** Select only landing pages that belong to this subaccount.
*/
public java.lang.Long getSubaccountId() {
return subaccountId;
}
/** Select only landing pages that belong to this subaccount. */
public List setSubaccountId(java.lang.Long subaccountId) {
this.subaccountId = subaccountId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing landing page. This method supports patch semantics.
*
* Create a request for the method "advertiserLandingPages.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Landing Page ID.
* @param content the {@link com.google.api.services.dfareporting.model.LandingPage}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.LandingPage content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserLandingPages";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing landing page. This method supports patch semantics.
*
* Create a request for the method "advertiserLandingPages.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Landing Page ID.
* @param content the {@link com.google.api.services.dfareporting.model.LandingPage}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.LandingPage content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.LandingPage.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. Landing Page ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. Landing Page ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. Landing Page ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing landing page.
*
* Create a request for the method "advertiserLandingPages.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.LandingPage}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.LandingPage content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertiserLandingPages";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing landing page.
*
* Create a request for the method "advertiserLandingPages.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.LandingPage}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.LandingPage content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.LandingPage.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Advertisers collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Advertisers.List request = dfareporting.advertisers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Advertisers advertisers() {
return new Advertisers();
}
/**
* The "advertisers" collection of methods.
*/
public class Advertisers {
/**
* Gets one advertiser by ID.
*
* Create a request for the method "advertisers.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Advertiser ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertisers/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one advertiser by ID.
*
* Create a request for the method "advertisers.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Advertiser ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.Advertiser.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Advertiser ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Advertiser ID.
*/
public java.lang.Long getId() {
return id;
}
/** Advertiser ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new advertiser.
*
* Create a request for the method "advertisers.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Advertiser}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Advertiser content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertisers";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new advertiser.
*
* Create a request for the method "advertisers.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Advertiser}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Advertiser content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.Advertiser.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of advertisers, possibly filtered. This method supports paging.
*
* Create a request for the method "advertisers.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertisers";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of advertisers, possibly filtered. This method supports paging.
*
* Create a request for the method "advertisers.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.AdvertisersListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only advertisers with these advertiser group IDs. */
@com.google.api.client.util.Key
private java.util.List advertiserGroupIds;
/** Select only advertisers with these advertiser group IDs.
*/
public java.util.List getAdvertiserGroupIds() {
return advertiserGroupIds;
}
/** Select only advertisers with these advertiser group IDs. */
public List setAdvertiserGroupIds(java.util.List advertiserGroupIds) {
this.advertiserGroupIds = advertiserGroupIds;
return this;
}
/** Select only advertisers with these floodlight configuration IDs. */
@com.google.api.client.util.Key
private java.util.List floodlightConfigurationIds;
/** Select only advertisers with these floodlight configuration IDs.
*/
public java.util.List getFloodlightConfigurationIds() {
return floodlightConfigurationIds;
}
/** Select only advertisers with these floodlight configuration IDs. */
public List setFloodlightConfigurationIds(java.util.List floodlightConfigurationIds) {
this.floodlightConfigurationIds = floodlightConfigurationIds;
return this;
}
/** Select only advertisers with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only advertisers with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only advertisers with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Select only advertisers which do not belong to any advertiser group. */
@com.google.api.client.util.Key
private java.lang.Boolean includeAdvertisersWithoutGroupsOnly;
/** Select only advertisers which do not belong to any advertiser group.
*/
public java.lang.Boolean getIncludeAdvertisersWithoutGroupsOnly() {
return includeAdvertisersWithoutGroupsOnly;
}
/** Select only advertisers which do not belong to any advertiser group. */
public List setIncludeAdvertisersWithoutGroupsOnly(java.lang.Boolean includeAdvertisersWithoutGroupsOnly) {
this.includeAdvertisersWithoutGroupsOnly = includeAdvertisersWithoutGroupsOnly;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Select only advertisers which use another advertiser's floodlight configuration. */
@com.google.api.client.util.Key
private java.lang.Boolean onlyParent;
/** Select only advertisers which use another advertiser's floodlight configuration.
*/
public java.lang.Boolean getOnlyParent() {
return onlyParent;
}
/** Select only advertisers which use another advertiser's floodlight configuration. */
public List setOnlyParent(java.lang.Boolean onlyParent) {
this.onlyParent = onlyParent;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "advertiser*2015" will return objects with names like "advertiser June 2015", "advertiser
* April 2015", or simply "advertiser 2015". Most of the searches also add wildcards
* implicitly at the start and the end of the search string. For example, a search string of
* "advertiser" will match objects with name "my advertiser", "advertiser 2015", or simply
* "advertiser" .
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
"advertiser*2015" will return objects with names like "advertiser June 2015", "advertiser April
2015", or simply "advertiser 2015". Most of the searches also add wildcards implicitly at the start
and the end of the search string. For example, a search string of "advertiser" will match objects
with name "my advertiser", "advertiser 2015", or simply "advertiser" .
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "advertiser*2015" will return objects with names like "advertiser June 2015", "advertiser
* April 2015", or simply "advertiser 2015". Most of the searches also add wildcards
* implicitly at the start and the end of the search string. For example, a search string of
* "advertiser" will match objects with name "my advertiser", "advertiser 2015", or simply
* "advertiser" .
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/** Select only advertisers with the specified status. */
@com.google.api.client.util.Key
private java.lang.String status;
/** Select only advertisers with the specified status.
*/
public java.lang.String getStatus() {
return status;
}
/** Select only advertisers with the specified status. */
public List setStatus(java.lang.String status) {
this.status = status;
return this;
}
/** Select only advertisers with these subaccount IDs. */
@com.google.api.client.util.Key
private java.lang.Long subaccountId;
/** Select only advertisers with these subaccount IDs.
*/
public java.lang.Long getSubaccountId() {
return subaccountId;
}
/** Select only advertisers with these subaccount IDs. */
public List setSubaccountId(java.lang.Long subaccountId) {
this.subaccountId = subaccountId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing advertiser. This method supports patch semantics.
*
* Create a request for the method "advertisers.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Advertiser ID.
* @param content the {@link com.google.api.services.dfareporting.model.Advertiser}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Advertiser content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertisers";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing advertiser. This method supports patch semantics.
*
* Create a request for the method "advertisers.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Advertiser ID.
* @param content the {@link com.google.api.services.dfareporting.model.Advertiser}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Advertiser content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.Advertiser.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. Advertiser ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. Advertiser ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. Advertiser ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing advertiser.
*
* Create a request for the method "advertisers.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Advertiser}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Advertiser content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/advertisers";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing advertiser.
*
* Create a request for the method "advertisers.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Advertiser}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Advertiser content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.Advertiser.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BillingAssignments collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.BillingAssignments.List request = dfareporting.billingAssignments().list(parameters ...)}
*
*
* @return the resource collection
*/
public BillingAssignments billingAssignments() {
return new BillingAssignments();
}
/**
* The "billingAssignments" collection of methods.
*/
public class BillingAssignments {
/**
* Inserts a new billing assignment and returns the new assignment. Only one of advertiser_id or
* campaign_id is support per request. If the new assignment has no effect (assigning a campaign to
* the parent advertiser billing profile or assigning an advertiser to the account billing profile),
* no assignment will be returned.
*
* Create a request for the method "billingAssignments.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param billingProfileId Billing profile ID of this billing assignment.
* @param content the {@link com.google.api.services.dfareporting.model.BillingAssignment}
* @return the request
*/
public Insert insert(java.lang.Long profileId, java.lang.Long billingProfileId, com.google.api.services.dfareporting.model.BillingAssignment content) throws java.io.IOException {
Insert result = new Insert(profileId, billingProfileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/billingProfiles/{+billingProfileId}/billingAssignments";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern BILLING_PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new billing assignment and returns the new assignment. Only one of advertiser_id or
* campaign_id is support per request. If the new assignment has no effect (assigning a campaign
* to the parent advertiser billing profile or assigning an advertiser to the account billing
* profile), no assignment will be returned.
*
* Create a request for the method "billingAssignments.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param billingProfileId Billing profile ID of this billing assignment.
* @param content the {@link com.google.api.services.dfareporting.model.BillingAssignment}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, java.lang.Long billingProfileId, com.google.api.services.dfareporting.model.BillingAssignment content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.BillingAssignment.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.billingProfileId = com.google.api.client.util.Preconditions.checkNotNull(billingProfileId, "Required parameter billingProfileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Billing profile ID of this billing assignment. */
@com.google.api.client.util.Key
private java.lang.Long billingProfileId;
/** Billing profile ID of this billing assignment.
*/
public java.lang.Long getBillingProfileId() {
return billingProfileId;
}
/** Billing profile ID of this billing assignment. */
public Insert setBillingProfileId(java.lang.Long billingProfileId) {
this.billingProfileId = billingProfileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of billing assignments.
*
* Create a request for the method "billingAssignments.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param billingProfileId Billing profile ID of this billing assignment.
* @return the request
*/
public List list(java.lang.Long profileId, java.lang.Long billingProfileId) throws java.io.IOException {
List result = new List(profileId, billingProfileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/billingProfiles/{+billingProfileId}/billingAssignments";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern BILLING_PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of billing assignments.
*
* Create a request for the method "billingAssignments.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param billingProfileId Billing profile ID of this billing assignment.
* @since 1.13
*/
protected List(java.lang.Long profileId, java.lang.Long billingProfileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.BillingAssignmentsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.billingProfileId = com.google.api.client.util.Preconditions.checkNotNull(billingProfileId, "Required parameter billingProfileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Billing profile ID of this billing assignment. */
@com.google.api.client.util.Key
private java.lang.Long billingProfileId;
/** Billing profile ID of this billing assignment.
*/
public java.lang.Long getBillingProfileId() {
return billingProfileId;
}
/** Billing profile ID of this billing assignment. */
public List setBillingProfileId(java.lang.Long billingProfileId) {
this.billingProfileId = billingProfileId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BillingProfiles collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.BillingProfiles.List request = dfareporting.billingProfiles().list(parameters ...)}
*
*
* @return the resource collection
*/
public BillingProfiles billingProfiles() {
return new BillingProfiles();
}
/**
* The "billingProfiles" collection of methods.
*/
public class BillingProfiles {
/**
* Gets one billing profile by ID.
*
* Create a request for the method "billingProfiles.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Billing Profile ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/billingProfiles/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one billing profile by ID.
*
* Create a request for the method "billingProfiles.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Billing Profile ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.BillingProfile.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Billing Profile ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Billing Profile ID.
*/
public java.lang.Long getId() {
return id;
}
/** Billing Profile ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a list of billing profiles, possibly filtered. This method supports paging.
*
* Create a request for the method "billingProfiles.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/billingProfiles";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of billing profiles, possibly filtered. This method supports paging.
*
* Create a request for the method "billingProfiles.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.BillingProfilesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only billing profile with currency. */
@com.google.api.client.util.Key("currency_code")
private java.lang.String currencyCode;
/** Select only billing profile with currency.
*/
public java.lang.String getCurrencyCode() {
return currencyCode;
}
/** Select only billing profile with currency. */
public List setCurrencyCode(java.lang.String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/** Select only billing profile with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only billing profile with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only billing profile with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Allows searching for billing profiles by name. Wildcards (*) are allowed. For example,
* "profile*2020" will return objects with names like "profile June 2020", "profile April
* 2020", or simply "profile 2020". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "profile" will
* match objects with name "my profile", "profile 2021", or simply "profile".
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Allows searching for billing profiles by name. Wildcards (*) are allowed. For example,
"profile*2020" will return objects with names like "profile June 2020", "profile April 2020", or
simply "profile 2020". Most of the searches also add wildcards implicitly at the start and the end
of the search string. For example, a search string of "profile" will match objects with name "my
profile", "profile 2021", or simply "profile".
*/
public java.lang.String getName() {
return name;
}
/**
* Allows searching for billing profiles by name. Wildcards (*) are allowed. For example,
* "profile*2020" will return objects with names like "profile June 2020", "profile April
* 2020", or simply "profile 2020". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "profile" will
* match objects with name "my profile", "profile 2021", or simply "profile".
*/
public List setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Select only billing profile which is suggested for the currency_code & subaccount_id using
* the Billing Suggestion API.
*/
@com.google.api.client.util.Key
private java.lang.Boolean onlySuggestion;
/** Select only billing profile which is suggested for the currency_code & subaccount_id using the
Billing Suggestion API.
*/
public java.lang.Boolean getOnlySuggestion() {
return onlySuggestion;
}
/**
* Select only billing profile which is suggested for the currency_code & subaccount_id using
* the Billing Suggestion API.
*/
public List setOnlySuggestion(java.lang.Boolean onlySuggestion) {
this.onlySuggestion = onlySuggestion;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/** Select only billing profile with the specified status. */
@com.google.api.client.util.Key
private java.util.List status;
/** Select only billing profile with the specified status.
*/
public java.util.List getStatus() {
return status;
}
/** Select only billing profile with the specified status. */
public List setStatus(java.util.List status) {
this.status = status;
return this;
}
/**
* Select only billing profile with the specified subaccount.When only_suggestion is true,
* only a single subaccount_id is supported.
*/
@com.google.api.client.util.Key
private java.util.List subaccountIds;
/** Select only billing profile with the specified subaccount.When only_suggestion is true, only a
single subaccount_id is supported.
*/
public java.util.List getSubaccountIds() {
return subaccountIds;
}
/**
* Select only billing profile with the specified subaccount.When only_suggestion is true,
* only a single subaccount_id is supported.
*/
public List setSubaccountIds(java.util.List subaccountIds) {
this.subaccountIds = subaccountIds;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing billing profile.
*
* Create a request for the method "billingProfiles.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.BillingProfile}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.BillingProfile content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/billingProfiles";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing billing profile.
*
* Create a request for the method "billingProfiles.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.BillingProfile}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.BillingProfile content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.BillingProfile.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BillingRates collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.BillingRates.List request = dfareporting.billingRates().list(parameters ...)}
*
*
* @return the resource collection
*/
public BillingRates billingRates() {
return new BillingRates();
}
/**
* The "billingRates" collection of methods.
*/
public class BillingRates {
/**
* Retrieves a list of billing rates. This method supports paging.
*
* Create a request for the method "billingRates.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param billingProfileId Billing profile ID of this billing rate.
* @return the request
*/
public List list(java.lang.Long profileId, java.lang.Long billingProfileId) throws java.io.IOException {
List result = new List(profileId, billingProfileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/billingProfiles/{+billingProfileId}/billingRates";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern BILLING_PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of billing rates. This method supports paging.
*
* Create a request for the method "billingRates.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param billingProfileId Billing profile ID of this billing rate.
* @since 1.13
*/
protected List(java.lang.Long profileId, java.lang.Long billingProfileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.BillingRatesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.billingProfileId = com.google.api.client.util.Preconditions.checkNotNull(billingProfileId, "Required parameter billingProfileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Billing profile ID of this billing rate. */
@com.google.api.client.util.Key
private java.lang.Long billingProfileId;
/** Billing profile ID of this billing rate.
*/
public java.lang.Long getBillingProfileId() {
return billingProfileId;
}
/** Billing profile ID of this billing rate. */
public List setBillingProfileId(java.lang.Long billingProfileId) {
this.billingProfileId = billingProfileId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Browsers collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Browsers.List request = dfareporting.browsers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Browsers browsers() {
return new Browsers();
}
/**
* The "browsers" collection of methods.
*/
public class Browsers {
/**
* Retrieves a list of browsers.
*
* Create a request for the method "browsers.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/browsers";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of browsers.
*
* Create a request for the method "browsers.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.BrowsersListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CampaignCreativeAssociations collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.CampaignCreativeAssociations.List request = dfareporting.campaignCreativeAssociations().list(parameters ...)}
*
*
* @return the resource collection
*/
public CampaignCreativeAssociations campaignCreativeAssociations() {
return new CampaignCreativeAssociations();
}
/**
* The "campaignCreativeAssociations" collection of methods.
*/
public class CampaignCreativeAssociations {
/**
* Associates a creative with the specified campaign. This method creates a default ad with
* dimensions matching the creative in the campaign if such a default ad does not exist already.
*
* Create a request for the method "campaignCreativeAssociations.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param campaignId Campaign ID in this association.
* @param content the {@link com.google.api.services.dfareporting.model.CampaignCreativeAssociation}
* @return the request
*/
public Insert insert(java.lang.Long profileId, java.lang.Long campaignId, com.google.api.services.dfareporting.model.CampaignCreativeAssociation content) throws java.io.IOException {
Insert result = new Insert(profileId, campaignId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/campaigns/{+campaignId}/campaignCreativeAssociations";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CAMPAIGN_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Associates a creative with the specified campaign. This method creates a default ad with
* dimensions matching the creative in the campaign if such a default ad does not exist already.
*
* Create a request for the method "campaignCreativeAssociations.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param campaignId Campaign ID in this association.
* @param content the {@link com.google.api.services.dfareporting.model.CampaignCreativeAssociation}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, java.lang.Long campaignId, com.google.api.services.dfareporting.model.CampaignCreativeAssociation content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.CampaignCreativeAssociation.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.campaignId = com.google.api.client.util.Preconditions.checkNotNull(campaignId, "Required parameter campaignId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Campaign ID in this association. */
@com.google.api.client.util.Key
private java.lang.Long campaignId;
/** Campaign ID in this association.
*/
public java.lang.Long getCampaignId() {
return campaignId;
}
/** Campaign ID in this association. */
public Insert setCampaignId(java.lang.Long campaignId) {
this.campaignId = campaignId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the list of creative IDs associated with the specified campaign. This method supports
* paging.
*
* Create a request for the method "campaignCreativeAssociations.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param campaignId Campaign ID in this association.
* @return the request
*/
public List list(java.lang.Long profileId, java.lang.Long campaignId) throws java.io.IOException {
List result = new List(profileId, campaignId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/campaigns/{+campaignId}/campaignCreativeAssociations";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CAMPAIGN_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves the list of creative IDs associated with the specified campaign. This method supports
* paging.
*
* Create a request for the method "campaignCreativeAssociations.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param campaignId Campaign ID in this association.
* @since 1.13
*/
protected List(java.lang.Long profileId, java.lang.Long campaignId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CampaignCreativeAssociationsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.campaignId = com.google.api.client.util.Preconditions.checkNotNull(campaignId, "Required parameter campaignId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Campaign ID in this association. */
@com.google.api.client.util.Key
private java.lang.Long campaignId;
/** Campaign ID in this association.
*/
public java.lang.Long getCampaignId() {
return campaignId;
}
/** Campaign ID in this association. */
public List setCampaignId(java.lang.Long campaignId) {
this.campaignId = campaignId;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Campaigns collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Campaigns.List request = dfareporting.campaigns().list(parameters ...)}
*
*
* @return the resource collection
*/
public Campaigns campaigns() {
return new Campaigns();
}
/**
* The "campaigns" collection of methods.
*/
public class Campaigns {
/**
* Gets one campaign by ID.
*
* Create a request for the method "campaigns.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Campaign ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/campaigns/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one campaign by ID.
*
* Create a request for the method "campaigns.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Campaign ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.Campaign.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Campaign ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Campaign ID.
*/
public java.lang.Long getId() {
return id;
}
/** Campaign ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new campaign.
*
* Create a request for the method "campaigns.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Campaign}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Campaign content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/campaigns";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new campaign.
*
* Create a request for the method "campaigns.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Campaign}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Campaign content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.Campaign.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of campaigns, possibly filtered. This method supports paging.
*
* Create a request for the method "campaigns.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/campaigns";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of campaigns, possibly filtered. This method supports paging.
*
* Create a request for the method "campaigns.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CampaignsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only campaigns whose advertisers belong to these advertiser groups. */
@com.google.api.client.util.Key
private java.util.List advertiserGroupIds;
/** Select only campaigns whose advertisers belong to these advertiser groups.
*/
public java.util.List getAdvertiserGroupIds() {
return advertiserGroupIds;
}
/** Select only campaigns whose advertisers belong to these advertiser groups. */
public List setAdvertiserGroupIds(java.util.List advertiserGroupIds) {
this.advertiserGroupIds = advertiserGroupIds;
return this;
}
/** Select only campaigns that belong to these advertisers. */
@com.google.api.client.util.Key
private java.util.List advertiserIds;
/** Select only campaigns that belong to these advertisers.
*/
public java.util.List getAdvertiserIds() {
return advertiserIds;
}
/** Select only campaigns that belong to these advertisers. */
public List setAdvertiserIds(java.util.List advertiserIds) {
this.advertiserIds = advertiserIds;
return this;
}
/**
* Select only archived campaigns. Don't set this field to select both archived and non-
* archived campaigns.
*/
@com.google.api.client.util.Key
private java.lang.Boolean archived;
/** Select only archived campaigns. Don't set this field to select both archived and non-archived
campaigns.
*/
public java.lang.Boolean getArchived() {
return archived;
}
/**
* Select only archived campaigns. Don't set this field to select both archived and non-
* archived campaigns.
*/
public List setArchived(java.lang.Boolean archived) {
this.archived = archived;
return this;
}
/** Select only campaigns that have at least one optimization activity. */
@com.google.api.client.util.Key
private java.lang.Boolean atLeastOneOptimizationActivity;
/** Select only campaigns that have at least one optimization activity.
*/
public java.lang.Boolean getAtLeastOneOptimizationActivity() {
return atLeastOneOptimizationActivity;
}
/** Select only campaigns that have at least one optimization activity. */
public List setAtLeastOneOptimizationActivity(java.lang.Boolean atLeastOneOptimizationActivity) {
this.atLeastOneOptimizationActivity = atLeastOneOptimizationActivity;
return this;
}
/** Exclude campaigns with these IDs. */
@com.google.api.client.util.Key
private java.util.List excludedIds;
/** Exclude campaigns with these IDs.
*/
public java.util.List getExcludedIds() {
return excludedIds;
}
/** Exclude campaigns with these IDs. */
public List setExcludedIds(java.util.List excludedIds) {
this.excludedIds = excludedIds;
return this;
}
/** Select only campaigns with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only campaigns with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only campaigns with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Select only campaigns that have overridden this event tag ID. */
@com.google.api.client.util.Key
private java.lang.Long overriddenEventTagId;
/** Select only campaigns that have overridden this event tag ID.
*/
public java.lang.Long getOverriddenEventTagId() {
return overriddenEventTagId;
}
/** Select only campaigns that have overridden this event tag ID. */
public List setOverriddenEventTagId(java.lang.Long overriddenEventTagId) {
this.overriddenEventTagId = overriddenEventTagId;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for campaigns by name or ID. Wildcards (*) are allowed. For example,
* "campaign*2015" will return campaigns with names like "campaign June 2015", "campaign April
* 2015", or simply "campaign 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "campaign" will
* match campaigns with name "my campaign", "campaign 2015", or simply "campaign".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for campaigns by name or ID. Wildcards (*) are allowed. For example,
"campaign*2015" will return campaigns with names like "campaign June 2015", "campaign April 2015",
or simply "campaign 2015". Most of the searches also add wildcards implicitly at the start and the
end of the search string. For example, a search string of "campaign" will match campaigns with name
"my campaign", "campaign 2015", or simply "campaign".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for campaigns by name or ID. Wildcards (*) are allowed. For example,
* "campaign*2015" will return campaigns with names like "campaign June 2015", "campaign April
* 2015", or simply "campaign 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "campaign" will
* match campaigns with name "my campaign", "campaign 2015", or simply "campaign".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/** Select only campaigns that belong to this subaccount. */
@com.google.api.client.util.Key
private java.lang.Long subaccountId;
/** Select only campaigns that belong to this subaccount.
*/
public java.lang.Long getSubaccountId() {
return subaccountId;
}
/** Select only campaigns that belong to this subaccount. */
public List setSubaccountId(java.lang.Long subaccountId) {
this.subaccountId = subaccountId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing campaign. This method supports patch semantics.
*
* Create a request for the method "campaigns.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Campaign ID.
* @param content the {@link com.google.api.services.dfareporting.model.Campaign}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Campaign content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/campaigns";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing campaign. This method supports patch semantics.
*
* Create a request for the method "campaigns.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Campaign ID.
* @param content the {@link com.google.api.services.dfareporting.model.Campaign}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Campaign content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.Campaign.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. Campaign ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. Campaign ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. Campaign ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing campaign.
*
* Create a request for the method "campaigns.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Campaign}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Campaign content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/campaigns";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing campaign.
*
* Create a request for the method "campaigns.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Campaign}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Campaign content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.Campaign.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ChangeLogs collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.ChangeLogs.List request = dfareporting.changeLogs().list(parameters ...)}
*
*
* @return the resource collection
*/
public ChangeLogs changeLogs() {
return new ChangeLogs();
}
/**
* The "changeLogs" collection of methods.
*/
public class ChangeLogs {
/**
* Gets one change log by ID.
*
* Create a request for the method "changeLogs.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Change log ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/changeLogs/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one change log by ID.
*
* Create a request for the method "changeLogs.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Change log ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.ChangeLog.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Change log ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Change log ID.
*/
public java.lang.Long getId() {
return id;
}
/** Change log ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a list of change logs. This method supports paging.
*
* Create a request for the method "changeLogs.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/changeLogs";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of change logs. This method supports paging.
*
* Create a request for the method "changeLogs.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.ChangeLogsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only change logs with the specified action. */
@com.google.api.client.util.Key
private java.lang.String action;
/** Select only change logs with the specified action.
*/
public java.lang.String getAction() {
return action;
}
/** Select only change logs with the specified action. */
public List setAction(java.lang.String action) {
this.action = action;
return this;
}
/** Select only change logs with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only change logs with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only change logs with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/**
* Select only change logs whose change time is before the specified maxChangeTime.The time
* should be formatted as an RFC3339 date/time string. For example, for 10:54 PM on July 18th,
* 2015, in the America/New York time zone, the format is "2015-07-18T22:54:00-04:00". In
* other words, the year, month, day, the letter T, the hour (24-hour clock system), minute,
* second, and then the time zone offset.
*/
@com.google.api.client.util.Key
private java.lang.String maxChangeTime;
/** Select only change logs whose change time is before the specified maxChangeTime.The time should be
formatted as an RFC3339 date/time string. For example, for 10:54 PM on July 18th, 2015, in the
America/New York time zone, the format is "2015-07-18T22:54:00-04:00". In other words, the year,
month, day, the letter T, the hour (24-hour clock system), minute, second, and then the time zone
offset.
*/
public java.lang.String getMaxChangeTime() {
return maxChangeTime;
}
/**
* Select only change logs whose change time is before the specified maxChangeTime.The time
* should be formatted as an RFC3339 date/time string. For example, for 10:54 PM on July 18th,
* 2015, in the America/New York time zone, the format is "2015-07-18T22:54:00-04:00". In
* other words, the year, month, day, the letter T, the hour (24-hour clock system), minute,
* second, and then the time zone offset.
*/
public List setMaxChangeTime(java.lang.String maxChangeTime) {
this.maxChangeTime = maxChangeTime;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* Select only change logs whose change time is after the specified minChangeTime.The time
* should be formatted as an RFC3339 date/time string. For example, for 10:54 PM on July 18th,
* 2015, in the America/New York time zone, the format is "2015-07-18T22:54:00-04:00". In
* other words, the year, month, day, the letter T, the hour (24-hour clock system), minute,
* second, and then the time zone offset.
*/
@com.google.api.client.util.Key
private java.lang.String minChangeTime;
/** Select only change logs whose change time is after the specified minChangeTime.The time should be
formatted as an RFC3339 date/time string. For example, for 10:54 PM on July 18th, 2015, in the
America/New York time zone, the format is "2015-07-18T22:54:00-04:00". In other words, the year,
month, day, the letter T, the hour (24-hour clock system), minute, second, and then the time zone
offset.
*/
public java.lang.String getMinChangeTime() {
return minChangeTime;
}
/**
* Select only change logs whose change time is after the specified minChangeTime.The time
* should be formatted as an RFC3339 date/time string. For example, for 10:54 PM on July 18th,
* 2015, in the America/New York time zone, the format is "2015-07-18T22:54:00-04:00". In
* other words, the year, month, day, the letter T, the hour (24-hour clock system), minute,
* second, and then the time zone offset.
*/
public List setMinChangeTime(java.lang.String minChangeTime) {
this.minChangeTime = minChangeTime;
return this;
}
/** Select only change logs with these object IDs. */
@com.google.api.client.util.Key
private java.util.List objectIds;
/** Select only change logs with these object IDs.
*/
public java.util.List getObjectIds() {
return objectIds;
}
/** Select only change logs with these object IDs. */
public List setObjectIds(java.util.List objectIds) {
this.objectIds = objectIds;
return this;
}
/** Select only change logs with the specified object type. */
@com.google.api.client.util.Key
private java.lang.String objectType;
/** Select only change logs with the specified object type.
*/
public java.lang.String getObjectType() {
return objectType;
}
/** Select only change logs with the specified object type. */
public List setObjectType(java.lang.String objectType) {
this.objectType = objectType;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Select only change logs whose object ID, user name, old or new values match the search
* string.
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Select only change logs whose object ID, user name, old or new values match the search string.
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Select only change logs whose object ID, user name, old or new values match the search
* string.
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Select only change logs with these user profile IDs. */
@com.google.api.client.util.Key
private java.util.List userProfileIds;
/** Select only change logs with these user profile IDs.
*/
public java.util.List getUserProfileIds() {
return userProfileIds;
}
/** Select only change logs with these user profile IDs. */
public List setUserProfileIds(java.util.List userProfileIds) {
this.userProfileIds = userProfileIds;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Cities collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Cities.List request = dfareporting.cities().list(parameters ...)}
*
*
* @return the resource collection
*/
public Cities cities() {
return new Cities();
}
/**
* The "cities" collection of methods.
*/
public class Cities {
/**
* Retrieves a list of cities, possibly filtered.
*
* Create a request for the method "cities.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/cities";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of cities, possibly filtered.
*
* Create a request for the method "cities.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CitiesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only cities from these countries. */
@com.google.api.client.util.Key
private java.util.List countryDartIds;
/** Select only cities from these countries.
*/
public java.util.List getCountryDartIds() {
return countryDartIds;
}
/** Select only cities from these countries. */
public List setCountryDartIds(java.util.List countryDartIds) {
this.countryDartIds = countryDartIds;
return this;
}
/** Select only cities with these DART IDs. */
@com.google.api.client.util.Key
private java.util.List dartIds;
/** Select only cities with these DART IDs.
*/
public java.util.List getDartIds() {
return dartIds;
}
/** Select only cities with these DART IDs. */
public List setDartIds(java.util.List dartIds) {
this.dartIds = dartIds;
return this;
}
/** Select only cities with names starting with this prefix. */
@com.google.api.client.util.Key
private java.lang.String namePrefix;
/** Select only cities with names starting with this prefix.
*/
public java.lang.String getNamePrefix() {
return namePrefix;
}
/** Select only cities with names starting with this prefix. */
public List setNamePrefix(java.lang.String namePrefix) {
this.namePrefix = namePrefix;
return this;
}
/** Select only cities from these regions. */
@com.google.api.client.util.Key
private java.util.List regionDartIds;
/** Select only cities from these regions.
*/
public java.util.List getRegionDartIds() {
return regionDartIds;
}
/** Select only cities from these regions. */
public List setRegionDartIds(java.util.List regionDartIds) {
this.regionDartIds = regionDartIds;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ConnectionTypes collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.ConnectionTypes.List request = dfareporting.connectionTypes().list(parameters ...)}
*
*
* @return the resource collection
*/
public ConnectionTypes connectionTypes() {
return new ConnectionTypes();
}
/**
* The "connectionTypes" collection of methods.
*/
public class ConnectionTypes {
/**
* Gets one connection type by ID.
*
* Create a request for the method "connectionTypes.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Connection type ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/connectionTypes/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one connection type by ID.
*
* Create a request for the method "connectionTypes.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Connection type ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.ConnectionType.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Connection type ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Connection type ID.
*/
public java.lang.Long getId() {
return id;
}
/** Connection type ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a list of connection types.
*
* Create a request for the method "connectionTypes.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/connectionTypes";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of connection types.
*
* Create a request for the method "connectionTypes.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.ConnectionTypesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ContentCategories collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.ContentCategories.List request = dfareporting.contentCategories().list(parameters ...)}
*
*
* @return the resource collection
*/
public ContentCategories contentCategories() {
return new ContentCategories();
}
/**
* The "contentCategories" collection of methods.
*/
public class ContentCategories {
/**
* Deletes an existing content category.
*
* Create a request for the method "contentCategories.delete".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Content category ID.
* @return the request
*/
public Delete delete(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(profileId, id);
initialize(result);
return result;
}
public class Delete extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/contentCategories/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Deletes an existing content category.
*
* Create a request for the method "contentCategories.delete".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Content category ID.
* @since 1.13
*/
protected Delete(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "DELETE", REST_PATH, null, Void.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Delete setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Content category ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Content category ID.
*/
public java.lang.Long getId() {
return id;
}
/** Content category ID. */
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets one content category by ID.
*
* Create a request for the method "contentCategories.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Content category ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/contentCategories/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one content category by ID.
*
* Create a request for the method "contentCategories.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Content category ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.ContentCategory.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Content category ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Content category ID.
*/
public java.lang.Long getId() {
return id;
}
/** Content category ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new content category.
*
* Create a request for the method "contentCategories.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ContentCategory}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.ContentCategory content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/contentCategories";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new content category.
*
* Create a request for the method "contentCategories.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ContentCategory}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.ContentCategory content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.ContentCategory.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of content categories, possibly filtered. This method supports paging.
*
* Create a request for the method "contentCategories.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/contentCategories";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of content categories, possibly filtered. This method supports paging.
*
* Create a request for the method "contentCategories.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.ContentCategoriesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only content categories with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only content categories with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only content categories with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "contentcategory*2015" will return objects with names like "contentcategory June 2015",
* "contentcategory April 2015", or simply "contentcategory 2015". Most of the searches also
* add wildcards implicitly at the start and the end of the search string. For example, a
* search string of "contentcategory" will match objects with name "my contentcategory",
* "contentcategory 2015", or simply "contentcategory".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
"contentcategory*2015" will return objects with names like "contentcategory June 2015",
"contentcategory April 2015", or simply "contentcategory 2015". Most of the searches also add
wildcards implicitly at the start and the end of the search string. For example, a search string of
"contentcategory" will match objects with name "my contentcategory", "contentcategory 2015", or
simply "contentcategory".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "contentcategory*2015" will return objects with names like "contentcategory June 2015",
* "contentcategory April 2015", or simply "contentcategory 2015". Most of the searches also
* add wildcards implicitly at the start and the end of the search string. For example, a
* search string of "contentcategory" will match objects with name "my contentcategory",
* "contentcategory 2015", or simply "contentcategory".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing content category. This method supports patch semantics.
*
* Create a request for the method "contentCategories.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. ContentCategory ID.
* @param content the {@link com.google.api.services.dfareporting.model.ContentCategory}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.ContentCategory content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/contentCategories";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing content category. This method supports patch semantics.
*
* Create a request for the method "contentCategories.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. ContentCategory ID.
* @param content the {@link com.google.api.services.dfareporting.model.ContentCategory}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.ContentCategory content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.ContentCategory.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. ContentCategory ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. ContentCategory ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. ContentCategory ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing content category.
*
* Create a request for the method "contentCategories.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ContentCategory}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.ContentCategory content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/contentCategories";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing content category.
*
* Create a request for the method "contentCategories.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ContentCategory}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.ContentCategory content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.ContentCategory.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Conversions collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Conversions.List request = dfareporting.conversions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Conversions conversions() {
return new Conversions();
}
/**
* The "conversions" collection of methods.
*/
public class Conversions {
/**
* Inserts conversions.
*
* Create a request for the method "conversions.batchinsert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Batchinsert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ConversionsBatchInsertRequest}
* @return the request
*/
public Batchinsert batchinsert(java.lang.Long profileId, com.google.api.services.dfareporting.model.ConversionsBatchInsertRequest content) throws java.io.IOException {
Batchinsert result = new Batchinsert(profileId, content);
initialize(result);
return result;
}
public class Batchinsert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{profileId}/conversions/batchinsert";
/**
* Inserts conversions.
*
* Create a request for the method "conversions.batchinsert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Batchinsert#execute()} method to invoke the remote
* operation. {@link
* Batchinsert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ConversionsBatchInsertRequest}
* @since 1.13
*/
protected Batchinsert(java.lang.Long profileId, com.google.api.services.dfareporting.model.ConversionsBatchInsertRequest content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.ConversionsBatchInsertResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Batchinsert set$Xgafv(java.lang.String $Xgafv) {
return (Batchinsert) super.set$Xgafv($Xgafv);
}
@Override
public Batchinsert setAccessToken(java.lang.String accessToken) {
return (Batchinsert) super.setAccessToken(accessToken);
}
@Override
public Batchinsert setAlt(java.lang.String alt) {
return (Batchinsert) super.setAlt(alt);
}
@Override
public Batchinsert setCallback(java.lang.String callback) {
return (Batchinsert) super.setCallback(callback);
}
@Override
public Batchinsert setFields(java.lang.String fields) {
return (Batchinsert) super.setFields(fields);
}
@Override
public Batchinsert setKey(java.lang.String key) {
return (Batchinsert) super.setKey(key);
}
@Override
public Batchinsert setOauthToken(java.lang.String oauthToken) {
return (Batchinsert) super.setOauthToken(oauthToken);
}
@Override
public Batchinsert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Batchinsert) super.setPrettyPrint(prettyPrint);
}
@Override
public Batchinsert setQuotaUser(java.lang.String quotaUser) {
return (Batchinsert) super.setQuotaUser(quotaUser);
}
@Override
public Batchinsert setUploadType(java.lang.String uploadType) {
return (Batchinsert) super.setUploadType(uploadType);
}
@Override
public Batchinsert setUploadProtocol(java.lang.String uploadProtocol) {
return (Batchinsert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Batchinsert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Batchinsert set(String parameterName, Object value) {
return (Batchinsert) super.set(parameterName, value);
}
}
/**
* Updates existing conversions.
*
* Create a request for the method "conversions.batchupdate".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Batchupdate#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ConversionsBatchUpdateRequest}
* @return the request
*/
public Batchupdate batchupdate(java.lang.Long profileId, com.google.api.services.dfareporting.model.ConversionsBatchUpdateRequest content) throws java.io.IOException {
Batchupdate result = new Batchupdate(profileId, content);
initialize(result);
return result;
}
public class Batchupdate extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{profileId}/conversions/batchupdate";
/**
* Updates existing conversions.
*
* Create a request for the method "conversions.batchupdate".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Batchupdate#execute()} method to invoke the remote
* operation. {@link
* Batchupdate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.ConversionsBatchUpdateRequest}
* @since 1.13
*/
protected Batchupdate(java.lang.Long profileId, com.google.api.services.dfareporting.model.ConversionsBatchUpdateRequest content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.ConversionsBatchUpdateResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Batchupdate set$Xgafv(java.lang.String $Xgafv) {
return (Batchupdate) super.set$Xgafv($Xgafv);
}
@Override
public Batchupdate setAccessToken(java.lang.String accessToken) {
return (Batchupdate) super.setAccessToken(accessToken);
}
@Override
public Batchupdate setAlt(java.lang.String alt) {
return (Batchupdate) super.setAlt(alt);
}
@Override
public Batchupdate setCallback(java.lang.String callback) {
return (Batchupdate) super.setCallback(callback);
}
@Override
public Batchupdate setFields(java.lang.String fields) {
return (Batchupdate) super.setFields(fields);
}
@Override
public Batchupdate setKey(java.lang.String key) {
return (Batchupdate) super.setKey(key);
}
@Override
public Batchupdate setOauthToken(java.lang.String oauthToken) {
return (Batchupdate) super.setOauthToken(oauthToken);
}
@Override
public Batchupdate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Batchupdate) super.setPrettyPrint(prettyPrint);
}
@Override
public Batchupdate setQuotaUser(java.lang.String quotaUser) {
return (Batchupdate) super.setQuotaUser(quotaUser);
}
@Override
public Batchupdate setUploadType(java.lang.String uploadType) {
return (Batchupdate) super.setUploadType(uploadType);
}
@Override
public Batchupdate setUploadProtocol(java.lang.String uploadProtocol) {
return (Batchupdate) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Batchupdate setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Batchupdate set(String parameterName, Object value) {
return (Batchupdate) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Countries collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Countries.List request = dfareporting.countries().list(parameters ...)}
*
*
* @return the resource collection
*/
public Countries countries() {
return new Countries();
}
/**
* The "countries" collection of methods.
*/
public class Countries {
/**
* Gets one country by ID.
*
* Create a request for the method "countries.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param dartId Country DART ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long dartId) throws java.io.IOException {
Get result = new Get(profileId, dartId);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/countries/{+dartId}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern DART_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one country by ID.
*
* Create a request for the method "countries.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param dartId Country DART ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long dartId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.Country.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.dartId = com.google.api.client.util.Preconditions.checkNotNull(dartId, "Required parameter dartId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Country DART ID. */
@com.google.api.client.util.Key
private java.lang.Long dartId;
/** Country DART ID.
*/
public java.lang.Long getDartId() {
return dartId;
}
/** Country DART ID. */
public Get setDartId(java.lang.Long dartId) {
this.dartId = dartId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a list of countries.
*
* Create a request for the method "countries.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/countries";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of countries.
*
* Create a request for the method "countries.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CountriesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CreativeAssets collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.CreativeAssets.List request = dfareporting.creativeAssets().list(parameters ...)}
*
*
* @return the resource collection
*/
public CreativeAssets creativeAssets() {
return new CreativeAssets();
}
/**
* The "creativeAssets" collection of methods.
*/
public class CreativeAssets {
/**
* Inserts a new creative asset.
*
* Create a request for the method "creativeAssets.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param advertiserId Advertiser ID of this creative. This is a required field.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeAssetMetadata}
* @return the request
*/
public Insert insert(java.lang.Long profileId, java.lang.Long advertiserId, com.google.api.services.dfareporting.model.CreativeAssetMetadata content) throws java.io.IOException {
Insert result = new Insert(profileId, advertiserId, content);
initialize(result);
return result;
}
/**
* Inserts a new creative asset.
*
* Create a request for the method "creativeAssets.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param profileId User profile ID associated with this request.@param advertiserId Advertiser ID of this creative. This is a required field.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeAssetMetadata} media metadata or {@code null} if none
* @param mediaContent The media HTTP content.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Insert insert(java.lang.Long profileId, java.lang.Long advertiserId, com.google.api.services.dfareporting.model.CreativeAssetMetadata content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Insert result = new Insert(profileId, advertiserId, content, mediaContent);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeAssets/{+advertiserId}/creativeAssets";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ADVERTISER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new creative asset.
*
* Create a request for the method "creativeAssets.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param advertiserId Advertiser ID of this creative. This is a required field.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeAssetMetadata}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, java.lang.Long advertiserId, com.google.api.services.dfareporting.model.CreativeAssetMetadata content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeAssetMetadata.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.advertiserId = com.google.api.client.util.Preconditions.checkNotNull(advertiserId, "Required parameter advertiserId must be specified.");
}
/**
* Inserts a new creative asset.
*
* Create a request for the method "creativeAssets.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param profileId User profile ID associated with this request.@param advertiserId Advertiser ID of this creative. This is a required field.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeAssetMetadata} media metadata or {@code null} if none
* @param mediaContent The media HTTP content.
* @since 1.13
*/
protected Insert(java.lang.Long profileId, java.lang.Long advertiserId, com.google.api.services.dfareporting.model.CreativeAssetMetadata content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(Dfareporting.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.dfareporting.model.CreativeAssetMetadata.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.advertiserId = com.google.api.client.util.Preconditions.checkNotNull(advertiserId, "Required parameter advertiserId must be specified.");
com.google.api.client.util.Preconditions.checkNotNull(mediaContent, "Required parameter mediaContent must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Advertiser ID of this creative. This is a required field. */
@com.google.api.client.util.Key
private java.lang.Long advertiserId;
/** Advertiser ID of this creative. This is a required field.
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/** Advertiser ID of this creative. This is a required field. */
public Insert setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CreativeFieldValues collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.CreativeFieldValues.List request = dfareporting.creativeFieldValues().list(parameters ...)}
*
*
* @return the resource collection
*/
public CreativeFieldValues creativeFieldValues() {
return new CreativeFieldValues();
}
/**
* The "creativeFieldValues" collection of methods.
*/
public class CreativeFieldValues {
/**
* Deletes an existing creative field value.
*
* Create a request for the method "creativeFieldValues.delete".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param id Creative Field Value ID
* @return the request
*/
public Delete delete(java.lang.Long profileId, java.lang.Long creativeFieldId, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(profileId, creativeFieldId, id);
initialize(result);
return result;
}
public class Delete extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+creativeFieldId}/creativeFieldValues/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CREATIVE_FIELD_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Deletes an existing creative field value.
*
* Create a request for the method "creativeFieldValues.delete".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param id Creative Field Value ID
* @since 1.13
*/
protected Delete(java.lang.Long profileId, java.lang.Long creativeFieldId, java.lang.Long id) {
super(Dfareporting.this, "DELETE", REST_PATH, null, Void.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.creativeFieldId = com.google.api.client.util.Preconditions.checkNotNull(creativeFieldId, "Required parameter creativeFieldId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Delete setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative field ID for this creative field value. */
@com.google.api.client.util.Key
private java.lang.Long creativeFieldId;
/** Creative field ID for this creative field value.
*/
public java.lang.Long getCreativeFieldId() {
return creativeFieldId;
}
/** Creative field ID for this creative field value. */
public Delete setCreativeFieldId(java.lang.Long creativeFieldId) {
this.creativeFieldId = creativeFieldId;
return this;
}
/** Creative Field Value ID */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Creative Field Value ID
*/
public java.lang.Long getId() {
return id;
}
/** Creative Field Value ID */
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets one creative field value by ID.
*
* Create a request for the method "creativeFieldValues.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param id Creative Field Value ID
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long creativeFieldId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, creativeFieldId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+creativeFieldId}/creativeFieldValues/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CREATIVE_FIELD_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one creative field value by ID.
*
* Create a request for the method "creativeFieldValues.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param id Creative Field Value ID
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long creativeFieldId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CreativeFieldValue.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.creativeFieldId = com.google.api.client.util.Preconditions.checkNotNull(creativeFieldId, "Required parameter creativeFieldId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative field ID for this creative field value. */
@com.google.api.client.util.Key
private java.lang.Long creativeFieldId;
/** Creative field ID for this creative field value.
*/
public java.lang.Long getCreativeFieldId() {
return creativeFieldId;
}
/** Creative field ID for this creative field value. */
public Get setCreativeFieldId(java.lang.Long creativeFieldId) {
this.creativeFieldId = creativeFieldId;
return this;
}
/** Creative Field Value ID */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Creative Field Value ID
*/
public java.lang.Long getId() {
return id;
}
/** Creative Field Value ID */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new creative field value.
*
* Create a request for the method "creativeFieldValues.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeFieldValue}
* @return the request
*/
public Insert insert(java.lang.Long profileId, java.lang.Long creativeFieldId, com.google.api.services.dfareporting.model.CreativeFieldValue content) throws java.io.IOException {
Insert result = new Insert(profileId, creativeFieldId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+creativeFieldId}/creativeFieldValues";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CREATIVE_FIELD_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new creative field value.
*
* Create a request for the method "creativeFieldValues.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeFieldValue}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, java.lang.Long creativeFieldId, com.google.api.services.dfareporting.model.CreativeFieldValue content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeFieldValue.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.creativeFieldId = com.google.api.client.util.Preconditions.checkNotNull(creativeFieldId, "Required parameter creativeFieldId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative field ID for this creative field value. */
@com.google.api.client.util.Key
private java.lang.Long creativeFieldId;
/** Creative field ID for this creative field value.
*/
public java.lang.Long getCreativeFieldId() {
return creativeFieldId;
}
/** Creative field ID for this creative field value. */
public Insert setCreativeFieldId(java.lang.Long creativeFieldId) {
this.creativeFieldId = creativeFieldId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of creative field values, possibly filtered. This method supports paging.
*
* Create a request for the method "creativeFieldValues.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @return the request
*/
public List list(java.lang.Long profileId, java.lang.Long creativeFieldId) throws java.io.IOException {
List result = new List(profileId, creativeFieldId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+creativeFieldId}/creativeFieldValues";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CREATIVE_FIELD_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of creative field values, possibly filtered. This method supports paging.
*
* Create a request for the method "creativeFieldValues.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @since 1.13
*/
protected List(java.lang.Long profileId, java.lang.Long creativeFieldId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CreativeFieldValuesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.creativeFieldId = com.google.api.client.util.Preconditions.checkNotNull(creativeFieldId, "Required parameter creativeFieldId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative field ID for this creative field value. */
@com.google.api.client.util.Key
private java.lang.Long creativeFieldId;
/** Creative field ID for this creative field value.
*/
public java.lang.Long getCreativeFieldId() {
return creativeFieldId;
}
/** Creative field ID for this creative field value. */
public List setCreativeFieldId(java.lang.Long creativeFieldId) {
this.creativeFieldId = creativeFieldId;
return this;
}
/** Select only creative field values with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only creative field values with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only creative field values with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for creative field values by their values. Wildcards (e.g. *) are not
* allowed.
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for creative field values by their values. Wildcards (e.g. *) are not allowed.
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for creative field values by their values. Wildcards (e.g. *) are not
* allowed.
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing creative field value. This method supports patch semantics.
*
* Create a request for the method "creativeFieldValues.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId CreativeField ID.
* @param id CreativeFieldValue ID.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeFieldValue}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long creativeFieldId, java.lang.Long id, com.google.api.services.dfareporting.model.CreativeFieldValue content) throws java.io.IOException {
Patch result = new Patch(profileId, creativeFieldId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+creativeFieldId}/creativeFieldValues";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CREATIVE_FIELD_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative field value. This method supports patch semantics.
*
* Create a request for the method "creativeFieldValues.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId CreativeField ID.
* @param id CreativeFieldValue ID.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeFieldValue}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long creativeFieldId, java.lang.Long id, com.google.api.services.dfareporting.model.CreativeFieldValue content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeFieldValue.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.creativeFieldId = com.google.api.client.util.Preconditions.checkNotNull(creativeFieldId, "Required parameter creativeFieldId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** CreativeField ID. */
@com.google.api.client.util.Key
private java.lang.Long creativeFieldId;
/** CreativeField ID.
*/
public java.lang.Long getCreativeFieldId() {
return creativeFieldId;
}
/** CreativeField ID. */
public Patch setCreativeFieldId(java.lang.Long creativeFieldId) {
this.creativeFieldId = creativeFieldId;
return this;
}
/** CreativeFieldValue ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** CreativeFieldValue ID.
*/
public java.lang.Long getId() {
return id;
}
/** CreativeFieldValue ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing creative field value.
*
* Create a request for the method "creativeFieldValues.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeFieldValue}
* @return the request
*/
public Update update(java.lang.Long profileId, java.lang.Long creativeFieldId, com.google.api.services.dfareporting.model.CreativeFieldValue content) throws java.io.IOException {
Update result = new Update(profileId, creativeFieldId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+creativeFieldId}/creativeFieldValues";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern CREATIVE_FIELD_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative field value.
*
* Create a request for the method "creativeFieldValues.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param creativeFieldId Creative field ID for this creative field value.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeFieldValue}
* @since 1.13
*/
protected Update(java.lang.Long profileId, java.lang.Long creativeFieldId, com.google.api.services.dfareporting.model.CreativeFieldValue content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeFieldValue.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.creativeFieldId = com.google.api.client.util.Preconditions.checkNotNull(creativeFieldId, "Required parameter creativeFieldId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative field ID for this creative field value. */
@com.google.api.client.util.Key
private java.lang.Long creativeFieldId;
/** Creative field ID for this creative field value.
*/
public java.lang.Long getCreativeFieldId() {
return creativeFieldId;
}
/** Creative field ID for this creative field value. */
public Update setCreativeFieldId(java.lang.Long creativeFieldId) {
this.creativeFieldId = creativeFieldId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CreativeFields collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.CreativeFields.List request = dfareporting.creativeFields().list(parameters ...)}
*
*
* @return the resource collection
*/
public CreativeFields creativeFields() {
return new CreativeFields();
}
/**
* The "creativeFields" collection of methods.
*/
public class CreativeFields {
/**
* Deletes an existing creative field.
*
* Create a request for the method "creativeFields.delete".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Creative Field ID
* @return the request
*/
public Delete delete(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(profileId, id);
initialize(result);
return result;
}
public class Delete extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Deletes an existing creative field.
*
* Create a request for the method "creativeFields.delete".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Creative Field ID
* @since 1.13
*/
protected Delete(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "DELETE", REST_PATH, null, Void.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Delete setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative Field ID */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Creative Field ID
*/
public java.lang.Long getId() {
return id;
}
/** Creative Field ID */
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets one creative field by ID.
*
* Create a request for the method "creativeFields.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Creative Field ID
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one creative field by ID.
*
* Create a request for the method "creativeFields.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Creative Field ID
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CreativeField.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative Field ID */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Creative Field ID
*/
public java.lang.Long getId() {
return id;
}
/** Creative Field ID */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new creative field.
*
* Create a request for the method "creativeFields.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeField}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeField content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new creative field.
*
* Create a request for the method "creativeFields.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeField}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeField content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeField.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of creative fields, possibly filtered. This method supports paging.
*
* Create a request for the method "creativeFields.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of creative fields, possibly filtered. This method supports paging.
*
* Create a request for the method "creativeFields.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CreativeFieldsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only creative fields that belong to these advertisers. */
@com.google.api.client.util.Key
private java.util.List advertiserIds;
/** Select only creative fields that belong to these advertisers.
*/
public java.util.List getAdvertiserIds() {
return advertiserIds;
}
/** Select only creative fields that belong to these advertisers. */
public List setAdvertiserIds(java.util.List advertiserIds) {
this.advertiserIds = advertiserIds;
return this;
}
/** Select only creative fields with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only creative fields with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only creative fields with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for creative fields by name or ID. Wildcards (*) are allowed. For example,
* "creativefield*2015" will return creative fields with names like "creativefield June 2015",
* "creativefield April 2015", or simply "creativefield 2015". Most of the searches also add
* wild-cards implicitly at the start and the end of the search string. For example, a search
* string of "creativefield" will match creative fields with the name "my creativefield",
* "creativefield 2015", or simply "creativefield".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for creative fields by name or ID. Wildcards (*) are allowed. For example,
"creativefield*2015" will return creative fields with names like "creativefield June 2015",
"creativefield April 2015", or simply "creativefield 2015". Most of the searches also add wild-
cards implicitly at the start and the end of the search string. For example, a search string of
"creativefield" will match creative fields with the name "my creativefield", "creativefield 2015",
or simply "creativefield".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for creative fields by name or ID. Wildcards (*) are allowed. For example,
* "creativefield*2015" will return creative fields with names like "creativefield June 2015",
* "creativefield April 2015", or simply "creativefield 2015". Most of the searches also add
* wild-cards implicitly at the start and the end of the search string. For example, a search
* string of "creativefield" will match creative fields with the name "my creativefield",
* "creativefield 2015", or simply "creativefield".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing creative field. This method supports patch semantics.
*
* Create a request for the method "creativeFields.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id CreativeField ID.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeField}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.CreativeField content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative field. This method supports patch semantics.
*
* Create a request for the method "creativeFields.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id CreativeField ID.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeField}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.CreativeField content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeField.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** CreativeField ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** CreativeField ID.
*/
public java.lang.Long getId() {
return id;
}
/** CreativeField ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing creative field.
*
* Create a request for the method "creativeFields.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeField}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeField content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeFields";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative field.
*
* Create a request for the method "creativeFields.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeField}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeField content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeField.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the CreativeGroups collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.CreativeGroups.List request = dfareporting.creativeGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public CreativeGroups creativeGroups() {
return new CreativeGroups();
}
/**
* The "creativeGroups" collection of methods.
*/
public class CreativeGroups {
/**
* Gets one creative group by ID.
*
* Create a request for the method "creativeGroups.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Creative group ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeGroups/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one creative group by ID.
*
* Create a request for the method "creativeGroups.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Creative group ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CreativeGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative group ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Creative group ID.
*/
public java.lang.Long getId() {
return id;
}
/** Creative group ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new creative group.
*
* Create a request for the method "creativeGroups.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeGroup}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeGroup content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new creative group.
*
* Create a request for the method "creativeGroups.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeGroup}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeGroup content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of creative groups, possibly filtered. This method supports paging.
*
* Create a request for the method "creativeGroups.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of creative groups, possibly filtered. This method supports paging.
*
* Create a request for the method "creativeGroups.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CreativeGroupsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only creative groups that belong to these advertisers. */
@com.google.api.client.util.Key
private java.util.List advertiserIds;
/** Select only creative groups that belong to these advertisers.
*/
public java.util.List getAdvertiserIds() {
return advertiserIds;
}
/** Select only creative groups that belong to these advertisers. */
public List setAdvertiserIds(java.util.List advertiserIds) {
this.advertiserIds = advertiserIds;
return this;
}
/** Select only creative groups that belong to this subgroup. */
@com.google.api.client.util.Key
private java.lang.Integer groupNumber;
/** Select only creative groups that belong to this subgroup.
*/
public java.lang.Integer getGroupNumber() {
return groupNumber;
}
/** Select only creative groups that belong to this subgroup. */
public List setGroupNumber(java.lang.Integer groupNumber) {
this.groupNumber = groupNumber;
return this;
}
/** Select only creative groups with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only creative groups with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only creative groups with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for creative groups by name or ID. Wildcards (*) are allowed. For example,
* "creativegroup*2015" will return creative groups with names like "creativegroup June 2015",
* "creativegroup April 2015", or simply "creativegroup 2015". Most of the searches also add
* wild-cards implicitly at the start and the end of the search string. For example, a search
* string of "creativegroup" will match creative groups with the name "my creativegroup",
* "creativegroup 2015", or simply "creativegroup".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for creative groups by name or ID. Wildcards (*) are allowed. For example,
"creativegroup*2015" will return creative groups with names like "creativegroup June 2015",
"creativegroup April 2015", or simply "creativegroup 2015". Most of the searches also add wild-
cards implicitly at the start and the end of the search string. For example, a search string of
"creativegroup" will match creative groups with the name "my creativegroup", "creativegroup 2015",
or simply "creativegroup".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for creative groups by name or ID. Wildcards (*) are allowed. For example,
* "creativegroup*2015" will return creative groups with names like "creativegroup June 2015",
* "creativegroup April 2015", or simply "creativegroup 2015". Most of the searches also add
* wild-cards implicitly at the start and the end of the search string. For example, a search
* string of "creativegroup" will match creative groups with the name "my creativegroup",
* "creativegroup 2015", or simply "creativegroup".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing creative group. This method supports patch semantics.
*
* Create a request for the method "creativeGroups.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Creative Group ID.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeGroup}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.CreativeGroup content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative group. This method supports patch semantics.
*
* Create a request for the method "creativeGroups.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Creative Group ID.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeGroup}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.CreativeGroup content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. Creative Group ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. Creative Group ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. Creative Group ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing creative group.
*
* Create a request for the method "creativeGroups.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeGroup}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeGroup content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creativeGroups";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative group.
*
* Create a request for the method "creativeGroups.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.CreativeGroup}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.CreativeGroup content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.CreativeGroup.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Creatives collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Creatives.List request = dfareporting.creatives().list(parameters ...)}
*
*
* @return the resource collection
*/
public Creatives creatives() {
return new Creatives();
}
/**
* The "creatives" collection of methods.
*/
public class Creatives {
/**
* Gets one creative by ID.
*
* Create a request for the method "creatives.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Creative ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creatives/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one creative by ID.
*
* Create a request for the method "creatives.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Creative ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.Creative.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Creative ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Creative ID.
*/
public java.lang.Long getId() {
return id;
}
/** Creative ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new creative.
*
* Create a request for the method "creatives.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Creative}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Creative content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creatives";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new creative.
*
* Create a request for the method "creatives.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Creative}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.Creative content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.Creative.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of creatives, possibly filtered. This method supports paging.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creatives";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of creatives, possibly filtered. This method supports paging.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.CreativesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only active creatives. Leave blank to select active and inactive creatives. */
@com.google.api.client.util.Key
private java.lang.Boolean active;
/** Select only active creatives. Leave blank to select active and inactive creatives.
*/
public java.lang.Boolean getActive() {
return active;
}
/** Select only active creatives. Leave blank to select active and inactive creatives. */
public List setActive(java.lang.Boolean active) {
this.active = active;
return this;
}
/** Select only creatives with this advertiser ID. */
@com.google.api.client.util.Key
private java.lang.Long advertiserId;
/** Select only creatives with this advertiser ID.
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/** Select only creatives with this advertiser ID. */
public List setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/**
* Select only archived creatives. Leave blank to select archived and unarchived creatives.
*/
@com.google.api.client.util.Key
private java.lang.Boolean archived;
/** Select only archived creatives. Leave blank to select archived and unarchived creatives.
*/
public java.lang.Boolean getArchived() {
return archived;
}
/**
* Select only archived creatives. Leave blank to select archived and unarchived creatives.
*/
public List setArchived(java.lang.Boolean archived) {
this.archived = archived;
return this;
}
/** Select only creatives with this campaign ID. */
@com.google.api.client.util.Key
private java.lang.Long campaignId;
/** Select only creatives with this campaign ID.
*/
public java.lang.Long getCampaignId() {
return campaignId;
}
/** Select only creatives with this campaign ID. */
public List setCampaignId(java.lang.Long campaignId) {
this.campaignId = campaignId;
return this;
}
/** Select only in-stream video creatives with these companion IDs. */
@com.google.api.client.util.Key
private java.util.List companionCreativeIds;
/** Select only in-stream video creatives with these companion IDs.
*/
public java.util.List getCompanionCreativeIds() {
return companionCreativeIds;
}
/** Select only in-stream video creatives with these companion IDs. */
public List setCompanionCreativeIds(java.util.List companionCreativeIds) {
this.companionCreativeIds = companionCreativeIds;
return this;
}
/** Select only creatives with these creative field IDs. */
@com.google.api.client.util.Key
private java.util.List creativeFieldIds;
/** Select only creatives with these creative field IDs.
*/
public java.util.List getCreativeFieldIds() {
return creativeFieldIds;
}
/** Select only creatives with these creative field IDs. */
public List setCreativeFieldIds(java.util.List creativeFieldIds) {
this.creativeFieldIds = creativeFieldIds;
return this;
}
/** Select only creatives with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only creatives with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only creatives with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Select only creatives with these rendering IDs. */
@com.google.api.client.util.Key
private java.util.List renderingIds;
/** Select only creatives with these rendering IDs.
*/
public java.util.List getRenderingIds() {
return renderingIds;
}
/** Select only creatives with these rendering IDs. */
public List setRenderingIds(java.util.List renderingIds) {
this.renderingIds = renderingIds;
return this;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "creative*2015" will return objects with names like "creative June 2015", "creative April
* 2015", or simply "creative 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "creative" will
* match objects with name "my creative", "creative 2015", or simply "creative".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name or ID. Wildcards (*) are allowed. For example, "creative*2015"
will return objects with names like "creative June 2015", "creative April 2015", or simply
"creative 2015". Most of the searches also add wildcards implicitly at the start and the end of the
search string. For example, a search string of "creative" will match objects with name "my
creative", "creative 2015", or simply "creative".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "creative*2015" will return objects with names like "creative June 2015", "creative April
* 2015", or simply "creative 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "creative" will
* match objects with name "my creative", "creative 2015", or simply "creative".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Select only creatives with these size IDs. */
@com.google.api.client.util.Key
private java.util.List sizeIds;
/** Select only creatives with these size IDs.
*/
public java.util.List getSizeIds() {
return sizeIds;
}
/** Select only creatives with these size IDs. */
public List setSizeIds(java.util.List sizeIds) {
this.sizeIds = sizeIds;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
/** Select only creatives corresponding to this Studio creative ID. */
@com.google.api.client.util.Key
private java.lang.Long studioCreativeId;
/** Select only creatives corresponding to this Studio creative ID.
*/
public java.lang.Long getStudioCreativeId() {
return studioCreativeId;
}
/** Select only creatives corresponding to this Studio creative ID. */
public List setStudioCreativeId(java.lang.Long studioCreativeId) {
this.studioCreativeId = studioCreativeId;
return this;
}
/** Select only creatives with these creative types. */
@com.google.api.client.util.Key
private java.util.List types;
/** Select only creatives with these creative types.
*/
public java.util.List getTypes() {
return types;
}
/** Select only creatives with these creative types. */
public List setTypes(java.util.List types) {
this.types = types;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing creative. This method supports patch semantics.
*
* Create a request for the method "creatives.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Creative ID.
* @param content the {@link com.google.api.services.dfareporting.model.Creative}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Creative content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creatives";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative. This method supports patch semantics.
*
* Create a request for the method "creatives.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. Creative ID.
* @param content the {@link com.google.api.services.dfareporting.model.Creative}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.Creative content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.Creative.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. Creative ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. Creative ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. Creative ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing creative.
*
* Create a request for the method "creatives.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Creative}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Creative content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/creatives";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing creative.
*
* Create a request for the method "creatives.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.Creative}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.Creative content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.Creative.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the DimensionValues collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.DimensionValues.List request = dfareporting.dimensionValues().list(parameters ...)}
*
*
* @return the resource collection
*/
public DimensionValues dimensionValues() {
return new DimensionValues();
}
/**
* The "dimensionValues" collection of methods.
*/
public class DimensionValues {
/**
* Retrieves list of report dimension values for a list of filters.
*
* Create a request for the method "dimensionValues.query".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Query#execute()} method to invoke the remote operation.
*
* @param profileId The Campaign Manager 360 user profile ID.
* @param content the {@link com.google.api.services.dfareporting.model.DimensionValueRequest}
* @return the request
*/
public Query query(java.lang.Long profileId, com.google.api.services.dfareporting.model.DimensionValueRequest content) throws java.io.IOException {
Query result = new Query(profileId, content);
initialize(result);
return result;
}
public class Query extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{profileId}/dimensionvalues/query";
/**
* Retrieves list of report dimension values for a list of filters.
*
* Create a request for the method "dimensionValues.query".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Query#execute()} method to invoke the remote operation.
* {@link
* Query#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId The Campaign Manager 360 user profile ID.
* @param content the {@link com.google.api.services.dfareporting.model.DimensionValueRequest}
* @since 1.13
*/
protected Query(java.lang.Long profileId, com.google.api.services.dfareporting.model.DimensionValueRequest content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.DimensionValueList.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Query set$Xgafv(java.lang.String $Xgafv) {
return (Query) super.set$Xgafv($Xgafv);
}
@Override
public Query setAccessToken(java.lang.String accessToken) {
return (Query) super.setAccessToken(accessToken);
}
@Override
public Query setAlt(java.lang.String alt) {
return (Query) super.setAlt(alt);
}
@Override
public Query setCallback(java.lang.String callback) {
return (Query) super.setCallback(callback);
}
@Override
public Query setFields(java.lang.String fields) {
return (Query) super.setFields(fields);
}
@Override
public Query setKey(java.lang.String key) {
return (Query) super.setKey(key);
}
@Override
public Query setOauthToken(java.lang.String oauthToken) {
return (Query) super.setOauthToken(oauthToken);
}
@Override
public Query setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Query) super.setPrettyPrint(prettyPrint);
}
@Override
public Query setQuotaUser(java.lang.String quotaUser) {
return (Query) super.setQuotaUser(quotaUser);
}
@Override
public Query setUploadType(java.lang.String uploadType) {
return (Query) super.setUploadType(uploadType);
}
@Override
public Query setUploadProtocol(java.lang.String uploadProtocol) {
return (Query) super.setUploadProtocol(uploadProtocol);
}
/** The Campaign Manager 360 user profile ID. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** The Campaign Manager 360 user profile ID.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** The Campaign Manager 360 user profile ID. */
public Query setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 100]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public Query setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The value of the nextToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The value of the nextToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous result page. */
public Query setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public Query set(String parameterName, Object value) {
return (Query) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the DirectorySites collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.DirectorySites.List request = dfareporting.directorySites().list(parameters ...)}
*
*
* @return the resource collection
*/
public DirectorySites directorySites() {
return new DirectorySites();
}
/**
* The "directorySites" collection of methods.
*/
public class DirectorySites {
/**
* Gets one directory site by ID.
*
* Create a request for the method "directorySites.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Directory site ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/directorySites/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one directory site by ID.
*
* Create a request for the method "directorySites.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Directory site ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.DirectorySite.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Directory site ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Directory site ID.
*/
public java.lang.Long getId() {
return id;
}
/** Directory site ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new directory site.
*
* Create a request for the method "directorySites.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.DirectorySite}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.DirectorySite content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/directorySites";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new directory site.
*
* Create a request for the method "directorySites.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.DirectorySite}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.DirectorySite content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.DirectorySite.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of directory sites, possibly filtered. This method supports paging.
*
* Create a request for the method "directorySites.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/directorySites";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of directory sites, possibly filtered. This method supports paging.
*
* Create a request for the method "directorySites.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.DirectorySitesListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/**
* This search filter is no longer supported and will have no effect on the results returned.
*/
@com.google.api.client.util.Key
private java.lang.Boolean acceptsInStreamVideoPlacements;
/** This search filter is no longer supported and will have no effect on the results returned.
*/
public java.lang.Boolean getAcceptsInStreamVideoPlacements() {
return acceptsInStreamVideoPlacements;
}
/**
* This search filter is no longer supported and will have no effect on the results returned.
*/
public List setAcceptsInStreamVideoPlacements(java.lang.Boolean acceptsInStreamVideoPlacements) {
this.acceptsInStreamVideoPlacements = acceptsInStreamVideoPlacements;
return this;
}
/**
* This search filter is no longer supported and will have no effect on the results returned.
*/
@com.google.api.client.util.Key
private java.lang.Boolean acceptsInterstitialPlacements;
/** This search filter is no longer supported and will have no effect on the results returned.
*/
public java.lang.Boolean getAcceptsInterstitialPlacements() {
return acceptsInterstitialPlacements;
}
/**
* This search filter is no longer supported and will have no effect on the results returned.
*/
public List setAcceptsInterstitialPlacements(java.lang.Boolean acceptsInterstitialPlacements) {
this.acceptsInterstitialPlacements = acceptsInterstitialPlacements;
return this;
}
/**
* Select only directory sites that accept publisher paid placements. This field can be left
* blank.
*/
@com.google.api.client.util.Key
private java.lang.Boolean acceptsPublisherPaidPlacements;
/** Select only directory sites that accept publisher paid placements. This field can be left blank.
*/
public java.lang.Boolean getAcceptsPublisherPaidPlacements() {
return acceptsPublisherPaidPlacements;
}
/**
* Select only directory sites that accept publisher paid placements. This field can be left
* blank.
*/
public List setAcceptsPublisherPaidPlacements(java.lang.Boolean acceptsPublisherPaidPlacements) {
this.acceptsPublisherPaidPlacements = acceptsPublisherPaidPlacements;
return this;
}
/**
* Select only active directory sites. Leave blank to retrieve both active and inactive
* directory sites.
*/
@com.google.api.client.util.Key
private java.lang.Boolean active;
/** Select only active directory sites. Leave blank to retrieve both active and inactive directory
sites.
*/
public java.lang.Boolean getActive() {
return active;
}
/**
* Select only active directory sites. Leave blank to retrieve both active and inactive
* directory sites.
*/
public List setActive(java.lang.Boolean active) {
this.active = active;
return this;
}
/** Select only directory sites with this Ad Manager network code. */
@com.google.api.client.util.Key
private java.lang.String dfpNetworkCode;
/** Select only directory sites with this Ad Manager network code.
*/
public java.lang.String getDfpNetworkCode() {
return dfpNetworkCode;
}
/** Select only directory sites with this Ad Manager network code. */
public List setDfpNetworkCode(java.lang.String dfpNetworkCode) {
this.dfpNetworkCode = dfpNetworkCode;
return this;
}
/** Select only directory sites with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only directory sites with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only directory sites with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 1000]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** Value of the nextPageToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Value of the nextPageToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Value of the nextPageToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Allows searching for objects by name, ID or URL. Wildcards (*) are allowed. For example,
* "directory site*2015" will return objects with names like "directory site June 2015",
* "directory site April 2015", or simply "directory site 2015". Most of the searches also add
* wildcards implicitly at the start and the end of the search string. For example, a search
* string of "directory site" will match objects with name "my directory site", "directory
* site 2015" or simply, "directory site".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name, ID or URL. Wildcards (*) are allowed. For example, "directory
site*2015" will return objects with names like "directory site June 2015", "directory site April
2015", or simply "directory site 2015". Most of the searches also add wildcards implicitly at the
start and the end of the search string. For example, a search string of "directory site" will match
objects with name "my directory site", "directory site 2015" or simply, "directory site".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name, ID or URL. Wildcards (*) are allowed. For example,
* "directory site*2015" will return objects with names like "directory site June 2015",
* "directory site April 2015", or simply "directory site 2015". Most of the searches also add
* wildcards implicitly at the start and the end of the search string. For example, a search
* string of "directory site" will match objects with name "my directory site", "directory
* site 2015" or simply, "directory site".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the DynamicTargetingKeys collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.DynamicTargetingKeys.List request = dfareporting.dynamicTargetingKeys().list(parameters ...)}
*
*
* @return the resource collection
*/
public DynamicTargetingKeys dynamicTargetingKeys() {
return new DynamicTargetingKeys();
}
/**
* The "dynamicTargetingKeys" collection of methods.
*/
public class DynamicTargetingKeys {
/**
* Deletes an existing dynamic targeting key.
*
* Create a request for the method "dynamicTargetingKeys.delete".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param objectId ID of the object of this dynamic targeting key. This is a required field.
* @param name Required. Name of this dynamic targeting key. This is a required field. Must be less than 256
* characters long and cannot contain commas. All characters are converted to lowercase.
* @param objectType Required. Type of the object of this dynamic targeting key. This is a required field.
* @return the request
*/
public Delete delete(java.lang.Long profileId, java.lang.Long objectId, java.lang.String name, java.lang.String objectType) throws java.io.IOException {
Delete result = new Delete(profileId, objectId, name, objectType);
initialize(result);
return result;
}
public class Delete extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/dynamicTargetingKeys/{+objectId}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern OBJECT_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Deletes an existing dynamic targeting key.
*
* Create a request for the method "dynamicTargetingKeys.delete".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param objectId ID of the object of this dynamic targeting key. This is a required field.
* @param name Required. Name of this dynamic targeting key. This is a required field. Must be less than 256
* characters long and cannot contain commas. All characters are converted to lowercase.
* @param objectType Required. Type of the object of this dynamic targeting key. This is a required field.
* @since 1.13
*/
protected Delete(java.lang.Long profileId, java.lang.Long objectId, java.lang.String name, java.lang.String objectType) {
super(Dfareporting.this, "DELETE", REST_PATH, null, Void.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.objectId = com.google.api.client.util.Preconditions.checkNotNull(objectId, "Required parameter objectId must be specified.");
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
this.objectType = com.google.api.client.util.Preconditions.checkNotNull(objectType, "Required parameter objectType must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Delete setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** ID of the object of this dynamic targeting key. This is a required field. */
@com.google.api.client.util.Key
private java.lang.Long objectId;
/** ID of the object of this dynamic targeting key. This is a required field.
*/
public java.lang.Long getObjectId() {
return objectId;
}
/** ID of the object of this dynamic targeting key. This is a required field. */
public Delete setObjectId(java.lang.Long objectId) {
this.objectId = objectId;
return this;
}
/**
* Required. Name of this dynamic targeting key. This is a required field. Must be less than
* 256 characters long and cannot contain commas. All characters are converted to lowercase.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of this dynamic targeting key. This is a required field. Must be less than 256
characters long and cannot contain commas. All characters are converted to lowercase.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of this dynamic targeting key. This is a required field. Must be less than
* 256 characters long and cannot contain commas. All characters are converted to lowercase.
*/
public Delete setName(java.lang.String name) {
this.name = name;
return this;
}
/** Required. Type of the object of this dynamic targeting key. This is a required field. */
@com.google.api.client.util.Key
private java.lang.String objectType;
/** Required. Type of the object of this dynamic targeting key. This is a required field.
*/
public java.lang.String getObjectType() {
return objectType;
}
/** Required. Type of the object of this dynamic targeting key. This is a required field. */
public Delete setObjectType(java.lang.String objectType) {
this.objectType = objectType;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Inserts a new dynamic targeting key. Keys must be created at the advertiser level before being
* assigned to the advertiser's ads, creatives, or placements. There is a maximum of 1000 keys per
* advertiser, out of which a maximum of 20 keys can be assigned per ad, creative, or placement.
*
* Create a request for the method "dynamicTargetingKeys.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.DynamicTargetingKey}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.DynamicTargetingKey content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/dynamicTargetingKeys";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new dynamic targeting key. Keys must be created at the advertiser level before being
* assigned to the advertiser's ads, creatives, or placements. There is a maximum of 1000 keys per
* advertiser, out of which a maximum of 20 keys can be assigned per ad, creative, or placement.
*
* Create a request for the method "dynamicTargetingKeys.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.DynamicTargetingKey}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.DynamicTargetingKey content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.DynamicTargetingKey.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of dynamic targeting keys.
*
* Create a request for the method "dynamicTargetingKeys.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/dynamicTargetingKeys";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of dynamic targeting keys.
*
* Create a request for the method "dynamicTargetingKeys.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.DynamicTargetingKeysListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only dynamic targeting keys whose object has this advertiser ID. */
@com.google.api.client.util.Key
private java.lang.Long advertiserId;
/** Select only dynamic targeting keys whose object has this advertiser ID.
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/** Select only dynamic targeting keys whose object has this advertiser ID. */
public List setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/** Select only dynamic targeting keys exactly matching these names. */
@com.google.api.client.util.Key
private java.util.List names;
/** Select only dynamic targeting keys exactly matching these names.
*/
public java.util.List getNames() {
return names;
}
/** Select only dynamic targeting keys exactly matching these names. */
public List setNames(java.util.List names) {
this.names = names;
return this;
}
/** Select only dynamic targeting keys with this object ID. */
@com.google.api.client.util.Key
private java.lang.Long objectId;
/** Select only dynamic targeting keys with this object ID.
*/
public java.lang.Long getObjectId() {
return objectId;
}
/** Select only dynamic targeting keys with this object ID. */
public List setObjectId(java.lang.Long objectId) {
this.objectId = objectId;
return this;
}
/** Select only dynamic targeting keys with this object type. */
@com.google.api.client.util.Key
private java.lang.String objectType;
/** Select only dynamic targeting keys with this object type.
*/
public java.lang.String getObjectType() {
return objectType;
}
/** Select only dynamic targeting keys with this object type. */
public List setObjectType(java.lang.String objectType) {
this.objectType = objectType;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the EventTags collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.EventTags.List request = dfareporting.eventTags().list(parameters ...)}
*
*
* @return the resource collection
*/
public EventTags eventTags() {
return new EventTags();
}
/**
* The "eventTags" collection of methods.
*/
public class EventTags {
/**
* Deletes an existing event tag.
*
* Create a request for the method "eventTags.delete".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Event tag ID.
* @return the request
*/
public Delete delete(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(profileId, id);
initialize(result);
return result;
}
public class Delete extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/eventTags/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Deletes an existing event tag.
*
* Create a request for the method "eventTags.delete".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Event tag ID.
* @since 1.13
*/
protected Delete(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "DELETE", REST_PATH, null, Void.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Delete setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Event tag ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Event tag ID.
*/
public java.lang.Long getId() {
return id;
}
/** Event tag ID. */
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets one event tag by ID.
*
* Create a request for the method "eventTags.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Event tag ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/eventTags/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one event tag by ID.
*
* Create a request for the method "eventTags.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Event tag ID.
* @since 1.13
*/
protected Get(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.EventTag.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Get setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Event tag ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Event tag ID.
*/
public java.lang.Long getId() {
return id;
}
/** Event tag ID. */
public Get setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new event tag.
*
* Create a request for the method "eventTags.insert".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.EventTag}
* @return the request
*/
public Insert insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.EventTag content) throws java.io.IOException {
Insert result = new Insert(profileId, content);
initialize(result);
return result;
}
public class Insert extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/eventTags";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Inserts a new event tag.
*
* Create a request for the method "eventTags.insert".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.EventTag}
* @since 1.13
*/
protected Insert(java.lang.Long profileId, com.google.api.services.dfareporting.model.EventTag content) {
super(Dfareporting.this, "POST", REST_PATH, content, com.google.api.services.dfareporting.model.EventTag.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Insert setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves a list of event tags, possibly filtered.
*
* Create a request for the method "eventTags.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/eventTags";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Retrieves a list of event tags, possibly filtered.
*
* Create a request for the method "eventTags.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.EventTagsListResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Select only event tags that belong to this ad. */
@com.google.api.client.util.Key
private java.lang.Long adId;
/** Select only event tags that belong to this ad.
*/
public java.lang.Long getAdId() {
return adId;
}
/** Select only event tags that belong to this ad. */
public List setAdId(java.lang.Long adId) {
this.adId = adId;
return this;
}
/** Select only event tags that belong to this advertiser. */
@com.google.api.client.util.Key
private java.lang.Long advertiserId;
/** Select only event tags that belong to this advertiser.
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/** Select only event tags that belong to this advertiser. */
public List setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/** Select only event tags that belong to this campaign. */
@com.google.api.client.util.Key
private java.lang.Long campaignId;
/** Select only event tags that belong to this campaign.
*/
public java.lang.Long getCampaignId() {
return campaignId;
}
/** Select only event tags that belong to this campaign. */
public List setCampaignId(java.lang.Long campaignId) {
this.campaignId = campaignId;
return this;
}
/**
* Examine only the specified campaign or advertiser's event tags for matching selector
* criteria. When set to false, the parent advertiser and parent campaign of the specified ad
* or campaign is examined as well. In addition, when set to false, the status field is
* examined as well, along with the enabledByDefault field. This parameter can not be set to
* true when adId is specified as ads do not define their own even tags.
*/
@com.google.api.client.util.Key
private java.lang.Boolean definitionsOnly;
/** Examine only the specified campaign or advertiser's event tags for matching selector criteria. When
set to false, the parent advertiser and parent campaign of the specified ad or campaign is examined
as well. In addition, when set to false, the status field is examined as well, along with the
enabledByDefault field. This parameter can not be set to true when adId is specified as ads do not
define their own even tags.
*/
public java.lang.Boolean getDefinitionsOnly() {
return definitionsOnly;
}
/**
* Examine only the specified campaign or advertiser's event tags for matching selector
* criteria. When set to false, the parent advertiser and parent campaign of the specified ad
* or campaign is examined as well. In addition, when set to false, the status field is
* examined as well, along with the enabledByDefault field. This parameter can not be set to
* true when adId is specified as ads do not define their own even tags.
*/
public List setDefinitionsOnly(java.lang.Boolean definitionsOnly) {
this.definitionsOnly = definitionsOnly;
return this;
}
/**
* Select only enabled event tags. What is considered enabled or disabled depends on the
* definitionsOnly parameter. When definitionsOnly is set to true, only the specified
* advertiser or campaign's event tags' enabledByDefault field is examined. When
* definitionsOnly is set to false, the specified ad or specified campaign's parent
* advertiser's or parent campaign's event tags' enabledByDefault and status fields are
* examined as well.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enabled;
/** Select only enabled event tags. What is considered enabled or disabled depends on the
definitionsOnly parameter. When definitionsOnly is set to true, only the specified advertiser or
campaign's event tags' enabledByDefault field is examined. When definitionsOnly is set to false,
the specified ad or specified campaign's parent advertiser's or parent campaign's event tags'
enabledByDefault and status fields are examined as well.
*/
public java.lang.Boolean getEnabled() {
return enabled;
}
/**
* Select only enabled event tags. What is considered enabled or disabled depends on the
* definitionsOnly parameter. When definitionsOnly is set to true, only the specified
* advertiser or campaign's event tags' enabledByDefault field is examined. When
* definitionsOnly is set to false, the specified ad or specified campaign's parent
* advertiser's or parent campaign's event tags' enabledByDefault and status fields are
* examined as well.
*/
public List setEnabled(java.lang.Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Select only event tags with the specified event tag types. Event tag types can be used to
* specify whether to use a third-party pixel, a third-party JavaScript URL, or a third-party
* click-through URL for either impression or click tracking.
*/
@com.google.api.client.util.Key
private java.util.List eventTagTypes;
/** Select only event tags with the specified event tag types. Event tag types can be used to specify
whether to use a third-party pixel, a third-party JavaScript URL, or a third-party click-through
URL for either impression or click tracking.
*/
public java.util.List getEventTagTypes() {
return eventTagTypes;
}
/**
* Select only event tags with the specified event tag types. Event tag types can be used to
* specify whether to use a third-party pixel, a third-party JavaScript URL, or a third-party
* click-through URL for either impression or click tracking.
*/
public List setEventTagTypes(java.util.List eventTagTypes) {
this.eventTagTypes = eventTagTypes;
return this;
}
/** Select only event tags with these IDs. */
@com.google.api.client.util.Key
private java.util.List ids;
/** Select only event tags with these IDs.
*/
public java.util.List getIds() {
return ids;
}
/** Select only event tags with these IDs. */
public List setIds(java.util.List ids) {
this.ids = ids;
return this;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "eventtag*2015" will return objects with names like "eventtag June 2015", "eventtag April
* 2015", or simply "eventtag 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "eventtag" will
* match objects with name "my eventtag", "eventtag 2015", or simply "eventtag".
*/
@com.google.api.client.util.Key
private java.lang.String searchString;
/** Allows searching for objects by name or ID. Wildcards (*) are allowed. For example, "eventtag*2015"
will return objects with names like "eventtag June 2015", "eventtag April 2015", or simply
"eventtag 2015". Most of the searches also add wildcards implicitly at the start and the end of the
search string. For example, a search string of "eventtag" will match objects with name "my
eventtag", "eventtag 2015", or simply "eventtag".
*/
public java.lang.String getSearchString() {
return searchString;
}
/**
* Allows searching for objects by name or ID. Wildcards (*) are allowed. For example,
* "eventtag*2015" will return objects with names like "eventtag June 2015", "eventtag April
* 2015", or simply "eventtag 2015". Most of the searches also add wildcards implicitly at the
* start and the end of the search string. For example, a search string of "eventtag" will
* match objects with name "my eventtag", "eventtag 2015", or simply "eventtag".
*/
public List setSearchString(java.lang.String searchString) {
this.searchString = searchString;
return this;
}
/** Field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** Field by which to sort the list. [default: ID]
*/
public java.lang.String getSortField() {
return sortField;
}
/** Field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: ASCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing event tag. This method supports patch semantics.
*
* Create a request for the method "eventTags.patch".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Required. EventTag ID.
* @param content the {@link com.google.api.services.dfareporting.model.EventTag}
* @return the request
*/
public Patch patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.EventTag content) throws java.io.IOException {
Patch result = new Patch(profileId, id, content);
initialize(result);
return result;
}
public class Patch extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/eventTags";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing event tag. This method supports patch semantics.
*
* Create a request for the method "eventTags.patch".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Required. EventTag ID.
* @param content the {@link com.google.api.services.dfareporting.model.EventTag}
* @since 1.13
*/
protected Patch(java.lang.Long profileId, java.lang.Long id, com.google.api.services.dfareporting.model.EventTag content) {
super(Dfareporting.this, "PATCH", REST_PATH, content, com.google.api.services.dfareporting.model.EventTag.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Patch setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Required. EventTag ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Required. EventTag ID.
*/
public java.lang.Long getId() {
return id;
}
/** Required. EventTag ID. */
public Patch setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates an existing event tag.
*
* Create a request for the method "eventTags.update".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.EventTag}
* @return the request
*/
public Update update(java.lang.Long profileId, com.google.api.services.dfareporting.model.EventTag content) throws java.io.IOException {
Update result = new Update(profileId, content);
initialize(result);
return result;
}
public class Update extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/eventTags";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates an existing event tag.
*
* Create a request for the method "eventTags.update".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param content the {@link com.google.api.services.dfareporting.model.EventTag}
* @since 1.13
*/
protected Update(java.lang.Long profileId, com.google.api.services.dfareporting.model.EventTag content) {
super(Dfareporting.this, "PUT", REST_PATH, content, com.google.api.services.dfareporting.model.EventTag.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Update setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Files collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.Files.List request = dfareporting.files().list(parameters ...)}
*
*
* @return the resource collection
*/
public Files files() {
return new Files();
}
/**
* The "files" collection of methods.
*/
public class Files {
/**
* Retrieves a report file by its report ID and file ID. This method supports media download.
*
* Create a request for the method "files.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param reportId The ID of the report.
* @param fileId The ID of the report file.
* @return the request
*/
public Get get(java.lang.Long reportId, java.lang.Long fileId) throws java.io.IOException {
Get result = new Get(reportId, fileId);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "reports/{reportId}/files/{fileId}";
/**
* Retrieves a report file by its report ID and file ID. This method supports media download.
*
* Create a request for the method "files.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param reportId The ID of the report.
* @param fileId The ID of the report file.
* @since 1.13
*/
protected Get(java.lang.Long reportId, java.lang.Long fileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.File.class);
this.reportId = com.google.api.client.util.Preconditions.checkNotNull(reportId, "Required parameter reportId must be specified.");
this.fileId = com.google.api.client.util.Preconditions.checkNotNull(fileId, "Required parameter fileId must be specified.");
initializeMediaDownload();
}
@Override
public void executeMediaAndDownloadTo(java.io.OutputStream outputStream) throws java.io.IOException {
super.executeMediaAndDownloadTo(outputStream);
}
@Override
public java.io.InputStream executeMediaAsInputStream() throws java.io.IOException {
return super.executeMediaAsInputStream();
}
@Override
public com.google.api.client.http.HttpResponse executeMedia() throws java.io.IOException {
return super.executeMedia();
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the report. */
@com.google.api.client.util.Key
private java.lang.Long reportId;
/** The ID of the report.
*/
public java.lang.Long getReportId() {
return reportId;
}
/** The ID of the report. */
public Get setReportId(java.lang.Long reportId) {
this.reportId = reportId;
return this;
}
/** The ID of the report file. */
@com.google.api.client.util.Key
private java.lang.Long fileId;
/** The ID of the report file.
*/
public java.lang.Long getFileId() {
return fileId;
}
/** The ID of the report file. */
public Get setFileId(java.lang.Long fileId) {
this.fileId = fileId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists files for a user profile.
*
* Create a request for the method "files.list".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param profileId The Campaign Manager 360 user profile ID.
* @return the request
*/
public List list(java.lang.Long profileId) throws java.io.IOException {
List result = new List(profileId);
initialize(result);
return result;
}
public class List extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{profileId}/files";
/**
* Lists files for a user profile.
*
* Create a request for the method "files.list".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId The Campaign Manager 360 user profile ID.
* @since 1.13
*/
protected List(java.lang.Long profileId) {
super(Dfareporting.this, "GET", REST_PATH, null, com.google.api.services.dfareporting.model.FileList.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The Campaign Manager 360 user profile ID. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** The Campaign Manager 360 user profile ID.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** The Campaign Manager 360 user profile ID. */
public List setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Maximum number of results to return. */
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** Maximum number of results to return. [default: 10]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/** Maximum number of results to return. */
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The value of the nextToken from the previous result page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The value of the nextToken from the previous result page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The value of the nextToken from the previous result page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The scope that defines which results are returned. */
@com.google.api.client.util.Key
private java.lang.String scope;
/** The scope that defines which results are returned. [default: MINE]
*/
public java.lang.String getScope() {
return scope;
}
/** The scope that defines which results are returned. */
public List setScope(java.lang.String scope) {
this.scope = scope;
return this;
}
/** The field by which to sort the list. */
@com.google.api.client.util.Key
private java.lang.String sortField;
/** The field by which to sort the list. [default: LAST_MODIFIED_TIME]
*/
public java.lang.String getSortField() {
return sortField;
}
/** The field by which to sort the list. */
public List setSortField(java.lang.String sortField) {
this.sortField = sortField;
return this;
}
/** Order of sorted results. */
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/** Order of sorted results. [default: DESCENDING]
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/** Order of sorted results. */
public List setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the FloodlightActivities collection.
*
* The typical use is:
*
* {@code Dfareporting dfareporting = new Dfareporting(...);}
* {@code Dfareporting.FloodlightActivities.List request = dfareporting.floodlightActivities().list(parameters ...)}
*
*
* @return the resource collection
*/
public FloodlightActivities floodlightActivities() {
return new FloodlightActivities();
}
/**
* The "floodlightActivities" collection of methods.
*/
public class FloodlightActivities {
/**
* Deletes an existing floodlight activity.
*
* Create a request for the method "floodlightActivities.delete".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Floodlight activity ID.
* @return the request
*/
public Delete delete(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Delete result = new Delete(profileId, id);
initialize(result);
return result;
}
public class Delete extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/floodlightActivities/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Deletes an existing floodlight activity.
*
* Create a request for the method "floodlightActivities.delete".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @param id Floodlight activity ID.
* @since 1.13
*/
protected Delete(java.lang.Long profileId, java.lang.Long id) {
super(Dfareporting.this, "DELETE", REST_PATH, null, Void.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Delete setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Floodlight activity ID. */
@com.google.api.client.util.Key
private java.lang.Long id;
/** Floodlight activity ID.
*/
public java.lang.Long getId() {
return id;
}
/** Floodlight activity ID. */
public Delete setId(java.lang.Long id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Generates a tag for a floodlight activity.
*
* Create a request for the method "floodlightActivities.generatetag".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Generatetag#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @return the request
*/
public Generatetag generatetag(java.lang.Long profileId) throws java.io.IOException {
Generatetag result = new Generatetag(profileId);
initialize(result);
return result;
}
public class Generatetag extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/floodlightActivities/generatetag";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Generates a tag for a floodlight activity.
*
* Create a request for the method "floodlightActivities.generatetag".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Generatetag#execute()} method to invoke the remote
* operation. {@link
* Generatetag#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param profileId User profile ID associated with this request.
* @since 1.13
*/
protected Generatetag(java.lang.Long profileId) {
super(Dfareporting.this, "POST", REST_PATH, null, com.google.api.services.dfareporting.model.FloodlightActivitiesGenerateTagResponse.class);
this.profileId = com.google.api.client.util.Preconditions.checkNotNull(profileId, "Required parameter profileId must be specified.");
}
@Override
public Generatetag set$Xgafv(java.lang.String $Xgafv) {
return (Generatetag) super.set$Xgafv($Xgafv);
}
@Override
public Generatetag setAccessToken(java.lang.String accessToken) {
return (Generatetag) super.setAccessToken(accessToken);
}
@Override
public Generatetag setAlt(java.lang.String alt) {
return (Generatetag) super.setAlt(alt);
}
@Override
public Generatetag setCallback(java.lang.String callback) {
return (Generatetag) super.setCallback(callback);
}
@Override
public Generatetag setFields(java.lang.String fields) {
return (Generatetag) super.setFields(fields);
}
@Override
public Generatetag setKey(java.lang.String key) {
return (Generatetag) super.setKey(key);
}
@Override
public Generatetag setOauthToken(java.lang.String oauthToken) {
return (Generatetag) super.setOauthToken(oauthToken);
}
@Override
public Generatetag setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Generatetag) super.setPrettyPrint(prettyPrint);
}
@Override
public Generatetag setQuotaUser(java.lang.String quotaUser) {
return (Generatetag) super.setQuotaUser(quotaUser);
}
@Override
public Generatetag setUploadType(java.lang.String uploadType) {
return (Generatetag) super.setUploadType(uploadType);
}
@Override
public Generatetag setUploadProtocol(java.lang.String uploadProtocol) {
return (Generatetag) super.setUploadProtocol(uploadProtocol);
}
/** User profile ID associated with this request. */
@com.google.api.client.util.Key
private java.lang.Long profileId;
/** User profile ID associated with this request.
*/
public java.lang.Long getProfileId() {
return profileId;
}
/** User profile ID associated with this request. */
public Generatetag setProfileId(java.lang.Long profileId) {
this.profileId = profileId;
return this;
}
/** Floodlight activity ID for which we want to generate a tag. */
@com.google.api.client.util.Key
private java.lang.Long floodlightActivityId;
/** Floodlight activity ID for which we want to generate a tag.
*/
public java.lang.Long getFloodlightActivityId() {
return floodlightActivityId;
}
/** Floodlight activity ID for which we want to generate a tag. */
public Generatetag setFloodlightActivityId(java.lang.Long floodlightActivityId) {
this.floodlightActivityId = floodlightActivityId;
return this;
}
@Override
public Generatetag set(String parameterName, Object value) {
return (Generatetag) super.set(parameterName, value);
}
}
/**
* Gets one floodlight activity by ID.
*
* Create a request for the method "floodlightActivities.get".
*
* This request holds the parameters needed by the dfareporting server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param profileId User profile ID associated with this request.
* @param id Floodlight activity ID.
* @return the request
*/
public Get get(java.lang.Long profileId, java.lang.Long id) throws java.io.IOException {
Get result = new Get(profileId, id);
initialize(result);
return result;
}
public class Get extends DfareportingRequest {
private static final String REST_PATH = "userprofiles/{+profileId}/floodlightActivities/{+id}";
private final java.util.regex.Pattern PROFILE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets one floodlight activity by ID.
*
* Create a request for the method "floodlightActivities.get".
*
* This request holds the parameters needed by the the dfareporting server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.