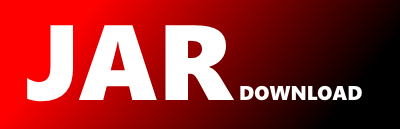
com.google.api.services.dfareporting.model.Advertiser Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dfareporting.model;
/**
* Contains properties of a Campaign Manager advertiser.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Campaign Manager 360 API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Advertiser extends com.google.api.client.json.GenericJson {
/**
* Account ID of this advertiser.This is a read-only field that can be left blank.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long accountId;
/**
* ID of the advertiser group this advertiser belongs to. You can group advertisers for reporting
* purposes, allowing you to see aggregated information for all advertisers in each group.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long advertiserGroupId;
/**
* Suffix added to click-through URL of ad creative associations under this advertiser. Must be
* less than 129 characters long.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clickThroughUrlSuffix;
/**
* ID of the click-through event tag to apply by default to the landing pages of this advertiser's
* campaigns.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long defaultClickThroughEventTagId;
/**
* Default email address used in sender field for tag emails.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultEmail;
/**
* Floodlight configuration ID of this advertiser. The floodlight configuration ID will be created
* automatically, so on insert this field should be left blank. This field can be set to another
* advertiser's floodlight configuration ID in order to share that advertiser's floodlight
* configuration with this advertiser, so long as: - This advertiser's original floodlight
* configuration is not already associated with floodlight activities or floodlight activity
* groups. - This advertiser's original floodlight configuration is not already shared with
* another advertiser.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long floodlightConfigurationId;
/**
* Dimension value for the ID of the floodlight configuration. This is a read-only, auto-generated
* field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DimensionValue floodlightConfigurationIdDimensionValue;
/**
* ID of this advertiser. This is a read-only, auto-generated field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long id;
/**
* Dimension value for the ID of this advertiser. This is a read-only, auto-generated field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DimensionValue idDimensionValue;
/**
* Identifies what kind of resource this is. Value: the fixed string "dfareporting#advertiser".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Measurement partner advertiser link for tag wrapping.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MeasurementPartnerAdvertiserLink measurementPartnerLink;
/**
* Name of this advertiser. This is a required field and must be less than 256 characters long and
* unique among advertisers of the same account.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Original floodlight configuration before any sharing occurred. Set the
* floodlightConfigurationId of this advertiser to originalFloodlightConfigurationId to unshare
* the advertiser's current floodlight configuration. You cannot unshare an advertiser's
* floodlight configuration if the shared configuration has activities associated with any
* campaign or placement.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long originalFloodlightConfigurationId;
/**
* Status of this advertiser.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* Subaccount ID of this advertiser.This is a read-only field that can be left blank.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long subaccountId;
/**
* Suspension status of this advertiser.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean suspended;
/**
* Account ID of this advertiser.This is a read-only field that can be left blank.
* @return value or {@code null} for none
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Account ID of this advertiser.This is a read-only field that can be left blank.
* @param accountId accountId or {@code null} for none
*/
public Advertiser setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* ID of the advertiser group this advertiser belongs to. You can group advertisers for reporting
* purposes, allowing you to see aggregated information for all advertisers in each group.
* @return value or {@code null} for none
*/
public java.lang.Long getAdvertiserGroupId() {
return advertiserGroupId;
}
/**
* ID of the advertiser group this advertiser belongs to. You can group advertisers for reporting
* purposes, allowing you to see aggregated information for all advertisers in each group.
* @param advertiserGroupId advertiserGroupId or {@code null} for none
*/
public Advertiser setAdvertiserGroupId(java.lang.Long advertiserGroupId) {
this.advertiserGroupId = advertiserGroupId;
return this;
}
/**
* Suffix added to click-through URL of ad creative associations under this advertiser. Must be
* less than 129 characters long.
* @return value or {@code null} for none
*/
public java.lang.String getClickThroughUrlSuffix() {
return clickThroughUrlSuffix;
}
/**
* Suffix added to click-through URL of ad creative associations under this advertiser. Must be
* less than 129 characters long.
* @param clickThroughUrlSuffix clickThroughUrlSuffix or {@code null} for none
*/
public Advertiser setClickThroughUrlSuffix(java.lang.String clickThroughUrlSuffix) {
this.clickThroughUrlSuffix = clickThroughUrlSuffix;
return this;
}
/**
* ID of the click-through event tag to apply by default to the landing pages of this advertiser's
* campaigns.
* @return value or {@code null} for none
*/
public java.lang.Long getDefaultClickThroughEventTagId() {
return defaultClickThroughEventTagId;
}
/**
* ID of the click-through event tag to apply by default to the landing pages of this advertiser's
* campaigns.
* @param defaultClickThroughEventTagId defaultClickThroughEventTagId or {@code null} for none
*/
public Advertiser setDefaultClickThroughEventTagId(java.lang.Long defaultClickThroughEventTagId) {
this.defaultClickThroughEventTagId = defaultClickThroughEventTagId;
return this;
}
/**
* Default email address used in sender field for tag emails.
* @return value or {@code null} for none
*/
public java.lang.String getDefaultEmail() {
return defaultEmail;
}
/**
* Default email address used in sender field for tag emails.
* @param defaultEmail defaultEmail or {@code null} for none
*/
public Advertiser setDefaultEmail(java.lang.String defaultEmail) {
this.defaultEmail = defaultEmail;
return this;
}
/**
* Floodlight configuration ID of this advertiser. The floodlight configuration ID will be created
* automatically, so on insert this field should be left blank. This field can be set to another
* advertiser's floodlight configuration ID in order to share that advertiser's floodlight
* configuration with this advertiser, so long as: - This advertiser's original floodlight
* configuration is not already associated with floodlight activities or floodlight activity
* groups. - This advertiser's original floodlight configuration is not already shared with
* another advertiser.
* @return value or {@code null} for none
*/
public java.lang.Long getFloodlightConfigurationId() {
return floodlightConfigurationId;
}
/**
* Floodlight configuration ID of this advertiser. The floodlight configuration ID will be created
* automatically, so on insert this field should be left blank. This field can be set to another
* advertiser's floodlight configuration ID in order to share that advertiser's floodlight
* configuration with this advertiser, so long as: - This advertiser's original floodlight
* configuration is not already associated with floodlight activities or floodlight activity
* groups. - This advertiser's original floodlight configuration is not already shared with
* another advertiser.
* @param floodlightConfigurationId floodlightConfigurationId or {@code null} for none
*/
public Advertiser setFloodlightConfigurationId(java.lang.Long floodlightConfigurationId) {
this.floodlightConfigurationId = floodlightConfigurationId;
return this;
}
/**
* Dimension value for the ID of the floodlight configuration. This is a read-only, auto-generated
* field.
* @return value or {@code null} for none
*/
public DimensionValue getFloodlightConfigurationIdDimensionValue() {
return floodlightConfigurationIdDimensionValue;
}
/**
* Dimension value for the ID of the floodlight configuration. This is a read-only, auto-generated
* field.
* @param floodlightConfigurationIdDimensionValue floodlightConfigurationIdDimensionValue or {@code null} for none
*/
public Advertiser setFloodlightConfigurationIdDimensionValue(DimensionValue floodlightConfigurationIdDimensionValue) {
this.floodlightConfigurationIdDimensionValue = floodlightConfigurationIdDimensionValue;
return this;
}
/**
* ID of this advertiser. This is a read-only, auto-generated field.
* @return value or {@code null} for none
*/
public java.lang.Long getId() {
return id;
}
/**
* ID of this advertiser. This is a read-only, auto-generated field.
* @param id id or {@code null} for none
*/
public Advertiser setId(java.lang.Long id) {
this.id = id;
return this;
}
/**
* Dimension value for the ID of this advertiser. This is a read-only, auto-generated field.
* @return value or {@code null} for none
*/
public DimensionValue getIdDimensionValue() {
return idDimensionValue;
}
/**
* Dimension value for the ID of this advertiser. This is a read-only, auto-generated field.
* @param idDimensionValue idDimensionValue or {@code null} for none
*/
public Advertiser setIdDimensionValue(DimensionValue idDimensionValue) {
this.idDimensionValue = idDimensionValue;
return this;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "dfareporting#advertiser".
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "dfareporting#advertiser".
* @param kind kind or {@code null} for none
*/
public Advertiser setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Measurement partner advertiser link for tag wrapping.
* @return value or {@code null} for none
*/
public MeasurementPartnerAdvertiserLink getMeasurementPartnerLink() {
return measurementPartnerLink;
}
/**
* Measurement partner advertiser link for tag wrapping.
* @param measurementPartnerLink measurementPartnerLink or {@code null} for none
*/
public Advertiser setMeasurementPartnerLink(MeasurementPartnerAdvertiserLink measurementPartnerLink) {
this.measurementPartnerLink = measurementPartnerLink;
return this;
}
/**
* Name of this advertiser. This is a required field and must be less than 256 characters long and
* unique among advertisers of the same account.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Name of this advertiser. This is a required field and must be less than 256 characters long and
* unique among advertisers of the same account.
* @param name name or {@code null} for none
*/
public Advertiser setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Original floodlight configuration before any sharing occurred. Set the
* floodlightConfigurationId of this advertiser to originalFloodlightConfigurationId to unshare
* the advertiser's current floodlight configuration. You cannot unshare an advertiser's
* floodlight configuration if the shared configuration has activities associated with any
* campaign or placement.
* @return value or {@code null} for none
*/
public java.lang.Long getOriginalFloodlightConfigurationId() {
return originalFloodlightConfigurationId;
}
/**
* Original floodlight configuration before any sharing occurred. Set the
* floodlightConfigurationId of this advertiser to originalFloodlightConfigurationId to unshare
* the advertiser's current floodlight configuration. You cannot unshare an advertiser's
* floodlight configuration if the shared configuration has activities associated with any
* campaign or placement.
* @param originalFloodlightConfigurationId originalFloodlightConfigurationId or {@code null} for none
*/
public Advertiser setOriginalFloodlightConfigurationId(java.lang.Long originalFloodlightConfigurationId) {
this.originalFloodlightConfigurationId = originalFloodlightConfigurationId;
return this;
}
/**
* Status of this advertiser.
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* Status of this advertiser.
* @param status status or {@code null} for none
*/
public Advertiser setStatus(java.lang.String status) {
this.status = status;
return this;
}
/**
* Subaccount ID of this advertiser.This is a read-only field that can be left blank.
* @return value or {@code null} for none
*/
public java.lang.Long getSubaccountId() {
return subaccountId;
}
/**
* Subaccount ID of this advertiser.This is a read-only field that can be left blank.
* @param subaccountId subaccountId or {@code null} for none
*/
public Advertiser setSubaccountId(java.lang.Long subaccountId) {
this.subaccountId = subaccountId;
return this;
}
/**
* Suspension status of this advertiser.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSuspended() {
return suspended;
}
/**
* Suspension status of this advertiser.
* @param suspended suspended or {@code null} for none
*/
public Advertiser setSuspended(java.lang.Boolean suspended) {
this.suspended = suspended;
return this;
}
@Override
public Advertiser set(String fieldName, Object value) {
return (Advertiser) super.set(fieldName, value);
}
@Override
public Advertiser clone() {
return (Advertiser) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy